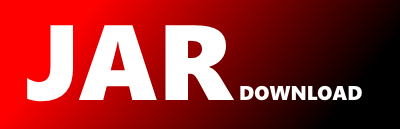
JavaScript.src.antlr4.atn.LexerAction.js Maven / Gradle / Ivy
Show all versions of antlr4-runtime-testsuite Show documentation
//
//[The "BSD license"]
// Copyright (c) 2013 Terence Parr
// Copyright (c) 2013 Sam Harwell
// Copyright (c) 2014 Eric Vergnaud
// All rights reserved.
//
// Redistribution and use in source and binary forms, with or without
// modification, are permitted provided that the following conditions
// are met:
//
// 1. Redistributions of source code must retain the above copyright
// notice, this list of conditions and the following disclaimer.
// 2. Redistributions in binary form must reproduce the above copyright
// notice, this list of conditions and the following disclaimer in the
// documentation and/or other materials provided with the distribution.
// 3. The name of the author may not be used to endorse or promote products
// derived from this software without specific prior written permission.
//
// THIS SOFTWARE IS PROVIDED BY THE AUTHOR ``AS IS'' AND ANY EXPRESS OR
// IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES
// OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED.
// IN NO EVENT SHALL THE AUTHOR BE LIABLE FOR ANY DIRECT, INDIRECT,
// INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT
// NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE,
// DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY
// THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT
// (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF
// THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
//
function LexerActionType() {
}
LexerActionType.CHANNEL = 0; //The type of a {@link LexerChannelAction} action.
LexerActionType.CUSTOM = 1; //The type of a {@link LexerCustomAction} action.
LexerActionType.MODE = 2; //The type of a {@link LexerModeAction} action.
LexerActionType.MORE = 3; //The type of a {@link LexerMoreAction} action.
LexerActionType.POP_MODE = 4; //The type of a {@link LexerPopModeAction} action.
LexerActionType.PUSH_MODE = 5; //The type of a {@link LexerPushModeAction} action.
LexerActionType.SKIP = 6; //The type of a {@link LexerSkipAction} action.
LexerActionType.TYPE = 7; //The type of a {@link LexerTypeAction} action.
function LexerAction(action) {
this.actionType = action;
this.isPositionDependent = false;
return this;
}
LexerAction.prototype.hashString = function() {
return "" + this.actionType;
};
LexerAction.prototype.equals = function(other) {
return this === other;
};
//
// Implements the {@code skip} lexer action by calling {@link Lexer//skip}.
//
// The {@code skip} command does not have any parameters, so this action is
// implemented as a singleton instance exposed by {@link //INSTANCE}.
function LexerSkipAction() {
LexerAction.call(this, LexerActionType.SKIP);
return this;
}
LexerSkipAction.prototype = Object.create(LexerAction.prototype);
LexerSkipAction.prototype.constructor = LexerSkipAction;
// Provides a singleton instance of this parameterless lexer action.
LexerSkipAction.INSTANCE = new LexerSkipAction();
LexerSkipAction.prototype.execute = function(lexer) {
lexer.skip();
};
LexerSkipAction.prototype.toString = function() {
return "skip";
};
// Implements the {@code type} lexer action by calling {@link Lexer//setType}
// with the assigned type.
function LexerTypeAction(type) {
LexerAction.call(this, LexerActionType.TYPE);
this.type = type;
return this;
}
LexerTypeAction.prototype = Object.create(LexerAction.prototype);
LexerTypeAction.prototype.constructor = LexerTypeAction;
LexerTypeAction.prototype.execute = function(lexer) {
lexer.type = this.type;
};
LexerTypeAction.prototype.hashString = function() {
return "" + this.actionType + this.type;
};
LexerTypeAction.prototype.equals = function(other) {
if(this === other) {
return true;
} else if (! (other instanceof LexerTypeAction)) {
return false;
} else {
return this.type === other.type;
}
};
LexerTypeAction.prototype.toString = function() {
return "type(" + this.type + ")";
};
// Implements the {@code pushMode} lexer action by calling
// {@link Lexer//pushMode} with the assigned mode.
function LexerPushModeAction(mode) {
LexerAction.call(this, LexerActionType.PUSH_MODE);
this.mode = mode;
return this;
}
LexerPushModeAction.prototype = Object.create(LexerAction.prototype);
LexerPushModeAction.prototype.constructor = LexerPushModeAction;
// This action is implemented by calling {@link Lexer//pushMode} with the
// value provided by {@link //getMode}.
LexerPushModeAction.prototype.execute = function(lexer) {
lexer.pushMode(this.mode);
};
LexerPushModeAction.prototype.hashString = function() {
return "" + this.actionType + this.mode;
};
LexerPushModeAction.prototype.equals = function(other) {
if (this === other) {
return true;
} else if (! (other instanceof LexerPushModeAction)) {
return false;
} else {
return this.mode === other.mode;
}
};
LexerPushModeAction.prototype.toString = function() {
return "pushMode(" + this.mode + ")";
};
// Implements the {@code popMode} lexer action by calling {@link Lexer//popMode}.
//
// The {@code popMode} command does not have any parameters, so this action is
// implemented as a singleton instance exposed by {@link //INSTANCE}.
function LexerPopModeAction() {
LexerAction.call(this,LexerActionType.POP_MODE);
return this;
}
LexerPopModeAction.prototype = Object.create(LexerAction.prototype);
LexerPopModeAction.prototype.constructor = LexerPopModeAction;
LexerPopModeAction.INSTANCE = new LexerPopModeAction();
// This action is implemented by calling {@link Lexer//popMode}.
LexerPopModeAction.prototype.execute = function(lexer) {
lexer.popMode();
};
LexerPopModeAction.prototype.toString = function() {
return "popMode";
};
// Implements the {@code more} lexer action by calling {@link Lexer//more}.
//
// The {@code more} command does not have any parameters, so this action is
// implemented as a singleton instance exposed by {@link //INSTANCE}.
function LexerMoreAction() {
LexerAction.call(this, LexerActionType.MORE);
return this;
}
LexerMoreAction.prototype = Object.create(LexerAction.prototype);
LexerMoreAction.prototype.constructor = LexerMoreAction;
LexerMoreAction.INSTANCE = new LexerMoreAction();
// This action is implemented by calling {@link Lexer//popMode}.
LexerMoreAction.prototype.execute = function(lexer) {
lexer.more();
};
LexerMoreAction.prototype.toString = function() {
return "more";
};
// Implements the {@code mode} lexer action by calling {@link Lexer//mode} with
// the assigned mode.
function LexerModeAction(mode) {
LexerAction.call(this, LexerActionType.MODE);
this.mode = mode;
return this;
}
LexerModeAction.prototype = Object.create(LexerAction.prototype);
LexerModeAction.prototype.constructor = LexerModeAction;
// This action is implemented by calling {@link Lexer//mode} with the
// value provided by {@link //getMode}.
LexerModeAction.prototype.execute = function(lexer) {
lexer.mode(this.mode);
};
LexerModeAction.prototype.hashString = function() {
return "" + this.actionType + this.mode;
};
LexerModeAction.prototype.equals = function(other) {
if (this === other) {
return true;
} else if (! (other instanceof LexerModeAction)) {
return false;
} else {
return this.mode === other.mode;
}
};
LexerModeAction.prototype.toString = function() {
return "mode(" + this.mode + ")";
};
// Executes a custom lexer action by calling {@link Recognizer//action} with the
// rule and action indexes assigned to the custom action. The implementation of
// a custom action is added to the generated code for the lexer in an override
// of {@link Recognizer//action} when the grammar is compiled.
//
// This class may represent embedded actions created with the {...}
// syntax in ANTLR 4, as well as actions created for lexer commands where the
// command argument could not be evaluated when the grammar was compiled.
// Constructs a custom lexer action with the specified rule and action
// indexes.
//
// @param ruleIndex The rule index to use for calls to
// {@link Recognizer//action}.
// @param actionIndex The action index to use for calls to
// {@link Recognizer//action}.
function LexerCustomAction(ruleIndex, actionIndex) {
LexerAction.call(this, LexerActionType.CUSTOM);
this.ruleIndex = ruleIndex;
this.actionIndex = actionIndex;
this.isPositionDependent = true;
return this;
}
LexerCustomAction.prototype = Object.create(LexerAction.prototype);
LexerCustomAction.prototype.constructor = LexerCustomAction;
// Custom actions are implemented by calling {@link Lexer//action} with the
// appropriate rule and action indexes.
LexerCustomAction.prototype.execute = function(lexer) {
lexer.action(null, this.ruleIndex, this.actionIndex);
};
LexerCustomAction.prototype.hashString = function() {
return "" + this.actionType + this.ruleIndex + this.actionIndex;
};
LexerCustomAction.prototype.equals = function(other) {
if (this === other) {
return true;
} else if (! (other instanceof LexerCustomAction)) {
return false;
} else {
return this.ruleIndex === other.ruleIndex && this.actionIndex === other.actionIndex;
}
};
// Implements the {@code channel} lexer action by calling
// {@link Lexer//setChannel} with the assigned channel.
// Constructs a new {@code channel} action with the specified channel value.
// @param channel The channel value to pass to {@link Lexer//setChannel}.
function LexerChannelAction(channel) {
LexerAction.call(this, LexerActionType.CHANNEL);
this.channel = channel;
return this;
}
LexerChannelAction.prototype = Object.create(LexerAction.prototype);
LexerChannelAction.prototype.constructor = LexerChannelAction;
// This action is implemented by calling {@link Lexer//setChannel} with the
// value provided by {@link //getChannel}.
LexerChannelAction.prototype.execute = function(lexer) {
lexer._channel = this.channel;
};
LexerChannelAction.prototype.hashString = function() {
return "" + this.actionType + this.channel;
};
LexerChannelAction.prototype.equals = function(other) {
if (this === other) {
return true;
} else if (! (other instanceof LexerChannelAction)) {
return false;
} else {
return this.channel === other.channel;
}
};
LexerChannelAction.prototype.toString = function() {
return "channel(" + this.channel + ")";
};
// This implementation of {@link LexerAction} is used for tracking input offsets
// for position-dependent actions within a {@link LexerActionExecutor}.
//
// This action is not serialized as part of the ATN, and is only required for
// position-dependent lexer actions which appear at a location other than the
// end of a rule. For more information about DFA optimizations employed for
// lexer actions, see {@link LexerActionExecutor//append} and
// {@link LexerActionExecutor//fixOffsetBeforeMatch}.
// Constructs a new indexed custom action by associating a character offset
// with a {@link LexerAction}.
//
// Note: This class is only required for lexer actions for which
// {@link LexerAction//isPositionDependent} returns {@code true}.
//
// @param offset The offset into the input {@link CharStream}, relative to
// the token start index, at which the specified lexer action should be
// executed.
// @param action The lexer action to execute at a particular offset in the
// input {@link CharStream}.
function LexerIndexedCustomAction(offset, action) {
LexerAction.call(this, action.actionType);
this.offset = offset;
this.action = action;
this.isPositionDependent = true;
return this;
}
LexerIndexedCustomAction.prototype = Object.create(LexerAction.prototype);
LexerIndexedCustomAction.prototype.constructor = LexerIndexedCustomAction;
// This method calls {@link //execute} on the result of {@link //getAction}
// using the provided {@code lexer}.
LexerIndexedCustomAction.prototype.execute = function(lexer) {
// assume the input stream position was properly set by the calling code
this.action.execute(lexer);
};
LexerIndexedCustomAction.prototype.hashString = function() {
return "" + this.actionType + this.offset + this.action;
};
LexerIndexedCustomAction.prototype.equals = function(other) {
if (this === other) {
return true;
} else if (! (other instanceof LexerIndexedCustomAction)) {
return false;
} else {
return this.offset === other.offset && this.action === other.action;
}
};
exports.LexerActionType = LexerActionType;
exports.LexerSkipAction = LexerSkipAction;
exports.LexerChannelAction = LexerChannelAction;
exports.LexerCustomAction = LexerCustomAction;
exports.LexerIndexedCustomAction = LexerIndexedCustomAction;
exports.LexerMoreAction = LexerMoreAction;
exports.LexerTypeAction = LexerTypeAction;
exports.LexerPushModeAction = LexerPushModeAction;
exports.LexerPopModeAction = LexerPopModeAction;
exports.LexerModeAction = LexerModeAction;