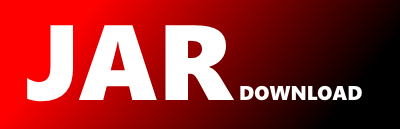
jquery-fracs-0.15.0.jquery.fracs-0.15.0.min.js Maven / Gradle / Ivy
/* jQuery.fracs 0.15.0 - http://larsjung.de/jquery-fracs/ */
!function(){"use strict";function t(t,n){return typeof t===n}function n(t,n){return t instanceof n}function i(t){return t&&t.nodeType}function e(t){return i(t)?t:n(t,g)?t[0]:void 0}function o(t,n,i){return g.each(t,function(t,e){i=n.call(e,i,t,e)}),i}function r(t,n,i){var e,o,r;if(t===n)return!0;if(!t||!n||t.constructor!==n.constructor)return!1;for(e=0,o=i.length;o>e;e+=1){if(r=i[e],t[r]&&y(t[r].equals)&&!t[r].equals(n[r]))return!1;if(t[r]!==n[r])return!1}return!0}function s(t,n,i,e){this.left=E(t),this.top=E(n),this.width=E(i),this.height=E(e),this.right=this.left+this.width,this.bottom=this.top+this.height}function h(t,n,i,e){this.visible=t||0,this.viewport=n||0,this.possible=i||0,this.rects=e&&T({},e)||null}function l(t,n){this.els=t,this.viewport=n}function c(t,n,i){var e;return g.inArray(i,q)>=0?e=s.ofElement(t):g.inArray(i,S)>=0&&(e=h.of(t,n)),e?e[i]:0}function u(t,n){return t.val-n.val}function a(t,n){return n.val-t.val}function f(t){var n=s.ofContent(t,!0),i=s.ofViewport(t,!0),e=n.width-i.width,o=n.height-i.height;this.content=n,this.viewport=i,this.width=0>=e?null:i.left/e,this.height=0>=o?null:i.top/o,this.left=i.left,this.top=i.top,this.right=n.right-i.right,this.bottom=n.bottom-i.bottom}function p(t){this.el=t||window}function d(t,n){this.context=t,this.viewport=n,this.init()}function v(t,n,i,e){this.context=new l(t,n),this.property=i,this.descending=e,this.init()}function w(t){t&&t!==window&&t!==document?(this.context=t,this.$autoTarget=g(t)):this.context=window,this.init()}var g=jQuery,m=g(window),b=g(document),T=g.extend,y=g.isFunction,k=Math.max,V=Math.min,E=Math.round,x=function(){var t={},n=1;return function(i){return i?(t[i]||(t[i]=n,n+=1),t[i]):0}}();T(s.prototype,{equals:function(t){return r(this,t,["left","top","width","height"])},area:function(){return this.width*this.height},relativeTo:function(t){return new s(this.left-t.left,this.top-t.top,this.width,this.height)},intersection:function(t){if(!n(t,s))return null;var i=k(this.left,t.left),e=V(this.right,t.right),o=k(this.top,t.top),r=V(this.bottom,t.bottom),h=e-i,l=r-o;return h>=0&&l>=0?new s(i,o,h,l):null},envelope:function(t){if(!n(t,s))return this;var i=V(this.left,t.left),e=k(this.right,t.right),o=V(this.top,t.top),r=k(this.bottom,t.bottom),h=e-i,l=r-o;return new s(i,o,h,l)}}),T(s,{ofContent:function(t,n){return t&&t!==document&&t!==window?n?new s(0,0,t.scrollWidth,t.scrollHeight):new s(t.offsetLeft-t.scrollLeft,t.offsetTop-t.scrollTop,t.scrollWidth,t.scrollHeight):new s(0,0,b.width(),b.height())},ofViewport:function(t,n){return t&&t!==document&&t!==window?n?new s(t.scrollLeft,t.scrollTop,t.clientWidth,t.clientHeight):new s(t.offsetLeft,t.offsetTop,t.clientWidth,t.clientHeight):new s(m.scrollLeft(),m.scrollTop(),m.width(),m.height())},ofElement:function(t){var n=g(t);if(!n.is(":visible"))return null;var i=n.offset();return new s(i.left,i.top,n.outerWidth(),n.outerHeight())}}),T(h.prototype,{equals:function(t){return this.fracsEqual(t)&&this.rectsEqual(t)},fracsEqual:function(t){return r(this,t,["visible","viewport","possible"])},rectsEqual:function(t){return r(this.rects,t.rects,["document","element","viewport"])}}),T(h,{of:function(t,n){var e,o,r;return t=i(t)&&s.ofElement(t)||t,n=i(n)&&s.ofViewport(n)||n||s.ofViewport(),t instanceof s&&(e=t.intersection(n))?(o=e.area(),r=V(t.width,n.width)*V(t.height,n.height),new h(o/t.area(),o/n.area(),o/r,{document:e,element:e.relativeTo(t),viewport:e.relativeTo(n)})):new h}});var q=["width","height","left","right","top","bottom"],S=["possible","visible","viewport"];T(l.prototype,{sorted:function(t,n){var i=this.viewport;return g.map(this.els,function(n){return{el:n,val:c(n,i,t)}}).sort(n?a:u)},best:function(t,n){return this.els.length?this.sorted(t,n)[0]:null}}),T(f.prototype,{equals:function(t){return r(this,t,["width","height","left","top","right","bottom","content","viewport"])}}),T(p.prototype,{equals:function(t){return r(this,t,["el"])},scrollState:function(){return new f(this.el)},scrollTo:function(t,n,i){var e=g(this.el===window?"html,body":this.el);t=t||0,n=n||0,i=isNaN(i)?1e3:i,e.stop(!0).animate({scrollLeft:t,scrollTop:n},i)},scroll:function(t,n,i){var e=this.el===window?m:g(this.el);t=t||0,n=n||0,this.scrollTo(e.scrollLeft()+t,e.scrollTop()+n,i)},scrollToRect:function(t,n,i,e){n=n||0,i=i||0,this.scrollTo(t.left-n,t.top-i,e)},scrollToElement:function(t,n,i,e){var o=s.ofElement(t).relativeTo(s.ofContent(this.el));this.scrollToRect(o,n,i,e)}});var C={init:function(){this.callbacks=g.Callbacks("memory unique"),this.currVal=null,this.prevVal=null,this.checkProxy=g.proxy(this.check,this),this.autoCheck()},bind:function(t){this.callbacks.add(t)},unbind:function(t){t?this.callbacks.remove(t):this.callbacks.empty()},trigger:function(){this.callbacks.fireWith(this.context,[this.currVal,this.prevVal])},check:function(t){var n=this.updatedValue(t);return void 0===n?!1:(this.prevVal=this.currVal,this.currVal=n,this.trigger(),!0)},$autoTarget:m,autoEvents:"load resize scroll",autoCheck:function(t){this.$autoTarget[t===!1?"off":"on"](this.autoEvents,this.checkProxy)}};T(d.prototype,C,{updatedValue:function(){var t=h.of(this.context,this.viewport);return this.currVal&&this.currVal.equals(t)?void 0:t}}),T(v.prototype,C,{updatedValue:function(){var t=this.context.best(this.property,this.descending);return t&&(t=t.val>0?t.el:null,this.currVal!==t)?t:void 0}}),T(w.prototype,C,{updatedValue:function(){var t=new f(this.context);return this.currVal&&this.currVal.equals(t)?void 0:t}});var L=function(t,n){var i=[].slice,e=jQuery,o=e.extend,r=e.isFunction,s=o({},n),h=function(n,i,o,s){return o=r(o)?o.apply(n,i):o,r(s[o])?s[o].apply(n,i):void e.error('Method "'+o+'" does not exist on jQuery.'+t)},l=function(){return h(this,i.call(arguments),s.defaultStatic,l)},c=function(t){return r(c[t])?c[t].apply(this,i.call(arguments,1)):h(this,i.call(arguments),s.defaultMethod,c)},u=function(t){t&&(o(l,t.statics),o(c,t.methods)),l.modplug=u};u.prev={statics:e[t],methods:e.fn[t]},u(n),e[t]=l,e.fn[t]=c},M="fracs";L(M,{statics:{version:"0.15.0",Rect:s,Fractions:h,Group:l,ScrollState:f,Viewport:p,FracsCallbacks:d,GroupCallbacks:v,ScrollStateCallbacks:w,fracs:function(t,n){return h.of(t,n)}},methods:{content:function(t){return this.length?s.ofContent(this[0],t):null},envelope:function(){return o(this,function(t){var n=s.ofElement(this);return t?t.envelope(n):n})},fracs:function(n,i,o){t(n,"string")||(o=i,i=n,n=null),y(i)||(o=i,i=null),o=e(o);var r=M+".fracs."+x(o);return"unbind"===n?this.each(function(){var t=g(this).data(r);t&&t.unbind(i)}):"check"===n?this.each(function(){var t=g(this).data(r);t&&t.check()}):y(i)?this.each(function(){var t=g(this),n=t.data(r);n||(n=new d(this,o),t.data(r,n)),n.bind(i)}):this.length?h.of(this[0],o):null},intersection:function(){return o(this,function(t){var n=s.ofElement(this);return t?t.intersection(n):n})},max:function(t,n,i){return y(n)||(i=n,n=null),i=e(i),n?(new v(this,i,t,!0).bind(n),this):this.pushStack(new l(this,i).best(t,!0).el)},min:function(t,n,i){return y(n)||(i=n,n=null),i=e(i),n?(new v(this,i,t).bind(n),this):this.pushStack(new l(this,i).best(t).el)},rect:function(){return this.length?s.ofElement(this[0]):null},scrollState:function(n,i){var e=M+".scrollState";return t(n,"string")||(i=n,n=null),"unbind"===n?this.each(function(){var t=g(this).data(e);t&&t.unbind(i)}):"check"===n?this.each(function(){var t=g(this).data(e);t&&t.check()}):y(i)?this.each(function(){var t=g(this),n=t.data(e);n||(n=new w(this),t.data(e,n)),n.bind(i)}):this.length?new f(this[0]):null},scroll:function(t,n,i){return this.each(function(){new p(this).scroll(t,n,i)})},scrollTo:function(t,n,i,o){return g.isNumeric(t)&&(o=i,i=n,n=t,t=null),t=e(t),this.each(function(){t?new p(this).scrollToElement(t,n,i,o):new p(this).scrollTo(n,i,o)})},scrollToThis:function(t,n,i,o){return o=new p(e(o)),o.scrollToElement(this[0],t,n,i),this},softLink:function(t,n,i,o){return o=new p(e(o)),this.filter("a[href^=#]").each(function(){var e=g(this);e.on("click",function(){o.scrollToElement(g(e.attr("href"))[0],t,n,i)})})},sort:function(n,i,o){return t(i,"boolean")||(o=i,i=null),o=e(o),this.pushStack(g.map(new l(this,o).sorted(n,!i),function(t){return t.el}))},viewport:function(t){return this.length?s.ofViewport(this[0],t):null}},defaultStatic:"fracs",defaultMethod:"fracs"})}();
© 2015 - 2025 Weber Informatics LLC | Privacy Policy