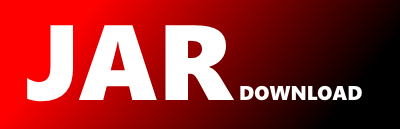
package.dist.esm.hooks.use-media-query.js Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of react Show documentation
Show all versions of react Show documentation
Responsive and accessible React UI components built with React and Emotion
The newest version!
"use strict";
"use client";
import { useState, useEffect } from 'react';
import { useCallbackRef } from './use-callback-ref.js';
function listen(query, callback) {
try {
query.addEventListener("change", callback);
return () => query.removeEventListener("change", callback);
} catch (e) {
query.addListener(callback);
return () => query.removeListener(callback);
}
}
function useMediaQuery(query, options) {
const { fallback: _fallback, ssr = true, getWindow } = options;
const getWin = useCallbackRef(getWindow);
const queries = Array.isArray(query) ? query : [query];
const fallback = _fallback?.filter((v) => v != null);
const [value, setValue] = useState(() => {
return queries.map((query2, index) => ({
media: query2,
matches: !ssr ? (getWindow?.() ?? window).matchMedia?.(query2)?.matches : !!fallback[index]
}));
});
useEffect(() => {
const win = getWin() ?? window;
setValue((prev) => {
const current = queries.map((query2) => ({
media: query2,
matches: win.matchMedia(query2).matches
}));
return prev.every(
(v, i) => v.matches === current[i].matches && v.media === current[i].media
) ? prev : current;
});
const mql = queries.map((query2) => win.matchMedia(query2));
const handler = (evt) => {
setValue((prev) => {
return prev.slice().map((item) => {
if (item.media === evt.media) return { ...item, matches: evt.matches };
return item;
});
});
};
const cleanups = mql.map((v) => listen(v, handler));
return () => cleanups.forEach((fn) => fn());
}, [getWin]);
return value.map((item) => item.matches);
}
export { useMediaQuery };
© 2015 - 2025 Weber Informatics LLC | Privacy Policy