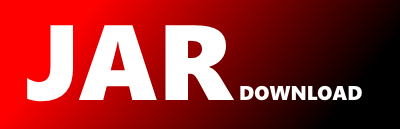
echopointng.ui.resource.js.stackedpaneex.js Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ibis-echo2 Show documentation
Show all versions of ibis-echo2 Show documentation
Echo2 bundled with Echo2_Extras, Echo2_FileTransfer and echopointing and various improvements/bugfixes
/*
* This file is part of the Echo Point Project. This project is a collection of
* Components that have extended the Echo Web Application Framework.
*
* Version: MPL 1.1/GPL 2.0/LGPL 2.1
*
* The contents of this file are subject to the Mozilla Public License Version
* 1.1 (the "License"); you may not use this file except in compliance with the
* License. You may obtain a copy of the License at http://www.mozilla.org/MPL/
*
* Software distributed under the License is distributed on an "AS IS" basis,
* WITHOUT WARRANTY OF ANY KIND, either express or implied. See the License for
* the specific language governing rights and limitations under the License.
*
* Alternatively, the contents of this file may be used under the terms of
* either the GNU General Public License Version 2 or later (the "GPL"), or the
* GNU Lesser General Public License Version 2.1 or later (the "LGPL"), in which
* case the provisions of the GPL or the LGPL are applicable instead of those
* above. If you wish to allow use of your version of this file only under the
* terms of either the GPL or the LGPL, and not to allow others to use your
* version of this file under the terms of the MPL, indicate your decision by
* deleting the provisions above and replace them with the notice and other
* provisions required by the GPL or the LGPL. If you do not delete the
* provisions above, a recipient may use your version of this file under the
* terms of any one of the MPL, the GPL or the LGPL.
*/
/*
* This design for this file was taken from the Echo2
* TabPane which is Copyright (C) 2005-2006 NextApp, Inc.
*
*/
/*
* This file is part of the Echo2 Extras Project.
* Copyright (C) 2005-2006 NextApp, Inc.
*
* Version: MPL 1.1/GPL 2.0/LGPL 2.1
*
* The contents of this file are subject to the Mozilla Public License Version
* 1.1 (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
* http://www.mozilla.org/MPL/
*
* Software distributed under the License is distributed on an "AS IS" basis,
* WITHOUT WARRANTY OF ANY KIND, either express or implied. See the License
* for the specific language governing rights and limitations under the
* License.
*
* Alternatively, the contents of this file may be used under the terms of
* either the GNU General Public License Version 2 or later (the "GPL"), or
* the GNU Lesser General Public License Version 2.1 or later (the "LGPL"),
* in which case the provisions of the GPL or the LGPL are applicable instead
* of those above. If you wish to allow use of your version of this file only
* under the terms of either the GPL or the LGPL, and not to allow others to
* use your version of this file under the terms of the MPL, indicate your
* decision by deleting the provisions above and replace them with the notice
* and other provisions required by the GPL or the LGPL. If you do not delete
* the provisions above, a recipient may use your version of this file under
* the terms of any one of the MPL, the GPL or the LGPL.
*/
/**
* EPStackedPaneEx Object/Namespace/Constructor.
*
* @param elementId the root id of the tab pane.
* @param containerElementId the id of the DOM element which will contain the
* tab pane.
* @param activePaneId the id of the active tab (if applicable)
*/
EPStackedPaneEx = function(elementId, containerElementId, activePaneId) {
this.elementId = elementId;
this.containerElementId = containerElementId;
this.activePaneId = activePaneId;
this.panes = new Array();
};
EPStackedPaneEx.prototype.create = function() {
var containerElement = document.getElementById(this.containerElementId);
if (!containerElement) {
throw "Container element of StackedPaneEx not found: " + this.containerElementId;
}
this.stackedPaneDivElement = document.createElement("div");
this.stackedPaneDivElement.id = this.elementId;
this.stackedPaneDivElement.style.position = "absolute";
this.stackedPaneDivElement.style.width = "100%";
this.stackedPaneDivElement.style.height = "100%";
this.stackedPaneDivElement.style.overflow = "auto";
//
// rendered on the server and sent down to us
EchoCssUtil.applyStyle(this.stackedPaneDivElement, this.styleText);
//
//
var attrs = this.standardWebSupportE.attributes;
for(var index=0; index array.length) {
throw "Array index of bounds: " + index + " (size=" + array.length + ")";
} else {
for (var i = array.length - 1; i >= index; --i) {
array[i + 1] = array[i];
}
array[index] = element;
}
};
EPStackedPaneEx.Arrays.removeElement = function(array, element) {
var index = EPStackedPaneEx.Arrays.indexOf(array, element);
if (index == -1) {
return;
}
EPStackedPaneEx.Arrays.removeIndex(array, index);
};
EPStackedPaneEx.Arrays.indexOf = function(array, element) {
for (var i = 0; i < array.length; ++i) {
if (array[i] == element) {
return i;
}
}
return -1;
}
EPStackedPaneEx.Arrays.removeIndex = function(array, index) {
for (i = index; i < array.length - 1; ++i) {
array[i] = array[i + 1];
}
array.length = array.length - 1;
};
/**
* Static object/namespace for StackedPaneEx MessageProcessor
* implementation.
*/
EPStackedPaneEx.MessageProcessor = function() { };
/**
* MessageProcessor process() implementation
* (invoked by ServerMessage processor).
*
* @param messagePartElement the message-part
element to process.
*/
EPStackedPaneEx.MessageProcessor.process = function(messagePartElement) {
for (var i = 0; i < messagePartElement.childNodes.length; ++i) {
if (messagePartElement.childNodes[i].nodeType === 1) {
switch (messagePartElement.childNodes[i].tagName) {
case "add-pane":
EPStackedPaneEx.MessageProcessor.processAddPane(messagePartElement.childNodes[i]);
break;
case "dispose":
EPStackedPaneEx.MessageProcessor.processDispose(messagePartElement.childNodes[i]);
break;
case "init":
EPStackedPaneEx.MessageProcessor.processInit(messagePartElement.childNodes[i]);
break;
case "remove-pane":
EPStackedPaneEx.MessageProcessor.processRemovePane(messagePartElement.childNodes[i]);
break;
case "set-active-pane":
EPStackedPaneEx.MessageProcessor.processSetActivePane(messagePartElement.childNodes[i]);
break;
}
}
}
};
/**
* Processes an add-pane
message to add a new pane to the StackedPaneEx.
*
* @param addPaneMessageElement the add-pane
element to process
*/
EPStackedPaneEx.MessageProcessor.processAddPane = function(addPaneMessageElement) {
var elementId = addPaneMessageElement.getAttribute("eid");
var stackedPane = EPStackedPaneEx.getComponent(elementId);
if (!stackedPane) {
throw "StackedPaneEx not found with id: " + elementId;
}
var paneId = addPaneMessageElement.getAttribute("pane-id");
var paneIndex = addPaneMessageElement.getAttribute("pane-index");
var rendered = addPaneMessageElement.getAttribute("rendered") == "true";
var pane = new EPStackedPaneEx.Pane(paneId, rendered);
stackedPane.addPane(pane, paneIndex);
};
/**
* Processes an dispose
message to dispose the state of a
* StackedPaneEx component that is being removed.
*
* @param disposeMessageElement the dispose
element to process
*/
EPStackedPaneEx.MessageProcessor.processDispose = function(disposeMessageElement) {
var elementId = disposeMessageElement.getAttribute("eid");
var stackedPane = EPStackedPaneEx.getComponent(elementId);
if (stackedPane) {
stackedPane.dispose();
}
};
/**
* Processes an init
message to initialize the state of a
* StackedPaneEx component that is being added.
*
* @param initMessageElement the init
element to process
*/
EPStackedPaneEx.MessageProcessor.processInit = function(initMessageElement) {
var elementId = initMessageElement.getAttribute("eid");
var containerElementId = initMessageElement.getAttribute("container-eid");
var activePaneId = initMessageElement.getAttribute("active-pane");
var stackedPane = new EPStackedPaneEx(elementId, containerElementId, activePaneId);
stackedPane.enabled = initMessageElement.getAttribute("enabled") != "false";
stackedPane.styleText = initMessageElement.getAttribute("style");
stackedPane.standardWebSupportE = initMessageElement.getElementsByTagName('standardWebSupport')[0];
stackedPane.create();
};
/**
* Processes a remove-pane
message to remove a pane from the StackedPaneEx.
*
* @param removePaneMessageElement the remove-pane
element to process
*/
EPStackedPaneEx.MessageProcessor.processRemovePane = function(removePaneMessageElement) {
var elementId = removePaneMessageElement.getAttribute("eid");
var paneId = removePaneMessageElement.getAttribute("pane-id");
var stackedPane = EPStackedPaneEx.getComponent(elementId);
var pane = stackedPane.getPaneById(paneId);
if (pane) {
stackedPane.removePane(pane);
}
};
/**
* Processes a set-active-pane
message to set the active pane.
*
* @param setActivePaneMessageElement the set-active-pane
element to process
*/
EPStackedPaneEx.MessageProcessor.processSetActivePane = function(setActivePaneMessageElement) {
var stackedPaneId = setActivePaneMessageElement.getAttribute("eid");
var paneId = setActivePaneMessageElement.getAttribute("active-pane");
var stackedPane = EPStackedPaneEx.getComponent(stackedPaneId);
if (paneId != null) {
stackedPane.getPaneById(paneId).rendered = true;
}
stackedPane.selectPane(paneId);
};
© 2015 - 2025 Weber Informatics LLC | Privacy Policy