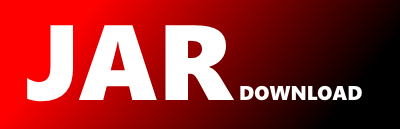
open_admin_style.js.admin.lib.blc-conditions-builder.js Maven / Gradle / Ivy
/**
* Broadleaf Commerce Conditions Rule Builder
* Javascript Conditions Builder that handles both simple targeting rules
* and complex quantitative item rules.
* @author: elbertbautista
*
* Based off the Javascript component "business-rules"
* @author: chris j. powers
* https://github.com/chrisjpowers/business-rules
* Copyright 2013 Chris Powers
* http://chrisjpowers.com
*
* Permission is hereby granted, free of charge, to any person obtaining
* a copy of this software and associated documentation files (the
* "Software"), to deal in the Software without restriction, including
* without limitation the rights to use, copy, modify, merge, publish,
* distribute, sublicense, and/or sell copies of the Software, and to
* permit persons to whom the Software is furnished to do so, subject to
* the following conditions:
*
* The above copyright notice and this permission notice shall be
* included in all copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
* EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
* MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
* NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE
* LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION
* OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION
* WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
*
*/
(function($) {
var uniqueModifier = 1;
$.fn.conditionsBuilder = function(options) {
if(options == "data") {
var builder = $(this).eq(0).data("conditionsBuilder");
return builder.collectData();
} else if (options == "builder") {
var builder = $(this).eq(0).data("conditionsBuilder");
return builder;
} else {
return $(this).each(function() {
var builder = new ConditionsBuilder(this, options);
$(this).data("conditionsBuilder", builder);
});
}
};
function ConditionsBuilder(element, options) {
this.element = $(element);
this.options = options || {};
this.init();
}
ConditionsBuilder.prototype = {
init: function() {
this.fields = this.options[0].fields;
this.data = this.options[1].data;
this.error = this.options[1].error;
var rules = this.buildRules(this.data);
this.element.html(rules);
this.element.find(".conditional-rules").unwrap();
if (this.data[0] != null && this.data[0].quantity != null) {
var addMainConditionLink = this.getAddMainConditionLink();
this.element.append(addMainConditionLink);
}
},
collectData: function() {
var elements = this.element.find("> .conditional-rules > .conditional");
var dataWrapper = {};
dataWrapper.data = [];
for (var i=0;i .all-any-none-wrapper > .all-any-none").val();
if ("all" == klass) {klass = "AND";}
if ("any" == klass) {klass = "OR";}
if ("none" == klass) {klass = "NOT";}
qty = $element.find("> .all-any-none-wrapper > .conditional-qty").val();
id = $element.find("> .all-any-none-wrapper > .conditional-id").val();
}
if (klass) {
var out = {};
if (qty) {
out.quantity = qty;
} else {
out.quantity = null;
}
if (id) {
out.id = id;
} else {
out.id = null;
}
out.groupOperator = klass;
out.groups = [];
$element.find("> .conditional-rules > .conditional, > .rule").each(function() {
var data = _this.collectDataFromNode($(this));
if (data != null) {
out.groups.push(data);
}
});
return out;
} else {
var value;
var trueRadio = $element.find(".true");
var falseRadio = $element.find(".false");
if (trueRadio != null && trueRadio.is(':checked')) {
value = "true";
} else if (falseRadio != null && falseRadio.is(':checked')) {
value = "false";
} else {
value = $element.find(".value").val();
}
return {
id:null,
quantity:null,
groupOperator:null,
groups:[],
name: $element.find(".field").val(),
operator: $element.find(".operator").val(),
value: value,
start: $element.find(".start").val(),
end: $element.find(".end").val()
};
}
},
buildAddNewRule: function(rules) {
var _this = this;
var f = _this.fields[0];
var newField = {id:null, quantity:null, groupOperator: "AND", groups: []};
rules.append(_this.buildConditional(newField));
},
buildAddNewItemRule: function(rules, isAdditional) {
var _this = this;
var f = _this.fields[0];
var newField = {id:null, quantity:1, groupOperator: "AND", groups: []};
if (!isAdditional) {
rules.append(_this.buildConditional(newField, isAdditional));
var addMainConditionLink = this.getAddMainConditionLink();
rules.append(addMainConditionLink);
} else {
rules.children(':last').before(_this.buildConditional(newField, isAdditional));
}
},
buildRules: function(ruleDataArray) {
var container = $("");
for (var i=0; i", {"class": "conditional-rules"});
var kind;
if(ruleData.groupOperator == "AND") { kind = "all"; }
else if(ruleData.groupOperator == "OR") { kind = "any"; }
else if (ruleData.groupOperator == "NOT") { kind = "none"; }
if(!kind) { return; }
var div = $("", {"class": "conditional " + kind});
var selectWrapper = $("", {"class": "all-any-none-wrapper"});
selectWrapper.append($("", {text: "Match", "class": "conditional-spacer"}));
var qty = ruleData.quantity;
if (qty != null) {
var quantity = $("", {"class": "conditional-qty", "type": "text", "value": qty});
selectWrapper.append(quantity);
selectWrapper.append($("", {text: "of", "class": "conditional-spacer"}));
}
var id = ruleData.id;
if (id != null) {
var idHidden = $("", {"class": "conditional-id", "type": "hidden", "value": id});
selectWrapper.append(idHidden);
}
var removeLink = $("", {"class": "remove tiny secondary radius button remove-subcondition", "href": "#", "text": "X"});
removeLink.click(function(e) {
e.preventDefault();
$(this).parent().remove();
});
div.append(removeLink);
var select = $("