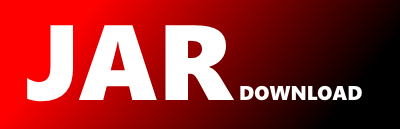
testmodel-js.constant_tck.js Maven / Gradle / Ivy
/*
* Copyright 2014 Red Hat, Inc.
*
* Red Hat licenses this file to you under the Apache License, version 2.0
* (the "License"); you may not use this file except in compliance with the
* License. You may obtain a copy of the License at:
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations
* under the License.
*/
/** @module testmodel-js/constant_tck */
var utils = require('vertx-js/util/utils');
var RefedInterface1 = require('testmodel-js/refed_interface1');
var io = Packages.io;
var JsonObject = io.vertx.core.json.JsonObject;
var JConstantTCK = Java.type('io.vertx.codegen.testmodel.ConstantTCK');
var TestDataObject = Java.type('io.vertx.codegen.testmodel.TestDataObject');
/**
@class
*/
var ConstantTCK = function(j_val) {
var j_constantTCK = j_val;
var that = this;
// A reference to the underlying Java delegate
// NOTE! This is an internal API and must not be used in user code.
// If you rely on this property your code is likely to break if we change it / remove it without warning.
this._jdel = j_constantTCK;
};
ConstantTCK._jclass = utils.getJavaClass("io.vertx.codegen.testmodel.ConstantTCK");
ConstantTCK._jtype = {accept: function(obj) {
return ConstantTCK._jclass.isInstance(obj._jdel);
},wrap: function(jdel) {
var obj = Object.create(ConstantTCK.prototype, {});
ConstantTCK.apply(obj, arguments);
return obj;
},
unwrap: function(obj) {
return obj._jdel;
}
};
ConstantTCK._create = function(jdel) {var obj = Object.create(ConstantTCK.prototype, {});
ConstantTCK.apply(obj, arguments);
return obj;
}
ConstantTCK.BYTE = JConstantTCK.BYTE;
ConstantTCK.BOXED_BYTE = JConstantTCK.BOXED_BYTE;
ConstantTCK.SHORT = JConstantTCK.SHORT;
ConstantTCK.BOXED_SHORT = JConstantTCK.BOXED_SHORT;
ConstantTCK.INT = JConstantTCK.INT;
ConstantTCK.BOXED_INT = JConstantTCK.BOXED_INT;
ConstantTCK.LONG = JConstantTCK.LONG;
ConstantTCK.BOXED_LONG = utils.convReturnLong(JConstantTCK.BOXED_LONG);
ConstantTCK.FLOAT = JConstantTCK.FLOAT;
ConstantTCK.BOXED_FLOAT = JConstantTCK.BOXED_FLOAT;
ConstantTCK.DOUBLE = JConstantTCK.DOUBLE;
ConstantTCK.BOXED_DOUBLE = JConstantTCK.BOXED_DOUBLE;
ConstantTCK.BOOLEAN = JConstantTCK.BOOLEAN;
ConstantTCK.BOXED_BOOLEAN = JConstantTCK.BOXED_BOOLEAN;
ConstantTCK.CHAR = JConstantTCK.CHAR;
ConstantTCK.BOXED_CHAR = JConstantTCK.BOXED_CHAR;
ConstantTCK.STRING = JConstantTCK.STRING;
ConstantTCK.VERTX_GEN = utils.convReturnVertxGen(RefedInterface1, JConstantTCK.VERTX_GEN);
ConstantTCK.DATA_OBJECT = utils.convReturnDataObject(JConstantTCK.DATA_OBJECT);
ConstantTCK.JSON_OBJECT = utils.convReturnJson(JConstantTCK.JSON_OBJECT);
ConstantTCK.JSON_ARRAY = utils.convReturnJson(JConstantTCK.JSON_ARRAY);
ConstantTCK.ENUM = utils.convReturnEnum(JConstantTCK.ENUM);
ConstantTCK.THROWABLE = utils.convReturnThrowable(JConstantTCK.THROWABLE);
ConstantTCK.OBJECT = utils.convReturnTypeUnknown(JConstantTCK.OBJECT);
ConstantTCK.NULLABLE_NON_NULL = utils.convReturnVertxGen(RefedInterface1, JConstantTCK.NULLABLE_NON_NULL);
ConstantTCK.NULLABLE_NULL = utils.convReturnVertxGen(RefedInterface1, JConstantTCK.NULLABLE_NULL);
ConstantTCK.BYTE_LIST = JConstantTCK.BYTE_LIST;
ConstantTCK.SHORT_LIST = JConstantTCK.SHORT_LIST;
ConstantTCK.INT_LIST = JConstantTCK.INT_LIST;
ConstantTCK.LONG_LIST = utils.convReturnListSetLong(JConstantTCK.LONG_LIST);
ConstantTCK.FLOAT_LIST = JConstantTCK.FLOAT_LIST;
ConstantTCK.DOUBLE_LIST = JConstantTCK.DOUBLE_LIST;
ConstantTCK.BOOLEAN_LIST = JConstantTCK.BOOLEAN_LIST;
ConstantTCK.CHAR_LIST = JConstantTCK.CHAR_LIST;
ConstantTCK.STRING_LIST = JConstantTCK.STRING_LIST;
ConstantTCK.VERTX_GEN_LIST = utils.convReturnListSetVertxGen(JConstantTCK.VERTX_GEN_LIST, RefedInterface1);
ConstantTCK.JSON_OBJECT_LIST = utils.convReturnListSetJson(JConstantTCK.JSON_OBJECT_LIST);
ConstantTCK.JSON_ARRAY_LIST = utils.convReturnListSetJson(JConstantTCK.JSON_ARRAY_LIST);
ConstantTCK.DATA_OBJECT_LIST = utils.convReturnListSetDataObject(JConstantTCK.DATA_OBJECT_LIST);
ConstantTCK.ENUM_LIST = utils.convReturnListSetEnum(JConstantTCK.ENUM_LIST);
ConstantTCK.BYTE_SET = utils.convReturnSet(JConstantTCK.BYTE_SET);
ConstantTCK.SHORT_SET = utils.convReturnSet(JConstantTCK.SHORT_SET);
ConstantTCK.INT_SET = utils.convReturnSet(JConstantTCK.INT_SET);
ConstantTCK.LONG_SET = utils.convReturnListSetLong(JConstantTCK.LONG_SET);
ConstantTCK.FLOAT_SET = utils.convReturnSet(JConstantTCK.FLOAT_SET);
ConstantTCK.DOUBLE_SET = utils.convReturnSet(JConstantTCK.DOUBLE_SET);
ConstantTCK.BOOLEAN_SET = utils.convReturnSet(JConstantTCK.BOOLEAN_SET);
ConstantTCK.CHAR_SET = utils.convReturnSet(JConstantTCK.CHAR_SET);
ConstantTCK.STRING_SET = utils.convReturnSet(JConstantTCK.STRING_SET);
ConstantTCK.VERTX_GEN_SET = utils.convReturnListSetVertxGen(JConstantTCK.VERTX_GEN_SET, RefedInterface1);
ConstantTCK.JSON_OBJECT_SET = utils.convReturnListSetJson(JConstantTCK.JSON_OBJECT_SET);
ConstantTCK.JSON_ARRAY_SET = utils.convReturnListSetJson(JConstantTCK.JSON_ARRAY_SET);
ConstantTCK.DATA_OBJECT_SET = utils.convReturnListSetDataObject(JConstantTCK.DATA_OBJECT_SET);
ConstantTCK.ENUM_SET = utils.convReturnListSetEnum(JConstantTCK.ENUM_SET);
ConstantTCK.BYTE_MAP = utils.convReturnMap(JConstantTCK.BYTE_MAP);
ConstantTCK.SHORT_MAP = utils.convReturnMap(JConstantTCK.SHORT_MAP);
ConstantTCK.INT_MAP = utils.convReturnMap(JConstantTCK.INT_MAP);
ConstantTCK.LONG_MAP = utils.convReturnMap(JConstantTCK.LONG_MAP);
ConstantTCK.FLOAT_MAP = utils.convReturnMap(JConstantTCK.FLOAT_MAP);
ConstantTCK.DOUBLE_MAP = utils.convReturnMap(JConstantTCK.DOUBLE_MAP);
ConstantTCK.BOOLEAN_MAP = utils.convReturnMap(JConstantTCK.BOOLEAN_MAP);
ConstantTCK.CHAR_MAP = utils.convReturnMap(JConstantTCK.CHAR_MAP);
ConstantTCK.STRING_MAP = utils.convReturnMap(JConstantTCK.STRING_MAP);
ConstantTCK.JSON_OBJECT_MAP = utils.convReturnMap(JConstantTCK.JSON_OBJECT_MAP);
ConstantTCK.JSON_ARRAY_MAP = utils.convReturnMap(JConstantTCK.JSON_ARRAY_MAP);
module.exports = ConstantTCK;
© 2015 - 2024 Weber Informatics LLC | Privacy Policy