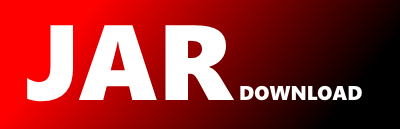
JavaScript.src.antlr4.Recognizer.js Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of antlr4-runtime-testsuite Show documentation
Show all versions of antlr4-runtime-testsuite Show documentation
A collection of tests for ANTLR 4 Runtime libraries.
//
// [The "BSD license"]
// Copyright (c) 2012 Terence Parr
// Copyright (c) 2012 Sam Harwell
// Copyright (c) 2014 Eric Vergnaud
// All rights reserved.
//
// Redistribution and use in source and binary forms, with or without
// modification, are permitted provided that the following conditions
// are met:
//
// 1. Redistributions of source code must retain the above copyright
// notice, this list of conditions and the following disclaimer.
// 2. Redistributions in binary form must reproduce the above copyright
// notice, this list of conditions and the following disclaimer in the
// documentation and/or other materials provided with the distribution.
// 3. The name of the author may not be used to endorse or promote products
// derived from this software without specific prior written permission.
//
// THIS SOFTWARE IS PROVIDED BY THE AUTHOR ``AS IS'' AND ANY EXPRESS OR
// IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES
// OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED.
// IN NO EVENT SHALL THE AUTHOR BE LIABLE FOR ANY DIRECT, INDIRECT,
// INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT
// NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE,
// DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY
// THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT
// (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF
// THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
//
var Token = require('./Token').Token;
var ConsoleErrorListener = require('./error/ErrorListener').ConsoleErrorListener;
var ProxyErrorListener = require('./error/ErrorListener').ProxyErrorListener;
function Recognizer() {
this._listeners = [ ConsoleErrorListener.INSTANCE ];
this._interp = null;
this._stateNumber = -1;
return this;
}
Recognizer.tokenTypeMapCache = {};
Recognizer.ruleIndexMapCache = {};
Recognizer.prototype.checkVersion = function(toolVersion) {
var runtimeVersion = "4.5.3";
if (runtimeVersion!==toolVersion) {
console.log("ANTLR runtime and generated code versions disagree: "+runtimeVersion+"!="+toolVersion);
}
};
Recognizer.prototype.addErrorListener = function(listener) {
this._listeners.push(listener);
};
Recognizer.prototype.removeErrorListeners = function() {
this._listeners = [];
};
Recognizer.prototype.getTokenTypeMap = function() {
var tokenNames = this.getTokenNames();
if (tokenNames===null) {
throw("The current recognizer does not provide a list of token names.");
}
var result = this.tokenTypeMapCache[tokenNames];
if(result===undefined) {
result = tokenNames.reduce(function(o, k, i) { o[k] = i; });
result.EOF = Token.EOF;
this.tokenTypeMapCache[tokenNames] = result;
}
return result;
};
// Get a map from rule names to rule indexes.
//
// Used for XPath and tree pattern compilation.
//
Recognizer.prototype.getRuleIndexMap = function() {
var ruleNames = this.getRuleNames();
if (ruleNames===null) {
throw("The current recognizer does not provide a list of rule names.");
}
var result = this.ruleIndexMapCache[ruleNames];
if(result===undefined) {
result = ruleNames.reduce(function(o, k, i) { o[k] = i; });
this.ruleIndexMapCache[ruleNames] = result;
}
return result;
};
Recognizer.prototype.getTokenType = function(tokenName) {
var ttype = this.getTokenTypeMap()[tokenName];
if (ttype !==undefined) {
return ttype;
} else {
return Token.INVALID_TYPE;
}
};
// What is the error header, normally line/character position information?//
Recognizer.prototype.getErrorHeader = function(e) {
var line = e.getOffendingToken().line;
var column = e.getOffendingToken().column;
return "line " + line + ":" + column;
};
// How should a token be displayed in an error message? The default
// is to display just the text, but during development you might
// want to have a lot of information spit out. Override in that case
// to use t.toString() (which, for CommonToken, dumps everything about
// the token). This is better than forcing you to override a method in
// your token objects because you don't have to go modify your lexer
// so that it creates a new Java type.
//
// @deprecated This method is not called by the ANTLR 4 Runtime. Specific
// implementations of {@link ANTLRErrorStrategy} may provide a similar
// feature when necessary. For example, see
// {@link DefaultErrorStrategy//getTokenErrorDisplay}.
//
Recognizer.prototype.getTokenErrorDisplay = function(t) {
if (t===null) {
return "";
}
var s = t.text;
if (s===null) {
if (t.type===Token.EOF) {
s = "";
} else {
s = "<" + t.type + ">";
}
}
s = s.replace("\n","\\n").replace("\r","\\r").replace("\t","\\t");
return "'" + s + "'";
};
Recognizer.prototype.getErrorListenerDispatch = function() {
return new ProxyErrorListener(this._listeners);
};
// subclass needs to override these if there are sempreds or actions
// that the ATN interp needs to execute
Recognizer.prototype.sempred = function(localctx, ruleIndex, actionIndex) {
return true;
};
Recognizer.prototype.precpred = function(localctx , precedence) {
return true;
};
//Indicate that the recognizer has changed internal state that is
//consistent with the ATN state passed in. This way we always know
//where we are in the ATN as the parser goes along. The rule
//context objects form a stack that lets us see the stack of
//invoking rules. Combine this and we have complete ATN
//configuration information.
Object.defineProperty(Recognizer.prototype, "state", {
get : function() {
return this._stateNumber;
},
set : function(state) {
this._stateNumber = state;
}
});
exports.Recognizer = Recognizer;
© 2015 - 2025 Weber Informatics LLC | Privacy Policy