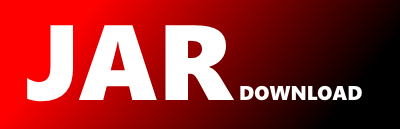
goog.useragent.useragent_test.js Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of google-closure-library
Show all versions of google-closure-library
The Google Closure Library is a collection of JavaScript code
designed for use with the Google Closure JavaScript Compiler.
This non-official distribution was prepared by the ClojureScript
team at http://clojure.org/
// Copyright 2006 The Closure Library Authors. All Rights Reserved.
//
// Licensed under the Apache License, Version 2.0 (the "License");
// you may not use this file except in compliance with the License.
// You may obtain a copy of the License at
//
// http://www.apache.org/licenses/LICENSE-2.0
//
// Unless required by applicable law or agreed to in writing, software
// distributed under the License is distributed on an "AS-IS" BASIS,
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// See the License for the specific language governing permissions and
// limitations under the License.
goog.provide('goog.userAgentTest');
goog.setTestOnly('goog.userAgentTest');
goog.require('goog.array');
goog.require('goog.labs.userAgent.platform');
goog.require('goog.labs.userAgent.testAgents');
goog.require('goog.labs.userAgent.util');
goog.require('goog.testing.PropertyReplacer');
goog.require('goog.testing.jsunit');
goog.require('goog.userAgent');
goog.require('goog.userAgentTestUtil');
var documentMode;
goog.userAgent.getDocumentMode_ = function() {
return documentMode;
};
var propertyReplacer = new goog.testing.PropertyReplacer();
var UserAgents =
{GECKO: 'GECKO', IE: 'IE', OPERA: 'OPERA', WEBKIT: 'WEBKIT', EDGE: 'EDGE'};
function tearDown() {
goog.labs.userAgent.util.setUserAgent(null);
documentMode = undefined;
propertyReplacer.reset();
}
/**
* Test browser detection for a user agent configuration.
* @param {Array} expectedAgents Array of expected userAgents.
* @param {string} uaString User agent string.
* @param {string=} opt_product Navigator product string.
* @param {string=} opt_vendor Navigator vendor string.
*/
function assertUserAgent(expectedAgents, uaString, opt_product, opt_vendor) {
var mockGlobal = {
'navigator':
{'userAgent': uaString, 'product': opt_product, 'vendor': opt_vendor}
};
propertyReplacer.set(goog, 'global', mockGlobal);
goog.labs.userAgent.util.setUserAgent(null);
goog.userAgentTestUtil.reinitializeUserAgent();
for (var ua in UserAgents) {
var isExpected = goog.array.contains(expectedAgents, UserAgents[ua]);
assertEquals(
isExpected,
goog.userAgentTestUtil.getUserAgentDetected(UserAgents[ua]));
}
}
function testOperaInit() {
// Check Opera Mini version strings are detected properly
var mockGlobal = {
'navigator': {'userAgent': goog.labs.userAgent.testAgents.OPERA_MINI}
};
propertyReplacer.set(goog, 'global', mockGlobal);
propertyReplacer.set(goog.userAgent, 'getUserAgentString', function() {
return goog.labs.userAgent.testAgents.OPERA_MINI;
});
goog.labs.userAgent.util.setUserAgent(null);
goog.userAgentTestUtil.reinitializeUserAgent();
assertTrue(goog.userAgent.OPERA);
assertEquals('11.10', goog.userAgent.VERSION);
// Check Opera + Blink versions are detected as Chromium
mockGlobal = {
'navigator': {'userAgent': goog.labs.userAgent.testAgents.OPERA_15},
};
propertyReplacer.set(goog, 'global', mockGlobal);
propertyReplacer.set(goog.userAgent, 'getUserAgentString', function() {
return goog.labs.userAgent.testAgents.OPERA_15;
});
goog.labs.userAgent.util.setUserAgent(null);
goog.userAgentTestUtil.reinitializeUserAgent();
// TODO(johnlenz): Chrome/Blink is miscategorized as Webkit
assertTrue(goog.userAgent.WEBKIT);
assertEquals('537.36', goog.userAgent.VERSION);
}
function testCompare() {
assertTrue(
'exact equality broken', goog.userAgent.compare('1.0', '1.0') == 0);
assertTrue(
'mutlidot equality broken',
goog.userAgent.compare('1.0.0.0', '1.0') == 0);
assertTrue('less than broken', goog.userAgent.compare('1.0.2.1', '1.1') < 0);
assertTrue(
'greater than broken', goog.userAgent.compare('1.1', '1.0.2.1') > 0);
assertTrue('b broken', goog.userAgent.compare('1.1', '1.1b') > 0);
assertTrue('b broken', goog.userAgent.compare('1.1b', '1.1') < 0);
assertTrue('b broken', goog.userAgent.compare('1.1b', '1.1b') == 0);
assertTrue('b>a broken', goog.userAgent.compare('1.1b', '1.1a') > 0);
assertTrue('a
© 2015 - 2025 Weber Informatics LLC | Privacy Policy