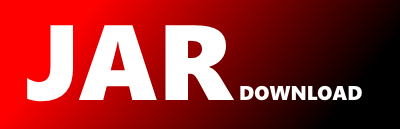
s.fisco-bcos-tars-sdk.3.5.0.source-code.Transaction Maven / Gradle / Ivy
The newest version!
/* ----------------------------------------------------------------------------
* This file was automatically generated by SWIG (https://www.swig.org).
* Version 4.1.1
*
* Do not make changes to this file unless you know what you are doing - modify
* the SWIG interface file instead.
* ----------------------------------------------------------------------------- */
package org.fisco.bcos.sdk.tars;
public class Transaction {
private transient long swigCPtr;
private transient boolean swigCMemOwn;
protected Transaction(long cPtr, boolean cMemoryOwn) {
swigCMemOwn = cMemoryOwn;
swigCPtr = cPtr;
}
protected static long getCPtr(Transaction obj) {
return (obj == null) ? 0 : obj.swigCPtr;
}
protected void swigSetCMemOwn(boolean own) {
swigCMemOwn = own;
}
@SuppressWarnings("deprecation")
protected void finalize() {
delete();
}
public synchronized void delete() {
if (swigCPtr != 0) {
if (swigCMemOwn) {
swigCMemOwn = false;
bcosJNI.delete_Transaction(swigCPtr);
}
swigCPtr = 0;
}
}
public void decode(SWIGTYPE_p_bytesConstRef _txData) {
bcosJNI.Transaction_decode(swigCPtr, this, SWIGTYPE_p_bytesConstRef.getCPtr(_txData));
}
public void encode(SWIGTYPE_p_std__vectorT_unsigned_char_t txData) {
bcosJNI.Transaction_encode(swigCPtr, this, SWIGTYPE_p_std__vectorT_unsigned_char_t.getCPtr(txData));
}
public SWIGTYPE_p_bcos__h256 hash() {
return new SWIGTYPE_p_bcos__h256(bcosJNI.Transaction_hash(swigCPtr, this), true);
}
public void verify(Hash hashImpl, SignatureCrypto signatureImpl) {
bcosJNI.Transaction_verify(swigCPtr, this, Hash.getCPtr(hashImpl), hashImpl, SignatureCrypto.getCPtr(signatureImpl), signatureImpl);
}
public int version() {
return bcosJNI.Transaction_version(swigCPtr, this);
}
public SWIGTYPE_p_std__string_view chainId() {
return new SWIGTYPE_p_std__string_view(bcosJNI.Transaction_chainId(swigCPtr, this), true);
}
public SWIGTYPE_p_std__string_view groupId() {
return new SWIGTYPE_p_std__string_view(bcosJNI.Transaction_groupId(swigCPtr, this), true);
}
public long blockLimit() {
return bcosJNI.Transaction_blockLimit(swigCPtr, this);
}
public String nonce() {
return bcosJNI.Transaction_nonce(swigCPtr, this);
}
public void setNonce(String arg0) {
bcosJNI.Transaction_setNonce(swigCPtr, this, arg0);
}
public SWIGTYPE_p_std__string_view to() {
return new SWIGTYPE_p_std__string_view(bcosJNI.Transaction_to(swigCPtr, this), true);
}
public SWIGTYPE_p_std__string_view abi() {
return new SWIGTYPE_p_std__string_view(bcosJNI.Transaction_abi(swigCPtr, this), true);
}
public SWIGTYPE_p_std__string_view extraData() {
return new SWIGTYPE_p_std__string_view(bcosJNI.Transaction_extraData(swigCPtr, this), true);
}
public void setExtraData(String _extraData) {
bcosJNI.Transaction_setExtraData(swigCPtr, this, _extraData);
}
public SWIGTYPE_p_std__string_view sender() {
return new SWIGTYPE_p_std__string_view(bcosJNI.Transaction_sender(swigCPtr, this), true);
}
public SWIGTYPE_p_bcos__bytesConstRef input() {
return new SWIGTYPE_p_bcos__bytesConstRef(bcosJNI.Transaction_input(swigCPtr, this), true);
}
public long importTime() {
return bcosJNI.Transaction_importTime(swigCPtr, this);
}
public void setImportTime(long _importTime) {
bcosJNI.Transaction_setImportTime(swigCPtr, this, _importTime);
}
public TransactionType type() {
return TransactionType.swigToEnum(bcosJNI.Transaction_type(swigCPtr, this));
}
public void forceSender(SWIGTYPE_p_std__vectorT_unsigned_char_t _sender) {
bcosJNI.Transaction_forceSender(swigCPtr, this, SWIGTYPE_p_std__vectorT_unsigned_char_t.getCPtr(_sender));
}
public SWIGTYPE_p_bcos__bytesConstRef signatureData() {
return new SWIGTYPE_p_bcos__bytesConstRef(bcosJNI.Transaction_signatureData(swigCPtr, this), true);
}
public int attribute() {
return bcosJNI.Transaction_attribute(swigCPtr, this);
}
public void setAttribute(int attribute) {
bcosJNI.Transaction_setAttribute(swigCPtr, this, attribute);
}
public SWIGTYPE_p_std__functionT_void_fError__Ptr_bcos__protocol__TransactionSubmitResult__PtrF_t takeSubmitCallback() {
return new SWIGTYPE_p_std__functionT_void_fError__Ptr_bcos__protocol__TransactionSubmitResult__PtrF_t(bcosJNI.Transaction_takeSubmitCallback(swigCPtr, this), true);
}
public SWIGTYPE_p_std__functionT_void_fError__Ptr_bcos__protocol__TransactionSubmitResult__PtrF_t submitCallback() {
return new SWIGTYPE_p_std__functionT_void_fError__Ptr_bcos__protocol__TransactionSubmitResult__PtrF_t(bcosJNI.Transaction_submitCallback(swigCPtr, this), false);
}
public void setSubmitCallback(SWIGTYPE_p_std__functionT_void_fError__Ptr_bcos__protocol__TransactionSubmitResult__PtrF_t _submitCallback) {
bcosJNI.Transaction_setSubmitCallback(swigCPtr, this, SWIGTYPE_p_std__functionT_void_fError__Ptr_bcos__protocol__TransactionSubmitResult__PtrF_t.getCPtr(_submitCallback));
}
public boolean synced() {
return bcosJNI.Transaction_synced(swigCPtr, this);
}
public void setSynced(boolean _synced) {
bcosJNI.Transaction_setSynced(swigCPtr, this, _synced);
}
public boolean sealed() {
return bcosJNI.Transaction_sealed(swigCPtr, this);
}
public void setSealed(boolean _sealed) {
bcosJNI.Transaction_setSealed(swigCPtr, this, _sealed);
}
public boolean invalid() {
return bcosJNI.Transaction_invalid(swigCPtr, this);
}
public void setInvalid(boolean _invalid) {
bcosJNI.Transaction_setInvalid(swigCPtr, this, _invalid);
}
public void setSystemTx(boolean _systemTx) {
bcosJNI.Transaction_setSystemTx(swigCPtr, this, _systemTx);
}
public boolean systemTx() {
return bcosJNI.Transaction_systemTx(swigCPtr, this);
}
public void setBatchId(int _batchId) {
bcosJNI.Transaction_setBatchId(swigCPtr, this, _batchId);
}
public int batchId() {
return bcosJNI.Transaction_batchId(swigCPtr, this);
}
public void setBatchHash(SWIGTYPE_p_bcos__h256 _hash) {
bcosJNI.Transaction_setBatchHash(swigCPtr, this, SWIGTYPE_p_bcos__h256.getCPtr(_hash));
}
public SWIGTYPE_p_bcos__h256 batchHash() {
return new SWIGTYPE_p_bcos__h256(bcosJNI.Transaction_batchHash(swigCPtr, this), false);
}
public boolean storeToBackend() {
return bcosJNI.Transaction_storeToBackend(swigCPtr, this);
}
public void setStoreToBackend(boolean _storeToBackend) {
bcosJNI.Transaction_setStoreToBackend(swigCPtr, this, _storeToBackend);
}
public final static class Attribute {
public final static Transaction.Attribute EVM_ABI_CODEC = new Transaction.Attribute("EVM_ABI_CODEC", bcosJNI.Transaction_EVM_ABI_CODEC_get());
public final static Transaction.Attribute LIQUID_SCALE_CODEC = new Transaction.Attribute("LIQUID_SCALE_CODEC", bcosJNI.Transaction_LIQUID_SCALE_CODEC_get());
public final static Transaction.Attribute DAG = new Transaction.Attribute("DAG", bcosJNI.Transaction_DAG_get());
public final static Transaction.Attribute LIQUID_CREATE = new Transaction.Attribute("LIQUID_CREATE", bcosJNI.Transaction_LIQUID_CREATE_get());
public final int swigValue() {
return swigValue;
}
public String toString() {
return swigName;
}
public static Attribute swigToEnum(int swigValue) {
if (swigValue < swigValues.length && swigValue >= 0 && swigValues[swigValue].swigValue == swigValue)
return swigValues[swigValue];
for (int i = 0; i < swigValues.length; i++)
if (swigValues[i].swigValue == swigValue)
return swigValues[i];
throw new IllegalArgumentException("No enum " + Attribute.class + " with value " + swigValue);
}
private Attribute(String swigName) {
this.swigName = swigName;
this.swigValue = swigNext++;
}
private Attribute(String swigName, int swigValue) {
this.swigName = swigName;
this.swigValue = swigValue;
swigNext = swigValue+1;
}
private Attribute(String swigName, Attribute swigEnum) {
this.swigName = swigName;
this.swigValue = swigEnum.swigValue;
swigNext = this.swigValue+1;
}
private static Attribute[] swigValues = { EVM_ABI_CODEC, LIQUID_SCALE_CODEC, DAG, LIQUID_CREATE };
private static int swigNext = 0;
private final int swigValue;
private final String swigName;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy