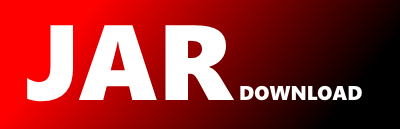
package.dist.NumberFormatter.module.js Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of number Show documentation
Show all versions of number Show documentation
Internationalized number formatting and parsing utilities
The newest version!
/*
* Copyright 2020 Adobe. All rights reserved.
* This file is licensed to you under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License. You may obtain a copy
* of the License at http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under
* the License is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR REPRESENTATIONS
* OF ANY KIND, either express or implied. See the License for the specific language
* governing permissions and limitations under the License.
*/ let $488c6ddbf4ef74c2$var$formatterCache = new Map();
let $488c6ddbf4ef74c2$var$supportsSignDisplay = false;
try {
// @ts-ignore
$488c6ddbf4ef74c2$var$supportsSignDisplay = new Intl.NumberFormat('de-DE', {
signDisplay: 'exceptZero'
}).resolvedOptions().signDisplay === 'exceptZero';
// eslint-disable-next-line no-empty
} catch (e) {}
let $488c6ddbf4ef74c2$var$supportsUnit = false;
try {
// @ts-ignore
$488c6ddbf4ef74c2$var$supportsUnit = new Intl.NumberFormat('de-DE', {
style: 'unit',
unit: 'degree'
}).resolvedOptions().style === 'unit';
// eslint-disable-next-line no-empty
} catch (e) {}
// Polyfill for units since Safari doesn't support them yet. See https://bugs.webkit.org/show_bug.cgi?id=215438.
// Currently only polyfilling the unit degree in narrow format for ColorSlider in our supported locales.
// Values were determined by switching to each locale manually in Chrome.
const $488c6ddbf4ef74c2$var$UNITS = {
degree: {
narrow: {
default: "\xb0",
'ja-JP': " \u5EA6",
'zh-TW': "\u5EA6",
'sl-SI': " \xb0"
}
}
};
class $488c6ddbf4ef74c2$export$cc77c4ff7e8673c5 {
/** Formats a number value as a string, according to the locale and options provided to the constructor. */ format(value) {
let res = '';
if (!$488c6ddbf4ef74c2$var$supportsSignDisplay && this.options.signDisplay != null) res = $488c6ddbf4ef74c2$export$711b50b3c525e0f2(this.numberFormatter, this.options.signDisplay, value);
else res = this.numberFormatter.format(value);
if (this.options.style === 'unit' && !$488c6ddbf4ef74c2$var$supportsUnit) {
var _UNITS_unit;
let { unit: unit, unitDisplay: unitDisplay = 'short', locale: locale } = this.resolvedOptions();
if (!unit) return res;
let values = (_UNITS_unit = $488c6ddbf4ef74c2$var$UNITS[unit]) === null || _UNITS_unit === void 0 ? void 0 : _UNITS_unit[unitDisplay];
res += values[locale] || values.default;
}
return res;
}
/** Formats a number to an array of parts such as separators, digits, punctuation, and more. */ formatToParts(value) {
// TODO: implement signDisplay for formatToParts
// @ts-ignore
return this.numberFormatter.formatToParts(value);
}
/** Formats a number range as a string. */ formatRange(start, end) {
// @ts-ignore
if (typeof this.numberFormatter.formatRange === 'function') // @ts-ignore
return this.numberFormatter.formatRange(start, end);
if (end < start) throw new RangeError('End date must be >= start date');
// Very basic fallback for old browsers.
return `${this.format(start)} \u{2013} ${this.format(end)}`;
}
/** Formats a number range as an array of parts. */ formatRangeToParts(start, end) {
// @ts-ignore
if (typeof this.numberFormatter.formatRangeToParts === 'function') // @ts-ignore
return this.numberFormatter.formatRangeToParts(start, end);
if (end < start) throw new RangeError('End date must be >= start date');
let startParts = this.numberFormatter.formatToParts(start);
let endParts = this.numberFormatter.formatToParts(end);
return [
...startParts.map((p)=>({
...p,
source: 'startRange'
})),
{
type: 'literal',
value: " \u2013 ",
source: 'shared'
},
...endParts.map((p)=>({
...p,
source: 'endRange'
}))
];
}
/** Returns the resolved formatting options based on the values passed to the constructor. */ resolvedOptions() {
let options = this.numberFormatter.resolvedOptions();
if (!$488c6ddbf4ef74c2$var$supportsSignDisplay && this.options.signDisplay != null) options = {
...options,
signDisplay: this.options.signDisplay
};
if (!$488c6ddbf4ef74c2$var$supportsUnit && this.options.style === 'unit') options = {
...options,
style: 'unit',
unit: this.options.unit,
unitDisplay: this.options.unitDisplay
};
return options;
}
constructor(locale, options = {}){
this.numberFormatter = $488c6ddbf4ef74c2$var$getCachedNumberFormatter(locale, options);
this.options = options;
}
}
function $488c6ddbf4ef74c2$var$getCachedNumberFormatter(locale, options = {}) {
let { numberingSystem: numberingSystem } = options;
if (numberingSystem && locale.includes('-nu-')) {
if (!locale.includes('-u-')) locale += '-u-';
locale += `-nu-${numberingSystem}`;
}
if (options.style === 'unit' && !$488c6ddbf4ef74c2$var$supportsUnit) {
var _UNITS_unit;
let { unit: unit, unitDisplay: unitDisplay = 'short' } = options;
if (!unit) throw new Error('unit option must be provided with style: "unit"');
if (!((_UNITS_unit = $488c6ddbf4ef74c2$var$UNITS[unit]) === null || _UNITS_unit === void 0 ? void 0 : _UNITS_unit[unitDisplay])) throw new Error(`Unsupported unit ${unit} with unitDisplay = ${unitDisplay}`);
options = {
...options,
style: 'decimal'
};
}
let cacheKey = locale + (options ? Object.entries(options).sort((a, b)=>a[0] < b[0] ? -1 : 1).join() : '');
if ($488c6ddbf4ef74c2$var$formatterCache.has(cacheKey)) return $488c6ddbf4ef74c2$var$formatterCache.get(cacheKey);
let numberFormatter = new Intl.NumberFormat(locale, options);
$488c6ddbf4ef74c2$var$formatterCache.set(cacheKey, numberFormatter);
return numberFormatter;
}
function $488c6ddbf4ef74c2$export$711b50b3c525e0f2(numberFormat, signDisplay, num) {
if (signDisplay === 'auto') return numberFormat.format(num);
else if (signDisplay === 'never') return numberFormat.format(Math.abs(num));
else {
let needsPositiveSign = false;
if (signDisplay === 'always') needsPositiveSign = num > 0 || Object.is(num, 0);
else if (signDisplay === 'exceptZero') {
if (Object.is(num, -0) || Object.is(num, 0)) num = Math.abs(num);
else needsPositiveSign = num > 0;
}
if (needsPositiveSign) {
let negative = numberFormat.format(-num);
let noSign = numberFormat.format(num);
// ignore RTL/LTR marker character
let minus = negative.replace(noSign, '').replace(/\u200e|\u061C/, '');
if ([
...minus
].length !== 1) console.warn('@react-aria/i18n polyfill for NumberFormat signDisplay: Unsupported case');
let positive = negative.replace(noSign, '!!!').replace(minus, '+').replace('!!!', noSign);
return positive;
} else return numberFormat.format(num);
}
}
export {$488c6ddbf4ef74c2$export$cc77c4ff7e8673c5 as NumberFormatter, $488c6ddbf4ef74c2$export$711b50b3c525e0f2 as numberFormatSignDisplayPolyfill};
//# sourceMappingURL=NumberFormatter.module.js.map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy