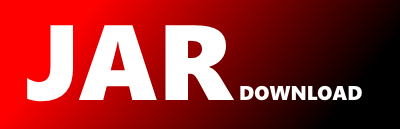
package.dist.esm.components.group.group.js Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of react Show documentation
Show all versions of react Show documentation
Responsive and accessible React UI components built with React and Emotion
The newest version!
"use strict";
"use client";
import { jsx } from 'react/jsx-runtime';
import { memo, forwardRef, Children, useMemo, isValidElement, cloneElement } from 'react';
import { dataAttr } from '../../utils/attr.js';
import { chakra } from '../../styled-system/factory.js';
const StyledGroup = chakra("div", {
base: {
display: "inline-flex",
gap: "0.5rem",
isolation: "isolate",
position: "relative",
"& [data-group-item]": {
_focusVisible: {
zIndex: 1
}
}
},
variants: {
orientation: {
horizontal: {
flexDirection: "row"
},
vertical: {
flexDirection: "column"
}
},
attached: {
true: {
gap: "0!"
}
},
grow: {
true: {
display: "flex",
"& > *": {
flex: 1
}
}
},
stacking: {
"first-on-top": {
"& > [data-group-item]": {
zIndex: "calc(var(--group-count) - var(--group-index))"
}
},
"last-on-top": {
"& > [data-group-item]": {
zIndex: "var(--group-index)"
}
}
}
},
compoundVariants: [
{
orientation: "horizontal",
attached: true,
css: {
"& > *[data-first]": {
borderEndRadius: "0!",
marginEnd: "-1px"
},
"& > *[data-between]": {
borderRadius: "0!",
marginEnd: "-1px"
},
"& > *[data-last]": {
borderStartRadius: "0!"
}
}
},
{
orientation: "vertical",
attached: true,
css: {
"& > *[data-first]": {
borderBottomRadius: "0!",
marginBottom: "-1px"
},
"& > *[data-between]": {
borderRadius: "0!",
marginBottom: "-1px"
},
"& > *[data-last]": {
borderTopRadius: "0!"
}
}
}
],
defaultVariants: {
orientation: "horizontal"
}
});
const Group = memo(
forwardRef(function Group2(props, ref) {
const {
align = "center",
justify = "flex-start",
children,
wrap,
...rest
} = props;
const count = Children.count(children);
const _children = useMemo(() => {
const childArray = Children.toArray(children).filter(
isValidElement
);
return childArray.map((child, index) => {
const childProps = child.props;
return cloneElement(child, {
...childProps,
"data-group-item": "",
"data-first": dataAttr(index === 0),
"data-last": dataAttr(index === count - 1),
"data-between": dataAttr(index > 0 && index < count - 1),
style: {
"--group-count": count,
"--group-index": index,
...childProps?.style ?? {}
}
});
});
}, [children, count]);
return /* @__PURE__ */ jsx(
StyledGroup,
{
ref,
alignItems: align,
justifyContent: justify,
flexWrap: wrap,
...rest,
children: _children
}
);
})
);
export { Group };
© 2015 - 2025 Weber Informatics LLC | Privacy Policy