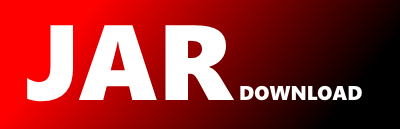
package.dist.esm.styled-system.unit-conversion.js Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of react Show documentation
Show all versions of react Show documentation
Responsive and accessible React UI components built with React and Emotion
The newest version!
"use strict";
const BASE_FONT_SIZE = 16;
const UNIT_PX = "px";
const UNIT_EM = "em";
const UNIT_REM = "rem";
function getUnit(value = "") {
const DIGIT_REGEX = new RegExp(String.raw`-?\d+(?:\.\d+|\d*)`);
const UNIT_REGEX = new RegExp(`${UNIT_PX}|${UNIT_EM}|${UNIT_REM}`);
const unit = value.match(
new RegExp(`${DIGIT_REGEX.source}(${UNIT_REGEX.source})`)
);
return unit?.[1];
}
function toPx(value = "") {
if (typeof value === "number") {
return `${value}px`;
}
const unit = getUnit(value);
if (!unit) return value;
if (unit === UNIT_PX) {
return value;
}
if (unit === UNIT_EM || unit === UNIT_REM) {
return `${parseFloat(value) * BASE_FONT_SIZE}${UNIT_PX}`;
}
}
function toEm(value = "", fontSize = BASE_FONT_SIZE) {
const unit = getUnit(value);
if (!unit) return value;
if (unit === UNIT_EM) {
return value;
}
if (unit === UNIT_PX) {
return `${parseFloat(value) / fontSize}${UNIT_EM}`;
}
if (unit === UNIT_REM) {
return `${parseFloat(value) * BASE_FONT_SIZE / fontSize}${UNIT_EM}`;
}
}
function toRem(value = "") {
const unit = getUnit(value);
if (!unit) return value;
if (unit === UNIT_REM) {
return value;
}
if (unit === UNIT_EM) {
return `${parseFloat(value)}${UNIT_REM}`;
}
if (unit === UNIT_PX) {
return `${parseFloat(value) / BASE_FONT_SIZE}${UNIT_REM}`;
}
}
export { getUnit, toEm, toPx, toRem };
© 2015 - 2025 Weber Informatics LLC | Privacy Policy