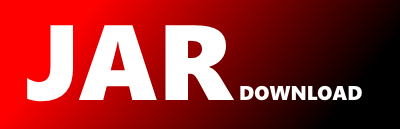
elements.index.js Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mutation-testing-elements Show documentation
Show all versions of mutation-testing-elements Show documentation
A suite of web components for a mutation testing report.
var qi = Object.defineProperty;
var Vi = (r, e, t) => e in r ? qi(r, e, { enumerable: !0, configurable: !0, writable: !0, value: t }) : r[e] = t;
var x = (r, e, t) => (Vi(r, typeof e != "symbol" ? e + "" : e, t), t), qt = (r, e, t) => {
if (!e.has(r))
throw TypeError("Cannot " + t);
};
var D = (r, e, t) => (qt(r, e, "read from private field"), t ? t.call(r) : e.get(r)), me = (r, e, t) => {
if (e.has(r))
throw TypeError("Cannot add the same private member more than once");
e instanceof WeakSet ? e.add(r) : e.set(r, t);
}, Vt = (r, e, t, n) => (qt(r, e, "write to private field"), n ? n.call(r, t) : e.set(r, t), t);
var Wt = (r, e, t) => (qt(r, e, "access private method"), t);
/**
* @license
* Copyright 2019 Google LLC
* SPDX-License-Identifier: BSD-3-Clause
*/
const yt = globalThis, yr = yt.ShadowRoot && (yt.ShadyCSS === void 0 || yt.ShadyCSS.nativeShadow) && "adoptedStyleSheets" in Document.prototype && "replace" in CSSStyleSheet.prototype, $n = Symbol(), Ur = /* @__PURE__ */ new WeakMap();
let Wi = class {
constructor(e, t, n) {
if (this._$cssResult$ = !0, n !== $n)
throw Error("CSSResult is not constructable. Use `unsafeCSS` or `css` instead.");
this.cssText = e, this.t = t;
}
get styleSheet() {
let e = this.o;
const t = this.t;
if (yr && e === void 0) {
const n = t !== void 0 && t.length === 1;
n && (e = Ur.get(t)), e === void 0 && ((this.o = e = new CSSStyleSheet()).replaceSync(this.cssText), n && Ur.set(t, e));
}
return e;
}
toString() {
return this.cssText;
}
};
const q = (r) => new Wi(typeof r == "string" ? r : r + "", void 0, $n), Zi = (r, e) => {
if (yr)
r.adoptedStyleSheets = e.map((t) => t instanceof CSSStyleSheet ? t : t.styleSheet);
else
for (const t of e) {
const n = document.createElement("style"), i = yt.litNonce;
i !== void 0 && n.setAttribute("nonce", i), n.textContent = t.cssText, r.appendChild(n);
}
}, Hr = yr ? (r) => r : (r) => r instanceof CSSStyleSheet ? ((e) => {
let t = "";
for (const n of e.cssRules)
t += n.cssText;
return q(t);
})(r) : r;
/**
* @license
* Copyright 2017 Google LLC
* SPDX-License-Identifier: BSD-3-Clause
*/
const { is: Gi, defineProperty: Yi, getOwnPropertyDescriptor: Ji, getOwnPropertyNames: Qi, getOwnPropertySymbols: Xi, getPrototypeOf: es } = Object, ue = globalThis, Kr = ue.trustedTypes, ts = Kr ? Kr.emptyScript : "", Zt = ue.reactiveElementPolyfillSupport, Ge = (r, e) => r, kt = { toAttribute(r, e) {
switch (e) {
case Boolean:
r = r ? ts : null;
break;
case Object:
case Array:
r = r == null ? r : JSON.stringify(r);
}
return r;
}, fromAttribute(r, e) {
let t = r;
switch (e) {
case Boolean:
t = r !== null;
break;
case Number:
t = r === null ? null : Number(r);
break;
case Object:
case Array:
try {
t = JSON.parse(r);
} catch {
t = null;
}
}
return t;
} }, wr = (r, e) => !Gi(r, e), qr = { attribute: !0, type: String, converter: kt, reflect: !1, hasChanged: wr };
Symbol.metadata ?? (Symbol.metadata = Symbol("metadata")), ue.litPropertyMetadata ?? (ue.litPropertyMetadata = /* @__PURE__ */ new WeakMap());
let Ie = class extends HTMLElement {
static addInitializer(e) {
this._$Ei(), (this.l ?? (this.l = [])).push(e);
}
static get observedAttributes() {
return this.finalize(), this._$Eh && [...this._$Eh.keys()];
}
static createProperty(e, t = qr) {
if (t.state && (t.attribute = !1), this._$Ei(), this.elementProperties.set(e, t), !t.noAccessor) {
const n = Symbol(), i = this.getPropertyDescriptor(e, n, t);
i !== void 0 && Yi(this.prototype, e, i);
}
}
static getPropertyDescriptor(e, t, n) {
const { get: i, set: s } = Ji(this.prototype, e) ?? { get() {
return this[t];
}, set(a) {
this[t] = a;
} };
return { get() {
return i == null ? void 0 : i.call(this);
}, set(a) {
const o = i == null ? void 0 : i.call(this);
s.call(this, a), this.requestUpdate(e, o, n);
}, configurable: !0, enumerable: !0 };
}
static getPropertyOptions(e) {
return this.elementProperties.get(e) ?? qr;
}
static _$Ei() {
if (this.hasOwnProperty(Ge("elementProperties")))
return;
const e = es(this);
e.finalize(), e.l !== void 0 && (this.l = [...e.l]), this.elementProperties = new Map(e.elementProperties);
}
static finalize() {
if (this.hasOwnProperty(Ge("finalized")))
return;
if (this.finalized = !0, this._$Ei(), this.hasOwnProperty(Ge("properties"))) {
const t = this.properties, n = [...Qi(t), ...Xi(t)];
for (const i of n)
this.createProperty(i, t[i]);
}
const e = this[Symbol.metadata];
if (e !== null) {
const t = litPropertyMetadata.get(e);
if (t !== void 0)
for (const [n, i] of t)
this.elementProperties.set(n, i);
}
this._$Eh = /* @__PURE__ */ new Map();
for (const [t, n] of this.elementProperties) {
const i = this._$Eu(t, n);
i !== void 0 && this._$Eh.set(i, t);
}
this.elementStyles = this.finalizeStyles(this.styles);
}
static finalizeStyles(e) {
const t = [];
if (Array.isArray(e)) {
const n = new Set(e.flat(1 / 0).reverse());
for (const i of n)
t.unshift(Hr(i));
} else
e !== void 0 && t.push(Hr(e));
return t;
}
static _$Eu(e, t) {
const n = t.attribute;
return n === !1 ? void 0 : typeof n == "string" ? n : typeof e == "string" ? e.toLowerCase() : void 0;
}
constructor() {
super(), this._$Ep = void 0, this.isUpdatePending = !1, this.hasUpdated = !1, this._$Em = null, this._$Ev();
}
_$Ev() {
var e;
this._$Eg = new Promise((t) => this.enableUpdating = t), this._$AL = /* @__PURE__ */ new Map(), this._$E_(), this.requestUpdate(), (e = this.constructor.l) == null || e.forEach((t) => t(this));
}
addController(e) {
var t;
(this._$ES ?? (this._$ES = [])).push(e), this.renderRoot !== void 0 && this.isConnected && ((t = e.hostConnected) == null || t.call(e));
}
removeController(e) {
var t;
(t = this._$ES) == null || t.splice(this._$ES.indexOf(e) >>> 0, 1);
}
_$E_() {
const e = /* @__PURE__ */ new Map(), t = this.constructor.elementProperties;
for (const n of t.keys())
this.hasOwnProperty(n) && (e.set(n, this[n]), delete this[n]);
e.size > 0 && (this._$Ep = e);
}
createRenderRoot() {
const e = this.shadowRoot ?? this.attachShadow(this.constructor.shadowRootOptions);
return Zi(e, this.constructor.elementStyles), e;
}
connectedCallback() {
var e;
this.renderRoot ?? (this.renderRoot = this.createRenderRoot()), this.enableUpdating(!0), (e = this._$ES) == null || e.forEach((t) => {
var n;
return (n = t.hostConnected) == null ? void 0 : n.call(t);
});
}
enableUpdating(e) {
}
disconnectedCallback() {
var e;
(e = this._$ES) == null || e.forEach((t) => {
var n;
return (n = t.hostDisconnected) == null ? void 0 : n.call(t);
});
}
attributeChangedCallback(e, t, n) {
this._$AK(e, n);
}
_$EO(e, t) {
var s;
const n = this.constructor.elementProperties.get(e), i = this.constructor._$Eu(e, n);
if (i !== void 0 && n.reflect === !0) {
const a = (((s = n.converter) == null ? void 0 : s.toAttribute) !== void 0 ? n.converter : kt).toAttribute(t, n.type);
this._$Em = e, a == null ? this.removeAttribute(i) : this.setAttribute(i, a), this._$Em = null;
}
}
_$AK(e, t) {
var s;
const n = this.constructor, i = n._$Eh.get(e);
if (i !== void 0 && this._$Em !== i) {
const a = n.getPropertyOptions(i), o = typeof a.converter == "function" ? { fromAttribute: a.converter } : ((s = a.converter) == null ? void 0 : s.fromAttribute) !== void 0 ? a.converter : kt;
this._$Em = i, this[i] = o.fromAttribute(t, a.type), this._$Em = null;
}
}
requestUpdate(e, t, n, i = !1, s) {
if (e !== void 0) {
if (n ?? (n = this.constructor.getPropertyOptions(e)), !(n.hasChanged ?? wr)(i ? s : this[e], t))
return;
this.C(e, t, n);
}
this.isUpdatePending === !1 && (this._$Eg = this._$EP());
}
C(e, t, n) {
this._$AL.has(e) || this._$AL.set(e, t), n.reflect === !0 && this._$Em !== e && (this._$Ej ?? (this._$Ej = /* @__PURE__ */ new Set())).add(e);
}
async _$EP() {
this.isUpdatePending = !0;
try {
await this._$Eg;
} catch (t) {
Promise.reject(t);
}
const e = this.scheduleUpdate();
return e != null && await e, !this.isUpdatePending;
}
scheduleUpdate() {
return this.performUpdate();
}
performUpdate() {
var n;
if (!this.isUpdatePending)
return;
if (!this.hasUpdated) {
if (this._$Ep) {
for (const [s, a] of this._$Ep)
this[s] = a;
this._$Ep = void 0;
}
const i = this.constructor.elementProperties;
if (i.size > 0)
for (const [s, a] of i)
a.wrapped !== !0 || this._$AL.has(s) || this[s] === void 0 || this.C(s, this[s], a);
}
let e = !1;
const t = this._$AL;
try {
e = this.shouldUpdate(t), e ? (this.willUpdate(t), (n = this._$ES) == null || n.forEach((i) => {
var s;
return (s = i.hostUpdate) == null ? void 0 : s.call(i);
}), this.update(t)) : this._$ET();
} catch (i) {
throw e = !1, this._$ET(), i;
}
e && this._$AE(t);
}
willUpdate(e) {
}
_$AE(e) {
var t;
(t = this._$ES) == null || t.forEach((n) => {
var i;
return (i = n.hostUpdated) == null ? void 0 : i.call(n);
}), this.hasUpdated || (this.hasUpdated = !0, this.firstUpdated(e)), this.updated(e);
}
_$ET() {
this._$AL = /* @__PURE__ */ new Map(), this.isUpdatePending = !1;
}
get updateComplete() {
return this.getUpdateComplete();
}
getUpdateComplete() {
return this._$Eg;
}
shouldUpdate(e) {
return !0;
}
update(e) {
this._$Ej && (this._$Ej = this._$Ej.forEach((t) => this._$EO(t, this[t]))), this._$ET();
}
updated(e) {
}
firstUpdated(e) {
}
};
Ie.elementStyles = [], Ie.shadowRootOptions = { mode: "open" }, Ie[Ge("elementProperties")] = /* @__PURE__ */ new Map(), Ie[Ge("finalized")] = /* @__PURE__ */ new Map(), Zt == null || Zt({ ReactiveElement: Ie }), (ue.reactiveElementVersions ?? (ue.reactiveElementVersions = [])).push("2.0.1");
/**
* @license
* Copyright 2017 Google LLC
* SPDX-License-Identifier: BSD-3-Clause
*/
const Ye = globalThis, _t = Ye.trustedTypes, Vr = _t ? _t.createPolicy("lit-html", { createHTML: (r) => r }) : void 0, xr = "$lit$", te = `lit$${(Math.random() + "").slice(9)}$`, $r = "?" + te, rs = `<${$r}>`, Ae = document, Xe = () => Ae.createComment(""), et = (r) => r === null || typeof r != "object" && typeof r != "function", kn = Array.isArray, _n = (r) => kn(r) || typeof (r == null ? void 0 : r[Symbol.iterator]) == "function", Gt = `[
\f\r]`, Ve = /<(?:(!--|\/[^a-zA-Z])|(\/?[a-zA-Z][^>\s]*)|(\/?$))/g, Wr = /-->/g, Zr = />/g, be = RegExp(`>|${Gt}(?:([^\\s"'>=/]+)(${Gt}*=${Gt}*(?:[^
\f\r"'\`<>=]|("|')|))|$)`, "g"), Gr = /'/g, Yr = /"/g, An = /^(?:script|style|textarea|title)$/i, Sn = (r) => (e, ...t) => ({ _$litType$: r, strings: e, values: t }), v = Sn(1), N = Sn(2), ne = Symbol.for("lit-noChange"), A = Symbol.for("lit-nothing"), Jr = /* @__PURE__ */ new WeakMap(), we = Ae.createTreeWalker(Ae, 129);
function Cn(r, e) {
if (!Array.isArray(r) || !r.hasOwnProperty("raw"))
throw Error("invalid template strings array");
return Vr !== void 0 ? Vr.createHTML(e) : e;
}
const En = (r, e) => {
const t = r.length - 1, n = [];
let i, s = e === 2 ? "" : "")), n];
};
class tt {
constructor({ strings: e, _$litType$: t }, n) {
let i;
this.parts = [];
let s = 0, a = 0;
const o = e.length - 1, l = this.parts, [c, g] = En(e, t);
if (this.el = tt.createElement(c, n), we.currentNode = this.el.content, t === 2) {
const d = this.el.content.firstChild;
d.replaceWith(...d.childNodes);
}
for (; (i = we.nextNode()) !== null && l.length < o; ) {
if (i.nodeType === 1) {
if (i.hasAttributes())
for (const d of i.getAttributeNames())
if (d.endsWith(xr)) {
const m = g[a++], y = i.getAttribute(d).split(te), $ = /([.?@])?(.*)/.exec(m);
l.push({ type: 1, index: s, name: $[2], strings: y, ctor: $[1] === "." ? Pn : $[1] === "?" ? Tn : $[1] === "@" ? Fn : ut }), i.removeAttribute(d);
} else
d.startsWith(te) && (l.push({ type: 6, index: s }), i.removeAttribute(d));
if (An.test(i.tagName)) {
const d = i.textContent.split(te), m = d.length - 1;
if (m > 0) {
i.textContent = _t ? _t.emptyScript : "";
for (let y = 0; y < m; y++)
i.append(d[y], Xe()), we.nextNode(), l.push({ type: 2, index: ++s });
i.append(d[m], Xe());
}
}
} else if (i.nodeType === 8)
if (i.data === $r)
l.push({ type: 2, index: s });
else {
let d = -1;
for (; (d = i.data.indexOf(te, d + 1)) !== -1; )
l.push({ type: 7, index: s }), d += te.length - 1;
}
s++;
}
}
static createElement(e, t) {
const n = Ae.createElement("template");
return n.innerHTML = e, n;
}
}
function Se(r, e, t = r, n) {
var a, o;
if (e === ne)
return e;
let i = n !== void 0 ? (a = t._$Co) == null ? void 0 : a[n] : t._$Cl;
const s = et(e) ? void 0 : e._$litDirective$;
return (i == null ? void 0 : i.constructor) !== s && ((o = i == null ? void 0 : i._$AO) == null || o.call(i, !1), s === void 0 ? i = void 0 : (i = new s(r), i._$AT(r, t, n)), n !== void 0 ? (t._$Co ?? (t._$Co = []))[n] = i : t._$Cl = i), i !== void 0 && (e = Se(r, i._$AS(r, e.values), i, n)), e;
}
class Mn {
constructor(e, t) {
this._$AV = [], this._$AN = void 0, this._$AD = e, this._$AM = t;
}
get parentNode() {
return this._$AM.parentNode;
}
get _$AU() {
return this._$AM._$AU;
}
u(e) {
const { el: { content: t }, parts: n } = this._$AD, i = ((e == null ? void 0 : e.creationScope) ?? Ae).importNode(t, !0);
we.currentNode = i;
let s = we.nextNode(), a = 0, o = 0, l = n[0];
for (; l !== void 0; ) {
if (a === l.index) {
let c;
l.type === 2 ? c = new Re(s, s.nextSibling, this, e) : l.type === 1 ? c = new l.ctor(s, l.name, l.strings, this, e) : l.type === 6 && (c = new zn(s, this, e)), this._$AV.push(c), l = n[++o];
}
a !== (l == null ? void 0 : l.index) && (s = we.nextNode(), a++);
}
return we.currentNode = Ae, i;
}
p(e) {
let t = 0;
for (const n of this._$AV)
n !== void 0 && (n.strings !== void 0 ? (n._$AI(e, n, t), t += n.strings.length - 2) : n._$AI(e[t])), t++;
}
}
class Re {
get _$AU() {
var e;
return ((e = this._$AM) == null ? void 0 : e._$AU) ?? this._$Cv;
}
constructor(e, t, n, i) {
this.type = 2, this._$AH = A, this._$AN = void 0, this._$AA = e, this._$AB = t, this._$AM = n, this.options = i, this._$Cv = (i == null ? void 0 : i.isConnected) ?? !0;
}
get parentNode() {
let e = this._$AA.parentNode;
const t = this._$AM;
return t !== void 0 && (e == null ? void 0 : e.nodeType) === 11 && (e = t.parentNode), e;
}
get startNode() {
return this._$AA;
}
get endNode() {
return this._$AB;
}
_$AI(e, t = this) {
e = Se(this, e, t), et(e) ? e === A || e == null || e === "" ? (this._$AH !== A && this._$AR(), this._$AH = A) : e !== this._$AH && e !== ne && this._(e) : e._$litType$ !== void 0 ? this.g(e) : e.nodeType !== void 0 ? this.$(e) : _n(e) ? this.T(e) : this._(e);
}
k(e) {
return this._$AA.parentNode.insertBefore(e, this._$AB);
}
$(e) {
this._$AH !== e && (this._$AR(), this._$AH = this.k(e));
}
_(e) {
this._$AH !== A && et(this._$AH) ? this._$AA.nextSibling.data = e : this.$(Ae.createTextNode(e)), this._$AH = e;
}
g(e) {
var s;
const { values: t, _$litType$: n } = e, i = typeof n == "number" ? this._$AC(e) : (n.el === void 0 && (n.el = tt.createElement(Cn(n.h, n.h[0]), this.options)), n);
if (((s = this._$AH) == null ? void 0 : s._$AD) === i)
this._$AH.p(t);
else {
const a = new Mn(i, this), o = a.u(this.options);
a.p(t), this.$(o), this._$AH = a;
}
}
_$AC(e) {
let t = Jr.get(e.strings);
return t === void 0 && Jr.set(e.strings, t = new tt(e)), t;
}
T(e) {
kn(this._$AH) || (this._$AH = [], this._$AR());
const t = this._$AH;
let n, i = 0;
for (const s of e)
i === t.length ? t.push(n = new Re(this.k(Xe()), this.k(Xe()), this, this.options)) : n = t[i], n._$AI(s), i++;
i < t.length && (this._$AR(n && n._$AB.nextSibling, i), t.length = i);
}
_$AR(e = this._$AA.nextSibling, t) {
var n;
for ((n = this._$AP) == null ? void 0 : n.call(this, !1, !0, t); e && e !== this._$AB; ) {
const i = e.nextSibling;
e.remove(), e = i;
}
}
setConnected(e) {
var t;
this._$AM === void 0 && (this._$Cv = e, (t = this._$AP) == null || t.call(this, e));
}
}
class ut {
get tagName() {
return this.element.tagName;
}
get _$AU() {
return this._$AM._$AU;
}
constructor(e, t, n, i, s) {
this.type = 1, this._$AH = A, this._$AN = void 0, this.element = e, this.name = t, this._$AM = i, this.options = s, n.length > 2 || n[0] !== "" || n[1] !== "" ? (this._$AH = Array(n.length - 1).fill(new String()), this.strings = n) : this._$AH = A;
}
_$AI(e, t = this, n, i) {
const s = this.strings;
let a = !1;
if (s === void 0)
e = Se(this, e, t, 0), a = !et(e) || e !== this._$AH && e !== ne, a && (this._$AH = e);
else {
const o = e;
let l, c;
for (e = s[0], l = 0; l < s.length - 1; l++)
c = Se(this, o[n + l], t, l), c === ne && (c = this._$AH[l]), a || (a = !et(c) || c !== this._$AH[l]), c === A ? e = A : e !== A && (e += (c ?? "") + s[l + 1]), this._$AH[l] = c;
}
a && !i && this.O(e);
}
O(e) {
e === A ? this.element.removeAttribute(this.name) : this.element.setAttribute(this.name, e ?? "");
}
}
class Pn extends ut {
constructor() {
super(...arguments), this.type = 3;
}
O(e) {
this.element[this.name] = e === A ? void 0 : e;
}
}
class Tn extends ut {
constructor() {
super(...arguments), this.type = 4;
}
O(e) {
this.element.toggleAttribute(this.name, !!e && e !== A);
}
}
class Fn extends ut {
constructor(e, t, n, i, s) {
super(e, t, n, i, s), this.type = 5;
}
_$AI(e, t = this) {
if ((e = Se(this, e, t, 0) ?? A) === ne)
return;
const n = this._$AH, i = e === A && n !== A || e.capture !== n.capture || e.once !== n.once || e.passive !== n.passive, s = e !== A && (n === A || i);
i && this.element.removeEventListener(this.name, this, n), s && this.element.addEventListener(this.name, this, e), this._$AH = e;
}
handleEvent(e) {
var t;
typeof this._$AH == "function" ? this._$AH.call(((t = this.options) == null ? void 0 : t.host) ?? this.element, e) : this._$AH.handleEvent(e);
}
}
class zn {
constructor(e, t, n) {
this.element = e, this.type = 6, this._$AN = void 0, this._$AM = t, this.options = n;
}
get _$AU() {
return this._$AM._$AU;
}
_$AI(e) {
Se(this, e);
}
}
const ns = { j: xr, P: te, A: $r, C: 1, M: En, L: Mn, R: _n, V: Se, D: Re, I: ut, H: Tn, N: Fn, U: Pn, B: zn }, Yt = Ye.litHtmlPolyfillSupport;
Yt == null || Yt(tt, Re), (Ye.litHtmlVersions ?? (Ye.litHtmlVersions = [])).push("3.1.0");
const is = (r, e, t) => {
const n = (t == null ? void 0 : t.renderBefore) ?? e;
let i = n._$litPart$;
if (i === void 0) {
const s = (t == null ? void 0 : t.renderBefore) ?? null;
n._$litPart$ = i = new Re(e.insertBefore(Xe(), s), s, void 0, t ?? {});
}
return i._$AI(r), i;
};
/**
* @license
* Copyright 2017 Google LLC
* SPDX-License-Identifier: BSD-3-Clause
*/
let Z = class extends Ie {
constructor() {
super(...arguments), this.renderOptions = { host: this }, this._$Do = void 0;
}
createRenderRoot() {
var t;
const e = super.createRenderRoot();
return (t = this.renderOptions).renderBefore ?? (t.renderBefore = e.firstChild), e;
}
update(e) {
const t = this.render();
this.hasUpdated || (this.renderOptions.isConnected = this.isConnected), super.update(e), this._$Do = is(t, this.renderRoot, this.renderOptions);
}
connectedCallback() {
var e;
super.connectedCallback(), (e = this._$Do) == null || e.setConnected(!0);
}
disconnectedCallback() {
var e;
super.disconnectedCallback(), (e = this._$Do) == null || e.setConnected(!1);
}
render() {
return ne;
}
};
var xn;
Z._$litElement$ = !0, Z.finalized = !0, (xn = globalThis.litElementHydrateSupport) == null || xn.call(globalThis, { LitElement: Z });
const Jt = globalThis.litElementPolyfillSupport;
Jt == null || Jt({ LitElement: Z });
(globalThis.litElementVersions ?? (globalThis.litElementVersions = [])).push("4.0.1");
/**
* @license
* Copyright 2017 Google LLC
* SPDX-License-Identifier: BSD-3-Clause
*/
const B = (r) => (e, t) => {
t !== void 0 ? t.addInitializer(() => {
customElements.define(r, e);
}) : customElements.define(r, e);
};
/**
* @license
* Copyright 2017 Google LLC
* SPDX-License-Identifier: BSD-3-Clause
*/
const ss = { attribute: !0, type: String, converter: kt, reflect: !1, hasChanged: wr }, as = (r = ss, e, t) => {
const { kind: n, metadata: i } = t;
let s = globalThis.litPropertyMetadata.get(i);
if (s === void 0 && globalThis.litPropertyMetadata.set(i, s = /* @__PURE__ */ new Map()), s.set(t.name, r), n === "accessor") {
const { name: a } = t;
return { set(o) {
const l = e.get.call(this);
e.set.call(this, o), this.requestUpdate(a, l, r);
}, init(o) {
return o !== void 0 && this.C(a, void 0, r), o;
} };
}
if (n === "setter") {
const { name: a } = t;
return function(o) {
const l = this[a];
e.call(this, o), this.requestUpdate(a, l, r);
};
}
throw Error("Unsupported decorator location: " + n);
};
function S(r) {
return (e, t) => typeof t == "object" ? as(r, e, t) : ((n, i, s) => {
const a = i.hasOwnProperty(s);
return i.constructor.createProperty(s, a ? { ...n, wrapped: !0 } : n), a ? Object.getOwnPropertyDescriptor(i, s) : void 0;
})(r, e, t);
}
/**
* @license
* Copyright 2017 Google LLC
* SPDX-License-Identifier: BSD-3-Clause
*/
function G(r) {
return S({ ...r, state: !0, attribute: !1 });
}
function os(r, e) {
return r.reduce((t, n) => {
const i = e(n);
return Object.prototype.hasOwnProperty.call(t, i) || (t[i] = []), t[i].push(n), t;
}, {});
}
const ls = "/";
function Qr(r, e, t) {
const n = Object.keys(r), i = cs(n), s = /* @__PURE__ */ Object.create(null);
return n.forEach((a) => {
const o = Xr(a.startsWith(e) ? a.substr(e.length) : a);
s[Xr(a.substr(i.length))] = t(r[a], o);
}), s;
}
function Xr(r) {
return r.split(/\/|\\/).filter(Boolean).join("/");
}
function cs(r) {
const e = r.map((n) => n.split(/\/|\\/).slice(0, -1));
if (r.length)
return e.reduce(t).join(ls);
return "";
function t(n, i) {
for (let s = 0; s < n.length; s++)
if (n[s] !== i[s])
return n.splice(0, s);
return n;
}
}
function us(r, e) {
const t = (n) => n.file ? `1${n.name}` : `0${n.name}`;
return t(r).localeCompare(t(e));
}
var X;
(function(r) {
r[r.maxAsciiCharacter = 127] = "maxAsciiCharacter", r[r.lineFeed = 10] = "lineFeed", r[r.carriageReturn = 13] = "carriageReturn", r[r.lineSeparator = 8232] = "lineSeparator", r[r.paragraphSeparator = 8233] = "paragraphSeparator";
})(X || (X = {}));
function ds(r) {
return r === X.lineFeed || r === X.carriageReturn || r === X.lineSeparator || r === X.paragraphSeparator;
}
function ps(r) {
const e = [];
let t = 0, n = 0;
function i(s) {
e.push(n), n = s;
}
for (i(0); t < r.length; ) {
const s = r.charCodeAt(t);
switch (t++, s) {
case X.carriageReturn:
r.charCodeAt(t) === X.lineFeed && t++, i(t);
break;
case X.lineFeed:
i(t);
break;
default:
s > X.maxAsciiCharacter && ds(s) && i(t);
break;
}
}
return e.push(n), e;
}
function Qt(r) {
if (r === void 0)
throw new Error("mutant.sourceFile was not defined");
}
var xe, $e;
class hs {
constructor(e) {
// MutantResult properties
x(this, "coveredBy");
x(this, "description");
x(this, "duration");
x(this, "id");
x(this, "killedBy");
x(this, "location");
x(this, "mutatorName");
x(this, "replacement");
x(this, "static");
x(this, "status");
x(this, "statusReason");
x(this, "testsCompleted");
me(this, xe, /* @__PURE__ */ new Map());
me(this, $e, /* @__PURE__ */ new Map());
this.coveredBy = e.coveredBy, this.description = e.description, this.duration = e.duration, this.id = e.id, this.killedBy = e.killedBy, this.location = e.location, this.mutatorName = e.mutatorName, this.replacement = e.replacement, this.static = e.static, this.status = e.status, this.statusReason = e.statusReason, this.testsCompleted = e.testsCompleted;
}
// New fields
get coveredByTests() {
if (D(this, xe).size)
return Array.from(D(this, xe).values());
}
set coveredByTests(e) {
Vt(this, xe, new Map(e.map((t) => [t.id, t])));
}
get killedByTests() {
if (D(this, $e).size)
return Array.from(D(this, $e).values());
}
set killedByTests(e) {
Vt(this, $e, new Map(e.map((t) => [t.id, t])));
}
addCoveredBy(e) {
D(this, xe).set(e.id, e);
}
addKilledBy(e) {
D(this, $e).set(e.id, e);
}
/**
* Retrieves the lines of code with the mutant applied to it, to be shown in a diff view.
*/
getMutatedLines() {
return Qt(this.sourceFile), this.sourceFile.getMutationLines(this);
}
/**
* Retrieves the original source lines for this mutant, to be shown in a diff view.
*/
getOriginalLines() {
return Qt(this.sourceFile), this.sourceFile.getLines(this.location);
}
/**
* Helper property to retrieve the source file name
* @throws When the `sourceFile` is not defined.
*/
get fileName() {
return Qt(this.sourceFile), this.sourceFile.name;
}
// TODO: https://github.com/stryker-mutator/mutation-testing-elements/pull/2453#discussion_r1178769871
update() {
var e, t;
(t = (e = this.sourceFile) == null ? void 0 : e.result) != null && t.file && this.sourceFile.result.updateAllMetrics();
}
}
xe = new WeakMap(), $e = new WeakMap();
function en(r) {
if (r === void 0)
throw new Error("sourceFile.source is undefined");
}
class On {
constructor() {
x(this, "lineMap");
}
getLineMap() {
return en(this.source), this.lineMap ?? (this.lineMap = ps(this.source));
}
/**
* Retrieves the source lines based on the `start.line` and `end.line` property.
*/
getLines(e) {
en(this.source);
const t = this.getLineMap();
return this.source.substring(t[e.start.line], t[(e.end ?? e.start).line + 1]);
}
}
class fs extends On {
/**
* @param input The file result content
* @param name The file name
*/
constructor(t, n) {
super();
x(this, "name");
/**
* Programming language that is used. Used for code highlighting, see https://prismjs.com/#examples.
*/
x(this, "language");
/**
* Full source code of the mutated file, this is used for highlighting.
*/
x(this, "source");
/**
* The mutants inside this file.
*/
x(this, "mutants");
/**
* The associated MetricsResult of this file.
*/
x(this, "result");
this.name = n, this.language = t.language, this.source = t.source, this.mutants = t.mutants.map((i) => {
const s = new hs(i);
return s.sourceFile = this, s;
});
}
/**
* Retrieves the lines of code with the mutant applied to it, to be shown in a diff view.
*/
getMutationLines(t) {
const n = this.getLineMap(), i = n[t.location.start.line], s = n[t.location.end.line], a = n[t.location.end.line + 1];
return `${this.source.substr(i, t.location.start.column - 1)}${t.replacement ?? t.description ?? t.mutatorName}${this.source.substring(s + t.location.end.column - 1, a)}`;
}
}
var lt, or;
class In {
constructor(e, t, n, i) {
me(this, lt);
/**
* The parent of this result (if it has one)
*/
x(this, "parent");
/**
* The name of this result
*/
x(this, "name");
/**
* The file belonging to this metric result (if it represents a single file)
*/
x(this, "file");
/**
* The the child results
*/
x(this, "childResults");
/**
* The actual metrics
*/
x(this, "metrics");
this.name = e, this.childResults = t, this.metrics = n, this.file = i;
}
updateParent(e) {
this.parent = e, this.childResults.forEach((t) => t.updateParent(this));
}
updateAllMetrics() {
if (this.parent !== void 0) {
this.parent.updateAllMetrics();
return;
}
this.updateMetrics();
}
updateMetrics() {
if (this.file === void 0) {
this.childResults.forEach((t) => {
t.updateMetrics();
});
const e = Wt(this, lt, or).call(this, this.childResults);
if (e.length === 0)
return;
e[0].tests ? this.metrics = lr(e) : this.metrics = At(e);
return;
}
this.file.tests ? this.metrics = lr([this.file]) : this.metrics = At([this.file]);
}
}
lt = new WeakSet(), or = function(e) {
const t = [];
return e.length === 0 || e.forEach((n) => {
if (n.file) {
t.push(n.file);
return;
}
t.push(...Wt(this, lt, or).call(this, n.childResults));
}), t;
};
function tn(r) {
if (r === void 0)
throw new Error("test.sourceFile was not defined");
}
function gs(r) {
if (r === void 0)
throw new Error("test.location was not defined");
}
var j;
(function(r) {
r.Killing = "Killing", r.Covering = "Covering", r.NotCovering = "NotCovering";
})(j || (j = {}));
var ke, _e;
class ms {
constructor(e) {
x(this, "id");
x(this, "name");
x(this, "location");
me(this, ke, /* @__PURE__ */ new Map());
me(this, _e, /* @__PURE__ */ new Map());
Object.entries(e).forEach(([t, n]) => {
this[t] = n;
});
}
get killedMutants() {
if (D(this, ke).size)
return Array.from(D(this, ke).values());
}
get coveredMutants() {
if (D(this, _e).size)
return Array.from(D(this, _e).values());
}
addCovered(e) {
D(this, _e).set(e.id, e);
}
addKilled(e) {
D(this, ke).set(e.id, e);
}
/**
* Retrieves the original source lines where this test is defined.
* @throws if source file or location is not defined
*/
getLines() {
return tn(this.sourceFile), gs(this.location), this.sourceFile.getLines(this.location);
}
/**
* Helper property to retrieve the source file name
* @throws When the `sourceFile` is not defined.
*/
get fileName() {
return tn(this.sourceFile), this.sourceFile.name;
}
get status() {
return D(this, ke).size ? j.Killing : D(this, _e).size ? j.Covering : j.NotCovering;
}
update() {
var e, t;
(t = (e = this.sourceFile) == null ? void 0 : e.result) != null && t.file && this.sourceFile.result.updateAllMetrics();
}
}
ke = new WeakMap(), _e = new WeakMap();
class bs extends On {
/**
* @param input the test file content
* @param name the file name
*/
constructor(t, n) {
super();
x(this, "name");
x(this, "tests");
x(this, "source");
/**
* The associated MetricsResult of this file.
*/
x(this, "result");
this.name = n, this.source = t.source, this.tests = t.tests.map((i) => {
const s = new ms(i);
return s.sourceFile = this, s;
});
}
}
const rn = NaN, nn = "All files", vs = "All tests";
function ys(r) {
const { files: e, testFiles: t, projectRoot: n = "" } = r, i = Qr(e, n, (s, a) => new fs(s, a));
if (t && Object.keys(t).length) {
const s = Qr(t, n, (a, o) => new bs(a, o));
return xs(Object.values(i).flatMap((a) => a.mutants), Object.values(s).flatMap((a) => a.tests)), {
systemUnderTestMetrics: Xt(nn, i, At),
testMetrics: Xt(vs, s, lr)
};
}
return {
systemUnderTestMetrics: Xt(nn, i, At),
testMetrics: void 0
};
}
function Xt(r, e, t) {
const n = Object.keys(e);
return n.length === 1 && n[0] === "" ? Dn(r, e[n[0]], t) : jn(r, e, t);
}
function jn(r, e, t) {
const n = t(Object.values(e)), i = ws(e, t);
return new In(r, i, n);
}
function Dn(r, e, t) {
return new In(r, [], t([e]), e);
}
function ws(r, e) {
const t = os(Object.entries(r), (n) => n[0].split("/")[0]);
return Object.keys(t).map((n) => {
if (t[n].length > 1 || t[n][0][0] !== n) {
const i = {};
return t[n].forEach((s) => i[s[0].substr(n.length + 1)] = s[1]), jn(n, i, e);
} else {
const [i, s] = t[n][0];
return Dn(i, s, e);
}
}).sort(us);
}
function xs(r, e) {
const t = new Map(e.map((n) => [n.id, n]));
for (const n of r) {
const i = n.coveredBy ?? [];
for (const a of i) {
const o = t.get(a);
o && (n.addCoveredBy(o), o.addCovered(n));
}
const s = n.killedBy ?? [];
for (const a of s) {
const o = t.get(a);
o && (n.addKilledBy(o), o.addKilled(n));
}
}
}
function lr(r) {
const e = r.flatMap((n) => n.tests), t = (n) => e.filter((i) => i.status === n).length;
return {
total: e.length,
killing: t(j.Killing),
covering: t(j.Covering),
notCovering: t(j.NotCovering)
};
}
function At(r) {
const e = r.flatMap((F) => F.mutants), t = (F) => e.filter((z) => z.status === F).length, n = t("Pending"), i = t("Killed"), s = t("Timeout"), a = t("Survived"), o = t("NoCoverage"), l = t("RuntimeError"), c = t("CompileError"), g = t("Ignored"), d = s + i, m = a + o, y = d + a, $ = m + d, k = l + c;
return {
pending: n,
killed: i,
timeout: s,
survived: a,
noCoverage: o,
runtimeErrors: l,
compileErrors: c,
ignored: g,
totalDetected: d,
totalUndetected: m,
totalCovered: y,
totalValid: $,
totalInvalid: k,
mutationScore: $ > 0 ? d / $ * 100 : rn,
totalMutants: $ + k + g + n,
mutationScoreBasedOnCoveredCode: $ > 0 ? d / y * 100 || 0 : rn
};
}
const $s = `/*! tailwindcss v3.3.5 | MIT License | https://tailwindcss.com*/*,:after,:before{border-color:rgb(var(--mut-gray-200,228 228 231)/1);border-style:solid;border-width:0;box-sizing:border-box}:after,:before{--tw-content:""}html{-webkit-text-size-adjust:100%;font-feature-settings:normal;font-family:ui-sans-serif,system-ui,-apple-system,BlinkMacSystemFont,Segoe UI,Roboto,Helvetica Neue,Arial,Noto Sans,sans-serif,Apple Color Emoji,Segoe UI Emoji,Segoe UI Symbol,Noto Color Emoji;font-variation-settings:normal;line-height:1.5;-moz-tab-size:4;tab-size:4}body{line-height:inherit;margin:0}hr{border-top-width:1px;color:inherit;height:0}abbr:where([title]){-webkit-text-decoration:underline dotted;text-decoration:underline dotted}h1,h2,h3,h4,h5,h6{font-size:inherit;font-weight:inherit}a{color:inherit;text-decoration:inherit}b,strong{font-weight:bolder}code,kbd,pre,samp{font-family:ui-monospace,SFMono-Regular,Menlo,Monaco,Consolas,Liberation Mono,Courier New,monospace;font-size:1em}small{font-size:80%}sub,sup{font-size:75%;line-height:0;position:relative;vertical-align:baseline}sub{bottom:-.25em}sup{top:-.5em}table{border-collapse:collapse;border-color:inherit;text-indent:0}button,input,optgroup,select,textarea{font-feature-settings:inherit;color:inherit;font-family:inherit;font-size:100%;font-variation-settings:inherit;font-weight:inherit;line-height:inherit;margin:0;padding:0}button,select{text-transform:none}[type=button],[type=reset],[type=submit],button{-webkit-appearance:button;background-color:transparent;background-image:none}:-moz-focusring{outline:auto}:-moz-ui-invalid{box-shadow:none}progress{vertical-align:baseline}::-webkit-inner-spin-button,::-webkit-outer-spin-button{height:auto}[type=search]{-webkit-appearance:textfield;outline-offset:-2px}::-webkit-search-decoration{-webkit-appearance:none}::-webkit-file-upload-button{-webkit-appearance:button;font:inherit}summary{display:list-item}blockquote,dd,dl,figure,h1,h2,h3,h4,h5,h6,hr,p,pre{margin:0}fieldset{margin:0}fieldset,legend{padding:0}menu,ol,ul{list-style:none;margin:0;padding:0}dialog{padding:0}textarea{resize:vertical}input::placeholder,textarea::placeholder{color:rgb(var(--mut-gray-400,161 161 170)/1);opacity:1}[role=button],button{cursor:pointer}:disabled{cursor:default}audio,canvas,embed,iframe,img,object,svg,video{display:block;vertical-align:middle}img,video{height:auto;max-width:100%}[hidden]{display:none}[multiple],[type=date],[type=datetime-local],[type=email],[type=month],[type=number],[type=password],[type=search],[type=tel],[type=text],[type=time],[type=url],[type=week],input:where(:not([type])),select,textarea{--tw-shadow:0 0 #0000;-webkit-appearance:none;-moz-appearance:none;appearance:none;background-color:#fff;border-color:rgb(var(--mut-gray-500,113 113 122)/var(--tw-border-opacity,1));border-radius:0;border-width:1px;font-size:1rem;line-height:1.5rem;padding:.5rem .75rem}[multiple]:focus,[type=date]:focus,[type=datetime-local]:focus,[type=email]:focus,[type=month]:focus,[type=number]:focus,[type=password]:focus,[type=search]:focus,[type=tel]:focus,[type=text]:focus,[type=time]:focus,[type=url]:focus,[type=week]:focus,input:where(:not([type])):focus,select:focus,textarea:focus{--tw-ring-inset:var(--tw-empty, );--tw-ring-offset-width:0px;--tw-ring-offset-color:#fff;--tw-ring-color:#2563eb;--tw-ring-offset-shadow:var(--tw-ring-inset) 0 0 0 var(--tw-ring-offset-width) var(--tw-ring-offset-color);--tw-ring-shadow:var(--tw-ring-inset) 0 0 0 calc(1px + var(--tw-ring-offset-width)) var(--tw-ring-color);border-color:#2563eb;box-shadow:var(--tw-ring-offset-shadow),var(--tw-ring-shadow),var(--tw-shadow);outline:2px solid transparent;outline-offset:2px}input::placeholder,textarea::placeholder{color:rgb(var(--mut-gray-500,113 113 122)/var(--tw-text-opacity,1));opacity:1}::-webkit-datetime-edit-fields-wrapper{padding:0}::-webkit-date-and-time-value{min-height:1.5em;text-align:inherit}::-webkit-datetime-edit{display:inline-flex}::-webkit-datetime-edit,::-webkit-datetime-edit-day-field,::-webkit-datetime-edit-hour-field,::-webkit-datetime-edit-meridiem-field,::-webkit-datetime-edit-millisecond-field,::-webkit-datetime-edit-minute-field,::-webkit-datetime-edit-month-field,::-webkit-datetime-edit-second-field,::-webkit-datetime-edit-year-field{padding-bottom:0;padding-top:0}select{background-image:url("data:image/svg+xml;charset=utf-8,%3Csvg xmlns='http://www.w3.org/2000/svg' fill='none' viewBox='0 0 20 20'%3E%3Cpath stroke='rgb(var(--mut-gray-500, 113 113 122) / var(--tw-stroke-opacity, 1))' stroke-linecap='round' stroke-linejoin='round' stroke-width='1.5' d='m6 8 4 4 4-4'/%3E%3C/svg%3E");background-position:right .5rem center;background-repeat:no-repeat;background-size:1.5em 1.5em;padding-right:2.5rem;-webkit-print-color-adjust:exact;print-color-adjust:exact}[multiple],[size]:where(select:not([size="1"])){background-image:none;background-position:0 0;background-repeat:unset;background-size:initial;padding-right:.75rem;-webkit-print-color-adjust:unset;print-color-adjust:unset}[type=checkbox],[type=radio]{--tw-shadow:0 0 #0000;-webkit-appearance:none;-moz-appearance:none;appearance:none;background-color:#fff;background-origin:border-box;border-color:rgb(var(--mut-gray-500,113 113 122)/var(--tw-border-opacity,1));border-width:1px;color:#2563eb;display:inline-block;flex-shrink:0;height:1rem;padding:0;-webkit-print-color-adjust:exact;print-color-adjust:exact;-webkit-user-select:none;user-select:none;vertical-align:middle;width:1rem}[type=checkbox]{border-radius:0}[type=radio]{border-radius:100%}[type=checkbox]:focus,[type=radio]:focus{--tw-ring-inset:var(--tw-empty, );--tw-ring-offset-width:2px;--tw-ring-offset-color:#fff;--tw-ring-color:#2563eb;--tw-ring-offset-shadow:var(--tw-ring-inset) 0 0 0 var(--tw-ring-offset-width) var(--tw-ring-offset-color);--tw-ring-shadow:var(--tw-ring-inset) 0 0 0 calc(2px + var(--tw-ring-offset-width)) var(--tw-ring-color);box-shadow:var(--tw-ring-offset-shadow),var(--tw-ring-shadow),var(--tw-shadow);outline:2px solid transparent;outline-offset:2px}[type=checkbox]:checked,[type=radio]:checked{background-color:currentColor;background-position:50%;background-repeat:no-repeat;background-size:100% 100%;border-color:transparent}[type=checkbox]:checked{background-image:url("data:image/svg+xml;charset=utf-8,%3Csvg xmlns='http://www.w3.org/2000/svg' fill='%23fff' viewBox='0 0 16 16'%3E%3Cpath d='M12.207 4.793a1 1 0 0 1 0 1.414l-5 5a1 1 0 0 1-1.414 0l-2-2a1 1 0 0 1 1.414-1.414L6.5 9.086l4.293-4.293a1 1 0 0 1 1.414 0z'/%3E%3C/svg%3E")}@media (forced-colors:active){[type=checkbox]:checked{-webkit-appearance:auto;-moz-appearance:auto;appearance:auto}}[type=radio]:checked{background-image:url("data:image/svg+xml;charset=utf-8,%3Csvg xmlns='http://www.w3.org/2000/svg' fill='%23fff' viewBox='0 0 16 16'%3E%3Ccircle cx='8' cy='8' r='3'/%3E%3C/svg%3E")}@media (forced-colors:active){[type=radio]:checked{-webkit-appearance:auto;-moz-appearance:auto;appearance:auto}}[type=checkbox]:checked:focus,[type=checkbox]:checked:hover,[type=radio]:checked:focus,[type=radio]:checked:hover{background-color:currentColor;border-color:transparent}[type=checkbox]:indeterminate{background-color:currentColor;background-image:url("data:image/svg+xml;charset=utf-8,%3Csvg xmlns='http://www.w3.org/2000/svg' fill='none' viewBox='0 0 16 16'%3E%3Cpath stroke='%23fff' stroke-linecap='round' stroke-linejoin='round' stroke-width='2' d='M4 8h8'/%3E%3C/svg%3E");background-position:50%;background-repeat:no-repeat;background-size:100% 100%;border-color:transparent}@media (forced-colors:active){[type=checkbox]:indeterminate{-webkit-appearance:auto;-moz-appearance:auto;appearance:auto}}[type=checkbox]:indeterminate:focus,[type=checkbox]:indeterminate:hover{background-color:currentColor;border-color:transparent}[type=file]{background:unset;border-color:inherit;border-radius:0;border-width:0;font-size:unset;line-height:inherit;padding:0}[type=file]:focus{outline:1px solid ButtonText;outline:1px auto -webkit-focus-ring-color}*,:after,:before{--tw-border-spacing-x:0;--tw-border-spacing-y:0;--tw-translate-x:0;--tw-translate-y:0;--tw-rotate:0;--tw-skew-x:0;--tw-skew-y:0;--tw-scale-x:1;--tw-scale-y:1;--tw-pan-x: ;--tw-pan-y: ;--tw-pinch-zoom: ;--tw-scroll-snap-strictness:proximity;--tw-gradient-from-position: ;--tw-gradient-via-position: ;--tw-gradient-to-position: ;--tw-ordinal: ;--tw-slashed-zero: ;--tw-numeric-figure: ;--tw-numeric-spacing: ;--tw-numeric-fraction: ;--tw-ring-inset: ;--tw-ring-offset-width:0px;--tw-ring-offset-color:#fff;--tw-ring-color:rgba(59,130,246,.5);--tw-ring-offset-shadow:0 0 #0000;--tw-ring-shadow:0 0 #0000;--tw-shadow:0 0 #0000;--tw-shadow-colored:0 0 #0000;--tw-blur: ;--tw-brightness: ;--tw-contrast: ;--tw-grayscale: ;--tw-hue-rotate: ;--tw-invert: ;--tw-saturate: ;--tw-sepia: ;--tw-drop-shadow: ;--tw-backdrop-blur: ;--tw-backdrop-brightness: ;--tw-backdrop-contrast: ;--tw-backdrop-grayscale: ;--tw-backdrop-hue-rotate: ;--tw-backdrop-invert: ;--tw-backdrop-opacity: ;--tw-backdrop-saturate: ;--tw-backdrop-sepia: }::backdrop{--tw-border-spacing-x:0;--tw-border-spacing-y:0;--tw-translate-x:0;--tw-translate-y:0;--tw-rotate:0;--tw-skew-x:0;--tw-skew-y:0;--tw-scale-x:1;--tw-scale-y:1;--tw-pan-x: ;--tw-pan-y: ;--tw-pinch-zoom: ;--tw-scroll-snap-strictness:proximity;--tw-gradient-from-position: ;--tw-gradient-via-position: ;--tw-gradient-to-position: ;--tw-ordinal: ;--tw-slashed-zero: ;--tw-numeric-figure: ;--tw-numeric-spacing: ;--tw-numeric-fraction: ;--tw-ring-inset: ;--tw-ring-offset-width:0px;--tw-ring-offset-color:#fff;--tw-ring-color:rgba(59,130,246,.5);--tw-ring-offset-shadow:0 0 #0000;--tw-ring-shadow:0 0 #0000;--tw-shadow:0 0 #0000;--tw-shadow-colored:0 0 #0000;--tw-blur: ;--tw-brightness: ;--tw-contrast: ;--tw-grayscale: ;--tw-hue-rotate: ;--tw-invert: ;--tw-saturate: ;--tw-sepia: ;--tw-drop-shadow: ;--tw-backdrop-blur: ;--tw-backdrop-brightness: ;--tw-backdrop-contrast: ;--tw-backdrop-grayscale: ;--tw-backdrop-hue-rotate: ;--tw-backdrop-invert: ;--tw-backdrop-opacity: ;--tw-backdrop-saturate: ;--tw-backdrop-sepia: }.container{margin-left:auto;margin-right:auto;width:100%}@media (min-width:640px){.container{max-width:640px}}@media (min-width:768px){.container{max-width:768px}}@media (min-width:1024px){.container{max-width:1024px}}@media (min-width:1280px){.container{max-width:1280px}}@media (min-width:1536px){.container{max-width:1536px}}@media (min-width:2000px){.container{max-width:2000px}}.sr-only{clip:rect(0,0,0,0);border-width:0;height:1px;margin:-1px;overflow:hidden;padding:0;position:absolute;white-space:nowrap;width:1px}.pointer-events-none{pointer-events:none}.visible{visibility:visible}.static{position:static}.fixed{position:fixed}.absolute{position:absolute}.relative{position:relative}.sticky{position:sticky}.bottom-0{bottom:0}.left-0{left:0}.top-0{top:0}.top-offset{top:var(--top-offset,0)}.z-10{z-index:10}.z-20{z-index:20}.float-right{float:right}.mx-1{margin-left:.25rem;margin-right:.25rem}.mx-2{margin-left:.5rem;margin-right:.5rem}.mx-6{margin-left:1.5rem;margin-right:1.5rem}.my-3{margin-bottom:.75rem;margin-top:.75rem}.my-4{margin-bottom:1rem;margin-top:1rem}.-mb-px{margin-bottom:-1px}.mb-6{margin-bottom:1.5rem}.ml-1{margin-left:.25rem}.ml-2{margin-left:.5rem}.ml-4{margin-left:1rem}.mr-12{margin-right:3rem}.mr-2{margin-right:.5rem}.mr-3{margin-right:.75rem}.mr-4{margin-right:1rem}.mr-6{margin-right:1.5rem}.mr-auto{margin-right:auto}.ms-3{margin-inline-start:.75rem}.mt-2{margin-top:.5rem}.block{display:block}.inline-block{display:inline-block}.inline{display:inline}.flex{display:flex}.inline-flex{display:inline-flex}.table{display:table}.h-2{height:.5rem}.h-3{height:.75rem}.h-4{height:1rem}.h-5{height:1.25rem}.h-8{height:2rem}.w-12{width:3rem}.w-24{width:6rem}.w-4{width:1rem}.w-5{width:1.25rem}.w-full{width:100%}.min-w-\\[24px\\]{min-width:24px}.max-w-6xl{max-width:72rem}.table-auto{table-layout:auto}.rotate-180{--tw-rotate:180deg;transform:translate(var(--tw-translate-x),var(--tw-translate-y)) rotate(var(--tw-rotate)) skew(var(--tw-skew-x)) skewY(var(--tw-skew-y)) scaleX(var(--tw-scale-x)) scaleY(var(--tw-scale-y))}.cursor-help{cursor:help}.cursor-pointer{cursor:pointer}.flex-row{flex-direction:row}.flex-col{flex-direction:column}.flex-wrap{flex-wrap:wrap}.items-center{align-items:center}.justify-start{justify-content:flex-start}.justify-center{justify-content:center}.justify-around{justify-content:space-around}.gap-4{gap:1rem}.space-y-4>:not([hidden])~:not([hidden]){--tw-space-y-reverse:0;margin-bottom:calc(1rem*var(--tw-space-y-reverse));margin-top:calc(1rem*(1 - var(--tw-space-y-reverse)))}.divide-y>:not([hidden])~:not([hidden]){--tw-divide-y-reverse:0;border-bottom-width:calc(1px*var(--tw-divide-y-reverse));border-top-width:calc(1px*(1 - var(--tw-divide-y-reverse)))}.divide-gray-200>:not([hidden])~:not([hidden]){--tw-divide-opacity:1;border-color:rgb(var(--mut-gray-200,228 228 231)/var(--tw-divide-opacity))}.overflow-hidden{overflow:hidden}.overflow-x-auto{overflow-x:auto}.rounded{border-radius:.25rem}.rounded-full{border-radius:9999px}.rounded-lg{border-radius:.5rem}.rounded-md{border-radius:.375rem}.rounded-t-3xl{border-top-left-radius:1.5rem;border-top-right-radius:1.5rem}.rounded-t-lg{border-top-left-radius:.5rem;border-top-right-radius:.5rem}.border{border-width:1px}.border-b{border-bottom-width:1px}.border-b-2{border-bottom-width:2px}.border-none{border-style:none}.border-gray-200{--tw-border-opacity:1;border-color:rgb(var(--mut-gray-200,228 228 231)/var(--tw-border-opacity))}.border-gray-300{--tw-border-opacity:1;border-color:rgb(var(--mut-gray-300,212 212 216)/var(--tw-border-opacity))}.border-primary-600{--tw-border-opacity:1;border-color:rgb(var(--mut-primary-600,2 132 199)/var(--tw-border-opacity))}.border-transparent{border-color:transparent}.bg-blue-600{--tw-bg-opacity:1;background-color:rgb(37 99 235/var(--tw-bg-opacity))}.bg-gray-100{--tw-bg-opacity:1;background-color:rgb(var(--mut-gray-100,244 244 245)/var(--tw-bg-opacity))}.bg-gray-200{--tw-bg-opacity:1;background-color:rgb(var(--mut-gray-200,228 228 231)/var(--tw-bg-opacity))}.bg-gray-200\\/60{background-color:rgb(var(--mut-gray-200,228 228 231)/.6)}.bg-gray-300{--tw-bg-opacity:1;background-color:rgb(var(--mut-gray-300,212 212 216)/var(--tw-bg-opacity))}.bg-green-100{--tw-bg-opacity:1;background-color:rgb(220 252 231/var(--tw-bg-opacity))}.bg-green-600{--tw-bg-opacity:1;background-color:rgb(22 163 74/var(--tw-bg-opacity))}.bg-orange-100{--tw-bg-opacity:1;background-color:rgb(255 237 213/var(--tw-bg-opacity))}.bg-primary-100{--tw-bg-opacity:1;background-color:rgb(var(--mut-primary-100,224 242 254)/var(--tw-bg-opacity))}.bg-red-100{--tw-bg-opacity:1;background-color:rgb(254 226 226/var(--tw-bg-opacity))}.bg-red-600{--tw-bg-opacity:1;background-color:rgb(220 38 38/var(--tw-bg-opacity))}.bg-white{--tw-bg-opacity:1;background-color:rgb(var(--mut-white,255 255 255)/var(--tw-bg-opacity))}.bg-yellow-100{--tw-bg-opacity:1;background-color:rgb(254 249 195/var(--tw-bg-opacity))}.bg-yellow-400{--tw-bg-opacity:1;background-color:rgb(250 204 21/var(--tw-bg-opacity))}.bg-yellow-600{--tw-bg-opacity:1;background-color:rgb(202 138 4/var(--tw-bg-opacity))}.p-1{padding:.25rem}.p-3{padding:.75rem}.p-4{padding:1rem}.px-2{padding-left:.5rem;padding-right:.5rem}.px-2\\.5{padding-left:.625rem;padding-right:.625rem}.px-4{padding-left:1rem;padding-right:1rem}.py-0{padding-bottom:0;padding-top:0}.py-0\\.5{padding-bottom:.125rem;padding-top:.125rem}.py-2{padding-bottom:.5rem;padding-top:.5rem}.py-3{padding-bottom:.75rem;padding-top:.75rem}.py-4{padding-bottom:1rem;padding-top:1rem}.py-6{padding-bottom:1.5rem;padding-top:1.5rem}.pb-4{padding-bottom:1rem}.pl-1{padding-left:.25rem}.pr-2{padding-right:.5rem}.pt-6{padding-top:1.5rem}.text-left{text-align:left}.text-center{text-align:center}.align-middle{vertical-align:middle}.font-sans{font-family:ui-sans-serif,system-ui,-apple-system,BlinkMacSystemFont,Segoe UI,Roboto,Helvetica Neue,Arial,Noto Sans,sans-serif,Apple Color Emoji,Segoe UI Emoji,Segoe UI Symbol,Noto Color Emoji}.text-5xl{font-size:3rem;line-height:1}.text-lg{font-size:1.125rem;line-height:1.75rem}.text-sm{font-size:.875rem;line-height:1.25rem}.font-bold{font-weight:700}.font-light{font-weight:300}.font-medium{font-weight:500}.font-semibold{font-weight:600}.tracking-tight{letter-spacing:-.025em}.text-gray-200{--tw-text-opacity:1;color:rgb(var(--mut-gray-200,228 228 231)/var(--tw-text-opacity))}.text-gray-600{--tw-text-opacity:1;color:rgb(var(--mut-gray-600,82 82 91)/var(--tw-text-opacity))}.text-gray-700{--tw-text-opacity:1;color:rgb(var(--mut-gray-700,63 63 70)/var(--tw-text-opacity))}.text-gray-800{--tw-text-opacity:1;color:rgb(var(--mut-gray-800,39 39 42)/var(--tw-text-opacity))}.text-green-700{--tw-text-opacity:1;color:rgb(21 128 61/var(--tw-text-opacity))}.text-green-800{--tw-text-opacity:1;color:rgb(22 101 52/var(--tw-text-opacity))}.text-orange-800{--tw-text-opacity:1;color:rgb(154 52 18/var(--tw-text-opacity))}.text-primary-800{--tw-text-opacity:1;color:rgb(var(--mut-primary-800,7 89 133)/var(--tw-text-opacity))}.text-primary-on{--tw-text-opacity:1;color:rgb(var(--mut-primary-on,3 105 161)/var(--tw-text-opacity))}.text-red-700{--tw-text-opacity:1;color:rgb(185 28 28/var(--tw-text-opacity))}.text-red-800{--tw-text-opacity:1;color:rgb(153 27 27/var(--tw-text-opacity))}.text-yellow-600{--tw-text-opacity:1;color:rgb(202 138 4/var(--tw-text-opacity))}.text-yellow-800{--tw-text-opacity:1;color:rgb(133 77 14/var(--tw-text-opacity))}.underline{text-decoration-line:underline}.decoration-dotted{text-decoration-style:dotted}.opacity-0{opacity:0}.shadow{--tw-shadow:0 1px 3px 0 rgba(0,0,0,.1),0 1px 2px -1px rgba(0,0,0,.1);--tw-shadow-colored:0 1px 3px 0 var(--tw-shadow-color),0 1px 2px -1px var(--tw-shadow-color)}.shadow,.shadow-xl{box-shadow:var(--tw-ring-offset-shadow,0 0 #0000),var(--tw-ring-shadow,0 0 #0000),var(--tw-shadow)}.shadow-xl{--tw-shadow:0 20px 25px -5px rgba(0,0,0,.1),0 8px 10px -6px rgba(0,0,0,.1);--tw-shadow-colored:0 20px 25px -5px var(--tw-shadow-color),0 8px 10px -6px var(--tw-shadow-color)}.\\!ring-offset-gray-200{--tw-ring-offset-color:rgb(var(--mut-gray-200,228 228 231)/1)!important}.filter{filter:var(--tw-blur) var(--tw-brightness) var(--tw-contrast) var(--tw-grayscale) var(--tw-hue-rotate) var(--tw-invert) var(--tw-saturate) var(--tw-sepia) var(--tw-drop-shadow)}.backdrop-blur-lg{--tw-backdrop-blur:blur(16px);-webkit-backdrop-filter:var(--tw-backdrop-blur) var(--tw-backdrop-brightness) var(--tw-backdrop-contrast) var(--tw-backdrop-grayscale) var(--tw-backdrop-hue-rotate) var(--tw-backdrop-invert) var(--tw-backdrop-opacity) var(--tw-backdrop-saturate) var(--tw-backdrop-sepia);backdrop-filter:var(--tw-backdrop-blur) var(--tw-backdrop-brightness) var(--tw-backdrop-contrast) var(--tw-backdrop-grayscale) var(--tw-backdrop-hue-rotate) var(--tw-backdrop-invert) var(--tw-backdrop-opacity) var(--tw-backdrop-saturate) var(--tw-backdrop-sepia)}.transition-all{transition-duration:.15s;transition-property:all;transition-timing-function:cubic-bezier(.4,0,.2,1)}.transition-colors{transition-duration:.15s;transition-property:color,background-color,border-color,text-decoration-color,fill,stroke;transition-timing-function:cubic-bezier(.4,0,.2,1)}.after\\:text-gray-800:after{--tw-text-opacity:1;color:rgb(var(--mut-gray-800,39 39 42)/var(--tw-text-opacity));content:var(--tw-content)}.after\\:content-\\[\\'\\/\\'\\]:after{--tw-content:"/";content:var(--tw-content)}.even\\:bg-gray-100:nth-child(2n),.odd\\:bg-gray-100:nth-child(odd){--tw-bg-opacity:1;background-color:rgb(var(--mut-gray-100,244 244 245)/var(--tw-bg-opacity))}.hover\\:cursor-pointer:hover{cursor:pointer}.hover\\:border-gray-300:hover{--tw-border-opacity:1;border-color:rgb(var(--mut-gray-300,212 212 216)/var(--tw-border-opacity))}.hover\\:bg-gray-100:hover{--tw-bg-opacity:1;background-color:rgb(var(--mut-gray-100,244 244 245)/var(--tw-bg-opacity))}.hover\\:bg-gray-200:hover{--tw-bg-opacity:1;background-color:rgb(var(--mut-gray-200,228 228 231)/var(--tw-bg-opacity))}.hover\\:text-gray-700:hover{--tw-text-opacity:1;color:rgb(var(--mut-gray-700,63 63 70)/var(--tw-text-opacity))}.hover\\:text-gray-900:hover{--tw-text-opacity:1;color:rgb(var(--mut-gray-900,24 24 27)/var(--tw-text-opacity))}.hover\\:text-primary-on:hover{--tw-text-opacity:1;color:rgb(var(--mut-primary-on,3 105 161)/var(--tw-text-opacity))}.hover\\:underline:hover{text-decoration-line:underline}.focus\\:outline-none:focus{outline:2px solid transparent;outline-offset:2px}.focus\\:ring-2:focus{--tw-ring-offset-shadow:var(--tw-ring-inset) 0 0 0 var(--tw-ring-offset-width) var(--tw-ring-offset-color);--tw-ring-shadow:var(--tw-ring-inset) 0 0 0 calc(2px + var(--tw-ring-offset-width)) var(--tw-ring-color);box-shadow:var(--tw-ring-offset-shadow),var(--tw-ring-shadow),var(--tw-shadow,0 0 #0000)}.focus\\:ring-primary-500:focus{--tw-ring-opacity:1;--tw-ring-color:rgb(var(--mut-primary-500,14 165 233)/var(--tw-ring-opacity))}.active\\:bg-gray-200:active,.group:hover .group-hover\\:bg-gray-200{--tw-bg-opacity:1;background-color:rgb(var(--mut-gray-200,228 228 231)/var(--tw-bg-opacity))}.aria-selected\\:border-b-\\[3px\\][aria-selected=true]{border-bottom-width:3px}.aria-selected\\:border-primary-700[aria-selected=true]{--tw-border-opacity:1;border-color:rgb(var(--mut-primary-700,3 105 161)/var(--tw-border-opacity))}.aria-selected\\:text-primary-on[aria-selected=true]{--tw-text-opacity:1;color:rgb(var(--mut-primary-on,3 105 161)/var(--tw-text-opacity))}@media (prefers-reduced-motion:no-preference){.motion-safe\\:transition-\\[height\\,max-width\\]{transition-duration:.15s;transition-property:height,max-width;transition-timing-function:cubic-bezier(.4,0,.2,1)}.motion-safe\\:transition-max-width{transition-duration:.15s;transition-property:max-width;transition-timing-function:cubic-bezier(.4,0,.2,1)}.motion-safe\\:duration-200{transition-duration:.2s}}@media (min-width:768px){.md\\:ml-2{margin-left:.5rem}.md\\:after\\:pl-1:after{content:var(--tw-content);padding-left:.25rem}}@media (min-width:1536px){.\\32xl\\:w-28{width:7rem}}`, ks = "code[class*=language-],pre[class*=language-]{color:var(--prism-maintext);direction:ltr;font-family:Consolas,Monaco,Andale Mono,Ubuntu Mono,monospace;font-size:1em;-webkit-hyphens:none;hyphens:none;line-height:1.5;-moz-tab-size:4;tab-size:4;text-align:left;white-space:pre;word-break:normal;word-spacing:normal}pre>code[class*=language-]{font-size:1em}pre[class*=language-]{border:1px solid var(--prism-border);border-radius:.25rem;margin:.5em 0;overflow:auto;padding:1em}:not(pre)>code[class*=language-],pre[class*=language-]{background:var(--prism-background)}.token.cdata,.token.comment,.token.doctype,.token.italic,.token.prolog{font-style:italic}.token.bold,.token.function,.token.important{font-weight:700}.token.namespace{opacity:.7}.token.atrule{color:var(--prism-atrule)}.token.attr{color:var(--prism-attr)}.token.attr-name{color:var(--prism-attr-name)}.token.boolean{color:var(--prism-boolean)}.token.builtin{color:var(--prism-builtin)}.token.cdata{color:var(--prism-cdata)}.token.changed{color:var(--prism-changed)}.token.char{color:var(--prism-char)}.token.comment{color:var(--prism-comment)}.token.constant{color:var(--prism-constant)}.token.deleted{color:var(--prism-deleted)}.token.doctype{color:var(--prism-doctype)}.token.entity{color:var(--prism-entity);cursor:help}.token.function{color:var(--prism-function)}.token.function-variable{color:var(--prism-function-variable,var(--prism-function))}.token.inserted{color:var(--prism-inserted)}.token.keyword{color:var(--prism-keyword)}.token.number{color:var(--prism-number)}.token.operator{color:var(--prism-operator)}.token.prolog{color:var(--prism-prolog)}.token.property{color:var(--prism-property)}.token.punctuation{color:var(--prism-punctuation)}.token.regex{color:var(--prism-regex)}.token.selector{color:var(--prism-selector)}.token.string{color:var(--prism-string)}.token.symbol{color:var(--prism-symbol)}.token.tag{color:var(--prism-tag)}.token.url{color:var(--prism-url)}.token.variable{color:var(--prism-variable)}.token.placeholder{color:var(--prism-placeholder)}.token.statement{color:var(--prism-statement)}.token.attr-value{color:var(--prism-attr-value)}.token.control{color:var(--prism-control)}.token.directive{color:var(--prism-directive)}.token.unit{color:var(--prism-unit)}.token.important{color:var(--prism-important)}.token.class-name{color:var(--prism-class-name)}pre[class*=language-].line-numbers{counter-reset:linenumber;padding-left:3.8em;position:relative}pre[class*=language-].line-numbers>code{position:relative;white-space:inherit}.line-numbers .line-numbers-rows{border-right:1px solid #999;font-size:100%;left:-3.8em;letter-spacing:-1px;pointer-events:none;position:absolute;top:0;-webkit-user-select:none;user-select:none;width:3em}.line-numbers-rows>span{counter-increment:linenumber;display:block}.line-numbers-rows>span:before{color:#999;content:counter(linenumber);display:block;padding-right:.8em;text-align:right}", _s = "";
var sn = typeof globalThis < "u" ? globalThis : typeof window < "u" ? window : typeof global < "u" ? global : typeof self < "u" ? self : {}, Bn = { exports: {} };
(function(r) {
var e = typeof window < "u" ? window : typeof WorkerGlobalScope < "u" && self instanceof WorkerGlobalScope ? self : {};
/**
* Prism: Lightweight, robust, elegant syntax highlighting
*
* @license MIT
* @author Lea Verou
* @namespace
* @public
*/
var t = function(n) {
var i = /(?:^|\s)lang(?:uage)?-([\w-]+)(?=\s|$)/i, s = 0, a = {}, o = {
/**
* By default, Prism will attempt to highlight all code elements (by calling {@link Prism.highlightAll}) on the
* current page after the page finished loading. This might be a problem if e.g. you wanted to asynchronously load
* additional languages or plugins yourself.
*
* By setting this value to `true`, Prism will not automatically highlight all code elements on the page.
*
* You obviously have to change this value before the automatic highlighting started. To do this, you can add an
* empty Prism object into the global scope before loading the Prism script like this:
*
* ```js
* window.Prism = window.Prism || {};
* Prism.manual = true;
* // add a new