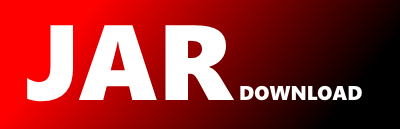
.kotlinx.kotlinx-coroutines-core-common.0.25.3-eap13.source-code.Dispatched.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of kotlinx-coroutines-core-common Show documentation
Show all versions of kotlinx-coroutines-core-common Show documentation
Coroutines support libraries for Kotlin
/*
* Copyright 2016-2018 JetBrains s.r.o. Use of this source code is governed by the Apache 2.0 license.
*/
package kotlinx.coroutines
import kotlinx.coroutines.internal.*
import kotlin.coroutines.*
@Suppress("PrivatePropertyName")
private val UNDEFINED = Symbol("UNDEFINED")
internal class DispatchedContinuation(
@JvmField val dispatcher: CoroutineDispatcher,
@JvmField val continuation: Continuation
) : Continuation by continuation, DispatchedTask {
private var _state: Any? = UNDEFINED
public override var resumeMode: Int = 0
override fun takeState(): Any? {
val state = _state
check(state !== UNDEFINED) // fail-fast if repeatedly invoked
_state = UNDEFINED
return state
}
override val delegate: Continuation
get() = this
override fun resumeWith(result: SuccessOrFailure) {
val context = continuation.context
if (dispatcher.isDispatchNeeded(context)) {
_state = result.toState()
resumeMode = MODE_ATOMIC_DEFAULT
dispatcher.dispatch(context, this)
} else
resumeUndispatchedWith(result)
}
@Suppress("NOTHING_TO_INLINE") // we need it inline to save us an entry on the stack
inline fun resumeCancellable(value: T) {
val context = continuation.context
if (dispatcher.isDispatchNeeded(context)) {
_state = value
resumeMode = MODE_CANCELLABLE
dispatcher.dispatch(context, this)
} else
resumeUndispatched(value)
}
@Suppress("NOTHING_TO_INLINE") // we need it inline to save us an entry on the stack
inline fun resumeCancellableWithException(exception: Throwable) {
val context = continuation.context
if (dispatcher.isDispatchNeeded(context)) {
_state = CompletedExceptionally(exception)
resumeMode = MODE_CANCELLABLE
dispatcher.dispatch(context, this)
} else
resumeUndispatchedWithException(exception)
}
@Suppress("NOTHING_TO_INLINE") // we need it inline to save us an entry on the stack
inline fun resumeUndispatchedWith(result: SuccessOrFailure) {
withCoroutineContext(context) {
continuation.resumeWith(result)
}
}
@Suppress("NOTHING_TO_INLINE") // we need it inline to save us an entry on the stack
inline fun resumeUndispatched(value: T) {
withCoroutineContext(context) {
continuation.resume(value)
}
}
@Suppress("NOTHING_TO_INLINE") // we need it inline to save us an entry on the stack
inline fun resumeUndispatchedWithException(exception: Throwable) {
withCoroutineContext(context) {
continuation.resumeWithException(exception)
}
}
// used by "yield" implementation
internal fun dispatchYield(value: T) {
val context = continuation.context
_state = value
resumeMode = MODE_CANCELLABLE
dispatcher.dispatchYield(context, this)
}
override fun toString(): String =
"DispatchedContinuation[$dispatcher, ${continuation.toDebugString()}]"
}
internal fun Continuation.resumeCancellable(value: T) = when (this) {
is DispatchedContinuation -> resumeCancellable(value)
else -> resume(value)
}
internal fun Continuation.resumeCancellableWithException(exception: Throwable) = when (this) {
is DispatchedContinuation -> resumeCancellableWithException(exception)
else -> resumeWithException(exception)
}
internal fun Continuation.resumeDirect(value: T) = when (this) {
is DispatchedContinuation -> continuation.resume(value)
else -> resume(value)
}
internal fun Continuation.resumeDirectWithException(exception: Throwable) = when (this) {
is DispatchedContinuation -> continuation.resumeWithException(exception)
else -> resumeWithException(exception)
}
/**
* @suppress **This is unstable API and it is subject to change.**
*/
public interface DispatchedTask : Runnable {
public val delegate: Continuation
public val resumeMode: Int get() = MODE_CANCELLABLE
public fun takeState(): Any?
@Suppress("UNCHECKED_CAST")
public fun getSuccessfulResult(state: Any?): T =
state as T
public fun getExceptionalResult(state: Any?): Throwable? =
(state as? CompletedExceptionally)?.cause
public override fun run() {
try {
val delegate = delegate as DispatchedContinuation
val continuation = delegate.continuation
val context = continuation.context
val job = if (resumeMode.isCancellableMode) context[Job] else null
val state = takeState() // NOTE: Must take state in any case, even if cancelled
withCoroutineContext(context) {
if (job != null && !job.isActive)
continuation.resumeWithException(job.getCancellationException())
else {
val exception = getExceptionalResult(state)
if (exception != null)
continuation.resumeWithException(exception)
else
continuation.resume(getSuccessfulResult(state))
}
}
} catch (e: Throwable) {
throw DispatchException("Unexpected exception running $this", e)
}
}
}
/**
* @suppress **This is unstable API and it is subject to change.**
*/
public fun DispatchedTask.dispatch(mode: Int = MODE_CANCELLABLE) {
var useMode = mode
val delegate = this.delegate
if (mode.isDispatchedMode && delegate is DispatchedContinuation<*> && mode.isCancellableMode == resumeMode.isCancellableMode) {
// dispatch directly using this instance's Runnable implementation
val dispatcher = delegate.dispatcher
val context = delegate.context
if (dispatcher.isDispatchNeeded(context)) {
dispatcher.dispatch(context, this)
return // and that's it -- dispatched via fast-path
} else {
useMode = MODE_UNDISPATCHED
}
}
// slow-path - use delegate
val state = takeState()
val exception = getExceptionalResult(state)
if (exception != null) {
delegate.resumeWithExceptionMode(exception, useMode)
} else {
delegate.resumeMode(getSuccessfulResult(state), useMode)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy