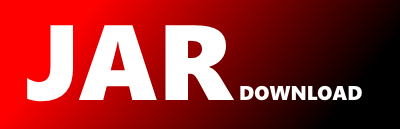
rimitive.1.0.source-code.Primitive Maven / Gradle / Ivy
package net.mintern.primitive;
import java.util.Arrays;
import net.mintern.primitive.comparators.BooleanComparator;
import net.mintern.primitive.comparators.ByteComparator;
import net.mintern.primitive.comparators.CharComparator;
import net.mintern.primitive.comparators.DoubleComparator;
import net.mintern.primitive.comparators.FloatComparator;
import net.mintern.primitive.comparators.IntComparator;
import net.mintern.primitive.comparators.LongComparator;
import net.mintern.primitive.comparators.ShortComparator;
/**
* A utility class that provides comparator-based sorting methods for all
* primitive arrays. It also provides non-comparator sort methods for
* {@code boolean} arrays since {@link Arrays} does not.
*/
public final class Primitive {
/**
* Sorts the given array so that all the {@code false} values are at the
* beginning.
*
* @param a the array to sort
* @throws NullPointerException if {@code a == null}
*/
public static void sort(boolean[] a) {
sort(a, 0, a.length);
}
/**
* Sorts the indicated portion of the given array so that all the
* {@code false} values are at the beginning.
*
* @param a the array to sort
* @param fromIndex the index (inclusive) marking the beginning of the array
* portion
* @param toIndex the index (exclusive) marking the end of the array portion
* @throws NullPointerException if {@code a == null}
* @throws ArrayIndexOutOfBoundsException if {@code fromIndex < 0} or
* {@code toIndex > a.length}
* @throws IllegalArgumentException if {@code fromIndex > toIndex}
*/
public static void sort(boolean[] a, int fromIndex, int toIndex) {
checkBounds(a.length, fromIndex, toIndex);
while (fromIndex < toIndex && !a[fromIndex]) {
fromIndex++;
}
if (fromIndex == toIndex) {
// all false values
return;
}
int nextFalse = fromIndex;
for (int i = fromIndex + 1; i < toIndex; i++) {
if (!a[i]) {
a[nextFalse] = false;
a[i] = true;
nextFalse++;
}
}
}
/**
* Sorts the given array by the given comparator. The sorting algorithm used
* is a stable sort, so two items that compare to 0 will be kept in the same
* order when the sort is complete.
*
* @param a the array to sort
* @param c the comparator to use for sorting the array, or {@code null} for
* natural ordering
* @throws NullPointerException if {@code a == null}
* @throws IllegalArgumentException if sorting finds that {@code c} violates
* the {@link BooleanComparator} contract
*/
public static void sort(boolean[] a, BooleanComparator c) {
sort(a, 0, a.length, c);
}
/**
* Sorts the indicated portion of the given array by the given comparator.
* The sorting algorithm used is a stable sort, so two items that compare to
* 0 will be kept in the same order when the sort is complete.
*
* @param a the array to sort
* @param fromIndex the index (inclusive) marking the beginning of the array
* portion
* @param toIndex the index (exclusive) marking the end of the array portion
* @param c the comparator to use for sorting the array, or {@code null} for
* natural ordering
* @throws NullPointerException if {@code a == null}
* @throws ArrayIndexOutOfBoundsException if {@code fromIndex < 0} or
* {@code toIndex > a.length}
* @throws IllegalArgumentException if {@code fromIndex > toIndex} or
* sorting finds that {@code c} violates the {@link BooleanComparator}
* contract
*/
public static void sort(boolean[] a, int fromIndex, int toIndex, BooleanComparator c) {
if (c == null) {
sort(a, fromIndex, toIndex);
} else {
checkBounds(a.length, fromIndex, toIndex);
BooleanTimSort.sort(a, fromIndex, toIndex, c, null, 0, 0);
}
}
/**
* Sorts the given array by the given comparator. The sorting algorithm used
* is a stable sort, so two items that compare to 0 will be kept in the same
* order when the sort is complete.
*
* @param a the array to sort
* @param c the comparator to use for sorting the array, or {@code null} for
* natural ordering
* @throws NullPointerException if {@code a == null}
* @throws IllegalArgumentException if sorting finds that {@code c} violates
* the {@link ByteComparator} contract
*/
public static void sort(byte[] a, ByteComparator c) {
sort(a, 0, a.length, c);
}
/**
* Sorts the indicated portion of the given array by the given comparator.
* The sorting algorithm used is a stable sort, so two items that compare to
* 0 will be kept in the same order when the sort is complete.
*
* @param a the array to sort
* @param fromIndex the index (inclusive) marking the beginning of the array
* portion
* @param toIndex the index (exclusive) marking the end of the array portion
* @param c the comparator to use for sorting the array, or {@code null} for
* natural ordering
* @throws NullPointerException if {@code a == null}
* @throws ArrayIndexOutOfBoundsException if {@code fromIndex < 0} or
* {@code toIndex > a.length}
* @throws IllegalArgumentException if {@code fromIndex > toIndex} or
* sorting finds that {@code c} violates the {@link ByteComparator}
* contract
*/
public static void sort(byte[] a, int fromIndex, int toIndex, ByteComparator c) {
if (c == null) {
Arrays.sort(a, fromIndex, toIndex);
} else {
checkBounds(a.length, fromIndex, toIndex);
ByteTimSort.sort(a, fromIndex, toIndex, c, null, 0, 0);
}
}
/**
* Sorts the given array by the given comparator. The sorting algorithm used
* is a stable sort, so two items that compare to 0 will be kept in the same
* order when the sort is complete.
*
* @param a the array to sort
* @param c the comparator to use for sorting the array, or {@code null} for
* natural ordering
* @throws NullPointerException if {@code a == null}
* @throws IllegalArgumentException if sorting finds that {@code c} violates
* the {@link CharComparator} contract
*/
public static void sort(char[] a, CharComparator c) {
sort(a, 0, a.length, c);
}
/**
* Sorts the indicated portion of the given array by the given comparator.
* The sorting algorithm used is a stable sort, so two items that compare to
* 0 will be kept in the same order when the sort is complete.
*
* @param a the array to sort
* @param fromIndex the index (inclusive) marking the beginning of the array
* portion
* @param toIndex the index (exclusive) marking the end of the array portion
* @param c the comparator to use for sorting the array, or {@code null} for
* natural ordering
* @throws NullPointerException if {@code a == null}
* @throws ArrayIndexOutOfBoundsException if {@code fromIndex < 0} or
* {@code toIndex > a.length}
* @throws IllegalArgumentException if {@code fromIndex > toIndex} or
* sorting finds that {@code c} violates the {@link CharComparator}
* contract
*/
public static void sort(char[] a, int fromIndex, int toIndex, CharComparator c) {
if (c == null) {
Arrays.sort(a, fromIndex, toIndex);
} else {
checkBounds(a.length, fromIndex, toIndex);
CharTimSort.sort(a, fromIndex, toIndex, c, null, 0, 0);
}
}
/**
* Sorts the given array by the given comparator. The sorting algorithm used
* is a stable sort, so two items that compare to 0 will be kept in the same
* order when the sort is complete.
*
* @param a the array to sort
* @param c the comparator to use for sorting the array, or {@code null} for
* natural ordering
* @throws NullPointerException if {@code a == null}
* @throws IllegalArgumentException if sorting finds that {@code c} violates
* the {@link DoubleComparator} contract
*/
public static void sort(double[] a, DoubleComparator c) {
sort(a, 0, a.length, c);
}
/**
* Sorts the indicated portion of the given array by the given comparator.
* The sorting algorithm used is a stable sort, so two items that compare to
* 0 will be kept in the same order when the sort is complete.
*
* @param a the array to sort
* @param fromIndex the index (inclusive) marking the beginning of the array
* portion
* @param toIndex the index (exclusive) marking the end of the array portion
* @param c the comparator to use for sorting the array, or {@code null} for
* natural ordering
* @throws NullPointerException if {@code a == null}
* @throws ArrayIndexOutOfBoundsException if {@code fromIndex < 0} or
* {@code toIndex > a.length}
* @throws IllegalArgumentException if {@code fromIndex > toIndex} or
* sorting finds that {@code c} violates the {@link DoubleComparator}
* contract
*/
public static void sort(double[] a, int fromIndex, int toIndex, DoubleComparator c) {
if (c == null) {
Arrays.sort(a, fromIndex, toIndex);
} else {
checkBounds(a.length, fromIndex, toIndex);
DoubleTimSort.sort(a, fromIndex, toIndex, c, null, 0, 0);
}
}
/**
* Sorts the given array by the given comparator. The sorting algorithm used
* is a stable sort, so two items that compare to 0 will be kept in the same
* order when the sort is complete.
*
* @param a the array to sort
* @param c the comparator to use for sorting the array, or {@code null} for
* natural ordering
* @throws NullPointerException if {@code a == null}
* @throws IllegalArgumentException if sorting finds that {@code c} violates
* the {@link FloatComparator} contract
*/
public static void sort(float[] a, FloatComparator c) {
sort(a, 0, a.length, c);
}
/**
* Sorts the indicated portion of the given array by the given comparator.
* The sorting algorithm used is a stable sort, so two items that compare to
* 0 will be kept in the same order when the sort is complete.
*
* @param a the array to sort
* @param fromIndex the index (inclusive) marking the beginning of the array
* portion
* @param toIndex the index (exclusive) marking the end of the array portion
* @param c the comparator to use for sorting the array, or {@code null} for
* natural ordering
* @throws NullPointerException if {@code a == null}
* @throws ArrayIndexOutOfBoundsException if {@code fromIndex < 0} or
* {@code toIndex > a.length}
* @throws IllegalArgumentException if {@code fromIndex > toIndex} or
* sorting finds that {@code c} violates the {@link FloatComparator}
* contract
*/
public static void sort(float[] a, int fromIndex, int toIndex, FloatComparator c) {
if (c == null) {
Arrays.sort(a, fromIndex, toIndex);
} else {
checkBounds(a.length, fromIndex, toIndex);
FloatTimSort.sort(a, fromIndex, toIndex, c, null, 0, 0);
}
}
/**
* Sorts the given array by the given comparator. The sorting algorithm used
* is a stable sort, so two items that compare to 0 will be kept in the same
* order when the sort is complete.
*
* @param a the array to sort
* @param c the comparator to use for sorting the array, or {@code null} for
* natural ordering
* @throws NullPointerException if {@code a == null}
* @throws IllegalArgumentException if sorting finds that {@code c} violates
* the {@link IntComparator} contract
*/
public static void sort(int[] a, IntComparator c) {
sort(a, 0, a.length, c);
}
/**
* Sorts the indicated portion of the given array by the given comparator.
* The sorting algorithm used is a stable sort, so two items that compare to
* 0 will be kept in the same order when the sort is complete.
*
* @param a the array to sort
* @param fromIndex the index (inclusive) marking the beginning of the array
* portion
* @param toIndex the index (exclusive) marking the end of the array portion
* @param c the comparator to use for sorting the array, or {@code null} for
* natural ordering
* @throws NullPointerException if {@code a == null}
* @throws ArrayIndexOutOfBoundsException if {@code fromIndex < 0} or
* {@code toIndex > a.length}
* @throws IllegalArgumentException if {@code fromIndex > toIndex} or
* sorting finds that {@code c} violates the {@link IntComparator}
* contract
*/
public static void sort(int[] a, int fromIndex, int toIndex, IntComparator c) {
if (c == null) {
Arrays.sort(a, fromIndex, toIndex);
} else {
checkBounds(a.length, fromIndex, toIndex);
IntTimSort.sort(a, fromIndex, toIndex, c, null, 0, 0);
}
}
/**
* Sorts the given array by the given comparator. The sorting algorithm used
* is a stable sort, so two items that compare to 0 will be kept in the same
* order when the sort is complete.
*
* @param a the array to sort
* @param c the comparator to use for sorting the array, or {@code null} for
* natural ordering
* @throws NullPointerException if {@code a == null}
* @throws IllegalArgumentException if sorting finds that {@code c} violates
* the {@link LongComparator} contract
*/
public static void sort(long[] a, LongComparator c) {
sort(a, 0, a.length, c);
}
/**
* Sorts the indicated portion of the given array by the given comparator.
* The sorting algorithm used is a stable sort, so two items that compare to
* 0 will be kept in the same order when the sort is complete.
*
* @param a the array to sort
* @param fromIndex the index (inclusive) marking the beginning of the array
* portion
* @param toIndex the index (exclusive) marking the end of the array portion
* @param c the comparator to use for sorting the array, or {@code null} for
* natural ordering
* @throws NullPointerException if {@code a == null}
* @throws ArrayIndexOutOfBoundsException if {@code fromIndex < 0} or
* {@code toIndex > a.length}
* @throws IllegalArgumentException if {@code fromIndex > toIndex} or
* sorting finds that {@code c} violates the {@link LongComparator}
* contract
*/
public static void sort(long[] a, int fromIndex, int toIndex, LongComparator c) {
if (c == null) {
Arrays.sort(a, fromIndex, toIndex);
} else {
checkBounds(a.length, fromIndex, toIndex);
LongTimSort.sort(a, fromIndex, toIndex, c, null, 0, 0);
}
}
/**
* Sorts the given array by the given comparator. The sorting algorithm used
* is a stable sort, so two items that compare to 0 will be kept in the same
* order when the sort is complete.
*
* @param a the array to sort
* @param c the comparator to use for sorting the array, or {@code null} for
* natural ordering
* @throws NullPointerException if {@code a == null}
* @throws IllegalArgumentException if sorting finds that {@code c} violates
* the {@link ShortComparator} contract
*/
public static void sort(short[] a, ShortComparator c) {
sort(a, 0, a.length, c);
}
/**
* Sorts the indicated portion of the given array by the given comparator.
* The sorting algorithm used is a stable sort, so two items that compare to
* 0 will be kept in the same order when the sort is complete.
*
* @param a the array to sort
* @param fromIndex the index (inclusive) marking the beginning of the array
* portion
* @param toIndex the index (exclusive) marking the end of the array portion
* @param c the comparator to use for sorting the array, or {@code null} for
* natural ordering
* @throws NullPointerException if {@code a == null}
* @throws ArrayIndexOutOfBoundsException if {@code fromIndex < 0} or
* {@code toIndex > a.length}
* @throws IllegalArgumentException if {@code fromIndex > toIndex} or
* sorting finds that {@code c} violates the {@link ShortComparator}
* contract
*/
public static void sort(short[] a, int fromIndex, int toIndex, ShortComparator c) {
if (c == null) {
Arrays.sort(a, fromIndex, toIndex);
} else {
checkBounds(a.length, fromIndex, toIndex);
ShortTimSort.sort(a, fromIndex, toIndex, c, null, 0, 0);
}
}
private static void checkBounds(int len, int fromIndex, int toIndex) {
if (fromIndex < 0) {
throw new ArrayIndexOutOfBoundsException("fromIndex < 0");
}
if (toIndex > len) {
throw new ArrayIndexOutOfBoundsException("toIndex > a.length");
}
if (fromIndex > toIndex) {
throw new IllegalArgumentException("fromIndex > toIndex");
}
}
private Primitive(){}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy