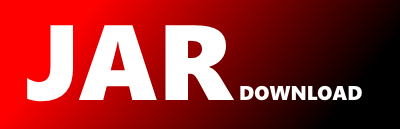
JavaScript.src.antlr4.atn.ATNState.js Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of antlr4-runtime-testsuite Show documentation
Show all versions of antlr4-runtime-testsuite Show documentation
A collection of tests for ANTLR 4 Runtime libraries.
//
// [The "BSD license"]
// Copyright (c) 2012 Terence Parr
// Copyright (c) 2012 Sam Harwell
// Copyright (c) 2014 Eric Vergnaud
// All rights reserved.
//
// Redistribution and use in source and binary forms, with or without
// modification, are permitted provided that the following conditions
// are met:
//
// 1. Redistributions of source code must retain the above copyright
// notice, this list of conditions and the following disclaimer.
// 2. Redistributions in binary form must reproduce the above copyright
// notice, this list of conditions and the following disclaimer in the
// documentation and/or other materials provided with the distribution.
// 3. The name of the author may not be used to endorse or promote products
// derived from this software without specific prior written permission.
//
// THIS SOFTWARE IS PROVIDED BY THE AUTHOR ``AS IS'' AND ANY EXPRESS OR
// IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES
// OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED.
// IN NO EVENT SHALL THE AUTHOR BE LIABLE FOR ANY DIRECT, INDIRECT,
// INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT
// NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE,
// DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY
// THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT
// (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF
// THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
//
// The following images show the relation of states and
// {@link ATNState//transitions} for various grammar constructs.
//
//
//
// - Solid edges marked with an &//0949; indicate a required
// {@link EpsilonTransition}.
//
// - Dashed edges indicate locations where any transition derived from
// {@link Transition} might appear.
//
// - Dashed nodes are place holders for either a sequence of linked
// {@link BasicState} states or the inclusion of a block representing a nested
// construct in one of the forms below.
//
// - Nodes showing multiple outgoing alternatives with a {@code ...} support
// any number of alternatives (one or more). Nodes without the {@code ...} only
// support the exact number of alternatives shown in the diagram.
//
//
//
// Basic Blocks
//
// Rule
//
//
//
// Block of 1 or more alternatives
//
//
//
// Greedy Loops
//
// Greedy Closure: {@code (...)*}
//
//
//
// Greedy Positive Closure: {@code (...)+}
//
//
//
// Greedy Optional: {@code (...)?}
//
//
//
// Non-Greedy Loops
//
// Non-Greedy Closure: {@code (...)*?}
//
//
//
// Non-Greedy Positive Closure: {@code (...)+?}
//
//
//
// Non-Greedy Optional: {@code (...)??}
//
//
//
var INITIAL_NUM_TRANSITIONS = 4;
function ATNState() {
// Which ATN are we in?
this.atn = null;
this.stateNumber = ATNState.INVALID_STATE_NUMBER;
this.stateType = null;
this.ruleIndex = 0; // at runtime, we don't have Rule objects
this.epsilonOnlyTransitions = false;
// Track the transitions emanating from this ATN state.
this.transitions = [];
// Used to cache lookahead during parsing, not used during construction
this.nextTokenWithinRule = null;
return this;
}
// constants for serialization
ATNState.INVALID_TYPE = 0;
ATNState.BASIC = 1;
ATNState.RULE_START = 2;
ATNState.BLOCK_START = 3;
ATNState.PLUS_BLOCK_START = 4;
ATNState.STAR_BLOCK_START = 5;
ATNState.TOKEN_START = 6;
ATNState.RULE_STOP = 7;
ATNState.BLOCK_END = 8;
ATNState.STAR_LOOP_BACK = 9;
ATNState.STAR_LOOP_ENTRY = 10;
ATNState.PLUS_LOOP_BACK = 11;
ATNState.LOOP_END = 12;
ATNState.serializationNames = [
"INVALID",
"BASIC",
"RULE_START",
"BLOCK_START",
"PLUS_BLOCK_START",
"STAR_BLOCK_START",
"TOKEN_START",
"RULE_STOP",
"BLOCK_END",
"STAR_LOOP_BACK",
"STAR_LOOP_ENTRY",
"PLUS_LOOP_BACK",
"LOOP_END" ];
ATNState.INVALID_STATE_NUMBER = -1;
ATNState.prototype.toString = function() {
return this.stateNumber;
};
ATNState.prototype.equals = function(other) {
if (other instanceof ATNState) {
return this.stateNumber===other.stateNumber;
} else {
return false;
}
};
ATNState.prototype.isNonGreedyExitState = function() {
return false;
};
ATNState.prototype.addTransition = function(trans, index) {
if(index===undefined) {
index = -1;
}
if (this.transitions.length===0) {
this.epsilonOnlyTransitions = trans.isEpsilon;
} else if(this.epsilonOnlyTransitions !== trans.isEpsilon) {
this.epsilonOnlyTransitions = false;
}
if (index===-1) {
this.transitions.push(trans);
} else {
this.transitions.splice(index, 1, trans);
}
};
function BasicState() {
ATNState.call(this);
this.stateType = ATNState.BASIC;
return this;
}
BasicState.prototype = Object.create(ATNState.prototype);
BasicState.prototype.constructor = BasicState;
function DecisionState() {
ATNState.call(this);
this.decision = -1;
this.nonGreedy = false;
return this;
}
DecisionState.prototype = Object.create(ATNState.prototype);
DecisionState.prototype.constructor = DecisionState;
// The start of a regular {@code (...)} block.
function BlockStartState() {
DecisionState.call(this);
this.endState = null;
return this;
}
BlockStartState.prototype = Object.create(DecisionState.prototype);
BlockStartState.prototype.constructor = BlockStartState;
function BasicBlockStartState() {
BlockStartState.call(this);
this.stateType = ATNState.BLOCK_START;
return this;
}
BasicBlockStartState.prototype = Object.create(BlockStartState.prototype);
BasicBlockStartState.prototype.constructor = BasicBlockStartState;
// Terminal node of a simple {@code (a|b|c)} block.
function BlockEndState() {
ATNState.call(this);
this.stateType = ATNState.BLOCK_END;
this.startState = null;
return this;
}
BlockEndState.prototype = Object.create(ATNState.prototype);
BlockEndState.prototype.constructor = BlockEndState;
// The last node in the ATN for a rule, unless that rule is the start symbol.
// In that case, there is one transition to EOF. Later, we might encode
// references to all calls to this rule to compute FOLLOW sets for
// error handling.
//
function RuleStopState() {
ATNState.call(this);
this.stateType = ATNState.RULE_STOP;
return this;
}
RuleStopState.prototype = Object.create(ATNState.prototype);
RuleStopState.prototype.constructor = RuleStopState;
function RuleStartState() {
ATNState.call(this);
this.stateType = ATNState.RULE_START;
this.stopState = null;
this.isPrecedenceRule = false;
return this;
}
RuleStartState.prototype = Object.create(ATNState.prototype);
RuleStartState.prototype.constructor = RuleStartState;
// Decision state for {@code A+} and {@code (A|B)+}. It has two transitions:
// one to the loop back to start of the block and one to exit.
//
function PlusLoopbackState() {
DecisionState.call(this);
this.stateType = ATNState.PLUS_LOOP_BACK;
return this;
}
PlusLoopbackState.prototype = Object.create(DecisionState.prototype);
PlusLoopbackState.prototype.constructor = PlusLoopbackState;
// Start of {@code (A|B|...)+} loop. Technically a decision state, but
// we don't use for code generation; somebody might need it, so I'm defining
// it for completeness. In reality, the {@link PlusLoopbackState} node is the
// real decision-making note for {@code A+}.
//
function PlusBlockStartState() {
BlockStartState.call(this);
this.stateType = ATNState.PLUS_BLOCK_START;
this.loopBackState = null;
return this;
}
PlusBlockStartState.prototype = Object.create(BlockStartState.prototype);
PlusBlockStartState.prototype.constructor = PlusBlockStartState;
// The block that begins a closure loop.
function StarBlockStartState() {
BlockStartState.call(this);
this.stateType = ATNState.STAR_BLOCK_START;
return this;
}
StarBlockStartState.prototype = Object.create(BlockStartState.prototype);
StarBlockStartState.prototype.constructor = StarBlockStartState;
function StarLoopbackState() {
ATNState.call(this);
this.stateType = ATNState.STAR_LOOP_BACK;
return this;
}
StarLoopbackState.prototype = Object.create(ATNState.prototype);
StarLoopbackState.prototype.constructor = StarLoopbackState;
function StarLoopEntryState() {
DecisionState.call(this);
this.stateType = ATNState.STAR_LOOP_ENTRY;
this.loopBackState = null;
// Indicates whether this state can benefit from a precedence DFA during SLL decision making.
this.precedenceRuleDecision = null;
return this;
}
StarLoopEntryState.prototype = Object.create(DecisionState.prototype);
StarLoopEntryState.prototype.constructor = StarLoopEntryState;
// Mark the end of a * or + loop.
function LoopEndState() {
ATNState.call(this);
this.stateType = ATNState.LOOP_END;
this.loopBackState = null;
return this;
}
LoopEndState.prototype = Object.create(ATNState.prototype);
LoopEndState.prototype.constructor = LoopEndState;
// The Tokens rule start state linking to each lexer rule start state */
function TokensStartState() {
DecisionState.call(this);
this.stateType = ATNState.TOKEN_START;
return this;
}
TokensStartState.prototype = Object.create(DecisionState.prototype);
TokensStartState.prototype.constructor = TokensStartState;
exports.ATNState = ATNState;
exports.BasicState = BasicState;
exports.DecisionState = DecisionState;
exports.BlockStartState = BlockStartState;
exports.BlockEndState = BlockEndState;
exports.LoopEndState = LoopEndState;
exports.RuleStartState = RuleStartState;
exports.RuleStopState = RuleStopState;
exports.TokensStartState = TokensStartState;
exports.PlusLoopbackState = PlusLoopbackState;
exports.StarLoopbackState = StarLoopbackState;
exports.StarLoopEntryState = StarLoopEntryState;
exports.PlusBlockStartState = PlusBlockStartState;
exports.StarBlockStartState = StarBlockStartState;
exports.BasicBlockStartState = BasicBlockStartState;
© 2015 - 2025 Weber Informatics LLC | Privacy Policy