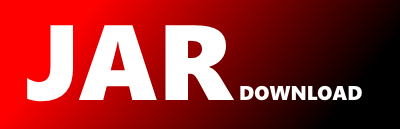
goog.labs.html.scrubber_test.js Maven / Gradle / Ivy
Show all versions of google-closure-library
// Copyright 2013 The Closure Library Authors. All Rights Reserved.
//
// Licensed under the Apache License, Version 2.0 (the "License");
// you may not use this file except in compliance with the License.
// You may obtain a copy of the License at
//
// http://www.apache.org/licenses/LICENSE-2.0
//
// Unless required by applicable law or agreed to in writing, software
// distributed under the License is distributed on an "AS-IS" BASIS,
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// See the License for the specific language governing permissions and
// limitations under the License.
goog.provide('goog.html.ScrubberTest');
goog.require('goog.labs.html.scrubber');
goog.require('goog.object');
goog.require('goog.string');
goog.require('goog.testing.jsunit');
goog.setTestOnly('goog.html.ScrubberTest');
var tagWhitelist = goog.object.createSet(
'a', 'b', 'i', 'p', 'font', 'hr', 'br', 'span', 'ol', 'ul', 'li', 'table',
'tr', 'td', 'th', 'tbody', 'h1', 'h2', 'h3', 'h4', 'h5', 'h6', 'img',
'html', 'head', 'body', 'title');
var attrWhitelist = {
// On any element,
'*': {
// allow unfiltered title attributes, and
'title': function(title) { return title; },
// specific dir values.
'dir': function(dir) { return dir === 'ltr' || dir === 'rtl' ? dir : null; }
},
// Specifically on elements,
'a': {
// allow an href but verify and rewrite, and
'href': function(href) {
return /^https?:\/\/google\.[a-z]{2,3}\/search\?/.test(href) ?
href.replace(
/[^A-Za-z0-9_\-.~:\/?#\[\]@!\$&()*+,;=%]+/, encodeURIComponent) :
null;
},
// mask the generic title handler for no good reason.
'title': function(title) { return '<' + title + '>'; }
}
};
function run(input, golden, desc) {
var actual =
goog.labs.html.scrubber.scrub(tagWhitelist, attrWhitelist, input);
assertEquals(desc, golden, actual);
}
function testEmptyString() {
run('', '', 'Empty string');
}
function testHelloWorld() {
run('Hello, World!', 'Hello, World!', 'Hello World');
}
function testNoEndTag() {
run('Hello, World!', 'Hello, World!',
'Hello World no end tag');
}
function testUnclosedTags() {
run('Hello, <>! ' +
'Hello,
<>!',
'Hello, <>! ' +
'Hello,
<>!
',
'RCDATA content, different case, unclosed tags');
}
function testListInList() {
run('- foo
- bar
',
'- foo
- bar
',
'list in list directly');
}
function testHeaders() {
run('header
body' +
'sub-headersub-body' +
'sub-sub-header
sub-sub-body
',
'header
body' +
'sub-header
sub-body' +
'sub-sub-header
sub-sub-body',
'headers');
}
function testListNesting() {
run('- foo
- bar',
'
- foo
- bar
',
'list nesting');
}
function testTableNesting() {
run('foo bar
',
'foo ' +
'bar
' +
'
',
'table nesting');
}
function testNestingLimit() {
run(goog.string.repeat('', 264) + goog.string.repeat('', 264),
goog.string.repeat('', 256) + goog.string.repeat('', 256),
'264 open spans');
}
function testTableScopes() {
run('Hi
How are you
\n' +
'' +
'Cell
\n ' +
'Cell
\n ' +
'
\n' +
'x
',
'Hi
How are you
\n' +
'' +
'Cell
\n ' +
// The close tag does not close the whole table. +
'Cell
\n ' +
'
\n' +
'x
',
'Table Scopes');
}
function testConcatSafe() {
run('<