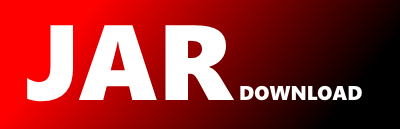
jangaroo-runtime.2.0.19.source-code.Arguments.as Maven / Gradle / Ivy
package {
/**
* An arguments object is used to store and access a function's arguments. Within a function's body, you can access its arguments object by using the local arguments variable.
* The arguments are stored as array elements: the first is accessed as arguments[0]
, the second as arguments[1]
, and so on. The arguments.length
property indicates the number of arguments passed to the function. There may be a different number of arguments passed than the function declares.
* Unlike previous versions of ActionScript, ActionScript 3.0 has no arguments.caller
property. To get a reference to the function that called the current function, you must pass a reference to that function as an argument. An example of this technique can be found in the example for arguments.callee
.
* ActionScript 3.0 includes a new ...(rest)
keyword that is recommended instead of the arguments class.
*
* @see http://help.adobe.com/en_US/FlashPlatform/reference/actionscript/3/statements.html#..._(rest)_parameter ...(rest)
* @see Function
*
*/
[Native]
public interface Arguments {
/**
* A reference to the currently executing function.
* @example The following code shows how to get a reference to the function that calls the function named secondFunction()
. The firstFunction()
function has the Boolean argument of true
to demonstrate that secondFunction()
successfully calls firstFunction()
and to prevent an infinite loop of each function calling the other.
* Because the callSecond
parameter is true
, firstFunction()
calls secondFunction()
and passes a reference to itself as the only argument. The function secondFunction()
receives this argument and stores it using a parameter named caller
, which is of data type Function. From within secondFunction()
, the caller
parameter is then used to call the firstFunction
function, but this time with the callSecond
argument set to false
.
* When execution returns to firstFunction()
, the trace()
statement is executed because callSecond
is false
.
*
* package {
* import flash.display.Sprite;
*
* public class ArgumentsExample extends Sprite {
* private var count:int = 1;
*
* public function ArgumentsExample() {
* firstFunction(true);
* }
*
* public function firstFunction(callSecond:Boolean) {
* trace(count + ": firstFunction");
* if(callSecond) {
* secondFunction(arguments.callee);
* }
* else {
* trace("CALLS STOPPED");
* }
* }
*
* public function secondFunction(caller:Function) {
* trace(count + ": secondFunction\n");
* count++;
* caller(false);
* }
* }
* }
*
*/
function get callee():Function;
/**
* The number of arguments passed to the function. This may be more or less than the function declares.
*/
function get length():uint;
}
}