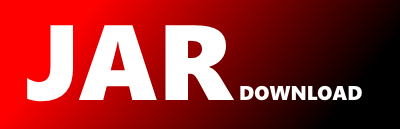
JavaScript.src.antlr4.atn.ATNDeserializer.js Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of antlr4-runtime-testsuite Show documentation
Show all versions of antlr4-runtime-testsuite Show documentation
A collection of tests for ANTLR 4 Runtime libraries.
// [The "BSD license"]
// Copyright (c) 2013 Terence Parr
// Copyright (c) 2013 Sam Harwell
// Copyright (c) 2014 Eric Vergnaud
// All rights reserved.
//
// Redistribution and use in source and binary forms, with or without
// modification, are permitted provided that the following conditions
// are met:
//
// 1. Redistributions of source code must retain the above copyright
// notice, this list of conditions and the following disclaimer.
// 2. Redistributions in binary form must reproduce the above copyright
// notice, this list of conditions and the following disclaimer in the
// documentation and/or other materials provided with the distribution.
// 3. The name of the author may not be used to endorse or promote products
// derived from this software without specific prior written permission.
//
// THIS SOFTWARE IS PROVIDED BY THE AUTHOR ``AS IS'' AND ANY EXPRESS OR
// IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES
// OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED.
// IN NO EVENT SHALL THE AUTHOR BE LIABLE FOR ANY DIRECT, INDIRECT,
// INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT
// NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE,
// DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY
// THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT
// (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF
// THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
var Token = require('./../Token').Token;
var ATN = require('./ATN').ATN;
var ATNType = require('./ATNType').ATNType;
var ATNStates = require('./ATNState');
var ATNState = ATNStates.ATNState;
var BasicState = ATNStates.BasicState;
var DecisionState = ATNStates.DecisionState;
var BlockStartState = ATNStates.BlockStartState;
var BlockEndState = ATNStates.BlockEndState;
var LoopEndState = ATNStates.LoopEndState;
var RuleStartState = ATNStates.RuleStartState;
var RuleStopState = ATNStates.RuleStopState;
var TokensStartState = ATNStates.TokensStartState;
var PlusLoopbackState = ATNStates.PlusLoopbackState;
var StarLoopbackState = ATNStates.StarLoopbackState;
var StarLoopEntryState = ATNStates.StarLoopEntryState;
var PlusBlockStartState = ATNStates.PlusBlockStartState;
var StarBlockStartState = ATNStates.StarBlockStartState;
var BasicBlockStartState = ATNStates.BasicBlockStartState;
var Transitions = require('./Transition');
var Transition = Transitions.Transition;
var AtomTransition = Transitions.AtomTransition;
var SetTransition = Transitions.SetTransition;
var NotSetTransition = Transitions.NotSetTransition;
var RuleTransition = Transitions.RuleTransition;
var RangeTransition = Transitions.RangeTransition;
var ActionTransition = Transitions.ActionTransition;
var EpsilonTransition = Transitions.EpsilonTransition;
var WildcardTransition = Transitions.WildcardTransition;
var PredicateTransition = Transitions.PredicateTransition;
var PrecedencePredicateTransition = Transitions.PrecedencePredicateTransition;
var IntervalSet = require('./../IntervalSet').IntervalSet;
var Interval = require('./../IntervalSet').Interval;
var ATNDeserializationOptions = require('./ATNDeserializationOptions').ATNDeserializationOptions;
var LexerActions = require('./LexerAction');
var LexerActionType = LexerActions.LexerActionType;
var LexerSkipAction = LexerActions.LexerSkipAction;
var LexerChannelAction = LexerActions.LexerChannelAction;
var LexerCustomAction = LexerActions.LexerCustomAction;
var LexerMoreAction = LexerActions.LexerMoreAction;
var LexerTypeAction = LexerActions.LexerTypeAction;
var LexerPushModeAction = LexerActions.LexerPushModeAction;
var LexerPopModeAction = LexerActions.LexerPopModeAction;
var LexerModeAction = LexerActions.LexerModeAction;
// This is the earliest supported serialized UUID.
// stick to serialized version for now, we don't need a UUID instance
var BASE_SERIALIZED_UUID = "AADB8D7E-AEEF-4415-AD2B-8204D6CF042E";
// This list contains all of the currently supported UUIDs, ordered by when
// the feature first appeared in this branch.
var SUPPORTED_UUIDS = [ BASE_SERIALIZED_UUID ];
var SERIALIZED_VERSION = 3;
// This is the current serialized UUID.
var SERIALIZED_UUID = BASE_SERIALIZED_UUID;
function initArray( length, value) {
var tmp = [];
tmp[length-1] = value;
return tmp.map(function(i) {return value;});
}
function ATNDeserializer (options) {
if ( options=== undefined || options === null ) {
options = ATNDeserializationOptions.defaultOptions;
}
this.deserializationOptions = options;
this.stateFactories = null;
this.actionFactories = null;
return this;
}
// Determines if a particular serialized representation of an ATN supports
// a particular feature, identified by the {@link UUID} used for serializing
// the ATN at the time the feature was first introduced.
//
// @param feature The {@link UUID} marking the first time the feature was
// supported in the serialized ATN.
// @param actualUuid The {@link UUID} of the actual serialized ATN which is
// currently being deserialized.
// @return {@code true} if the {@code actualUuid} value represents a
// serialized ATN at or after the feature identified by {@code feature} was
// introduced; otherwise, {@code false}.
ATNDeserializer.prototype.isFeatureSupported = function(feature, actualUuid) {
var idx1 = SUPPORTED_UUIDS.index(feature);
if (idx1<0) {
return false;
}
var idx2 = SUPPORTED_UUIDS.index(actualUuid);
return idx2 >= idx1;
};
ATNDeserializer.prototype.deserialize = function(data) {
this.reset(data);
this.checkVersion();
this.checkUUID();
var atn = this.readATN();
this.readStates(atn);
this.readRules(atn);
this.readModes(atn);
var sets = this.readSets(atn);
this.readEdges(atn, sets);
this.readDecisions(atn);
this.readLexerActions(atn);
this.markPrecedenceDecisions(atn);
this.verifyATN(atn);
if (this.deserializationOptions.generateRuleBypassTransitions && atn.grammarType === ATNType.PARSER ) {
this.generateRuleBypassTransitions(atn);
// re-verify after modification
this.verifyATN(atn);
}
return atn;
};
ATNDeserializer.prototype.reset = function(data) {
var adjust = function(c) {
var v = c.charCodeAt(0);
return v>1 ? v-2 : -1;
};
var temp = data.split("").map(adjust);
// don't adjust the first value since that's the version number
temp[0] = data.charCodeAt(0);
this.data = temp;
this.pos = 0;
};
ATNDeserializer.prototype.checkVersion = function() {
var version = this.readInt();
if ( version !== SERIALIZED_VERSION ) {
throw ("Could not deserialize ATN with version " + version + " (expected " + SERIALIZED_VERSION + ").");
}
};
ATNDeserializer.prototype.checkUUID = function() {
var uuid = this.readUUID();
if (SUPPORTED_UUIDS.indexOf(uuid)<0) {
throw ("Could not deserialize ATN with UUID: " + uuid +
" (expected " + SERIALIZED_UUID + " or a legacy UUID).", uuid, SERIALIZED_UUID);
}
this.uuid = uuid;
};
ATNDeserializer.prototype.readATN = function() {
var grammarType = this.readInt();
var maxTokenType = this.readInt();
return new ATN(grammarType, maxTokenType);
};
ATNDeserializer.prototype.readStates = function(atn) {
var j, pair, stateNumber;
var loopBackStateNumbers = [];
var endStateNumbers = [];
var nstates = this.readInt();
for(var i=0; i 0) {
bypassStart.addTransition(ruleToStartState.transitions[count-1]);
ruleToStartState.transitions = ruleToStartState.transitions.slice(-1);
}
// link the new states
atn.ruleToStartState[idx].addTransition(new EpsilonTransition(bypassStart));
bypassStop.addTransition(new EpsilonTransition(endState));
var matchState = new BasicState();
atn.addState(matchState);
matchState.addTransition(new AtomTransition(bypassStop, atn.ruleToTokenType[idx]));
bypassStart.addTransition(new EpsilonTransition(matchState));
};
ATNDeserializer.prototype.stateIsEndStateFor = function(state, idx) {
if ( state.ruleIndex !== idx) {
return null;
}
if (!( state instanceof StarLoopEntryState)) {
return null;
}
var maybeLoopEndState = state.transitions[state.transitions.length - 1].target;
if (!( maybeLoopEndState instanceof LoopEndState)) {
return null;
}
if (maybeLoopEndState.epsilonOnlyTransitions &&
(maybeLoopEndState.transitions[0].target instanceof RuleStopState)) {
return state;
} else {
return null;
}
};
//
// Analyze the {@link StarLoopEntryState} states in the specified ATN to set
// the {@link StarLoopEntryState//precedenceRuleDecision} field to the
// correct value.
//
// @param atn The ATN.
//
ATNDeserializer.prototype.markPrecedenceDecisions = function(atn) {
for(var i=0; i= 0);
} else {
this.checkCondition(state.transitions.length <= 1 || (state instanceof RuleStopState));
}
}
};
ATNDeserializer.prototype.checkCondition = function(condition, message) {
if (!condition) {
if (message === undefined || message===null) {
message = "IllegalState";
}
throw (message);
}
};
ATNDeserializer.prototype.readInt = function() {
return this.data[this.pos++];
};
ATNDeserializer.prototype.readInt32 = function() {
var low = this.readInt();
var high = this.readInt();
return low | (high << 16);
};
ATNDeserializer.prototype.readLong = function() {
var low = this.readInt32();
var high = this.readInt32();
return (low & 0x00000000FFFFFFFF) | (high << 32);
};
function createByteToHex() {
var bth = [];
for (var i = 0; i < 256; i++) {
bth[i] = (i + 0x100).toString(16).substr(1).toUpperCase();
}
return bth;
}
var byteToHex = createByteToHex();
ATNDeserializer.prototype.readUUID = function() {
var bb = [];
for(var i=7;i>=0;i--) {
var int = this.readInt();
/* jshint bitwise: false */
bb[(2*i)+1] = int & 0xFF;
bb[2*i] = (int >> 8) & 0xFF;
}
return byteToHex[bb[0]] + byteToHex[bb[1]] +
byteToHex[bb[2]] + byteToHex[bb[3]] + '-' +
byteToHex[bb[4]] + byteToHex[bb[5]] + '-' +
byteToHex[bb[6]] + byteToHex[bb[7]] + '-' +
byteToHex[bb[8]] + byteToHex[bb[9]] + '-' +
byteToHex[bb[10]] + byteToHex[bb[11]] +
byteToHex[bb[12]] + byteToHex[bb[13]] +
byteToHex[bb[14]] + byteToHex[bb[15]];
};
ATNDeserializer.prototype.edgeFactory = function(atn, type, src, trg, arg1, arg2, arg3, sets) {
var target = atn.states[trg];
switch(type) {
case Transition.EPSILON:
return new EpsilonTransition(target);
case Transition.RANGE:
return arg3 !== 0 ? new RangeTransition(target, Token.EOF, arg2) : new RangeTransition(target, arg1, arg2);
case Transition.RULE:
return new RuleTransition(atn.states[arg1], arg2, arg3, target);
case Transition.PREDICATE:
return new PredicateTransition(target, arg1, arg2, arg3 !== 0);
case Transition.PRECEDENCE:
return new PrecedencePredicateTransition(target, arg1);
case Transition.ATOM:
return arg3 !== 0 ? new AtomTransition(target, Token.EOF) : new AtomTransition(target, arg1);
case Transition.ACTION:
return new ActionTransition(target, arg1, arg2, arg3 !== 0);
case Transition.SET:
return new SetTransition(target, sets[arg1]);
case Transition.NOT_SET:
return new NotSetTransition(target, sets[arg1]);
case Transition.WILDCARD:
return new WildcardTransition(target);
default:
throw "The specified transition type: " + type + " is not valid.";
}
};
ATNDeserializer.prototype.stateFactory = function(type, ruleIndex) {
if (this.stateFactories === null) {
var sf = [];
sf[ATNState.INVALID_TYPE] = null;
sf[ATNState.BASIC] = function() { return new BasicState(); };
sf[ATNState.RULE_START] = function() { return new RuleStartState(); };
sf[ATNState.BLOCK_START] = function() { return new BasicBlockStartState(); };
sf[ATNState.PLUS_BLOCK_START] = function() { return new PlusBlockStartState(); };
sf[ATNState.STAR_BLOCK_START] = function() { return new StarBlockStartState(); };
sf[ATNState.TOKEN_START] = function() { return new TokensStartState(); };
sf[ATNState.RULE_STOP] = function() { return new RuleStopState(); };
sf[ATNState.BLOCK_END] = function() { return new BlockEndState(); };
sf[ATNState.STAR_LOOP_BACK] = function() { return new StarLoopbackState(); };
sf[ATNState.STAR_LOOP_ENTRY] = function() { return new StarLoopEntryState(); };
sf[ATNState.PLUS_LOOP_BACK] = function() { return new PlusLoopbackState(); };
sf[ATNState.LOOP_END] = function() { return new LoopEndState(); };
this.stateFactories = sf;
}
if (type>this.stateFactories.length || this.stateFactories[type] === null) {
throw("The specified state type " + type + " is not valid.");
} else {
var s = this.stateFactories[type]();
if (s!==null) {
s.ruleIndex = ruleIndex;
return s;
}
}
};
ATNDeserializer.prototype.lexerActionFactory = function(type, data1, data2) {
if (this.actionFactories === null) {
var af = [];
af[LexerActionType.CHANNEL] = function(data1, data2) { return new LexerChannelAction(data1); };
af[LexerActionType.CUSTOM] = function(data1, data2) { return new LexerCustomAction(data1, data2); };
af[LexerActionType.MODE] = function(data1, data2) { return new LexerModeAction(data1); };
af[LexerActionType.MORE] = function(data1, data2) { return LexerMoreAction.INSTANCE; };
af[LexerActionType.POP_MODE] = function(data1, data2) { return LexerPopModeAction.INSTANCE; };
af[LexerActionType.PUSH_MODE] = function(data1, data2) { return new LexerPushModeAction(data1); };
af[LexerActionType.SKIP] = function(data1, data2) { return LexerSkipAction.INSTANCE; };
af[LexerActionType.TYPE] = function(data1, data2) { return new LexerTypeAction(data1); };
this.actionFactories = af;
}
if (type>this.actionFactories.length || this.actionFactories[type] === null) {
throw("The specified lexer action type " + type + " is not valid.");
} else {
return this.actionFactories[type](data1, data2);
}
};
exports.ATNDeserializer = ATNDeserializer;
© 2015 - 2025 Weber Informatics LLC | Privacy Policy