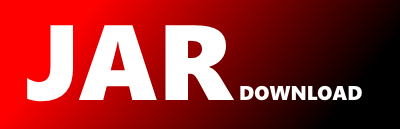
package.dist.esm.hooks.use-controllable-state.js Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of react Show documentation
Show all versions of react Show documentation
Responsive and accessible React UI components built with React and Emotion
The newest version!
"use strict";
"use client";
import { useMemo, useState } from 'react';
import { useCallbackRef } from './use-callback-ref.js';
function useControllableProp(prop, state) {
const controlled = typeof prop !== "undefined";
const value = controlled ? prop : state;
return useMemo(() => [controlled, value], [controlled, value]);
}
function useControllableState(props) {
const {
value: valueProp,
defaultValue,
onChange,
shouldUpdate = (prev, next) => prev !== next
} = props;
const onChangeProp = useCallbackRef(onChange);
const shouldUpdateProp = useCallbackRef(shouldUpdate);
const [uncontrolledState, setUncontrolledState] = useState(defaultValue);
const controlled = valueProp !== void 0;
const value = controlled ? valueProp : uncontrolledState;
const setValue = useCallbackRef(
(next) => {
const setter = next;
const nextValue = typeof next === "function" ? setter(value) : next;
if (!shouldUpdateProp(value, nextValue)) {
return;
}
if (!controlled) {
setUncontrolledState(nextValue);
}
onChangeProp(nextValue);
},
[controlled, onChangeProp, value, shouldUpdateProp]
);
return [value, setValue];
}
export { useControllableProp, useControllableState };
© 2015 - 2025 Weber Informatics LLC | Privacy Policy