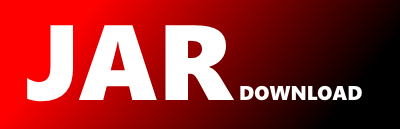
jangaroo-runtime.2.0.19.source-code.XML.as Maven / Gradle / Ivy
package {
/**
* The XML class contains methods and properties for working with XML objects. The XML class (along with the XMLList, Namespace, and QName classes) implements the powerful XML-handling standards defined in ECMAScript for XML (E4X) specification (ECMA-357 edition 2).
* Use the toXMLString()
method to return a string representation of the XML object regardless of whether the XML object has simple content or complex content.
* Note: The XML class (along with related classes) from ActionScript 2.0 has been renamed XMLDocument and moved into the flash.xml package. It is included in ActionScript 3.0 for backward compatibility.
*
* @see Namespace
* @see QName
* @see XMLList
* @see #toXMLString()
* @see http://www.ecma-international.org/publications/standards/Ecma-357.htm ECMAScript for XML (E4X) specification (ECMA-357 edition 2)
* @see http://help.adobe.com/en_US/as3/dev/WS5b3ccc516d4fbf351e63e3d118a9b90204-7e71.html XML objects
*
*/
[Native]
public final dynamic class XML {
/**
* Determines whether XML comments are ignored when XML objects parse the source XML data. By default, the comments are ignored (true
). To include XML comments, set this property to false
. The ignoreComments
property is used only during the XML parsing, not during the call to any method such as myXMLObject.child(*).toXMLString()
. If the source XML includes comment nodes, they are kept or discarded during the XML parsing.
* @see #child()
* @see #toXMLString()
*
* @example This example shows the effect of setting XML.ignoreComments
to false
and to true
:
*
* XML.ignoreComments = false;
* var xml1:XML =
* <foo>
* <!-- comment -->
* </foo>;
* trace(xml1.toXMLString()); // <foo><!-- comment --></foo>
*
* XML.ignoreComments = true;
* var xml2:XML =
* <foo>
* <!-- example -->
* </foo>;
* trace(xml2.toXMLString()); // <foo/>
*
*/
public static function get ignoreComments():Boolean {
throw new Error('not implemented'); // TODO: implement!
}
/**
* @private
*/
public static function set ignoreComments(value:Boolean):void {
throw new Error('not implemented'); // TODO: implement!
}
/**
* Determines whether XML processing instructions are ignored when XML objects parse the source XML data. By default, the processing instructions are ignored (true
). To include XML processing instructions, set this property to false
. The ignoreProcessingInstructions
property is used only during the XML parsing, not during the call to any method such as myXMLObject.child(*).toXMLString()
. If the source XML includes processing instructions nodes, they are kept or discarded during the XML parsing.
* @see #child()
* @see #toXMLString()
*
* @example This example shows the effect of setting XML.ignoreProcessingInstructions
to false
and to true
:
*
* XML.ignoreProcessingInstructions = false;
* var xml1:XML =
* <foo>
* <?exampleInstruction ?>
* </foo>;
* trace(xml1.toXMLString()); // <foo><?exampleInstruction ?></foo>
*
* XML.ignoreProcessingInstructions = true;
* var xml2:XML =
* <foo>
* <?exampleInstruction ?>
* </foo>;
* trace(xml2.toXMLString()); // <foo/>
*
*/
public static function get ignoreProcessingInstructions():Boolean {
throw new Error('not implemented'); // TODO: implement!
}
/**
* @private
*/
public static function set ignoreProcessingInstructions(value:Boolean):void {
throw new Error('not implemented'); // TODO: implement!
}
/**
* Determines whether white space characters at the beginning and end of text nodes are ignored during parsing. By default, white space is ignored (true
). If a text node is 100% white space and the ignoreWhitespace
property is set to true
, then the node is not created. To show white space in a text node, set the ignoreWhitespace
property to false
.
* When you create an XML object, it caches the current value of the ignoreWhitespace
property. Changing the ignoreWhitespace
does not change the behavior of existing XML objects.
* @example This example shows the effect of setting XML.ignoreWhitespace
to false
and to true
:
*
* XML.ignoreWhitespace = false;
* var xml1:XML = <foo> </foo>;
* trace(xml1.children().length()); // 1
*
* XML.ignoreWhitespace = true;
* var xml2:XML = <foo> </foo>;
* trace(xml2.children().length()); // 0
*
*/
public static function get ignoreWhitespace():Boolean {
throw new Error('not implemented'); // TODO: implement!
}
/**
* @private
*/
public static function set ignoreWhitespace(value:Boolean):void {
throw new Error('not implemented'); // TODO: implement!
}
/**
* Determines the amount of indentation applied by the toString()
and toXMLString()
methods when the XML.prettyPrinting
property is set to true
. Indentation is applied with the space character, not the tab character. The default value is 2
.
* @see #prettyPrinting
* @see #toString()
* @see #toXMLString()
*
* @example This example shows the effect of setting the XML.prettyIndent
static property:
*
* var xml:XML = <foo><bar/></foo>;
* XML.prettyIndent = 0;
* trace(xml.toXMLString());
*
* XML.prettyIndent = 1;
* trace(xml.toXMLString());
*
* XML.prettyIndent = 2;
* trace(xml.toXMLString());
*
*/
public static function get prettyIndent():int {
throw new Error('not implemented'); // TODO: implement!
}
/**
* @private
*/
public static function set prettyIndent(value:int):void {
throw new Error('not implemented'); // TODO: implement!
}
/**
* Determines whether the toString()
and toXMLString()
methods normalize white space characters between some tags. The default value is true
.
* @see #prettyIndent
* @see #toString()
* @see #toXMLString()
*
* @example This example shows the effect of setting XML.prettyPrinting
static property:
*
* var xml:XML = <foo><bar/></foo>;
* XML.prettyPrinting = false;
* trace(xml.toXMLString());
*
* XML.prettyPrinting = true;
* trace(xml.toXMLString());
*
*/
public static function get prettyPrinting():Boolean {
throw new Error('not implemented'); // TODO: implement!
}
/**
* @private
*/
public static function set prettyPrinting(value:Boolean):void {
throw new Error('not implemented'); // TODO: implement!
}
/**
* Creates a new XML object. You must use the constructor to create an XML object before you call any of the methods of the XML class.
* Use the toXMLString()
method to return a string representation of the XML object regardless of whether the XML object has simple content or complex content.
* @param value Any object that can be converted to XML with the top-level XML()
function.
*
* @see #XML()
* @see #toXMLString()
* @see http://help.adobe.com/en_US/as3/dev/WS5b3ccc516d4fbf351e63e3d118a9b90204-7e71.html XML objects
*
* @example The following example shows how you can load a remote XML document in ActionScript 3.0 using the URLLoader class in Flash Professional. Example provided by ActionScriptExamples.com.
*
* //
* // Requires:
* // - TextArea control UI component in the Flash Professional Library.
* //
* import fl.controls.TextArea;
*
* var xml:XML;
*
* var urlRequest:URLRequest = new URLRequest("http://www.helpexamples.com/flash/xml/menu.xml");
*
* var urlLoader:URLLoader = new URLLoader();
* urlLoader.addEventListener(Event.COMPLETE, urlLoader_complete);
* urlLoader.load(urlRequest);
*
* var textArea:TextArea = new TextArea();
* textArea.move(5, 5);
* textArea.setSize(stage.stageWidth - 10, stage.stageHeight - 10);
* addChild(textArea);
*
* function urlLoader_complete(evt:Event):void {
* xml = new XML(evt.currentTarget.data);
* textArea.text = xml.toXMLString();
* }
*
* Here's another variation using all ActionScript. Example provided by ActionScriptExamples.com.
*
* var xml:XML;
* var textArea:TextField = new TextField();
* textArea.width = 300;
*
* var urlRequest:URLRequest = new URLRequest("http://www.helpexamples.com/flash/xml/menu.xml");
* var urlLoader:URLLoader = new URLLoader();
* urlLoader.dataFormat = URLLoaderDataFormat.TEXT;
* urlLoader.addEventListener(Event.COMPLETE, urlLoader_complete);
* urlLoader.load(urlRequest);
*
* function urlLoader_complete(evt:Event):void {
* xml = new XML(evt.target.data);
* textArea.text = xml.toXMLString();
* addChild(textArea);
* }
*
*/
public function XML(value:Object) {
throw new Error('not implemented'); // TODO: implement!
}
/**
* Adds a namespace to the set of in-scope namespaces for the XML object. If the namespace already exists in the in-scope namespaces for the XML object (with a prefix matching that of the given parameter), then the prefix of the existing namespace is set to undefined
. If the input parameter is a Namespace object, it's used directly. If it's a QName object, the input parameter's URI is used to create a new namespace; otherwise, it's converted to a String and a namespace is created from the String.
* @param ns The namespace to add to the XML object.
*
* @return The new XML object, with the namespace added.
*
* @see http://help.adobe.com/en_US/as3/dev/WS5b3ccc516d4fbf351e63e3d118a9b90204-7e71.html XML objects
*
* @example This example uses a namespace defined in one XML object and applies it to another XML object:
*
* var xml1:XML = <ns:foo xmlns:ns="www.example.com/ns" />;
* var nsNamespace:Namespace = xml1.namespace();
*
* var xml2:XML = <bar />;
* xml2.addNamespace(nsNamespace);
* trace(xml2.toXMLString()); // <bar xmlns:ns="www.example.com/ns"/>
*
*/
public native function addNamespace(ns:Object):XML;
/**
* Appends the given child to the end of the XML object's properties. The appendChild()
method takes an XML object, an XMLList object, or any other data type that is then converted to a String.
* Use the delete
(XML) operator to remove XML nodes.
* @param child The XML object to append.
*
* @return The resulting XML object.
*
* @see http://help.adobe.com/en_US/FlashPlatform/reference/actionscript/3/operators.html#delete_(XML)
* @see http://help.adobe.com/en_US/as3/dev/WS5b3ccc516d4fbf351e63e3d118a9b90204-7e72.html The E4X approach to XML processing
* @see http://help.adobe.com/en_US/as3/dev/WS5b3ccc516d4fbf351e63e3d118a9b90204-7e71.html XML objects
* @see http://help.adobe.com/en_US/as3/dev/WS5b3ccc516d4fbf351e63e3d118a9b90204-7e68.html Assembling and transforming XML objects
*
* @example This example appends a new element to the end of the child list of an XML object:
*
* var xml:XML =
* <body>
* <p>hello</p>
* </body>;
*
* xml.appendChild(<p>world</p>);
* trace(xml.p[0].toXMLString()); // <p>hello</p>
* trace(xml.p[1].toXMLString()); // <p>world</p>
*
*/
public native function appendChild(child:Object):XML;
/**
* Returns the XML value of the attribute that has the name matching the attributeName
parameter. Attributes are found within XML elements. In the following example, the element has an attribute named "gender
" with the value "boy
": John
.
* The attributeName
parameter can be any data type; however, String is the most common data type to use. When passing any object other than a QName object, the attributeName
parameter uses the toString()
method to convert the parameter to a string.
* If you need a qualified name reference, you can pass in a QName object. A QName object defines a namespace and the local name, which you can use to define the qualified name of an attribute. Therefore calling attribute(qname)
is not the same as calling attribute(qname.toString())
.
* @param attributeName The name of the attribute.
*
* @return An XMLList object or an empty XMLList object. Returns an empty XMLList object when an attribute value has not been defined.
*
* @see #attributes()
* @see QName
* @see Namespace
* @see #elements()
* @see http://help.adobe.com/en_US/FlashPlatform/reference/actionscript/3/operators.html#attribute_identifier
* @see http://help.adobe.com/en_US/as3/dev/WS5b3ccc516d4fbf351e63e3d118a9b90204-7e71.html XML objects
* @see http://help.adobe.com/en_US/as3/dev/WS5b3ccc516d4fbf351e63e3d118a9b90204-7e6b.html Traversing XML structures
*
* @example This example shows a QName object passed into the attribute()
method. The localName
property is attr
and the namespace
property is ns
.
*
* var xml:XML = <ns:node xmlns:ns = "http://uri" ns:attr = '7' />
* var qn:QName = new QName("http://uri", "attr");
* trace (xml.attribute(qn)); // 7
*
* To return an attribute with a name that matches an ActionScript reserved word, use the attribute()
method instead of the attribute identifier (@) operator, as in the following example:
*
* var xml:XML = <example class="first" />
* trace(xml.attribute("class"));
*
*
*/
public native function attribute(attributeName:*):XMLList;
/**
* Returns a list of attribute values for the given XML object. Use the name()
method with the attributes()
method to return the name of an attribute. Use of xml.attributes()
is equivalent to xml.@*
.
* @return The list of attribute values.
*
* @see #attribute()
* @see #name()
* @see http://help.adobe.com/en_US/FlashPlatform/reference/actionscript/3/operators.html#attribute_identifier
* @see http://help.adobe.com/en_US/as3/dev/WS5b3ccc516d4fbf351e63e3d118a9b90204-7e71.html XML objects
* @see http://help.adobe.com/en_US/as3/dev/WS5b3ccc516d4fbf351e63e3d118a9b90204-7e6b.html Traversing XML structures
*
* @example The following example returns the name of the attribute:
*
* var xml:XML=<example id='123' color='blue'/>
* trace(xml.attributes()[1].name()); //color
*
* This example returns the names of all the attributes:
*
* var xml:XML = <example id='123' color='blue'/>
* var attNamesList:XMLList = xml.@*;
*
* trace (attNamesList is XMLList); // true
* trace (attNamesList.length()); // 2
*
* for (var i:int = 0; i < attNamesList.length(); i++)
* {
* trace (typeof (attNamesList[i])); // xml
* trace (attNamesList[i].nodeKind()); // attribute
* trace (attNamesList[i].name()); // id and color
* }
*
*
*/
public native function attributes():XMLList;
/**
* Lists the children of an XML object. An XML child is an XML element, text node, comment, or processing instruction.
* Use the propertyName
parameter to list the contents of a specific XML child. For example, to return the contents of a child named
, use child.name("first")
. You can generate the same result by using the child's index number. The index number identifies the child's position in the list of other XML children. For example, name.child(0)
returns the first child in a list.
* Use an asterisk (*) to output all the children in an XML document. For example, doc.child("*")
.
* Use the length()
method with the asterisk (*) parameter of the child()
method to output the total number of children. For example, numChildren = doc.child("*").length()
.
* @param propertyName The element name or integer of the XML child.
*
* @return An XMLList object of child nodes that match the input parameter.
*
* @see #elements()
* @see XMLList
* @see #length()
* @see http://help.adobe.com/en_US/as3/dev/WS5b3ccc516d4fbf351e63e3d118a9b90204-7e71.html XML objects
* @see http://help.adobe.com/en_US/as3/dev/WS5b3ccc516d4fbf351e63e3d118a9b90204-7e6b.html Traversing XML structures
*
* @example This example shows the use of the child()
method to identify child elements with a specified name:
*
* var xml:XML =
* <foo>
* <bar>text1</bar>
* <bar>text2</bar>
* </foo>;
* trace(xml.child("bar").length()); // 2
* trace(xml.child("bar")[0].toXMLString()); // <bar>text1</bar>
* trace(xml.child("bar")[1].toXMLString()); // <bar>text2</bar>
*
*/
public native function child(propertyName:Object):XMLList;
/**
* Identifies the zero-indexed position of this XML object within the context of its parent.
* @return The position of the object. Returns -1 as well as positive integers.
*
* @see http://help.adobe.com/en_US/as3/dev/WS5b3ccc516d4fbf351e63e3d118a9b90204-7e71.html XML objects
*
* @example This example shows the use of the childIndex()
method:
*
* var xml:XML =
* <foo>
* <bar />
* text
* <bob />
* </foo>;
* trace(xml.bar.childIndex()); // 0
* trace(xml.bob.childIndex()); // 2
*
*/
public native function childIndex():int;
/**
* Lists the children of the XML object in the sequence in which they appear. An XML child is an XML element, text node, comment, or processing instruction.
* @return An XMLList object of the XML object's children.
*
* @see http://help.adobe.com/en_US/as3/dev/WS5b3ccc516d4fbf351e63e3d118a9b90204-7e71.html XML objects
*
* @example This example shows the use of the children()
method:
*
* XML.ignoreComments = false;
* XML.ignoreProcessingInstructions = false;
* var xml:XML =
* <foo id="22">
* <bar>44</bar>
* text
* <!-- comment -->
* <?instruction ?>
* </foo>;
* trace(xml.children().length()); // 4
* trace(xml.children()[0].toXMLString()); // <bar>44</bar>
* trace(xml.children()[1].toXMLString()); // text
* trace(xml.children()[2].toXMLString()); // <!-- comment -->
* trace(xml.children()[3].toXMLString()); // <?instruction ?>
*
*/
public native function children():XMLList;
/**
* Lists the properties of the XML object that contain XML comments.
* @return An XMLList object of the properties that contain comments.
*
* @see http://help.adobe.com/en_US/as3/dev/WS5b3ccc516d4fbf351e63e3d118a9b90204-7e71.html XML objects
*
* @example This example shows the use of the comments()
method:
*
* XML.ignoreComments = false;
* var xml:XML =
* <foo>
* <!-- example -->
* <!-- example2 -->
* </foo>;
* trace(xml.comments().length()); // 2
* trace(xml.comments()[1].toXMLString()); // <!-- example2 -->
*
*/
public native function comments():XMLList;
/**
* Compares the XML object against the given value
parameter.
* @param value A value to compare against the current XML object.
*
* @return If the XML object matches the value
parameter, then true
; otherwise false
.
*
* @see http://help.adobe.com/en_US/as3/dev/WS5b3ccc516d4fbf351e63e3d118a9b90204-7e71.html XML objects
*
* @example This example shows the use of the contains()
method:
*
* var xml:XML =
* <order>
* <item>Rice</item>
* <item>Kung Pao Shrimp</item>
* </order>;
* trace(xml.item[0].contains(<item>Rice</item>)); // true
* trace(xml.item[1].contains(<item>Kung Pao Shrimp</item>)); // true
* trace(xml.item[1].contains(<item>MSG</item>)); // false
*
*/
public native function contains(value:XML):Boolean;
/**
* Returns a copy of the given XML object. The copy is a duplicate of the entire tree of nodes. The copied XML object has no parent and returns null
if you attempt to call the parent()
method.
* @return The copy of the object.
*
* @see http://help.adobe.com/en_US/as3/dev/WS5b3ccc516d4fbf351e63e3d118a9b90204-7e71.html XML objects
*
* @example This example shows that the copy()
method creates a new instance of an XML object. When you modify the copy, the original remains unchanged:
*
* var xml1:XML = <foo />;
* var xml2:XML = xml1.copy();
* xml2.appendChild(<bar />);
* trace(xml1.bar.length()); // 0
* trace(xml2.bar.length()); // 1
*
*/
public native function copy():XML;
/**
* Returns an object with the following properties set to the default values: ignoreComments
, ignoreProcessingInstructions
, ignoreWhitespace
, prettyIndent
, and prettyPrinting
. The default values are as follows:
*
* ignoreComments = true
* ignoreProcessingInstructions = true
* ignoreWhitespace = true
* prettyIndent = 2
* prettyPrinting = true
* Note: You do not apply this method to an instance of the XML class; you apply it to XML
, as in the following code: var df:Object = XML.defaultSettings()
.
* @return An object with properties set to the default settings.
*
* @see #ignoreComments
* @see #ignoreProcessingInstructions
* @see #ignoreWhitespace
* @see #prettyIndent
* @see #prettyPrinting
* @see #setSettings()
* @see #settings()
* @see http://help.adobe.com/en_US/as3/dev/WS5b3ccc516d4fbf351e63e3d118a9b90204-7e71.html XML objects
*
* @example The following example shows: how to apply some custom settings (for including comments and processing instructions) prior to setting an XML object; how to then revert back to the default settings before setting another XML object; and then how to set the custom settings again (for setting any more XML objects):
*
* XML.ignoreComments = false;
* XML.ignoreProcessingInstructions = false;
* var customSettings:Object = XML.settings();
*
* var xml1:XML =
* <foo>
* <!-- comment -->
* <?instruction ?>
* </foo>;
* trace(xml1.toXMLString());
* // <foo>
* // <!-- comment -->
* // <?instruction ?>
* // </foo>
*
* XML.setSettings(XML.defaultSettings());
* var xml2:XML =
* <foo>
* <!-- comment -->
* <?instruction ?>
* </foo>;
* trace(xml2.toXMLString());
*
*/
public native static function defaultSettings():Object;
/**
* Returns all descendants (children, grandchildren, great-grandchildren, and so on) of the XML object that have the given name
parameter. The name
parameter is optional. The name
parameter can be a QName object, a String data type or any other data type that is then converted to a String data type.
* To return all descendants, use the "*" parameter. If no parameter is passed, the string "*" is passed and returns all descendants of the XML object.
* @param name The name of the element to match.
*
* @return An XMLList object of matching descendants. If there are no descendants, returns an empty XMLList object.
*
* @see http://help.adobe.com/en_US/FlashPlatform/reference/actionscript/3/operators.html#descendant_accessor
*
* @example To return descendants with names that match ActionScript reserved words, use the descendants()
method instead of the descendant (..) operator, as in the following example:
*
* var xml:XML =
* <enrollees>
* <student id="239">
* <class name="Algebra" />
* <class name="Spanish 2"/>
* </student>
* <student id="206">
* <class name="Trigonometry" />
* <class name="Spanish 2" />
* </student>
* </enrollees>
* trace(xml.descendants("class"));
*
* The following example shows that the descendants()
method returns an XMLList object that contains all descendant objects, including children, grandchildren, and so on:
*
* XML.ignoreComments = false;
* var xml:XML =
* <body>
* <!-- comment -->
* text1
* <a>
* <b>text2</b>
* </a>
* </body>;
* trace(xml.descendants("*").length()); // 5
* trace(xml.descendants("*")[0]); // // <!-- comment -->
* trace(xml.descendants("*")[1].toXMLString()); // text1
* trace(xml.descendants("a").toXMLString()); // <a><b>text2</b></a>
* trace(xml.descendants("b").toXMLString()); // <b>text2</b>
*
*/
public native function descendants(name:Object = "*"):XMLList;
/**
* Lists the elements of an XML object. An element consists of a start and an end tag; for example
. The name
parameter is optional. The name
parameter can be a QName object, a String data type, or any other data type that is then converted to a String data type. Use the name
parameter to list a specific element. For example, the element "first
" returns "John
" in this example: John
.
* To list all elements, use the asterisk (*) as the parameter. The asterisk is also the default parameter.
* Use the length()
method with the asterisk parameter to output the total number of elements. For example, numElement = addressbook.elements("*").length()
.
* @param name The name of the element. An element's name is surrounded by angle brackets. For example, "first
" is the name
in this example:
.
*
* @return An XMLList object of the element's content. The element's content falls between the start and end tags. If you use the asterisk (*) to call all elements, both the element's tags and content are returned.
*
* @see #child()
* @see XMLList
* @see #length()
* @see #attribute()
* @see http://help.adobe.com/en_US/FlashPlatform/reference/actionscript/3/operators.html#dot_(XML)
* @see http://help.adobe.com/en_US/as3/dev/WS5b3ccc516d4fbf351e63e3d118a9b90204-7e71.html XML objects
*
* @example The following example shows that the elements()
method returns a list of elements only ? not comments, text properties, or processing instructions:
*
* var xml:XML =
* <foo>
* <!-- comment -->
* <?instruction ?>
* text
* <a>1</a>
* <b>2</b>
* </foo>;
* trace(xml.elements("*").length()); // 2
* trace(xml.elements("*")[0].toXMLString()); // <a>1</a>
* trace(xml.elements("b").length()); // 1
* trace(xml.elements("b")[0].toXMLString()); // <b>2</b>
*
* To return elements with names that match ActionScript reserved words, use the elements()
method instead of the XML dot (.) operator, as in the following example:
*
* var xml:XML =
* <student id="206">
* <class name="Trigonometry" />
* <class name="Spanish 2" />
* </student>
* trace(xml.elements("class"));
*
*/
public native function elements(name:Object = "*"):XMLList;
/**
* Checks to see whether the XML object contains complex content. An XML object contains complex content if it has child elements. XML objects that representing attributes, comments, processing instructions, and text nodes do not have complex content. However, an object that contains these can still be considered to contain complex content (if the object has child elements).
* @return If the XML object contains complex content, true
; otherwise false
.
*
* @see #hasSimpleContent()
* @see http://help.adobe.com/en_US/as3/dev/WS5b3ccc516d4fbf351e63e3d118a9b90204-7e71.html XML objects
*
* @example The following example shows an XML object with one property named a
that has simple content and one property named a
that has complex content:
*
* var xml:XML =
* <foo>
* <a>
* text
* </a>
* <a>
* <b/>
* </a>
* </foo>;
* trace(xml.a[0].hasComplexContent()); // false
* trace(xml.a[1].hasComplexContent()); // true
*
* trace(xml.a[0].hasSimpleContent()); // true
* trace(xml.a[1].hasSimpleContent()); // false
*
*/
public native function hasComplexContent():Boolean;
/**
* Checks to see whether the object has the property specified by the p
parameter.
* @param p The property to match.
*
* @return If the property exists, true
; otherwise false
.
*
* @see http://help.adobe.com/en_US/as3/dev/WS5b3ccc516d4fbf351e63e3d118a9b90204-7e71.html XML objects
*
* @example The following example uses the hasOwnProperty()
method to ensure that a property (b
) exists prior to evaluating an expression (b == "11"
) that uses the property:
*
* var xml:XML =
* <foo>
* <a />
* <a>
* <b>10</b>
* </a>
* <a>
* <b>11</b>
* </a>
* </foo>;
* trace(xml.a.(hasOwnProperty("b") && b == "11"));
* If the last line in this example were the following, Flash Player would throw an exception since the first element named a
does not have a property named b
:
* trace(xml.a.(b == "11"));
* The following example uses the hasOwnProperty()
method to ensure that a property (item
) exists prior to evaluating an expression (item.contains("toothbrush")
) that uses the property:
*
* var xml:XML =
* <orders>
* <order id='1'>
* <item>toothbrush</item>
* <item>toothpaste</item>
* </order>
* <order>
* <returnItem>shoe polish</returnItem>
* </order>
* </orders>;
* trace(xml.order.(hasOwnProperty("item") && item.contains("toothbrush")));
*
*/
public native function hasOwnProperty(p:String):Boolean;
/**
* Checks to see whether the XML object contains simple content. An XML object contains simple content if it represents a text node, an attribute node, or an XML element that has no child elements. XML objects that represent comments and processing instructions do not contain simple content.
* @return If the XML object contains simple content, true
; otherwise false
.
*
* @see #hasComplexContent()
* @see http://help.adobe.com/en_US/as3/dev/WS5b3ccc516d4fbf351e63e3d118a9b90204-7e71.html XML objects
*
* @example The following example shows an XML object with one property named a
that has simple content and one property named a
that has complex content:
*
* var xml:XML =
* <foo>
* <a>
* text
* </a>
* <a>
* <b/>
* </a>
* </foo>;
* trace(xml.a[0].hasComplexContent()); // false
* trace(xml.a[1].hasComplexContent()); // true
*
* trace(xml.a[0].hasSimpleContent()); // true
* trace(xml.a[1].hasSimpleContent()); // false
*
*/
public native function hasSimpleContent():Boolean;
/**
* Lists the namespaces for the XML object, based on the object's parent.
* @return An array of Namespace objects.
*
* @see http://help.adobe.com/en_US/as3/dev/WS5b3ccc516d4fbf351e63e3d118a9b90204-7e71.html XML objects
*
*/
public native function inScopeNamespaces():Array;
/**
* Inserts the given child2
parameter after the child1
parameter in this XML object and returns the resulting object. If the child1
parameter is null
, the method inserts the contents of child2
before all children of the XML object (in other words, after none). If child1
is provided, but it does not exist in the XML object, the XML object is not modified and undefined
is returned.
* If you call this method on an XML child that is not an element (text, attributes, comments, pi, and so on) undefined
is returned.
* Use the delete
(XML) operator to remove XML nodes.
* @param child1 The object in the source object that you insert before child2
.
* @param child2 The object to insert.
*
* @return The resulting XML object or undefined
.
*
* @see #insertChildBefore()
* @see http://help.adobe.com/en_US/FlashPlatform/reference/actionscript/3/operators.html#delete_(XML)
* @see http://help.adobe.com/en_US/as3/dev/WS5b3ccc516d4fbf351e63e3d118a9b90204-7e71.html XML objects
* @see http://help.adobe.com/en_US/as3/dev/WS5b3ccc516d4fbf351e63e3d118a9b90204-7e68.html Assembling and transforming XML objects
*
* @example The following example appends an element to the end of the child elements of an XML object:
*
* var xml:XML =
* <menu>
* <item>burger</item>
* <item>soda</item>
* </menu>;
* xml.insertChildAfter(xml.item[0], <saleItem>fries</saleItem>);
* trace(xml);
* The trace()
output is the following:
*
* <code><menu>
* <item>burger</item>
* <saleItem>fries</saleItem>
* <item>soda</item>
* </menu></code>
*
*/
public native function insertChildAfter(child1:Object, child2:Object):*;
/**
* Inserts the given child2
parameter before the child1
parameter in this XML object and returns the resulting object. If the child1
parameter is null
, the method inserts the contents of child2
after all children of the XML object (in other words, before none). If child1
is provided, but it does not exist in the XML object, the XML object is not modified and undefined
is returned.
* If you call this method on an XML child that is not an element (text, attributes, comments, pi, and so on) undefined
is returned.
* Use the delete
(XML) operator to remove XML nodes.
* @param child1 The object in the source object that you insert after child2
.
* @param child2 The object to insert.
*
* @return The resulting XML object or undefined
.
*
* @see #insertChildAfter()
* @see http://help.adobe.com/en_US/FlashPlatform/reference/actionscript/3/operators.html#delete_(XML)
* @see http://help.adobe.com/en_US/as3/dev/WS5b3ccc516d4fbf351e63e3d118a9b90204-7e71.html XML objects
* @see http://help.adobe.com/en_US/as3/dev/WS5b3ccc516d4fbf351e63e3d118a9b90204-7e68.html Assembling and transforming XML objects
*
* @example The following example appends an element to the end of the child elements of an XML object:
*
* var xml:XML =
* <menu>
* <item>burger</item>
* <item>soda</item>
* </menu>;
* xml.insertChildBefore(xml.item[0], <saleItem>fries</saleItem>);
* trace(xml);
* The trace()
output is the following:
*
*
*/
public native function insertChildBefore(child1:Object, child2:Object):*;
/**
* For XML objects, this method always returns the integer 1
. The length()
method of the XMLList class returns a value of 1
for an XMLList object that contains only one value.
* @return Always returns 1
for any XML object.
*
* @see http://help.adobe.com/en_US/as3/dev/WS5b3ccc516d4fbf351e63e3d118a9b90204-7e71.html XML objects
*
*/
public native function length():int;
/**
* Gives the local name portion of the qualified name of the XML object.
* @return The local name as either a String or null
.
*
* @see http://help.adobe.com/en_US/as3/dev/WS5b3ccc516d4fbf351e63e3d118a9b90204-7e71.html XML objects
*
* @example The following example illustrates the use of the localName()
method:
*
* var xml:XML =
* <soap:Envelope xmlns:soap="http://www.w3.org/2001/12/soap-envelope"
* soap:encodingStyle="http://www.w3.org/2001/12/soap-encoding">
*
* <soap:Body xmlns:wx = "http://example.com/weather">
* <wx:forecast>
* <wx:city>Quito</wx:city>
* </wx:forecast>
* </soap:Body>
* </soap:Envelope>;
*
* trace(xml.localName()); // Envelope
*
*/
public native function localName():Object;
/**
* Gives the qualified name for the XML object.
* @return The qualified name is either a QName or null
.
*
* @see #attributes()
* @see http://help.adobe.com/en_US/FlashPlatform/reference/actionscript/3/operators.html#attribute_identifier
* @see http://help.adobe.com/en_US/as3/dev/WS5b3ccc516d4fbf351e63e3d118a9b90204-7e71.html XML objects
*
* @example The following example illustrates the use of the name()
method to get the qualified name of an XML object:
*
* var xml:XML =
* <soap:Envelope xmlns:soap="http://www.w3.org/2001/12/soap-envelope"
* soap:encodingStyle="http://www.w3.org/2001/12/soap-encoding">
*
* <soap:Body xmlns:wx = "http://example.com/weather">
* <wx:forecast>
* <wx:city>Quito</wx:city>
* </wx:forecast>
* </soap:Body>
* </soap:Envelope>;
*
* trace(xml.name().localName); // Envelope
* trace(xml.name().uri); // "http://www.w3.org/2001/12/soap-envelope"
*
* The following example illustrates the use of the name()
method called on an XML property, on a text element, and on an attribute:
*
* var xml:XML =
* <foo x="15" y="22">
* text
* </foo>;
*
* trace(xml.name().localName); // foo
* trace(xml.name().uri == ""); // true
* trace(xml.children()[0]); // text
* trace(xml.children()[0].name()); // null
* trace(xml.attributes()[0]); // 15
* trace(xml.attributes()[0].name()); // x
*
*/
public native function name():Object;
/**
* If no parameter is provided, gives the namespace associated with the qualified name of this XML object. If a prefix
parameter is specified, the method returns the namespace that matches the prefix
parameter and that is in scope for the XML object. If there is no such namespace, the method returns undefined
.
* @param prefix The prefix you want to match.
*
* @return Returns null
, undefined
, or a namespace.
*
* @see http://help.adobe.com/en_US/as3/dev/WS5b3ccc516d4fbf351e63e3d118a9b90204-7e71.html XML objects
*
* @example The following example uses the namespace()
method to get the namespace of an XML object and assign it to a Namespace object named soap
which is then used in identifying a property of the xml
object (xml.soap::Body[0]
):
*
* var xml:XML =
* <soap:Envelope xmlns:soap="http://www.w3.org/2001/12/soap-envelope"
* soap:encodingStyle="http://www.w3.org/2001/12/soap-encoding">
*
* <soap:Body xmlns:wx = "http://example.com/weather">
* <wx:forecast>
* <wx:city>Quito</wx:city>
* </wx:forecast>
* </soap:Body>
* </soap:Envelope>;
*
* var soap:Namespace = xml.namespace();
* trace(soap.prefix); // soap
* trace(soap.uri); // http://www.w3.org/2001/12/soap-envelope
*
* var body:XML = xml.soap::Body[0];
* trace(body.namespace().prefix); // soap
* trace(xml.namespace().uri); // http://www.w3.org/2001/12/soap-envelope
* trace(body.namespace("wx").uri); // "http://example.com/weather"
*
* The following example uses the namespace()
method to get the default namespace for a node, as well as the namespace for a specific prefix ("dc"
):
*
* var xml:XML =
* <rdf:RDF xmlns:rdf="http://www.w3.org/1999/02/22-rdf-syntax-ns#"
* xmlns:dc="http://purl.org/dc/elements/1.1/"
* xmlns="http://purl.org/rss/1.0/">
* <!-- ... -->
* </rdf:RDF>;
*
* trace(xml.namespace()); // http://www.w3.org/1999/02/22-rdf-syntax-ns#
* trace(xml.namespace("dc")); // http://purl.org/dc/elements/1.1/
* trace(xml.namespace("foo")); // undefined
*
*/
public native function namespace(prefix:String = null):*;
/**
* Lists namespace declarations associated with the XML object in the context of its parent.
* @return An array of Namespace objects.
*
* @see #namespace()
* @see http://help.adobe.com/en_US/as3/dev/WS5b3ccc516d4fbf351e63e3d118a9b90204-7e71.html XML objects
*
* @example The following example outputs the namespace declarations of an XML object:
*
* var xml:XML =
* <rdf:RDF xmlns:rdf="http://www.w3.org/1999/02/22-rdf-syntax-ns#"
* xmlns:dc="http://purl.org/dc/elements/1.1/"
* xmlns="http://purl.org/rss/1.0/">
*
* <!-- ... -->
*
* </rdf:RDF>;
*
* for (var i:uint = 0; i < xml.namespaceDeclarations().length; i++) {
* var ns:Namespace = xml.namespaceDeclarations()[i];
* var prefix:String = ns.prefix;
* if (prefix == "") {
* prefix = "(default)";
* }
* trace(prefix + ":" , ns.uri);
* }
*
* The trace()
output is the following:
*
* <code>rdf: http://www.w3.org/1999/02/22-rdf-syntax-ns#
* dc: http://purl.org/dc/elements/1.1/
* (default): http://purl.org/rss/1.0/</code>
*
*/
public native function namespaceDeclarations():Array;
/**
* Specifies the type of node: text, comment, processing-instruction, attribute, or element.
* @return The node type used.
*
* @see http://help.adobe.com/en_US/FlashPlatform/reference/actionscript/3/operators.html#attribute_identifier
* @see http://help.adobe.com/en_US/as3/dev/WS5b3ccc516d4fbf351e63e3d118a9b90204-7e71.html XML objects
*
* @example This example traces all five node types:
*
* XML.ignoreComments = false;
* XML.ignoreProcessingInstructions = false;
*
* var xml:XML =
* <example id="10">
* <!-- this is a comment -->
* <?test this is a pi ?>
* and some text
* </example>;
*
* trace(xml.nodeKind()); // element
* trace(xml.children()[0].nodeKind()); // comment
* trace(xml.children()[1].nodeKind()); // processing-instruction
* trace(xml.children()[2].nodeKind()); // text
* trace(xml.@id[0].nodeKind()); // attribute
*
*/
public native function nodeKind():String;
/**
* For the XML object and all descendant XML objects, merges adjacent text nodes and eliminates empty text nodes.
* @return The resulting normalized XML object.
*
* @see http://help.adobe.com/en_US/as3/dev/WS5b3ccc516d4fbf351e63e3d118a9b90204-7e71.html XML objects
*
* @example The following example shows the effect of calling the normalize()
method:
*
* var xml:XML = <body></body>;
* xml.appendChild("hello");
* xml.appendChild(" world");
* trace(xml.children().length()); // 2
* xml.normalize();
* trace(xml.children().length()); // 1
*
*/
public native function normalize():XML;
/**
* Returns the parent of the XML object. If the XML object has no parent, the method returns undefined
.
* @return Either an XML reference of the parent node, or undefined
if the XML object has no parent.
*
* @see http://help.adobe.com/en_US/as3/dev/WS5b3ccc516d4fbf351e63e3d118a9b90204-7e71.html XML objects
* @see http://help.adobe.com/en_US/as3/dev/WS5b3ccc516d4fbf351e63e3d118a9b90204-7e6b.html Traversing XML structures
*
* @example The following example uses the parent()
method to identify the parent element of a specific element in an XML structure:
*
* var xml:XML =
* <body>
* <p id="p1">Hello</p>
* <p id="p2">Test:
* <ul>
* <li>1</li>
* <li>2</li>
* </ul>
* </p>
* </body>;
* var node:XML = xml.p.ul.(li.contains("1"))[0]; // == <ul> ... </ul>
* trace(node.parent().@id); // p2
*
*/
public native function parent():*;
/**
* Inserts a copy of the provided child
object into the XML element before any existing XML properties for that element.
* Use the delete
(XML) operator to remove XML nodes.
* @param value The object to insert.
*
* @return The resulting XML object.
*
* @see http://help.adobe.com/en_US/FlashPlatform/reference/actionscript/3/operators.html#delete_(XML)
* @see http://help.adobe.com/en_US/as3/dev/WS5b3ccc516d4fbf351e63e3d118a9b90204-7e71.html XML objects
* @see http://help.adobe.com/en_US/as3/dev/WS5b3ccc516d4fbf351e63e3d118a9b90204-7e68.html Assembling and transforming XML objects
*
* @example The following example uses the prependChild()
method to add an element to the begining of a child list of an XML object:
*
* var xml:XML =
* <body>
* <p>hello</p>
* </body>;
*
* xml.prependChild(<p>world</p>);
* trace(xml.p[0].toXMLString()); // <p>world</p>
* trace(xml.p[1].toXMLString()); // <p>hello</p>
*
*/
public native function prependChild(value:Object):XML;
/**
* If a name
parameter is provided, lists all the children of the XML object that contain processing instructions with that name
. With no parameters, the method lists all the children of the XML object that contain any processing instructions.
* @param name The name of the processing instructions to match.
*
* @return A list of matching child objects.
*
* @see http://help.adobe.com/en_US/as3/dev/WS5b3ccc516d4fbf351e63e3d118a9b90204-7e71.html XML objects
*
* @example The following example uses the processingInstructions()
method to get an array of processing instructions for an XML object:
*
* XML.ignoreProcessingInstructions = false;
* var xml:XML =
* <body>
* foo
* <?xml-stylesheet href="headlines.css" type="text/css" ?>
* <?instructionX ?>
*
* </body>;
*
* trace(xml.processingInstructions().length()); // 2
* trace(xml.processingInstructions()[0].name()); // xml-stylesheet
*
*/
public native function processingInstructions(name:String = "*"):XMLList;
/**
* Checks whether the property p
is in the set of properties that can be iterated in a for..in
statement applied to the XML object. Returns true
only if toString(p) == "0"
.
* @param p The property that you want to check.
*
* @return If the property can be iterated in a for..in
statement, true
; otherwise, false
.
*
* @see http://help.adobe.com/en_US/as3/dev/WS5b3ccc516d4fbf351e63e3d118a9b90204-7e71.html XML objects
*
* @example The following example shows that, for an XML object, the propertyNameIsEnumerable()
method returns a value of true
only for the value 0
; whereas for an XMLList object, the return value is true
for each valid index value for the XMLList object:
*
* var xml:XML =
* <body>
* <p>Hello</p>
* <p>World</p>
* </body>;
*
* trace(xml.propertyIsEnumerable(0)); // true
* trace(xml.propertyIsEnumerable(1)); // false
*
* for (var propertyName:String in xml) {
* trace(xml[propertyName]);
* }
*
* var list:XMLList = xml.p;
* trace(list.propertyIsEnumerable(0)); // true
* trace(list.propertyIsEnumerable(1)); // true
* trace(list.propertyIsEnumerable(2)); // false
*
* for (var propertyName:String in list) {
* trace(list[propertyName]);
* }
*
*/
public native function propertyIsEnumerable(p:String):Boolean;
/**
* Removes the given namespace for this object and all descendants. The removeNamespaces()
method does not remove a namespace if it is referenced by the object's qualified name or the qualified name of the object's attributes.
* @param ns The namespace to remove.
*
* @return A copy of the resulting XML object.
*
* @see http://help.adobe.com/en_US/as3/dev/WS5b3ccc516d4fbf351e63e3d118a9b90204-7e71.html XML objects
*
* @example The following example shows how to remove a namespace declaration from an XML object:
*
* var xml:XML =
* <rdf:RDF xmlns:rdf="http://www.w3.org/1999/02/22-rdf-syntax-ns#"
* xmlns:dc="http://purl.org/dc/elements/1.1/"
* xmlns="http://purl.org/rss/1.0/">
*
* <!-- ... -->
*
* </rdf:RDF>;
*
* trace(xml.namespaceDeclarations().length); // 3
* trace(xml.namespaceDeclarations()[0] is String); //
* var dc:Namespace = xml.namespace("dc");
* xml.removeNamespace(dc);
* trace(xml.namespaceDeclarations().length); // 2
*
*/
public native function removeNamespace(ns:Namespace):XML;
/**
* Replaces the properties specified by the propertyName
parameter with the given value
parameter. If no properties match propertyName
, the XML object is left unmodified.
* @param propertyName Can be a numeric value, an unqualified name for a set of XML elements, a qualified name for a set of XML elements, or the asterisk wildcard ("*"). Use an unqualified name to identify XML elements in the default namespace.
* @param value The replacement value. This can be an XML object, an XMLList object, or any value that can be converted with toString()
.
*
* @return The resulting XML object, with the matching properties replaced.
*
* @see http://help.adobe.com/en_US/as3/dev/WS5b3ccc516d4fbf351e63e3d118a9b90204-7e71.html XML objects
*
* @example The following example illustrates calling the replace()
method with an integer as the first parameter:
*
* var xml:XML =
* <body>
* <p>Hello</p>
* <p>World</p>
* <hr/>
* </body>;
*
* xml.replace(1, <p>Bob</p>);
* trace(xml);
*
* This results in the following trace()
output:
*
* <code><body>
* <p>Hello</p>
* <p>Bob</p>
* <hr/>
* </body>
* </code>
*
* The following example calls replace()
method with a string as the first parameter:
*
* var xml:XML =
* <body>
* <p>Hello</p>
* <p>World</p>
* <hr/>
* </body>;
*
* xml.replace("p", <p>Hi</p>);
* trace(xml);
*
* This results in the following trace()
output:
*
* <code><body>
* <p>Hi</p>
* <hr/>
* </body>;
* </code>
*
*
* The following example illustrates calling the replace()
method with a QName as the first parameter:
*
* var xml:XML =
* <ns:body xmlns:ns = "myNS">
* <ns:p>Hello</ns:p>
* <ns:p>World</ns:p>
* <hr/>
* </ns:body>;
*
* var qname:QName = new QName("myNS", "p");
* xml.replace(qname, <p>Bob</p>);
* trace(xml);
*
*
* This results in the following trace()
output:
*
* <code><ns:body xmlns:ns = "myNS">
* <p>Bob</p>
* <hr/>
* </ns:body>
* </code>
*
*
* The following example illustrates calling the replace()
method with the string "*"
as the first parameter:
*
* var xml:XML =
* <body>
* <p>Hello</p>
* <p>World</p>
* <hr/>
* </body>;
*
* xml.replace("*", <img src = "hello.jpg"/>);
* trace(xml);
*
* This results in the following trace()
output:
*
* <code><body>
* <img src="hello.jpg"/>
* </body>
* </code>
*
*
*/
public native function replace(propertyName:Object, value:XML):XML;
/**
* Replaces the child properties of the XML object with the specified set of XML properties, provided in the value
parameter.
* @param value The replacement XML properties. Can be a single XML object or an XMLList object.
*
* @return The resulting XML object.
*
* @see http://help.adobe.com/en_US/as3/dev/WS5b3ccc516d4fbf351e63e3d118a9b90204-7e71.html XML objects
*
* @example The following example illustrates calling the setChildren()
method, first using an XML object as the parameter, and then using an XMLList object as the parameter:
*
* var xml:XML =
* <body>
* <p>Hello</p>
* <p>World</p>
* </body>;
*
* var list:XMLList = xml.p;
*
* xml.setChildren(<p>hello</p>);
* trace(xml);
*
* // <body>
* // <p>hello</p>
* // </body>
*
* xml.setChildren(list);
* trace(xml);
*
* // <body>
* // <p>Hello</p>
* // <p>World</p>
* // </body>
*
*/
public native function setChildren(value:Object):XML;
/**
* Changes the local name of the XML object to the given name
parameter.
* @param name The replacement name for the local name.
*
* @see http://help.adobe.com/en_US/as3/dev/WS5b3ccc516d4fbf351e63e3d118a9b90204-7e71.html XML objects
*
* @example The following example uses the setLocalName()
method to change the local name of an XML element:
*
* var xml:XML =
* <ns:item xmlns:ns="http://example.com">
* toothbrush
* </ns:item>;
*
* xml.setLocalName("orderItem");
* trace(xml.toXMLString()); // <ns:orderItem xmlns:ns="http://example.com">toothbrush</ns:orderItem>
*
*/
public native function setLocalName(name:String):void;
/**
* Sets the name of the XML object to the given qualified name or attribute name.
* @param name The new name for the object.
*
* @see http://help.adobe.com/en_US/as3/dev/WS5b3ccc516d4fbf351e63e3d118a9b90204-7e71.html XML objects
*
* @example The following example uses the setName()
method to change the name of an XML element:
*
* var xml:XML =
* <item>
* toothbrush
* </item>;
*
* xml.setName("orderItem");
* trace(xml.toXMLString()); // <orderItem>toothbrush</orderItem>
*
*/
public native function setName(name:String):void;
/**
* Sets the namespace associated with the XML object.
* @param ns The new namespace.
*
* @see http://help.adobe.com/en_US/as3/dev/WS5b3ccc516d4fbf351e63e3d118a9b90204-7e71.html XML objects
*
* @example The following example uses the soap
namespace defined in one XML object and applies it to the namespace of another XML object (xml2
):
*
* var xml1:XML =
* <soap:Envelope xmlns:soap="http://www.w3.org/2001/12/soap-envelope"
* soap:encodingStyle="http://www.w3.org/2001/12/soap-encoding">
* <!-- ... -->
* </soap:Envelope>;
* var ns:Namespace = xml1.namespace("soap");
*
* var xml2:XML =
* <Envelope>
* <Body/>
* </Envelope>;
*
* xml2.setNamespace(ns);
*
* trace(xml2);
*
*/
public native function setNamespace(ns:Namespace):void;
/**
* Sets values for the following XML properties: ignoreComments
, ignoreProcessingInstructions
, ignoreWhitespace
, prettyIndent
, and prettyPrinting
. The following are the default settings, which are applied if no setObj
parameter is provided:
*
* XML.ignoreComments = true
* XML.ignoreProcessingInstructions = true
* XML.ignoreWhitespace = true
* XML.prettyIndent = 2
* XML.prettyPrinting = true
* Note: You do not apply this method to an instance of the XML class; you apply it to XML
, as in the following code: XML.setSettings()
.
* @param rest An object with each of the following properties:
*
* ignoreComments
* ignoreProcessingInstructions
* ignoreWhitespace
* prettyIndent
* prettyPrinting
*
* @see #ignoreComments
* @see #ignoreProcessingInstructions
* @see #ignoreWhitespace
* @see #prettyIndent
* @see #prettyPrinting
* @see #defaultSettings()
* @see #settings()
* @see http://help.adobe.com/en_US/as3/dev/WS5b3ccc516d4fbf351e63e3d118a9b90204-7e71.html XML objects
* @see http://help.adobe.com/en_US/as3/learn/WS5b3ccc516d4fbf351e63e3d118a9b90204-7fd0.html Functions
* @see http://help.adobe.com/en_US/as3/learn/WS5b3ccc516d4fbf351e63e3d118a9b90204-7f56.html Function parameters
*
* @example The following example shows: how to apply some custom settings (for including comments and processing instructions) prior to setting an XML object; how to then revert back to the default settings before setting another XML object; and then how to set the custom settings again (for setting any more XML objects):
*
* XML.ignoreComments = false;
* XML.ignoreProcessingInstructions = false;
* var customSettings:Object = XML.settings();
*
* var xml1:XML =
* <foo>
* <!-- comment -->
* <?instruction ?>
* </foo>;
* trace(xml1.toXMLString());
* // <foo>
* // <!-- comment -->
* // <?instruction ?>
* // </foo>
*
* XML.setSettings(XML.defaultSettings());
* var xml2:XML =
* <foo>
* <!-- comment -->
* <?instruction ?>
* </foo>;
* trace(xml2.toXMLString());
*
*/
public native static function setSettings(...rest):void;
/**
* Retrieves the following properties: ignoreComments
, ignoreProcessingInstructions
, ignoreWhitespace
, prettyIndent
, and prettyPrinting
.
* @return An object with the following XML properties:
*
* ignoreComments
* ignoreProcessingInstructions
* ignoreWhitespace
* prettyIndent
* prettyPrinting
*
* @see #ignoreComments
* @see #ignoreProcessingInstructions
* @see #ignoreWhitespace
* @see #prettyIndent
* @see #prettyPrinting
* @see #defaultSettings()
* @see #setSettings()
*
* @example The following example shows: how to apply some custom settings (for including comments and processing instructions) prior to setting an XML object; how to then revert back to the default settings before setting another XML object; and then how to set the custom settings again (for setting any more XML objects):
*
* XML.ignoreComments = false;
* XML.ignoreProcessingInstructions = false;
* var customSettings:Object = XML.settings();
*
* var xml1:XML =
* <foo>
* <!-- comment -->
* <?instruction ?>
* </foo>;
* trace(xml1.toXMLString());
* // <foo>
* // <!-- comment -->
* // <?instruction ?>
* // </foo>
*
* XML.setSettings(XML.defaultSettings());
* var xml2:XML =
* <foo>
* <!-- comment -->
* <?instruction ?>
* </foo>;
* trace(xml2.toXMLString());
*
*/
public native static function settings():Object;
/**
* Returns an XMLList object of all XML properties of the XML object that represent XML text nodes.
* @return The list of properties.
*
* @see http://help.adobe.com/en_US/as3/dev/WS5b3ccc516d4fbf351e63e3d118a9b90204-7e71.html XML objects
*
* @example The following example uses the text()
method to get the text nodes of an XML object:
*
* var xml:XML =
* <body>
* text1
* <hr/>
* text2
* </body>;
* trace(xml.text()[0]); // text1
* trace(xml.text()[1]); // text2
*
*/
[Native]
public native function text():XMLList;
/**
* Returns a string representation of the XML object. The rules for this conversion depend on whether the XML object has simple content or complex content:
*
* - If the XML object has simple content,
toString()
returns the String contents of the XML object with the following stripped out: the start tag, attributes, namespace declarations, and end tag.
*
* - If the XML object has complex content,
toString()
returns an XML encoded String representing the entire XML object, including the start tag, attributes, namespace declarations, and end tag.
* To return the entire XML object every time, use toXMLString()
.
* @return The string representation of the XML object.
*
* @see #hasSimpleContent()
* @see #hasComplexContent()
* @see #toXMLString()
* @see http://help.adobe.com/en_US/as3/dev/WS5b3ccc516d4fbf351e63e3d118a9b90204-7e6d.html XML type conversion
*
* @example The following example shows what the toString()
method returns when the XML object has simple content:
*
* var test:XML = <type name="Joe">example</type>;
* trace(test.toString()); //example
*
* The following example shows what the toString()
method returns when the XML object has complex content:
*
* var test:XML =
* <type name="Joe">
* <base name="Bob"></base>
* example
* </type>;
* trace(test.toString());
* // <type name="Joe">
* // <base name="Bob"/>
* // example
* // </type>
*
*/
[Native]
public native function toString():String;
/**
* Returns a string representation of the XML object. Unlike the toString()
method, the toXMLString()
method always returns the start tag, attributes, and end tag of the XML object, regardless of whether the XML object has simple content or complex content. (The toString()
method strips out these items for XML objects that contain simple content.)
* @return The string representation of the XML object.
*
* @see #toString()
* @see http://help.adobe.com/en_US/as3/dev/WS5b3ccc516d4fbf351e63e3d118a9b90204-7e71.html XML objects
* @see http://help.adobe.com/en_US/as3/dev/WS5b3ccc516d4fbf351e63e3d118a9b90204-7e6d.html XML type conversion
*
* @example The following example shows the difference between using the toString()
method (which is applied to all parameters of a trace()
method, by default) and using the toXMLString()
method:
*
* var xml:XML =
* <p>hello</p>;
* trace(xml); // hello
* trace(xml.toXMLString()); // <p>hello</p>
*
*/
[Native]
public native function toXMLString():String;
/**
* Returns the XML object.
* @return The primitive value of an XML instance.
*
* @see http://help.adobe.com/en_US/as3/dev/WS5b3ccc516d4fbf351e63e3d118a9b90204-7e71.html XML objects
*
* @example The following example shows that the value returned by the valueOf()
method is the same as the source XML object:
*
* var xml:XML = <p>hello</p>;
* trace(xml.valueOf() === xml); // true
*
*/
[Native]
public native function valueOf():XML;
}
}