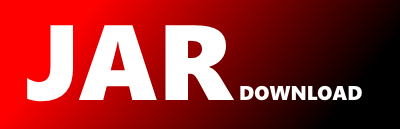
jangaroo-runtime.2.0.19.source-code.Boolean.as Maven / Gradle / Ivy
package {
/**
* A Boolean object is a data type that can have one of two values, either true
or false
, used for logical operations. Use the Boolean class to retrieve the primitive data type or string representation of a Boolean object.
* To create a Boolean object, you can use the constructor or the global function, or assign a literal value. It doesn't matter which technique you use; in ActionScript 3.0, all three techniques are equivalent. (This is different from JavaScript, where a Boolean object is distinct from the Boolean primitive type.)
* The following lines of code are equivalent:
*
* var flag:Boolean = true;
* var flag:Boolean = new Boolean(true);
* var flag:Boolean = Boolean(true);
*
*
* @see http://help.adobe.com/en_US/as3/learn/WS5b3ccc516d4fbf351e63e3d118a9b90204-7f9c.html Data types
* @see http://help.adobe.com/en_US/as3/learn/WS5b3ccc516d4fbf351e63e3d118a9b90204-7f88.html Data type descriptions
* @see http://help.adobe.com/en_US/as3/learn/WS5b3ccc516d4fbf351e63e3d118a9b90204-7f87.html Type conversions
*
*/
[Native]
public final class Boolean {
/**
* Creates a Boolean object with the specified value. If you omit the expression
parameter, the Boolean object is initialized with a value of false
. If you specify a value for the expression
parameter, the method evaluates it and returns the result as a Boolean value according to the rules in the global Boolean()
function.
* When called as a function (not as constructor), it converts the expression
parameter to a Boolean value and returns the value.
* Unlike previous versions of ActionScript, the Boolean()
function returns the same results as does the Boolean class constructor.
* The result depends on the data type and value of the argument, as described in the following table:
*
* Input Value Example Return Value
*
* 0
* Boolean(0)
* false
*
* NaN
* Boolean(NaN)
* false
*
* Number (not 0
or NaN
)
* Boolean(4)
* true
*
* Empty string
* Boolean("")
* false
*
* Non-empty string
* Boolean("6")
* true
*
* null
* Boolean(null)
* false
*
* undefined
* Boolean(undefined)
* false
*
* Instance of Object class
* Boolean(new Object())
* true
*
* No argument
* Boolean()
* false
*
* @param expression Any expression.
*
* @return A new Boolean or the result of the conversion to Boolean.
*
* @see http://help.adobe.com/en_US/as3/learn/WS5b3ccc516d4fbf351e63e3d118a9b90204-7f9c.html Data types
* @see http://help.adobe.com/en_US/as3/learn/WS5b3ccc516d4fbf351e63e3d118a9b90204-7f88.html Data type descriptions
* @see http://help.adobe.com/en_US/as3/learn/WS5b3ccc516d4fbf351e63e3d118a9b90204-7f87.html Type conversions
*
* @example The following code creates a new Boolean object, initialized to a value of false
called myBoolean
:
*
* var myBoolean:Boolean = new Boolean();
*
*/
public native function Boolean(expression:Object = false);
/**
* Returns the string representation ("true"
or "false"
) of the Boolean object. The output is not localized, and is "true"
or "false"
regardless of the system language.
* @return The string "true"
or "false"
.
*
* @example This example creates a variable of type Boolean and then uses the toString()
method to convert the value to a string for use in an array of strings:
*
* var myStringArray:Array = new Array("yes", "could be");
* var myBool:Boolean = 0;
* myBool.toString();
* myStringArray.push(myBool);
* trace(myStringArray); // yes,could be,false
*
*/
public native function toString():String;
/**
* Returns true
if the value of the specified Boolean object is true; false
otherwise.
* @return A Boolean value.
*
* @example The following example shows how this method works, and also shows that the value of a new Boolean object is false
:
*
* var myBool:Boolean = new Boolean();
* trace(myBool.valueOf()); // false
* myBool = (6==3+3);
* trace(myBool.valueOf()); // true
*
*/
public native function valueOf():Boolean;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy