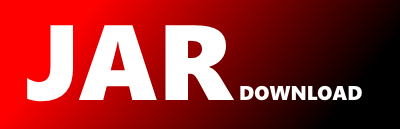
isb-ui.scripts.8bcb0ac5bcb6a64a1ac4.bundle.js Maven / Gradle / Ivy
webpackJsonp([1],{2:function(n,e,t){t("HLyl"),n.exports=t("uexX")},"3KOC":function(n,e){n.exports='/* Copyright 2014+, Federico Zivolo, LICENSE at https://github.com/FezVrasta/bootstrap-material-design/blob/master/LICENSE.md */\n/* globals jQuery, navigator */\n\n(function($, window, document, undefined) {\n\n "use strict";\n\n /**\n * Define the name of the plugin\n */\n var ripples = "ripples";\n\n\n /**\n * Get an instance of the plugin\n */\n var self = null;\n\n\n /**\n * Define the defaults of the plugin\n */\n var defaults = {};\n\n\n /**\n * Create the main plugin function\n */\n function Ripples(element, options) {\n self = this;\n\n this.element = $(element);\n\n this.options = $.extend({}, defaults, options);\n\n this._defaults = defaults;\n this._name = ripples;\n\n this.init();\n }\n\n\n /**\n * Initialize the plugin\n */\n Ripples.prototype.init = function() {\n var $element = this.element;\n\n $element.on("mousedown touchstart", function(event) {\n /**\n * Verify if the user is just touching on a device and return if so\n */\n if(self.isTouch() && event.type === "mousedown") {\n return;\n }\n\n\n /**\n * Verify if the current element already has a ripple wrapper element and\n * creates if it doesn\'t\n */\n if(!($element.find(".ripple-container").length)) {\n $element.append("");\n }\n\n\n /**\n * Find the ripple wrapper\n */\n var $wrapper = $element.children(".ripple-container");\n\n\n /**\n * Get relY and relX positions\n */\n var relY = self.getRelY($wrapper, event);\n var relX = self.getRelX($wrapper, event);\n\n\n /**\n * If relY and/or relX are false, return the event\n */\n if(!relY && !relX) {\n return;\n }\n\n\n /**\n * Get the ripple color\n */\n var rippleColor = self.getRipplesColor($element);\n\n\n /**\n * Create the ripple element\n */\n var $ripple = $("");\n\n $ripple\n .addClass("ripple")\n .css({\n "left": relX,\n "top": relY,\n "background-color": rippleColor\n });\n\n\n /**\n * Append the ripple to the wrapper\n */\n $wrapper.append($ripple);\n\n\n /**\n * Make sure the ripple has the styles applied (ugly hack but it works)\n */\n (function() { return window.getComputedStyle($ripple[0]).opacity; })();\n\n\n /**\n * Turn on the ripple animation\n */\n self.rippleOn($element, $ripple);\n\n\n /**\n * Call the rippleEnd function when the transition "on" ends\n */\n setTimeout(function() {\n self.rippleEnd($ripple);\n }, 500);\n\n\n /**\n * Detect when the user leaves the element\n */\n $element.on("mouseup mouseleave touchend", function() {\n $ripple.data("mousedown", "off");\n\n if($ripple.data("animating") === "off") {\n self.rippleOut($ripple);\n }\n });\n\n });\n };\n\n\n /**\n * Get the new size based on the element height/width and the ripple width\n */\n Ripples.prototype.getNewSize = function($element, $ripple) {\n\n return (Math.max($element.outerWidth(), $element.outerHeight()) / $ripple.outerWidth()) * 2.5;\n };\n\n\n /**\n * Get the relX\n */\n Ripples.prototype.getRelX = function($wrapper, event) {\n var wrapperOffset = $wrapper.offset();\n\n if(!self.isTouch()) {\n /**\n * Get the mouse position relative to the ripple wrapper\n */\n return event.pageX - wrapperOffset.left;\n } else {\n /**\n * Make sure the user is using only one finger and then get the touch\n * position relative to the ripple wrapper\n */\n event = event.originalEvent;\n\n if(event.touches.length === 1) {\n return event.touches[0].pageX - wrapperOffset.left;\n }\n\n return false;\n }\n };\n\n\n /**\n * Get the relY\n */\n Ripples.prototype.getRelY = function($wrapper, event) {\n var wrapperOffset = $wrapper.offset();\n\n if(!self.isTouch()) {\n /**\n * Get the mouse position relative to the ripple wrapper\n */\n return event.pageY - wrapperOffset.top;\n } else {\n /**\n * Make sure the user is using only one finger and then get the touch\n * position relative to the ripple wrapper\n */\n event = event.originalEvent;\n\n if(event.touches.length === 1) {\n return event.touches[0].pageY - wrapperOffset.top;\n }\n\n return false;\n }\n };\n\n\n /**\n * Get the ripple color\n */\n Ripples.prototype.getRipplesColor = function($element) {\n\n var color = $element.data("ripple-color") ? $element.data("ripple-color") : window.getComputedStyle($element[0]).color;\n\n return color;\n };\n\n\n /**\n * Verify if the client browser has transistion support\n */\n Ripples.prototype.hasTransitionSupport = function() {\n var thisBody = document.body || document.documentElement;\n var thisStyle = thisBody.style;\n\n var support = (\n thisStyle.transition !== undefined ||\n thisStyle.WebkitTransition !== undefined ||\n thisStyle.MozTransition !== undefined ||\n thisStyle.MsTransition !== undefined ||\n thisStyle.OTransition !== undefined\n );\n\n return support;\n };\n\n\n /**\n * Verify if the client is using a mobile device\n */\n Ripples.prototype.isTouch = function() {\n return /Android|webOS|iPhone|iPad|iPod|BlackBerry|IEMobile|Opera Mini/i.test(navigator.userAgent);\n };\n\n\n /**\n * End the animation of the ripple\n */\n Ripples.prototype.rippleEnd = function($ripple) {\n $ripple.data("animating", "off");\n\n if($ripple.data("mousedown") === "off") {\n self.rippleOut($ripple);\n }\n };\n\n\n /**\n * Turn off the ripple effect\n */\n Ripples.prototype.rippleOut = function($ripple) {\n $ripple.off();\n\n if(self.hasTransitionSupport()) {\n $ripple.addClass("ripple-out");\n } else {\n $ripple.animate({"opacity": 0}, 100, function() {\n $ripple.trigger("transitionend");\n });\n }\n\n $ripple.on("transitionend webkitTransitionEnd oTransitionEnd MSTransitionEnd", function() {\n $ripple.remove();\n });\n };\n\n\n /**\n * Turn on the ripple effect\n */\n Ripples.prototype.rippleOn = function($element, $ripple) {\n var size = self.getNewSize($element, $ripple);\n\n if(self.hasTransitionSupport()) {\n $ripple\n .css({\n "-ms-transform": "scale(" + size + ")",\n "-moz-transform": "scale(" + size + ")",\n "-webkit-transform": "scale(" + size + ")",\n "transform": "scale(" + size + ")"\n })\n .addClass("ripple-on")\n .data("animating", "on")\n .data("mousedown", "on");\n } else {\n $ripple.animate({\n "width": Math.max($element.outerWidth(), $element.outerHeight()) * 2,\n "height": Math.max($element.outerWidth(), $element.outerHeight()) * 2,\n "margin-left": Math.max($element.outerWidth(), $element.outerHeight()) * (-1),\n "margin-top": Math.max($element.outerWidth(), $element.outerHeight()) * (-1),\n "opacity": 0.2\n }, 500, function() {\n $ripple.trigger("transitionend");\n });\n }\n };\n\n\n /**\n * Create the jquery plugin function\n */\n $.fn.ripples = function(options) {\n return this.each(function() {\n if(!$.data(this, "plugin_" + ripples)) {\n $.data(this, "plugin_" + ripples, new Ripples(this, options));\n }\n });\n };\n\n})(jQuery, window, document);\n'},HLyl:function(n,e,t){t("KF6U")(t("r3cr"))},KF6U:function(n,e){n.exports=function(n){"undefined"!=typeof execScript?execScript(n):eval.call(null,n)}},r3cr:function(n,e){n.exports='/* globals jQuery */\n\n(function ($) {\n // Selector to select only not already processed elements\n $.expr[":"].notmdproc = function (obj) {\n if ($(obj).data("mdproc")) {\n return false;\n } else {\n return true;\n }\n };\n\n function _isChar(evt) {\n if (typeof evt.which == "undefined") {\n return true;\n } else if (typeof evt.which == "number" && evt.which > 0) {\n return (\n !evt.ctrlKey\n && !evt.metaKey\n && !evt.altKey\n && evt.which != 8 // backspace\n && evt.which != 9 // tab\n && evt.which != 13 // enter\n && evt.which != 16 // shift\n && evt.which != 17 // ctrl\n && evt.which != 20 // caps lock\n && evt.which != 27 // escape\n );\n }\n return false;\n }\n\n function _addFormGroupFocus(element) {\n var $element = $(element);\n if (!$element.prop(\'disabled\')) { // this is showing as undefined on chrome but works fine on firefox??\n $element.closest(".form-group").addClass("is-focused");\n }\n }\n\n function _toggleDisabledState($element, state) {\n var $target;\n if ($element.hasClass(\'checkbox-inline\') || $element.hasClass(\'radio-inline\')) {\n $target = $element;\n } else {\n $target = $element.closest(\'.checkbox\').length ? $element.closest(\'.checkbox\') : $element.closest(\'.radio\');\n }\n return $target.toggleClass(\'disabled\', state);\n }\n\n function _toggleTypeFocus($input) {\n var disabledToggleType = false;\n if ($input.is($.material.options.checkboxElements) || $input.is($.material.options.radioElements)) {\n disabledToggleType = true;\n }\n $input.closest(\'label\').hover(function () {\n var $i = $(this).find(\'input\');\n var isDisabled = $i.prop(\'disabled\'); // hack because the _addFormGroupFocus() wasn\'t identifying the property on chrome\n if (disabledToggleType) {\n _toggleDisabledState($(this), isDisabled);\n }\n if (!isDisabled) {\n _addFormGroupFocus($i); // need to find the input so we can check disablement\n }\n },\n function () {\n _removeFormGroupFocus($(this).find(\'input\'));\n });\n }\n\n function _removeFormGroupFocus(element) {\n $(element).closest(".form-group").removeClass("is-focused"); // remove class from form-group\n }\n\n $.material = {\n "options": {\n // These options set what will be started by $.material.init()\n "validate": true,\n "input": true,\n "ripples": true,\n "checkbox": true,\n "togglebutton": true,\n "radio": true,\n "arrive": true,\n "autofill": false,\n\n "withRipples": [\n ".btn:not(.btn-link)",\n ".card-image",\n ".navbar a:not(.withoutripple)",\n ".dropdown-menu a",\n ".nav-tabs a:not(.withoutripple)",\n ".withripple",\n ".pagination li:not(.active):not(.disabled) a:not(.withoutripple)"\n ].join(","),\n "inputElements": "input.form-control, textarea.form-control, select.form-control",\n "checkboxElements": ".checkbox > label > input[type=checkbox], label.checkbox-inline > input[type=checkbox]",\n "togglebuttonElements": ".togglebutton > label > input[type=checkbox]",\n "radioElements": ".radio > label > input[type=radio], label.radio-inline > input[type=radio]"\n },\n "checkbox": function (selector) {\n // Add fake-checkbox to material checkboxes\n var $input = $((selector) ? selector : this.options.checkboxElements)\n .filter(":notmdproc")\n .data("mdproc", true)\n .after("");\n\n _toggleTypeFocus($input);\n },\n "togglebutton": function (selector) {\n // Add fake-checkbox to material checkboxes\n var $input = $((selector) ? selector : this.options.togglebuttonElements)\n .filter(":notmdproc")\n .data("mdproc", true)\n .after("");\n\n _toggleTypeFocus($input);\n },\n "radio": function (selector) {\n // Add fake-radio to material radios\n var $input = $((selector) ? selector : this.options.radioElements)\n .filter(":notmdproc")\n .data("mdproc", true)\n .after("");\n\n _toggleTypeFocus($input);\n },\n "input": function (selector) {\n $((selector) ? selector : this.options.inputElements)\n .filter(":notmdproc")\n .data("mdproc", true)\n .each(function () {\n var $input = $(this);\n\n // Requires form-group standard markup (will add it if necessary)\n var $formGroup = $input.closest(".form-group"); // note that form-group may be grandparent in the case of an input-group\n if ($formGroup.length === 0 && $input.attr(\'type\') !== "hidden" && !$input.attr(\'hidden\')) {\n $input.wrap("");\n $formGroup = $input.closest(".form-group"); // find node after attached (otherwise additional attachments don\'t work)\n }\n\n // Legacy - Add hint label if using the old shorthand data-hint attribute on the input\n if ($input.attr("data-hint")) {\n $input.after("" + $input.attr("data-hint") + "
");\n $input.removeAttr("data-hint");\n }\n\n // Legacy - Change input-sm/lg to form-group-sm/lg instead (preferred standard and simpler css/less variants)\n var legacySizes = {\n "input-lg": "form-group-lg",\n "input-sm": "form-group-sm"\n };\n $.each(legacySizes, function (legacySize, standardSize) {\n if ($input.hasClass(legacySize)) {\n $input.removeClass(legacySize);\n $formGroup.addClass(standardSize);\n }\n });\n\n // Legacy - Add label-floating if using old shorthand \n if ($input.hasClass("floating-label")) {\n var placeholder = $input.attr("placeholder");\n $input.attr("placeholder", null).removeClass("floating-label");\n var id = $input.attr("id");\n var forAttribute = "";\n if (id) {\n forAttribute = "for=\'" + id + "\'";\n }\n $formGroup.addClass("label-floating");\n $input.after("");\n }\n\n // Set as empty if is empty (damn I must improve this...)\n if ($input.val() === null || $input.val() == "undefined" || $input.val() === "") {\n $formGroup.addClass("is-empty");\n }\n\n // Support for file input\n if ($formGroup.find("input[type=file]").length > 0) {\n $formGroup.addClass("is-fileinput");\n }\n });\n },\n "attachInputEventHandlers": function () {\n var validate = this.options.validate;\n\n $(document)\n .on("keydown paste", ".form-control", function (e) {\n if (_isChar(e)) {\n $(this).closest(".form-group").removeClass("is-empty");\n }\n })\n .on("keyup change", ".form-control", function () {\n var $input = $(this);\n var $formGroup = $input.closest(".form-group");\n var isValid = (typeof $input[0].checkValidity === "undefined" || $input[0].checkValidity());\n\n if ($input.val() === "") {\n $formGroup.addClass("is-empty");\n }\n else {\n $formGroup.removeClass("is-empty");\n }\n\n // Validation events do not bubble, so they must be attached directly to the input: http://jsfiddle.net/PEpRM/1/\n // Further, even the bind method is being caught, but since we are already calling #checkValidity here, just alter\n // the form-group on change.\n //\n // NOTE: I\'m not sure we should be intervening regarding validation, this seems better as a README and snippet of code.\n // BUT, I\'ve left it here for backwards compatibility.\n if (validate) {\n if (isValid) {\n $formGroup.removeClass("has-error");\n }\n else {\n $formGroup.addClass("has-error");\n }\n }\n })\n .on("focus", ".form-control, .form-group.is-fileinput", function () {\n _addFormGroupFocus(this);\n })\n .on("blur", ".form-control, .form-group.is-fileinput", function () {\n _removeFormGroupFocus(this);\n })\n // make sure empty is added back when there is a programmatic value change.\n // NOTE: programmatic changing of value using $.val() must trigger the change event i.e. $.val(\'x\').trigger(\'change\')\n .on("change", ".form-group input", function () {\n var $input = $(this);\n if ($input.attr("type") == "file") {\n return;\n }\n\n var $formGroup = $input.closest(".form-group");\n var value = $input.val();\n if (value) {\n $formGroup.removeClass("is-empty");\n } else {\n $formGroup.addClass("is-empty");\n }\n })\n // set the fileinput readonly field with the name of the file\n .on("change", ".form-group.is-fileinput input[type=\'file\']", function () {\n var $input = $(this);\n var $formGroup = $input.closest(".form-group");\n var value = "";\n $.each(this.files, function (i, file) {\n value += file.name + ", ";\n });\n value = value.substring(0, value.length - 2);\n if (value) {\n $formGroup.removeClass("is-empty");\n } else {\n $formGroup.addClass("is-empty");\n }\n $formGroup.find("input.form-control[readonly]").val(value);\n });\n },\n "ripples": function (selector) {\n $((selector) ? selector : this.options.withRipples).ripples();\n },\n "autofill": function () {\n // This part of code will detect autofill when the page is loading (username and password inputs for example)\n var loading = setInterval(function () {\n $("input[type!=checkbox]").each(function () {\n var $this = $(this);\n if ($this.val() && $this.val() !== $this.attr("value")) {\n $this.trigger("change");\n }\n });\n }, 100);\n\n // After 10 seconds we are quite sure all the needed inputs are autofilled then we can stop checking them\n setTimeout(function () {\n clearInterval(loading);\n }, 10000);\n },\n "attachAutofillEventHandlers": function () {\n // Listen on inputs of the focused form (because user can select from the autofill dropdown only when the input has focus)\n var focused;\n $(document)\n .on("focus", "input", function () {\n var $inputs = $(this).parents("form").find("input").not("[type=file]");\n focused = setInterval(function () {\n $inputs.each(function () {\n var $this = $(this);\n if ($this.val() !== $this.attr("value")) {\n $this.trigger("change");\n }\n });\n }, 100);\n })\n .on("blur", ".form-group input", function () {\n clearInterval(focused);\n });\n },\n "init": function (options) {\n this.options = $.extend({}, this.options, options);\n var $document = $(document);\n\n if ($.fn.ripples && this.options.ripples) {\n this.ripples();\n }\n if (this.options.input) {\n this.input();\n this.attachInputEventHandlers();\n }\n if (this.options.checkbox) {\n this.checkbox();\n }\n if (this.options.togglebutton) {\n this.togglebutton();\n }\n if (this.options.radio) {\n this.radio();\n }\n if (this.options.autofill) {\n this.autofill();\n this.attachAutofillEventHandlers();\n }\n\n if (document.arrive && this.options.arrive) {\n if ($.fn.ripples && this.options.ripples) {\n $document.arrive(this.options.withRipples, function () {\n $.material.ripples($(this));\n });\n }\n if (this.options.input) {\n $document.arrive(this.options.inputElements, function () {\n $.material.input($(this));\n });\n }\n if (this.options.checkbox) {\n $document.arrive(this.options.checkboxElements, function () {\n $.material.checkbox($(this));\n });\n }\n if (this.options.radio) {\n $document.arrive(this.options.radioElements, function () {\n $.material.radio($(this));\n });\n }\n if (this.options.togglebutton) {\n $document.arrive(this.options.togglebuttonElements, function () {\n $.material.togglebutton($(this));\n });\n }\n\n }\n }\n };\n\n})(jQuery);\n'},uexX:function(n,e,t){t("KF6U")(t("3KOC"))}},[2]);
© 2015 - 2025 Weber Informatics LLC | Privacy Policy