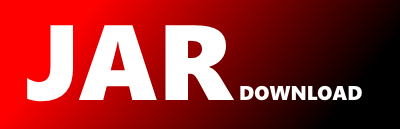
META-INF.dirigible.ide-schema.js.serializer.js Maven / Gradle / Ivy
/*
* Copyright (c) 2024 Eclipse Dirigible contributors
*
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v2.0
* which accompanies this distribution, and is available at
* http://www.eclipse.org/legal/epl-v20.html
*
* SPDX-FileCopyrightText: Eclipse Dirigible contributors
* SPDX-License-Identifier: EPL-2.0
*/
function createSchema(graph) {
let schema = [];
schema.push('\n');
schema.push(' \n');
let parent = graph.getDefaultParent();
let childCount = graph.model.getChildCount(parent);
for (let i = 0; i < childCount; i++) {
let child = graph.model.getChildAt(parent, i);
if (!graph.model.isEdge(child)) {
schema.push(' \n');
let columnCount = graph.model.getChildCount(child);
if (columnCount > 0) {
for (let j = 0; j < columnCount; j++) {
let column = graph.model.getChildAt(child, j).value;
if (column.isSQL) {
schema.push(' \n');
} else {
schema.push(' \n');
}
}
}
schema.push(' \n');
} else {
schema.push(' \n');
schema.push(' \n');
}
}
schema.push(' \n');
let enc = new mxCodec(mxUtils.createXmlDocument());
let node = enc.encode(graph.getModel());
let model = mxUtils.getXml(node);
schema.push(' ' + model);
schema.push('\n ');
return schema.join('');
}
function createSchemaJson(graph) {
let root = {};
root.schema = {};
root.schema.structures = [];
let parent = graph.getDefaultParent();
let childCount = graph.model.getChildCount(parent);
for (let i = 0; i < childCount; i++) {
let child = graph.model.getChildAt(parent, i);
let structure = {};
if (!graph.model.isEdge(child)) {
structure.name = child.value.name;
structure.type = child.value.type.toUpperCase();
structure.columns = [];
let columnCount = graph.model.getChildCount(child);
if (columnCount > 0) {
for (let j = 0; j < columnCount; j++) {
let childColumn = graph.model.getChildAt(child, j).value;
let column = {};
if (childColumn.isSQL) {
column.query = childColumn.name;
} else {
column.name = childColumn.name;
column.type = childColumn.type;
column.length = childColumn.columnLength;
column.nullable = childColumn.notNull === 'true' ? !childColumn.notNull : true;
column.primaryKey = childColumn.primaryKey === 'true' ? childColumn.primaryKey : false;
column.identity = childColumn.autoIncrement === 'true' ? childColumn.autoIncrement : false;
column.unique = childColumn.unique === 'true' ? childColumn.unique : false;
column.defaultValue = childColumn.defaultValue !== null && childColumn.defaultValue !== '' ? childColumn.defaultValue : null;
column.precision = childColumn.precision === 'true' ? childColumn.precision : null;
column.scale = childColumn.scale === 'true' ? childColumn.scale : null;
}
structure.columns.push(column);
}
}
} else {
structure.name = child.source.parent.value.name + '_' + child.target.parent.value.name;
structure.type = 'foreignKey';
structure.table = child.source.parent.value.name;
structure.constraintName = child.source.parent.value.name + '_' + child.target.parent.value.name;
structure.columns = child.source.value.name;
structure.referencedTable = child.target.parent.value.name;
structure.referencedColumns = child.target.value.name;
}
root.schema.structures.push(structure);
}
let serialized = JSON.stringify(root, null, 4);
return serialized;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy