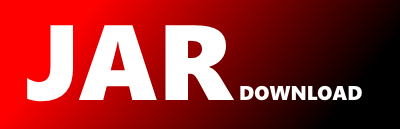
static.scripts.beangle.beangle-ui.js Maven / Gradle / Ivy
/**
* Beangle, Agile Java/Scala Development Scaffold and Toolkit
*
* Copyright (c) 2005-2013, Beangle Software.
*
* Beangle is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* Beangle is distributed in the hope that it will be useful.
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with Beangle. If not, see .
*/
/*----------------------------------------------
* Beangle UI
* include ToolBar,Grid,EntityAction
*/
(function( bg, undefined ) {
bg.alert=function(msg){
alert(msg);
}
bg.uitheme="default"
function NamedFunction(name,func,objectCount){
this.name=name;
this.func=func;
this.objectCount=(null==objectCount)?'ge0':objectCount;
}
/**
* 生成一个工具栏
* @param divId 工具栏对应的div
* @param title 工具栏的标题
* @param imageName 工具栏顶头的图片名称
*/
function ToolBar(divId,title,imageName){
this.itemCount=0;
this.bar=document.getElementById(divId);
if(null==this.bar){
//bg.alert("cannot find div with id " + divId);
return;
}
this.bar.innerHTML="";
this.id=divId;
this.separator=" ";
this.bar.className="toolbar notprint";
var imageRoot=beangle.getContextPath()+"/static/themes/"+ bg.uitheme +"/icons/",
imagePath=imageRoot + "16x16/actions/";
this.setTitle=function(newTitle,imageName){
if(!newTitle) return;
if(imageName==null)imageName="action-info";
this.title_div.innerHTML=genIconElement(null,imageName) + ''+newTitle+"";
}
this.setSeparator=function(separator){
this.separator=separator;
}
/**
* 设置抬头
*/
this.init = function (title,imageName){
var title_div = document.createElement('div'), msg_div, items_div;
title_div.className="toolbar-title";
this.bar.appendChild(title_div);
this.title_div=title_div;
this.setTitle(title,imageName);
items_div = document.createElement('div');
items_div.className="toolbar-items";
items_div.id=this.id+"_items";
this.items_div=items_div;
this.bar.appendChild(items_div);
msg_div = document.createElement('div');
msg_div.className="toolbar-msg";
msg_div.style.display="none";
msg_div.id=this.id+"_msg";
this.bar.appendChild(msg_div);
}
this.init(title,imageName);
this.addHr=function(){
hrdiv=this.appendDiv(null,"toolbar-line");
hrdiv.innerHTML='
';
}
function genIconElement(action,cssClassOrIconPath){
var cssClass ="action-default";
if(null != cssClassOrIconPath){
if(cssClassOrIconPath.indexOf('.')>-1){
if(cssClassOrIconPath.charAt(0)!='/'){
cssClassOrIconPath=imagePath+cssClassOrIconPath;
}
return '
';
}
else cssClass=cssClassOrIconPath;
}
if(null==cssClassOrIconPath && null != action){
if(typeof action == "object") action = action.name;
if(typeof action=="string"){
if(action.indexOf("add")==0 || action.indexOf("batchAdd")==0 ||action.indexOf("new")==0) cssClass= "action-new";
else if(action.indexOf("remove")==0||action.indexOf("delete")==0) cssClass="action-edit-delete";
else if(action.indexOf("update")==0||action.indexOf("edit")==0||action.indexOf("batchEdit")==0) cssClass= "action-update";
else if(action.indexOf("export")==0) cssClass="action-excel";
else if(action.indexOf("copy")==0) cssClass="action-edit-copy";
else if(action.indexOf("print")==0) cssClass= "action-print";
else if(action.indexOf("refresh")==0) cssClass="action-refresh";
else if(action.indexOf("close")==0) cssClass="action-close";
else if(action.indexOf("save")==0) cssClass= "action-save";
else if(action.indexOf("download")==0) cssClass="action-download";
}
}
return '';
}
/**
* 设置按钮的动作
*/
function setAction(item,action){
if(null==action){
bg.alert("action should not be null");
return;
}
if(typeof action=='function'){
item.onclick=action;
return;
}
if(typeof action=='string'){
if (action.indexOf('(')!=-1){
item.onclick= function (){eval(action);}
}
else if(action.indexOf('.action')!=-1){
item.onclick=function (){Go(action)}
}else{
bg.alert("unsuported action description:"+action);
}
}
if(typeof action=='object'){
item.onclick=action.func;
return;
}
}
this.addBack = function (title){
if(null==title){
this.addItem("返回",function (){history.back(-1)},'action-backward');
}else{
this.addItem(title,function (){history.back(-1)},'action-backward');
}
}
this.addHelp = function (module){
this.addItem("帮助",function (){
if(null==module) bg.alert("施工中..");
else window.open("help.action?helpId="+module);
},'action-help-contents');
}
this.addPrint = function (msg){
if(null==msg) this.addItem("打印","print()");
else this.addItem(msg,"print()");
}
this.addClose = function (msg){
if(''==msg|| null==msg) msg="关闭";
this.addItem(msg,"window.close()",'action-close');
}
/**
* 添加按钮
*/
this.addItem = function(title,action,imageName,alt,objectCount){
this.addSeparatorAsNeed();
var item_div = document.createElement('div');
item_div.innerHTML=genIconElement(action,imageName)+title;
item_div.onmouseout=MouseOutItem;
item_div.onmouseover=MouseOverItem;
setAction(item_div,action);
if(!objectCount) { if(typeof action=='object'){objectCount=action.objectCount;}}
if(!objectCount) objectCount='ge0';
item_div.className=("toolbar-item toolbar-item-"+objectCount) + ((objectCount!='ge0')?" toolbar-item-disabled":"");
item_div.title=(alt==null?title:alt);
this.items_div.appendChild(item_div);
this.itemCount++;
return item_div;
}
this.addDiv=function(className){
var newDiv = document.createElement('div');
if(className)newDiv.className=className;
this.items_div.appendChild(newDiv);
return newDiv;
}
this.appendDiv=function(id,className){
var newDiv = document.createElement('div');
if(id)newDiv.setAttribute("id",id);
if(className)newDiv.className=className;
document.getElementById(this.id).appendChild(newDiv);
return newDiv;
}
/**
* 添加分隔符
*
*/
this.addSeparator = function (){
if(this.separator){
this.addDiv("toolbar-separator").innerHTML=this.separator;
}
}
this.addSeparatorAsNeed = function (){
if(this.itemCount!=0){
this.addSeparator();
}
}
this.addBackOrClose = function (backCaption, closeCaption) {
if (parent.location == self.location && (window.history.length <= 1 || window.history.length == null)) {
this.addClose((null == closeCaption) ? "关闭" : closeCaption);
} else {
this.addBack((null == backCaption) ? "后退" : backCaption);
}
}
// 增加空白功能点
this.addBlankItem = function () {
this.addDiv("toolbar-group-separator").innerHTML=" ";
this.itemCount++;
}
/**
* 设置工具栏的消息区
*
*/
this.setMessage = function (msg){
if (typeof msg == "undefined") return;
document.getElementById(this.id+"_msg").innerHTML=msg;
}
/**
* 在工具栏中添加一个菜单
*/
this.addMenu = function(title,action,imageName,alt){
this.addSeparatorAsNeed();
var item_div = document.createElement('div');
item_div.className="toolbar-item";
var menuTableId=this.id+this.itemCount+"_menu";
item_div.id=menuTableId;
item_div.tabIndex = 0;
item_div.title=alt||title;
item_div.onmouseout=MouseOutItem;
item_div.onmouseover=MouseOverItem;
this.items_div.appendChild(item_div);
if(action == null){
item_div.innerHTML=genIconElement(null,imageName) + title + ' '+ genIconElement(null,'action-downarrow');
item_div.onclick=function (event){displayMenu(event);};
}else{
var span1 = document.createElement("span");
span1.innerHTML=genIconElement(action,imageName)+title;
setAction(span1,action);
var span2 = document.createElement("span");
span2.innerHTML=genIconElement(action,"action-downarrow");
span2.onclick = function (event){displayMenu(event);};
item_div.appendChild(span1);
item_div.appendChild(span2);
}
item_div.onblur = function (event){hiddenMenu(event);};
var menu = new Menu(menuTableId,item_div);
this.itemCount++;
return menu;
}
function hiddenMenu(event){
var div=bg.event.getTarget(event);
while(div && div.tagName.toLowerCase()!='div'){
div=div.parentNode;
}
var menu=div.lastElementChild || div.lastChild;
if(null==menu || menu.tagName.toLowerCase()!='table'){alert('menu is null then return and target is '+div);return;}
if(menu.style.visibility!=""&&menu.style.visibility!="hidden"){
for(var i = 0;i < menu.rows.length;i++){
if(menu.rows[i].cells[0].className=='toolbar-menuitem-transfer'){
return;
}
}
menu.style.visibility="hidden";
}
}
function displayMenu(event){
var div=bg.event.getTarget(event);
while(div && div.tagName.toLowerCase()!='div'){
div=div.parentNode;
}
var menu=div.lastElementChild || div.lastChild;
if(null==menu){alert('menu is null then return and target is '+div);return;}
if(menu.style.visibility!=""&&menu.style.visibility!="hidden"){
menu.style.visibility="hidden";
div.className="toolbar-item-transfer";
}else{
menu.style.visibility="visible";
div.className="toolbar-item-selected";
}
}
/**
* 生成一个菜单
*/
function Menu(id,item_div){
var table=document.createElement("table");
table.className="toolbar-menu";
table.id=id+"Table";
var mytablebody = document.createElement("tbody");
table.appendChild(mytablebody);
if (jQuery("#" + id).find("span").length>0) {
table.onclick = function (event){displayMenu(event);};
}
item_div.appendChild(table);
this.table=table;
/**
* 在菜单中添加一个条目
*/
this.addItem = function (title,action,imageName,alt){
var itemTd = document.createElement('td');
itemTd.innerHTML=genIconElement(action,imageName)+title;
itemTd.onmouseout=MouseOutMenuItem;
itemTd.onmouseover=MouseOverMenuItem;
itemTd.title=alt||title;
setAction(itemTd,action);
itemTd.className="toolbar-menuitem";
itemTd.width="100%";
var tr = document.createElement('tr');
tr.appendChild(itemTd);
if(this.table.tBodies.length==0) this.table.appendChild(document.createElement("tbody"));
this.table.tBodies[0].appendChild(tr);
}
}
// /菜单条目的鼠标进入和离开事件响应方法
function MouseOutMenuItem(e){
var o=bg.event.getTarget(e);
while (o && o.tagName.toLowerCase()!="td"){o=o.parentNode;}
if(o)o.className="toolbar-menuitem";
}
function MouseOverMenuItem(e){
var o=bg.event.getTarget(e);
while (o && o.tagName.toLowerCase()!="td"){o=o.parentNode;}
if(o)o.className="toolbar-menuitem-transfer";
}
/**
* 当鼠标经过工具栏的按钮时
*
*/
function MouseOverItem(e){
var o=bg.event.getTarget(e);
while (o&&o.tagName.toLowerCase()!="div"){o=o.parentNode;}
if(o) jQuery(o).removeClass("toolbar-item").addClass("toolbar-item-transfer");
}
/**
* 当鼠标离开工具栏的按钮时
*/
function MouseOutItem(e){
var o=bg.event.getTarget(e);
while (o&&o.tagName.toLowerCase()!="div"){o=o.parentNode;}
if(o) jQuery(o).removeClass("toolbar-item-transfer").removeClass("toolbar-item-selected").addClass("toolbar-item");
}
}
bg.extend({'ui.toolbar':function (divId,title,imageName){
return new ToolBar(divId,title,imageName);
}
});
bg.extend({'ui.gridbar':function(divIds,title){
this.divIds=divIds;
this.pageId=null;
this.title=title;
this.toolbars=[];
for(var i=0;i1){
addAnchor(titles['first'],1);
addAnchor(titles['previous'],onePage.pageNo-1);
}
var labelspan=document.createElement('span');
labelspan.innerHTML="" + onePage.startNo +" - "+ onePage.endNo + " of " + onePage.total + "";
labelspan.style.padding="0px 2px 0px 2px";
pageDiv.appendChild(labelspan);
var numSpan=jQuery(labelspan)
numSpan.attr('title',titles['change'])
numSpan.mouseover(function (){this.className='pgbar-label'});
numSpan.mouseout(function(){this.className=''});
// 为了防止其他信息上下移动错误
numSpan.click(function(){this.parentNode.style.marginTop="0px";this.nextSibling.style.display='';this.style.display='none'});
var pagespan=document.createElement('span');
pagespan.style.display="none";
//add pagesize select
if(ranks && (ranks.length>0)){
var pageNoSelect=document.createElement('select');
pageNoSelect.id=pageDiv.id+"_select";
pagespan.appendChild(pageNoSelect);
pageNoSelect.className="pgbar-selbox";
pageNoSelect.title=titles['pagesize']||'Page Size';
var selectIndex=0;
for(var i=0;i=2) {
if(jQuery(this).hasClass("toolbar-item-e1")) jQuery(this).addClass('toolbar-item-disabled');
else jQuery(this).removeClass('toolbar-item-disabled');
} else if(selectedCount==1) {
if(jQuery(this).hasClass("toolbar-item-ge2")) jQuery(this).addClass('toolbar-item-disabled');
else jQuery(this).removeClass('toolbar-item-disabled');
} else{
if(jQuery(this).hasClass("toolbar-item-ge0")) jQuery(this).removeClass('toolbar-item-disabled');
else jQuery(this).addClass('toolbar-item-disabled');
}
};
if(bg.ui.grid.enableDynaBar){
jQuery('#'+gridId+'_bar1_items .toolbar-item').each(changeToolbarItem);
jQuery('#'+gridId+'_bar2_items .toolbar-item').each(changeToolbarItem);
}
if(bg.ui.grid.enableSelectTip){
if(selectedCount>1) bg.ui.grid.setGridMessage(gridId,"已选 "+selectedCount+" 条");
else bg.ui.grid.setGridMessage(gridId,"");
}
},
/**
* 行选函数。单击行内的任一处,可以选定行头的checkbox或者radio 用法:onclick="toggleRow(event)"
*/
toggleRow : function (event){
var ele = bg.event.getTarget(event);
//find fired grid table
var ownGridTable=ele;
while(ownGridTable.tagName != null && ownGridTable.tagName.toLowerCase()!="table"){
ownGridTable=ownGridTable.parentNode;
if(null==ownGridTable) break;
}
var changed=true;
var firstCell=null;
var isFireOnBoxCell=false;
if(null!=ele && ele.tagName.toLowerCase()=="td"){
firstCell = ele.parentNode.firstChild;
//find first cell
while(firstCell.tagName == null || firstCell.tagName.toLowerCase()!="td"){
firstCell=firstCell.nextSibling;
}
isFireOnBoxCell=(ele==firstCell);
//shall we reserve other select
// find box input
ele=firstCell.firstChild;
while(((typeof ele.tagName)=="undefined")||ele.tagName.toLowerCase()!="input"){
ele=ele.nextSibling;
if(ele==null)return;
}
ele.checked = !ele.checked;
}else if((ele.type=="checkbox")||(ele.type=="radio")){
firstCell=ele.parentNode;
isFireOnBoxCell=true;
}else{
changed=false;
}
if(null==ele || null==firstCell || null==ownGridTable || !changed) return;
// 改变选定行的颜色
var row=firstCell.parentNode;
if((typeof row.className)=="undefined") return;
if(ele.checked) jQuery(row).removeClass("griddata-over").addClass("griddata-selected");
else jQuery(row).removeClass("griddata-selected").addClass("griddata-over");
var selectedCount=0;
if(ele.type=="radio") {
if(ele.checked) selectedCount=1;
}else{
var isReserveOtherSelect = !bg.ui.grid.enableSingleRowSelect || isFireOnBoxCell || event.ctrlKey ;
jQuery("#"+ownGridTable.id + " .gridselect").each(function(){
if(jQuery(this).find("input").is(":checked")){
if(firstCell != this && !isReserveOtherSelect){
jQuery(this).find("input").prop("checked",false);
jQuery(this).parent("tr").removeClass("griddata-selected");
}else{
selectedCount++;
}
}
});
if(!isReserveOtherSelect){
jQuery("#"+ownGridTable.id + " .gridselect-top").each(function(){
jQuery(this).find("input").prop("checked",false);
});
}
}
bg.ui.grid.notifyGridbar(ownGridTable.id,selectedCount);
// 激发自定义事件
if(typeof ele.onchange =="function") ele.onchange();
},
//列排序对应的onePage和选中的列
sort : function (onePage,ele){
if(null==onePage){
bg.alert("无法找到元素对应的排序表格!");return;
}
var orderByStr=null;
if(ele.className=="gridhead-sortable"){
if(typeof ele.asc!="undefined"){
orderByStr=ele.asc;
}
else{
orderByStr=ele.id+" asc";
}
}else if(ele.className=="gridhead-asc"){
if(typeof ele.desc!="undefined"){
orderByStr=ele.desc;
}
else{
orderByStr=ele.id.replace(/\,/g," desc,")+" desc";
}
}else{
orderByStr="";
}
onePage.goPage(1,null,orderByStr);
},
/**
* 初始化排序表格
* 此函数主要是向已经待排序表格的列头1)添加鼠标事件响应和显示效果. 2)负责将事件传递到用户定义的函数中.
*
* 凡是要排序的列,请注名排序单元格的id 和class. 其中id是排序要传递的字段,class为定值gridhead-kable.
* 除此之外,用户(使用该方法的人)需要自定义一个钩子函数"sortBy(what)",以备调用.
*
* @param tableId 待排序表格的id
* @param onePage 与表格对应的page对象
*/
init : function (tableId,onePage){
var table= document.getElementById(tableId), thead = table.tHead, tbody, orderBy, columnSort ,i ,j, head, row, cell, desc, asc, orignRowCls;
if(!thead || thead.rows.length==0){
//bg.alert("sortTable ["+tableId+"] without thead");
return;
}
orderBy=onePage.orderby;
columnSort = function(){// this is a td/th
bg.ui.grid.sort(onePage,this);
}
for(j=0;j'
continue;
}
asc = cell.id+" asc";
if(typeof cell.asc !="undefined"){
asc = cell.asc;
}
if(orderBy==asc){
cell.className="gridhead-asc"
cell.innerHTML=cell.innerHTML+''
continue;
}
}
}
}
tbody=document.getElementById(tableId+"_data");
if(!tbody) return;
for(j=0;j7) emptyCnt=7;
heightpx=emptyCnt*16;
if(size==0){
emptyLabel=document.createElement("div");
emptyLabel.innerHTML=(msg||'No result matched your search.');
emptyLabel.style.paddingTop=heightpx/2-16 +"px";
emptydiv.appendChild(emptyLabel);
}
emptydiv.style.height=heightpx+"px";
}
}
});
// Action---------------------------------------------------------------------
//this.action,this.paramstring,this.target
function EntityAction(entity,onePage){
this.entity=entity;
this.page=onePage;
this.formid="form_" + bg.randomInt();
//record self for closure method
var selfaction = this;
function applyMethod(action,method){
var last1=action.lastIndexOf("!"), lastDot=action.lastIndexOf("."), shortAction=action, sufix="";
if(-1 == last1) last1 = lastDot;
if(-1!=last1){
shortAction=action.substring(0,last1);
}
if(-1!=lastDot){
sufix=action.substring(lastDot);
}
return shortAction+"!"+method+sufix;
}
this.getForm=function (){
return this.page.getForm();
};
this.addParam = function(name,value){
bg.form.addInput(this.getForm(),name,value);
}
if(null!=this.page.target&&''!=this.page.target){
var fm = this.getForm();
if(null!=fm) fm.target=this.page.target;
}
this.beforeSubmmitId = function(method) {
var ids = bg.input.getCheckBoxValues(entity+".id");
if (ids == null || ids == "") {
bg.alert("你没有选择要操作的记录!");
return false;
}
var form=this.getForm();
form.action = applyMethod(this.page.actionurl, method);
if(this.page.paramstr){
bg.form.addHiddens(form,this.page.paramstr);
bg.form.addParamsInput(form,this.page.paramstr);
}
return true;
}
this.submitIdAction=function (method,multiId,confirmMsg,ajax){
if (this.beforeSubmmitId(method)) {
if(null!=confirmMsg && ''!=confirmMsg){
if(!confirm(confirmMsg))return;
}
bg.form.submitId(this.getForm(),this.entity + ".id",multiId,null,null,ajax);
}
}
this.remove=function(confirmMsg){
confirmMsg=confirmMsg||'确认删除?';
return new NamedFunction('remove',function(){
selfaction.submitIdAction('remove',true,confirmMsg);
},bg.ui.grid.enableDynaBar?'ge1':'ge0');
}
this.add = function(){
return new NamedFunction('add',function(){
var form=selfaction.getForm();
if(""!=selfaction.page.paramstr) bg.form.addHiddens(form,selfaction.page.paramstr);
bg.form.addInput(form,selfaction.entity + '.id',"");
if(""!=selfaction.page.paramstr) bg.form.addParamsInput(form,selfaction.page.paramstr);
bg.form.submit(form,applyMethod(selfaction.page.actionurl,"edit"));
});
}
this.info = function(){
return new NamedFunction('info',function(){
selfaction.submitIdAction('info',false)
},bg.ui.grid.enableDynaBar?'e1':'ge0');
}
this.edit = function (){
return new NamedFunction('edit',function(){
selfaction.submitIdAction('edit',false);
},bg.ui.grid.enableDynaBar?'e1':'ge0');
}
this.single = function(methodName,confirmMsg,extparams){
return new NamedFunction(methodName,function(){
var form=selfaction.getForm();
if(null!=extparams) bg.form.addHiddens(form,extparams);
selfaction.submitIdAction(methodName,false,confirmMsg);
},bg.ui.grid.enableDynaBar?'e1':'ge0');
}
this.multi = function(methodName,confirmMsg,extparams,ajax){
return new NamedFunction(methodName,function(){
try {
var form = selfaction.getForm();
if(null!=extparams) bg.form.addHiddens(form, extparams);
selfaction.submitIdAction(methodName, true, confirmMsg,ajax);
}catch(e){
bg.alert(e)
}
},bg.ui.grid.enableDynaBar?'ge1':'ge0');
}
this.method=function(methodName,confirmMsg,extparams,ajax){
return new NamedFunction(methodName,function(){
var form=selfaction.getForm();
if(null!=confirmMsg && ''!=confirmMsg){
if(!confirm(confirmMsg))return;
}
if(null!=extparams){
bg.form.addHiddens(form,extparams);
}
if(""!=selfaction.page.paramstr){
bg.form.addHiddens(form,selfaction.page.paramstr);
bg.form.addParamsInput(form,selfaction.page.paramstr);
}
bg.form.submit(form,applyMethod(selfaction.page.actionurl ,methodName),null,null,ajax);
});
}
this.exportData=function(properties,format,extparams){
format = format || "xls";
properties = properties||"";
extparams = extparams||"";
if(extparams.indexOf("&") != 0) extparams = "&" + extparams;
extparams = "&format=" + format +"&properties=" + properties + extparams;
return selfaction.method('export',null,extparams,false);
}
}
bg.extend({entityaction:EntityAction});
bg.extend({'ui.module':{
moduleClick:function (moudleId){
var id= document.getElementById(moudleId);
if(id.className=="module collapsed"){
id.className="module expanded";
}else{
id.className="module collapsed";
}
}
}
});
bg.extend({'ui.load':
function (module,callback){
var base="/static";
if(bg.getContextPath().length>1)
base = bg.getContextPath() + base;
if(module=="validity"){
jQuery.struts2_jquery.requireCss("/themes/" + bg.uitheme + "/jquery.validity.css",base);
jQuery.struts2_jquery.require("/scripts/plugins/jquery-validity.js",null,base);
jQuery.struts2_jquery.require("/scripts/i18n/zh_CN/jquery.validity.js",callback,base);
}else if(module=="tabletree"){
jQuery.struts2_jquery.requireCss("/themes/" + bg.uitheme + "/beangle-ui-tabletree.css",base);
jQuery.struts2_jquery.require("/scripts/beangle/beangle-ui-tabletree.js",callback,base);
}else if(module=="colorbox"){
jQuery.struts2_jquery.requireCss("/themes/" + bg.uitheme + "/colorbox.css",base);
jQuery.struts2_jquery.require("/scripts/plugins/jquery-colorbox.min.js",callback,base);
}else if(module=="jquery.pstrength"){
jQuery.struts2_jquery.requireCss("/themes/" + bg.uitheme + "/jquery-pstrength.css",base);
jQuery.struts2_jquery.require("/scripts/plugins/jquery-pstrength.js",callback,base);
jQuery.struts2_jquery.require("/scripts/i18n/zh_CN/jquery-pstrength.js",callback,base);
}
}
});
})(beangle);
© 2015 - 2025 Weber Informatics LLC | Privacy Policy