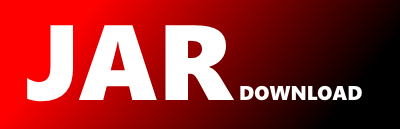
package.dist.esm.styled-system.calc.js Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of react Show documentation
Show all versions of react Show documentation
Responsive and accessible React UI components built with React and Emotion
The newest version!
"use strict";
import { isObject } from '../utils/is.js';
function resolveReference(operand) {
if (isObject(operand) && operand.reference) {
return operand.reference;
}
return String(operand);
}
const toExpression = (operator, ...operands) => operands.map(resolveReference).join(` ${operator} `).replace(/calc/g, "");
const add = (...operands) => `calc(${toExpression("+", ...operands)})`;
const subtract = (...operands) => `calc(${toExpression("-", ...operands)})`;
const multiply = (...operands) => `calc(${toExpression("*", ...operands)})`;
const divide = (...operands) => `calc(${toExpression("/", ...operands)})`;
const negate = (x) => {
const value = resolveReference(x);
if (value != null && !Number.isNaN(parseFloat(value))) {
return String(value).startsWith("-") ? String(value).slice(1) : `-${value}`;
}
return multiply(value, -1);
};
const calc = Object.assign(
(x) => ({
add: (...operands) => calc(add(x, ...operands)),
subtract: (...operands) => calc(subtract(x, ...operands)),
multiply: (...operands) => calc(multiply(x, ...operands)),
divide: (...operands) => calc(divide(x, ...operands)),
negate: () => calc(negate(x)),
toString: () => x.toString()
}),
{
add,
subtract,
multiply,
divide,
negate
}
);
export { calc };
© 2015 - 2025 Weber Informatics LLC | Privacy Policy