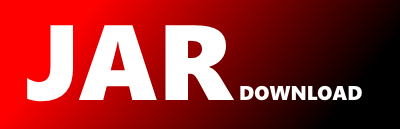
js.GalenCore.js Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of galen Show documentation
Show all versions of galen Show documentation
A library for layout testing of websites
/*******************************************************************************
* Copyright 2014 Ivan Shubin http://galenframework.com
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
* ******************************************************************************/
var GalenCore = {
settings: {
parameterization: {
stackBackwards: false
}
},
futureData: {
stack: [],
push: function (name, value) {
this.stack.push({
name: name,
value: value
});
},
pop: function () {
if (this.stack.length > 0) {
this.stack.pop();
}
},
fetchAll: function () {
var data = {};
for (var i = 0; i < this.stack.length; i++) {
var name = this.stack[i].name;
var value = this.stack[i].value;
if (data[name] == null || data[name] == undefined) {
if (Array.isArray(value)) {
data[name] = value;
}
else {
data[name] = [value];
}
}
else {
if (Array.isArray(value)) {
for (var k = 0; k < value.length; k++) {
data[name].push(value[k]);
}
}
else {
data[name].push(value);
}
}
}
return data;
}
},
parametersStack: {
stack: [],
add: function (parameters) {
this.stack.push(parameters);
},
pop: function () {
if (this.stack.length > 0) {
this.stack.pop();
}
},
last: function() {
if (this.stack.length > 0) {
return this.stack[this.stack.length - 1];
}
else return null;
},
fetchAll: function () {
var args = [];
if (GalenCore.settings.parameterization.stackBackwards) {
for (var i = this.stack.length - 1; i >= 0; i--) {
for (var j = 0; j < this.stack[i].length; j++) {
args.push(this.stack[i][j]);
}
}
}
else {
for (var i = 0; i < this.stack.length; i++) {
for (var j = 0; j < this.stack[i].length; j++) {
args.push(this.stack[i][j]);
}
}
}
return args;
}
},
processVariable: function (varName) {
var args = GalenCore.parametersStack.last();
if (args != null) {
if (args.length == 1) {
if (args[0] != null && typeof args[0] == "object") {
var argObject = args[0];
var value = eval("argObject." + varName);
return value;
}
}
}
return "";
},
processTestName: function (name) {
var parameters = this.parametersStack.last();
if (parameters != null) {
var text = "";
var processing = true;
var id = 0;
var varName = "";
// 0 - appending to text, 1 - appending to varName
var state = 0;
while(processing) {
var sym = name.charAt(id);
if (sym == "$" && id < name.length - 1 && name.charAt(id + 1) == "{") {
varName = "";
id++;
state = 1;
}
else if (state == 1 && name.charAt(id) == "}") {
state = 0;
if (varName.length > 0) {
text = text + GalenCore.processVariable(varName);
}
}
else {
if (state == 0) {
text = text + sym;
}
else {
varName = varName + sym;
}
}
id++;
processing = (id < name.length);
}
return text;
}
else return name;
}
};
function test(name, callback) {
var callbacks = [];
if (typeof callback == "function") {
callbacks = [callback];
}
var aTest = {
testName: GalenCore.processTestName(name),
callbacks: callbacks,
arguments: GalenCore.parametersStack.fetchAll(),
data: GalenCore.futureData.fetchAll(),
on: function (arguments, callback) {
if (Array.isArray(arguments)){
this.arguments = arguments;
} else {
this.arguments = [arguments];
}
return this.do(callback);
},
do: function (callback) {
if (typeof callback == "function") {
this.callbacks.push(callback);
}
return this;
},
/* All the following functions are implementations of GalenTest interface */
getName: function () {
return this.testName;
},
beforeTest: function () {
},
afterTest: function () {
},
checkLayout: function (driver, specFile, includedTags, excludedTags) {
return _galenCore.checkLayout(this.report, driver, includedTags, excludedTags);
},
execute: function (report, listener) {
this.report = report || null;
this.listener = listener || null;
if (this.callbacks != null) {
for (var i = 0; i < this.callbacks.length; i++) {
invokeFunc(this, this.arguments, this.callbacks[i]);
}
}
}
};
// A GalenTest is a Java interface which will be used by Galen Framework in order to execute js-based tests
_galenCore.addTest(new GalenTest(aTest));
return aTest;
};
function parameterizeByArray(rows, callback) {
for (var i = 0; i 0) {
try {
return callback(times);
}
catch (ex) {
return retry(times - 1, callback);
}
}
else return callback(times);
}
function createTestDataProvider(varName) {
return function (varValue, callback) {
GalenCore.futureData.push(varName, varValue);
callback();
GalenCore.futureData.pop();
};
}
(function (exports) {
exports.test = test;
exports.forAll = forAll;
exports.forOnly = forOnly;
exports.beforeTestSuite = beforeTestSuite;
exports.afterTestSuite = afterTestSuite;
exports.beforeTest = beforeTest;
exports.afterTest = afterTest;
exports.retry = retry;
exports.createTestDataProvider = createTestDataProvider;
exports.GalenCore = GalenCore;
})(this);
© 2015 - 2025 Weber Informatics LLC | Privacy Policy