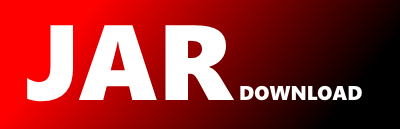
testmodel-js.generics_tck.js Maven / Gradle / Ivy
/*
* Copyright 2014 Red Hat, Inc.
*
* Red Hat licenses this file to you under the Apache License, version 2.0
* (the "License"); you may not use this file except in compliance with the
* License. You may obtain a copy of the License at:
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations
* under the License.
*/
/** @module testmodel-js/generics_tck */
var utils = require('vertx-js/util/utils');
var GenericRefedInterface = require('testmodel-js/generic_refed_interface');
var InterfaceWithApiArg = require('testmodel-js/interface_with_api_arg');
var InterfaceWithVariableArg = require('testmodel-js/interface_with_variable_arg');
var InterfaceWithStringArg = require('testmodel-js/interface_with_string_arg');
var RefedInterface1 = require('testmodel-js/refed_interface1');
var GenericNullableRefedInterface = require('testmodel-js/generic_nullable_refed_interface');
var io = Packages.io;
var JsonObject = io.vertx.core.json.JsonObject;
var JGenericsTCK = Java.type('io.vertx.codegen.testmodel.GenericsTCK');
var TestDataObject = Java.type('io.vertx.codegen.testmodel.TestDataObject');
/**
@class
*/
var GenericsTCK = function(j_val) {
var j_genericsTCK = j_val;
var that = this;
var __super_methodWithByteParameterizedReturn = this.methodWithByteParameterizedReturn;
var __super_methodWithShortParameterizedReturn = this.methodWithShortParameterizedReturn;
var __super_methodWithIntegerParameterizedReturn = this.methodWithIntegerParameterizedReturn;
var __super_methodWithLongParameterizedReturn = this.methodWithLongParameterizedReturn;
var __super_methodWithFloatParameterizedReturn = this.methodWithFloatParameterizedReturn;
var __super_methodWithDoubleParameterizedReturn = this.methodWithDoubleParameterizedReturn;
var __super_methodWithBooleanParameterizedReturn = this.methodWithBooleanParameterizedReturn;
var __super_methodWithCharacterParameterizedReturn = this.methodWithCharacterParameterizedReturn;
var __super_methodWithStringParameterizedReturn = this.methodWithStringParameterizedReturn;
var __super_methodWithJsonObjectParameterizedReturn = this.methodWithJsonObjectParameterizedReturn;
var __super_methodWithJsonArrayParameterizedReturn = this.methodWithJsonArrayParameterizedReturn;
var __super_methodWithDataObjectParameterizedReturn = this.methodWithDataObjectParameterizedReturn;
var __super_methodWithEnumParameterizedReturn = this.methodWithEnumParameterizedReturn;
var __super_methodWithGenEnumParameterizedReturn = this.methodWithGenEnumParameterizedReturn;
var __super_methodWithUserTypeParameterizedReturn = this.methodWithUserTypeParameterizedReturn;
var __super_methodWithHandlerByteParameterized = this.methodWithHandlerByteParameterized;
var __super_methodWithHandlerShortParameterized = this.methodWithHandlerShortParameterized;
var __super_methodWithHandlerIntegerParameterized = this.methodWithHandlerIntegerParameterized;
var __super_methodWithHandlerLongParameterized = this.methodWithHandlerLongParameterized;
var __super_methodWithHandlerFloatParameterized = this.methodWithHandlerFloatParameterized;
var __super_methodWithHandlerDoubleParameterized = this.methodWithHandlerDoubleParameterized;
var __super_methodWithHandlerBooleanParameterized = this.methodWithHandlerBooleanParameterized;
var __super_methodWithHandlerCharacterParameterized = this.methodWithHandlerCharacterParameterized;
var __super_methodWithHandlerStringParameterized = this.methodWithHandlerStringParameterized;
var __super_methodWithHandlerJsonObjectParameterized = this.methodWithHandlerJsonObjectParameterized;
var __super_methodWithHandlerJsonArrayParameterized = this.methodWithHandlerJsonArrayParameterized;
var __super_methodWithHandlerDataObjectParameterized = this.methodWithHandlerDataObjectParameterized;
var __super_methodWithHandlerEnumParameterized = this.methodWithHandlerEnumParameterized;
var __super_methodWithHandlerGenEnumParameterized = this.methodWithHandlerGenEnumParameterized;
var __super_methodWithHandlerUserTypeParameterized = this.methodWithHandlerUserTypeParameterized;
var __super_methodWithHandlerAsyncResultByteParameterized = this.methodWithHandlerAsyncResultByteParameterized;
var __super_methodWithHandlerAsyncResultShortParameterized = this.methodWithHandlerAsyncResultShortParameterized;
var __super_methodWithHandlerAsyncResultIntegerParameterized = this.methodWithHandlerAsyncResultIntegerParameterized;
var __super_methodWithHandlerAsyncResultLongParameterized = this.methodWithHandlerAsyncResultLongParameterized;
var __super_methodWithHandlerAsyncResultFloatParameterized = this.methodWithHandlerAsyncResultFloatParameterized;
var __super_methodWithHandlerAsyncResultDoubleParameterized = this.methodWithHandlerAsyncResultDoubleParameterized;
var __super_methodWithHandlerAsyncResultBooleanParameterized = this.methodWithHandlerAsyncResultBooleanParameterized;
var __super_methodWithHandlerAsyncResultCharacterParameterized = this.methodWithHandlerAsyncResultCharacterParameterized;
var __super_methodWithHandlerAsyncResultStringParameterized = this.methodWithHandlerAsyncResultStringParameterized;
var __super_methodWithHandlerAsyncResultJsonObjectParameterized = this.methodWithHandlerAsyncResultJsonObjectParameterized;
var __super_methodWithHandlerAsyncResultJsonArrayParameterized = this.methodWithHandlerAsyncResultJsonArrayParameterized;
var __super_methodWithHandlerAsyncResultDataObjectParameterized = this.methodWithHandlerAsyncResultDataObjectParameterized;
var __super_methodWithHandlerAsyncResultEnumParameterized = this.methodWithHandlerAsyncResultEnumParameterized;
var __super_methodWithHandlerAsyncResultGenEnumParameterized = this.methodWithHandlerAsyncResultGenEnumParameterized;
var __super_methodWithHandlerAsyncResultUserTypeParameterized = this.methodWithHandlerAsyncResultUserTypeParameterized;
var __super_methodWithFunctionParamByteParameterized = this.methodWithFunctionParamByteParameterized;
var __super_methodWithFunctionParamShortParameterized = this.methodWithFunctionParamShortParameterized;
var __super_methodWithFunctionParamIntegerParameterized = this.methodWithFunctionParamIntegerParameterized;
var __super_methodWithFunctionParamLongParameterized = this.methodWithFunctionParamLongParameterized;
var __super_methodWithFunctionParamFloatParameterized = this.methodWithFunctionParamFloatParameterized;
var __super_methodWithFunctionParamDoubleParameterized = this.methodWithFunctionParamDoubleParameterized;
var __super_methodWithFunctionParamBooleanParameterized = this.methodWithFunctionParamBooleanParameterized;
var __super_methodWithFunctionParamCharacterParameterized = this.methodWithFunctionParamCharacterParameterized;
var __super_methodWithFunctionParamStringParameterized = this.methodWithFunctionParamStringParameterized;
var __super_methodWithFunctionParamJsonObjectParameterized = this.methodWithFunctionParamJsonObjectParameterized;
var __super_methodWithFunctionParamJsonArrayParameterized = this.methodWithFunctionParamJsonArrayParameterized;
var __super_methodWithFunctionParamDataObjectParameterized = this.methodWithFunctionParamDataObjectParameterized;
var __super_methodWithFunctionParamEnumParameterized = this.methodWithFunctionParamEnumParameterized;
var __super_methodWithFunctionParamGenEnumParameterized = this.methodWithFunctionParamGenEnumParameterized;
var __super_methodWithFunctionParamUserTypeParameterized = this.methodWithFunctionParamUserTypeParameterized;
var __super_methodWithClassTypeParameterizedReturn = this.methodWithClassTypeParameterizedReturn;
var __super_methodWithHandlerClassTypeParameterized = this.methodWithHandlerClassTypeParameterized;
var __super_methodWithHandlerAsyncResultClassTypeParameterized = this.methodWithHandlerAsyncResultClassTypeParameterized;
var __super_methodWithFunctionParamClassTypeParameterized = this.methodWithFunctionParamClassTypeParameterized;
var __super_methodWithClassTypeParam = this.methodWithClassTypeParam;
var __super_methodWithClassTypeReturn = this.methodWithClassTypeReturn;
var __super_methodWithClassTypeHandler = this.methodWithClassTypeHandler;
var __super_methodWithClassTypeHandlerAsyncResult = this.methodWithClassTypeHandlerAsyncResult;
var __super_methodWithClassTypeFunctionParam = this.methodWithClassTypeFunctionParam;
var __super_methodWithClassTypeFunctionReturn = this.methodWithClassTypeFunctionReturn;
var __super_interfaceWithApiArg = this.interfaceWithApiArg;
var __super_interfaceWithStringArg = this.interfaceWithStringArg;
var __super_interfaceWithVariableArg = this.interfaceWithVariableArg;
var __super_methodWithHandlerGenericNullableApi = this.methodWithHandlerGenericNullableApi;
var __super_methodWithHandlerAsyncResultGenericNullableApi = this.methodWithHandlerAsyncResultGenericNullableApi;
var __super_methodWithGenericNullableApiReturn = this.methodWithGenericNullableApiReturn;
var __super_methodWithParamInferedReturn = this.methodWithParamInferedReturn;
var __super_methodWithHandlerParamInfered = this.methodWithHandlerParamInfered;
var __super_methodWithHandlerAsyncResultParamInfered = this.methodWithHandlerAsyncResultParamInfered;
/**
@public
@return {GenericRefedInterface}
*/
this.methodWithByteParameterizedReturn = function() {
var __args = arguments;
if (__args.length === 0) {
return utils.convReturnVertxGen(GenericRefedInterface, j_genericsTCK["methodWithByteParameterizedReturn()"](), undefined) ;
} else if (typeof __super_methodWithByteParameterizedReturn != 'undefined') {
return __super_methodWithByteParameterizedReturn.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@return {GenericRefedInterface}
*/
this.methodWithShortParameterizedReturn = function() {
var __args = arguments;
if (__args.length === 0) {
return utils.convReturnVertxGen(GenericRefedInterface, j_genericsTCK["methodWithShortParameterizedReturn()"](), undefined) ;
} else if (typeof __super_methodWithShortParameterizedReturn != 'undefined') {
return __super_methodWithShortParameterizedReturn.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@return {GenericRefedInterface}
*/
this.methodWithIntegerParameterizedReturn = function() {
var __args = arguments;
if (__args.length === 0) {
return utils.convReturnVertxGen(GenericRefedInterface, j_genericsTCK["methodWithIntegerParameterizedReturn()"](), undefined) ;
} else if (typeof __super_methodWithIntegerParameterizedReturn != 'undefined') {
return __super_methodWithIntegerParameterizedReturn.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@return {GenericRefedInterface}
*/
this.methodWithLongParameterizedReturn = function() {
var __args = arguments;
if (__args.length === 0) {
return utils.convReturnVertxGen(GenericRefedInterface, j_genericsTCK["methodWithLongParameterizedReturn()"](), undefined) ;
} else if (typeof __super_methodWithLongParameterizedReturn != 'undefined') {
return __super_methodWithLongParameterizedReturn.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@return {GenericRefedInterface}
*/
this.methodWithFloatParameterizedReturn = function() {
var __args = arguments;
if (__args.length === 0) {
return utils.convReturnVertxGen(GenericRefedInterface, j_genericsTCK["methodWithFloatParameterizedReturn()"](), undefined) ;
} else if (typeof __super_methodWithFloatParameterizedReturn != 'undefined') {
return __super_methodWithFloatParameterizedReturn.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@return {GenericRefedInterface}
*/
this.methodWithDoubleParameterizedReturn = function() {
var __args = arguments;
if (__args.length === 0) {
return utils.convReturnVertxGen(GenericRefedInterface, j_genericsTCK["methodWithDoubleParameterizedReturn()"](), undefined) ;
} else if (typeof __super_methodWithDoubleParameterizedReturn != 'undefined') {
return __super_methodWithDoubleParameterizedReturn.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@return {GenericRefedInterface}
*/
this.methodWithBooleanParameterizedReturn = function() {
var __args = arguments;
if (__args.length === 0) {
return utils.convReturnVertxGen(GenericRefedInterface, j_genericsTCK["methodWithBooleanParameterizedReturn()"](), undefined) ;
} else if (typeof __super_methodWithBooleanParameterizedReturn != 'undefined') {
return __super_methodWithBooleanParameterizedReturn.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@return {GenericRefedInterface}
*/
this.methodWithCharacterParameterizedReturn = function() {
var __args = arguments;
if (__args.length === 0) {
return utils.convReturnVertxGen(GenericRefedInterface, j_genericsTCK["methodWithCharacterParameterizedReturn()"](), undefined) ;
} else if (typeof __super_methodWithCharacterParameterizedReturn != 'undefined') {
return __super_methodWithCharacterParameterizedReturn.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@return {GenericRefedInterface}
*/
this.methodWithStringParameterizedReturn = function() {
var __args = arguments;
if (__args.length === 0) {
return utils.convReturnVertxGen(GenericRefedInterface, j_genericsTCK["methodWithStringParameterizedReturn()"](), undefined) ;
} else if (typeof __super_methodWithStringParameterizedReturn != 'undefined') {
return __super_methodWithStringParameterizedReturn.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@return {GenericRefedInterface}
*/
this.methodWithJsonObjectParameterizedReturn = function() {
var __args = arguments;
if (__args.length === 0) {
return utils.convReturnVertxGen(GenericRefedInterface, j_genericsTCK["methodWithJsonObjectParameterizedReturn()"](), undefined) ;
} else if (typeof __super_methodWithJsonObjectParameterizedReturn != 'undefined') {
return __super_methodWithJsonObjectParameterizedReturn.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@return {GenericRefedInterface}
*/
this.methodWithJsonArrayParameterizedReturn = function() {
var __args = arguments;
if (__args.length === 0) {
return utils.convReturnVertxGen(GenericRefedInterface, j_genericsTCK["methodWithJsonArrayParameterizedReturn()"](), undefined) ;
} else if (typeof __super_methodWithJsonArrayParameterizedReturn != 'undefined') {
return __super_methodWithJsonArrayParameterizedReturn.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@return {GenericRefedInterface}
*/
this.methodWithDataObjectParameterizedReturn = function() {
var __args = arguments;
if (__args.length === 0) {
return utils.convReturnVertxGen(GenericRefedInterface, j_genericsTCK["methodWithDataObjectParameterizedReturn()"](), undefined) ;
} else if (typeof __super_methodWithDataObjectParameterizedReturn != 'undefined') {
return __super_methodWithDataObjectParameterizedReturn.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@return {GenericRefedInterface}
*/
this.methodWithEnumParameterizedReturn = function() {
var __args = arguments;
if (__args.length === 0) {
return utils.convReturnVertxGen(GenericRefedInterface, j_genericsTCK["methodWithEnumParameterizedReturn()"](), utils.enum_jtype(io.vertx.codegen.testmodel.TestEnum)) ;
} else if (typeof __super_methodWithEnumParameterizedReturn != 'undefined') {
return __super_methodWithEnumParameterizedReturn.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@return {GenericRefedInterface}
*/
this.methodWithGenEnumParameterizedReturn = function() {
var __args = arguments;
if (__args.length === 0) {
return utils.convReturnVertxGen(GenericRefedInterface, j_genericsTCK["methodWithGenEnumParameterizedReturn()"](), utils.enum_jtype(io.vertx.codegen.testmodel.TestGenEnum)) ;
} else if (typeof __super_methodWithGenEnumParameterizedReturn != 'undefined') {
return __super_methodWithGenEnumParameterizedReturn.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@return {GenericRefedInterface}
*/
this.methodWithUserTypeParameterizedReturn = function() {
var __args = arguments;
if (__args.length === 0) {
return utils.convReturnVertxGen(GenericRefedInterface, j_genericsTCK["methodWithUserTypeParameterizedReturn()"](), RefedInterface1._jtype) ;
} else if (typeof __super_methodWithUserTypeParameterizedReturn != 'undefined') {
return __super_methodWithUserTypeParameterizedReturn.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param handler {function}
*/
this.methodWithHandlerByteParameterized = function(handler) {
var __args = arguments;
if (__args.length === 1 && typeof __args[0] === 'function') {
j_genericsTCK["methodWithHandlerByteParameterized(io.vertx.core.Handler)"](function(jVal) {
__args[0](utils.convReturnVertxGen(GenericRefedInterface, jVal, undefined));
});
} else if (typeof __super_methodWithHandlerByteParameterized != 'undefined') {
return __super_methodWithHandlerByteParameterized.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param handler {function}
*/
this.methodWithHandlerShortParameterized = function(handler) {
var __args = arguments;
if (__args.length === 1 && typeof __args[0] === 'function') {
j_genericsTCK["methodWithHandlerShortParameterized(io.vertx.core.Handler)"](function(jVal) {
__args[0](utils.convReturnVertxGen(GenericRefedInterface, jVal, undefined));
});
} else if (typeof __super_methodWithHandlerShortParameterized != 'undefined') {
return __super_methodWithHandlerShortParameterized.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param handler {function}
*/
this.methodWithHandlerIntegerParameterized = function(handler) {
var __args = arguments;
if (__args.length === 1 && typeof __args[0] === 'function') {
j_genericsTCK["methodWithHandlerIntegerParameterized(io.vertx.core.Handler)"](function(jVal) {
__args[0](utils.convReturnVertxGen(GenericRefedInterface, jVal, undefined));
});
} else if (typeof __super_methodWithHandlerIntegerParameterized != 'undefined') {
return __super_methodWithHandlerIntegerParameterized.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param handler {function}
*/
this.methodWithHandlerLongParameterized = function(handler) {
var __args = arguments;
if (__args.length === 1 && typeof __args[0] === 'function') {
j_genericsTCK["methodWithHandlerLongParameterized(io.vertx.core.Handler)"](function(jVal) {
__args[0](utils.convReturnVertxGen(GenericRefedInterface, jVal, undefined));
});
} else if (typeof __super_methodWithHandlerLongParameterized != 'undefined') {
return __super_methodWithHandlerLongParameterized.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param handler {function}
*/
this.methodWithHandlerFloatParameterized = function(handler) {
var __args = arguments;
if (__args.length === 1 && typeof __args[0] === 'function') {
j_genericsTCK["methodWithHandlerFloatParameterized(io.vertx.core.Handler)"](function(jVal) {
__args[0](utils.convReturnVertxGen(GenericRefedInterface, jVal, undefined));
});
} else if (typeof __super_methodWithHandlerFloatParameterized != 'undefined') {
return __super_methodWithHandlerFloatParameterized.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param handler {function}
*/
this.methodWithHandlerDoubleParameterized = function(handler) {
var __args = arguments;
if (__args.length === 1 && typeof __args[0] === 'function') {
j_genericsTCK["methodWithHandlerDoubleParameterized(io.vertx.core.Handler)"](function(jVal) {
__args[0](utils.convReturnVertxGen(GenericRefedInterface, jVal, undefined));
});
} else if (typeof __super_methodWithHandlerDoubleParameterized != 'undefined') {
return __super_methodWithHandlerDoubleParameterized.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param handler {function}
*/
this.methodWithHandlerBooleanParameterized = function(handler) {
var __args = arguments;
if (__args.length === 1 && typeof __args[0] === 'function') {
j_genericsTCK["methodWithHandlerBooleanParameterized(io.vertx.core.Handler)"](function(jVal) {
__args[0](utils.convReturnVertxGen(GenericRefedInterface, jVal, undefined));
});
} else if (typeof __super_methodWithHandlerBooleanParameterized != 'undefined') {
return __super_methodWithHandlerBooleanParameterized.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param handler {function}
*/
this.methodWithHandlerCharacterParameterized = function(handler) {
var __args = arguments;
if (__args.length === 1 && typeof __args[0] === 'function') {
j_genericsTCK["methodWithHandlerCharacterParameterized(io.vertx.core.Handler)"](function(jVal) {
__args[0](utils.convReturnVertxGen(GenericRefedInterface, jVal, undefined));
});
} else if (typeof __super_methodWithHandlerCharacterParameterized != 'undefined') {
return __super_methodWithHandlerCharacterParameterized.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param handler {function}
*/
this.methodWithHandlerStringParameterized = function(handler) {
var __args = arguments;
if (__args.length === 1 && typeof __args[0] === 'function') {
j_genericsTCK["methodWithHandlerStringParameterized(io.vertx.core.Handler)"](function(jVal) {
__args[0](utils.convReturnVertxGen(GenericRefedInterface, jVal, undefined));
});
} else if (typeof __super_methodWithHandlerStringParameterized != 'undefined') {
return __super_methodWithHandlerStringParameterized.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param handler {function}
*/
this.methodWithHandlerJsonObjectParameterized = function(handler) {
var __args = arguments;
if (__args.length === 1 && typeof __args[0] === 'function') {
j_genericsTCK["methodWithHandlerJsonObjectParameterized(io.vertx.core.Handler)"](function(jVal) {
__args[0](utils.convReturnVertxGen(GenericRefedInterface, jVal, undefined));
});
} else if (typeof __super_methodWithHandlerJsonObjectParameterized != 'undefined') {
return __super_methodWithHandlerJsonObjectParameterized.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param handler {function}
*/
this.methodWithHandlerJsonArrayParameterized = function(handler) {
var __args = arguments;
if (__args.length === 1 && typeof __args[0] === 'function') {
j_genericsTCK["methodWithHandlerJsonArrayParameterized(io.vertx.core.Handler)"](function(jVal) {
__args[0](utils.convReturnVertxGen(GenericRefedInterface, jVal, undefined));
});
} else if (typeof __super_methodWithHandlerJsonArrayParameterized != 'undefined') {
return __super_methodWithHandlerJsonArrayParameterized.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param handler {function}
*/
this.methodWithHandlerDataObjectParameterized = function(handler) {
var __args = arguments;
if (__args.length === 1 && typeof __args[0] === 'function') {
j_genericsTCK["methodWithHandlerDataObjectParameterized(io.vertx.core.Handler)"](function(jVal) {
__args[0](utils.convReturnVertxGen(GenericRefedInterface, jVal, undefined));
});
} else if (typeof __super_methodWithHandlerDataObjectParameterized != 'undefined') {
return __super_methodWithHandlerDataObjectParameterized.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param handler {function}
*/
this.methodWithHandlerEnumParameterized = function(handler) {
var __args = arguments;
if (__args.length === 1 && typeof __args[0] === 'function') {
j_genericsTCK["methodWithHandlerEnumParameterized(io.vertx.core.Handler)"](function(jVal) {
__args[0](utils.convReturnVertxGen(GenericRefedInterface, jVal, utils.enum_jtype(io.vertx.codegen.testmodel.TestEnum)));
});
} else if (typeof __super_methodWithHandlerEnumParameterized != 'undefined') {
return __super_methodWithHandlerEnumParameterized.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param handler {function}
*/
this.methodWithHandlerGenEnumParameterized = function(handler) {
var __args = arguments;
if (__args.length === 1 && typeof __args[0] === 'function') {
j_genericsTCK["methodWithHandlerGenEnumParameterized(io.vertx.core.Handler)"](function(jVal) {
__args[0](utils.convReturnVertxGen(GenericRefedInterface, jVal, utils.enum_jtype(io.vertx.codegen.testmodel.TestGenEnum)));
});
} else if (typeof __super_methodWithHandlerGenEnumParameterized != 'undefined') {
return __super_methodWithHandlerGenEnumParameterized.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param handler {function}
*/
this.methodWithHandlerUserTypeParameterized = function(handler) {
var __args = arguments;
if (__args.length === 1 && typeof __args[0] === 'function') {
j_genericsTCK["methodWithHandlerUserTypeParameterized(io.vertx.core.Handler)"](function(jVal) {
__args[0](utils.convReturnVertxGen(GenericRefedInterface, jVal, RefedInterface1._jtype));
});
} else if (typeof __super_methodWithHandlerUserTypeParameterized != 'undefined') {
return __super_methodWithHandlerUserTypeParameterized.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param handler {function}
*/
this.methodWithHandlerAsyncResultByteParameterized = function(handler) {
var __args = arguments;
if (__args.length === 1 && typeof __args[0] === 'function') {
j_genericsTCK["methodWithHandlerAsyncResultByteParameterized(io.vertx.core.Handler)"](function(ar) {
if (ar.succeeded()) {
__args[0](utils.convReturnVertxGen(GenericRefedInterface, ar.result(), undefined), null);
} else {
__args[0](null, ar.cause());
}
});
} else if (typeof __super_methodWithHandlerAsyncResultByteParameterized != 'undefined') {
return __super_methodWithHandlerAsyncResultByteParameterized.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param handler {function}
*/
this.methodWithHandlerAsyncResultShortParameterized = function(handler) {
var __args = arguments;
if (__args.length === 1 && typeof __args[0] === 'function') {
j_genericsTCK["methodWithHandlerAsyncResultShortParameterized(io.vertx.core.Handler)"](function(ar) {
if (ar.succeeded()) {
__args[0](utils.convReturnVertxGen(GenericRefedInterface, ar.result(), undefined), null);
} else {
__args[0](null, ar.cause());
}
});
} else if (typeof __super_methodWithHandlerAsyncResultShortParameterized != 'undefined') {
return __super_methodWithHandlerAsyncResultShortParameterized.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param handler {function}
*/
this.methodWithHandlerAsyncResultIntegerParameterized = function(handler) {
var __args = arguments;
if (__args.length === 1 && typeof __args[0] === 'function') {
j_genericsTCK["methodWithHandlerAsyncResultIntegerParameterized(io.vertx.core.Handler)"](function(ar) {
if (ar.succeeded()) {
__args[0](utils.convReturnVertxGen(GenericRefedInterface, ar.result(), undefined), null);
} else {
__args[0](null, ar.cause());
}
});
} else if (typeof __super_methodWithHandlerAsyncResultIntegerParameterized != 'undefined') {
return __super_methodWithHandlerAsyncResultIntegerParameterized.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param handler {function}
*/
this.methodWithHandlerAsyncResultLongParameterized = function(handler) {
var __args = arguments;
if (__args.length === 1 && typeof __args[0] === 'function') {
j_genericsTCK["methodWithHandlerAsyncResultLongParameterized(io.vertx.core.Handler)"](function(ar) {
if (ar.succeeded()) {
__args[0](utils.convReturnVertxGen(GenericRefedInterface, ar.result(), undefined), null);
} else {
__args[0](null, ar.cause());
}
});
} else if (typeof __super_methodWithHandlerAsyncResultLongParameterized != 'undefined') {
return __super_methodWithHandlerAsyncResultLongParameterized.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param handler {function}
*/
this.methodWithHandlerAsyncResultFloatParameterized = function(handler) {
var __args = arguments;
if (__args.length === 1 && typeof __args[0] === 'function') {
j_genericsTCK["methodWithHandlerAsyncResultFloatParameterized(io.vertx.core.Handler)"](function(ar) {
if (ar.succeeded()) {
__args[0](utils.convReturnVertxGen(GenericRefedInterface, ar.result(), undefined), null);
} else {
__args[0](null, ar.cause());
}
});
} else if (typeof __super_methodWithHandlerAsyncResultFloatParameterized != 'undefined') {
return __super_methodWithHandlerAsyncResultFloatParameterized.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param handler {function}
*/
this.methodWithHandlerAsyncResultDoubleParameterized = function(handler) {
var __args = arguments;
if (__args.length === 1 && typeof __args[0] === 'function') {
j_genericsTCK["methodWithHandlerAsyncResultDoubleParameterized(io.vertx.core.Handler)"](function(ar) {
if (ar.succeeded()) {
__args[0](utils.convReturnVertxGen(GenericRefedInterface, ar.result(), undefined), null);
} else {
__args[0](null, ar.cause());
}
});
} else if (typeof __super_methodWithHandlerAsyncResultDoubleParameterized != 'undefined') {
return __super_methodWithHandlerAsyncResultDoubleParameterized.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param handler {function}
*/
this.methodWithHandlerAsyncResultBooleanParameterized = function(handler) {
var __args = arguments;
if (__args.length === 1 && typeof __args[0] === 'function') {
j_genericsTCK["methodWithHandlerAsyncResultBooleanParameterized(io.vertx.core.Handler)"](function(ar) {
if (ar.succeeded()) {
__args[0](utils.convReturnVertxGen(GenericRefedInterface, ar.result(), undefined), null);
} else {
__args[0](null, ar.cause());
}
});
} else if (typeof __super_methodWithHandlerAsyncResultBooleanParameterized != 'undefined') {
return __super_methodWithHandlerAsyncResultBooleanParameterized.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param handler {function}
*/
this.methodWithHandlerAsyncResultCharacterParameterized = function(handler) {
var __args = arguments;
if (__args.length === 1 && typeof __args[0] === 'function') {
j_genericsTCK["methodWithHandlerAsyncResultCharacterParameterized(io.vertx.core.Handler)"](function(ar) {
if (ar.succeeded()) {
__args[0](utils.convReturnVertxGen(GenericRefedInterface, ar.result(), undefined), null);
} else {
__args[0](null, ar.cause());
}
});
} else if (typeof __super_methodWithHandlerAsyncResultCharacterParameterized != 'undefined') {
return __super_methodWithHandlerAsyncResultCharacterParameterized.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param handler {function}
*/
this.methodWithHandlerAsyncResultStringParameterized = function(handler) {
var __args = arguments;
if (__args.length === 1 && typeof __args[0] === 'function') {
j_genericsTCK["methodWithHandlerAsyncResultStringParameterized(io.vertx.core.Handler)"](function(ar) {
if (ar.succeeded()) {
__args[0](utils.convReturnVertxGen(GenericRefedInterface, ar.result(), undefined), null);
} else {
__args[0](null, ar.cause());
}
});
} else if (typeof __super_methodWithHandlerAsyncResultStringParameterized != 'undefined') {
return __super_methodWithHandlerAsyncResultStringParameterized.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param handler {function}
*/
this.methodWithHandlerAsyncResultJsonObjectParameterized = function(handler) {
var __args = arguments;
if (__args.length === 1 && typeof __args[0] === 'function') {
j_genericsTCK["methodWithHandlerAsyncResultJsonObjectParameterized(io.vertx.core.Handler)"](function(ar) {
if (ar.succeeded()) {
__args[0](utils.convReturnVertxGen(GenericRefedInterface, ar.result(), undefined), null);
} else {
__args[0](null, ar.cause());
}
});
} else if (typeof __super_methodWithHandlerAsyncResultJsonObjectParameterized != 'undefined') {
return __super_methodWithHandlerAsyncResultJsonObjectParameterized.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param handler {function}
*/
this.methodWithHandlerAsyncResultJsonArrayParameterized = function(handler) {
var __args = arguments;
if (__args.length === 1 && typeof __args[0] === 'function') {
j_genericsTCK["methodWithHandlerAsyncResultJsonArrayParameterized(io.vertx.core.Handler)"](function(ar) {
if (ar.succeeded()) {
__args[0](utils.convReturnVertxGen(GenericRefedInterface, ar.result(), undefined), null);
} else {
__args[0](null, ar.cause());
}
});
} else if (typeof __super_methodWithHandlerAsyncResultJsonArrayParameterized != 'undefined') {
return __super_methodWithHandlerAsyncResultJsonArrayParameterized.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param handler {function}
*/
this.methodWithHandlerAsyncResultDataObjectParameterized = function(handler) {
var __args = arguments;
if (__args.length === 1 && typeof __args[0] === 'function') {
j_genericsTCK["methodWithHandlerAsyncResultDataObjectParameterized(io.vertx.core.Handler)"](function(ar) {
if (ar.succeeded()) {
__args[0](utils.convReturnVertxGen(GenericRefedInterface, ar.result(), undefined), null);
} else {
__args[0](null, ar.cause());
}
});
} else if (typeof __super_methodWithHandlerAsyncResultDataObjectParameterized != 'undefined') {
return __super_methodWithHandlerAsyncResultDataObjectParameterized.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param handler {function}
*/
this.methodWithHandlerAsyncResultEnumParameterized = function(handler) {
var __args = arguments;
if (__args.length === 1 && typeof __args[0] === 'function') {
j_genericsTCK["methodWithHandlerAsyncResultEnumParameterized(io.vertx.core.Handler)"](function(ar) {
if (ar.succeeded()) {
__args[0](utils.convReturnVertxGen(GenericRefedInterface, ar.result(), utils.enum_jtype(io.vertx.codegen.testmodel.TestEnum)), null);
} else {
__args[0](null, ar.cause());
}
});
} else if (typeof __super_methodWithHandlerAsyncResultEnumParameterized != 'undefined') {
return __super_methodWithHandlerAsyncResultEnumParameterized.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param handler {function}
*/
this.methodWithHandlerAsyncResultGenEnumParameterized = function(handler) {
var __args = arguments;
if (__args.length === 1 && typeof __args[0] === 'function') {
j_genericsTCK["methodWithHandlerAsyncResultGenEnumParameterized(io.vertx.core.Handler)"](function(ar) {
if (ar.succeeded()) {
__args[0](utils.convReturnVertxGen(GenericRefedInterface, ar.result(), utils.enum_jtype(io.vertx.codegen.testmodel.TestGenEnum)), null);
} else {
__args[0](null, ar.cause());
}
});
} else if (typeof __super_methodWithHandlerAsyncResultGenEnumParameterized != 'undefined') {
return __super_methodWithHandlerAsyncResultGenEnumParameterized.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param handler {function}
*/
this.methodWithHandlerAsyncResultUserTypeParameterized = function(handler) {
var __args = arguments;
if (__args.length === 1 && typeof __args[0] === 'function') {
j_genericsTCK["methodWithHandlerAsyncResultUserTypeParameterized(io.vertx.core.Handler)"](function(ar) {
if (ar.succeeded()) {
__args[0](utils.convReturnVertxGen(GenericRefedInterface, ar.result(), RefedInterface1._jtype), null);
} else {
__args[0](null, ar.cause());
}
});
} else if (typeof __super_methodWithHandlerAsyncResultUserTypeParameterized != 'undefined') {
return __super_methodWithHandlerAsyncResultUserTypeParameterized.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param handler {function}
*/
this.methodWithFunctionParamByteParameterized = function(handler) {
var __args = arguments;
if (__args.length === 1 && typeof __args[0] === 'function') {
j_genericsTCK["methodWithFunctionParamByteParameterized(java.util.function.Function)"](function(jVal) {
var jRet = __args[0](utils.convReturnVertxGen(GenericRefedInterface, jVal, undefined));
return jRet;
});
} else if (typeof __super_methodWithFunctionParamByteParameterized != 'undefined') {
return __super_methodWithFunctionParamByteParameterized.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param handler {function}
*/
this.methodWithFunctionParamShortParameterized = function(handler) {
var __args = arguments;
if (__args.length === 1 && typeof __args[0] === 'function') {
j_genericsTCK["methodWithFunctionParamShortParameterized(java.util.function.Function)"](function(jVal) {
var jRet = __args[0](utils.convReturnVertxGen(GenericRefedInterface, jVal, undefined));
return jRet;
});
} else if (typeof __super_methodWithFunctionParamShortParameterized != 'undefined') {
return __super_methodWithFunctionParamShortParameterized.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param handler {function}
*/
this.methodWithFunctionParamIntegerParameterized = function(handler) {
var __args = arguments;
if (__args.length === 1 && typeof __args[0] === 'function') {
j_genericsTCK["methodWithFunctionParamIntegerParameterized(java.util.function.Function)"](function(jVal) {
var jRet = __args[0](utils.convReturnVertxGen(GenericRefedInterface, jVal, undefined));
return jRet;
});
} else if (typeof __super_methodWithFunctionParamIntegerParameterized != 'undefined') {
return __super_methodWithFunctionParamIntegerParameterized.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param handler {function}
*/
this.methodWithFunctionParamLongParameterized = function(handler) {
var __args = arguments;
if (__args.length === 1 && typeof __args[0] === 'function') {
j_genericsTCK["methodWithFunctionParamLongParameterized(java.util.function.Function)"](function(jVal) {
var jRet = __args[0](utils.convReturnVertxGen(GenericRefedInterface, jVal, undefined));
return jRet;
});
} else if (typeof __super_methodWithFunctionParamLongParameterized != 'undefined') {
return __super_methodWithFunctionParamLongParameterized.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param handler {function}
*/
this.methodWithFunctionParamFloatParameterized = function(handler) {
var __args = arguments;
if (__args.length === 1 && typeof __args[0] === 'function') {
j_genericsTCK["methodWithFunctionParamFloatParameterized(java.util.function.Function)"](function(jVal) {
var jRet = __args[0](utils.convReturnVertxGen(GenericRefedInterface, jVal, undefined));
return jRet;
});
} else if (typeof __super_methodWithFunctionParamFloatParameterized != 'undefined') {
return __super_methodWithFunctionParamFloatParameterized.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param handler {function}
*/
this.methodWithFunctionParamDoubleParameterized = function(handler) {
var __args = arguments;
if (__args.length === 1 && typeof __args[0] === 'function') {
j_genericsTCK["methodWithFunctionParamDoubleParameterized(java.util.function.Function)"](function(jVal) {
var jRet = __args[0](utils.convReturnVertxGen(GenericRefedInterface, jVal, undefined));
return jRet;
});
} else if (typeof __super_methodWithFunctionParamDoubleParameterized != 'undefined') {
return __super_methodWithFunctionParamDoubleParameterized.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param handler {function}
*/
this.methodWithFunctionParamBooleanParameterized = function(handler) {
var __args = arguments;
if (__args.length === 1 && typeof __args[0] === 'function') {
j_genericsTCK["methodWithFunctionParamBooleanParameterized(java.util.function.Function)"](function(jVal) {
var jRet = __args[0](utils.convReturnVertxGen(GenericRefedInterface, jVal, undefined));
return jRet;
});
} else if (typeof __super_methodWithFunctionParamBooleanParameterized != 'undefined') {
return __super_methodWithFunctionParamBooleanParameterized.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param handler {function}
*/
this.methodWithFunctionParamCharacterParameterized = function(handler) {
var __args = arguments;
if (__args.length === 1 && typeof __args[0] === 'function') {
j_genericsTCK["methodWithFunctionParamCharacterParameterized(java.util.function.Function)"](function(jVal) {
var jRet = __args[0](utils.convReturnVertxGen(GenericRefedInterface, jVal, undefined));
return jRet;
});
} else if (typeof __super_methodWithFunctionParamCharacterParameterized != 'undefined') {
return __super_methodWithFunctionParamCharacterParameterized.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param handler {function}
*/
this.methodWithFunctionParamStringParameterized = function(handler) {
var __args = arguments;
if (__args.length === 1 && typeof __args[0] === 'function') {
j_genericsTCK["methodWithFunctionParamStringParameterized(java.util.function.Function)"](function(jVal) {
var jRet = __args[0](utils.convReturnVertxGen(GenericRefedInterface, jVal, undefined));
return jRet;
});
} else if (typeof __super_methodWithFunctionParamStringParameterized != 'undefined') {
return __super_methodWithFunctionParamStringParameterized.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param handler {function}
*/
this.methodWithFunctionParamJsonObjectParameterized = function(handler) {
var __args = arguments;
if (__args.length === 1 && typeof __args[0] === 'function') {
j_genericsTCK["methodWithFunctionParamJsonObjectParameterized(java.util.function.Function)"](function(jVal) {
var jRet = __args[0](utils.convReturnVertxGen(GenericRefedInterface, jVal, undefined));
return jRet;
});
} else if (typeof __super_methodWithFunctionParamJsonObjectParameterized != 'undefined') {
return __super_methodWithFunctionParamJsonObjectParameterized.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param handler {function}
*/
this.methodWithFunctionParamJsonArrayParameterized = function(handler) {
var __args = arguments;
if (__args.length === 1 && typeof __args[0] === 'function') {
j_genericsTCK["methodWithFunctionParamJsonArrayParameterized(java.util.function.Function)"](function(jVal) {
var jRet = __args[0](utils.convReturnVertxGen(GenericRefedInterface, jVal, undefined));
return jRet;
});
} else if (typeof __super_methodWithFunctionParamJsonArrayParameterized != 'undefined') {
return __super_methodWithFunctionParamJsonArrayParameterized.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param handler {function}
*/
this.methodWithFunctionParamDataObjectParameterized = function(handler) {
var __args = arguments;
if (__args.length === 1 && typeof __args[0] === 'function') {
j_genericsTCK["methodWithFunctionParamDataObjectParameterized(java.util.function.Function)"](function(jVal) {
var jRet = __args[0](utils.convReturnVertxGen(GenericRefedInterface, jVal, undefined));
return jRet;
});
} else if (typeof __super_methodWithFunctionParamDataObjectParameterized != 'undefined') {
return __super_methodWithFunctionParamDataObjectParameterized.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param handler {function}
*/
this.methodWithFunctionParamEnumParameterized = function(handler) {
var __args = arguments;
if (__args.length === 1 && typeof __args[0] === 'function') {
j_genericsTCK["methodWithFunctionParamEnumParameterized(java.util.function.Function)"](function(jVal) {
var jRet = __args[0](utils.convReturnVertxGen(GenericRefedInterface, jVal, utils.enum_jtype(io.vertx.codegen.testmodel.TestEnum)));
return jRet;
});
} else if (typeof __super_methodWithFunctionParamEnumParameterized != 'undefined') {
return __super_methodWithFunctionParamEnumParameterized.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param handler {function}
*/
this.methodWithFunctionParamGenEnumParameterized = function(handler) {
var __args = arguments;
if (__args.length === 1 && typeof __args[0] === 'function') {
j_genericsTCK["methodWithFunctionParamGenEnumParameterized(java.util.function.Function)"](function(jVal) {
var jRet = __args[0](utils.convReturnVertxGen(GenericRefedInterface, jVal, utils.enum_jtype(io.vertx.codegen.testmodel.TestGenEnum)));
return jRet;
});
} else if (typeof __super_methodWithFunctionParamGenEnumParameterized != 'undefined') {
return __super_methodWithFunctionParamGenEnumParameterized.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param handler {function}
*/
this.methodWithFunctionParamUserTypeParameterized = function(handler) {
var __args = arguments;
if (__args.length === 1 && typeof __args[0] === 'function') {
j_genericsTCK["methodWithFunctionParamUserTypeParameterized(java.util.function.Function)"](function(jVal) {
var jRet = __args[0](utils.convReturnVertxGen(GenericRefedInterface, jVal, RefedInterface1._jtype));
return jRet;
});
} else if (typeof __super_methodWithFunctionParamUserTypeParameterized != 'undefined') {
return __super_methodWithFunctionParamUserTypeParameterized.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param type {todo}
@return {GenericRefedInterface}
*/
this.methodWithClassTypeParameterizedReturn = function(type) {
var __args = arguments;
if (__args.length === 1 && typeof __args[0] === 'function') {
return utils.convReturnVertxGen(GenericRefedInterface, j_genericsTCK["methodWithClassTypeParameterizedReturn(java.lang.Class)"](utils.get_jclass(__args[0])), utils.get_jtype(__args[0])) ;
} else if (typeof __super_methodWithClassTypeParameterizedReturn != 'undefined') {
return __super_methodWithClassTypeParameterizedReturn.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param type {todo}
@param handler {function}
*/
this.methodWithHandlerClassTypeParameterized = function(type, handler) {
var __args = arguments;
if (__args.length === 2 && typeof __args[0] === 'function' && typeof __args[1] === 'function') {
j_genericsTCK["methodWithHandlerClassTypeParameterized(java.lang.Class,io.vertx.core.Handler)"](utils.get_jclass(__args[0]), function(jVal) {
__args[1](utils.convReturnVertxGen(GenericRefedInterface, jVal, utils.get_jtype(__args[0])));
});
} else if (typeof __super_methodWithHandlerClassTypeParameterized != 'undefined') {
return __super_methodWithHandlerClassTypeParameterized.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param type {todo}
@param handler {function}
*/
this.methodWithHandlerAsyncResultClassTypeParameterized = function(type, handler) {
var __args = arguments;
if (__args.length === 2 && typeof __args[0] === 'function' && typeof __args[1] === 'function') {
j_genericsTCK["methodWithHandlerAsyncResultClassTypeParameterized(java.lang.Class,io.vertx.core.Handler)"](utils.get_jclass(__args[0]), function(ar) {
if (ar.succeeded()) {
__args[1](utils.convReturnVertxGen(GenericRefedInterface, ar.result(), utils.get_jtype(__args[0])), null);
} else {
__args[1](null, ar.cause());
}
});
} else if (typeof __super_methodWithHandlerAsyncResultClassTypeParameterized != 'undefined') {
return __super_methodWithHandlerAsyncResultClassTypeParameterized.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param type {todo}
@param handler {function}
*/
this.methodWithFunctionParamClassTypeParameterized = function(type, handler) {
var __args = arguments;
if (__args.length === 2 && typeof __args[0] === 'function' && typeof __args[1] === 'function') {
j_genericsTCK["methodWithFunctionParamClassTypeParameterized(java.lang.Class,java.util.function.Function)"](utils.get_jclass(__args[0]), function(jVal) {
var jRet = __args[1](utils.convReturnVertxGen(GenericRefedInterface, jVal, utils.get_jtype(__args[0])));
return jRet;
});
} else if (typeof __super_methodWithFunctionParamClassTypeParameterized != 'undefined') {
return __super_methodWithFunctionParamClassTypeParameterized.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param type {todo}
@param u {Object}
*/
this.methodWithClassTypeParam = function(type, u) {
var __args = arguments;
if (__args.length === 2 && typeof __args[0] === 'function' && typeof __args[1] !== 'function') {
j_genericsTCK["methodWithClassTypeParam(java.lang.Class,java.lang.Object)"](utils.get_jclass(__args[0]), utils.get_jtype(__args[0]).unwrap(__args[1]));
} else if (typeof __super_methodWithClassTypeParam != 'undefined') {
return __super_methodWithClassTypeParam.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param type {todo}
@return {Object}
*/
this.methodWithClassTypeReturn = function(type) {
var __args = arguments;
if (__args.length === 1 && typeof __args[0] === 'function') {
return utils.get_jtype(__args[0]).wrap(j_genericsTCK["methodWithClassTypeReturn(java.lang.Class)"](utils.get_jclass(__args[0]))) ;
} else if (typeof __super_methodWithClassTypeReturn != 'undefined') {
return __super_methodWithClassTypeReturn.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param type {todo}
@param f {function}
*/
this.methodWithClassTypeHandler = function(type, f) {
var __args = arguments;
if (__args.length === 2 && typeof __args[0] === 'function' && typeof __args[1] === 'function') {
j_genericsTCK["methodWithClassTypeHandler(java.lang.Class,io.vertx.core.Handler)"](utils.get_jclass(__args[0]), function(jVal) {
__args[1](utils.get_jtype(__args[0]).wrap(jVal));
});
} else if (typeof __super_methodWithClassTypeHandler != 'undefined') {
return __super_methodWithClassTypeHandler.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param type {todo}
@param f {function}
*/
this.methodWithClassTypeHandlerAsyncResult = function(type, f) {
var __args = arguments;
if (__args.length === 2 && typeof __args[0] === 'function' && typeof __args[1] === 'function') {
j_genericsTCK["methodWithClassTypeHandlerAsyncResult(java.lang.Class,io.vertx.core.Handler)"](utils.get_jclass(__args[0]), function(ar) {
if (ar.succeeded()) {
__args[1](utils.get_jtype(__args[0]).wrap(ar.result()), null);
} else {
__args[1](null, ar.cause());
}
});
} else if (typeof __super_methodWithClassTypeHandlerAsyncResult != 'undefined') {
return __super_methodWithClassTypeHandlerAsyncResult.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param type {todo}
@param f {function}
*/
this.methodWithClassTypeFunctionParam = function(type, f) {
var __args = arguments;
if (__args.length === 2 && typeof __args[0] === 'function' && typeof __args[1] === 'function') {
j_genericsTCK["methodWithClassTypeFunctionParam(java.lang.Class,java.util.function.Function)"](utils.get_jclass(__args[0]), function(jVal) {
var jRet = __args[1](utils.get_jtype(__args[0]).wrap(jVal));
return jRet;
});
} else if (typeof __super_methodWithClassTypeFunctionParam != 'undefined') {
return __super_methodWithClassTypeFunctionParam.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param type {todo}
@param f {function}
*/
this.methodWithClassTypeFunctionReturn = function(type, f) {
var __args = arguments;
if (__args.length === 2 && typeof __args[0] === 'function' && typeof __args[1] === 'function') {
j_genericsTCK["methodWithClassTypeFunctionReturn(java.lang.Class,java.util.function.Function)"](utils.get_jclass(__args[0]), function(jVal) {
var jRet = __args[1](jVal);
return utils.get_jtype(__args[0]).unwrap(jRet);
});
} else if (typeof __super_methodWithClassTypeFunctionReturn != 'undefined') {
return __super_methodWithClassTypeFunctionReturn.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param value {RefedInterface1}
@return {InterfaceWithApiArg}
*/
this.interfaceWithApiArg = function(value) {
var __args = arguments;
if (__args.length === 1 && typeof __args[0] === 'object' && __args[0]._jdel) {
return utils.convReturnVertxGen(InterfaceWithApiArg, j_genericsTCK["interfaceWithApiArg(io.vertx.codegen.testmodel.RefedInterface1)"](__args[0]._jdel)) ;
} else if (typeof __super_interfaceWithApiArg != 'undefined') {
return __super_interfaceWithApiArg.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param value {string}
@return {InterfaceWithStringArg}
*/
this.interfaceWithStringArg = function(value) {
var __args = arguments;
if (__args.length === 1 && typeof __args[0] === 'string') {
return utils.convReturnVertxGen(InterfaceWithStringArg, j_genericsTCK["interfaceWithStringArg(java.lang.String)"](__args[0])) ;
} else if (typeof __super_interfaceWithStringArg != 'undefined') {
return __super_interfaceWithStringArg.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param value1 {Object}
@param type {todo}
@param value2 {Object}
@return {InterfaceWithVariableArg}
*/
this.interfaceWithVariableArg = function(value1, type, value2) {
var __args = arguments;
if (__args.length === 3 && typeof __args[0] !== 'function' && typeof __args[1] === 'function' && typeof __args[2] !== 'function') {
return utils.convReturnVertxGen(InterfaceWithVariableArg, j_genericsTCK["interfaceWithVariableArg(java.lang.Object,java.lang.Class,java.lang.Object)"](utils.convParamTypeUnknown(__args[0]), utils.get_jclass(__args[1]), utils.get_jtype(__args[1]).unwrap(__args[2])), undefined, utils.get_jtype(__args[1])) ;
} else if (typeof __super_interfaceWithVariableArg != 'undefined') {
return __super_interfaceWithVariableArg.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param notNull {boolean}
@param handler {function}
*/
this.methodWithHandlerGenericNullableApi = function(notNull, handler) {
var __args = arguments;
if (__args.length === 2 && typeof __args[0] ==='boolean' && typeof __args[1] === 'function') {
j_genericsTCK["methodWithHandlerGenericNullableApi(boolean,io.vertx.core.Handler)"](__args[0], function(jVal) {
__args[1](utils.convReturnVertxGen(GenericNullableRefedInterface, jVal, RefedInterface1._jtype));
});
} else if (typeof __super_methodWithHandlerGenericNullableApi != 'undefined') {
return __super_methodWithHandlerGenericNullableApi.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param notNull {boolean}
@param handler {function}
*/
this.methodWithHandlerAsyncResultGenericNullableApi = function(notNull, handler) {
var __args = arguments;
if (__args.length === 2 && typeof __args[0] ==='boolean' && typeof __args[1] === 'function') {
j_genericsTCK["methodWithHandlerAsyncResultGenericNullableApi(boolean,io.vertx.core.Handler)"](__args[0], function(ar) {
if (ar.succeeded()) {
__args[1](utils.convReturnVertxGen(GenericNullableRefedInterface, ar.result(), RefedInterface1._jtype), null);
} else {
__args[1](null, ar.cause());
}
});
} else if (typeof __super_methodWithHandlerAsyncResultGenericNullableApi != 'undefined') {
return __super_methodWithHandlerAsyncResultGenericNullableApi.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param notNull {boolean}
@return {GenericNullableRefedInterface}
*/
this.methodWithGenericNullableApiReturn = function(notNull) {
var __args = arguments;
if (__args.length === 1 && typeof __args[0] ==='boolean') {
return utils.convReturnVertxGen(GenericNullableRefedInterface, j_genericsTCK["methodWithGenericNullableApiReturn(boolean)"](__args[0]), RefedInterface1._jtype) ;
} else if (typeof __super_methodWithGenericNullableApiReturn != 'undefined') {
return __super_methodWithGenericNullableApiReturn.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param param {GenericRefedInterface}
@return {GenericRefedInterface}
*/
this.methodWithParamInferedReturn = function(param) {
var __args = arguments;
if (__args.length === 1 && typeof __args[0] === 'object' && __args[0]._jdel) {
return utils.convReturnVertxGen(GenericRefedInterface, j_genericsTCK["methodWithParamInferedReturn(io.vertx.codegen.testmodel.GenericRefedInterface)"](__args[0]._jdel), undefined) ;
} else if (typeof __super_methodWithParamInferedReturn != 'undefined') {
return __super_methodWithParamInferedReturn.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param param {GenericRefedInterface}
@param handler {function}
*/
this.methodWithHandlerParamInfered = function(param, handler) {
var __args = arguments;
if (__args.length === 2 && typeof __args[0] === 'object' && __args[0]._jdel && typeof __args[1] === 'function') {
j_genericsTCK["methodWithHandlerParamInfered(io.vertx.codegen.testmodel.GenericRefedInterface,io.vertx.core.Handler)"](__args[0]._jdel, function(jVal) {
__args[1](utils.convReturnVertxGen(GenericRefedInterface, jVal, undefined));
});
} else if (typeof __super_methodWithHandlerParamInfered != 'undefined') {
return __super_methodWithHandlerParamInfered.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param param {GenericRefedInterface}
@param handler {function}
*/
this.methodWithHandlerAsyncResultParamInfered = function(param, handler) {
var __args = arguments;
if (__args.length === 2 && typeof __args[0] === 'object' && __args[0]._jdel && typeof __args[1] === 'function') {
j_genericsTCK["methodWithHandlerAsyncResultParamInfered(io.vertx.codegen.testmodel.GenericRefedInterface,io.vertx.core.Handler)"](__args[0]._jdel, function(ar) {
if (ar.succeeded()) {
__args[1](utils.convReturnVertxGen(GenericRefedInterface, ar.result(), undefined), null);
} else {
__args[1](null, ar.cause());
}
});
} else if (typeof __super_methodWithHandlerAsyncResultParamInfered != 'undefined') {
return __super_methodWithHandlerAsyncResultParamInfered.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
// A reference to the underlying Java delegate
// NOTE! This is an internal API and must not be used in user code.
// If you rely on this property your code is likely to break if we change it / remove it without warning.
this._jdel = j_genericsTCK;
};
GenericsTCK._jclass = utils.getJavaClass("io.vertx.codegen.testmodel.GenericsTCK");
GenericsTCK._jtype = {accept: function(obj) {
return GenericsTCK._jclass.isInstance(obj._jdel);
},wrap: function(jdel) {
var obj = Object.create(GenericsTCK.prototype, {});
GenericsTCK.apply(obj, arguments);
return obj;
},
unwrap: function(obj) {
return obj._jdel;
}
};
GenericsTCK._create = function(jdel) {var obj = Object.create(GenericsTCK.prototype, {});
GenericsTCK.apply(obj, arguments);
return obj;
}
module.exports = GenericsTCK;
© 2015 - 2024 Weber Informatics LLC | Privacy Policy