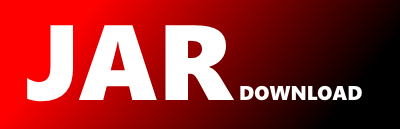
js.GalenPages.js Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of galen Show documentation
Show all versions of galen Show documentation
A library for layout testing of websites
/*******************************************************************************
* Copyright 2014 Ivan Shubin http://galenframework.com
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
* ******************************************************************************/
String.prototype.trim=function(){return this.replace(/^\s+|\s+$/g, '');};
function listToArray(list) {
return GalenUtils.listToArray(list);
}
var GalenPages = {
settings: {
cacheWebElements: true,
allowReporting: true,
},
report: function (name, details) {
if (GalenPages.settings.allowReporting) {
var testSession = TestSession.current();
if (testSession != null) {
var node = testSession.getReport().info(name);
if (details != undefined && details != null) {
node.withDetails(details);
}
}
}
},
Locator: function (type, value) {
this.type = type;
this.value = value;
},
parseLocator: function (locatorText) {
var index = locatorText.indexOf(":");
if (index > 0 ) {
var typeText = locatorText.substr(0, index).trim();
var value = locatorText.substr(index + 1, locatorText.length - 1 - index).trim();
var type = "css";
if (typeText == "id") {
type = typeText;
}
else if (typeText == "xpath") {
type = typeText;
}
else if (typeText == "css") {
type = typeText;
}
else {
throw new Error("Unknown locator type: " + typeText);
}
return new this.Locator(type, value);
}
else return {
type: "css",
value: locatorText
};
},
create: function (driver) {
return new GalenPages.Driver(driver);
},
extendPage: function (page, driver, mainFields, secondaryFields) {
var obj = new GalenPages.Page(driver, mainFields, secondaryFields);
for (key in obj) {
if (obj.hasOwnProperty(key)) {
page[key] = obj[key];
}
}
},
Driver: function (driver) {
this.driver = driver;
this.page = function (mainFields, secondaryFields) {
return new GalenPages.Page(this.driver, mainFields, secondaryFields);
};
this.component = this.page;
//basic functions
this.get = function (url){this.driver.get(url);};
this.refresh = function () {this.driver.navigate().reload();};
this.back = function (){this.driver.navigate().back();};
this.currentUrl = function (){return this.driver.getCurrentUrl();};
this.pageSource = function () {return this.driver.getPageSource();};
this.title = function () {return this.driver.getTitle();};
},
convertLocator: function(galenLocator) {
if (galenLocator.type == "id") {
return By.id(galenLocator.value);
}
else if (galenLocator.type == "css") {
return By.cssSelector(galenLocator.value);
}
else if (galenLocator.type == "xpath") {
return By.xpath(galenLocator.value);
}
},
convertTimeToMillis: function (userTime) {
if (typeof userTime == "string") {
var number = parseInt(userTime);
var type = userTime.replace(new RegExp("([0-9]| )", "g"), "");
if (type == "") {
return number;
}
else {
if (type == "m") {
return number * 60000;
}
else if(type == "s") {
return number * 1000;
}
else throw new Error("Cannot convert time. Uknown metric: " + type);
}
}
else return userTime;
},
Wait: function (settings) {
this.settings = settings;
if (settings.time == undefined) {
throw new Error("time was not defined");
}
var period = settings.period;
if (period == undefined) {
period = 1000;
}
this.message = null;
if (typeof settings.message == "string") {
this.message = settings.message;
}
else this.message = "timeout error waiting for:"
this.time = GalenPages.convertTimeToMillis(settings.time);
this.period = GalenPages.convertTimeToMillis(period);
//conditions is a map of functions which should return boolean
this.untilAll = function (conditions) {
var waitFuncs = [];
for (var property in conditions) {
if (conditions.hasOwnProperty(property)) {
var value = conditions[property];
waitFuncs[waitFuncs.length] = {
message: property,
func: value
};
}
}
if (waitFuncs.length > 0) {
var t = 0;
while (t < this.time) {
t = t + this.period;
if (this._checkAll(waitFuncs)) {
return;
}
GalenPages.sleep(this.period);
}
var errors = "";
for (var i = 0; i 0) {
throw new Error(this.message + errors);
}
}
else throw new Error("You are waiting for nothing");
};
this.forEach = function (items, itemConditionName, conditionFunc) {
var conditions = {};
for (var i=0; i 0 ) {
var conditions = {};
var primaryFields = this.primaryFields;
var page = this;
for (var i = 0; i
© 2015 - 2025 Weber Informatics LLC | Privacy Policy