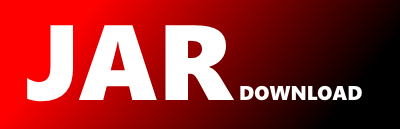
jangaroo-runtime.2.0.19.source-code.QName.as Maven / Gradle / Ivy
package {
/**
* QName objects represent qualified names of XML elements and attributes. Each QName object has a local name and a namespace Uniform Resource Identifier (URI). When the value of the namespace URI is null
, the QName object matches any namespace. Use the QName constructor to create a new QName object that is either a copy of another QName object or a new QName object with a uri
from a Namespace object and a localName
from a QName object.
* Methods specific to E4X can use QName objects interchangeably with strings. E4X methods are in the QName, Namespace, XML, and XMLList classes. These E4X methods, which take a string, can also take a QName object. This interchangeability is how namespace support works with, for example, the XML.child()
method.
* The QName class (along with the XML, XMLList, and Namespace classes) implements powerful XML-handling standards defined in ECMAScript for XML (E4X) specification (ECMA-357 edition 2).
* A qualified identifier evaluates to a QName object. If the QName object of an XML element is specified without identifying a namespace, the uri
property of the associated QName object is set to the global default namespace. If the QName object of an XML attribute is specified without identifying a namespace, the uri
property is set to an empty string.
*
* @see XML
* @see XMLList
* @see Namespace
* @see http://www.ecma-international.org/publications/standards/Ecma-357.htm ECMAScript for XML (E4X) specification (ECMA-357 edition 2)
*
*/
[Native]
public final class QName {
/**
* The local name of the QName object.
*/
public function get localName():String {
throw new Error('not implemented'); // TODO: implement!
}
/**
* The Uniform Resource Identifier (URI) of the QName object.
*/
public function get uri():String {
throw new Error('not implemented'); // TODO: implement!
}
/**
* a) Creates a QName object that is a copy of another QName object. If the parameter passed to the constructor is a QName object, a copy of the QName object is created. If the parameter is not a QName object, the parameter is converted to a string and assigned to the localName
property of the new QName instance. If the parameter is undefined
or unspecified, a new QName object is created with the localName
property set to the empty string.
* b) Creates a QName object with a URI object from a Namespace object and a localName
from a QName object. If either parameter is not the expected data type, the parameter is converted to a string and assigned to the corresponding property of the new QName object. For example, if both parameters are strings, a new QName object is returned with a uri
property set to the first parameter and a localName
property set to the second parameter. In other words, the following permutations, along with many others, are valid forms of the constructor:
*
* QName (uri:Namespace, localName:String);
* QName (uri:String, localName: QName);
* QName (uri:String, localName: String);
*
* If you pass null
for the qnameOrUri
parameter, the uri
property of the new QName object is set to null
.
* Note: This class shows two constructor entries because each form accepts different parameters. The constructor behaves differently depending on the type and number of parameters passed, as detailed in each entry. ActionSript 3.0 does not support method or constructor overloading.
* @param qnameOrUri a) The QName object to be copied. Objects of any other type are converted to a string that is assigned to the localName
property of the new QName object.
* b) A Namespace object from which to copy the uri
value. A parameter of any other type is converted to a string.
* @param localName A QName object from which to copy the localName
value. A parameter of any other type is converted to a string.
*
*/
public native function QName(qnameOrUri:Object/*QName or Namespace*/ = null, localName:Object/*String or QName*/ = null);
/**
* Returns a string composed of the URI, and the local name for the QName object, separated by "::".
* The format depends on the uri
property of the QName object:
* If uri
== ""
toString
returns localName
else if uri
== null
toString
returns *::localName
else
toString
returns uri
::localName
* @return The qualified name, as a string.
*
*/
public native function toString():String;
/**
* Returns the QName object.
* @return The primitive value of a QName instance.
*
*/
public native function valueOf():QName;
}
}