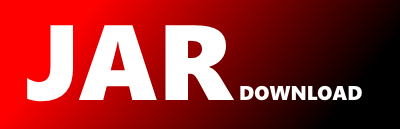
rhino1.7.7.testsrc.tests.menuhead.js Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of rhino Show documentation
Show all versions of rhino Show documentation
Rhino is an open-source implementation of JavaScript written entirely in Java. It is typically
embedded into Java applications to provide scripting to end users.
/* -*- Mode: C++; tab-width: 2; indent-tabs-mode: nil; c-basic-offset: 2 -*- */
/* This Source Code Form is subject to the terms of the Mozilla Public
* License, v. 2.0. If a copy of the MPL was not distributed with this
* file, You can obtain one at http://mozilla.org/MPL/2.0/. */
var gTotalCount = 0;
var gTotalSelected = 0;
function setCursor(aCursor)
{
if (document.body && document.body.style)
{
document.body.style.cursor = aCursor;
}
}
function selectAll(suiteName, testDirName)
{
setCursor('wait');
suiteName = suiteName || '';
testDirName = testDirName || '';
setTimeout('_selectAll("' + suiteName + '", "' + testDirName + '")', 200);
}
function _selectAll(suiteName, testDirName)
{
if (!suiteName)
{
for (suiteName in suites)
{
setAllDirs(suiteName, true);
}
}
else if (!testDirName)
{
setAllDirs(suiteName, true);
}
else
{
setAllTests(suiteName, testDirName, true);
}
setCursor('auto');
}
function selectNone(suiteName, testDirName)
{
setCursor('wait');
suiteName = suiteName || '';
testDirName = testDirName || '';
setTimeout('_selectNone("' + suiteName + '", "' + testDirName + '")', 200);
}
function _selectNone(suiteName, testDirName)
{
if (!suiteName)
{
for (suiteName in suites)
{
setAllDirs(suiteName, false);
}
}
else if (!testDirName)
{
setAllDirs(suiteName, false);
}
else
{
setAllTests(suiteName, testDirName, false);
}
setCursor('auto');
}
function updateCounts(suiteName)
{
var suite = suites[suiteName];
var pct = parseInt((suite.selected / suite.count) * 100);
if (isNaN(pct))
pct = 0;
document.forms.testCases.elements['SUMMARY_' + suiteName].value =
suite.selected + '/' + suite.count +
' (' + pct + '%) selected';
pct = parseInt((gTotalSelected / gTotalCount) * 100);
if (isNaN(pct))
pct = 0;
document.forms.testCases.elements['TOTAL'].value =
gTotalSelected + '/' + gTotalCount + ' (' +
pct + '%) selected';
}
function onRadioClick(radio)
{
var info = radio.id.split(':');
var suiteName = info[0];
var suite = suites[suiteName];
var incr = (radio.checked ? 1 : -1);
suite.selected += incr;
gTotalSelected += incr;
updateCounts(suiteName);
return true;
}
function updateTotals()
{
setCursor('wait');
setTimeout('_updateTotals()', 200);
}
function _updateTotals()
{
gTotalCount = 0;
gTotalSelected = 0;
for (var suiteName in suites)
{
gTotalCount += suites[suiteName].count;
}
for (suiteName in suites)
{
updateCounts(suiteName);
}
setCursor('auto');
}
function setAllDirs(suiteName, value)
{
var testDirs = suites[suiteName].testDirs;
for (var testDirName in testDirs)
{
setAllTests(suiteName, testDirName, value);
}
}
function setAllTests(suiteName, testDirName, value)
{
var suite = suites[suiteName];
var tests = suite.testDirs[testDirName].tests;
var elements = document.forms['testCases'].elements;
var incr = 0;
for (var testName in tests)
{
var radioName = tests[testName].id;
var radio = elements[radioName];
if (radio.checked && !value)
{
incr--;
}
else if (!radio.checked && value)
{
incr++;
}
radio.checked = value;
}
suite.selected += incr;
gTotalSelected += incr;
updateCounts(suiteName);
}
function createList()
{
var suiteName;
var elements = document.forms['testCases'].elements;
var win = window.open('about:blank', 'other_window');
var writer = new CachedWriter(win.document);
win.document.open();
writer.writeln('');
writer.writeln('# Created ' + new Date());
for (suiteName in suites)
{
writer.writeln('# ' + suiteName + ': ' +
elements['SUMMARY_' + suiteName].value);
}
writer.writeln('# TOTAL: ' + elements['TOTAL'].value);
for (suiteName in suites)
{
var testDirs = suites[suiteName].testDirs;
for (var testDirName in testDirs)
{
var tests = testDirs[testDirName].tests;
for (var testName in tests)
{
var radioName = tests[testName].id;
var radio = elements[radioName];
if (radio.checked)
writer.writeln(suiteName + '/' + testDirName + '/' + radio.value);
}
}
}
writer.writeln('<\/pre>');
writer.close();
}
var gTests;
var gTestNumber;
var gWindow;
function executeList()
{
var elements = document.forms['testCases'].elements;
gTests = [];
gTestNumber = -1;
for (var suiteName in suites)
{
var testDirs = suites[suiteName].testDirs;
for (var testDirName in testDirs)
{
var tests = testDirs[testDirName].tests;
for (var testName in tests)
{
var test = tests[testName];
var radioName = test.id;
var radio = elements[radioName];
delete test.testCases;
if (radio.checked)
{
gTests[gTests.length] = test;
test.path = suiteName + '/' + testDirName + '/' + radio.value;
}
}
}
}
runNextTest();
}
function runNextTest()
{
var iTestCase;
if (gTestNumber != -1)
{
// tests have already run in gWindow, collect the results
// for later reporting.
var e;
try
{
var test = gTests[gTestNumber];
test.testCases = [];
//test.testCases = test.testCases.concat(gWindow.gTestcases);
// note MSIE6 has a bug where instead of concating the new arrays
// it concats them to the first element. work around...
var origtestcases = gWindow.gTestcases;
for (iTestCase = 0; iTestCase < origtestcases.length; iTestCase++)
{
// test.testCases[test.testCases.length] = origtestcases[iTestCase];
var origtestcase = origtestcases[iTestCase];
var testCase = test.testCases[test.testCases.length] = {};
testCase.name = new String(origtestcase.name);
testCase.description = new String(origtestcase.description);
testCase.expect = new String(origtestcase.expect);
testCase.actual = new String(origtestcase.actual);
testCase.passed = origtestcase.passed ? true : false;
testCase.reason = new String(origtestcase.reason);
testCase.bugnumber = new String(origtestcase.bugnumber?origtestcase.bugnumber:'');
}
origtestcases = origtestcase = null;
}
catch(e)
{
;
}
}
++gTestNumber;
if (gTestNumber < gTests.length)
{
// run test
test = gTests[gTestNumber];
gWindow = window.open('js-test-driver-' +
document.forms.testCases.doctype.value +
'.html?test=' +
test.path +
';language=' +
document.forms.testCases.language.value,
'output');
if (!gWindow)
{
alert('This test suite requires popup windows.\n' +
'Please enable them for this site.');
}
}
else if (document.forms.testCases.outputformat.value == 'html')
{
// all tests completed, display report
reportHTML();
}
else if (document.forms.testCases.outputformat.value == 'javascript')
{
// all tests completed, display report
reportJavaScript();
}
}
function reportHTML()
{
var errorsOnly = document.forms.testCases.failures.checked;
var totalTestCases = 0;
var totalTestCasesPassed = 0;
var totalTestCasesFailed = 0;
gWindow.document.close();
var writer = new CachedWriter(gWindow.document);
writer.writeln('');
writer.writeln('');
writer.writeln('');
writer.writeln('JavaScript Tests Browser: ' +
navigator.userAgent + ' Language: ' +
document.forms.testCases.language.value +
'<\/title>');
writer.writeln('