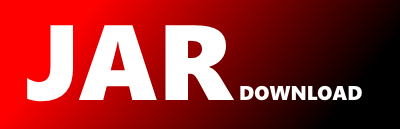
testmodel-js.test_interface.js Maven / Gradle / Ivy
/*
* Copyright 2014 Red Hat, Inc.
*
* Red Hat licenses this file to you under the Apache License, version 2.0
* (the "License"); you may not use this file except in compliance with the
* License. You may obtain a copy of the License at:
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations
* under the License.
*/
/** @module testmodel-js/test_interface */
var utils = require('vertx-js/util/utils');
var GenericRefedInterface = require('testmodel-js/generic_refed_interface');
var RefedInterface1 = require('testmodel-js/refed_interface1');
var AbstractHandlerUserType = require('testmodel-js/abstract_handler_user_type');
var ConcreteHandlerUserType = require('testmodel-js/concrete_handler_user_type');
var ConcreteHandlerUserTypeExtension = require('testmodel-js/concrete_handler_user_type_extension');
var SuperInterface1 = require('testmodel-js/super_interface1');
var RefedInterface2 = require('testmodel-js/refed_interface2');
var SuperInterface2 = require('testmodel-js/super_interface2');
var io = Packages.io;
var JsonObject = io.vertx.core.json.JsonObject;
var JTestInterface = Java.type('io.vertx.codegen.testmodel.TestInterface');
var TestDataObject = Java.type('io.vertx.codegen.testmodel.TestDataObject');
/**
@class
*/
var TestInterface = function(j_val) {
var j_testInterface = j_val;
var that = this;
SuperInterface1.call(this, j_val);
SuperInterface2.call(this, j_val);
var __super_otherSuperMethodWithBasicParams = this.otherSuperMethodWithBasicParams;
var __super_methodWithBasicParams = this.methodWithBasicParams;
var __super_methodWithBasicBoxedParams = this.methodWithBasicBoxedParams;
var __super_methodWithHandlerBasicTypes = this.methodWithHandlerBasicTypes;
var __super_methodWithHandlerStringReturn = this.methodWithHandlerStringReturn;
var __super_methodWithHandlerGenericReturn = this.methodWithHandlerGenericReturn;
var __super_methodWithHandlerVertxGenReturn = this.methodWithHandlerVertxGenReturn;
var __super_methodWithHandlerAsyncResultByte = this.methodWithHandlerAsyncResultByte;
var __super_methodWithHandlerAsyncResultShort = this.methodWithHandlerAsyncResultShort;
var __super_methodWithHandlerAsyncResultInteger = this.methodWithHandlerAsyncResultInteger;
var __super_methodWithHandlerAsyncResultLong = this.methodWithHandlerAsyncResultLong;
var __super_methodWithHandlerAsyncResultFloat = this.methodWithHandlerAsyncResultFloat;
var __super_methodWithHandlerAsyncResultDouble = this.methodWithHandlerAsyncResultDouble;
var __super_methodWithHandlerAsyncResultBoolean = this.methodWithHandlerAsyncResultBoolean;
var __super_methodWithHandlerAsyncResultCharacter = this.methodWithHandlerAsyncResultCharacter;
var __super_methodWithHandlerAsyncResultString = this.methodWithHandlerAsyncResultString;
var __super_methodWithHandlerAsyncResultDataObject = this.methodWithHandlerAsyncResultDataObject;
var __super_methodWithHandlerAsyncResultStringReturn = this.methodWithHandlerAsyncResultStringReturn;
var __super_methodWithHandlerAsyncResultGenericReturn = this.methodWithHandlerAsyncResultGenericReturn;
var __super_methodWithHandlerAsyncResultVertxGenReturn = this.methodWithHandlerAsyncResultVertxGenReturn;
var __super_methodWithUserTypes = this.methodWithUserTypes;
var __super_methodWithObjectParam = this.methodWithObjectParam;
var __super_methodWithDataObjectParam = this.methodWithDataObjectParam;
var __super_methodWithHandlerUserTypes = this.methodWithHandlerUserTypes;
var __super_methodWithHandlerAsyncResultUserTypes = this.methodWithHandlerAsyncResultUserTypes;
var __super_methodWithConcreteHandlerUserTypeSubtype = this.methodWithConcreteHandlerUserTypeSubtype;
var __super_methodWithAbstractHandlerUserTypeSubtype = this.methodWithAbstractHandlerUserTypeSubtype;
var __super_methodWithConcreteHandlerUserTypeSubtypeExtension = this.methodWithConcreteHandlerUserTypeSubtypeExtension;
var __super_methodWithHandlerVoid = this.methodWithHandlerVoid;
var __super_methodWithHandlerAsyncResultVoid = this.methodWithHandlerAsyncResultVoid;
var __super_methodWithHandlerThrowable = this.methodWithHandlerThrowable;
var __super_methodWithHandlerDataObject = this.methodWithHandlerDataObject;
var __super_methodWithHandlerGenericUserType = this.methodWithHandlerGenericUserType;
var __super_methodWithHandlerAsyncResultGenericUserType = this.methodWithHandlerAsyncResultGenericUserType;
var __super_methodWithByteReturn = this.methodWithByteReturn;
var __super_methodWithShortReturn = this.methodWithShortReturn;
var __super_methodWithIntReturn = this.methodWithIntReturn;
var __super_methodWithLongReturn = this.methodWithLongReturn;
var __super_methodWithFloatReturn = this.methodWithFloatReturn;
var __super_methodWithDoubleReturn = this.methodWithDoubleReturn;
var __super_methodWithBooleanReturn = this.methodWithBooleanReturn;
var __super_methodWithCharReturn = this.methodWithCharReturn;
var __super_methodWithStringReturn = this.methodWithStringReturn;
var __super_methodWithVertxGenReturn = this.methodWithVertxGenReturn;
var __super_methodWithVertxGenNullReturn = this.methodWithVertxGenNullReturn;
var __super_methodWithAbstractVertxGenReturn = this.methodWithAbstractVertxGenReturn;
var __super_methodWithDataObjectReturn = this.methodWithDataObjectReturn;
var __super_methodWithDataObjectNullReturn = this.methodWithDataObjectNullReturn;
var __super_methodWithGenericUserTypeReturn = this.methodWithGenericUserTypeReturn;
var __super_overloadedMethod = this.overloadedMethod;
var __super_overloadedMethod = this.overloadedMethod;
var __super_overloadedMethod = this.overloadedMethod;
var __super_overloadedMethod = this.overloadedMethod;
var __super_methodWithGenericReturn = this.methodWithGenericReturn;
var __super_methodWithGenericParam = this.methodWithGenericParam;
var __super_methodWithGenericHandler = this.methodWithGenericHandler;
var __super_methodWithGenericHandlerAsyncResult = this.methodWithGenericHandlerAsyncResult;
var __super_fluentMethod = this.fluentMethod;
var __super_staticFactoryMethod = this.staticFactoryMethod;
var __super_methodWithCachedReturn = this.methodWithCachedReturn;
var __super_methodWithCachedReturnPrimitive = this.methodWithCachedReturnPrimitive;
var __super_methodWithCachedListReturn = this.methodWithCachedListReturn;
var __super_methodWithJsonObjectReturn = this.methodWithJsonObjectReturn;
var __super_methodWithNullJsonObjectReturn = this.methodWithNullJsonObjectReturn;
var __super_methodWithComplexJsonObjectReturn = this.methodWithComplexJsonObjectReturn;
var __super_methodWithJsonArrayReturn = this.methodWithJsonArrayReturn;
var __super_methodWithNullJsonArrayReturn = this.methodWithNullJsonArrayReturn;
var __super_methodWithComplexJsonArrayReturn = this.methodWithComplexJsonArrayReturn;
var __super_methodWithJsonParams = this.methodWithJsonParams;
var __super_methodWithNullJsonParams = this.methodWithNullJsonParams;
var __super_methodWithHandlerJson = this.methodWithHandlerJson;
var __super_methodWithHandlerComplexJson = this.methodWithHandlerComplexJson;
var __super_methodWithHandlerAsyncResultJsonObject = this.methodWithHandlerAsyncResultJsonObject;
var __super_methodWithHandlerAsyncResultNullJsonObject = this.methodWithHandlerAsyncResultNullJsonObject;
var __super_methodWithHandlerAsyncResultComplexJsonObject = this.methodWithHandlerAsyncResultComplexJsonObject;
var __super_methodWithHandlerAsyncResultJsonArray = this.methodWithHandlerAsyncResultJsonArray;
var __super_methodWithHandlerAsyncResultNullJsonArray = this.methodWithHandlerAsyncResultNullJsonArray;
var __super_methodWithHandlerAsyncResultComplexJsonArray = this.methodWithHandlerAsyncResultComplexJsonArray;
var __super_methodWithEnumParam = this.methodWithEnumParam;
var __super_methodWithEnumReturn = this.methodWithEnumReturn;
var __super_methodWithGenEnumParam = this.methodWithGenEnumParam;
var __super_methodWithGenEnumReturn = this.methodWithGenEnumReturn;
var __super_methodWithThrowableReturn = this.methodWithThrowableReturn;
var __super_methodWithThrowableParam = this.methodWithThrowableParam;
var __super_superMethodOverloadedBySubclass = this.superMethodOverloadedBySubclass;
/**
@public
@param b {number}
@param s {number}
@param i {number}
@param l {number}
@param f {number}
@param d {number}
@param bool {boolean}
@param ch {string}
@param str {string}
*/
this.otherSuperMethodWithBasicParams = function(b, s, i, l, f, d, bool, ch, str) {
var __args = arguments;
if (__args.length === 9 && typeof __args[0] ==='number' && typeof __args[1] ==='number' && typeof __args[2] ==='number' && typeof __args[3] ==='number' && typeof __args[4] ==='number' && typeof __args[5] ==='number' && typeof __args[6] ==='boolean' && typeof __args[7] ==='string' && typeof __args[8] === 'string') {
j_testInterface["otherSuperMethodWithBasicParams(byte,short,int,long,float,double,boolean,char,java.lang.String)"](__args[0], __args[1], __args[2], __args[3], __args[4], __args[5], __args[6], __args[7], __args[8]);
} else if (typeof __super_otherSuperMethodWithBasicParams != 'undefined') {
return __super_otherSuperMethodWithBasicParams.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param b {number}
@param s {number}
@param i {number}
@param l {number}
@param f {number}
@param d {number}
@param bool {boolean}
@param ch {string}
@param str {string}
*/
this.methodWithBasicParams = function(b, s, i, l, f, d, bool, ch, str) {
var __args = arguments;
if (__args.length === 9 && typeof __args[0] ==='number' && typeof __args[1] ==='number' && typeof __args[2] ==='number' && typeof __args[3] ==='number' && typeof __args[4] ==='number' && typeof __args[5] ==='number' && typeof __args[6] ==='boolean' && typeof __args[7] ==='string' && typeof __args[8] === 'string') {
j_testInterface["methodWithBasicParams(byte,short,int,long,float,double,boolean,char,java.lang.String)"](__args[0], __args[1], __args[2], __args[3], __args[4], __args[5], __args[6], __args[7], __args[8]);
} else if (typeof __super_methodWithBasicParams != 'undefined') {
return __super_methodWithBasicParams.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param b {number}
@param s {number}
@param i {number}
@param l {number}
@param f {number}
@param d {number}
@param bool {boolean}
@param ch {string}
*/
this.methodWithBasicBoxedParams = function(b, s, i, l, f, d, bool, ch) {
var __args = arguments;
if (__args.length === 8 && typeof __args[0] ==='number' && typeof __args[1] ==='number' && typeof __args[2] ==='number' && typeof __args[3] ==='number' && typeof __args[4] ==='number' && typeof __args[5] ==='number' && typeof __args[6] ==='boolean' && typeof __args[7] ==='string') {
j_testInterface["methodWithBasicBoxedParams(java.lang.Byte,java.lang.Short,java.lang.Integer,java.lang.Long,java.lang.Float,java.lang.Double,java.lang.Boolean,java.lang.Character)"](utils.convParamByte(__args[0]), utils.convParamShort(__args[1]), utils.convParamInteger(__args[2]), utils.convParamLong(__args[3]), utils.convParamFloat(__args[4]), utils.convParamDouble(__args[5]), __args[6], utils.convParamCharacter(__args[7]));
} else if (typeof __super_methodWithBasicBoxedParams != 'undefined') {
return __super_methodWithBasicBoxedParams.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param byteHandler {function}
@param shortHandler {function}
@param intHandler {function}
@param longHandler {function}
@param floatHandler {function}
@param doubleHandler {function}
@param booleanHandler {function}
@param charHandler {function}
@param stringHandler {function}
*/
this.methodWithHandlerBasicTypes = function(byteHandler, shortHandler, intHandler, longHandler, floatHandler, doubleHandler, booleanHandler, charHandler, stringHandler) {
var __args = arguments;
if (__args.length === 9 && typeof __args[0] === 'function' && typeof __args[1] === 'function' && typeof __args[2] === 'function' && typeof __args[3] === 'function' && typeof __args[4] === 'function' && typeof __args[5] === 'function' && typeof __args[6] === 'function' && typeof __args[7] === 'function' && typeof __args[8] === 'function') {
j_testInterface["methodWithHandlerBasicTypes(io.vertx.core.Handler,io.vertx.core.Handler,io.vertx.core.Handler,io.vertx.core.Handler,io.vertx.core.Handler,io.vertx.core.Handler,io.vertx.core.Handler,io.vertx.core.Handler,io.vertx.core.Handler)"](function(jVal) {
__args[0](jVal);
}, function(jVal) {
__args[1](jVal);
}, function(jVal) {
__args[2](jVal);
}, function(jVal) {
__args[3](utils.convReturnLong(jVal));
}, function(jVal) {
__args[4](jVal);
}, function(jVal) {
__args[5](jVal);
}, function(jVal) {
__args[6](jVal);
}, function(jVal) {
__args[7](jVal);
}, function(jVal) {
__args[8](jVal);
});
} else if (typeof __super_methodWithHandlerBasicTypes != 'undefined') {
return __super_methodWithHandlerBasicTypes.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param expected {string}
@return {function}
*/
this.methodWithHandlerStringReturn = function(expected) {
var __args = arguments;
if (__args.length === 1 && typeof __args[0] === 'string') {
return utils.convReturnHandler(j_testInterface["methodWithHandlerStringReturn(java.lang.String)"](__args[0]), function(result) { return result; }) ;
} else if (typeof __super_methodWithHandlerStringReturn != 'undefined') {
return __super_methodWithHandlerStringReturn.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param handler {function}
@return {function}
*/
this.methodWithHandlerGenericReturn = function(handler) {
var __args = arguments;
if (__args.length === 1 && typeof __args[0] === 'function') {
return utils.convReturnHandler(j_testInterface["methodWithHandlerGenericReturn(io.vertx.core.Handler)"](function(jVal) {
__args[0](utils.convReturnTypeUnknown(jVal));
}), function(result) { return utils.convParamTypeUnknown(result); }) ;
} else if (typeof __super_methodWithHandlerGenericReturn != 'undefined') {
return __super_methodWithHandlerGenericReturn.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param expected {string}
@return {function}
*/
this.methodWithHandlerVertxGenReturn = function(expected) {
var __args = arguments;
if (__args.length === 1 && typeof __args[0] === 'string') {
return utils.convReturnHandler(j_testInterface["methodWithHandlerVertxGenReturn(java.lang.String)"](__args[0]), function(result) { return result._jdel; }) ;
} else if (typeof __super_methodWithHandlerVertxGenReturn != 'undefined') {
return __super_methodWithHandlerVertxGenReturn.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param sendFailure {boolean}
@param handler {function}
*/
this.methodWithHandlerAsyncResultByte = function(sendFailure, handler) {
var __args = arguments;
if (__args.length === 2 && typeof __args[0] ==='boolean' && typeof __args[1] === 'function') {
j_testInterface["methodWithHandlerAsyncResultByte(boolean,io.vertx.core.Handler)"](__args[0], function(ar) {
if (ar.succeeded()) {
__args[1](ar.result(), null);
} else {
__args[1](null, ar.cause());
}
});
} else if (typeof __super_methodWithHandlerAsyncResultByte != 'undefined') {
return __super_methodWithHandlerAsyncResultByte.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param sendFailure {boolean}
@param handler {function}
*/
this.methodWithHandlerAsyncResultShort = function(sendFailure, handler) {
var __args = arguments;
if (__args.length === 2 && typeof __args[0] ==='boolean' && typeof __args[1] === 'function') {
j_testInterface["methodWithHandlerAsyncResultShort(boolean,io.vertx.core.Handler)"](__args[0], function(ar) {
if (ar.succeeded()) {
__args[1](ar.result(), null);
} else {
__args[1](null, ar.cause());
}
});
} else if (typeof __super_methodWithHandlerAsyncResultShort != 'undefined') {
return __super_methodWithHandlerAsyncResultShort.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param sendFailure {boolean}
@param handler {function}
*/
this.methodWithHandlerAsyncResultInteger = function(sendFailure, handler) {
var __args = arguments;
if (__args.length === 2 && typeof __args[0] ==='boolean' && typeof __args[1] === 'function') {
j_testInterface["methodWithHandlerAsyncResultInteger(boolean,io.vertx.core.Handler)"](__args[0], function(ar) {
if (ar.succeeded()) {
__args[1](ar.result(), null);
} else {
__args[1](null, ar.cause());
}
});
} else if (typeof __super_methodWithHandlerAsyncResultInteger != 'undefined') {
return __super_methodWithHandlerAsyncResultInteger.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param sendFailure {boolean}
@param handler {function}
*/
this.methodWithHandlerAsyncResultLong = function(sendFailure, handler) {
var __args = arguments;
if (__args.length === 2 && typeof __args[0] ==='boolean' && typeof __args[1] === 'function') {
j_testInterface["methodWithHandlerAsyncResultLong(boolean,io.vertx.core.Handler)"](__args[0], function(ar) {
if (ar.succeeded()) {
__args[1](utils.convReturnLong(ar.result()), null);
} else {
__args[1](null, ar.cause());
}
});
} else if (typeof __super_methodWithHandlerAsyncResultLong != 'undefined') {
return __super_methodWithHandlerAsyncResultLong.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param sendFailure {boolean}
@param handler {function}
*/
this.methodWithHandlerAsyncResultFloat = function(sendFailure, handler) {
var __args = arguments;
if (__args.length === 2 && typeof __args[0] ==='boolean' && typeof __args[1] === 'function') {
j_testInterface["methodWithHandlerAsyncResultFloat(boolean,io.vertx.core.Handler)"](__args[0], function(ar) {
if (ar.succeeded()) {
__args[1](ar.result(), null);
} else {
__args[1](null, ar.cause());
}
});
} else if (typeof __super_methodWithHandlerAsyncResultFloat != 'undefined') {
return __super_methodWithHandlerAsyncResultFloat.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param sendFailure {boolean}
@param handler {function}
*/
this.methodWithHandlerAsyncResultDouble = function(sendFailure, handler) {
var __args = arguments;
if (__args.length === 2 && typeof __args[0] ==='boolean' && typeof __args[1] === 'function') {
j_testInterface["methodWithHandlerAsyncResultDouble(boolean,io.vertx.core.Handler)"](__args[0], function(ar) {
if (ar.succeeded()) {
__args[1](ar.result(), null);
} else {
__args[1](null, ar.cause());
}
});
} else if (typeof __super_methodWithHandlerAsyncResultDouble != 'undefined') {
return __super_methodWithHandlerAsyncResultDouble.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param sendFailure {boolean}
@param handler {function}
*/
this.methodWithHandlerAsyncResultBoolean = function(sendFailure, handler) {
var __args = arguments;
if (__args.length === 2 && typeof __args[0] ==='boolean' && typeof __args[1] === 'function') {
j_testInterface["methodWithHandlerAsyncResultBoolean(boolean,io.vertx.core.Handler)"](__args[0], function(ar) {
if (ar.succeeded()) {
__args[1](ar.result(), null);
} else {
__args[1](null, ar.cause());
}
});
} else if (typeof __super_methodWithHandlerAsyncResultBoolean != 'undefined') {
return __super_methodWithHandlerAsyncResultBoolean.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param sendFailure {boolean}
@param handler {function}
*/
this.methodWithHandlerAsyncResultCharacter = function(sendFailure, handler) {
var __args = arguments;
if (__args.length === 2 && typeof __args[0] ==='boolean' && typeof __args[1] === 'function') {
j_testInterface["methodWithHandlerAsyncResultCharacter(boolean,io.vertx.core.Handler)"](__args[0], function(ar) {
if (ar.succeeded()) {
__args[1](ar.result(), null);
} else {
__args[1](null, ar.cause());
}
});
} else if (typeof __super_methodWithHandlerAsyncResultCharacter != 'undefined') {
return __super_methodWithHandlerAsyncResultCharacter.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param sendFailure {boolean}
@param handler {function}
*/
this.methodWithHandlerAsyncResultString = function(sendFailure, handler) {
var __args = arguments;
if (__args.length === 2 && typeof __args[0] ==='boolean' && typeof __args[1] === 'function') {
j_testInterface["methodWithHandlerAsyncResultString(boolean,io.vertx.core.Handler)"](__args[0], function(ar) {
if (ar.succeeded()) {
__args[1](ar.result(), null);
} else {
__args[1](null, ar.cause());
}
});
} else if (typeof __super_methodWithHandlerAsyncResultString != 'undefined') {
return __super_methodWithHandlerAsyncResultString.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param sendFailure {boolean}
@param handler {function}
*/
this.methodWithHandlerAsyncResultDataObject = function(sendFailure, handler) {
var __args = arguments;
if (__args.length === 2 && typeof __args[0] ==='boolean' && typeof __args[1] === 'function') {
j_testInterface["methodWithHandlerAsyncResultDataObject(boolean,io.vertx.core.Handler)"](__args[0], function(ar) {
if (ar.succeeded()) {
__args[1](utils.convReturnDataObject(ar.result()), null);
} else {
__args[1](null, ar.cause());
}
});
} else if (typeof __super_methodWithHandlerAsyncResultDataObject != 'undefined') {
return __super_methodWithHandlerAsyncResultDataObject.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param expected {string}
@param fail {boolean}
@return {function}
*/
this.methodWithHandlerAsyncResultStringReturn = function(expected, fail) {
var __args = arguments;
if (__args.length === 2 && typeof __args[0] === 'string' && typeof __args[1] ==='boolean') {
return utils.convReturnHandlerAsyncResult(j_testInterface["methodWithHandlerAsyncResultStringReturn(java.lang.String,boolean)"](__args[0], __args[1]), function(result) { return result; }) ;
} else if (typeof __super_methodWithHandlerAsyncResultStringReturn != 'undefined') {
return __super_methodWithHandlerAsyncResultStringReturn.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param handler {function}
@return {function}
*/
this.methodWithHandlerAsyncResultGenericReturn = function(handler) {
var __args = arguments;
if (__args.length === 1 && typeof __args[0] === 'function') {
return utils.convReturnHandlerAsyncResult(j_testInterface["methodWithHandlerAsyncResultGenericReturn(io.vertx.core.Handler)"](function(ar) {
if (ar.succeeded()) {
__args[0](utils.convReturnTypeUnknown(ar.result()), null);
} else {
__args[0](null, ar.cause());
}
}), function(result) { return utils.convParamTypeUnknown(result); }) ;
} else if (typeof __super_methodWithHandlerAsyncResultGenericReturn != 'undefined') {
return __super_methodWithHandlerAsyncResultGenericReturn.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param expected {string}
@param fail {boolean}
@return {function}
*/
this.methodWithHandlerAsyncResultVertxGenReturn = function(expected, fail) {
var __args = arguments;
if (__args.length === 2 && typeof __args[0] === 'string' && typeof __args[1] ==='boolean') {
return utils.convReturnHandlerAsyncResult(j_testInterface["methodWithHandlerAsyncResultVertxGenReturn(java.lang.String,boolean)"](__args[0], __args[1]), function(result) { return result._jdel; }) ;
} else if (typeof __super_methodWithHandlerAsyncResultVertxGenReturn != 'undefined') {
return __super_methodWithHandlerAsyncResultVertxGenReturn.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param refed {RefedInterface1}
*/
this.methodWithUserTypes = function(refed) {
var __args = arguments;
if (__args.length === 1 && typeof __args[0] === 'object' && __args[0]._jdel) {
j_testInterface["methodWithUserTypes(io.vertx.codegen.testmodel.RefedInterface1)"](__args[0]._jdel);
} else if (typeof __super_methodWithUserTypes != 'undefined') {
return __super_methodWithUserTypes.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param str {string}
@param obj {Object}
*/
this.methodWithObjectParam = function(str, obj) {
var __args = arguments;
if (__args.length === 2 && typeof __args[0] === 'string' && typeof __args[1] !== 'function') {
j_testInterface["methodWithObjectParam(java.lang.String,java.lang.Object)"](__args[0], utils.convParamTypeUnknown(__args[1]));
} else if (typeof __super_methodWithObjectParam != 'undefined') {
return __super_methodWithObjectParam.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param dataObject {Object}
*/
this.methodWithDataObjectParam = function(dataObject) {
var __args = arguments;
if (__args.length === 1 && (typeof __args[0] === 'object' && __args[0] != null)) {
j_testInterface["methodWithDataObjectParam(io.vertx.codegen.testmodel.TestDataObject)"](__args[0] != null ? new TestDataObject(new JsonObject(Java.asJSONCompatible(__args[0]))) : null);
} else if (typeof __super_methodWithDataObjectParam != 'undefined') {
return __super_methodWithDataObjectParam.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param handler {function}
*/
this.methodWithHandlerUserTypes = function(handler) {
var __args = arguments;
if (__args.length === 1 && typeof __args[0] === 'function') {
j_testInterface["methodWithHandlerUserTypes(io.vertx.core.Handler)"](function(jVal) {
__args[0](utils.convReturnVertxGen(RefedInterface1, jVal));
});
} else if (typeof __super_methodWithHandlerUserTypes != 'undefined') {
return __super_methodWithHandlerUserTypes.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param handler {function}
*/
this.methodWithHandlerAsyncResultUserTypes = function(handler) {
var __args = arguments;
if (__args.length === 1 && typeof __args[0] === 'function') {
j_testInterface["methodWithHandlerAsyncResultUserTypes(io.vertx.core.Handler)"](function(ar) {
if (ar.succeeded()) {
__args[0](utils.convReturnVertxGen(RefedInterface1, ar.result()), null);
} else {
__args[0](null, ar.cause());
}
});
} else if (typeof __super_methodWithHandlerAsyncResultUserTypes != 'undefined') {
return __super_methodWithHandlerAsyncResultUserTypes.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param handler {ConcreteHandlerUserType}
*/
this.methodWithConcreteHandlerUserTypeSubtype = function(handler) {
var __args = arguments;
if (__args.length === 1 && typeof __args[0] === 'object' && __args[0]._jdel) {
j_testInterface["methodWithConcreteHandlerUserTypeSubtype(io.vertx.codegen.testmodel.ConcreteHandlerUserType)"](__args[0]._jdel);
} else if (typeof __super_methodWithConcreteHandlerUserTypeSubtype != 'undefined') {
return __super_methodWithConcreteHandlerUserTypeSubtype.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param handler {AbstractHandlerUserType}
*/
this.methodWithAbstractHandlerUserTypeSubtype = function(handler) {
var __args = arguments;
if (__args.length === 1 && typeof __args[0] === 'object' && __args[0]._jdel) {
j_testInterface["methodWithAbstractHandlerUserTypeSubtype(io.vertx.codegen.testmodel.AbstractHandlerUserType)"](__args[0]._jdel);
} else if (typeof __super_methodWithAbstractHandlerUserTypeSubtype != 'undefined') {
return __super_methodWithAbstractHandlerUserTypeSubtype.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param handler {ConcreteHandlerUserTypeExtension}
*/
this.methodWithConcreteHandlerUserTypeSubtypeExtension = function(handler) {
var __args = arguments;
if (__args.length === 1 && typeof __args[0] === 'object' && __args[0]._jdel) {
j_testInterface["methodWithConcreteHandlerUserTypeSubtypeExtension(io.vertx.codegen.testmodel.ConcreteHandlerUserTypeExtension)"](__args[0]._jdel);
} else if (typeof __super_methodWithConcreteHandlerUserTypeSubtypeExtension != 'undefined') {
return __super_methodWithConcreteHandlerUserTypeSubtypeExtension.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param handler {function}
*/
this.methodWithHandlerVoid = function(handler) {
var __args = arguments;
if (__args.length === 1 && typeof __args[0] === 'function') {
j_testInterface["methodWithHandlerVoid(io.vertx.core.Handler)"](__args[0]);
} else if (typeof __super_methodWithHandlerVoid != 'undefined') {
return __super_methodWithHandlerVoid.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param sendFailure {boolean}
@param handler {function}
*/
this.methodWithHandlerAsyncResultVoid = function(sendFailure, handler) {
var __args = arguments;
if (__args.length === 2 && typeof __args[0] ==='boolean' && typeof __args[1] === 'function') {
j_testInterface["methodWithHandlerAsyncResultVoid(boolean,io.vertx.core.Handler)"](__args[0], function(ar) {
if (ar.succeeded()) {
__args[1](null, null);
} else {
__args[1](null, ar.cause());
}
});
} else if (typeof __super_methodWithHandlerAsyncResultVoid != 'undefined') {
return __super_methodWithHandlerAsyncResultVoid.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param handler {function}
*/
this.methodWithHandlerThrowable = function(handler) {
var __args = arguments;
if (__args.length === 1 && typeof __args[0] === 'function') {
j_testInterface["methodWithHandlerThrowable(io.vertx.core.Handler)"](function(jVal) {
__args[0](utils.convReturnThrowable(jVal));
});
} else if (typeof __super_methodWithHandlerThrowable != 'undefined') {
return __super_methodWithHandlerThrowable.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param handler {function}
*/
this.methodWithHandlerDataObject = function(handler) {
var __args = arguments;
if (__args.length === 1 && typeof __args[0] === 'function') {
j_testInterface["methodWithHandlerDataObject(io.vertx.core.Handler)"](function(jVal) {
__args[0](utils.convReturnDataObject(jVal));
});
} else if (typeof __super_methodWithHandlerDataObject != 'undefined') {
return __super_methodWithHandlerDataObject.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param value {Object}
@param handler {function}
*/
this.methodWithHandlerGenericUserType = function(value, handler) {
var __args = arguments;
if (__args.length === 2 && typeof __args[0] !== 'function' && typeof __args[1] === 'function') {
j_testInterface["methodWithHandlerGenericUserType(java.lang.Object,io.vertx.core.Handler)"](utils.convParamTypeUnknown(__args[0]), function(jVal) {
__args[1](utils.convReturnVertxGen(GenericRefedInterface, jVal, undefined));
});
} else if (typeof __super_methodWithHandlerGenericUserType != 'undefined') {
return __super_methodWithHandlerGenericUserType.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param value {Object}
@param handler {function}
*/
this.methodWithHandlerAsyncResultGenericUserType = function(value, handler) {
var __args = arguments;
if (__args.length === 2 && typeof __args[0] !== 'function' && typeof __args[1] === 'function') {
j_testInterface["methodWithHandlerAsyncResultGenericUserType(java.lang.Object,io.vertx.core.Handler)"](utils.convParamTypeUnknown(__args[0]), function(ar) {
if (ar.succeeded()) {
__args[1](utils.convReturnVertxGen(GenericRefedInterface, ar.result(), undefined), null);
} else {
__args[1](null, ar.cause());
}
});
} else if (typeof __super_methodWithHandlerAsyncResultGenericUserType != 'undefined') {
return __super_methodWithHandlerAsyncResultGenericUserType.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@return {number}
*/
this.methodWithByteReturn = function() {
var __args = arguments;
if (__args.length === 0) {
return j_testInterface["methodWithByteReturn()"]() ;
} else if (typeof __super_methodWithByteReturn != 'undefined') {
return __super_methodWithByteReturn.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@return {number}
*/
this.methodWithShortReturn = function() {
var __args = arguments;
if (__args.length === 0) {
return j_testInterface["methodWithShortReturn()"]() ;
} else if (typeof __super_methodWithShortReturn != 'undefined') {
return __super_methodWithShortReturn.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@return {number}
*/
this.methodWithIntReturn = function() {
var __args = arguments;
if (__args.length === 0) {
return j_testInterface["methodWithIntReturn()"]() ;
} else if (typeof __super_methodWithIntReturn != 'undefined') {
return __super_methodWithIntReturn.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@return {number}
*/
this.methodWithLongReturn = function() {
var __args = arguments;
if (__args.length === 0) {
return j_testInterface["methodWithLongReturn()"]() ;
} else if (typeof __super_methodWithLongReturn != 'undefined') {
return __super_methodWithLongReturn.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@return {number}
*/
this.methodWithFloatReturn = function() {
var __args = arguments;
if (__args.length === 0) {
return j_testInterface["methodWithFloatReturn()"]() ;
} else if (typeof __super_methodWithFloatReturn != 'undefined') {
return __super_methodWithFloatReturn.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@return {number}
*/
this.methodWithDoubleReturn = function() {
var __args = arguments;
if (__args.length === 0) {
return j_testInterface["methodWithDoubleReturn()"]() ;
} else if (typeof __super_methodWithDoubleReturn != 'undefined') {
return __super_methodWithDoubleReturn.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@return {boolean}
*/
this.methodWithBooleanReturn = function() {
var __args = arguments;
if (__args.length === 0) {
return j_testInterface["methodWithBooleanReturn()"]() ;
} else if (typeof __super_methodWithBooleanReturn != 'undefined') {
return __super_methodWithBooleanReturn.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@return {string}
*/
this.methodWithCharReturn = function() {
var __args = arguments;
if (__args.length === 0) {
return j_testInterface["methodWithCharReturn()"]() ;
} else if (typeof __super_methodWithCharReturn != 'undefined') {
return __super_methodWithCharReturn.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@return {string}
*/
this.methodWithStringReturn = function() {
var __args = arguments;
if (__args.length === 0) {
return j_testInterface["methodWithStringReturn()"]() ;
} else if (typeof __super_methodWithStringReturn != 'undefined') {
return __super_methodWithStringReturn.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@return {RefedInterface1}
*/
this.methodWithVertxGenReturn = function() {
var __args = arguments;
if (__args.length === 0) {
return utils.convReturnVertxGen(RefedInterface1, j_testInterface["methodWithVertxGenReturn()"]()) ;
} else if (typeof __super_methodWithVertxGenReturn != 'undefined') {
return __super_methodWithVertxGenReturn.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@return {RefedInterface1}
*/
this.methodWithVertxGenNullReturn = function() {
var __args = arguments;
if (__args.length === 0) {
return utils.convReturnVertxGen(RefedInterface1, j_testInterface["methodWithVertxGenNullReturn()"]()) ;
} else if (typeof __super_methodWithVertxGenNullReturn != 'undefined') {
return __super_methodWithVertxGenNullReturn.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@return {RefedInterface2}
*/
this.methodWithAbstractVertxGenReturn = function() {
var __args = arguments;
if (__args.length === 0) {
return utils.convReturnVertxGen(RefedInterface2, j_testInterface["methodWithAbstractVertxGenReturn()"]()) ;
} else if (typeof __super_methodWithAbstractVertxGenReturn != 'undefined') {
return __super_methodWithAbstractVertxGenReturn.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@return {Object}
*/
this.methodWithDataObjectReturn = function() {
var __args = arguments;
if (__args.length === 0) {
return utils.convReturnDataObject(j_testInterface["methodWithDataObjectReturn()"]()) ;
} else if (typeof __super_methodWithDataObjectReturn != 'undefined') {
return __super_methodWithDataObjectReturn.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@return {Object}
*/
this.methodWithDataObjectNullReturn = function() {
var __args = arguments;
if (__args.length === 0) {
return utils.convReturnDataObject(j_testInterface["methodWithDataObjectNullReturn()"]()) ;
} else if (typeof __super_methodWithDataObjectNullReturn != 'undefined') {
return __super_methodWithDataObjectNullReturn.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param value {Object}
@return {GenericRefedInterface}
*/
this.methodWithGenericUserTypeReturn = function(value) {
var __args = arguments;
if (__args.length === 1 && typeof __args[0] !== 'function') {
return utils.convReturnVertxGen(GenericRefedInterface, j_testInterface["methodWithGenericUserTypeReturn(java.lang.Object)"](utils.convParamTypeUnknown(__args[0])), undefined) ;
} else if (typeof __super_methodWithGenericUserTypeReturn != 'undefined') {
return __super_methodWithGenericUserTypeReturn.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param str {string}
@param refed {RefedInterface1}
@param period {number}
@param handler {function}
@return {string}
*/
this.overloadedMethod = function() {
var __args = arguments;
if (__args.length === 2 && typeof __args[0] === 'string' && typeof __args[1] === 'function') {
return j_testInterface["overloadedMethod(java.lang.String,io.vertx.core.Handler)"](__args[0], function(jVal) {
__args[1](jVal);
}) ;
} else if (__args.length === 2 && typeof __args[0] === 'string' && typeof __args[1] === 'object' && __args[1]._jdel) {
return j_testInterface["overloadedMethod(java.lang.String,io.vertx.codegen.testmodel.RefedInterface1)"](__args[0], __args[1]._jdel) ;
} else if (__args.length === 3 && typeof __args[0] === 'string' && typeof __args[1] === 'object' && __args[1]._jdel && typeof __args[2] === 'function') {
return j_testInterface["overloadedMethod(java.lang.String,io.vertx.codegen.testmodel.RefedInterface1,io.vertx.core.Handler)"](__args[0], __args[1]._jdel, function(jVal) {
__args[2](jVal);
}) ;
} else if (__args.length === 4 && typeof __args[0] === 'string' && typeof __args[1] === 'object' && __args[1]._jdel && typeof __args[2] ==='number' && typeof __args[3] === 'function') {
return j_testInterface["overloadedMethod(java.lang.String,io.vertx.codegen.testmodel.RefedInterface1,long,io.vertx.core.Handler)"](__args[0], __args[1]._jdel, __args[2], function(jVal) {
__args[3](jVal);
}) ;
} else if (typeof __super_overloadedMethod != 'undefined') {
return __super_overloadedMethod.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param type {string}
@return {Object}
*/
this.methodWithGenericReturn = function(type) {
var __args = arguments;
if (__args.length === 1 && typeof __args[0] === 'string') {
return utils.convReturnTypeUnknown(j_testInterface["methodWithGenericReturn(java.lang.String)"](__args[0])) ;
} else if (typeof __super_methodWithGenericReturn != 'undefined') {
return __super_methodWithGenericReturn.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param type {string}
@param u {Object}
*/
this.methodWithGenericParam = function(type, u) {
var __args = arguments;
if (__args.length === 2 && typeof __args[0] === 'string' && typeof __args[1] !== 'function') {
j_testInterface["methodWithGenericParam(java.lang.String,java.lang.Object)"](__args[0], utils.convParamTypeUnknown(__args[1]));
} else if (typeof __super_methodWithGenericParam != 'undefined') {
return __super_methodWithGenericParam.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param type {string}
@param handler {function}
*/
this.methodWithGenericHandler = function(type, handler) {
var __args = arguments;
if (__args.length === 2 && typeof __args[0] === 'string' && typeof __args[1] === 'function') {
j_testInterface["methodWithGenericHandler(java.lang.String,io.vertx.core.Handler)"](__args[0], function(jVal) {
__args[1](utils.convReturnTypeUnknown(jVal));
});
} else if (typeof __super_methodWithGenericHandler != 'undefined') {
return __super_methodWithGenericHandler.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param type {string}
@param asyncResultHandler {function}
*/
this.methodWithGenericHandlerAsyncResult = function(type, asyncResultHandler) {
var __args = arguments;
if (__args.length === 2 && typeof __args[0] === 'string' && typeof __args[1] === 'function') {
j_testInterface["methodWithGenericHandlerAsyncResult(java.lang.String,io.vertx.core.Handler)"](__args[0], function(ar) {
if (ar.succeeded()) {
__args[1](utils.convReturnTypeUnknown(ar.result()), null);
} else {
__args[1](null, ar.cause());
}
});
} else if (typeof __super_methodWithGenericHandlerAsyncResult != 'undefined') {
return __super_methodWithGenericHandlerAsyncResult.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param str {string}
@return {TestInterface}
*/
this.fluentMethod = function(str) {
var __args = arguments;
if (__args.length === 1 && typeof __args[0] === 'string') {
j_testInterface["fluentMethod(java.lang.String)"](__args[0]) ;
return that;
} else if (typeof __super_fluentMethod != 'undefined') {
return __super_fluentMethod.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param foo {string}
@return {RefedInterface1}
*/
this.methodWithCachedReturn = function(foo) {
var __args = arguments;
if (__args.length === 1 && typeof __args[0] === 'string') {
if (that.cachedmethodWithCachedReturn == null) {
that.cachedmethodWithCachedReturn = utils.convReturnVertxGen(RefedInterface1, j_testInterface["methodWithCachedReturn(java.lang.String)"](__args[0]));
}
return that.cachedmethodWithCachedReturn;
} else if (typeof __super_methodWithCachedReturn != 'undefined') {
return __super_methodWithCachedReturn.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param arg {number}
@return {number}
*/
this.methodWithCachedReturnPrimitive = function(arg) {
var __args = arguments;
if (__args.length === 1 && typeof __args[0] ==='number') {
if (that.cachedmethodWithCachedReturnPrimitive == null) {
that.cachedmethodWithCachedReturnPrimitive = j_testInterface["methodWithCachedReturnPrimitive(int)"](__args[0]);
}
return that.cachedmethodWithCachedReturnPrimitive;
} else if (typeof __super_methodWithCachedReturnPrimitive != 'undefined') {
return __super_methodWithCachedReturnPrimitive.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@return {Array.}
*/
this.methodWithCachedListReturn = function() {
var __args = arguments;
if (__args.length === 0) {
if (that.cachedmethodWithCachedListReturn == null) {
that.cachedmethodWithCachedListReturn = utils.convReturnListSetVertxGen(j_testInterface["methodWithCachedListReturn()"](), RefedInterface1);
}
return that.cachedmethodWithCachedListReturn;
} else if (typeof __super_methodWithCachedListReturn != 'undefined') {
return __super_methodWithCachedListReturn.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@return {Object}
*/
this.methodWithJsonObjectReturn = function() {
var __args = arguments;
if (__args.length === 0) {
return utils.convReturnJson(j_testInterface["methodWithJsonObjectReturn()"]()) ;
} else if (typeof __super_methodWithJsonObjectReturn != 'undefined') {
return __super_methodWithJsonObjectReturn.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@return {Object}
*/
this.methodWithNullJsonObjectReturn = function() {
var __args = arguments;
if (__args.length === 0) {
return utils.convReturnJson(j_testInterface["methodWithNullJsonObjectReturn()"]()) ;
} else if (typeof __super_methodWithNullJsonObjectReturn != 'undefined') {
return __super_methodWithNullJsonObjectReturn.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@return {Object}
*/
this.methodWithComplexJsonObjectReturn = function() {
var __args = arguments;
if (__args.length === 0) {
return utils.convReturnJson(j_testInterface["methodWithComplexJsonObjectReturn()"]()) ;
} else if (typeof __super_methodWithComplexJsonObjectReturn != 'undefined') {
return __super_methodWithComplexJsonObjectReturn.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@return {Array}
*/
this.methodWithJsonArrayReturn = function() {
var __args = arguments;
if (__args.length === 0) {
return utils.convReturnJson(j_testInterface["methodWithJsonArrayReturn()"]()) ;
} else if (typeof __super_methodWithJsonArrayReturn != 'undefined') {
return __super_methodWithJsonArrayReturn.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@return {Array}
*/
this.methodWithNullJsonArrayReturn = function() {
var __args = arguments;
if (__args.length === 0) {
return utils.convReturnJson(j_testInterface["methodWithNullJsonArrayReturn()"]()) ;
} else if (typeof __super_methodWithNullJsonArrayReturn != 'undefined') {
return __super_methodWithNullJsonArrayReturn.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@return {Array}
*/
this.methodWithComplexJsonArrayReturn = function() {
var __args = arguments;
if (__args.length === 0) {
return utils.convReturnJson(j_testInterface["methodWithComplexJsonArrayReturn()"]()) ;
} else if (typeof __super_methodWithComplexJsonArrayReturn != 'undefined') {
return __super_methodWithComplexJsonArrayReturn.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param jsonObject {Object}
@param jsonArray {Array}
*/
this.methodWithJsonParams = function(jsonObject, jsonArray) {
var __args = arguments;
if (__args.length === 2 && (typeof __args[0] === 'object' && __args[0] != null) && typeof __args[1] === 'object' && __args[1] instanceof Array) {
j_testInterface["methodWithJsonParams(io.vertx.core.json.JsonObject,io.vertx.core.json.JsonArray)"](utils.convParamJsonObject(__args[0]), utils.convParamJsonArray(__args[1]));
} else if (typeof __super_methodWithJsonParams != 'undefined') {
return __super_methodWithJsonParams.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param jsonObject {Object}
@param jsonArray {Array}
*/
this.methodWithNullJsonParams = function(jsonObject, jsonArray) {
var __args = arguments;
if (__args.length === 2 && typeof __args[0] === 'object' && typeof __args[1] === 'object' && (__args[1] instanceof Array || __args[1] == null)) {
j_testInterface["methodWithNullJsonParams(io.vertx.core.json.JsonObject,io.vertx.core.json.JsonArray)"](utils.convParamJsonObject(__args[0]), utils.convParamJsonArray(__args[1]));
} else if (typeof __super_methodWithNullJsonParams != 'undefined') {
return __super_methodWithNullJsonParams.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param jsonObjectHandler {function}
@param jsonArrayHandler {function}
*/
this.methodWithHandlerJson = function(jsonObjectHandler, jsonArrayHandler) {
var __args = arguments;
if (__args.length === 2 && typeof __args[0] === 'function' && typeof __args[1] === 'function') {
j_testInterface["methodWithHandlerJson(io.vertx.core.Handler,io.vertx.core.Handler)"](function(jVal) {
__args[0](utils.convReturnJson(jVal));
}, function(jVal) {
__args[1](utils.convReturnJson(jVal));
});
} else if (typeof __super_methodWithHandlerJson != 'undefined') {
return __super_methodWithHandlerJson.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param jsonObjectHandler {function}
@param jsonArrayHandler {function}
*/
this.methodWithHandlerComplexJson = function(jsonObjectHandler, jsonArrayHandler) {
var __args = arguments;
if (__args.length === 2 && typeof __args[0] === 'function' && typeof __args[1] === 'function') {
j_testInterface["methodWithHandlerComplexJson(io.vertx.core.Handler,io.vertx.core.Handler)"](function(jVal) {
__args[0](utils.convReturnJson(jVal));
}, function(jVal) {
__args[1](utils.convReturnJson(jVal));
});
} else if (typeof __super_methodWithHandlerComplexJson != 'undefined') {
return __super_methodWithHandlerComplexJson.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param handler {function}
*/
this.methodWithHandlerAsyncResultJsonObject = function(handler) {
var __args = arguments;
if (__args.length === 1 && typeof __args[0] === 'function') {
j_testInterface["methodWithHandlerAsyncResultJsonObject(io.vertx.core.Handler)"](function(ar) {
if (ar.succeeded()) {
__args[0](utils.convReturnJson(ar.result()), null);
} else {
__args[0](null, ar.cause());
}
});
} else if (typeof __super_methodWithHandlerAsyncResultJsonObject != 'undefined') {
return __super_methodWithHandlerAsyncResultJsonObject.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param handler {function}
*/
this.methodWithHandlerAsyncResultNullJsonObject = function(handler) {
var __args = arguments;
if (__args.length === 1 && typeof __args[0] === 'function') {
j_testInterface["methodWithHandlerAsyncResultNullJsonObject(io.vertx.core.Handler)"](function(ar) {
if (ar.succeeded()) {
__args[0](utils.convReturnJson(ar.result()), null);
} else {
__args[0](null, ar.cause());
}
});
} else if (typeof __super_methodWithHandlerAsyncResultNullJsonObject != 'undefined') {
return __super_methodWithHandlerAsyncResultNullJsonObject.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param handler {function}
*/
this.methodWithHandlerAsyncResultComplexJsonObject = function(handler) {
var __args = arguments;
if (__args.length === 1 && typeof __args[0] === 'function') {
j_testInterface["methodWithHandlerAsyncResultComplexJsonObject(io.vertx.core.Handler)"](function(ar) {
if (ar.succeeded()) {
__args[0](utils.convReturnJson(ar.result()), null);
} else {
__args[0](null, ar.cause());
}
});
} else if (typeof __super_methodWithHandlerAsyncResultComplexJsonObject != 'undefined') {
return __super_methodWithHandlerAsyncResultComplexJsonObject.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param handler {function}
*/
this.methodWithHandlerAsyncResultJsonArray = function(handler) {
var __args = arguments;
if (__args.length === 1 && typeof __args[0] === 'function') {
j_testInterface["methodWithHandlerAsyncResultJsonArray(io.vertx.core.Handler)"](function(ar) {
if (ar.succeeded()) {
__args[0](utils.convReturnJson(ar.result()), null);
} else {
__args[0](null, ar.cause());
}
});
} else if (typeof __super_methodWithHandlerAsyncResultJsonArray != 'undefined') {
return __super_methodWithHandlerAsyncResultJsonArray.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param handler {function}
*/
this.methodWithHandlerAsyncResultNullJsonArray = function(handler) {
var __args = arguments;
if (__args.length === 1 && typeof __args[0] === 'function') {
j_testInterface["methodWithHandlerAsyncResultNullJsonArray(io.vertx.core.Handler)"](function(ar) {
if (ar.succeeded()) {
__args[0](utils.convReturnJson(ar.result()), null);
} else {
__args[0](null, ar.cause());
}
});
} else if (typeof __super_methodWithHandlerAsyncResultNullJsonArray != 'undefined') {
return __super_methodWithHandlerAsyncResultNullJsonArray.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param handler {function}
*/
this.methodWithHandlerAsyncResultComplexJsonArray = function(handler) {
var __args = arguments;
if (__args.length === 1 && typeof __args[0] === 'function') {
j_testInterface["methodWithHandlerAsyncResultComplexJsonArray(io.vertx.core.Handler)"](function(ar) {
if (ar.succeeded()) {
__args[0](utils.convReturnJson(ar.result()), null);
} else {
__args[0](null, ar.cause());
}
});
} else if (typeof __super_methodWithHandlerAsyncResultComplexJsonArray != 'undefined') {
return __super_methodWithHandlerAsyncResultComplexJsonArray.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param strVal {string}
@param weirdo {Object}
@return {string}
*/
this.methodWithEnumParam = function(strVal, weirdo) {
var __args = arguments;
if (__args.length === 2 && typeof __args[0] === 'string' && typeof __args[1] === 'string') {
return j_testInterface["methodWithEnumParam(java.lang.String,io.vertx.codegen.testmodel.TestEnum)"](__args[0], io.vertx.codegen.testmodel.TestEnum.valueOf(__args[1])) ;
} else if (typeof __super_methodWithEnumParam != 'undefined') {
return __super_methodWithEnumParam.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param strVal {string}
@return {Object}
*/
this.methodWithEnumReturn = function(strVal) {
var __args = arguments;
if (__args.length === 1 && typeof __args[0] === 'string') {
return utils.convReturnEnum(j_testInterface["methodWithEnumReturn(java.lang.String)"](__args[0])) ;
} else if (typeof __super_methodWithEnumReturn != 'undefined') {
return __super_methodWithEnumReturn.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param strVal {string}
@param weirdo {Object}
@return {string}
*/
this.methodWithGenEnumParam = function(strVal, weirdo) {
var __args = arguments;
if (__args.length === 2 && typeof __args[0] === 'string' && typeof __args[1] === 'string') {
return j_testInterface["methodWithGenEnumParam(java.lang.String,io.vertx.codegen.testmodel.TestGenEnum)"](__args[0], io.vertx.codegen.testmodel.TestGenEnum.valueOf(__args[1])) ;
} else if (typeof __super_methodWithGenEnumParam != 'undefined') {
return __super_methodWithGenEnumParam.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param strVal {string}
@return {Object}
*/
this.methodWithGenEnumReturn = function(strVal) {
var __args = arguments;
if (__args.length === 1 && typeof __args[0] === 'string') {
return utils.convReturnEnum(j_testInterface["methodWithGenEnumReturn(java.lang.String)"](__args[0])) ;
} else if (typeof __super_methodWithGenEnumReturn != 'undefined') {
return __super_methodWithGenEnumReturn.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param strVal {string}
@return {todo}
*/
this.methodWithThrowableReturn = function(strVal) {
var __args = arguments;
if (__args.length === 1 && typeof __args[0] === 'string') {
return utils.convReturnThrowable(j_testInterface["methodWithThrowableReturn(java.lang.String)"](__args[0])) ;
} else if (typeof __super_methodWithThrowableReturn != 'undefined') {
return __super_methodWithThrowableReturn.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param t {todo}
@return {string}
*/
this.methodWithThrowableParam = function(t) {
var __args = arguments;
if (__args.length === 1 && typeof __args[0] === 'object') {
return j_testInterface["methodWithThrowableParam(java.lang.Throwable)"](utils.convParamThrowable(__args[0])) ;
} else if (typeof __super_methodWithThrowableParam != 'undefined') {
return __super_methodWithThrowableParam.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
/**
@public
@param s {string}
@return {number}
*/
this.superMethodOverloadedBySubclass = function(s) {
var __args = arguments;
if (__args.length === 1 && typeof __args[0] === 'string') {
return j_testInterface["superMethodOverloadedBySubclass(java.lang.String)"](__args[0]) ;
} else if (typeof __super_superMethodOverloadedBySubclass != 'undefined') {
return __super_superMethodOverloadedBySubclass.apply(this, __args);
}
else throw new TypeError('function invoked with invalid arguments');
};
// A reference to the underlying Java delegate
// NOTE! This is an internal API and must not be used in user code.
// If you rely on this property your code is likely to break if we change it / remove it without warning.
this._jdel = j_testInterface;
};
TestInterface._jclass = utils.getJavaClass("io.vertx.codegen.testmodel.TestInterface");
TestInterface._jtype = {accept: function(obj) {
return TestInterface._jclass.isInstance(obj._jdel);
},wrap: function(jdel) {
var obj = Object.create(TestInterface.prototype, {});
TestInterface.apply(obj, arguments);
return obj;
},
unwrap: function(obj) {
return obj._jdel;
}
};
TestInterface._create = function(jdel) {var obj = Object.create(TestInterface.prototype, {});
TestInterface.apply(obj, arguments);
return obj;
}
/**
@memberof module:testmodel-js/test_interface
@param foo {string}
@return {RefedInterface1}
*/
TestInterface.staticFactoryMethod = function(foo) {
var __args = arguments;
if (__args.length === 1 && typeof __args[0] === 'string') {
return utils.convReturnVertxGen(RefedInterface1, JTestInterface["staticFactoryMethod(java.lang.String)"](__args[0])) ;
}else throw new TypeError('function invoked with invalid arguments');
};
module.exports = TestInterface;
© 2015 - 2024 Weber Informatics LLC | Privacy Policy