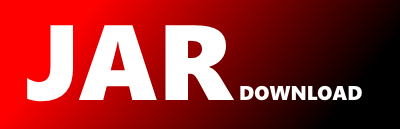
goog.ui.prompt.js Maven / Gradle / Ivy
// Copyright 2006 The Closure Library Authors. All Rights Reserved.
//
// Licensed under the Apache License, Version 2.0 (the "License");
// you may not use this file except in compliance with the License.
// You may obtain a copy of the License at
//
// http://www.apache.org/licenses/LICENSE-2.0
//
// Unless required by applicable law or agreed to in writing, software
// distributed under the License is distributed on an "AS-IS" BASIS,
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// See the License for the specific language governing permissions and
// limitations under the License.
/**
* @fileoverview DHTML prompt to replace javascript's prompt().
*
* @see ../demos/prompt.html
*/
goog.provide('goog.ui.Prompt');
goog.require('goog.Timer');
goog.require('goog.dom');
goog.require('goog.dom.InputType');
goog.require('goog.dom.TagName');
goog.require('goog.events');
goog.require('goog.events.EventType');
goog.require('goog.functions');
goog.require('goog.html.SafeHtml');
goog.require('goog.ui.Component');
goog.require('goog.ui.Dialog');
goog.require('goog.userAgent');
/**
* Creates an object that represents a prompt (used in place of javascript's
* prompt). The html structure of the prompt is the same as the layout for
* dialog.js except for the addition of a text box which is placed inside the
* "Content area" and has the default class-name 'modal-dialog-userInput'
*
* @param {string} promptTitle The title of the prompt.
* @param {string|!goog.html.SafeHtml} promptBody The body of the prompt.
* String is treated as plain text and it will be HTML-escaped.
* @param {Function} callback The function to call when the user selects Ok or
* Cancel. The function should expect a single argument which represents
* what the user entered into the prompt. If the user presses cancel, the
* value of the argument will be null.
* @param {string=} opt_defaultValue Optional default value that should be in
* the text box when the prompt appears.
* @param {string=} opt_class Optional prefix for the classes.
* @param {boolean=} opt_useIframeForIE For IE, workaround windowed controls
* z-index issue by using a an iframe instead of a div for bg element.
* @param {goog.dom.DomHelper=} opt_domHelper Optional DOM helper; see {@link
* goog.ui.Component} for semantics.
* @constructor
* @extends {goog.ui.Dialog}
*/
goog.ui.Prompt = function(
promptTitle, promptBody, callback, opt_defaultValue, opt_class,
opt_useIframeForIE, opt_domHelper) {
goog.ui.Prompt.base(
this, 'constructor', opt_class, opt_useIframeForIE, opt_domHelper);
/**
* The id of the input element.
* @type {string}
* @private
*/
this.inputElementId_ = this.makeId('ie');
this.setTitle(promptTitle);
var label = goog.html.SafeHtml.create(
'label', {'for': this.inputElementId_},
goog.html.SafeHtml.htmlEscapePreservingNewlines(promptBody));
var br = goog.html.SafeHtml.BR;
this.setSafeHtmlContent(goog.html.SafeHtml.concat(label, br, br));
this.callback_ = callback;
this.defaultValue_ = goog.isDef(opt_defaultValue) ? opt_defaultValue : '';
/** @desc label for a dialog button. */
var MSG_PROMPT_OK = goog.getMsg('OK');
/** @desc label for a dialog button. */
var MSG_PROMPT_CANCEL = goog.getMsg('Cancel');
var buttonSet = new goog.ui.Dialog.ButtonSet(opt_domHelper);
buttonSet.set(goog.ui.Dialog.DefaultButtonKeys.OK, MSG_PROMPT_OK, true);
buttonSet.set(
goog.ui.Dialog.DefaultButtonKeys.CANCEL, MSG_PROMPT_CANCEL, false, true);
this.setButtonSet(buttonSet);
};
goog.inherits(goog.ui.Prompt, goog.ui.Dialog);
goog.tagUnsealableClass(goog.ui.Prompt);
/**
* Callback function which is invoked with the response to the prompt
* @type {Function}
* @private
*/
goog.ui.Prompt.prototype.callback_ = goog.nullFunction;
/**
* Default value to display in prompt window
* @type {string}
* @private
*/
goog.ui.Prompt.prototype.defaultValue_ = '';
/**
* Element in which user enters response (HTML text box)
* @type {HTMLInputElement}
* @private
*/
goog.ui.Prompt.prototype.userInputEl_ = null;
/**
* Tracks whether the prompt is in the process of closing to prevent multiple
* calls to the callback when the user presses enter.
* @type {boolean}
* @private
*/
goog.ui.Prompt.prototype.isClosing_ = false;
/**
* Number of rows in the user input element.
* The default is 1 which means use an element.
* @type {number}
* @private
*/
goog.ui.Prompt.prototype.rows_ = 1;
/**
* Number of cols in the user input element.
* The default is 0 which means use browser default.
* @type {number}
* @private
*/
goog.ui.Prompt.prototype.cols_ = 0;
/**
* The input decorator function.
* @type {function(Element)?}
* @private
*/
goog.ui.Prompt.prototype.inputDecoratorFn_ = null;
/**
* A validation function that takes a string and returns true if the string is
* accepted, false otherwise.
* @type {function(string):boolean}
* @private
*/
goog.ui.Prompt.prototype.validationFn_ = goog.functions.TRUE;
/**
* Sets the validation function that takes a string and returns true if the
* string is accepted, false otherwise.
* @param {function(string): boolean} fn The validation function to use on user
* input.
*/
goog.ui.Prompt.prototype.setValidationFunction = function(fn) {
this.validationFn_ = fn;
};
/** @override */
goog.ui.Prompt.prototype.enterDocument = function() {
if (this.inputDecoratorFn_) {
this.inputDecoratorFn_(this.userInputEl_);
}
goog.ui.Prompt.superClass_.enterDocument.call(this);
this.getHandler().listen(
this, goog.ui.Dialog.EventType.SELECT, this.onPromptExit_);
this.getHandler().listen(
this.userInputEl_,
[goog.events.EventType.KEYUP, goog.events.EventType.CHANGE],
this.handleInputChanged_);
};
/**
* @return {HTMLInputElement} The user input element. May be null if the Prompt
* has not been rendered.
*/
goog.ui.Prompt.prototype.getInputElement = function() {
return this.userInputEl_;
};
/**
* Sets an input decorator function. This function will be called in
* #enterDocument and will be passed the input element. This is useful for
* attaching handlers to the input element for specific change events,
* for example.
* @param {function(Element)} inputDecoratorFn A function to call on the input
* element on #enterDocument.
*/
goog.ui.Prompt.prototype.setInputDecoratorFn = function(inputDecoratorFn) {
this.inputDecoratorFn_ = inputDecoratorFn;
};
/**
* Set the number of rows in the user input element.
* A values of 1 means use an `` element. If the prompt is already
* rendered then you cannot change from `` to `
© 2015 - 2025 Weber Informatics LLC | Privacy Policy