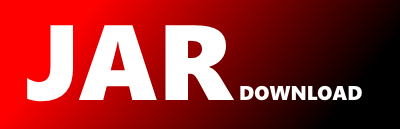
testmodel-js.function_param_tck.js Maven / Gradle / Ivy
/*
* Copyright 2014 Red Hat, Inc.
*
* Red Hat licenses this file to you under the Apache License, version 2.0
* (the "License"); you may not use this file except in compliance with the
* License. You may obtain a copy of the License at:
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations
* under the License.
*/
/** @module testmodel-js/function_param_tck */
var utils = require('vertx-js/util/utils');
var GenericRefedInterface = require('testmodel-js/generic_refed_interface');
var RefedInterface1 = require('testmodel-js/refed_interface1');
var io = Packages.io;
var JsonObject = io.vertx.core.json.JsonObject;
var JFunctionParamTCK = Java.type('io.vertx.codegen.testmodel.FunctionParamTCK');
var TestDataObject = Java.type('io.vertx.codegen.testmodel.TestDataObject');
/**
@class
*/
var FunctionParamTCK = function(j_val) {
var j_functionParamTCK = j_val;
var that = this;
var __super_methodWithBasicParam = this.methodWithBasicParam;
var __super_methodWithJsonParam = this.methodWithJsonParam;
var __super_methodWithVoidParam = this.methodWithVoidParam;
var __super_methodWithUserTypeParam = this.methodWithUserTypeParam;
var __super_methodWithObjectParam = this.methodWithObjectParam;
var __super_methodWithDataObjectParam = this.methodWithDataObjectParam;
var __super_methodWithEnumParam = this.methodWithEnumParam;
var __super_methodWithListParam = this.methodWithListParam;
var __super_methodWithSetParam = this.methodWithSetParam;
var __super_methodWithMapParam = this.methodWithMapParam;
var __super_methodWithGenericParam = this.methodWithGenericParam;
var __super_methodWithGenericUserTypeParam = this.methodWithGenericUserTypeParam;
var __super_methodWithBasicReturn = this.methodWithBasicReturn;
var __super_methodWithJsonReturn = this.methodWithJsonReturn;
var __super_methodWithObjectReturn = this.methodWithObjectReturn;
var __super_methodWithDataObjectReturn = this.methodWithDataObjectReturn;
var __super_methodWithEnumReturn = this.methodWithEnumReturn;
var __super_methodWithListReturn = this.methodWithListReturn;
var __super_methodWithSetReturn = this.methodWithSetReturn;
var __super_methodWithMapReturn = this.methodWithMapReturn;
var __super_methodWithGenericReturn = this.methodWithGenericReturn;
var __super_methodWithGenericUserTypeReturn = this.methodWithGenericUserTypeReturn;
var __super_methodWithNullableListParam = this.methodWithNullableListParam;
var __super_methodWithNullableListReturn = this.methodWithNullableListReturn;
/**
@public
@param byteFunc {function}
@param shortFunc {function}
@param integerFunc {function}
@param longFunc {function}
@param floatFunc {function}
@param doubleFunc {function}
@param booleanFunc {function}
@param charFunc {function}
@param stringFunc {function}
@return {Array.}
*/
this.methodWithBasicParam = function(byteFunc, shortFunc, integerFunc, longFunc, floatFunc, doubleFunc, booleanFunc, charFunc, stringFunc) {
var __args = arguments;
if (__args.length === 9 && typeof __args[0] === 'function' && typeof __args[1] === 'function' && typeof __args[2] === 'function' && typeof __args[3] === 'function' && typeof __args[4] === 'function' && typeof __args[5] === 'function' && typeof __args[6] === 'function' && typeof __args[7] === 'function' && typeof __args[8] === 'function') {
return j_functionParamTCK["methodWithBasicParam(java.util.function.Function,java.util.function.Function,java.util.function.Function,java.util.function.Function,java.util.function.Function,java.util.function.Function,java.util.function.Function,java.util.function.Function,java.util.function.Function)"](function(jVal) {
var jRet = __args[0](jVal);
return jRet;
}, function(jVal) {
var jRet = __args[1](jVal);
return jRet;
}, function(jVal) {
var jRet = __args[2](jVal);
return jRet;
}, function(jVal) {
var jRet = __args[3](utils.convReturnLong(jVal));
return jRet;
}, function(jVal) {
var jRet = __args[4](jVal);
return jRet;
}, function(jVal) {
var jRet = __args[5](jVal);
return jRet;
}, function(jVal) {
var jRet = __args[6](jVal);
return jRet;
}, function(jVal) {
var jRet = __args[7](jVal);
return jRet;
}, function(jVal) {
var jRet = __args[8](jVal);
return jRet;
}) ;
} else if (typeof __super_methodWithBasicParam != 'undefined') {
return __super_methodWithBasicParam.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param objectFunc {function}
@param arrayFunc {function}
@return {Array.}
*/
this.methodWithJsonParam = function(objectFunc, arrayFunc) {
var __args = arguments;
if (__args.length === 2 && typeof __args[0] === 'function' && typeof __args[1] === 'function') {
return j_functionParamTCK["methodWithJsonParam(java.util.function.Function,java.util.function.Function)"](function(jVal) {
var jRet = __args[0](utils.convReturnJson(jVal));
return jRet;
}, function(jVal) {
var jRet = __args[1](utils.convReturnJson(jVal));
return jRet;
}) ;
} else if (typeof __super_methodWithJsonParam != 'undefined') {
return __super_methodWithJsonParam.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param func {function}
@return {string}
*/
this.methodWithVoidParam = function(func) {
var __args = arguments;
if (__args.length === 1 && typeof __args[0] === 'function') {
return j_functionParamTCK["methodWithVoidParam(java.util.function.Function)"](__args[0]) ;
} else if (typeof __super_methodWithVoidParam != 'undefined') {
return __super_methodWithVoidParam.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param arg {RefedInterface1}
@param func {function}
@return {string}
*/
this.methodWithUserTypeParam = function(arg, func) {
var __args = arguments;
if (__args.length === 2 && typeof __args[0] === 'object' && __args[0]._jdel && typeof __args[1] === 'function') {
return j_functionParamTCK["methodWithUserTypeParam(io.vertx.codegen.testmodel.RefedInterface1,java.util.function.Function)"](__args[0]._jdel, function(jVal) {
var jRet = __args[1](utils.convReturnVertxGen(RefedInterface1, jVal));
return jRet;
}) ;
} else if (typeof __super_methodWithUserTypeParam != 'undefined') {
return __super_methodWithUserTypeParam.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param arg {Object}
@param func {function}
@return {string}
*/
this.methodWithObjectParam = function(arg, func) {
var __args = arguments;
if (__args.length === 2 && typeof __args[0] !== 'function' && typeof __args[1] === 'function') {
return j_functionParamTCK["methodWithObjectParam(java.lang.Object,java.util.function.Function)"](utils.convParamTypeUnknown(__args[0]), function(jVal) {
var jRet = __args[1](utils.convReturnTypeUnknown(jVal));
return jRet;
}) ;
} else if (typeof __super_methodWithObjectParam != 'undefined') {
return __super_methodWithObjectParam.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param func {function}
@return {string}
*/
this.methodWithDataObjectParam = function(func) {
var __args = arguments;
if (__args.length === 1 && typeof __args[0] === 'function') {
return j_functionParamTCK["methodWithDataObjectParam(java.util.function.Function)"](function(jVal) {
var jRet = __args[0](utils.convReturnDataObject(jVal));
return jRet;
}) ;
} else if (typeof __super_methodWithDataObjectParam != 'undefined') {
return __super_methodWithDataObjectParam.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param func {function}
@return {string}
*/
this.methodWithEnumParam = function(func) {
var __args = arguments;
if (__args.length === 1 && typeof __args[0] === 'function') {
return j_functionParamTCK["methodWithEnumParam(java.util.function.Function)"](function(jVal) {
var jRet = __args[0](utils.convReturnEnum(jVal));
return jRet;
}) ;
} else if (typeof __super_methodWithEnumParam != 'undefined') {
return __super_methodWithEnumParam.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param stringFunc {function}
@return {string}
*/
this.methodWithListParam = function(stringFunc) {
var __args = arguments;
if (__args.length === 1 && typeof __args[0] === 'function') {
return j_functionParamTCK["methodWithListParam(java.util.function.Function)"](function(jVal) {
var jRet = __args[0](jVal);
return jRet;
}) ;
} else if (typeof __super_methodWithListParam != 'undefined') {
return __super_methodWithListParam.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param func {function}
@return {string}
*/
this.methodWithSetParam = function(func) {
var __args = arguments;
if (__args.length === 1 && typeof __args[0] === 'function') {
return j_functionParamTCK["methodWithSetParam(java.util.function.Function)"](function(jVal) {
var jRet = __args[0](utils.convReturnSet(jVal));
return jRet;
}) ;
} else if (typeof __super_methodWithSetParam != 'undefined') {
return __super_methodWithSetParam.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param func {function}
@return {string}
*/
this.methodWithMapParam = function(func) {
var __args = arguments;
if (__args.length === 1 && typeof __args[0] === 'function') {
return j_functionParamTCK["methodWithMapParam(java.util.function.Function)"](function(jVal) {
var jRet = __args[0](utils.convReturnMap(jVal));
return jRet;
}) ;
} else if (typeof __super_methodWithMapParam != 'undefined') {
return __super_methodWithMapParam.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param t {Object}
@param func {function}
@return {string}
*/
this.methodWithGenericParam = function(t, func) {
var __args = arguments;
if (__args.length === 2 && typeof __args[0] !== 'function' && typeof __args[1] === 'function') {
return j_functionParamTCK["methodWithGenericParam(java.lang.Object,java.util.function.Function)"](utils.convParamTypeUnknown(__args[0]), function(jVal) {
var jRet = __args[1](utils.convReturnTypeUnknown(jVal));
return jRet;
}) ;
} else if (typeof __super_methodWithGenericParam != 'undefined') {
return __super_methodWithGenericParam.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param t {Object}
@param func {function}
@return {string}
*/
this.methodWithGenericUserTypeParam = function(t, func) {
var __args = arguments;
if (__args.length === 2 && typeof __args[0] !== 'function' && typeof __args[1] === 'function') {
return j_functionParamTCK["methodWithGenericUserTypeParam(java.lang.Object,java.util.function.Function)"](utils.convParamTypeUnknown(__args[0]), function(jVal) {
var jRet = __args[1](utils.convReturnVertxGen(GenericRefedInterface, jVal, undefined));
return jRet;
}) ;
} else if (typeof __super_methodWithGenericUserTypeParam != 'undefined') {
return __super_methodWithGenericUserTypeParam.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param byteFunc {function}
@param shortFunc {function}
@param integerFunc {function}
@param longFunc {function}
@param floatFunc {function}
@param doubleFunc {function}
@param booleanFunc {function}
@param charFunc {function}
@param stringFunc {function}
@return {string}
*/
this.methodWithBasicReturn = function(byteFunc, shortFunc, integerFunc, longFunc, floatFunc, doubleFunc, booleanFunc, charFunc, stringFunc) {
var __args = arguments;
if (__args.length === 9 && typeof __args[0] === 'function' && typeof __args[1] === 'function' && typeof __args[2] === 'function' && typeof __args[3] === 'function' && typeof __args[4] === 'function' && typeof __args[5] === 'function' && typeof __args[6] === 'function' && typeof __args[7] === 'function' && typeof __args[8] === 'function') {
return j_functionParamTCK["methodWithBasicReturn(java.util.function.Function,java.util.function.Function,java.util.function.Function,java.util.function.Function,java.util.function.Function,java.util.function.Function,java.util.function.Function,java.util.function.Function,java.util.function.Function)"](function(jVal) {
var jRet = __args[0](jVal);
return utils.convParamByte(jRet);
}, function(jVal) {
var jRet = __args[1](jVal);
return utils.convParamShort(jRet);
}, function(jVal) {
var jRet = __args[2](jVal);
return utils.convParamInteger(jRet);
}, function(jVal) {
var jRet = __args[3](jVal);
return utils.convParamLong(jRet);
}, function(jVal) {
var jRet = __args[4](jVal);
return utils.convParamFloat(jRet);
}, function(jVal) {
var jRet = __args[5](jVal);
return utils.convParamDouble(jRet);
}, function(jVal) {
var jRet = __args[6](jVal);
return jRet;
}, function(jVal) {
var jRet = __args[7](jVal);
return utils.convParamCharacter(jRet);
}, function(jVal) {
var jRet = __args[8](jVal);
return jRet;
}) ;
} else if (typeof __super_methodWithBasicReturn != 'undefined') {
return __super_methodWithBasicReturn.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param objectFunc {function}
@param arrayFunc {function}
@return {string}
*/
this.methodWithJsonReturn = function(objectFunc, arrayFunc) {
var __args = arguments;
if (__args.length === 2 && typeof __args[0] === 'function' && typeof __args[1] === 'function') {
return j_functionParamTCK["methodWithJsonReturn(java.util.function.Function,java.util.function.Function)"](function(jVal) {
var jRet = __args[0](jVal);
return utils.convParamJsonObject(jRet);
}, function(jVal) {
var jRet = __args[1](jVal);
return utils.convParamJsonArray(jRet);
}) ;
} else if (typeof __super_methodWithJsonReturn != 'undefined') {
return __super_methodWithJsonReturn.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param func {function}
@return {string}
*/
this.methodWithObjectReturn = function(func) {
var __args = arguments;
if (__args.length === 1 && typeof __args[0] === 'function') {
return j_functionParamTCK["methodWithObjectReturn(java.util.function.Function)"](function(jVal) {
var jRet = __args[0](jVal);
return utils.convParamTypeUnknown(jRet);
}) ;
} else if (typeof __super_methodWithObjectReturn != 'undefined') {
return __super_methodWithObjectReturn.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param func {function}
@return {string}
*/
this.methodWithDataObjectReturn = function(func) {
var __args = arguments;
if (__args.length === 1 && typeof __args[0] === 'function') {
return j_functionParamTCK["methodWithDataObjectReturn(java.util.function.Function)"](function(jVal) {
var jRet = __args[0](jVal);
return jRet != null ? new TestDataObject(new JsonObject(Java.asJSONCompatible(jRet))) : null;
}) ;
} else if (typeof __super_methodWithDataObjectReturn != 'undefined') {
return __super_methodWithDataObjectReturn.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param func {function}
@return {string}
*/
this.methodWithEnumReturn = function(func) {
var __args = arguments;
if (__args.length === 1 && typeof __args[0] === 'function') {
return j_functionParamTCK["methodWithEnumReturn(java.util.function.Function)"](function(jVal) {
var jRet = __args[0](jVal);
return io.vertx.codegen.testmodel.TestEnum.valueOf(jRet);
}) ;
} else if (typeof __super_methodWithEnumReturn != 'undefined') {
return __super_methodWithEnumReturn.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param func {function}
@return {string}
*/
this.methodWithListReturn = function(func) {
var __args = arguments;
if (__args.length === 1 && typeof __args[0] === 'function') {
return j_functionParamTCK["methodWithListReturn(java.util.function.Function)"](function(jVal) {
var jRet = __args[0](jVal);
return utils.convParamListBasicOther(jRet);
}) ;
} else if (typeof __super_methodWithListReturn != 'undefined') {
return __super_methodWithListReturn.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param func {function}
@return {string}
*/
this.methodWithSetReturn = function(func) {
var __args = arguments;
if (__args.length === 1 && typeof __args[0] === 'function') {
return j_functionParamTCK["methodWithSetReturn(java.util.function.Function)"](function(jVal) {
var jRet = __args[0](jVal);
return utils.convParamSetBasicOther(jRet);
}) ;
} else if (typeof __super_methodWithSetReturn != 'undefined') {
return __super_methodWithSetReturn.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param func {function}
@return {string}
*/
this.methodWithMapReturn = function(func) {
var __args = arguments;
if (__args.length === 1 && typeof __args[0] === 'function') {
return j_functionParamTCK["methodWithMapReturn(java.util.function.Function)"](function(jVal) {
var jRet = __args[0](jVal);
return jRet;
}) ;
} else if (typeof __super_methodWithMapReturn != 'undefined') {
return __super_methodWithMapReturn.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param func {function}
@return {string}
*/
this.methodWithGenericReturn = function(func) {
var __args = arguments;
if (__args.length === 1 && typeof __args[0] === 'function') {
return j_functionParamTCK["methodWithGenericReturn(java.util.function.Function)"](function(jVal) {
var jRet = __args[0](jVal);
return utils.convParamTypeUnknown(jRet);
}) ;
} else if (typeof __super_methodWithGenericReturn != 'undefined') {
return __super_methodWithGenericReturn.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param func {function}
@return {string}
*/
this.methodWithGenericUserTypeReturn = function(func) {
var __args = arguments;
if (__args.length === 1 && typeof __args[0] === 'function') {
return j_functionParamTCK["methodWithGenericUserTypeReturn(java.util.function.Function)"](function(jVal) {
var jRet = __args[0](utils.convReturnVertxGen(GenericRefedInterface, jVal, undefined));
return jRet._jdel;
}) ;
} else if (typeof __super_methodWithGenericUserTypeReturn != 'undefined') {
return __super_methodWithGenericUserTypeReturn.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param func {function}
@return {string}
*/
this.methodWithNullableListParam = function(func) {
var __args = arguments;
if (__args.length === 1 && typeof __args[0] === 'function') {
return j_functionParamTCK["methodWithNullableListParam(java.util.function.Function)"](function(jVal) {
var jRet = __args[0](jVal);
return jRet;
}) ;
} else if (typeof __super_methodWithNullableListParam != 'undefined') {
return __super_methodWithNullableListParam.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param func {function}
@return {string}
*/
this.methodWithNullableListReturn = function(func) {
var __args = arguments;
if (__args.length === 1 && typeof __args[0] === 'function') {
return j_functionParamTCK["methodWithNullableListReturn(java.util.function.Function)"](function(jVal) {
var jRet = __args[0](jVal);
return utils.convParamListBasicOther(jRet);
}) ;
} else if (typeof __super_methodWithNullableListReturn != 'undefined') {
return __super_methodWithNullableListReturn.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
// A reference to the underlying Java delegate
// NOTE! This is an internal API and must not be used in user code.
// If you rely on this property your code is likely to break if we change it / remove it without warning.
this._jdel = j_functionParamTCK;
};
FunctionParamTCK._jclass = utils.getJavaClass("io.vertx.codegen.testmodel.FunctionParamTCK");
FunctionParamTCK._jtype = {accept: function(obj) {
return FunctionParamTCK._jclass.isInstance(obj._jdel);
},wrap: function(jdel) {
var obj = Object.create(FunctionParamTCK.prototype, {});
FunctionParamTCK.apply(obj, arguments);
return obj;
},
unwrap: function(obj) {
return obj._jdel;
}
};
FunctionParamTCK._create = function(jdel) {var obj = Object.create(FunctionParamTCK.prototype, {});
FunctionParamTCK.apply(obj, arguments);
return obj;
}
module.exports = FunctionParamTCK;
© 2015 - 2024 Weber Informatics LLC | Privacy Policy