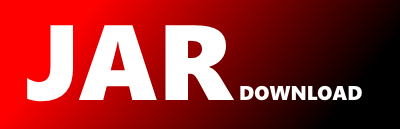
dev-ui.qwc-qosdk-controllers.js Maven / Gradle / Ivy
import {QwcHotReloadElement, css, html} from 'qwc-hot-reload-element';
import {JsonRpc} from 'jsonrpc';
import '@vaadin/details';
import '@vaadin/list-box';
import '@vaadin/item';
import '@vaadin/horizontal-layout';
import '@vaadin/vertical-layout';
import '@vaadin/icon';
import '@vaadin/vaadin-lumo-styles/vaadin-iconset.js'
import '@vaadin/form-layout';
import '@vaadin/text-field';
import 'qui-badge';
export class QWCQOSDKControllers extends QwcHotReloadElement {
jsonRpc = new JsonRpc(this);
constructor() {
super();
}
connectedCallback() {
super.connectedCallback();
this.hotReload();
}
hotReload() {
this.jsonRpc.getControllers().then(
jsonRpcResponse => this._controllers = jsonRpcResponse.result);
}
static properties = {
_controllers: {state: true},
}
render() {
if (this._controllers) {
return html`
Configured controllers (${this._controllers.length})
${this._controllers.map(this.controller, this)}
`
}
}
controller(controller) {
return html`
${nameImplAndResource(controller.name, controller.className,
controller.resourceClass)}
${this.namespaces(controller)}
${this.children(controller.dependents, "Dependents",
this.dependent)}
${this.children(controller.eventSources, "Event Sources",
this.eventSource)}
`
}
namespaces(controller) {
return html`
Configured:
${controller.configuredNamespaces.map(ns => html`${name(ns)}`)}
Effective:
${controller.effectiveNamespaces.map(ns => html`${name(ns)}`)}
`
}
eventSource(eventSource) {
return html`
${nameImplAndResource(eventSource.name, eventSource.type, eventSource.resourceClass)}
`
}
dependent(dependent) {
return html`
${nameImplAndResource(dependent.name, dependent.type,
dependent.resourceClass)}
${dependsOn(dependent)}
${conditions(dependent)}
`
}
children(children, childrenName, childRenderer) {
if (children) {
let count = children.length;
return html`
${children.map((child) => html`${childRenderer(child)}`)}
`
}
}
}
function name(name) {
return html`
${name} `
}
function nameImplAndResource(n, impl, resource) {
return html`
${name(n)}
${clazz(impl)}
${resourceClass(resource)}
`
}
const fabric8Prefix = 'io.fabric8.kubernetes.api.model.';
const josdkProcessingPrefix = 'io.javaoperatorsdk.operator.processing.';
const userProvided = 'success';
const k8sProvided = 'warning';
const josdkProvided = 'contrast';
function resourceClass(resourceClass) {
return clazz(resourceClass, true)
}
function clazz(impl, isPill) {
if (impl) {
let level = userProvided;
if (impl.startsWith(fabric8Prefix)) {
level = k8sProvided;
impl = impl.substring(fabric8Prefix.length);
} else if (impl.startsWith(josdkProcessingPrefix)) {
level = josdkProvided;
impl = impl.substring(josdkProcessingPrefix.length);
}
return isPill ?
html`${impl} ` :
html`${impl} `
}
}
function conditions(dependent) {
let hasConditions = dependent.hasConditions;
if (hasConditions) {
return html`
${field(dependent.readyCondition, "Ready")}
${field(dependent.reconcileCondition, "Reconcile")}
${field(dependent.deleteCondition, "Delete")}
`
}
}
function dependsOn(dependent) {
if (dependent.dependsOn && dependent.dependsOn.length > 0) {
return html`
${dependent.dependsOn.map(dep => html`${name(dep)}`)}
`
}
}
function field(value, label) {
if (value) {
return html`
${label}: ${clazz(value)}
`
}
}
customElements.define('qwc-qosdk-controllers', QWCQOSDKControllers);
© 2015 - 2025 Weber Informatics LLC | Privacy Policy