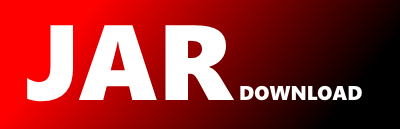
org.jbpm.designer.public.js.Plugins.arrangement.js Maven / Gradle / Ivy
/**
* Copyright (c) 2006
* Martin Czuchra, Nicolas Peters, Daniel Polak, Willi Tscheschner
*
* Permission is hereby granted, free of charge, to any person obtaining a
* copy of this software and associated documentation files (the "Software"),
* to deal in the Software without restriction, including without limitation
* the rights to use, copy, modify, merge, publish, distribute, sublicense,
* and/or sell copies of the Software, and to permit persons to whom the
* Software is furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in
* all copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING
* FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
* DEALINGS IN THE SOFTWARE.
**/
Array.prototype.insertFrom = function(from, to){
to = Math.max(0, to);
from = Math.min( Math.max(0, from), this.length-1 );
var el = this[from];
var old = this.without(el);
var newA = old.slice(0, to);
newA.push(el);
if(old.length > to ){
newA = newA.concat(old.slice(to))
};
return newA;
}
if(!ORYX.Plugins)
ORYX.Plugins = new Object();
ORYX.Plugins.Arrangement = Clazz.extend({
facade: undefined,
construct: function(facade) {
this.facade = facade;
if(!(ORYX.READONLY == true || ORYX.VIEWLOCKED == true)) {
// Z-Ordering
this.facade.offer({
'name':ORYX.I18N.Arrangement.btf,
'functionality': this.setZLevel.bind(this, this.setToTop),
'group': ORYX.I18N.Arrangement.groupZ,
'icon': ORYX.BASE_FILE_PATH + "images/shape_move_front.png",
dropDownGroupIcon : ORYX.BASE_FILE_PATH + "images/shape_move_backwards.png",
'description': ORYX.I18N.Arrangement.btfDesc,
'index': 1,
'minShape': 1});
this.facade.offer({
'name':ORYX.I18N.Arrangement.btb,
'functionality': this.setZLevel.bind(this, this.setToBack),
'group': ORYX.I18N.Arrangement.groupZ,
dropDownGroupIcon : ORYX.BASE_FILE_PATH + "images/shape_move_backwards.png",
'icon': ORYX.BASE_FILE_PATH + "images/shape_move_back.png",
'description': ORYX.I18N.Arrangement.btbDesc,
'index': 2,
'minShape': 1});
this.facade.offer({
'name':ORYX.I18N.Arrangement.bf,
'functionality': this.setZLevel.bind(this, this.setForward),
'group': ORYX.I18N.Arrangement.groupZ,
dropDownGroupIcon : ORYX.BASE_FILE_PATH + "images/shape_move_backwards.png",
'icon': ORYX.BASE_FILE_PATH + "images/shape_move_forwards.png",
'description': ORYX.I18N.Arrangement.bfDesc,
'index': 3,
'minShape': 1});
this.facade.offer({
'name':ORYX.I18N.Arrangement.bb,
'functionality': this.setZLevel.bind(this, this.setBackward),
'group': ORYX.I18N.Arrangement.groupZ,
dropDownGroupIcon : ORYX.BASE_FILE_PATH + "images/shape_move_backwards.png",
'icon': ORYX.BASE_FILE_PATH + "images/shape_move_backwards.png",
'description': ORYX.I18N.Arrangement.bbDesc,
'index': 4,
'minShape': 1});
// Aligment
this.facade.offer({
'name':ORYX.I18N.Arrangement.ab,
'functionality': this.alignShapes.bind(this, [ORYX.CONFIG.EDITOR_ALIGN_BOTTOM]),
'group': ORYX.I18N.Arrangement.groupA,
dropDownGroupIcon : ORYX.BASE_FILE_PATH + "images/shape_align_center.png",
'icon': ORYX.BASE_FILE_PATH + "images/shape_align_bottom.png",
'description': ORYX.I18N.Arrangement.abDesc,
'index': 1,
'minShape': 2});
this.facade.offer({
'name':ORYX.I18N.Arrangement.am,
'functionality': this.alignShapes.bind(this, [ORYX.CONFIG.EDITOR_ALIGN_MIDDLE]),
'group': ORYX.I18N.Arrangement.groupA,
dropDownGroupIcon : ORYX.BASE_FILE_PATH + "images/shape_align_center.png",
'icon': ORYX.BASE_FILE_PATH + "images/shape_align_middle.png",
'description': ORYX.I18N.Arrangement.amDesc,
'index': 2,
'minShape': 2});
this.facade.offer({
'name':ORYX.I18N.Arrangement.at,
'functionality': this.alignShapes.bind(this, [ORYX.CONFIG.EDITOR_ALIGN_TOP]),
'group': ORYX.I18N.Arrangement.groupA,
dropDownGroupIcon : ORYX.BASE_FILE_PATH + "images/shape_align_center.png",
'icon': ORYX.BASE_FILE_PATH + "images/shape_align_top.png",
'description': ORYX.I18N.Arrangement.atDesc,
'index': 3,
'minShape': 2});
this.facade.offer({
'name':ORYX.I18N.Arrangement.al,
'functionality': this.alignShapes.bind(this, [ORYX.CONFIG.EDITOR_ALIGN_LEFT]),
'group': ORYX.I18N.Arrangement.groupA,
dropDownGroupIcon : ORYX.BASE_FILE_PATH + "images/shape_align_center.png",
'icon': ORYX.BASE_FILE_PATH + "images/shape_align_left.png",
'description': ORYX.I18N.Arrangement.alDesc,
'index': 4,
'minShape': 2});
this.facade.offer({
'name':ORYX.I18N.Arrangement.ac,
'functionality': this.alignShapes.bind(this, [ORYX.CONFIG.EDITOR_ALIGN_CENTER]),
'group': ORYX.I18N.Arrangement.groupA,
dropDownGroupIcon : ORYX.BASE_FILE_PATH + "images/shape_align_center.png",
'icon': ORYX.BASE_FILE_PATH + "images/shape_align_center.png",
'description': ORYX.I18N.Arrangement.acDesc,
'index': 5,
'minShape': 2});
this.facade.offer({
'name':ORYX.I18N.Arrangement.ar,
'functionality': this.alignShapes.bind(this, [ORYX.CONFIG.EDITOR_ALIGN_RIGHT]),
'group': ORYX.I18N.Arrangement.groupA,
dropDownGroupIcon : ORYX.BASE_FILE_PATH + "images/shape_align_center.png",
'icon': ORYX.BASE_FILE_PATH + "images/shape_align_right.png",
'description': ORYX.I18N.Arrangement.arDesc,
'index': 6,
'minShape': 2});
this.facade.offer({
'name':ORYX.I18N.Arrangement.as,
'functionality': this.alignShapes.bind(this, [ORYX.CONFIG.EDITOR_ALIGN_SIZE]),
'group': ORYX.I18N.Arrangement.groupA,
dropDownGroupIcon : ORYX.BASE_FILE_PATH + "images/shape_align_center.png",
'icon': ORYX.BASE_FILE_PATH + "images/shape_align_size.png",
'description': ORYX.I18N.Arrangement.asDesc,
'index': 7,
'minShape': 2});
}
this.facade.registerOnEvent(ORYX.CONFIG.EVENT_ARRANGEMENT_TOP, this.setZLevel.bind(this, this.setToTop) );
this.facade.registerOnEvent(ORYX.CONFIG.EVENT_ARRANGEMENT_BACK, this.setZLevel.bind(this, this.setToBack) );
this.facade.registerOnEvent(ORYX.CONFIG.EVENT_ARRANGEMENT_FORWARD, this.setZLevel.bind(this, this.setForward) );
this.facade.registerOnEvent(ORYX.CONFIG.EVENT_ARRANGEMENT_BACKWARD, this.setZLevel.bind(this, this.setBackward) );
},
setZLevel:function(callback, event){
//Command-Pattern for dragging one docker
var zLevelCommand = ORYX.Core.Command.extend({
construct: function(callback, elements, facade){
this.callback = callback;
this.elements = elements;
// For redo, the previous elements get stored
this.elAndIndex = elements.map(function(el){ return {el:el, previous:el.parent.children[el.parent.children.indexOf(el)-1]} })
this.facade = facade;
},
execute: function(){
// Call the defined z-order callback with the elements
this.callback( this.elements )
this.facade.setSelection( this.elements )
},
rollback: function(){
// Sort all elements on the index of there containment
var sortedEl = this.elAndIndex.sortBy( function( el ) {
var value = el.el;
var t = $A(value.node.parentNode.childNodes);
return t.indexOf(value.node);
});
// Every element get setted back bevor the old previous element
for(var i=0; i maxSize.width) {
newBounds.a.x = shape.bounds.upperLeft().x -
(maxSize.width - shape.bounds.width())/2;
newBounds.b.x = shape.bounds.lowerRight().x + (maxSize.width - shape.bounds.width())/2
}
/* If the new height of shape exceeds the maximum height, set height value to maximum. */
if(this.maxHeight > maxSize.height) {
newBounds.a.y = shape.bounds.upperLeft().y -
(maxSize.height - shape.bounds.height())/2;
newBounds.b.y = shape.bounds.lowerRight().y + (maxSize.height - shape.bounds.height())/2
}
/* set bounds of shape */
shape.bounds.set(newBounds);
},
execute: function(){
// align each shape according to the way that was specified.
this.elements.each(function(shape, index) {
this.orgPos[index] = shape.bounds.upperLeft();
var relBounds = this.bounds.clone();
if (shape.parent && !(shape.parent instanceof ORYX.Core.Canvas) ) {
var upL = shape.parent.absoluteBounds().upperLeft();
relBounds.moveBy(-upL.x, -upL.y);
}
switch (this.way) {
// align the shapes in the requested way.
case ORYX.CONFIG.EDITOR_ALIGN_BOTTOM:
shape.bounds.moveTo({
x: shape.bounds.upperLeft().x,
y: relBounds.b.y - shape.bounds.height()
}); break;
case ORYX.CONFIG.EDITOR_ALIGN_MIDDLE:
shape.bounds.moveTo({
x: shape.bounds.upperLeft().x,
y: (relBounds.a.y + relBounds.b.y - shape.bounds.height()) / 2
}); break;
case ORYX.CONFIG.EDITOR_ALIGN_TOP:
shape.bounds.moveTo({
x: shape.bounds.upperLeft().x,
y: relBounds.a.y
}); break;
case ORYX.CONFIG.EDITOR_ALIGN_LEFT:
shape.bounds.moveTo({
x: relBounds.a.x,
y: shape.bounds.upperLeft().y
}); break;
case ORYX.CONFIG.EDITOR_ALIGN_CENTER:
shape.bounds.moveTo({
x: (relBounds.a.x + relBounds.b.x - shape.bounds.width()) / 2,
y: shape.bounds.upperLeft().y
}); break;
case ORYX.CONFIG.EDITOR_ALIGN_RIGHT:
shape.bounds.moveTo({
x: relBounds.b.x - shape.bounds.width(),
y: shape.bounds.upperLeft().y
}); break;
case ORYX.CONFIG.EDITOR_ALIGN_SIZE:
if(shape.isResizable) {
this.orgPos[index] = {a: shape.bounds.upperLeft(), b: shape.bounds.lowerRight()};
this.setBounds(shape, shape.maximumSize);
}
break;
}
//shape.update()
}.bind(this));
this.facade.getCanvas().update();
this.facade.updateSelection();
},
rollback: function(){
this.elements.each(function(shape, index) {
if (this.way == ORYX.CONFIG.EDITOR_ALIGN_SIZE) {
if(shape.isResizable) {shape.bounds.set(this.orgPos[index]);}
} else {shape.bounds.moveTo(this.orgPos[index]);}
}.bind(this));
this.facade.getCanvas().update();
this.facade.updateSelection();
}
})
var command = new commandClass(elements, bounds, maxHeight, maxWidth, parseInt(way), this.facade);
this.facade.executeCommands([command]);
}
});
© 2015 - 2025 Weber Informatics LLC | Privacy Policy