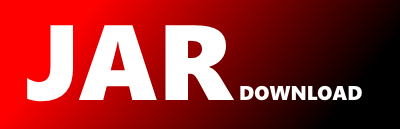
rwt.widgets.util.LayoutImpl.js Maven / Gradle / Ivy
/*******************************************************************************
* Copyright (c) 2004, 2014 1&1 Internet AG, Germany, http://www.1und1.de,
* EclipseSource and others.
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v1.0
* which accompanies this distribution, and is available at
* http://www.eclipse.org/legal/epl-v10.html
*
* Contributors:
* 1&1 Internet AG and others - original API and implementation
* EclipseSource - adaptation for the Eclipse Remote Application Platform
******************************************************************************/
/**
* Abstact base class of all layout implementations
*
* @param vWidget {rwt.widgets.base.Parent} reference to the associated widget
*/
rwt.qx.Class.define("rwt.widgets.util.LayoutImpl",
{
extend : rwt.qx.Object,
/*
*****************************************************************************
CONSTRUCTOR
*****************************************************************************
*/
construct : function(vWidget)
{
this.base(arguments);
this._widget = vWidget;
},
/*
*****************************************************************************
MEMBERS
*****************************************************************************
*/
members :
{
/**
* Returns the associated widget
*
* @type member
* @return {rwt.widgets.base.Parent} reference to the associated widget
*/
getWidget : function() {
return this._widget;
},
/*
Global Structure:
[01] COMPUTE BOX DIMENSIONS FOR AN INDIVIDUAL CHILD
[02] COMPUTE NEEDED DIMENSIONS FOR AN INDIVIDUAL CHILD
[03] COMPUTE NEEDED DIMENSIONS FOR ALL CHILDREN
[04] UPDATE LAYOUT WHEN A CHILD CHANGES ITS OUTER DIMENSIONS
[05] UPDATE CHILD ON INNER DIMENSION CHANGES OF LAYOUT
[06] UPDATE LAYOUT ON JOB QUEUE FLUSH
[07] UPDATE CHILDREN ON JOB QUEUE FLUSH
[08] CHILDREN ADD/REMOVE/MOVE HANDLING
[09] FLUSH LAYOUT QUEUES OF CHILDREN
[10] LAYOUT CHILD
*/
/*
---------------------------------------------------------------------------
[01] COMPUTE BOX DIMENSIONS FOR AN INDIVIDUAL CHILD
---------------------------------------------------------------------------
*/
/**
* Compute and return the box width of the given child
*
* @type member
* @param vChild {rwt.widgets.base.Widget} TODOC
* @return {Integer} box width of the given child
*/
computeChildBoxWidth : function(vChild) {
return vChild.getWidthValue() || vChild._computeBoxWidthFallback();
},
/**
* Compute and return the box height of the given child
*
* @type member
* @param vChild {rwt.widgets.base.Widget} TODOC
* @return {Integer} box height of the given child
*/
computeChildBoxHeight : function(vChild) {
return vChild.getHeightValue() || vChild._computeBoxHeightFallback();
},
/*
---------------------------------------------------------------------------
[02] COMPUTE NEEDED DIMENSIONS FOR AN INDIVIDUAL CHILD
---------------------------------------------------------------------------
*/
/**
* Compute and return the needed width of the given child
*
* @type member
* @param vChild {rwt.widgets.base.Widget} TODOC
* @return {Integer} needed width
*/
computeChildNeededWidth : function(vChild)
{
// omit ultra long lines, these two variables only needed once
// here, but this enhance the readability of the code :)
var vMinBox = vChild._computedMinWidthTypePercent ? null : vChild.getMinWidthValue();
var vMaxBox = vChild._computedMaxWidthTypePercent ? null : vChild.getMaxWidthValue();
var vBox = (vChild._computedWidthTypePercent || vChild._computedWidthTypeFlex ? null : vChild.getWidthValue()) || vChild.getPreferredBoxWidth() || 0;
return rwt.util.Numbers.limit(vBox, vMinBox, vMaxBox) + vChild.getMarginLeft() + vChild.getMarginRight();
},
/**
* Compute and return the needed height of the given child
*
* @type member
* @param vChild {rwt.widgets.base.Widget} TODOC
* @return {Integer} needed height
*/
computeChildNeededHeight : function(vChild)
{
// omit ultra long lines, these two variables only needed once
// here, but this enhance the readability of the code :)
var vMinBox = vChild._computedMinHeightTypePercent ? null : vChild.getMinHeightValue();
var vMaxBox = vChild._computedMaxHeightTypePercent ? null : vChild.getMaxHeightValue();
var vBox = (vChild._computedHeightTypePercent || vChild._computedHeightTypeFlex ? null : vChild.getHeightValue()) || vChild.getPreferredBoxHeight() || 0;
return rwt.util.Numbers.limit(vBox, vMinBox, vMaxBox) + vChild.getMarginTop() + vChild.getMarginBottom();
},
/*
---------------------------------------------------------------------------
[03] COMPUTE NEEDED DIMENSIONS FOR ALL CHILDREN
---------------------------------------------------------------------------
*/
/**
* Calculate the maximum needed width of all children
*
* @type member
* @return {Integer} maximum needed width of all children
*/
computeChildrenNeededWidth_max : function()
{
for (var i=0, ch=this.getWidget().getVisibleChildren(), chl=ch.length, maxv=0; i
© 2015 - 2025 Weber Informatics LLC | Privacy Policy