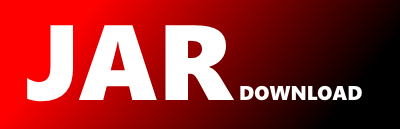
yale.compiler.compiler.0.9.12.source-code.CSSLexer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of compiler Show documentation
Show all versions of compiler Show documentation
The Apache Royale Compiler
The newest version!
// $ANTLR 3.5.2 CSS.g
package org.apache.royale.compiler.internal.css;
import org.apache.royale.compiler.problems.CSSParserProblem;
import org.apache.royale.compiler.problems.ICompilerProblem;
import org.antlr.runtime.*;
import java.util.Stack;
import java.util.List;
import java.util.ArrayList;
@SuppressWarnings("all")
public class CSSLexer extends Lexer {
public static final int EOF=-1;
public static final int T__81=81;
public static final int ALPHA_VALUE=4;
public static final int ARGUMENTS=5;
public static final int AT_FONT_FACE=6;
public static final int AT_KEYFRAMES=7;
public static final int AT_MEDIA=8;
public static final int AT_NAMESPACE=9;
public static final int AT_WEBKIT_KEYFRAMES=10;
public static final int BEGINS_WITH=11;
public static final int BLOCK_END=12;
public static final int BLOCK_OPEN=13;
public static final int CALC=14;
public static final int CHILD=15;
public static final int CLASS_REFERENCE=16;
public static final int COLON=17;
public static final int COMMA=18;
public static final int COMMENT=19;
public static final int CONTAINS=20;
public static final int DASHED_ID=21;
public static final int DIGIT=22;
public static final int DOT=23;
public static final int DOUBLE_COLON=24;
public static final int EMBED=25;
public static final int ENDS_WITH=26;
public static final int EQUALS=27;
public static final int ESCAPED_HEX=28;
public static final int FORMAT=29;
public static final int FUNCTIONS=30;
public static final int HASH_WORD=31;
public static final int HEX_DIGIT=32;
public static final int HREFLANG_MATCH=33;
public static final int ID=34;
public static final int IMPORTANT=35;
public static final int I_ARRAY=36;
public static final int I_CHILD_SELECTOR=37;
public static final int I_DECL=38;
public static final int I_MEDIUM_CONDITIONS=39;
public static final int I_MULTIVALUE=40;
public static final int I_PRECEDED_SELECTOR=41;
public static final int I_RULE=42;
public static final int I_RULES=43;
public static final int I_SELECTOR=44;
public static final int I_SELECTOR_GROUP=45;
public static final int I_SIBLING_SELECTOR=46;
public static final int I_SIMPLE_SELECTOR=47;
public static final int LETTER=48;
public static final int LIST_MATCH=49;
public static final int LOCAL=50;
public static final int MATRIX3D_VALUE=51;
public static final int MATRIX_VALUE=52;
public static final int NOT=53;
public static final int NULL=54;
public static final int NUMBER=55;
public static final int NUMBER_WITH_PERCENT=56;
public static final int NUMBER_WITH_UNIT=57;
public static final int ONLY=58;
public static final int OPERATOR=59;
public static final int PERCENT=60;
public static final int PIPE=61;
public static final int PRECEDED=62;
public static final int PROPERTY_REFERENCE=63;
public static final int RECT_VALUE=64;
public static final int RGB=65;
public static final int RGBA=66;
public static final int ROTATE_VALUE=67;
public static final int SCALE=68;
public static final int SCALE_VALUE=69;
public static final int SEMI_COLONS=70;
public static final int SQUARE_END=71;
public static final int SQUARE_OPEN=72;
public static final int STAR=73;
public static final int STRING=74;
public static final int STRING_QUOTE=75;
public static final int TILDE=76;
public static final int TRANSLATE3D_VALUE=77;
public static final int URL=78;
public static final int VAR=79;
public static final int WS=80;
/**
* Lexer problems.
*/
protected List problems = new ArrayList();
/**
* Collect lexer problems.
*/
@Override
public void displayRecognitionError(String[] tokenNames, RecognitionException e)
{
problems.add(CSSParserProblem.create(this, tokenNames, e));
}
// delegates
// delegators
public Lexer[] getDelegates() {
return new Lexer[] {};
}
public CSSLexer() {}
public CSSLexer(CharStream input) {
this(input, new RecognizerSharedState());
}
public CSSLexer(CharStream input, RecognizerSharedState state) {
super(input,state);
}
@Override public String getGrammarFileName() { return "CSS.g"; }
// $ANTLR start "T__81"
public final void mT__81() throws RecognitionException {
try {
int _type = T__81;
int _channel = DEFAULT_TOKEN_CHANNEL;
// CSS.g:30:7: ( 'and' )
// CSS.g:30:9: 'and'
{
match("and");
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "T__81"
// $ANTLR start "BEGINS_WITH"
public final void mBEGINS_WITH() throws RecognitionException {
try {
int _type = BEGINS_WITH;
int _channel = DEFAULT_TOKEN_CHANNEL;
// CSS.g:546:13: ( '^=' )
// CSS.g:546:15: '^='
{
match("^=");
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "BEGINS_WITH"
// $ANTLR start "ENDS_WITH"
public final void mENDS_WITH() throws RecognitionException {
try {
int _type = ENDS_WITH;
int _channel = DEFAULT_TOKEN_CHANNEL;
// CSS.g:547:11: ( '$=' )
// CSS.g:547:13: '$='
{
match("$=");
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "ENDS_WITH"
// $ANTLR start "CONTAINS"
public final void mCONTAINS() throws RecognitionException {
try {
int _type = CONTAINS;
int _channel = DEFAULT_TOKEN_CHANNEL;
// CSS.g:548:10: ( '*=' )
// CSS.g:548:12: '*='
{
match("*=");
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "CONTAINS"
// $ANTLR start "LIST_MATCH"
public final void mLIST_MATCH() throws RecognitionException {
try {
int _type = LIST_MATCH;
int _channel = DEFAULT_TOKEN_CHANNEL;
// CSS.g:549:12: ( '~=' )
// CSS.g:549:14: '~='
{
match("~=");
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "LIST_MATCH"
// $ANTLR start "HREFLANG_MATCH"
public final void mHREFLANG_MATCH() throws RecognitionException {
try {
int _type = HREFLANG_MATCH;
int _channel = DEFAULT_TOKEN_CHANNEL;
// CSS.g:550:16: ( '|=' )
// CSS.g:550:18: '|='
{
match("|=");
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "HREFLANG_MATCH"
// $ANTLR start "BLOCK_OPEN"
public final void mBLOCK_OPEN() throws RecognitionException {
try {
int _type = BLOCK_OPEN;
int _channel = DEFAULT_TOKEN_CHANNEL;
// CSS.g:551:12: ( '{' )
// CSS.g:551:14: '{'
{
match('{');
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "BLOCK_OPEN"
// $ANTLR start "BLOCK_END"
public final void mBLOCK_END() throws RecognitionException {
try {
int _type = BLOCK_END;
int _channel = DEFAULT_TOKEN_CHANNEL;
// CSS.g:552:11: ( '}' )
// CSS.g:552:14: '}'
{
match('}');
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "BLOCK_END"
// $ANTLR start "SQUARE_OPEN"
public final void mSQUARE_OPEN() throws RecognitionException {
try {
int _type = SQUARE_OPEN;
int _channel = DEFAULT_TOKEN_CHANNEL;
// CSS.g:553:13: ( '[' )
// CSS.g:553:15: '['
{
match('[');
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "SQUARE_OPEN"
// $ANTLR start "SQUARE_END"
public final void mSQUARE_END() throws RecognitionException {
try {
int _type = SQUARE_END;
int _channel = DEFAULT_TOKEN_CHANNEL;
// CSS.g:554:12: ( ']' )
// CSS.g:554:15: ']'
{
match(']');
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "SQUARE_END"
// $ANTLR start "COMMA"
public final void mCOMMA() throws RecognitionException {
try {
int _type = COMMA;
int _channel = DEFAULT_TOKEN_CHANNEL;
// CSS.g:555:7: ( ',' )
// CSS.g:555:9: ','
{
match(',');
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "COMMA"
// $ANTLR start "PERCENT"
public final void mPERCENT() throws RecognitionException {
try {
int _type = PERCENT;
int _channel = DEFAULT_TOKEN_CHANNEL;
// CSS.g:556:9: ( '%' )
// CSS.g:556:11: '%'
{
match('%');
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "PERCENT"
// $ANTLR start "PIPE"
public final void mPIPE() throws RecognitionException {
try {
int _type = PIPE;
int _channel = DEFAULT_TOKEN_CHANNEL;
// CSS.g:557:6: ( '|' )
// CSS.g:557:8: '|'
{
match('|');
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "PIPE"
// $ANTLR start "STAR"
public final void mSTAR() throws RecognitionException {
try {
int _type = STAR;
int _channel = DEFAULT_TOKEN_CHANNEL;
// CSS.g:558:6: ( '*' )
// CSS.g:558:8: '*'
{
match('*');
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "STAR"
// $ANTLR start "TILDE"
public final void mTILDE() throws RecognitionException {
try {
int _type = TILDE;
int _channel = DEFAULT_TOKEN_CHANNEL;
// CSS.g:559:7: ( '~' )
// CSS.g:559:9: '~'
{
match('~');
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "TILDE"
// $ANTLR start "DOT"
public final void mDOT() throws RecognitionException {
try {
int _type = DOT;
int _channel = DEFAULT_TOKEN_CHANNEL;
// CSS.g:560:5: ( '.' )
// CSS.g:560:7: '.'
{
match('.');
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "DOT"
// $ANTLR start "EQUALS"
public final void mEQUALS() throws RecognitionException {
try {
int _type = EQUALS;
int _channel = DEFAULT_TOKEN_CHANNEL;
// CSS.g:561:7: ( '=' )
// CSS.g:561:9: '='
{
match('=');
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "EQUALS"
// $ANTLR start "AT_NAMESPACE"
public final void mAT_NAMESPACE() throws RecognitionException {
try {
int _type = AT_NAMESPACE;
int _channel = DEFAULT_TOKEN_CHANNEL;
// CSS.g:562:14: ( '@namespace' )
// CSS.g:562:16: '@namespace'
{
match("@namespace");
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "AT_NAMESPACE"
// $ANTLR start "AT_MEDIA"
public final void mAT_MEDIA() throws RecognitionException {
try {
int _type = AT_MEDIA;
int _channel = DEFAULT_TOKEN_CHANNEL;
// CSS.g:563:10: ( '@media' )
// CSS.g:563:12: '@media'
{
match("@media");
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "AT_MEDIA"
// $ANTLR start "AT_KEYFRAMES"
public final void mAT_KEYFRAMES() throws RecognitionException {
try {
int _type = AT_KEYFRAMES;
int _channel = DEFAULT_TOKEN_CHANNEL;
// CSS.g:564:14: ( '@keyframes' )
// CSS.g:564:16: '@keyframes'
{
match("@keyframes");
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "AT_KEYFRAMES"
// $ANTLR start "AT_WEBKIT_KEYFRAMES"
public final void mAT_WEBKIT_KEYFRAMES() throws RecognitionException {
try {
int _type = AT_WEBKIT_KEYFRAMES;
int _channel = DEFAULT_TOKEN_CHANNEL;
// CSS.g:565:21: ( '@-webkit-keyframes' )
// CSS.g:565:23: '@-webkit-keyframes'
{
match("@-webkit-keyframes");
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "AT_WEBKIT_KEYFRAMES"
// $ANTLR start "DOUBLE_COLON"
public final void mDOUBLE_COLON() throws RecognitionException {
try {
int _type = DOUBLE_COLON;
int _channel = DEFAULT_TOKEN_CHANNEL;
// CSS.g:566:14: ( '::' )
// CSS.g:566:16: '::'
{
match("::");
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "DOUBLE_COLON"
// $ANTLR start "COLON"
public final void mCOLON() throws RecognitionException {
try {
int _type = COLON;
int _channel = DEFAULT_TOKEN_CHANNEL;
// CSS.g:567:7: ( ':' )
// CSS.g:567:9: ':'
{
match(':');
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "COLON"
// $ANTLR start "AT_FONT_FACE"
public final void mAT_FONT_FACE() throws RecognitionException {
try {
int _type = AT_FONT_FACE;
int _channel = DEFAULT_TOKEN_CHANNEL;
// CSS.g:568:14: ( '@font-face' )
// CSS.g:568:16: '@font-face'
{
match("@font-face");
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "AT_FONT_FACE"
// $ANTLR start "CLASS_REFERENCE"
public final void mCLASS_REFERENCE() throws RecognitionException {
try {
int _type = CLASS_REFERENCE;
int _channel = DEFAULT_TOKEN_CHANNEL;
// CSS.g:569:17: ( 'ClassReference' )
// CSS.g:569:19: 'ClassReference'
{
match("ClassReference");
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "CLASS_REFERENCE"
// $ANTLR start "PROPERTY_REFERENCE"
public final void mPROPERTY_REFERENCE() throws RecognitionException {
try {
int _type = PROPERTY_REFERENCE;
int _channel = DEFAULT_TOKEN_CHANNEL;
// CSS.g:570:20: ( 'PropertyReference' )
// CSS.g:570:22: 'PropertyReference'
{
match("PropertyReference");
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "PROPERTY_REFERENCE"
// $ANTLR start "IMPORTANT"
public final void mIMPORTANT() throws RecognitionException {
try {
int _type = IMPORTANT;
int _channel = DEFAULT_TOKEN_CHANNEL;
// CSS.g:571:11: ( '!important' )
// CSS.g:571:13: '!important'
{
match("!important");
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "IMPORTANT"
// $ANTLR start "EMBED"
public final void mEMBED() throws RecognitionException {
try {
int _type = EMBED;
int _channel = DEFAULT_TOKEN_CHANNEL;
// CSS.g:572:7: ( 'Embed' )
// CSS.g:572:9: 'Embed'
{
match("Embed");
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "EMBED"
// $ANTLR start "URL"
public final void mURL() throws RecognitionException {
try {
int _type = URL;
int _channel = DEFAULT_TOKEN_CHANNEL;
// CSS.g:573:5: ( 'url' )
// CSS.g:573:7: 'url'
{
match("url");
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "URL"
// $ANTLR start "FORMAT"
public final void mFORMAT() throws RecognitionException {
try {
int _type = FORMAT;
int _channel = DEFAULT_TOKEN_CHANNEL;
// CSS.g:574:8: ( 'format' )
// CSS.g:574:10: 'format'
{
match("format");
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "FORMAT"
// $ANTLR start "LOCAL"
public final void mLOCAL() throws RecognitionException {
try {
int _type = LOCAL;
int _channel = DEFAULT_TOKEN_CHANNEL;
// CSS.g:575:7: ( 'local' )
// CSS.g:575:9: 'local'
{
match("local");
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "LOCAL"
// $ANTLR start "CALC"
public final void mCALC() throws RecognitionException {
try {
int _type = CALC;
int _channel = DEFAULT_TOKEN_CHANNEL;
// CSS.g:576:6: ( 'calc' )
// CSS.g:576:8: 'calc'
{
match("calc");
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "CALC"
// $ANTLR start "SCALE"
public final void mSCALE() throws RecognitionException {
try {
int _type = SCALE;
int _channel = DEFAULT_TOKEN_CHANNEL;
// CSS.g:577:7: ( 'scale' )
// CSS.g:577:9: 'scale'
{
match("scale");
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "SCALE"
// $ANTLR start "VAR"
public final void mVAR() throws RecognitionException {
try {
int _type = VAR;
int _channel = DEFAULT_TOKEN_CHANNEL;
// CSS.g:578:5: ( 'var' )
// CSS.g:578:7: 'var'
{
match("var");
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "VAR"
// $ANTLR start "NULL"
public final void mNULL() throws RecognitionException {
try {
int _type = NULL;
int _channel = DEFAULT_TOKEN_CHANNEL;
// CSS.g:579:6: ( 'null' )
// CSS.g:579:8: 'null'
{
match("null");
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "NULL"
// $ANTLR start "ONLY"
public final void mONLY() throws RecognitionException {
try {
int _type = ONLY;
int _channel = DEFAULT_TOKEN_CHANNEL;
// CSS.g:580:6: ( 'only' )
// CSS.g:580:8: 'only'
{
match("only");
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "ONLY"
// $ANTLR start "CHILD"
public final void mCHILD() throws RecognitionException {
try {
int _type = CHILD;
int _channel = DEFAULT_TOKEN_CHANNEL;
// CSS.g:581:7: ( '>' )
// CSS.g:581:9: '>'
{
match('>');
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "CHILD"
// $ANTLR start "PRECEDED"
public final void mPRECEDED() throws RecognitionException {
try {
int _type = PRECEDED;
int _channel = DEFAULT_TOKEN_CHANNEL;
// CSS.g:582:10: ( '+' )
// CSS.g:582:12: '+'
{
match('+');
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "PRECEDED"
// $ANTLR start "FUNCTIONS"
public final void mFUNCTIONS() throws RecognitionException {
try {
int _type = FUNCTIONS;
int _channel = DEFAULT_TOKEN_CHANNEL;
// CSS.g:583:11: ( '-moz-linear-gradient' | '-webkit-linear-gradient' | 'linear-gradient' | 'radial-gradient' | 'conic-gradient' | 'repeating-linear-gradient' | 'repeating-radial-gradient' | 'repeating-conic-gradient' | 'progid:DXImageTransform.Microsoft.gradient' | 'translateX' | 'translateY' | 'translateZ' | 'translate' | 'rotateX' | 'rotateY' | 'rotateZ' | 'scaleX' | 'scaleY' | 'scaleZ' | 'skewX' | 'skewY' | 'skew' | 'perspective' | 'blur' | 'brightness' | 'contrast' | 'drop-shadow' | 'hue-rotate' | 'invert' | 'saturate' | 'sepia' )
int alt1=31;
alt1 = dfa1.predict(input);
switch (alt1) {
case 1 :
// CSS.g:583:13: '-moz-linear-gradient'
{
match("-moz-linear-gradient");
}
break;
case 2 :
// CSS.g:584:13: '-webkit-linear-gradient'
{
match("-webkit-linear-gradient");
}
break;
case 3 :
// CSS.g:585:13: 'linear-gradient'
{
match("linear-gradient");
}
break;
case 4 :
// CSS.g:586:13: 'radial-gradient'
{
match("radial-gradient");
}
break;
case 5 :
// CSS.g:587:13: 'conic-gradient'
{
match("conic-gradient");
}
break;
case 6 :
// CSS.g:588:13: 'repeating-linear-gradient'
{
match("repeating-linear-gradient");
}
break;
case 7 :
// CSS.g:589:13: 'repeating-radial-gradient'
{
match("repeating-radial-gradient");
}
break;
case 8 :
// CSS.g:590:13: 'repeating-conic-gradient'
{
match("repeating-conic-gradient");
}
break;
case 9 :
// CSS.g:591:13: 'progid:DXImageTransform.Microsoft.gradient'
{
match("progid:DXImageTransform.Microsoft.gradient");
}
break;
case 10 :
// CSS.g:592:13: 'translateX'
{
match("translateX");
}
break;
case 11 :
// CSS.g:593:13: 'translateY'
{
match("translateY");
}
break;
case 12 :
// CSS.g:594:13: 'translateZ'
{
match("translateZ");
}
break;
case 13 :
// CSS.g:595:13: 'translate'
{
match("translate");
}
break;
case 14 :
// CSS.g:596:13: 'rotateX'
{
match("rotateX");
}
break;
case 15 :
// CSS.g:597:13: 'rotateY'
{
match("rotateY");
}
break;
case 16 :
// CSS.g:598:13: 'rotateZ'
{
match("rotateZ");
}
break;
case 17 :
// CSS.g:599:13: 'scaleX'
{
match("scaleX");
}
break;
case 18 :
// CSS.g:600:13: 'scaleY'
{
match("scaleY");
}
break;
case 19 :
// CSS.g:601:13: 'scaleZ'
{
match("scaleZ");
}
break;
case 20 :
// CSS.g:602:13: 'skewX'
{
match("skewX");
}
break;
case 21 :
// CSS.g:603:13: 'skewY'
{
match("skewY");
}
break;
case 22 :
// CSS.g:604:13: 'skew'
{
match("skew");
}
break;
case 23 :
// CSS.g:605:13: 'perspective'
{
match("perspective");
}
break;
case 24 :
// CSS.g:606:13: 'blur'
{
match("blur");
}
break;
case 25 :
// CSS.g:607:13: 'brightness'
{
match("brightness");
}
break;
case 26 :
// CSS.g:608:13: 'contrast'
{
match("contrast");
}
break;
case 27 :
// CSS.g:609:13: 'drop-shadow'
{
match("drop-shadow");
}
break;
case 28 :
// CSS.g:610:13: 'hue-rotate'
{
match("hue-rotate");
}
break;
case 29 :
// CSS.g:611:13: 'invert'
{
match("invert");
}
break;
case 30 :
// CSS.g:612:13: 'saturate'
{
match("saturate");
}
break;
case 31 :
// CSS.g:613:13: 'sepia'
{
match("sepia");
}
break;
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "FUNCTIONS"
// $ANTLR start "NOT"
public final void mNOT() throws RecognitionException {
try {
int _type = NOT;
int _channel = DEFAULT_TOKEN_CHANNEL;
// CSS.g:628:5: ( 'not' )
// CSS.g:628:8: 'not'
{
match("not");
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "NOT"
// $ANTLR start "ALPHA_VALUE"
public final void mALPHA_VALUE() throws RecognitionException {
try {
int _type = ALPHA_VALUE;
int _channel = DEFAULT_TOKEN_CHANNEL;
// CSS.g:631:13: ( 'alpha(' ( options {greedy=false; } : . )* ')' )
// CSS.g:631:16: 'alpha(' ( options {greedy=false; } : . )* ')'
{
match("alpha(");
// CSS.g:631:25: ( options {greedy=false; } : . )*
loop2:
while (true) {
int alt2=2;
int LA2_0 = input.LA(1);
if ( (LA2_0==')') ) {
alt2=2;
}
else if ( ((LA2_0 >= '\u0000' && LA2_0 <= '(')||(LA2_0 >= '*' && LA2_0 <= '\uFFFF')) ) {
alt2=1;
}
switch (alt2) {
case 1 :
// CSS.g:631:52: .
{
matchAny();
}
break;
default :
break loop2;
}
}
match(')');
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "ALPHA_VALUE"
// $ANTLR start "ROTATE_VALUE"
public final void mROTATE_VALUE() throws RecognitionException {
try {
int _type = ROTATE_VALUE;
int _channel = DEFAULT_TOKEN_CHANNEL;
// CSS.g:636:14: ( 'rotate(' ( options {greedy=false; } : . )* ')' )
// CSS.g:636:17: 'rotate(' ( options {greedy=false; } : . )* ')'
{
match("rotate(");
// CSS.g:636:27: ( options {greedy=false; } : . )*
loop3:
while (true) {
int alt3=2;
int LA3_0 = input.LA(1);
if ( (LA3_0==')') ) {
alt3=2;
}
else if ( ((LA3_0 >= '\u0000' && LA3_0 <= '(')||(LA3_0 >= '*' && LA3_0 <= '\uFFFF')) ) {
alt3=1;
}
switch (alt3) {
case 1 :
// CSS.g:636:54: .
{
matchAny();
}
break;
default :
break loop3;
}
}
match(')');
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "ROTATE_VALUE"
// $ANTLR start "SCALE_VALUE"
public final void mSCALE_VALUE() throws RecognitionException {
try {
int _type = SCALE_VALUE;
int _channel = DEFAULT_TOKEN_CHANNEL;
// CSS.g:641:13: ( 'scale(' ( options {greedy=false; } : . )* ')' )
// CSS.g:641:16: 'scale(' ( options {greedy=false; } : . )* ')'
{
match("scale(");
// CSS.g:641:25: ( options {greedy=false; } : . )*
loop4:
while (true) {
int alt4=2;
int LA4_0 = input.LA(1);
if ( (LA4_0==')') ) {
alt4=2;
}
else if ( ((LA4_0 >= '\u0000' && LA4_0 <= '(')||(LA4_0 >= '*' && LA4_0 <= '\uFFFF')) ) {
alt4=1;
}
switch (alt4) {
case 1 :
// CSS.g:641:52: .
{
matchAny();
}
break;
default :
break loop4;
}
}
match(')');
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "SCALE_VALUE"
// $ANTLR start "TRANSLATE3D_VALUE"
public final void mTRANSLATE3D_VALUE() throws RecognitionException {
try {
int _type = TRANSLATE3D_VALUE;
int _channel = DEFAULT_TOKEN_CHANNEL;
// CSS.g:646:19: ( 'translate3d(' ( options {greedy=false; } : . )* ')' )
// CSS.g:646:22: 'translate3d(' ( options {greedy=false; } : . )* ')'
{
match("translate3d(");
// CSS.g:646:37: ( options {greedy=false; } : . )*
loop5:
while (true) {
int alt5=2;
int LA5_0 = input.LA(1);
if ( (LA5_0==')') ) {
alt5=2;
}
else if ( ((LA5_0 >= '\u0000' && LA5_0 <= '(')||(LA5_0 >= '*' && LA5_0 <= '\uFFFF')) ) {
alt5=1;
}
switch (alt5) {
case 1 :
// CSS.g:646:64: .
{
matchAny();
}
break;
default :
break loop5;
}
}
match(')');
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "TRANSLATE3D_VALUE"
// $ANTLR start "RECT_VALUE"
public final void mRECT_VALUE() throws RecognitionException {
try {
int _type = RECT_VALUE;
int _channel = DEFAULT_TOKEN_CHANNEL;
// CSS.g:651:12: ( 'rect(' ( options {greedy=false; } : . )* ')' )
// CSS.g:651:15: 'rect(' ( options {greedy=false; } : . )* ')'
{
match("rect(");
// CSS.g:651:23: ( options {greedy=false; } : . )*
loop6:
while (true) {
int alt6=2;
int LA6_0 = input.LA(1);
if ( (LA6_0==')') ) {
alt6=2;
}
else if ( ((LA6_0 >= '\u0000' && LA6_0 <= '(')||(LA6_0 >= '*' && LA6_0 <= '\uFFFF')) ) {
alt6=1;
}
switch (alt6) {
case 1 :
// CSS.g:651:50: .
{
matchAny();
}
break;
default :
break loop6;
}
}
match(')');
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "RECT_VALUE"
// $ANTLR start "MATRIX_VALUE"
public final void mMATRIX_VALUE() throws RecognitionException {
try {
int _type = MATRIX_VALUE;
int _channel = DEFAULT_TOKEN_CHANNEL;
// CSS.g:656:14: ( 'matrix(' ( ( WS )* NUMBER ( WS )* ) ',' ( ( WS )* NUMBER ( WS )* ) ',' ( ( WS )* NUMBER ( WS )* ) ',' ( ( WS )* NUMBER ( WS )* ) ',' ( ( WS )* NUMBER ( WS )* ) ',' ( ( WS )* NUMBER ( WS )* ) ')' )
// CSS.g:656:17: 'matrix(' ( ( WS )* NUMBER ( WS )* ) ',' ( ( WS )* NUMBER ( WS )* ) ',' ( ( WS )* NUMBER ( WS )* ) ',' ( ( WS )* NUMBER ( WS )* ) ',' ( ( WS )* NUMBER ( WS )* ) ',' ( ( WS )* NUMBER ( WS )* ) ')'
{
match("matrix(");
// CSS.g:656:28: ( ( WS )* NUMBER ( WS )* )
// CSS.g:656:30: ( WS )* NUMBER ( WS )*
{
// CSS.g:656:30: ( WS )*
loop7:
while (true) {
int alt7=2;
int LA7_0 = input.LA(1);
if ( ((LA7_0 >= '\t' && LA7_0 <= '\n')||LA7_0=='\r'||LA7_0==' ') ) {
alt7=1;
}
switch (alt7) {
case 1 :
// CSS.g:656:30: WS
{
mWS();
}
break;
default :
break loop7;
}
}
mNUMBER();
// CSS.g:656:41: ( WS )*
loop8:
while (true) {
int alt8=2;
int LA8_0 = input.LA(1);
if ( ((LA8_0 >= '\t' && LA8_0 <= '\n')||LA8_0=='\r'||LA8_0==' ') ) {
alt8=1;
}
switch (alt8) {
case 1 :
// CSS.g:656:41: WS
{
mWS();
}
break;
default :
break loop8;
}
}
}
match(',');
// CSS.g:657:17: ( ( WS )* NUMBER ( WS )* )
// CSS.g:657:19: ( WS )* NUMBER ( WS )*
{
// CSS.g:657:19: ( WS )*
loop9:
while (true) {
int alt9=2;
int LA9_0 = input.LA(1);
if ( ((LA9_0 >= '\t' && LA9_0 <= '\n')||LA9_0=='\r'||LA9_0==' ') ) {
alt9=1;
}
switch (alt9) {
case 1 :
// CSS.g:657:19: WS
{
mWS();
}
break;
default :
break loop9;
}
}
mNUMBER();
// CSS.g:657:30: ( WS )*
loop10:
while (true) {
int alt10=2;
int LA10_0 = input.LA(1);
if ( ((LA10_0 >= '\t' && LA10_0 <= '\n')||LA10_0=='\r'||LA10_0==' ') ) {
alt10=1;
}
switch (alt10) {
case 1 :
// CSS.g:657:30: WS
{
mWS();
}
break;
default :
break loop10;
}
}
}
match(',');
// CSS.g:658:17: ( ( WS )* NUMBER ( WS )* )
// CSS.g:658:19: ( WS )* NUMBER ( WS )*
{
// CSS.g:658:19: ( WS )*
loop11:
while (true) {
int alt11=2;
int LA11_0 = input.LA(1);
if ( ((LA11_0 >= '\t' && LA11_0 <= '\n')||LA11_0=='\r'||LA11_0==' ') ) {
alt11=1;
}
switch (alt11) {
case 1 :
// CSS.g:658:19: WS
{
mWS();
}
break;
default :
break loop11;
}
}
mNUMBER();
// CSS.g:658:30: ( WS )*
loop12:
while (true) {
int alt12=2;
int LA12_0 = input.LA(1);
if ( ((LA12_0 >= '\t' && LA12_0 <= '\n')||LA12_0=='\r'||LA12_0==' ') ) {
alt12=1;
}
switch (alt12) {
case 1 :
// CSS.g:658:30: WS
{
mWS();
}
break;
default :
break loop12;
}
}
}
match(',');
// CSS.g:659:17: ( ( WS )* NUMBER ( WS )* )
// CSS.g:659:19: ( WS )* NUMBER ( WS )*
{
// CSS.g:659:19: ( WS )*
loop13:
while (true) {
int alt13=2;
int LA13_0 = input.LA(1);
if ( ((LA13_0 >= '\t' && LA13_0 <= '\n')||LA13_0=='\r'||LA13_0==' ') ) {
alt13=1;
}
switch (alt13) {
case 1 :
// CSS.g:659:19: WS
{
mWS();
}
break;
default :
break loop13;
}
}
mNUMBER();
// CSS.g:659:30: ( WS )*
loop14:
while (true) {
int alt14=2;
int LA14_0 = input.LA(1);
if ( ((LA14_0 >= '\t' && LA14_0 <= '\n')||LA14_0=='\r'||LA14_0==' ') ) {
alt14=1;
}
switch (alt14) {
case 1 :
// CSS.g:659:30: WS
{
mWS();
}
break;
default :
break loop14;
}
}
}
match(',');
// CSS.g:660:17: ( ( WS )* NUMBER ( WS )* )
// CSS.g:660:19: ( WS )* NUMBER ( WS )*
{
// CSS.g:660:19: ( WS )*
loop15:
while (true) {
int alt15=2;
int LA15_0 = input.LA(1);
if ( ((LA15_0 >= '\t' && LA15_0 <= '\n')||LA15_0=='\r'||LA15_0==' ') ) {
alt15=1;
}
switch (alt15) {
case 1 :
// CSS.g:660:19: WS
{
mWS();
}
break;
default :
break loop15;
}
}
mNUMBER();
// CSS.g:660:30: ( WS )*
loop16:
while (true) {
int alt16=2;
int LA16_0 = input.LA(1);
if ( ((LA16_0 >= '\t' && LA16_0 <= '\n')||LA16_0=='\r'||LA16_0==' ') ) {
alt16=1;
}
switch (alt16) {
case 1 :
// CSS.g:660:30: WS
{
mWS();
}
break;
default :
break loop16;
}
}
}
match(',');
// CSS.g:661:17: ( ( WS )* NUMBER ( WS )* )
// CSS.g:661:19: ( WS )* NUMBER ( WS )*
{
// CSS.g:661:19: ( WS )*
loop17:
while (true) {
int alt17=2;
int LA17_0 = input.LA(1);
if ( ((LA17_0 >= '\t' && LA17_0 <= '\n')||LA17_0=='\r'||LA17_0==' ') ) {
alt17=1;
}
switch (alt17) {
case 1 :
// CSS.g:661:19: WS
{
mWS();
}
break;
default :
break loop17;
}
}
mNUMBER();
// CSS.g:661:30: ( WS )*
loop18:
while (true) {
int alt18=2;
int LA18_0 = input.LA(1);
if ( ((LA18_0 >= '\t' && LA18_0 <= '\n')||LA18_0=='\r'||LA18_0==' ') ) {
alt18=1;
}
switch (alt18) {
case 1 :
// CSS.g:661:30: WS
{
mWS();
}
break;
default :
break loop18;
}
}
}
match(')');
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "MATRIX_VALUE"
// $ANTLR start "MATRIX3D_VALUE"
public final void mMATRIX3D_VALUE() throws RecognitionException {
try {
int _type = MATRIX3D_VALUE;
int _channel = DEFAULT_TOKEN_CHANNEL;
// CSS.g:668:16: ( 'matrix3d(' ( ( WS )* NUMBER ( WS )* ) ',' ( ( WS )* NUMBER ( WS )* ) ',' ( ( WS )* NUMBER ( WS )* ) ',' ( ( WS )* NUMBER ( WS )* ) ',' ( ( WS )* NUMBER ( WS )* ) ',' ( ( WS )* NUMBER ( WS )* ) ',' ( ( WS )* NUMBER ( WS )* ) ',' ( ( WS )* NUMBER ( WS )* ) ',' ( ( WS )* NUMBER ( WS )* ) ',' ( ( WS )* NUMBER ( WS )* ) ',' ( ( WS )* NUMBER ( WS )* ) ',' ( ( WS )* NUMBER ( WS )* ) ',' ( ( WS )* NUMBER ( WS )* ) ',' ( ( WS )* NUMBER ( WS )* ) ',' ( ( WS )* NUMBER ( WS )* ) ',' ( ( WS )* NUMBER ( WS )* ) ')' )
// CSS.g:668:19: 'matrix3d(' ( ( WS )* NUMBER ( WS )* ) ',' ( ( WS )* NUMBER ( WS )* ) ',' ( ( WS )* NUMBER ( WS )* ) ',' ( ( WS )* NUMBER ( WS )* ) ',' ( ( WS )* NUMBER ( WS )* ) ',' ( ( WS )* NUMBER ( WS )* ) ',' ( ( WS )* NUMBER ( WS )* ) ',' ( ( WS )* NUMBER ( WS )* ) ',' ( ( WS )* NUMBER ( WS )* ) ',' ( ( WS )* NUMBER ( WS )* ) ',' ( ( WS )* NUMBER ( WS )* ) ',' ( ( WS )* NUMBER ( WS )* ) ',' ( ( WS )* NUMBER ( WS )* ) ',' ( ( WS )* NUMBER ( WS )* ) ',' ( ( WS )* NUMBER ( WS )* ) ',' ( ( WS )* NUMBER ( WS )* ) ')'
{
match("matrix3d(");
// CSS.g:668:32: ( ( WS )* NUMBER ( WS )* )
// CSS.g:668:34: ( WS )* NUMBER ( WS )*
{
// CSS.g:668:34: ( WS )*
loop19:
while (true) {
int alt19=2;
int LA19_0 = input.LA(1);
if ( ((LA19_0 >= '\t' && LA19_0 <= '\n')||LA19_0=='\r'||LA19_0==' ') ) {
alt19=1;
}
switch (alt19) {
case 1 :
// CSS.g:668:34: WS
{
mWS();
}
break;
default :
break loop19;
}
}
mNUMBER();
// CSS.g:668:45: ( WS )*
loop20:
while (true) {
int alt20=2;
int LA20_0 = input.LA(1);
if ( ((LA20_0 >= '\t' && LA20_0 <= '\n')||LA20_0=='\r'||LA20_0==' ') ) {
alt20=1;
}
switch (alt20) {
case 1 :
// CSS.g:668:45: WS
{
mWS();
}
break;
default :
break loop20;
}
}
}
match(',');
// CSS.g:669:21: ( ( WS )* NUMBER ( WS )* )
// CSS.g:669:23: ( WS )* NUMBER ( WS )*
{
// CSS.g:669:23: ( WS )*
loop21:
while (true) {
int alt21=2;
int LA21_0 = input.LA(1);
if ( ((LA21_0 >= '\t' && LA21_0 <= '\n')||LA21_0=='\r'||LA21_0==' ') ) {
alt21=1;
}
switch (alt21) {
case 1 :
// CSS.g:669:23: WS
{
mWS();
}
break;
default :
break loop21;
}
}
mNUMBER();
// CSS.g:669:34: ( WS )*
loop22:
while (true) {
int alt22=2;
int LA22_0 = input.LA(1);
if ( ((LA22_0 >= '\t' && LA22_0 <= '\n')||LA22_0=='\r'||LA22_0==' ') ) {
alt22=1;
}
switch (alt22) {
case 1 :
// CSS.g:669:34: WS
{
mWS();
}
break;
default :
break loop22;
}
}
}
match(',');
// CSS.g:670:21: ( ( WS )* NUMBER ( WS )* )
// CSS.g:670:23: ( WS )* NUMBER ( WS )*
{
// CSS.g:670:23: ( WS )*
loop23:
while (true) {
int alt23=2;
int LA23_0 = input.LA(1);
if ( ((LA23_0 >= '\t' && LA23_0 <= '\n')||LA23_0=='\r'||LA23_0==' ') ) {
alt23=1;
}
switch (alt23) {
case 1 :
// CSS.g:670:23: WS
{
mWS();
}
break;
default :
break loop23;
}
}
mNUMBER();
// CSS.g:670:34: ( WS )*
loop24:
while (true) {
int alt24=2;
int LA24_0 = input.LA(1);
if ( ((LA24_0 >= '\t' && LA24_0 <= '\n')||LA24_0=='\r'||LA24_0==' ') ) {
alt24=1;
}
switch (alt24) {
case 1 :
// CSS.g:670:34: WS
{
mWS();
}
break;
default :
break loop24;
}
}
}
match(',');
// CSS.g:671:21: ( ( WS )* NUMBER ( WS )* )
// CSS.g:671:23: ( WS )* NUMBER ( WS )*
{
// CSS.g:671:23: ( WS )*
loop25:
while (true) {
int alt25=2;
int LA25_0 = input.LA(1);
if ( ((LA25_0 >= '\t' && LA25_0 <= '\n')||LA25_0=='\r'||LA25_0==' ') ) {
alt25=1;
}
switch (alt25) {
case 1 :
// CSS.g:671:23: WS
{
mWS();
}
break;
default :
break loop25;
}
}
mNUMBER();
// CSS.g:671:34: ( WS )*
loop26:
while (true) {
int alt26=2;
int LA26_0 = input.LA(1);
if ( ((LA26_0 >= '\t' && LA26_0 <= '\n')||LA26_0=='\r'||LA26_0==' ') ) {
alt26=1;
}
switch (alt26) {
case 1 :
// CSS.g:671:34: WS
{
mWS();
}
break;
default :
break loop26;
}
}
}
match(',');
// CSS.g:672:21: ( ( WS )* NUMBER ( WS )* )
// CSS.g:672:23: ( WS )* NUMBER ( WS )*
{
// CSS.g:672:23: ( WS )*
loop27:
while (true) {
int alt27=2;
int LA27_0 = input.LA(1);
if ( ((LA27_0 >= '\t' && LA27_0 <= '\n')||LA27_0=='\r'||LA27_0==' ') ) {
alt27=1;
}
switch (alt27) {
case 1 :
// CSS.g:672:23: WS
{
mWS();
}
break;
default :
break loop27;
}
}
mNUMBER();
// CSS.g:672:34: ( WS )*
loop28:
while (true) {
int alt28=2;
int LA28_0 = input.LA(1);
if ( ((LA28_0 >= '\t' && LA28_0 <= '\n')||LA28_0=='\r'||LA28_0==' ') ) {
alt28=1;
}
switch (alt28) {
case 1 :
// CSS.g:672:34: WS
{
mWS();
}
break;
default :
break loop28;
}
}
}
match(',');
// CSS.g:673:21: ( ( WS )* NUMBER ( WS )* )
// CSS.g:673:23: ( WS )* NUMBER ( WS )*
{
// CSS.g:673:23: ( WS )*
loop29:
while (true) {
int alt29=2;
int LA29_0 = input.LA(1);
if ( ((LA29_0 >= '\t' && LA29_0 <= '\n')||LA29_0=='\r'||LA29_0==' ') ) {
alt29=1;
}
switch (alt29) {
case 1 :
// CSS.g:673:23: WS
{
mWS();
}
break;
default :
break loop29;
}
}
mNUMBER();
// CSS.g:673:34: ( WS )*
loop30:
while (true) {
int alt30=2;
int LA30_0 = input.LA(1);
if ( ((LA30_0 >= '\t' && LA30_0 <= '\n')||LA30_0=='\r'||LA30_0==' ') ) {
alt30=1;
}
switch (alt30) {
case 1 :
// CSS.g:673:34: WS
{
mWS();
}
break;
default :
break loop30;
}
}
}
match(',');
// CSS.g:674:21: ( ( WS )* NUMBER ( WS )* )
// CSS.g:674:23: ( WS )* NUMBER ( WS )*
{
// CSS.g:674:23: ( WS )*
loop31:
while (true) {
int alt31=2;
int LA31_0 = input.LA(1);
if ( ((LA31_0 >= '\t' && LA31_0 <= '\n')||LA31_0=='\r'||LA31_0==' ') ) {
alt31=1;
}
switch (alt31) {
case 1 :
// CSS.g:674:23: WS
{
mWS();
}
break;
default :
break loop31;
}
}
mNUMBER();
// CSS.g:674:34: ( WS )*
loop32:
while (true) {
int alt32=2;
int LA32_0 = input.LA(1);
if ( ((LA32_0 >= '\t' && LA32_0 <= '\n')||LA32_0=='\r'||LA32_0==' ') ) {
alt32=1;
}
switch (alt32) {
case 1 :
// CSS.g:674:34: WS
{
mWS();
}
break;
default :
break loop32;
}
}
}
match(',');
// CSS.g:675:21: ( ( WS )* NUMBER ( WS )* )
// CSS.g:675:23: ( WS )* NUMBER ( WS )*
{
// CSS.g:675:23: ( WS )*
loop33:
while (true) {
int alt33=2;
int LA33_0 = input.LA(1);
if ( ((LA33_0 >= '\t' && LA33_0 <= '\n')||LA33_0=='\r'||LA33_0==' ') ) {
alt33=1;
}
switch (alt33) {
case 1 :
// CSS.g:675:23: WS
{
mWS();
}
break;
default :
break loop33;
}
}
mNUMBER();
// CSS.g:675:34: ( WS )*
loop34:
while (true) {
int alt34=2;
int LA34_0 = input.LA(1);
if ( ((LA34_0 >= '\t' && LA34_0 <= '\n')||LA34_0=='\r'||LA34_0==' ') ) {
alt34=1;
}
switch (alt34) {
case 1 :
// CSS.g:675:34: WS
{
mWS();
}
break;
default :
break loop34;
}
}
}
match(',');
// CSS.g:676:21: ( ( WS )* NUMBER ( WS )* )
// CSS.g:676:23: ( WS )* NUMBER ( WS )*
{
// CSS.g:676:23: ( WS )*
loop35:
while (true) {
int alt35=2;
int LA35_0 = input.LA(1);
if ( ((LA35_0 >= '\t' && LA35_0 <= '\n')||LA35_0=='\r'||LA35_0==' ') ) {
alt35=1;
}
switch (alt35) {
case 1 :
// CSS.g:676:23: WS
{
mWS();
}
break;
default :
break loop35;
}
}
mNUMBER();
// CSS.g:676:34: ( WS )*
loop36:
while (true) {
int alt36=2;
int LA36_0 = input.LA(1);
if ( ((LA36_0 >= '\t' && LA36_0 <= '\n')||LA36_0=='\r'||LA36_0==' ') ) {
alt36=1;
}
switch (alt36) {
case 1 :
// CSS.g:676:34: WS
{
mWS();
}
break;
default :
break loop36;
}
}
}
match(',');
// CSS.g:677:21: ( ( WS )* NUMBER ( WS )* )
// CSS.g:677:23: ( WS )* NUMBER ( WS )*
{
// CSS.g:677:23: ( WS )*
loop37:
while (true) {
int alt37=2;
int LA37_0 = input.LA(1);
if ( ((LA37_0 >= '\t' && LA37_0 <= '\n')||LA37_0=='\r'||LA37_0==' ') ) {
alt37=1;
}
switch (alt37) {
case 1 :
// CSS.g:677:23: WS
{
mWS();
}
break;
default :
break loop37;
}
}
mNUMBER();
// CSS.g:677:34: ( WS )*
loop38:
while (true) {
int alt38=2;
int LA38_0 = input.LA(1);
if ( ((LA38_0 >= '\t' && LA38_0 <= '\n')||LA38_0=='\r'||LA38_0==' ') ) {
alt38=1;
}
switch (alt38) {
case 1 :
// CSS.g:677:34: WS
{
mWS();
}
break;
default :
break loop38;
}
}
}
match(',');
// CSS.g:678:21: ( ( WS )* NUMBER ( WS )* )
// CSS.g:678:23: ( WS )* NUMBER ( WS )*
{
// CSS.g:678:23: ( WS )*
loop39:
while (true) {
int alt39=2;
int LA39_0 = input.LA(1);
if ( ((LA39_0 >= '\t' && LA39_0 <= '\n')||LA39_0=='\r'||LA39_0==' ') ) {
alt39=1;
}
switch (alt39) {
case 1 :
// CSS.g:678:23: WS
{
mWS();
}
break;
default :
break loop39;
}
}
mNUMBER();
// CSS.g:678:34: ( WS )*
loop40:
while (true) {
int alt40=2;
int LA40_0 = input.LA(1);
if ( ((LA40_0 >= '\t' && LA40_0 <= '\n')||LA40_0=='\r'||LA40_0==' ') ) {
alt40=1;
}
switch (alt40) {
case 1 :
// CSS.g:678:34: WS
{
mWS();
}
break;
default :
break loop40;
}
}
}
match(',');
// CSS.g:679:21: ( ( WS )* NUMBER ( WS )* )
// CSS.g:679:23: ( WS )* NUMBER ( WS )*
{
// CSS.g:679:23: ( WS )*
loop41:
while (true) {
int alt41=2;
int LA41_0 = input.LA(1);
if ( ((LA41_0 >= '\t' && LA41_0 <= '\n')||LA41_0=='\r'||LA41_0==' ') ) {
alt41=1;
}
switch (alt41) {
case 1 :
// CSS.g:679:23: WS
{
mWS();
}
break;
default :
break loop41;
}
}
mNUMBER();
// CSS.g:679:34: ( WS )*
loop42:
while (true) {
int alt42=2;
int LA42_0 = input.LA(1);
if ( ((LA42_0 >= '\t' && LA42_0 <= '\n')||LA42_0=='\r'||LA42_0==' ') ) {
alt42=1;
}
switch (alt42) {
case 1 :
// CSS.g:679:34: WS
{
mWS();
}
break;
default :
break loop42;
}
}
}
match(',');
// CSS.g:680:21: ( ( WS )* NUMBER ( WS )* )
// CSS.g:680:23: ( WS )* NUMBER ( WS )*
{
// CSS.g:680:23: ( WS )*
loop43:
while (true) {
int alt43=2;
int LA43_0 = input.LA(1);
if ( ((LA43_0 >= '\t' && LA43_0 <= '\n')||LA43_0=='\r'||LA43_0==' ') ) {
alt43=1;
}
switch (alt43) {
case 1 :
// CSS.g:680:23: WS
{
mWS();
}
break;
default :
break loop43;
}
}
mNUMBER();
// CSS.g:680:34: ( WS )*
loop44:
while (true) {
int alt44=2;
int LA44_0 = input.LA(1);
if ( ((LA44_0 >= '\t' && LA44_0 <= '\n')||LA44_0=='\r'||LA44_0==' ') ) {
alt44=1;
}
switch (alt44) {
case 1 :
// CSS.g:680:34: WS
{
mWS();
}
break;
default :
break loop44;
}
}
}
match(',');
// CSS.g:681:21: ( ( WS )* NUMBER ( WS )* )
// CSS.g:681:23: ( WS )* NUMBER ( WS )*
{
// CSS.g:681:23: ( WS )*
loop45:
while (true) {
int alt45=2;
int LA45_0 = input.LA(1);
if ( ((LA45_0 >= '\t' && LA45_0 <= '\n')||LA45_0=='\r'||LA45_0==' ') ) {
alt45=1;
}
switch (alt45) {
case 1 :
// CSS.g:681:23: WS
{
mWS();
}
break;
default :
break loop45;
}
}
mNUMBER();
// CSS.g:681:34: ( WS )*
loop46:
while (true) {
int alt46=2;
int LA46_0 = input.LA(1);
if ( ((LA46_0 >= '\t' && LA46_0 <= '\n')||LA46_0=='\r'||LA46_0==' ') ) {
alt46=1;
}
switch (alt46) {
case 1 :
// CSS.g:681:34: WS
{
mWS();
}
break;
default :
break loop46;
}
}
}
match(',');
// CSS.g:682:21: ( ( WS )* NUMBER ( WS )* )
// CSS.g:682:23: ( WS )* NUMBER ( WS )*
{
// CSS.g:682:23: ( WS )*
loop47:
while (true) {
int alt47=2;
int LA47_0 = input.LA(1);
if ( ((LA47_0 >= '\t' && LA47_0 <= '\n')||LA47_0=='\r'||LA47_0==' ') ) {
alt47=1;
}
switch (alt47) {
case 1 :
// CSS.g:682:23: WS
{
mWS();
}
break;
default :
break loop47;
}
}
mNUMBER();
// CSS.g:682:34: ( WS )*
loop48:
while (true) {
int alt48=2;
int LA48_0 = input.LA(1);
if ( ((LA48_0 >= '\t' && LA48_0 <= '\n')||LA48_0=='\r'||LA48_0==' ') ) {
alt48=1;
}
switch (alt48) {
case 1 :
// CSS.g:682:34: WS
{
mWS();
}
break;
default :
break loop48;
}
}
}
match(',');
// CSS.g:683:21: ( ( WS )* NUMBER ( WS )* )
// CSS.g:683:23: ( WS )* NUMBER ( WS )*
{
// CSS.g:683:23: ( WS )*
loop49:
while (true) {
int alt49=2;
int LA49_0 = input.LA(1);
if ( ((LA49_0 >= '\t' && LA49_0 <= '\n')||LA49_0=='\r'||LA49_0==' ') ) {
alt49=1;
}
switch (alt49) {
case 1 :
// CSS.g:683:23: WS
{
mWS();
}
break;
default :
break loop49;
}
}
mNUMBER();
// CSS.g:683:34: ( WS )*
loop50:
while (true) {
int alt50=2;
int LA50_0 = input.LA(1);
if ( ((LA50_0 >= '\t' && LA50_0 <= '\n')||LA50_0=='\r'||LA50_0==' ') ) {
alt50=1;
}
switch (alt50) {
case 1 :
// CSS.g:683:34: WS
{
mWS();
}
break;
default :
break loop50;
}
}
}
match(')');
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "MATRIX3D_VALUE"
// $ANTLR start "RGBA"
public final void mRGBA() throws RecognitionException {
try {
int _type = RGBA;
int _channel = DEFAULT_TOKEN_CHANNEL;
// CSS.g:690:6: ( 'rgba(' ( ( WS )* NUMBER ( PERCENT |) ( WS )* ) ',' ( ( WS )* NUMBER ( PERCENT |) ( WS )* ) ',' ( ( WS )* NUMBER ( PERCENT |) ( WS )* ) ',' ( ( WS )* NUMBER ( PERCENT |) ( WS )* ) ')' | 'rgba(' ( ( WS )* NUMBER ( PERCENT |) ( WS )* ) ( ( WS )* NUMBER ( PERCENT |) ( WS )* ) ( ( WS )* NUMBER ( PERCENT |) ( WS )* ) '/' ( ( WS )* NUMBER ( PERCENT |) ( WS )* ) ')' )
int alt75=2;
alt75 = dfa75.predict(input);
switch (alt75) {
case 1 :
// CSS.g:690:9: 'rgba(' ( ( WS )* NUMBER ( PERCENT |) ( WS )* ) ',' ( ( WS )* NUMBER ( PERCENT |) ( WS )* ) ',' ( ( WS )* NUMBER ( PERCENT |) ( WS )* ) ',' ( ( WS )* NUMBER ( PERCENT |) ( WS )* ) ')'
{
match("rgba(");
// CSS.g:690:18: ( ( WS )* NUMBER ( PERCENT |) ( WS )* )
// CSS.g:690:20: ( WS )* NUMBER ( PERCENT |) ( WS )*
{
// CSS.g:690:20: ( WS )*
loop51:
while (true) {
int alt51=2;
int LA51_0 = input.LA(1);
if ( ((LA51_0 >= '\t' && LA51_0 <= '\n')||LA51_0=='\r'||LA51_0==' ') ) {
alt51=1;
}
switch (alt51) {
case 1 :
// CSS.g:690:20: WS
{
mWS();
}
break;
default :
break loop51;
}
}
mNUMBER();
// CSS.g:690:31: ( PERCENT |)
int alt52=2;
int LA52_0 = input.LA(1);
if ( (LA52_0=='%') ) {
alt52=1;
}
else if ( ((LA52_0 >= '\t' && LA52_0 <= '\n')||LA52_0=='\r'||LA52_0==' '||LA52_0==',') ) {
alt52=2;
}
else {
NoViableAltException nvae =
new NoViableAltException("", 52, 0, input);
throw nvae;
}
switch (alt52) {
case 1 :
// CSS.g:690:33: PERCENT
{
mPERCENT();
}
break;
case 2 :
// CSS.g:690:43:
{
}
break;
}
// CSS.g:690:45: ( WS )*
loop53:
while (true) {
int alt53=2;
int LA53_0 = input.LA(1);
if ( ((LA53_0 >= '\t' && LA53_0 <= '\n')||LA53_0=='\r'||LA53_0==' ') ) {
alt53=1;
}
switch (alt53) {
case 1 :
// CSS.g:690:45: WS
{
mWS();
}
break;
default :
break loop53;
}
}
}
match(',');
// CSS.g:691:5: ( ( WS )* NUMBER ( PERCENT |) ( WS )* )
// CSS.g:691:7: ( WS )* NUMBER ( PERCENT |) ( WS )*
{
// CSS.g:691:7: ( WS )*
loop54:
while (true) {
int alt54=2;
int LA54_0 = input.LA(1);
if ( ((LA54_0 >= '\t' && LA54_0 <= '\n')||LA54_0=='\r'||LA54_0==' ') ) {
alt54=1;
}
switch (alt54) {
case 1 :
// CSS.g:691:7: WS
{
mWS();
}
break;
default :
break loop54;
}
}
mNUMBER();
// CSS.g:691:18: ( PERCENT |)
int alt55=2;
int LA55_0 = input.LA(1);
if ( (LA55_0=='%') ) {
alt55=1;
}
else if ( ((LA55_0 >= '\t' && LA55_0 <= '\n')||LA55_0=='\r'||LA55_0==' '||LA55_0==',') ) {
alt55=2;
}
else {
NoViableAltException nvae =
new NoViableAltException("", 55, 0, input);
throw nvae;
}
switch (alt55) {
case 1 :
// CSS.g:691:20: PERCENT
{
mPERCENT();
}
break;
case 2 :
// CSS.g:691:30:
{
}
break;
}
// CSS.g:691:32: ( WS )*
loop56:
while (true) {
int alt56=2;
int LA56_0 = input.LA(1);
if ( ((LA56_0 >= '\t' && LA56_0 <= '\n')||LA56_0=='\r'||LA56_0==' ') ) {
alt56=1;
}
switch (alt56) {
case 1 :
// CSS.g:691:32: WS
{
mWS();
}
break;
default :
break loop56;
}
}
}
match(',');
// CSS.g:692:5: ( ( WS )* NUMBER ( PERCENT |) ( WS )* )
// CSS.g:692:7: ( WS )* NUMBER ( PERCENT |) ( WS )*
{
// CSS.g:692:7: ( WS )*
loop57:
while (true) {
int alt57=2;
int LA57_0 = input.LA(1);
if ( ((LA57_0 >= '\t' && LA57_0 <= '\n')||LA57_0=='\r'||LA57_0==' ') ) {
alt57=1;
}
switch (alt57) {
case 1 :
// CSS.g:692:7: WS
{
mWS();
}
break;
default :
break loop57;
}
}
mNUMBER();
// CSS.g:692:18: ( PERCENT |)
int alt58=2;
int LA58_0 = input.LA(1);
if ( (LA58_0=='%') ) {
alt58=1;
}
else if ( ((LA58_0 >= '\t' && LA58_0 <= '\n')||LA58_0=='\r'||LA58_0==' '||LA58_0==',') ) {
alt58=2;
}
else {
NoViableAltException nvae =
new NoViableAltException("", 58, 0, input);
throw nvae;
}
switch (alt58) {
case 1 :
// CSS.g:692:20: PERCENT
{
mPERCENT();
}
break;
case 2 :
// CSS.g:692:30:
{
}
break;
}
// CSS.g:692:32: ( WS )*
loop59:
while (true) {
int alt59=2;
int LA59_0 = input.LA(1);
if ( ((LA59_0 >= '\t' && LA59_0 <= '\n')||LA59_0=='\r'||LA59_0==' ') ) {
alt59=1;
}
switch (alt59) {
case 1 :
// CSS.g:692:32: WS
{
mWS();
}
break;
default :
break loop59;
}
}
}
match(',');
// CSS.g:693:5: ( ( WS )* NUMBER ( PERCENT |) ( WS )* )
// CSS.g:693:7: ( WS )* NUMBER ( PERCENT |) ( WS )*
{
// CSS.g:693:7: ( WS )*
loop60:
while (true) {
int alt60=2;
int LA60_0 = input.LA(1);
if ( ((LA60_0 >= '\t' && LA60_0 <= '\n')||LA60_0=='\r'||LA60_0==' ') ) {
alt60=1;
}
switch (alt60) {
case 1 :
// CSS.g:693:7: WS
{
mWS();
}
break;
default :
break loop60;
}
}
mNUMBER();
// CSS.g:693:18: ( PERCENT |)
int alt61=2;
int LA61_0 = input.LA(1);
if ( (LA61_0=='%') ) {
alt61=1;
}
else if ( ((LA61_0 >= '\t' && LA61_0 <= '\n')||LA61_0=='\r'||LA61_0==' '||LA61_0==')') ) {
alt61=2;
}
else {
NoViableAltException nvae =
new NoViableAltException("", 61, 0, input);
throw nvae;
}
switch (alt61) {
case 1 :
// CSS.g:693:20: PERCENT
{
mPERCENT();
}
break;
case 2 :
// CSS.g:693:30:
{
}
break;
}
// CSS.g:693:32: ( WS )*
loop62:
while (true) {
int alt62=2;
int LA62_0 = input.LA(1);
if ( ((LA62_0 >= '\t' && LA62_0 <= '\n')||LA62_0=='\r'||LA62_0==' ') ) {
alt62=1;
}
switch (alt62) {
case 1 :
// CSS.g:693:32: WS
{
mWS();
}
break;
default :
break loop62;
}
}
}
match(')');
}
break;
case 2 :
// CSS.g:695:9: 'rgba(' ( ( WS )* NUMBER ( PERCENT |) ( WS )* ) ( ( WS )* NUMBER ( PERCENT |) ( WS )* ) ( ( WS )* NUMBER ( PERCENT |) ( WS )* ) '/' ( ( WS )* NUMBER ( PERCENT |) ( WS )* ) ')'
{
match("rgba(");
// CSS.g:695:18: ( ( WS )* NUMBER ( PERCENT |) ( WS )* )
// CSS.g:695:20: ( WS )* NUMBER ( PERCENT |) ( WS )*
{
// CSS.g:695:20: ( WS )*
loop63:
while (true) {
int alt63=2;
int LA63_0 = input.LA(1);
if ( ((LA63_0 >= '\t' && LA63_0 <= '\n')||LA63_0=='\r'||LA63_0==' ') ) {
alt63=1;
}
switch (alt63) {
case 1 :
// CSS.g:695:20: WS
{
mWS();
}
break;
default :
break loop63;
}
}
mNUMBER();
// CSS.g:695:31: ( PERCENT |)
int alt64=2;
int LA64_0 = input.LA(1);
if ( (LA64_0=='%') ) {
alt64=1;
}
else if ( ((LA64_0 >= '\t' && LA64_0 <= '\n')||LA64_0=='\r'||LA64_0==' '||LA64_0=='+'||(LA64_0 >= '-' && LA64_0 <= '.')||(LA64_0 >= '0' && LA64_0 <= '9')) ) {
alt64=2;
}
else {
NoViableAltException nvae =
new NoViableAltException("", 64, 0, input);
throw nvae;
}
switch (alt64) {
case 1 :
// CSS.g:695:33: PERCENT
{
mPERCENT();
}
break;
case 2 :
// CSS.g:695:43:
{
}
break;
}
// CSS.g:695:45: ( WS )*
loop65:
while (true) {
int alt65=2;
int LA65_0 = input.LA(1);
if ( ((LA65_0 >= '\t' && LA65_0 <= '\n')||LA65_0=='\r'||LA65_0==' ') ) {
alt65=1;
}
switch (alt65) {
case 1 :
// CSS.g:695:45: WS
{
mWS();
}
break;
default :
break loop65;
}
}
}
// CSS.g:696:5: ( ( WS )* NUMBER ( PERCENT |) ( WS )* )
// CSS.g:696:7: ( WS )* NUMBER ( PERCENT |) ( WS )*
{
// CSS.g:696:7: ( WS )*
loop66:
while (true) {
int alt66=2;
int LA66_0 = input.LA(1);
if ( ((LA66_0 >= '\t' && LA66_0 <= '\n')||LA66_0=='\r'||LA66_0==' ') ) {
alt66=1;
}
switch (alt66) {
case 1 :
// CSS.g:696:7: WS
{
mWS();
}
break;
default :
break loop66;
}
}
mNUMBER();
// CSS.g:696:18: ( PERCENT |)
int alt67=2;
int LA67_0 = input.LA(1);
if ( (LA67_0=='%') ) {
alt67=1;
}
else if ( ((LA67_0 >= '\t' && LA67_0 <= '\n')||LA67_0=='\r'||LA67_0==' '||LA67_0=='+'||(LA67_0 >= '-' && LA67_0 <= '.')||(LA67_0 >= '0' && LA67_0 <= '9')) ) {
alt67=2;
}
else {
NoViableAltException nvae =
new NoViableAltException("", 67, 0, input);
throw nvae;
}
switch (alt67) {
case 1 :
// CSS.g:696:20: PERCENT
{
mPERCENT();
}
break;
case 2 :
// CSS.g:696:30:
{
}
break;
}
// CSS.g:696:32: ( WS )*
loop68:
while (true) {
int alt68=2;
int LA68_0 = input.LA(1);
if ( ((LA68_0 >= '\t' && LA68_0 <= '\n')||LA68_0=='\r'||LA68_0==' ') ) {
alt68=1;
}
switch (alt68) {
case 1 :
// CSS.g:696:32: WS
{
mWS();
}
break;
default :
break loop68;
}
}
}
// CSS.g:697:5: ( ( WS )* NUMBER ( PERCENT |) ( WS )* )
// CSS.g:697:7: ( WS )* NUMBER ( PERCENT |) ( WS )*
{
// CSS.g:697:7: ( WS )*
loop69:
while (true) {
int alt69=2;
int LA69_0 = input.LA(1);
if ( ((LA69_0 >= '\t' && LA69_0 <= '\n')||LA69_0=='\r'||LA69_0==' ') ) {
alt69=1;
}
switch (alt69) {
case 1 :
// CSS.g:697:7: WS
{
mWS();
}
break;
default :
break loop69;
}
}
mNUMBER();
// CSS.g:697:18: ( PERCENT |)
int alt70=2;
int LA70_0 = input.LA(1);
if ( (LA70_0=='%') ) {
alt70=1;
}
else if ( ((LA70_0 >= '\t' && LA70_0 <= '\n')||LA70_0=='\r'||LA70_0==' '||LA70_0=='/') ) {
alt70=2;
}
else {
NoViableAltException nvae =
new NoViableAltException("", 70, 0, input);
throw nvae;
}
switch (alt70) {
case 1 :
// CSS.g:697:20: PERCENT
{
mPERCENT();
}
break;
case 2 :
// CSS.g:697:30:
{
}
break;
}
// CSS.g:697:32: ( WS )*
loop71:
while (true) {
int alt71=2;
int LA71_0 = input.LA(1);
if ( ((LA71_0 >= '\t' && LA71_0 <= '\n')||LA71_0=='\r'||LA71_0==' ') ) {
alt71=1;
}
switch (alt71) {
case 1 :
// CSS.g:697:32: WS
{
mWS();
}
break;
default :
break loop71;
}
}
}
match('/');
// CSS.g:698:5: ( ( WS )* NUMBER ( PERCENT |) ( WS )* )
// CSS.g:698:7: ( WS )* NUMBER ( PERCENT |) ( WS )*
{
// CSS.g:698:7: ( WS )*
loop72:
while (true) {
int alt72=2;
int LA72_0 = input.LA(1);
if ( ((LA72_0 >= '\t' && LA72_0 <= '\n')||LA72_0=='\r'||LA72_0==' ') ) {
alt72=1;
}
switch (alt72) {
case 1 :
// CSS.g:698:7: WS
{
mWS();
}
break;
default :
break loop72;
}
}
mNUMBER();
// CSS.g:698:18: ( PERCENT |)
int alt73=2;
int LA73_0 = input.LA(1);
if ( (LA73_0=='%') ) {
alt73=1;
}
else if ( ((LA73_0 >= '\t' && LA73_0 <= '\n')||LA73_0=='\r'||LA73_0==' '||LA73_0==')') ) {
alt73=2;
}
else {
NoViableAltException nvae =
new NoViableAltException("", 73, 0, input);
throw nvae;
}
switch (alt73) {
case 1 :
// CSS.g:698:20: PERCENT
{
mPERCENT();
}
break;
case 2 :
// CSS.g:698:30:
{
}
break;
}
// CSS.g:698:32: ( WS )*
loop74:
while (true) {
int alt74=2;
int LA74_0 = input.LA(1);
if ( ((LA74_0 >= '\t' && LA74_0 <= '\n')||LA74_0=='\r'||LA74_0==' ') ) {
alt74=1;
}
switch (alt74) {
case 1 :
// CSS.g:698:32: WS
{
mWS();
}
break;
default :
break loop74;
}
}
}
match(')');
}
break;
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "RGBA"
// $ANTLR start "RGB"
public final void mRGB() throws RecognitionException {
try {
int _type = RGB;
int _channel = DEFAULT_TOKEN_CHANNEL;
// CSS.g:705:5: ( 'rgb(' ( ( WS )* NUMBER ( PERCENT |) ( WS )* ) ',' ( ( WS )* NUMBER ( PERCENT |) ( WS )* ) ',' ( ( WS )* NUMBER ( PERCENT |) ( WS )* ) ')' | 'rgb(' ( ( WS )* NUMBER ( PERCENT |) ( WS )* ) ( ( WS )* NUMBER ( PERCENT |) ( WS )* ) ( ( WS )* NUMBER ( PERCENT |) ( WS )* ) ')' )
int alt94=2;
alt94 = dfa94.predict(input);
switch (alt94) {
case 1 :
// CSS.g:705:8: 'rgb(' ( ( WS )* NUMBER ( PERCENT |) ( WS )* ) ',' ( ( WS )* NUMBER ( PERCENT |) ( WS )* ) ',' ( ( WS )* NUMBER ( PERCENT |) ( WS )* ) ')'
{
match("rgb(");
// CSS.g:705:16: ( ( WS )* NUMBER ( PERCENT |) ( WS )* )
// CSS.g:705:18: ( WS )* NUMBER ( PERCENT |) ( WS )*
{
// CSS.g:705:18: ( WS )*
loop76:
while (true) {
int alt76=2;
int LA76_0 = input.LA(1);
if ( ((LA76_0 >= '\t' && LA76_0 <= '\n')||LA76_0=='\r'||LA76_0==' ') ) {
alt76=1;
}
switch (alt76) {
case 1 :
// CSS.g:705:18: WS
{
mWS();
}
break;
default :
break loop76;
}
}
mNUMBER();
// CSS.g:705:29: ( PERCENT |)
int alt77=2;
int LA77_0 = input.LA(1);
if ( (LA77_0=='%') ) {
alt77=1;
}
else if ( ((LA77_0 >= '\t' && LA77_0 <= '\n')||LA77_0=='\r'||LA77_0==' '||LA77_0==',') ) {
alt77=2;
}
else {
NoViableAltException nvae =
new NoViableAltException("", 77, 0, input);
throw nvae;
}
switch (alt77) {
case 1 :
// CSS.g:705:31: PERCENT
{
mPERCENT();
}
break;
case 2 :
// CSS.g:705:41:
{
}
break;
}
// CSS.g:705:43: ( WS )*
loop78:
while (true) {
int alt78=2;
int LA78_0 = input.LA(1);
if ( ((LA78_0 >= '\t' && LA78_0 <= '\n')||LA78_0=='\r'||LA78_0==' ') ) {
alt78=1;
}
switch (alt78) {
case 1 :
// CSS.g:705:43: WS
{
mWS();
}
break;
default :
break loop78;
}
}
}
match(',');
// CSS.g:706:5: ( ( WS )* NUMBER ( PERCENT |) ( WS )* )
// CSS.g:706:7: ( WS )* NUMBER ( PERCENT |) ( WS )*
{
// CSS.g:706:7: ( WS )*
loop79:
while (true) {
int alt79=2;
int LA79_0 = input.LA(1);
if ( ((LA79_0 >= '\t' && LA79_0 <= '\n')||LA79_0=='\r'||LA79_0==' ') ) {
alt79=1;
}
switch (alt79) {
case 1 :
// CSS.g:706:7: WS
{
mWS();
}
break;
default :
break loop79;
}
}
mNUMBER();
// CSS.g:706:18: ( PERCENT |)
int alt80=2;
int LA80_0 = input.LA(1);
if ( (LA80_0=='%') ) {
alt80=1;
}
else if ( ((LA80_0 >= '\t' && LA80_0 <= '\n')||LA80_0=='\r'||LA80_0==' '||LA80_0==',') ) {
alt80=2;
}
else {
NoViableAltException nvae =
new NoViableAltException("", 80, 0, input);
throw nvae;
}
switch (alt80) {
case 1 :
// CSS.g:706:20: PERCENT
{
mPERCENT();
}
break;
case 2 :
// CSS.g:706:30:
{
}
break;
}
// CSS.g:706:32: ( WS )*
loop81:
while (true) {
int alt81=2;
int LA81_0 = input.LA(1);
if ( ((LA81_0 >= '\t' && LA81_0 <= '\n')||LA81_0=='\r'||LA81_0==' ') ) {
alt81=1;
}
switch (alt81) {
case 1 :
// CSS.g:706:32: WS
{
mWS();
}
break;
default :
break loop81;
}
}
}
match(',');
// CSS.g:707:5: ( ( WS )* NUMBER ( PERCENT |) ( WS )* )
// CSS.g:707:7: ( WS )* NUMBER ( PERCENT |) ( WS )*
{
// CSS.g:707:7: ( WS )*
loop82:
while (true) {
int alt82=2;
int LA82_0 = input.LA(1);
if ( ((LA82_0 >= '\t' && LA82_0 <= '\n')||LA82_0=='\r'||LA82_0==' ') ) {
alt82=1;
}
switch (alt82) {
case 1 :
// CSS.g:707:7: WS
{
mWS();
}
break;
default :
break loop82;
}
}
mNUMBER();
// CSS.g:707:18: ( PERCENT |)
int alt83=2;
int LA83_0 = input.LA(1);
if ( (LA83_0=='%') ) {
alt83=1;
}
else if ( ((LA83_0 >= '\t' && LA83_0 <= '\n')||LA83_0=='\r'||LA83_0==' '||LA83_0==')') ) {
alt83=2;
}
else {
NoViableAltException nvae =
new NoViableAltException("", 83, 0, input);
throw nvae;
}
switch (alt83) {
case 1 :
// CSS.g:707:20: PERCENT
{
mPERCENT();
}
break;
case 2 :
// CSS.g:707:30:
{
}
break;
}
// CSS.g:707:32: ( WS )*
loop84:
while (true) {
int alt84=2;
int LA84_0 = input.LA(1);
if ( ((LA84_0 >= '\t' && LA84_0 <= '\n')||LA84_0=='\r'||LA84_0==' ') ) {
alt84=1;
}
switch (alt84) {
case 1 :
// CSS.g:707:32: WS
{
mWS();
}
break;
default :
break loop84;
}
}
}
match(')');
}
break;
case 2 :
// CSS.g:709:8: 'rgb(' ( ( WS )* NUMBER ( PERCENT |) ( WS )* ) ( ( WS )* NUMBER ( PERCENT |) ( WS )* ) ( ( WS )* NUMBER ( PERCENT |) ( WS )* ) ')'
{
match("rgb(");
// CSS.g:709:16: ( ( WS )* NUMBER ( PERCENT |) ( WS )* )
// CSS.g:709:18: ( WS )* NUMBER ( PERCENT |) ( WS )*
{
// CSS.g:709:18: ( WS )*
loop85:
while (true) {
int alt85=2;
int LA85_0 = input.LA(1);
if ( ((LA85_0 >= '\t' && LA85_0 <= '\n')||LA85_0=='\r'||LA85_0==' ') ) {
alt85=1;
}
switch (alt85) {
case 1 :
// CSS.g:709:18: WS
{
mWS();
}
break;
default :
break loop85;
}
}
mNUMBER();
// CSS.g:709:29: ( PERCENT |)
int alt86=2;
int LA86_0 = input.LA(1);
if ( (LA86_0=='%') ) {
alt86=1;
}
else if ( ((LA86_0 >= '\t' && LA86_0 <= '\n')||LA86_0=='\r'||LA86_0==' '||LA86_0=='+'||(LA86_0 >= '-' && LA86_0 <= '.')||(LA86_0 >= '0' && LA86_0 <= '9')) ) {
alt86=2;
}
else {
NoViableAltException nvae =
new NoViableAltException("", 86, 0, input);
throw nvae;
}
switch (alt86) {
case 1 :
// CSS.g:709:31: PERCENT
{
mPERCENT();
}
break;
case 2 :
// CSS.g:709:41:
{
}
break;
}
// CSS.g:709:43: ( WS )*
loop87:
while (true) {
int alt87=2;
int LA87_0 = input.LA(1);
if ( ((LA87_0 >= '\t' && LA87_0 <= '\n')||LA87_0=='\r'||LA87_0==' ') ) {
alt87=1;
}
switch (alt87) {
case 1 :
// CSS.g:709:43: WS
{
mWS();
}
break;
default :
break loop87;
}
}
}
// CSS.g:710:5: ( ( WS )* NUMBER ( PERCENT |) ( WS )* )
// CSS.g:710:7: ( WS )* NUMBER ( PERCENT |) ( WS )*
{
// CSS.g:710:7: ( WS )*
loop88:
while (true) {
int alt88=2;
int LA88_0 = input.LA(1);
if ( ((LA88_0 >= '\t' && LA88_0 <= '\n')||LA88_0=='\r'||LA88_0==' ') ) {
alt88=1;
}
switch (alt88) {
case 1 :
// CSS.g:710:7: WS
{
mWS();
}
break;
default :
break loop88;
}
}
mNUMBER();
// CSS.g:710:18: ( PERCENT |)
int alt89=2;
int LA89_0 = input.LA(1);
if ( (LA89_0=='%') ) {
alt89=1;
}
else if ( ((LA89_0 >= '\t' && LA89_0 <= '\n')||LA89_0=='\r'||LA89_0==' '||LA89_0=='+'||(LA89_0 >= '-' && LA89_0 <= '.')||(LA89_0 >= '0' && LA89_0 <= '9')) ) {
alt89=2;
}
else {
NoViableAltException nvae =
new NoViableAltException("", 89, 0, input);
throw nvae;
}
switch (alt89) {
case 1 :
// CSS.g:710:20: PERCENT
{
mPERCENT();
}
break;
case 2 :
// CSS.g:710:30:
{
}
break;
}
// CSS.g:710:32: ( WS )*
loop90:
while (true) {
int alt90=2;
int LA90_0 = input.LA(1);
if ( ((LA90_0 >= '\t' && LA90_0 <= '\n')||LA90_0=='\r'||LA90_0==' ') ) {
alt90=1;
}
switch (alt90) {
case 1 :
// CSS.g:710:32: WS
{
mWS();
}
break;
default :
break loop90;
}
}
}
// CSS.g:711:5: ( ( WS )* NUMBER ( PERCENT |) ( WS )* )
// CSS.g:711:7: ( WS )* NUMBER ( PERCENT |) ( WS )*
{
// CSS.g:711:7: ( WS )*
loop91:
while (true) {
int alt91=2;
int LA91_0 = input.LA(1);
if ( ((LA91_0 >= '\t' && LA91_0 <= '\n')||LA91_0=='\r'||LA91_0==' ') ) {
alt91=1;
}
switch (alt91) {
case 1 :
// CSS.g:711:7: WS
{
mWS();
}
break;
default :
break loop91;
}
}
mNUMBER();
// CSS.g:711:18: ( PERCENT |)
int alt92=2;
int LA92_0 = input.LA(1);
if ( (LA92_0=='%') ) {
alt92=1;
}
else if ( ((LA92_0 >= '\t' && LA92_0 <= '\n')||LA92_0=='\r'||LA92_0==' '||LA92_0==')') ) {
alt92=2;
}
else {
NoViableAltException nvae =
new NoViableAltException("", 92, 0, input);
throw nvae;
}
switch (alt92) {
case 1 :
// CSS.g:711:20: PERCENT
{
mPERCENT();
}
break;
case 2 :
// CSS.g:711:30:
{
}
break;
}
// CSS.g:711:32: ( WS )*
loop93:
while (true) {
int alt93=2;
int LA93_0 = input.LA(1);
if ( ((LA93_0 >= '\t' && LA93_0 <= '\n')||LA93_0=='\r'||LA93_0==' ') ) {
alt93=1;
}
switch (alt93) {
case 1 :
// CSS.g:711:32: WS
{
mWS();
}
break;
default :
break loop93;
}
}
}
match(')');
}
break;
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "RGB"
// $ANTLR start "ARGUMENTS"
public final void mARGUMENTS() throws RecognitionException {
try {
int _type = ARGUMENTS;
int _channel = DEFAULT_TOKEN_CHANNEL;
// CSS.g:716:5: ( '(' ( options {greedy=false; } : ARGUMENTS | . )* ')' )
// CSS.g:716:9: '(' ( options {greedy=false; } : ARGUMENTS | . )* ')'
{
match('(');
// CSS.g:716:13: ( options {greedy=false; } : ARGUMENTS | . )*
loop95:
while (true) {
int alt95=3;
int LA95_0 = input.LA(1);
if ( (LA95_0==')') ) {
alt95=3;
}
else if ( (LA95_0=='(') ) {
alt95=1;
}
else if ( ((LA95_0 >= '\u0000' && LA95_0 <= '\'')||(LA95_0 >= '*' && LA95_0 <= '\uFFFF')) ) {
alt95=2;
}
switch (alt95) {
case 1 :
// CSS.g:716:40: ARGUMENTS
{
mARGUMENTS();
}
break;
case 2 :
// CSS.g:716:52: .
{
matchAny();
}
break;
default :
break loop95;
}
}
match(')');
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "ARGUMENTS"
// $ANTLR start "SEMI_COLONS"
public final void mSEMI_COLONS() throws RecognitionException {
try {
int _type = SEMI_COLONS;
int _channel = DEFAULT_TOKEN_CHANNEL;
// CSS.g:726:13: ( ( ';' )+ )
// CSS.g:726:15: ( ';' )+
{
// CSS.g:726:15: ( ';' )+
int cnt96=0;
loop96:
while (true) {
int alt96=2;
int LA96_0 = input.LA(1);
if ( (LA96_0==';') ) {
alt96=1;
}
switch (alt96) {
case 1 :
// CSS.g:726:15: ';'
{
match(';');
}
break;
default :
if ( cnt96 >= 1 ) break loop96;
EarlyExitException eee = new EarlyExitException(96, input);
throw eee;
}
cnt96++;
}
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "SEMI_COLONS"
// $ANTLR start "HASH_WORD"
public final void mHASH_WORD() throws RecognitionException {
try {
int _type = HASH_WORD;
int _channel = DEFAULT_TOKEN_CHANNEL;
// CSS.g:733:5: ( '#' ( LETTER | DIGIT | '-' | '_' )+ )
// CSS.g:733:9: '#' ( LETTER | DIGIT | '-' | '_' )+
{
match('#');
// CSS.g:733:13: ( LETTER | DIGIT | '-' | '_' )+
int cnt97=0;
loop97:
while (true) {
int alt97=2;
int LA97_0 = input.LA(1);
if ( (LA97_0=='-'||(LA97_0 >= '0' && LA97_0 <= '9')||(LA97_0 >= 'A' && LA97_0 <= 'Z')||LA97_0=='_'||(LA97_0 >= 'a' && LA97_0 <= 'z')) ) {
alt97=1;
}
switch (alt97) {
case 1 :
// CSS.g:
{
if ( input.LA(1)=='-'||(input.LA(1) >= '0' && input.LA(1) <= '9')||(input.LA(1) >= 'A' && input.LA(1) <= 'Z')||input.LA(1)=='_'||(input.LA(1) >= 'a' && input.LA(1) <= 'z') ) {
input.consume();
}
else {
MismatchedSetException mse = new MismatchedSetException(null,input);
recover(mse);
throw mse;
}
}
break;
default :
if ( cnt97 >= 1 ) break loop97;
EarlyExitException eee = new EarlyExitException(97, input);
throw eee;
}
cnt97++;
}
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "HASH_WORD"
// $ANTLR start "ID"
public final void mID() throws RecognitionException {
try {
int _type = ID;
int _channel = DEFAULT_TOKEN_CHANNEL;
// CSS.g:732:5: ( ( '-' | '_' | '__' | '___' )? LETTER ( LETTER | DIGIT | '-' | '_' )* )
// CSS.g:732:9: ( '-' | '_' | '__' | '___' )? LETTER ( LETTER | DIGIT | '-' | '_' )*
{
// CSS.g:732:9: ( '-' | '_' | '__' | '___' )?
int alt98=5;
int LA98_0 = input.LA(1);
if ( (LA98_0=='-') ) {
alt98=1;
}
else if ( (LA98_0=='_') ) {
int LA98_2 = input.LA(2);
if ( (LA98_2=='_') ) {
int LA98_4 = input.LA(3);
if ( (LA98_4=='_') ) {
alt98=4;
}
else if ( ((LA98_4 >= 'A' && LA98_4 <= 'Z')||(LA98_4 >= 'a' && LA98_4 <= 'z')) ) {
alt98=3;
}
}
else if ( ((LA98_2 >= 'A' && LA98_2 <= 'Z')||(LA98_2 >= 'a' && LA98_2 <= 'z')) ) {
alt98=2;
}
}
switch (alt98) {
case 1 :
// CSS.g:732:11: '-'
{
match('-');
}
break;
case 2 :
// CSS.g:732:17: '_'
{
match('_');
}
break;
case 3 :
// CSS.g:732:23: '__'
{
match("__");
}
break;
case 4 :
// CSS.g:732:30: '___'
{
match("___");
}
break;
}
mLETTER();
// CSS.g:732:46: ( LETTER | DIGIT | '-' | '_' )*
loop99:
while (true) {
int alt99=2;
int LA99_0 = input.LA(1);
if ( (LA99_0=='-'||(LA99_0 >= '0' && LA99_0 <= '9')||(LA99_0 >= 'A' && LA99_0 <= 'Z')||LA99_0=='_'||(LA99_0 >= 'a' && LA99_0 <= 'z')) ) {
alt99=1;
}
switch (alt99) {
case 1 :
// CSS.g:
{
if ( input.LA(1)=='-'||(input.LA(1) >= '0' && input.LA(1) <= '9')||(input.LA(1) >= 'A' && input.LA(1) <= 'Z')||input.LA(1)=='_'||(input.LA(1) >= 'a' && input.LA(1) <= 'z') ) {
input.consume();
}
else {
MismatchedSetException mse = new MismatchedSetException(null,input);
recover(mse);
throw mse;
}
}
break;
default :
break loop99;
}
}
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "ID"
// $ANTLR start "DASHED_ID"
public final void mDASHED_ID() throws RecognitionException {
try {
int _type = DASHED_ID;
int _channel = DEFAULT_TOKEN_CHANNEL;
// CSS.g:735:12: ( '--' LETTER ( LETTER | DIGIT | '-' | '_' )* )
// CSS.g:735:16: '--' LETTER ( LETTER | DIGIT | '-' | '_' )*
{
match("--");
mLETTER();
// CSS.g:735:28: ( LETTER | DIGIT | '-' | '_' )*
loop100:
while (true) {
int alt100=2;
int LA100_0 = input.LA(1);
if ( (LA100_0=='-'||(LA100_0 >= '0' && LA100_0 <= '9')||(LA100_0 >= 'A' && LA100_0 <= 'Z')||LA100_0=='_'||(LA100_0 >= 'a' && LA100_0 <= 'z')) ) {
alt100=1;
}
switch (alt100) {
case 1 :
// CSS.g:
{
if ( input.LA(1)=='-'||(input.LA(1) >= '0' && input.LA(1) <= '9')||(input.LA(1) >= 'A' && input.LA(1) <= 'Z')||input.LA(1)=='_'||(input.LA(1) >= 'a' && input.LA(1) <= 'z') ) {
input.consume();
}
else {
MismatchedSetException mse = new MismatchedSetException(null,input);
recover(mse);
throw mse;
}
}
break;
default :
break loop100;
}
}
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "DASHED_ID"
// $ANTLR start "OPERATOR"
public final void mOPERATOR() throws RecognitionException {
try {
int _type = OPERATOR;
int _channel = DEFAULT_TOKEN_CHANNEL;
// CSS.g:745:2: ( ( '+' | '-' | '*' | '/' ) )
// CSS.g:
{
if ( (input.LA(1) >= '*' && input.LA(1) <= '+')||input.LA(1)=='-'||input.LA(1)=='/' ) {
input.consume();
}
else {
MismatchedSetException mse = new MismatchedSetException(null,input);
recover(mse);
throw mse;
}
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "OPERATOR"
// $ANTLR start "LETTER"
public final void mLETTER() throws RecognitionException {
try {
// CSS.g:749:5: ( 'a' .. 'z' | 'A' .. 'Z' )
// CSS.g:
{
if ( (input.LA(1) >= 'A' && input.LA(1) <= 'Z')||(input.LA(1) >= 'a' && input.LA(1) <= 'z') ) {
input.consume();
}
else {
MismatchedSetException mse = new MismatchedSetException(null,input);
recover(mse);
throw mse;
}
}
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "LETTER"
// $ANTLR start "NUMBER"
public final void mNUMBER() throws RecognitionException {
try {
// CSS.g:761:2: ( ( '+' | '-' )? ( ( DIGIT )+ ( DOT ( DIGIT )+ )? | DOT ( DIGIT )+ ) )
// CSS.g:761:6: ( '+' | '-' )? ( ( DIGIT )+ ( DOT ( DIGIT )+ )? | DOT ( DIGIT )+ )
{
// CSS.g:761:6: ( '+' | '-' )?
int alt101=2;
int LA101_0 = input.LA(1);
if ( (LA101_0=='+'||LA101_0=='-') ) {
alt101=1;
}
switch (alt101) {
case 1 :
// CSS.g:
{
if ( input.LA(1)=='+'||input.LA(1)=='-' ) {
input.consume();
}
else {
MismatchedSetException mse = new MismatchedSetException(null,input);
recover(mse);
throw mse;
}
}
break;
}
// CSS.g:762:3: ( ( DIGIT )+ ( DOT ( DIGIT )+ )? | DOT ( DIGIT )+ )
int alt106=2;
int LA106_0 = input.LA(1);
if ( ((LA106_0 >= '0' && LA106_0 <= '9')) ) {
alt106=1;
}
else if ( (LA106_0=='.') ) {
alt106=2;
}
else {
NoViableAltException nvae =
new NoViableAltException("", 106, 0, input);
throw nvae;
}
switch (alt106) {
case 1 :
// CSS.g:762:5: ( DIGIT )+ ( DOT ( DIGIT )+ )?
{
// CSS.g:762:5: ( DIGIT )+
int cnt102=0;
loop102:
while (true) {
int alt102=2;
int LA102_0 = input.LA(1);
if ( ((LA102_0 >= '0' && LA102_0 <= '9')) ) {
alt102=1;
}
switch (alt102) {
case 1 :
// CSS.g:
{
if ( (input.LA(1) >= '0' && input.LA(1) <= '9') ) {
input.consume();
}
else {
MismatchedSetException mse = new MismatchedSetException(null,input);
recover(mse);
throw mse;
}
}
break;
default :
if ( cnt102 >= 1 ) break loop102;
EarlyExitException eee = new EarlyExitException(102, input);
throw eee;
}
cnt102++;
}
// CSS.g:762:12: ( DOT ( DIGIT )+ )?
int alt104=2;
int LA104_0 = input.LA(1);
if ( (LA104_0=='.') ) {
alt104=1;
}
switch (alt104) {
case 1 :
// CSS.g:762:13: DOT ( DIGIT )+
{
mDOT();
// CSS.g:762:17: ( DIGIT )+
int cnt103=0;
loop103:
while (true) {
int alt103=2;
int LA103_0 = input.LA(1);
if ( ((LA103_0 >= '0' && LA103_0 <= '9')) ) {
alt103=1;
}
switch (alt103) {
case 1 :
// CSS.g:
{
if ( (input.LA(1) >= '0' && input.LA(1) <= '9') ) {
input.consume();
}
else {
MismatchedSetException mse = new MismatchedSetException(null,input);
recover(mse);
throw mse;
}
}
break;
default :
if ( cnt103 >= 1 ) break loop103;
EarlyExitException eee = new EarlyExitException(103, input);
throw eee;
}
cnt103++;
}
}
break;
}
}
break;
case 2 :
// CSS.g:763:7: DOT ( DIGIT )+
{
mDOT();
// CSS.g:763:11: ( DIGIT )+
int cnt105=0;
loop105:
while (true) {
int alt105=2;
int LA105_0 = input.LA(1);
if ( ((LA105_0 >= '0' && LA105_0 <= '9')) ) {
alt105=1;
}
switch (alt105) {
case 1 :
// CSS.g:
{
if ( (input.LA(1) >= '0' && input.LA(1) <= '9') ) {
input.consume();
}
else {
MismatchedSetException mse = new MismatchedSetException(null,input);
recover(mse);
throw mse;
}
}
break;
default :
if ( cnt105 >= 1 ) break loop105;
EarlyExitException eee = new EarlyExitException(105, input);
throw eee;
}
cnt105++;
}
}
break;
}
}
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "NUMBER"
// $ANTLR start "NUMBER_WITH_PERCENT"
public final void mNUMBER_WITH_PERCENT() throws RecognitionException {
try {
int _type = NUMBER_WITH_PERCENT;
int _channel = DEFAULT_TOKEN_CHANNEL;
// CSS.g:791:5: ( NUMBER PERCENT )
// CSS.g:791:9: NUMBER PERCENT
{
mNUMBER();
mPERCENT();
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "NUMBER_WITH_PERCENT"
// $ANTLR start "NUMBER_WITH_UNIT"
public final void mNUMBER_WITH_UNIT() throws RecognitionException {
try {
int _type = NUMBER_WITH_UNIT;
int _channel = DEFAULT_TOKEN_CHANNEL;
// CSS.g:782:2: ( NUMBER ( ID )? )
// CSS.g:782:4: NUMBER ( ID )?
{
mNUMBER();
// CSS.g:782:11: ( ID )?
int alt107=2;
int LA107_0 = input.LA(1);
if ( (LA107_0=='-'||(LA107_0 >= 'A' && LA107_0 <= 'Z')||LA107_0=='_'||(LA107_0 >= 'a' && LA107_0 <= 'z')) ) {
alt107=1;
}
switch (alt107) {
case 1 :
// CSS.g:782:12: ID
{
mID();
}
break;
}
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "NUMBER_WITH_UNIT"
// $ANTLR start "DIGIT"
public final void mDIGIT() throws RecognitionException {
try {
// CSS.g:788:5: ( '0' .. '9' )
// CSS.g:
{
if ( (input.LA(1) >= '0' && input.LA(1) <= '9') ) {
input.consume();
}
else {
MismatchedSetException mse = new MismatchedSetException(null,input);
recover(mse);
throw mse;
}
}
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "DIGIT"
// $ANTLR start "COMMENT"
public final void mCOMMENT() throws RecognitionException {
try {
int _type = COMMENT;
int _channel = DEFAULT_TOKEN_CHANNEL;
// CSS.g:791:5: ( '/*' ( options {greedy=false; } : . )* '*/' )
// CSS.g:791:9: '/*' ( options {greedy=false; } : . )* '*/'
{
match("/*");
// CSS.g:791:14: ( options {greedy=false; } : . )*
loop108:
while (true) {
int alt108=2;
int LA108_0 = input.LA(1);
if ( (LA108_0=='*') ) {
int LA108_1 = input.LA(2);
if ( (LA108_1=='/') ) {
alt108=2;
}
else if ( ((LA108_1 >= '\u0000' && LA108_1 <= '.')||(LA108_1 >= '0' && LA108_1 <= '\uFFFF')) ) {
alt108=1;
}
}
else if ( ((LA108_0 >= '\u0000' && LA108_0 <= ')')||(LA108_0 >= '+' && LA108_0 <= '\uFFFF')) ) {
alt108=1;
}
switch (alt108) {
case 1 :
// CSS.g:791:41: .
{
matchAny();
}
break;
default :
break loop108;
}
}
match("*/");
_channel = HIDDEN;
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "COMMENT"
// $ANTLR start "WS"
public final void mWS() throws RecognitionException {
try {
int _type = WS;
int _channel = DEFAULT_TOKEN_CHANNEL;
// CSS.g:795:5: ( ( ' ' | '\\t' | '\\r' | '\\n' ) )
// CSS.g:795:9: ( ' ' | '\\t' | '\\r' | '\\n' )
{
if ( (input.LA(1) >= '\t' && input.LA(1) <= '\n')||input.LA(1)=='\r'||input.LA(1)==' ' ) {
input.consume();
}
else {
MismatchedSetException mse = new MismatchedSetException(null,input);
recover(mse);
throw mse;
}
_channel = HIDDEN;
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "WS"
// $ANTLR start "STRING"
public final void mSTRING() throws RecognitionException {
try {
int _type = STRING;
int _channel = DEFAULT_TOKEN_CHANNEL;
// CSS.g:799:5: ( STRING_QUOTE (~ ( '\\\\' | STRING_QUOTE ) | ESCAPED_HEX )* STRING_QUOTE )
// CSS.g:799:9: STRING_QUOTE (~ ( '\\\\' | STRING_QUOTE ) | ESCAPED_HEX )* STRING_QUOTE
{
mSTRING_QUOTE();
// CSS.g:800:9: (~ ( '\\\\' | STRING_QUOTE ) | ESCAPED_HEX )*
loop109:
while (true) {
int alt109=3;
int LA109_0 = input.LA(1);
if ( ((LA109_0 >= '\u0000' && LA109_0 <= '!')||(LA109_0 >= '#' && LA109_0 <= '&')||(LA109_0 >= '(' && LA109_0 <= '[')||(LA109_0 >= ']' && LA109_0 <= '\uFFFF')) ) {
alt109=1;
}
else if ( (LA109_0=='\\') ) {
alt109=2;
}
switch (alt109) {
case 1 :
// CSS.g:800:11: ~ ( '\\\\' | STRING_QUOTE )
{
if ( (input.LA(1) >= '\u0000' && input.LA(1) <= '!')||(input.LA(1) >= '#' && input.LA(1) <= '&')||(input.LA(1) >= '(' && input.LA(1) <= '[')||(input.LA(1) >= ']' && input.LA(1) <= '\uFFFF') ) {
input.consume();
}
else {
MismatchedSetException mse = new MismatchedSetException(null,input);
recover(mse);
throw mse;
}
}
break;
case 2 :
// CSS.g:800:38: ESCAPED_HEX
{
mESCAPED_HEX();
}
break;
default :
break loop109;
}
}
mSTRING_QUOTE();
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "STRING"
// $ANTLR start "STRING_QUOTE"
public final void mSTRING_QUOTE() throws RecognitionException {
try {
// CSS.g:807:5: ( '\"' | '\\'' )
// CSS.g:
{
if ( input.LA(1)=='\"'||input.LA(1)=='\'' ) {
input.consume();
}
else {
MismatchedSetException mse = new MismatchedSetException(null,input);
recover(mse);
throw mse;
}
}
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "STRING_QUOTE"
// $ANTLR start "HEX_DIGIT"
public final void mHEX_DIGIT() throws RecognitionException {
try {
// CSS.g:813:5: ( DIGIT | ( 'a' .. 'f' | 'A' .. 'F' ) )
// CSS.g:
{
if ( (input.LA(1) >= '0' && input.LA(1) <= '9')||(input.LA(1) >= 'A' && input.LA(1) <= 'F')||(input.LA(1) >= 'a' && input.LA(1) <= 'f') ) {
input.consume();
}
else {
MismatchedSetException mse = new MismatchedSetException(null,input);
recover(mse);
throw mse;
}
}
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "HEX_DIGIT"
// $ANTLR start "ESCAPED_HEX"
public final void mESCAPED_HEX() throws RecognitionException {
try {
// CSS.g:819:5: ( '\\\\' HEX_DIGIT HEX_DIGIT HEX_DIGIT HEX_DIGIT | '\\\\' HEX_DIGIT HEX_DIGIT HEX_DIGIT | '\\\\' HEX_DIGIT HEX_DIGIT | '\\\\' HEX_DIGIT )
int alt110=4;
int LA110_0 = input.LA(1);
if ( (LA110_0=='\\') ) {
int LA110_1 = input.LA(2);
if ( ((LA110_1 >= '0' && LA110_1 <= '9')||(LA110_1 >= 'A' && LA110_1 <= 'F')||(LA110_1 >= 'a' && LA110_1 <= 'f')) ) {
int LA110_2 = input.LA(3);
if ( ((LA110_2 >= '0' && LA110_2 <= '9')||(LA110_2 >= 'A' && LA110_2 <= 'F')||(LA110_2 >= 'a' && LA110_2 <= 'f')) ) {
int LA110_4 = input.LA(4);
if ( ((LA110_4 >= '0' && LA110_4 <= '9')||(LA110_4 >= 'A' && LA110_4 <= 'F')||(LA110_4 >= 'a' && LA110_4 <= 'f')) ) {
int LA110_6 = input.LA(5);
if ( ((LA110_6 >= '0' && LA110_6 <= '9')||(LA110_6 >= 'A' && LA110_6 <= 'F')||(LA110_6 >= 'a' && LA110_6 <= 'f')) ) {
alt110=1;
}
else {
alt110=2;
}
}
else {
alt110=3;
}
}
else {
alt110=4;
}
}
else {
int nvaeMark = input.mark();
try {
input.consume();
NoViableAltException nvae =
new NoViableAltException("", 110, 1, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
else {
NoViableAltException nvae =
new NoViableAltException("", 110, 0, input);
throw nvae;
}
switch (alt110) {
case 1 :
// CSS.g:819:10: '\\\\' HEX_DIGIT HEX_DIGIT HEX_DIGIT HEX_DIGIT
{
match('\\');
mHEX_DIGIT();
mHEX_DIGIT();
mHEX_DIGIT();
mHEX_DIGIT();
}
break;
case 2 :
// CSS.g:820:10: '\\\\' HEX_DIGIT HEX_DIGIT HEX_DIGIT
{
match('\\');
mHEX_DIGIT();
mHEX_DIGIT();
mHEX_DIGIT();
}
break;
case 3 :
// CSS.g:821:10: '\\\\' HEX_DIGIT HEX_DIGIT
{
match('\\');
mHEX_DIGIT();
mHEX_DIGIT();
}
break;
case 4 :
// CSS.g:822:10: '\\\\' HEX_DIGIT
{
match('\\');
mHEX_DIGIT();
}
break;
}
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "ESCAPED_HEX"
@Override
public void mTokens() throws RecognitionException {
// CSS.g:1:8: ( T__81 | BEGINS_WITH | ENDS_WITH | CONTAINS | LIST_MATCH | HREFLANG_MATCH | BLOCK_OPEN | BLOCK_END | SQUARE_OPEN | SQUARE_END | COMMA | PERCENT | PIPE | STAR | TILDE | DOT | EQUALS | AT_NAMESPACE | AT_MEDIA | AT_KEYFRAMES | AT_WEBKIT_KEYFRAMES | DOUBLE_COLON | COLON | AT_FONT_FACE | CLASS_REFERENCE | PROPERTY_REFERENCE | IMPORTANT | EMBED | URL | FORMAT | LOCAL | CALC | SCALE | VAR | NULL | ONLY | CHILD | PRECEDED | FUNCTIONS | NOT | ALPHA_VALUE | ROTATE_VALUE | SCALE_VALUE | TRANSLATE3D_VALUE | RECT_VALUE | MATRIX_VALUE | MATRIX3D_VALUE | RGBA | RGB | ARGUMENTS | SEMI_COLONS | HASH_WORD | ID | DASHED_ID | OPERATOR | NUMBER_WITH_PERCENT | NUMBER_WITH_UNIT | COMMENT | WS | STRING )
int alt111=60;
alt111 = dfa111.predict(input);
switch (alt111) {
case 1 :
// CSS.g:1:10: T__81
{
mT__81();
}
break;
case 2 :
// CSS.g:1:16: BEGINS_WITH
{
mBEGINS_WITH();
}
break;
case 3 :
// CSS.g:1:28: ENDS_WITH
{
mENDS_WITH();
}
break;
case 4 :
// CSS.g:1:38: CONTAINS
{
mCONTAINS();
}
break;
case 5 :
// CSS.g:1:47: LIST_MATCH
{
mLIST_MATCH();
}
break;
case 6 :
// CSS.g:1:58: HREFLANG_MATCH
{
mHREFLANG_MATCH();
}
break;
case 7 :
// CSS.g:1:73: BLOCK_OPEN
{
mBLOCK_OPEN();
}
break;
case 8 :
// CSS.g:1:84: BLOCK_END
{
mBLOCK_END();
}
break;
case 9 :
// CSS.g:1:94: SQUARE_OPEN
{
mSQUARE_OPEN();
}
break;
case 10 :
// CSS.g:1:106: SQUARE_END
{
mSQUARE_END();
}
break;
case 11 :
// CSS.g:1:117: COMMA
{
mCOMMA();
}
break;
case 12 :
// CSS.g:1:123: PERCENT
{
mPERCENT();
}
break;
case 13 :
// CSS.g:1:131: PIPE
{
mPIPE();
}
break;
case 14 :
// CSS.g:1:136: STAR
{
mSTAR();
}
break;
case 15 :
// CSS.g:1:141: TILDE
{
mTILDE();
}
break;
case 16 :
// CSS.g:1:147: DOT
{
mDOT();
}
break;
case 17 :
// CSS.g:1:151: EQUALS
{
mEQUALS();
}
break;
case 18 :
// CSS.g:1:158: AT_NAMESPACE
{
mAT_NAMESPACE();
}
break;
case 19 :
// CSS.g:1:171: AT_MEDIA
{
mAT_MEDIA();
}
break;
case 20 :
// CSS.g:1:180: AT_KEYFRAMES
{
mAT_KEYFRAMES();
}
break;
case 21 :
// CSS.g:1:193: AT_WEBKIT_KEYFRAMES
{
mAT_WEBKIT_KEYFRAMES();
}
break;
case 22 :
// CSS.g:1:213: DOUBLE_COLON
{
mDOUBLE_COLON();
}
break;
case 23 :
// CSS.g:1:226: COLON
{
mCOLON();
}
break;
case 24 :
// CSS.g:1:232: AT_FONT_FACE
{
mAT_FONT_FACE();
}
break;
case 25 :
// CSS.g:1:245: CLASS_REFERENCE
{
mCLASS_REFERENCE();
}
break;
case 26 :
// CSS.g:1:261: PROPERTY_REFERENCE
{
mPROPERTY_REFERENCE();
}
break;
case 27 :
// CSS.g:1:280: IMPORTANT
{
mIMPORTANT();
}
break;
case 28 :
// CSS.g:1:290: EMBED
{
mEMBED();
}
break;
case 29 :
// CSS.g:1:296: URL
{
mURL();
}
break;
case 30 :
// CSS.g:1:300: FORMAT
{
mFORMAT();
}
break;
case 31 :
// CSS.g:1:307: LOCAL
{
mLOCAL();
}
break;
case 32 :
// CSS.g:1:313: CALC
{
mCALC();
}
break;
case 33 :
// CSS.g:1:318: SCALE
{
mSCALE();
}
break;
case 34 :
// CSS.g:1:324: VAR
{
mVAR();
}
break;
case 35 :
// CSS.g:1:328: NULL
{
mNULL();
}
break;
case 36 :
// CSS.g:1:333: ONLY
{
mONLY();
}
break;
case 37 :
// CSS.g:1:338: CHILD
{
mCHILD();
}
break;
case 38 :
// CSS.g:1:344: PRECEDED
{
mPRECEDED();
}
break;
case 39 :
// CSS.g:1:353: FUNCTIONS
{
mFUNCTIONS();
}
break;
case 40 :
// CSS.g:1:363: NOT
{
mNOT();
}
break;
case 41 :
// CSS.g:1:367: ALPHA_VALUE
{
mALPHA_VALUE();
}
break;
case 42 :
// CSS.g:1:379: ROTATE_VALUE
{
mROTATE_VALUE();
}
break;
case 43 :
// CSS.g:1:392: SCALE_VALUE
{
mSCALE_VALUE();
}
break;
case 44 :
// CSS.g:1:404: TRANSLATE3D_VALUE
{
mTRANSLATE3D_VALUE();
}
break;
case 45 :
// CSS.g:1:422: RECT_VALUE
{
mRECT_VALUE();
}
break;
case 46 :
// CSS.g:1:433: MATRIX_VALUE
{
mMATRIX_VALUE();
}
break;
case 47 :
// CSS.g:1:446: MATRIX3D_VALUE
{
mMATRIX3D_VALUE();
}
break;
case 48 :
// CSS.g:1:461: RGBA
{
mRGBA();
}
break;
case 49 :
// CSS.g:1:466: RGB
{
mRGB();
}
break;
case 50 :
// CSS.g:1:470: ARGUMENTS
{
mARGUMENTS();
}
break;
case 51 :
// CSS.g:1:480: SEMI_COLONS
{
mSEMI_COLONS();
}
break;
case 52 :
// CSS.g:1:492: HASH_WORD
{
mHASH_WORD();
}
break;
case 53 :
// CSS.g:1:502: ID
{
mID();
}
break;
case 54 :
// CSS.g:1:505: DASHED_ID
{
mDASHED_ID();
}
break;
case 55 :
// CSS.g:1:515: OPERATOR
{
mOPERATOR();
}
break;
case 56 :
// CSS.g:1:524: NUMBER_WITH_PERCENT
{
mNUMBER_WITH_PERCENT();
}
break;
case 57 :
// CSS.g:1:544: NUMBER_WITH_UNIT
{
mNUMBER_WITH_UNIT();
}
break;
case 58 :
// CSS.g:1:561: COMMENT
{
mCOMMENT();
}
break;
case 59 :
// CSS.g:1:569: WS
{
mWS();
}
break;
case 60 :
// CSS.g:1:572: STRING
{
mSTRING();
}
break;
}
}
protected DFA1 dfa1 = new DFA1(this);
protected DFA75 dfa75 = new DFA75(this);
protected DFA94 dfa94 = new DFA94(this);
protected DFA111 dfa111 = new DFA111(this);
static final String DFA1_eotS =
"\47\uffff\1\56\25\uffff\1\102\10\uffff";
static final String DFA1_eofS =
"\106\uffff";
static final String DFA1_minS =
"\1\55\1\155\1\uffff\1\141\1\157\1\145\1\162\1\141\1\154\6\uffff\1\160"+
"\1\164\1\156\2\uffff\2\141\1\145\4\uffff\1\145\1\141\1\151\1\156\1\154"+
"\1\167\1\141\1\164\2\uffff\1\163\1\145\1\130\1\164\1\145\1\154\1\130\3"+
"\uffff\1\151\1\130\1\141\3\uffff\1\156\3\uffff\1\164\1\147\1\145\1\55"+
"\1\130\1\143\7\uffff";
static final String DFA1_maxS =
"\1\164\1\167\1\uffff\2\157\2\162\1\153\1\162\6\uffff\1\160\1\164\1\156"+
"\2\uffff\2\141\1\145\4\uffff\1\145\1\141\1\164\1\156\1\154\1\167\1\141"+
"\1\164\2\uffff\1\163\1\145\1\131\1\164\1\145\1\154\1\132\3\uffff\1\151"+
"\1\132\1\141\3\uffff\1\156\3\uffff\1\164\1\147\1\145\1\55\1\132\1\162"+
"\7\uffff";
static final String DFA1_acceptS =
"\2\uffff\1\3\6\uffff\1\33\1\34\1\35\1\1\1\2\1\4\3\uffff\1\11\1\27\3\uffff"+
"\1\36\1\37\1\30\1\31\10\uffff\1\5\1\32\7\uffff\1\24\1\25\1\26\3\uffff"+
"\1\21\1\22\1\23\1\uffff\1\16\1\17\1\20\6\uffff\1\12\1\13\1\14\1\15\1\6"+
"\1\7\1\10";
static final String DFA1_specialS =
"\106\uffff}>";
static final String[] DFA1_transitionS = {
"\1\1\64\uffff\1\10\1\4\1\11\3\uffff\1\12\1\13\2\uffff\1\2\3\uffff\1\5"+
"\1\uffff\1\3\1\7\1\6",
"\1\14\11\uffff\1\15",
"",
"\1\16\3\uffff\1\17\11\uffff\1\20",
"\1\21",
"\1\23\14\uffff\1\22",
"\1\24",
"\1\27\1\uffff\1\25\1\uffff\1\30\5\uffff\1\26",
"\1\31\5\uffff\1\32",
"",
"",
"",
"",
"",
"",
"\1\33",
"\1\34",
"\1\35",
"",
"",
"\1\36",
"\1\37",
"\1\40",
"",
"",
"",
"",
"\1\41",
"\1\42",
"\1\43\12\uffff\1\44",
"\1\45",
"\1\46",
"\1\47",
"\1\50",
"\1\51",
"",
"",
"\1\52",
"\1\53",
"\1\54\1\55",
"\1\57",
"\1\60",
"\1\61",
"\1\62\1\63\1\64",
"",
"",
"",
"\1\65",
"\1\66\1\67\1\70",
"\1\71",
"",
"",
"",
"\1\72",
"",
"",
"",
"\1\73",
"\1\74",
"\1\75",
"\1\76",
"\1\77\1\100\1\101",
"\1\105\10\uffff\1\103\5\uffff\1\104",
"",
"",
"",
"",
"",
"",
""
};
static final short[] DFA1_eot = DFA.unpackEncodedString(DFA1_eotS);
static final short[] DFA1_eof = DFA.unpackEncodedString(DFA1_eofS);
static final char[] DFA1_min = DFA.unpackEncodedStringToUnsignedChars(DFA1_minS);
static final char[] DFA1_max = DFA.unpackEncodedStringToUnsignedChars(DFA1_maxS);
static final short[] DFA1_accept = DFA.unpackEncodedString(DFA1_acceptS);
static final short[] DFA1_special = DFA.unpackEncodedString(DFA1_specialS);
static final short[][] DFA1_transition;
static {
int numStates = DFA1_transitionS.length;
DFA1_transition = new short[numStates][];
for (int i=0; i
© 2015 - 2025 Weber Informatics LLC | Privacy Policy