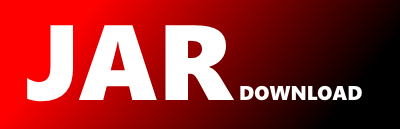
goog.transpile.js Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of google-closure-library
Show all versions of google-closure-library
The Google Closure Library is a collection of JavaScript code
designed for use with the Google Closure JavaScript Compiler.
This non-official distribution was prepared by the ClojureScript
team at http://clojure.org/
// Copyright 2016 The Closure Library Authors. All Rights Reserved.
//
// Licensed under the Apache License, Version 2.0 (the "License");
// you may not use this file except in compliance with the License.
// You may obtain a copy of the License at
//
// http://www.apache.org/licenses/LICENSE-2.0
//
// Unless required by applicable law or agreed to in writing, software
// distributed under the License is distributed on an "AS-IS" BASIS,
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// See the License for the specific language governing permissions and
// limitations under the License.
// NOTE: This is a generated file. Do not edit.
// clang-format off
/** @fileoverview @nocompile */
var $jscomp={};$jscomp.scope={};$jscomp.getGlobal=function(maybeGlobal){return typeof window!="undefined"&&window===maybeGlobal?maybeGlobal:typeof global!="undefined"?global:maybeGlobal};$jscomp.global=$jscomp.getGlobal(this);$jscomp.initSymbol=function(){if(!$jscomp.global.Symbol)$jscomp.global.Symbol=$jscomp.Symbol;$jscomp.initSymbol=function(){}};$jscomp.symbolCounter_=0;$jscomp.Symbol=function(description){return("jscomp_symbol_"+description+$jscomp.symbolCounter_++)};
$jscomp.initSymbolIterator=function(){$jscomp.initSymbol();if(!$jscomp.global.Symbol.iterator)$jscomp.global.Symbol.iterator=$jscomp.global.Symbol("iterator");$jscomp.initSymbolIterator=function(){}};$jscomp.makeIterator=function(iterable){$jscomp.initSymbolIterator();if(iterable[$jscomp.global.Symbol.iterator])return iterable[$jscomp.global.Symbol.iterator]();var index=0;return({next:function(){if(index==iterable.length)return{done:true};else return{done:false,value:iterable[index++]}}})};
$jscomp.arrayFromIterator=function(iterator){var i;var arr=[];while(!(i=iterator.next()).done)arr.push(i.value);return arr};$jscomp.arrayFromIterable=function(iterable){if(iterable instanceof Array)return iterable;else return $jscomp.arrayFromIterator($jscomp.makeIterator(iterable))};
$jscomp.inherits=function(childCtor,parentCtor){function tempCtor(){}tempCtor.prototype=parentCtor.prototype;childCtor.prototype=new tempCtor;childCtor.prototype.constructor=childCtor;for(var p in parentCtor)if($jscomp.global.Object.defineProperties){var descriptor=$jscomp.global.Object.getOwnPropertyDescriptor(parentCtor,p);if(descriptor)$jscomp.global.Object.defineProperty(childCtor,p,descriptor)}else childCtor[p]=parentCtor[p]};$jscomp.array=$jscomp.array||{};
$jscomp.array.done_=function(){return{done:true,value:void 0}};
$jscomp.array.arrayIterator_=function(array,func){if(array instanceof String)array=String(array);var i=0;$jscomp.initSymbol();$jscomp.initSymbolIterator();var $jscomp$compprop0={};var iter=($jscomp$compprop0.next=function(){if(istart)if(--opt_end in this)this[--target]=this[opt_end];else delete this[target]}return this};
$jscomp.array.copyWithin$install=function(){$jscomp.array.installHelper_("copyWithin",$jscomp.array.copyWithin)};$jscomp.array.fill=function(value,opt_start,opt_end){opt_start=opt_start===undefined?0:opt_start;var length=this.length||0;if(opt_start<0)opt_start=Math.max(0,length+(opt_start));if(opt_end==null||opt_end>length)opt_end=length;opt_end=+opt_end;if(opt_end<0)opt_end=Math.max(0,length+opt_end);for(var i=+(opt_start||0);i>>0;if(x===0)return 32;var result=0;if((x&4294901760)===0){x<<=16;result+=16}if((x&4278190080)===0){x<<=8;result+=8}if((x&4026531840)===0){x<<=4;result+=4}if((x&3221225472)===0){x<<=2;result+=2}if((x&2147483648)===0)result++;return result};$jscomp.math.imul=function(a,b){a=Number(a);b=Number(b);var ah=a>>>16&65535;var al=a&65535;var bh=b>>>16&65535;var bl=b&65535;var lh=ah*bl+al*bh<<16>>>0;return al*bl+lh|0};
$jscomp.math.sign=function(x){x=Number(x);return x===0||isNaN(x)?x:x>0?1:-1};$jscomp.math.log10=function(x){return Math.log(x)/Math.LN10};$jscomp.math.log2=function(x){return Math.log(x)/Math.LN2};$jscomp.math.log1p=function(x){x=Number(x);if(x<.25&&x>-.25){var y=x;var d=1;var z=x;var zPrev=0;var s=1;while(zPrev!=z){y*=x;s*=-1;z=(zPrev=z)+s*y/++d}return z}return Math.log(1+x)};
$jscomp.math.expm1=function(x){x=Number(x);if(x<.25&&x>-.25){var y=x;var d=1;var z=x;var zPrev=0;while(zPrev!=z){y*=x/++d;z=(zPrev=z)+y}return z}return Math.exp(x)-1};$jscomp.math.cosh=function(x){x=Number(x);return(Math.exp(x)+Math.exp(-x))/2};$jscomp.math.sinh=function(x){x=Number(x);if(x===0)return x;return(Math.exp(x)-Math.exp(-x))/2};$jscomp.math.tanh=function(x){x=Number(x);if(x===0)return x;var y=Math.exp(2*-Math.abs(x));var z=(1-y)/(1+y);return x<0?-z:z};
$jscomp.math.acosh=function(x){x=Number(x);return Math.log(x+Math.sqrt(x*x-1))};$jscomp.math.asinh=function(x){x=Number(x);if(x===0)return x;var y=Math.log(Math.abs(x)+Math.sqrt(x*x+1));return x<0?-y:y};$jscomp.math.atanh=function(x){x=Number(x);return($jscomp.math.log1p(x)-$jscomp.math.log1p(-x))/2};
$jscomp.math.hypot=function(x,y,rest){var $jscomp$restParams=[];for(var $jscomp$restIndex=2;$jscomp$restIndex1E100||max<1E-100){x=x/max;y=y/max;var sum=x*x+y*y;for(var $jscomp$iter$5=$jscomp.makeIterator(rest$11),$jscomp$key$z=$jscomp$iter$5.next();!$jscomp$key$z.done;$jscomp$key$z=$jscomp$iter$5.next()){var z$12=$jscomp$key$z.value;z$12=Number(z$12)/max;sum+=z$12*z$12}return Math.sqrt(sum)*max}else{var sum$13=x*x+y*y;for(var $jscomp$iter$6=$jscomp.makeIterator(rest$11),$jscomp$key$z=$jscomp$iter$6.next();!$jscomp$key$z.done;$jscomp$key$z=$jscomp$iter$6.next()){var z$14=$jscomp$key$z.value;
z$14=Number(z$14);sum$13+=z$14*z$14}return Math.sqrt(sum$13)}};$jscomp.math.trunc=function(x){x=Number(x);if(isNaN(x)||x===Infinity||x===-Infinity||x===0)return x;var y=Math.floor(Math.abs(x));return x<0?-y:y};$jscomp.math.cbrt=function(x){if(x===0)return x;x=Number(x);var y=Math.pow(Math.abs(x),1/3);return x<0?-y:y};$jscomp.number=$jscomp.number||{};$jscomp.number.isFinite=function(x){if(typeof x!=="number")return false;return!isNaN(x)&&x!==Infinity&&x!==-Infinity};
$jscomp.number.isInteger=function(x){if(!$jscomp.number.isFinite(x))return false;return x===Math.floor(x)};$jscomp.number.isNaN=function(x){return typeof x==="number"&&isNaN(x)};$jscomp.number.isSafeInteger=function(x){return $jscomp.number.isInteger(x)&&Math.abs(x)<=$jscomp.number.MAX_SAFE_INTEGER};$jscomp.number.EPSILON=Math.pow(2,-52);$jscomp.number.MAX_SAFE_INTEGER=9007199254740991;$jscomp.number.MIN_SAFE_INTEGER=-9007199254740991;$jscomp.object=$jscomp.object||{};
$jscomp.object.assign=function(target,sources){var $jscomp$restParams=[];for(var $jscomp$restIndex=1;$jscomp$restIndex1114111||
code!==Math.floor(code))throw new RangeError("invalid_code_point "+code);if(code<=65535)result+=String.fromCharCode(code);else{code-=65536;result+=String.fromCharCode(code>>>10&1023|55296);result+=String.fromCharCode(code&1023|56320)}}return result};
$jscomp.string.repeat=function(copies){$jscomp.string.noNullOrUndefined_(this,"repeat");var string=String(this);if(copies<0||copies>1342177279)throw new RangeError("Invalid count value");copies=copies|0;var result="";while(copies){if(copies&1)result+=string;if(copies>>>=1)string+=string}return result};$jscomp.string.repeat$install=function(){if(!String.prototype.repeat)String.prototype.repeat=$jscomp.string.repeat};
$jscomp.string.codePointAt=function(position){$jscomp.string.noNullOrUndefined_(this,"codePointAt");var string=String(this);var size=string.length;position=Number(position)||0;if(!(position>=0&&position56319||position+1===size)return first;var second=string.charCodeAt(position+1);if(second<56320||second>57343)return first;return(first-55296)*1024+second+9216};
$jscomp.string.codePointAt$install=function(){if(!String.prototype.codePointAt)String.prototype.codePointAt=$jscomp.string.codePointAt};$jscomp.string.includes=function(searchString,opt_position){opt_position=opt_position===undefined?0:opt_position;$jscomp.string.noRegExp_(searchString,"includes");$jscomp.string.noNullOrUndefined_(this,"includes");var string=String(this);return string.indexOf(searchString,opt_position)!==-1};
$jscomp.string.includes$install=function(){if(!String.prototype.includes)String.prototype.includes=$jscomp.string.includes};
$jscomp.string.startsWith=function(searchString,opt_position){opt_position=opt_position===undefined?0:opt_position;$jscomp.string.noRegExp_(searchString,"startsWith");$jscomp.string.noNullOrUndefined_(this,"startsWith");var string=String(this);searchString=searchString+"";var strLen=string.length;var searchLen=searchString.length;var i=Math.max(0,Math.min(opt_position|0,string.length));var j=0;while(j=searchLen};
$jscomp.string.startsWith$install=function(){if(!String.prototype.startsWith)String.prototype.startsWith=$jscomp.string.startsWith};
$jscomp.string.endsWith=function(searchString,opt_position){$jscomp.string.noRegExp_(searchString,"endsWith");$jscomp.string.noNullOrUndefined_(this,"endsWith");var string=String(this);searchString=searchString+"";if(opt_position===void 0)opt_position=string.length;var i=Math.max(0,Math.min(opt_position|0,string.length));var j=searchString.length;while(j>0&&i>0)if(string[--i]!=searchString[--j])return false;return j<=0};
$jscomp.string.endsWith$install=function(){if(!String.prototype.endsWith)String.prototype.endsWith=$jscomp.string.endsWith};(function(global,jscomp){if(global["$jscomp"])for(var key in global["$jscomp"])jscomp[key]=global["$jscomp"][key];global["$jscomp"]=jscomp})(window,$jscomp);function com_google_javascript_jscomp_gwt_TranspilerGwtModule(){var Jb="",Kb=0,Lb="gwt.codesvr=",Mb="gwt.hosted=",Nb="gwt.hybrid",Ob="com.google.javascript.jscomp.gwt.TranspilerGwtModule",Pb="__gwt_marker_com.google.javascript.jscomp.gwt.TranspilerGwtModule",Qb='