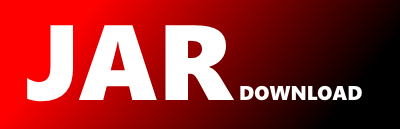
dev-ui.qwc-wsn-endpoints.js Maven / Gradle / Ivy
import { LitElement, html, css} from 'lit';
import { columnBodyRenderer } from '@vaadin/grid/lit.js';
import '@vaadin/grid';
import '@vaadin/text-field';
import '@vaadin/button';
import '@vaadin/tooltip';
import '@vaadin/message-input';
import '@vaadin/message-list';
import { notifier } from 'notifier';
import { JsonRpc } from 'jsonrpc';
import { endpoints } from 'build-time-data';
export class QwcWebSocketNextEndpoints extends LitElement {
jsonRpc = new JsonRpc(this);
static styles = css`
:host {
display: flex;
flex-direction: column;
gap: 10px;
}
.endpoints-table {
padding-bottom: 10px;
}
.annotation {
color: var(--lumo-contrast-50pct);
}
.connections-icon {
cursor: pointer;
}
.top-bar {
display: flex;
align-items: baseline;
gap: 20px;
padding-left: 20px;
padding-right: 20px;
}
.top-bar h4 {
color: var(--lumo-contrast-60pct);
}
vaadin-message.outgoing {
background-color: hsla(214, 61%, 25%, 0.05);
border: 2px solid rgb(255, 255, 255);
border-radius: 9px;
}
.message-list {
gap: 20px;
padding-left: 20px;
padding-right: 20px;
}
vaadin-message-input > vaadin-text-area > textarea {
font-family: monospace;
}
`;
static properties = {
_selectedEndpoint: {state: true},
_selectedConnection: {state: true},
_endpointsAndConnections: {state: true},
_textMessages: {state: true}
};
constructor() {
super();
// If not null then show the connections of the selected endpoint
this._selectedEndpoint = null;
// If not null then show the detail of a Dev UI connection
this._selectedConnection = null;
this._textMessages = [];
}
connectedCallback() {
super.connectedCallback();
const generatedEndpoints = endpoints.map(e => e.generatedClazz);
this.jsonRpc.getConnections({"endpoints": generatedEndpoints})
.then(jsonResponse => {
this._endpointsAndConnections = endpoints.map(e => {
e.connections = jsonResponse.result[e.generatedClazz];
return e;
});
})
.then(() => {
this._conntectionStatusStream = this.jsonRpc.connectionStatus().onNext(jsonResponse => {
const endpoint = this._endpointsAndConnections.find(e => e.generatedClazz == jsonResponse.result.endpoint);
if (endpoint) {
if (jsonResponse.result.removed) {
const connectionId = jsonResponse.result.id;
endpoint.connections = endpoint.connections.filter(c => c.id != connectionId);
} else {
endpoint.connections = [
...endpoint.connections,
jsonResponse.result
];
}
// TODO this is inefficient but I did not find a way to update the endpoint list
// when a connection is added/removed
// https://lit.dev/docs/components/properties/#mutating-properties
this._endpointsAndConnections = this._endpointsAndConnections.map(e => e);
}
}
);
this._textMessagesStream = this.jsonRpc.connectionMessages().onNext(jsonResponse => {
if (this._selectedConnection && jsonResponse.result.key == this._selectedConnection.devuiSocketKey) {
this._textMessages = [
jsonResponse.result,
...this._textMessages
];
}
});
});
}
disconnectedCallback() {
super.disconnectedCallback();
this._conntectionStatusStream.cancel();
}
render() {
if (this._endpointsAndConnections){
if(this._selectedConnection) {
if (this._textMessages) {
return this._renderConnection();
} else {
return html`Loading messages...`;
}
} else if (this._selectedEndpoint){
return this._renderConnections();
} else{
return this._renderEndpoints();
}
} else {
return html`Loading endpoints...`;
}
}
_renderEndpoints(){
return html`
`;
}
_renderConnections(){
return html`
${this._renderTopBar()}
`;
}
_renderConnection(){
return html`
${this._renderTopBarConnection()}
style="font-family: monospace;"
`;
}
_renderTopBar(){
return html`
`;
}
_renderTopBarConnection(){
return html`
`;
}
_renderPath(endpoint) {
const inputId = endpoint.clazz.replaceAll("\.","_");
const hasPathParam = endpoint.path.indexOf('{') != -1;
var inputPath;
var resetButton;
if (hasPathParam) {
inputPath = html`
`
resetButton = html`
this._resetPathInput(inputId, endpoint.path)}" label="Reset the original path" >
`
} else {
inputPath = html`
`
resetButton = html``;
}
return html`
${inputPath}
this._openDevConnection(inputId,endpoint)}" label="Open Dev UI connection">
Connect
${resetButton}
`;
}
_renderClazz(endpoint) {
return html`
${endpoint.clazz}
`;
}
_renderExecutionMode(endpoint) {
return html`
${endpoint.executionMode}
`;
}
_renderCallbacks(endpoint) {
return endpoint.callbacks ? html`
${ endpoint.callbacks.map(callback =>
html`${callback.annotation}
${callback.method}
`
)}
`: html``;
}
_renderConnectionsButton(endpoint) {
return html`
this._showConnections(endpoint)}>
${endpoint.connections.length}
`;
}
_renderType(connection) {
if(connection.devuiSocketKey) {
return html`
`
} else {
return html`
`
}
}
_renderId(connection) {
return html`
${connection.id}
`;
}
_renderHandshakePath(connection) {
return html`
${connection.handshakePath}
`;
}
_renderCreationTime(connection) {
return html`
${connection.creationTime}
`;
}
_renderDevButton(connection) {
if (connection.devuiSocketKey) {
return html`
this._showConnectionDetail(connection)}>
Manage
`;
} else {
return html``;
}
}
_showConnections(endpoint) {
this._selectedEndpoint = endpoint;
this._selectedConnection = null;
}
_showEndpoints() {
this._selectedEndpoint = null;
this._selectedConnection = null;
}
_showConnectionDetail(connection) {
this._selectedConnection = connection;
this._textMessages = null;
this.jsonRpc.getMessages({"connectionKey": connection.devuiSocketKey}).then(jsonResponse => {
this._textMessages = jsonResponse.result;
});
}
_openDevConnection(inputPathId, endpoint) {
const query = '#'+ inputPathId;
const path = this.renderRoot?.querySelector(query).value ?? null;
if (path) {
this.jsonRpc.openDevConnection({"path": path, "endpointPath": endpoint.path}).then(jsonResponse => {
if (jsonResponse.result.success) {
notifier.showSuccessMessage("Opened Dev UI connection");
this._selectedEndpoint = endpoint;
} else {
notifier.showErrorMessage("Unable to open Dev UI connection", "bottom-stretch");
}
});
} else {
notifier.showErrorMessage("Unable to obtain the endpoint path", "bottom-stretch");
}
}
_closeDevConnection() {
this.jsonRpc.closeDevConnection({"connectionKey": this._selectedConnection.devuiSocketKey}).then(jsonResponse => {
if (jsonResponse.result.success) {
notifier.showSuccessMessage("Closed Dev UI connection");
} else {
notifier.showErrorMessage("Unable to close Dev UI connection", "bottom-stretch");
}
this._selectedConnection = null;
this._showConnections(this._selectedEndpoint);
});
}
_clearMessages() {
if (this._selectedConnection && this._selectedConnection.devuiSocketKey) {
this.jsonRpc.clearMessages({"connectionKey": this._selectedConnection.devuiSocketKey}).then(jsonResponse => {
if (!jsonResponse.result.success) {
notifier.showErrorMessage("Unable to clear messages for Dev UI connection", "bottom-stretch");
}
this._textMessages = [];
});
}
}
_resetPathInput(endpointPathId, value) {
const query = '#'+ endpointPathId;
const input = this.renderRoot?.querySelector(query) ?? null;
if (input) {
input.value = value;
}
}
_sendMessage(e) {
if (this._selectedConnection && this._selectedConnection.devuiSocketKey) {
this.jsonRpc.sendTextMessage({"connectionKey": this._selectedConnection.devuiSocketKey, "message": e.detail.value}).then(jsonResponse => {
if (jsonResponse.result.success) {
notifier.showSuccessMessage("Text message sent to Dev UI connection");
} else {
notifier.showErrorMessage("Unable to send text message to Dev UI connection", "bottom-stretch");
}
});
}
}
}
customElements.define('qwc-wsn-endpoints', QwcWebSocketNextEndpoints);
© 2015 - 2025 Weber Informatics LLC | Privacy Policy