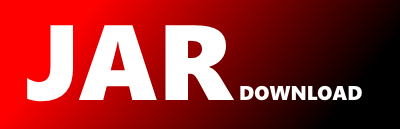
.cdm-java.6.0.0-dev.82.source-code.MetaFieldTypes.cs Maven / Gradle / Ivy
// This file is auto-generated from the ISDA Common Domain Model, do not edit.
//
// Version: 6.0.0-dev.82
//
#nullable enable // Allow nullable reference types
namespace Org.Isda.Cdm.MetaFields
{
using System.Collections.Generic;
using Newtonsoft.Json;
using Newtonsoft.Json.Converters;
using NodaTime;
using Rosetta.Lib.Meta;
public class Key
{
[JsonConstructor]
public Key(string keyValue, string? scope)
{
Scope = scope;
KeyValue = keyValue;
}
[JsonProperty("value")]
public string KeyValue { get; }
public string? Scope { get; }
}
public class Reference
{
[JsonConstructor]
public Reference(string? reference, string? scope)
{
Scope = scope;
ReferenceValue = reference;
}
[JsonProperty("value")]
public string? ReferenceValue { get; }
public string? Scope { get; }
}
public class ReferenceWithMetaParty : IReferenceWithMeta
{
[JsonConstructor]
public ReferenceWithMetaParty(Party? value, string? globalReference, string? externalReference, Reference? address)
{
Value = value;
GlobalReference = globalReference;
ExternalReference = externalReference;
Address = address;
}
public Party? Value { get; }
public string? GlobalReference { get; }
public string? ExternalReference { get; }
public Reference? Address { get; }
}
public class ReferenceWithMetaBusinessDayAdjustments : IReferenceWithMeta
{
[JsonConstructor]
public ReferenceWithMetaBusinessDayAdjustments(BusinessDayAdjustments? value, string? globalReference, string? externalReference, Reference? address)
{
Value = value;
GlobalReference = globalReference;
ExternalReference = externalReference;
Address = address;
}
public BusinessDayAdjustments? Value { get; }
public string? GlobalReference { get; }
public string? ExternalReference { get; }
public Reference? Address { get; }
}
public class ReferenceWithMetaTrade : IReferenceWithMeta
{
[JsonConstructor]
public ReferenceWithMetaTrade(Trade? value, string? globalReference, string? externalReference, Reference? address)
{
Value = value;
GlobalReference = globalReference;
ExternalReference = externalReference;
Address = address;
}
public Trade? Value { get; }
public string? GlobalReference { get; }
public string? ExternalReference { get; }
public Reference? Address { get; }
}
public class ReferenceWithMetaNonNegativeQuantitySchedule : IReferenceWithMeta
{
[JsonConstructor]
public ReferenceWithMetaNonNegativeQuantitySchedule(NonNegativeQuantitySchedule? value, string? globalReference, string? externalReference, Reference? address)
{
Value = value;
GlobalReference = globalReference;
ExternalReference = externalReference;
Address = address;
}
public NonNegativeQuantitySchedule? Value { get; }
public string? GlobalReference { get; }
public string? ExternalReference { get; }
public Reference? Address { get; }
}
public class ReferenceWithMetaPriceSchedule : IReferenceWithMeta
{
[JsonConstructor]
public ReferenceWithMetaPriceSchedule(PriceSchedule? value, string? globalReference, string? externalReference, Reference? address)
{
Value = value;
GlobalReference = globalReference;
ExternalReference = externalReference;
Address = address;
}
public PriceSchedule? Value { get; }
public string? GlobalReference { get; }
public string? ExternalReference { get; }
public Reference? Address { get; }
}
public class ReferenceWithMetaTradeState : IReferenceWithMeta
{
[JsonConstructor]
public ReferenceWithMetaTradeState(TradeState? value, string? globalReference, string? externalReference, Reference? address)
{
Value = value;
GlobalReference = globalReference;
ExternalReference = externalReference;
Address = address;
}
public TradeState? Value { get; }
public string? GlobalReference { get; }
public string? ExternalReference { get; }
public Reference? Address { get; }
}
public class ReferenceWithMetaBusinessCenters : IReferenceWithMeta
{
[JsonConstructor]
public ReferenceWithMetaBusinessCenters(BusinessCenters? value, string? globalReference, string? externalReference, Reference? address)
{
Value = value;
GlobalReference = globalReference;
ExternalReference = externalReference;
Address = address;
}
public BusinessCenters? Value { get; }
public string? GlobalReference { get; }
public string? ExternalReference { get; }
public Reference? Address { get; }
}
public class ReferenceWithMetaPayout : IReferenceWithMeta
{
[JsonConstructor]
public ReferenceWithMetaPayout(Payout? value, string? globalReference, string? externalReference, Reference? address)
{
Value = value;
GlobalReference = globalReference;
ExternalReference = externalReference;
Address = address;
}
public Payout? Value { get; }
public string? GlobalReference { get; }
public string? ExternalReference { get; }
public Reference? Address { get; }
}
public class ReferenceWithMetaCollateralPortfolio : IReferenceWithMeta
{
[JsonConstructor]
public ReferenceWithMetaCollateralPortfolio(CollateralPortfolio? value, string? globalReference, string? externalReference, Reference? address)
{
Value = value;
GlobalReference = globalReference;
ExternalReference = externalReference;
Address = address;
}
public CollateralPortfolio? Value { get; }
public string? GlobalReference { get; }
public string? ExternalReference { get; }
public Reference? Address { get; }
}
public class ReferenceWithMetaLegalAgreement : IReferenceWithMeta
{
[JsonConstructor]
public ReferenceWithMetaLegalAgreement(LegalAgreement? value, string? globalReference, string? externalReference, Reference? address)
{
Value = value;
GlobalReference = globalReference;
ExternalReference = externalReference;
Address = address;
}
public LegalAgreement? Value { get; }
public string? GlobalReference { get; }
public string? ExternalReference { get; }
public Reference? Address { get; }
}
public class ReferenceWithMetaCommodity : IReferenceWithMeta
{
[JsonConstructor]
public ReferenceWithMetaCommodity(Commodity? value, string? globalReference, string? externalReference, Reference? address)
{
Value = value;
GlobalReference = globalReference;
ExternalReference = externalReference;
Address = address;
}
public Commodity? Value { get; }
public string? GlobalReference { get; }
public string? ExternalReference { get; }
public Reference? Address { get; }
}
public class ReferenceWithMetaContractDetails : IReferenceWithMeta
{
[JsonConstructor]
public ReferenceWithMetaContractDetails(ContractDetails? value, string? globalReference, string? externalReference, Reference? address)
{
Value = value;
GlobalReference = globalReference;
ExternalReference = externalReference;
Address = address;
}
public ContractDetails? Value { get; }
public string? GlobalReference { get; }
public string? ExternalReference { get; }
public Reference? Address { get; }
}
public class ReferenceWithMetaExecutionDetails : IReferenceWithMeta
{
[JsonConstructor]
public ReferenceWithMetaExecutionDetails(ExecutionDetails? value, string? globalReference, string? externalReference, Reference? address)
{
Value = value;
GlobalReference = globalReference;
ExternalReference = externalReference;
Address = address;
}
public ExecutionDetails? Value { get; }
public string? GlobalReference { get; }
public string? ExternalReference { get; }
public Reference? Address { get; }
}
public class ReferenceWithMetaCollateral : IReferenceWithMeta
{
[JsonConstructor]
public ReferenceWithMetaCollateral(Collateral? value, string? globalReference, string? externalReference, Reference? address)
{
Value = value;
GlobalReference = globalReference;
ExternalReference = externalReference;
Address = address;
}
public Collateral? Value { get; }
public string? GlobalReference { get; }
public string? ExternalReference { get; }
public Reference? Address { get; }
}
public class ReferenceWithMetaCalculationPeriodDates : IReferenceWithMeta
{
[JsonConstructor]
public ReferenceWithMetaCalculationPeriodDates(CalculationPeriodDates? value, string? globalReference, string? externalReference, Reference? address)
{
Value = value;
GlobalReference = globalReference;
ExternalReference = externalReference;
Address = address;
}
public CalculationPeriodDates? Value { get; }
public string? GlobalReference { get; }
public string? ExternalReference { get; }
public Reference? Address { get; }
}
public class ReferenceWithMetaPaymentDates : IReferenceWithMeta
{
[JsonConstructor]
public ReferenceWithMetaPaymentDates(PaymentDates? value, string? globalReference, string? externalReference, Reference? address)
{
Value = value;
GlobalReference = globalReference;
ExternalReference = externalReference;
Address = address;
}
public PaymentDates? Value { get; }
public string? GlobalReference { get; }
public string? ExternalReference { get; }
public Reference? Address { get; }
}
public class ReferenceWithMetaPerformanceValuationDates : IReferenceWithMeta
{
[JsonConstructor]
public ReferenceWithMetaPerformanceValuationDates(PerformanceValuationDates? value, string? globalReference, string? externalReference, Reference? address)
{
Value = value;
GlobalReference = globalReference;
ExternalReference = externalReference;
Address = address;
}
public PerformanceValuationDates? Value { get; }
public string? GlobalReference { get; }
public string? ExternalReference { get; }
public Reference? Address { get; }
}
public class BasicReferenceWithMetaString : IReferenceWithMeta
{
[JsonConstructor]
public BasicReferenceWithMetaString(string? value, string? globalReference, string? externalReference, Reference? address)
{
Value = value;
GlobalReference = globalReference;
ExternalReference = externalReference;
Address = address;
}
public string? Value { get; }
public string? GlobalReference { get; }
public string? ExternalReference { get; }
public Reference? Address { get; }
}
public class ReferenceWithMetaAdjustableOrRelativeDate : IReferenceWithMeta
{
[JsonConstructor]
public ReferenceWithMetaAdjustableOrRelativeDate(AdjustableOrRelativeDate? value, string? globalReference, string? externalReference, Reference? address)
{
Value = value;
GlobalReference = globalReference;
ExternalReference = externalReference;
Address = address;
}
public AdjustableOrRelativeDate? Value { get; }
public string? GlobalReference { get; }
public string? ExternalReference { get; }
public Reference? Address { get; }
}
public class ReferenceWithMetaBasketConstituent : IReferenceWithMeta
{
[JsonConstructor]
public ReferenceWithMetaBasketConstituent(BasketConstituent? value, string? globalReference, string? externalReference, Reference? address)
{
Value = value;
GlobalReference = globalReference;
ExternalReference = externalReference;
Address = address;
}
public BasketConstituent? Value { get; }
public string? GlobalReference { get; }
public string? ExternalReference { get; }
public Reference? Address { get; }
}
public class ReferenceWithMetaMoney : IReferenceWithMeta
{
[JsonConstructor]
public ReferenceWithMetaMoney(Money? value, string? globalReference, string? externalReference, Reference? address)
{
Value = value;
GlobalReference = globalReference;
ExternalReference = externalReference;
Address = address;
}
public Money? Value { get; }
public string? GlobalReference { get; }
public string? ExternalReference { get; }
public Reference? Address { get; }
}
public class ReferenceWithMetaOptionPayout : IReferenceWithMeta
{
[JsonConstructor]
public ReferenceWithMetaOptionPayout(OptionPayout? value, string? globalReference, string? externalReference, Reference? address)
{
Value = value;
GlobalReference = globalReference;
ExternalReference = externalReference;
Address = address;
}
public OptionPayout? Value { get; }
public string? GlobalReference { get; }
public string? ExternalReference { get; }
public Reference? Address { get; }
}
public class ReferenceWithMetaPortfolioState : IReferenceWithMeta
{
[JsonConstructor]
public ReferenceWithMetaPortfolioState(PortfolioState? value, string? globalReference, string? externalReference, Reference? address)
{
Value = value;
GlobalReference = globalReference;
ExternalReference = externalReference;
Address = address;
}
public PortfolioState? Value { get; }
public string? GlobalReference { get; }
public string? ExternalReference { get; }
public Reference? Address { get; }
}
public class ReferenceWithMetaAdjustableOrRelativeDates : IReferenceWithMeta
{
[JsonConstructor]
public ReferenceWithMetaAdjustableOrRelativeDates(AdjustableOrRelativeDates? value, string? globalReference, string? externalReference, Reference? address)
{
Value = value;
GlobalReference = globalReference;
ExternalReference = externalReference;
Address = address;
}
public AdjustableOrRelativeDates? Value { get; }
public string? GlobalReference { get; }
public string? ExternalReference { get; }
public Reference? Address { get; }
}
public class ReferenceWithMetaInterestRatePayout : IReferenceWithMeta
{
[JsonConstructor]
public ReferenceWithMetaInterestRatePayout(InterestRatePayout? value, string? globalReference, string? externalReference, Reference? address)
{
Value = value;
GlobalReference = globalReference;
ExternalReference = externalReference;
Address = address;
}
public InterestRatePayout? Value { get; }
public string? GlobalReference { get; }
public string? ExternalReference { get; }
public Reference? Address { get; }
}
public class ReferenceWithMetaFloatingRateIndex : IReferenceWithMeta
{
[JsonConstructor]
public ReferenceWithMetaFloatingRateIndex(FloatingRateIndex? value, string? globalReference, string? externalReference, Reference? address)
{
Value = value;
GlobalReference = globalReference;
ExternalReference = externalReference;
Address = address;
}
public FloatingRateIndex? Value { get; }
public string? GlobalReference { get; }
public string? ExternalReference { get; }
public Reference? Address { get; }
}
public class ReferenceWithMetaWorkflowStep : IReferenceWithMeta
{
[JsonConstructor]
public ReferenceWithMetaWorkflowStep(WorkflowStep? value, string? globalReference, string? externalReference, Reference? address)
{
Value = value;
GlobalReference = globalReference;
ExternalReference = externalReference;
Address = address;
}
public WorkflowStep? Value { get; }
public string? GlobalReference { get; }
public string? ExternalReference { get; }
public Reference? Address { get; }
}
public class ReferenceWithMetaNaturalPerson : IReferenceWithMeta
{
[JsonConstructor]
public ReferenceWithMetaNaturalPerson(NaturalPerson? value, string? globalReference, string? externalReference, Reference? address)
{
Value = value;
GlobalReference = globalReference;
ExternalReference = externalReference;
Address = address;
}
public NaturalPerson? Value { get; }
public string? GlobalReference { get; }
public string? ExternalReference { get; }
public Reference? Address { get; }
}
public class ReferenceWithMetaFixedRateSpecification : IReferenceWithMeta
{
[JsonConstructor]
public ReferenceWithMetaFixedRateSpecification(FixedRateSpecification? value, string? globalReference, string? externalReference, Reference? address)
{
Value = value;
GlobalReference = globalReference;
ExternalReference = externalReference;
Address = address;
}
public FixedRateSpecification? Value { get; }
public string? GlobalReference { get; }
public string? ExternalReference { get; }
public Reference? Address { get; }
}
public class ReferenceWithMetaAccount : IReferenceWithMeta
{
[JsonConstructor]
public ReferenceWithMetaAccount(Account? value, string? globalReference, string? externalReference, Reference? address)
{
Value = value;
GlobalReference = globalReference;
ExternalReference = externalReference;
Address = address;
}
public Account? Value { get; }
public string? GlobalReference { get; }
public string? ExternalReference { get; }
public Reference? Address { get; }
}
public class ReferenceWithMetaObservable : IReferenceWithMeta
{
[JsonConstructor]
public ReferenceWithMetaObservable(Observable? value, string? globalReference, string? externalReference, Reference? address)
{
Value = value;
GlobalReference = globalReference;
ExternalReference = externalReference;
Address = address;
}
public Observable? Value { get; }
public string? GlobalReference { get; }
public string? ExternalReference { get; }
public Reference? Address { get; }
}
public class ReferenceWithMetaRateObservation : IReferenceWithMeta
{
[JsonConstructor]
public ReferenceWithMetaRateObservation(RateObservation? value, string? globalReference, string? externalReference, Reference? address)
{
Value = value;
GlobalReference = globalReference;
ExternalReference = externalReference;
Address = address;
}
public RateObservation? Value { get; }
public string? GlobalReference { get; }
public string? ExternalReference { get; }
public Reference? Address { get; }
}
public class ReferenceWithMetaLegalEntity : IReferenceWithMeta
{
[JsonConstructor]
public ReferenceWithMetaLegalEntity(LegalEntity? value, string? globalReference, string? externalReference, Reference? address)
{
Value = value;
GlobalReference = globalReference;
ExternalReference = externalReference;
Address = address;
}
public LegalEntity? Value { get; }
public string? GlobalReference { get; }
public string? ExternalReference { get; }
public Reference? Address { get; }
}
public class ReferenceWithMetaProtectionTerms : IReferenceWithMeta
{
[JsonConstructor]
public ReferenceWithMetaProtectionTerms(ProtectionTerms? value, string? globalReference, string? externalReference, Reference? address)
{
Value = value;
GlobalReference = globalReference;
ExternalReference = externalReference;
Address = address;
}
public ProtectionTerms? Value { get; }
public string? GlobalReference { get; }
public string? ExternalReference { get; }
public Reference? Address { get; }
}
public class ReferenceWithMetaCashSettlementTerms : IReferenceWithMeta
{
[JsonConstructor]
public ReferenceWithMetaCashSettlementTerms(CashSettlementTerms? value, string? globalReference, string? externalReference, Reference? address)
{
Value = value;
GlobalReference = globalReference;
ExternalReference = externalReference;
Address = address;
}
public CashSettlementTerms? Value { get; }
public string? GlobalReference { get; }
public string? ExternalReference { get; }
public Reference? Address { get; }
}
public class ReferenceWithMetaPhysicalSettlementTerms : IReferenceWithMeta
{
[JsonConstructor]
public ReferenceWithMetaPhysicalSettlementTerms(PhysicalSettlementTerms? value, string? globalReference, string? externalReference, Reference? address)
{
Value = value;
GlobalReference = globalReference;
ExternalReference = externalReference;
Address = address;
}
public PhysicalSettlementTerms? Value { get; }
public string? GlobalReference { get; }
public string? ExternalReference { get; }
public Reference? Address { get; }
}
public class BasicReferenceWithMetaLocalDate : IValueReferenceWithMeta
{
[JsonConstructor]
public BasicReferenceWithMetaLocalDate(NodaTime.LocalDate? value, string? globalReference, string? externalReference, Reference? address)
{
Value = value;
GlobalReference = globalReference;
ExternalReference = externalReference;
Address = address;
}
[JsonConverter(typeof(Rosetta.Lib.LocalDateConverter))]
public NodaTime.LocalDate? Value { get; }
public string? GlobalReference { get; }
public string? ExternalReference { get; }
public Reference? Address { get; }
}
public class ReferenceWithMetaObservation : IReferenceWithMeta
{
[JsonConstructor]
public ReferenceWithMetaObservation(Observation? value, string? globalReference, string? externalReference, Reference? address)
{
Value = value;
GlobalReference = globalReference;
ExternalReference = externalReference;
Address = address;
}
public Observation? Value { get; }
public string? GlobalReference { get; }
public string? ExternalReference { get; }
public Reference? Address { get; }
}
public class ReferenceWithMetaResolvablePriceQuantity : IReferenceWithMeta
{
[JsonConstructor]
public ReferenceWithMetaResolvablePriceQuantity(ResolvablePriceQuantity? value, string? globalReference, string? externalReference, Reference? address)
{
Value = value;
GlobalReference = globalReference;
ExternalReference = externalReference;
Address = address;
}
public ResolvablePriceQuantity? Value { get; }
public string? GlobalReference { get; }
public string? ExternalReference { get; }
public Reference? Address { get; }
}
public class ReferenceWithMetaCreditEvents : IReferenceWithMeta
{
[JsonConstructor]
public ReferenceWithMetaCreditEvents(CreditEvents? value, string? globalReference, string? externalReference, Reference? address)
{
Value = value;
GlobalReference = globalReference;
ExternalReference = externalReference;
Address = address;
}
public CreditEvents? Value { get; }
public string? GlobalReference { get; }
public string? ExternalReference { get; }
public Reference? Address { get; }
}
public class ReferenceWithMetaQuotedCurrencyPair : IReferenceWithMeta
{
[JsonConstructor]
public ReferenceWithMetaQuotedCurrencyPair(QuotedCurrencyPair? value, string? globalReference, string? externalReference, Reference? address)
{
Value = value;
GlobalReference = globalReference;
ExternalReference = externalReference;
Address = address;
}
public QuotedCurrencyPair? Value { get; }
public string? GlobalReference { get; }
public string? ExternalReference { get; }
public Reference? Address { get; }
}
public class FieldWithMetaString : IFieldWithMeta
{
[JsonConstructor]
public FieldWithMetaString(string value, MetaFields? meta)
{
Value = value;
Meta = meta;
}
public string Value { get; }
public MetaFields? Meta { get; }
}
public class FieldWithMetaAccountTypeEnum : IEnumFieldWithMeta
{
[JsonConstructor]
public FieldWithMetaAccountTypeEnum(Enums.AccountType value, MetaFields? meta)
{
Value = value;
Meta = meta;
}
[JsonConverter(typeof(StringEnumConverter))]
public Enums.AccountType Value { get; }
public MetaFields? Meta { get; }
}
public class FieldWithMetaLocalDate : IValueFieldWithMeta
{
[JsonConstructor]
public FieldWithMetaLocalDate(NodaTime.LocalDate value, MetaFields? meta)
{
Value = value;
Meta = meta;
}
[JsonConverter(typeof(Rosetta.Lib.LocalDateConverter))]
public NodaTime.LocalDate Value { get; }
public MetaFields? Meta { get; }
}
public class FieldWithMetaCreditSupportAgreementTypeEnum : IEnumFieldWithMeta
{
[JsonConstructor]
public FieldWithMetaCreditSupportAgreementTypeEnum(Enums.CreditSupportAgreementType value, MetaFields? meta)
{
Value = value;
Meta = meta;
}
[JsonConverter(typeof(StringEnumConverter))]
public Enums.CreditSupportAgreementType Value { get; }
public MetaFields? Meta { get; }
}
public class FieldWithMetaContractualDefinitionsEnum : IEnumFieldWithMeta
{
[JsonConstructor]
public FieldWithMetaContractualDefinitionsEnum(Enums.ContractualDefinitions value, MetaFields? meta)
{
Value = value;
Meta = meta;
}
[JsonConverter(typeof(StringEnumConverter))]
public Enums.ContractualDefinitions Value { get; }
public MetaFields? Meta { get; }
}
public class FieldWithMetaMasterAgreementTypeEnum : IEnumFieldWithMeta
{
[JsonConstructor]
public FieldWithMetaMasterAgreementTypeEnum(Enums.MasterAgreementType value, MetaFields? meta)
{
Value = value;
Meta = meta;
}
[JsonConverter(typeof(StringEnumConverter))]
public Enums.MasterAgreementType Value { get; }
public MetaFields? Meta { get; }
}
public class FieldWithMetaMasterConfirmationTypeEnum : IEnumFieldWithMeta
{
[JsonConstructor]
public FieldWithMetaMasterConfirmationTypeEnum(Enums.MasterConfirmationType value, MetaFields? meta)
{
Value = value;
Meta = meta;
}
[JsonConverter(typeof(StringEnumConverter))]
public Enums.MasterConfirmationType Value { get; }
public MetaFields? Meta { get; }
}
public class FieldWithMetaMasterConfirmationAnnexTypeEnum : IEnumFieldWithMeta
{
[JsonConstructor]
public FieldWithMetaMasterConfirmationAnnexTypeEnum(Enums.MasterConfirmationAnnexType value, MetaFields? meta)
{
Value = value;
Meta = meta;
}
[JsonConverter(typeof(StringEnumConverter))]
public Enums.MasterConfirmationAnnexType Value { get; }
public MetaFields? Meta { get; }
}
public class FieldWithMetaCommodity : IFieldWithMeta
{
[JsonConstructor]
public FieldWithMetaCommodity(Commodity value, MetaFields? meta)
{
Value = value;
Meta = meta;
}
public Commodity Value { get; }
public MetaFields? Meta { get; }
}
public class FieldWithMetaMarketDisruptionEnum : IEnumFieldWithMeta
{
[JsonConstructor]
public FieldWithMetaMarketDisruptionEnum(Enums.MarketDisruption value, MetaFields? meta)
{
Value = value;
Meta = meta;
}
[JsonConverter(typeof(StringEnumConverter))]
public Enums.MarketDisruption Value { get; }
public MetaFields? Meta { get; }
}
public class FieldWithMetaBasketConstituent : IFieldWithMeta
{
[JsonConstructor]
public FieldWithMetaBasketConstituent(BasketConstituent value, MetaFields? meta)
{
Value = value;
Meta = meta;
}
public BasketConstituent Value { get; }
public MetaFields? Meta { get; }
}
public class FieldWithMetaBusinessCenterEnum : IEnumFieldWithMeta
{
[JsonConstructor]
public FieldWithMetaBusinessCenterEnum(Enums.BusinessCenter value, MetaFields? meta)
{
Value = value;
Meta = meta;
}
[JsonConverter(typeof(StringEnumConverter))]
public Enums.BusinessCenter Value { get; }
public MetaFields? Meta { get; }
}
public class FieldWithMetaCommodityBusinessCalendarEnum : IEnumFieldWithMeta
{
[JsonConstructor]
public FieldWithMetaCommodityBusinessCalendarEnum(Enums.CommodityBusinessCalendar value, MetaFields? meta)
{
Value = value;
Meta = meta;
}
[JsonConverter(typeof(StringEnumConverter))]
public Enums.CommodityBusinessCalendar Value { get; }
public MetaFields? Meta { get; }
}
public class FieldWithMetaGoverningLawEnum : IEnumFieldWithMeta
{
[JsonConstructor]
public FieldWithMetaGoverningLawEnum(Enums.GoverningLaw value, MetaFields? meta)
{
Value = value;
Meta = meta;
}
[JsonConverter(typeof(StringEnumConverter))]
public Enums.GoverningLaw Value { get; }
public MetaFields? Meta { get; }
}
public class FieldWithMetaMatrixTypeEnum : IEnumFieldWithMeta
{
[JsonConstructor]
public FieldWithMetaMatrixTypeEnum(Enums.MatrixType value, MetaFields? meta)
{
Value = value;
Meta = meta;
}
[JsonConverter(typeof(StringEnumConverter))]
public Enums.MatrixType Value { get; }
public MetaFields? Meta { get; }
}
public class FieldWithMetaMatrixTermEnum : IEnumFieldWithMeta
{
[JsonConstructor]
public FieldWithMetaMatrixTermEnum(Enums.MatrixTerm value, MetaFields? meta)
{
Value = value;
Meta = meta;
}
[JsonConverter(typeof(StringEnumConverter))]
public Enums.MatrixTerm Value { get; }
public MetaFields? Meta { get; }
}
public class FieldWithMetaContractualSupplementTypeEnum : IEnumFieldWithMeta
{
[JsonConstructor]
public FieldWithMetaContractualSupplementTypeEnum(Enums.ContractualSupplementType value, MetaFields? meta)
{
Value = value;
Meta = meta;
}
[JsonConverter(typeof(StringEnumConverter))]
public Enums.ContractualSupplementType Value { get; }
public MetaFields? Meta { get; }
}
public class FieldWithMetaIndexAnnexSourceEnum : IEnumFieldWithMeta
{
[JsonConstructor]
public FieldWithMetaIndexAnnexSourceEnum(Enums.IndexAnnexSource value, MetaFields? meta)
{
Value = value;
Meta = meta;
}
[JsonConverter(typeof(StringEnumConverter))]
public Enums.IndexAnnexSource Value { get; }
public MetaFields? Meta { get; }
}
public class FieldWithMetaCommodityReferencePriceEnum : IEnumFieldWithMeta
{
[JsonConstructor]
public FieldWithMetaCommodityReferencePriceEnum(Enums.CommodityReferencePrice value, MetaFields? meta)
{
Value = value;
Meta = meta;
}
[JsonConverter(typeof(StringEnumConverter))]
public Enums.CommodityReferencePrice Value { get; }
public MetaFields? Meta { get; }
}
public class FieldWithMetaDayCountFractionEnum : IEnumFieldWithMeta
{
[JsonConstructor]
public FieldWithMetaDayCountFractionEnum(Enums.DayCountFraction value, MetaFields? meta)
{
Value = value;
Meta = meta;
}
[JsonConverter(typeof(StringEnumConverter))]
public Enums.DayCountFraction Value { get; }
public MetaFields? Meta { get; }
}
public class FieldWithMetaTimeZone : IFieldWithMeta
{
[JsonConstructor]
public FieldWithMetaTimeZone(TimeZone value, MetaFields? meta)
{
Value = value;
Meta = meta;
}
public TimeZone Value { get; }
public MetaFields? Meta { get; }
}
public class FieldWithMetaSettlementRateOptionEnum : IEnumFieldWithMeta
{
[JsonConstructor]
public FieldWithMetaSettlementRateOptionEnum(Enums.SettlementRateOption value, MetaFields? meta)
{
Value = value;
Meta = meta;
}
[JsonConverter(typeof(StringEnumConverter))]
public Enums.SettlementRateOption Value { get; }
public MetaFields? Meta { get; }
}
public class FieldWithMetaFloatingRateIndexEnum : IEnumFieldWithMeta
{
[JsonConstructor]
public FieldWithMetaFloatingRateIndexEnum(Enums.FloatingRateIndex value, MetaFields? meta)
{
Value = value;
Meta = meta;
}
[JsonConverter(typeof(StringEnumConverter))]
public Enums.FloatingRateIndex Value { get; }
public MetaFields? Meta { get; }
}
public class FieldWithMetaQuotedCurrencyPair : IFieldWithMeta
{
[JsonConstructor]
public FieldWithMetaQuotedCurrencyPair(QuotedCurrencyPair value, MetaFields? meta)
{
Value = value;
Meta = meta;
}
public QuotedCurrencyPair Value { get; }
public MetaFields? Meta { get; }
}
public class FieldWithMetaFloatingRateIndex : IFieldWithMeta
{
[JsonConstructor]
public FieldWithMetaFloatingRateIndex(FloatingRateIndex value, MetaFields? meta)
{
Value = value;
Meta = meta;
}
public FloatingRateIndex Value { get; }
public MetaFields? Meta { get; }
}
public class FieldWithMetaInflationRateIndexEnum : IEnumFieldWithMeta
{
[JsonConstructor]
public FieldWithMetaInflationRateIndexEnum(Enums.InflationRateIndex value, MetaFields? meta)
{
Value = value;
Meta = meta;
}
[JsonConverter(typeof(StringEnumConverter))]
public Enums.InflationRateIndex Value { get; }
public MetaFields? Meta { get; }
}
public class FieldWithMetaInterpolationMethodEnum : IEnumFieldWithMeta
{
[JsonConstructor]
public FieldWithMetaInterpolationMethodEnum(Enums.InterpolationMethod value, MetaFields? meta)
{
Value = value;
Meta = meta;
}
[JsonConverter(typeof(StringEnumConverter))]
public Enums.InterpolationMethod Value { get; }
public MetaFields? Meta { get; }
}
public class FieldWithMetaInformationProviderEnum : IEnumFieldWithMeta
{
[JsonConstructor]
public FieldWithMetaInformationProviderEnum(Enums.InformationProvider value, MetaFields? meta)
{
Value = value;
Meta = meta;
}
[JsonConverter(typeof(StringEnumConverter))]
public Enums.InformationProvider Value { get; }
public MetaFields? Meta { get; }
}
public class FieldWithMetaCreditLimitTypeEnum : IEnumFieldWithMeta
{
[JsonConstructor]
public FieldWithMetaCreditLimitTypeEnum(Enums.CreditLimitType value, MetaFields? meta)
{
Value = value;
Meta = meta;
}
[JsonConverter(typeof(StringEnumConverter))]
public Enums.CreditLimitType Value { get; }
public MetaFields? Meta { get; }
}
public class FieldWithMetaLimitLevelEnum : IEnumFieldWithMeta
{
[JsonConstructor]
public FieldWithMetaLimitLevelEnum(Enums.LimitLevel value, MetaFields? meta)
{
Value = value;
Meta = meta;
}
[JsonConverter(typeof(StringEnumConverter))]
public Enums.LimitLevel Value { get; }
public MetaFields? Meta { get; }
}
public class FieldWithMetaCreditNotation : IFieldWithMeta
{
[JsonConstructor]
public FieldWithMetaCreditNotation(CreditNotation value, MetaFields? meta)
{
Value = value;
Meta = meta;
}
public CreditNotation Value { get; }
public MetaFields? Meta { get; }
}
public class FieldWithMetaPersonIdentifier : IFieldWithMeta
{
[JsonConstructor]
public FieldWithMetaPersonIdentifier(PersonIdentifier value, MetaFields? meta)
{
Value = value;
Meta = meta;
}
public PersonIdentifier Value { get; }
public MetaFields? Meta { get; }
}
public class FieldWithMetaNaturalPersonRoleEnum : IEnumFieldWithMeta
{
[JsonConstructor]
public FieldWithMetaNaturalPersonRoleEnum(Enums.NaturalPersonRole value, MetaFields? meta)
{
Value = value;
Meta = meta;
}
[JsonConverter(typeof(StringEnumConverter))]
public Enums.NaturalPersonRole Value { get; }
public MetaFields? Meta { get; }
}
public class FieldWithMetaPriceSchedule : IFieldWithMeta
{
[JsonConstructor]
public FieldWithMetaPriceSchedule(PriceSchedule value, MetaFields? meta)
{
Value = value;
Meta = meta;
}
public PriceSchedule Value { get; }
public MetaFields? Meta { get; }
}
public class FieldWithMetaNonNegativeQuantitySchedule : IFieldWithMeta
{
[JsonConstructor]
public FieldWithMetaNonNegativeQuantitySchedule(NonNegativeQuantitySchedule value, MetaFields? meta)
{
Value = value;
Meta = meta;
}
public NonNegativeQuantitySchedule Value { get; }
public MetaFields? Meta { get; }
}
public class FieldWithMetaObservable : IFieldWithMeta
{
[JsonConstructor]
public FieldWithMetaObservable(Observable value, MetaFields? meta)
{
Value = value;
Meta = meta;
}
public Observable Value { get; }
public MetaFields? Meta { get; }
}
public class FieldWithMetaAssetClassEnum : IEnumFieldWithMeta
{
[JsonConstructor]
public FieldWithMetaAssetClassEnum(Enums.AssetClass value, MetaFields? meta)
{
Value = value;
Meta = meta;
}
[JsonConverter(typeof(StringEnumConverter))]
public Enums.AssetClass Value { get; }
public MetaFields? Meta { get; }
}
public class FieldWithMetaEntityTypeEnum : IEnumFieldWithMeta
{
[JsonConstructor]
public FieldWithMetaEntityTypeEnum(Enums.EntityType value, MetaFields? meta)
{
Value = value;
Meta = meta;
}
[JsonConverter(typeof(StringEnumConverter))]
public Enums.EntityType Value { get; }
public MetaFields? Meta { get; }
}
public class FieldWithMetaResourceTypeEnum : IEnumFieldWithMeta
{
[JsonConstructor]
public FieldWithMetaResourceTypeEnum(Enums.ResourceType value, MetaFields? meta)
{
Value = value;
Meta = meta;
}
[JsonConverter(typeof(StringEnumConverter))]
public Enums.ResourceType Value { get; }
public MetaFields? Meta { get; }
}
public class FieldWithMetaRestructuringEnum : IEnumFieldWithMeta
{
[JsonConstructor]
public FieldWithMetaRestructuringEnum(Enums.Restructuring value, MetaFields? meta)
{
Value = value;
Meta = meta;
}
[JsonConverter(typeof(StringEnumConverter))]
public Enums.Restructuring Value { get; }
public MetaFields? Meta { get; }
}
public class FieldWithMetaSettledEntityMatrixSourceEnum : IEnumFieldWithMeta
{
[JsonConstructor]
public FieldWithMetaSettledEntityMatrixSourceEnum(Enums.SettledEntityMatrixSource value, MetaFields? meta)
{
Value = value;
Meta = meta;
}
[JsonConverter(typeof(StringEnumConverter))]
public Enums.SettledEntityMatrixSource Value { get; }
public MetaFields? Meta { get; }
}
public class FieldWithMetaSpreadScheduleTypeEnum : IEnumFieldWithMeta
{
[JsonConstructor]
public FieldWithMetaSpreadScheduleTypeEnum(Enums.SpreadScheduleType value, MetaFields? meta)
{
Value = value;
Meta = meta;
}
[JsonConverter(typeof(StringEnumConverter))]
public Enums.SpreadScheduleType Value { get; }
public MetaFields? Meta { get; }
}
public class FieldWithMetaIdentifier : IFieldWithMeta
{
[JsonConstructor]
public FieldWithMetaIdentifier(Identifier value, MetaFields? meta)
{
Value = value;
Meta = meta;
}
public Identifier Value { get; }
public MetaFields? Meta { get; }
}
public class MetaFields
{
[JsonConstructor]
public MetaFields(string? scheme, string? globalKey, string? externalKey, IEnumerable location)
{
Scheme = scheme;
GlobalKey = globalKey;
ExternalKey = externalKey;
Location = location;
}
public string? Scheme { get; }
public string? GlobalKey { get; }
public string? ExternalKey { get; }
public IEnumerable Location { get; }
}
public class MetaAndTemplateFields
{
[JsonConstructor]
public MetaAndTemplateFields(string? scheme, string? globalKey, string? externalKey, string? templateGlobalReference, IEnumerable location)
{
Scheme = scheme;
GlobalKey = globalKey;
ExternalKey = externalKey;
TemplateGlobalReference = templateGlobalReference;
Location = location;
}
public string? Scheme { get; }
public string? GlobalKey { get; }
public string? ExternalKey { get; }
public string? TemplateGlobalReference { get; }
public IEnumerable Location { get; }
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy