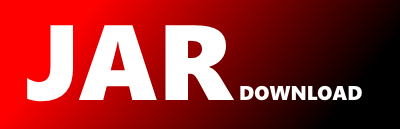
static.js.vendor.openlayers3.tasks.parse-examples.js Maven / Gradle / Ivy
The newest version!
var fs = require('fs');
var path = require('path');
var async = require('async');
var Parser = require('htmlparser2').Parser;
var exampleDir = path.join(__dirname, '..', 'examples');
/**
* List all .html files in the example directory (excluding index.html).
* @param {function(Error, Array.)} callback Called with any error or
* the list of paths to examples.
*/
function listExamples(callback) {
fs.readdir(exampleDir, function(err, items) {
if (err) {
return callback(err);
}
var examplePaths = items.filter(function(item) {
return /\.html$/i.test(item) && item !== 'index.html';
}).map(function(item) {
return path.join(exampleDir, item);
});
callback(null, examplePaths);
});
}
/**
* Parse info from examples.
* @param {Array.} examplePaths Paths to examples.
* @param {function(Error, Array.
© 2015 - 2025 Weber Informatics LLC | Privacy Policy