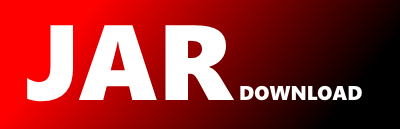
rwt.event.KeyEvent.js Maven / Gradle / Ivy
/*******************************************************************************
* Copyright (c) 2004, 2013 1&1 Internet AG, Germany, http://www.1und1.de,
* EclipseSource and others.
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v1.0
* which accompanies this distribution, and is available at
* http://www.eclipse.org/legal/epl-v10.html
*
* Contributors:
* 1&1 Internet AG and others - original API and implementation
* EclipseSource - adaptation for the Eclipse Remote Application Platform
******************************************************************************/
/**
* A key event instance contains all data for each occured key event
*/
rwt.qx.Class.define("rwt.event.KeyEvent",
{
extend : rwt.event.DomEvent,
/**
* @param vType {String} event type (keydown, keypress, keyup)
* @param vDomEvent {Element} DOM event object
* @param vDomTarget {Element} target element of the DOM event
* @param vTarget
* @param vOriginalTarget
* @param vKeyCode {Integer} emulated key code for compatibility with older qoodoo applications
* @param vCharCode {Integer} char code from the "keypress" event
* @param vKeyIdentifier {String} the key identifier
*/
construct : function(vType, vDomEvent, vDomTarget, vTarget, vOriginalTarget, vKeyCode, vCharCode, vKeyIdentifier)
{
this.base(arguments, vType, vDomEvent, vDomTarget, vTarget, vOriginalTarget);
this._keyCode = vKeyCode;
this.setCharCode(vCharCode);
this.setKeyIdentifier(vKeyIdentifier);
},
/*
*****************************************************************************
STATICS
*****************************************************************************
*/
statics :
{
/**
* Mapping of the old key identifiers to the key codes
* @deprecated
*/
keys :
{
esc : 27,
enter : 13,
tab : 9,
space : 32,
up : 38,
down : 40,
left : 37,
right : 39,
shift : 16,
ctrl : 17,
alt : 18,
f1 : 112,
f2 : 113,
f3 : 114,
f4 : 115,
f5 : 116,
f6 : 117,
f7 : 118,
f8 : 119,
f9 : 120,
f10 : 121,
f11 : 122,
f12 : 123,
print : 124,
del : 46,
backspace : 8,
insert : 45,
home : 36,
end : 35,
pageup : 33,
pagedown : 34,
numlock : 144,
numpad_0 : 96,
numpad_1 : 97,
numpad_2 : 98,
numpad_3 : 99,
numpad_4 : 100,
numpad_5 : 101,
numpad_6 : 102,
numpad_7 : 103,
numpad_8 : 104,
numpad_9 : 105,
numpad_divide : 111,
numpad_multiply : 106,
numpad_minus : 109,
numpad_plus : 107
},
/**
* Mapping of the key codes to the key identifiers
*/
codes : {}
},
/*
*****************************************************************************
PROPERTIES
*****************************************************************************
*/
properties :
{
/**
* Unicode number of the pressed character.
*/
charCode :
{
_fast : true,
setOnlyOnce : true,
noCompute : true
},
/**
* Identifier of the pressed key. This property is modeled after the KeyboardEvent.keyIdentifier property
* of the W3C DOM 3 event specification (http://www.w3.org/TR/2003/NOTE-DOM-Level-3-Events-20031107/events.html#Events-KeyboardEvent-keyIdentifier).
*
* Printable keys are represented by a unicode string, non-printable keys have one of the following
* values:
*
*
* Backspace The Backspace (Back) key.
* Tab The Horizontal Tabulation (Tab) key.
* Space The Space (Spacebar) key.
* Enter The Enter key. Note: This key identifier is also used for the Return (Macintosh numpad) key.
* Shift The Shift key.
* Control The Control (Ctrl) key.
* Alt The Alt (Menu) key.
* CapsLock The CapsLock key
* Meta The Meta key. (Apple Meta and Windows key)
* Escape The Escape (Esc) key.
* Left The Left Arrow key.
* Up The Up Arrow key.
* Right The Right Arrow key.
* Down The Down Arrow key.
* PageUp The Page Up key.
* PageDown The Page Down (Next) key.
* End The End key.
* Home The Home key.
* Insert The Insert (Ins) key. (Does not fire in Opera/Win)
* Delete The Delete (Del) Key.
* F1 The F1 key.
* F2 The F2 key.
* F3 The F3 key.
* F4 The F4 key.
* F5 The F5 key.
* F6 The F6 key.
* F7 The F7 key.
* F8 The F8 key.
* F9 The F9 key.
* F10 The F10 key.
* F11 The F11 key.
* F12 The F12 key.
* NumLock The Num Lock key.
* PrintScreen The Print Screen (PrintScrn, SnapShot) key.
* Scroll The scroll lock key
* Pause The pause/break key
* Win The Windows Logo key
* Apps The Application key (Windows Context Menu)
*
*/
keyIdentifier :
{
_fast : true,
setOnlyOnce : true,
noCompute : true
}
},
/*
*****************************************************************************
MEMBERS
*****************************************************************************
*/
members :
{
/**
* Legacy keycode
* @deprecated Will be removed with qooxdoo 0.7
*/
getKeyCode : function() {
return this._keyCode;
}
},
/*
*****************************************************************************
DEFER
*****************************************************************************
*/
defer : function(statics)
{
// create dynamic codes copy
for (var i in statics.keys) {
statics.codes[statics.keys[i]] = i;
}
}
});
© 2015 - 2025 Weber Informatics LLC | Privacy Policy