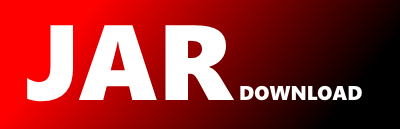
META-INF.resources.webjars.skeleton.js._polyfill.js Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of skeleton Show documentation
Show all versions of skeleton Show documentation
Common components for webapps
// Returns a function, that, as long as it continues to be invoked, will not
// be triggered. The function will be called after it stops being called for
// N milliseconds. If `immediate` is passed, trigger the function on the
// leading edge, instead of the trailing.
function debounce(func, wait, immediate) {
var timeout;
return function() {
var context = this, args = arguments;
var later = function() {
timeout = null;
if (!immediate) func.apply(context, args);
};
var callNow = immediate && !timeout;
clearTimeout(timeout);
timeout = setTimeout(later, wait);
if (callNow) func.apply(context, args);
};
};
// map is undefined on Safari
Uint8Array.prototype.map = Uint8Array.prototype.map || Array.prototype.map;
// Math.sign is undefined on Safari
// polyfill from MDN
Math.sign = Math.sign || function (x) {
x = +x; // convert to a number
if (x === 0 || isNaN(x)) {
return x;
}
return x > 0 ? 1 : -1;
};
String.prototype.startsWith = String.prototype.startsWith || function startsWith(search) {
/* http://mths.be/startswith v0.1.0 by @mathias */
var string = String(this);
if (this === null || Object.prototype.toString.call(search) === '[object RegExp]') {
throw TypeError();
}
var stringLength = string.length;
var searchString = String(search);
var position = (function (args) {
var p = args.length > 1 ? Number(args[1]) : 0;
return isNaN(p) ? 0 : p;
}(arguments));
var start = Math.min(Math.max(position, 0), stringLength);
return String.prototype.indexOf.call(string, searchString, position) === start;
};
// Replace placeholders in strings
// es. "{0}, {1}".replace("Hello", "World!") -> "Hello, World"
String.prototype.format = String.prototype.format || function () {
var parameters = arguments;
return this.replace(/{(\d+)}/g, function (placeholder, position) {
var parameter = parameters[position];
if (typeof parameter != 'undefined') {
return parameter;
}
return placeholder;
});
};
String.prototype.leftPad = String.prototype.leftPad || function (length, character) {
var text = "" + this;
var padding = "";
for (var i = 0; i < length - text.length; ++i) {
padding += character;
}
return padding + text;
};
String.isEmpty = String.isEmpty || function (string) {
return string === null || string === undefined || string === "";
}
String.prototype.replaceAll = function(search, replacement) {
var target = this;
return target.split(search).join(replacement);
};
Array.prototype.some = Array.prototype.some || function (fun, thisArg) {
'use strict';
if (this == null) {
throw new TypeError('Array.prototype.some called on null or undefined');
}
if (typeof fun !== 'function') {
throw new TypeError();
}
var t = Object(this);
var len = t.length >>> 0;
for (var i = 0; i < len; i++) {
if (i in t && fun.call(thisArg, t[i], i, t)) {
return true;
}
}
return false;
};
Array.prototype.contains = Array.prototype.contains || function (needle) {
return this.indexOf(needle) > -1;
};
Array.prototype.remove = Array.prototype.remove || function (item) {
var position = this.indexOf(item);
if (position === -1) {
return [];
}
return this.splice(position, 1);
};
Array.prototype.flatten = Array.prototype.flatten || function() {
return this.reduce(function (acc, item){ return acc.concat(item);}, []);
};
Array.prototype.split = Array.prototype.split || function (parts) {
if (parts < 1) {
throw "Cannot split array in zero or negative amounts";
}
var result = [];
var partLength = Math.ceil(this.length / parts);
var takenElements = 0;
for (var count = 0; count !== parts; ++count) {
var howMany = Math.min(partLength, this.length - takenElements);
result.push(this.slice(0 + takenElements, takenElements + howMany));
takenElements += howMany;
}
return result;
};
Array.prototype.groupBy = Array.prototype.groupBy || function(discriminator) {
var grouped = {};
for (var index = 0; index !== this.length; ++index) {
var key = discriminator(this[index]);
if (!grouped[key]) {
grouped[key] = [];
}
grouped[key].push(this[index]);
}
return grouped;
};
// Force redraw of dom element
function redraw(el) {
var empty = document.createTextNode(' ');
var previousDisplay = el.style.display;
el.appendChild(empty);
el.style.display = 'none';
setTimeout(function () {
el.style.display = previousDisplay;
empty.parentNode.removeChild(empty);
}, 20);
}
;
// Wrap a promise with a timeout. If the wrapped promise resolves before timeout,
// the wrapper will also resolve. Otherwise the wrapper will reject.
Promise.timeout = Promise.timeout || function (timeoutInMs, promise) {
return new Promise(function (resolve, reject) {
var timeoutId = setTimeout(function () {
reject("Timeout after " + timeoutInMs + "ms.");
}, timeoutInMs);
promise.then(function (val) {
clearTimeout(timeoutId);
resolve(val);
}).catch(function (e) {
clearTimeout(timeoutId);
reject(e);
});
});
};
// Return a promise that will resolve after the specified delay in ms
Promise.delay = Promise.delay || function (delayInMs) {
if (delayInMs !== 0) {
return new Promise(function (resolve, reject) {
setTimeout(resolve, delayInMs);
});
}
return Promise.resolve();
};
var Comparators = function () {};
Comparators.byProperty = function (property) {
return function (a, b) {
if (a[property] > b[property]) {
return 1;
}
if (a[property] < b[property]) {
return -1;
}
return 0;
};
};
Comparators.natural = function () {
return function (a, b) {
if (a > b) {
return 1;
}
if (a < b) {
return -1;
}
return 0;
};
};
Comparators.array = function () {
var compare = Comparators.natural();
return function (lhsArray, rhsArray) {
if (lhsArray.length !== rhsArray.length) {
return lhsArray.length - rhsArray.length;
}
for (var index = 0; index !== lhsArray.length; ++index) {
var comparison = compare(lhsArray[index], rhsArray[index]);
if (comparison !== 0) {
return comparison;
}
}
return 0;
};
};
Storage.prototype.setJson = function (key, object) {
localStorage.setItem(key, JSON.stringify(object));
};
Storage.prototype.getJson = function (key) {
return JSON.parse(localStorage.getItem(key));
};
© 2015 - 2025 Weber Informatics LLC | Privacy Policy