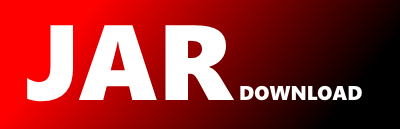
package.dist.vscode-form-container.vscode-form-container.js Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of elements Show documentation
Show all versions of elements Show documentation
Webcomponents for creating Visual Studio Code extensions
The newest version!
var __decorate = (this && this.__decorate) || function (decorators, target, key, desc) {
var c = arguments.length, r = c < 3 ? target : desc === null ? desc = Object.getOwnPropertyDescriptor(target, key) : desc, d;
if (typeof Reflect === "object" && typeof Reflect.decorate === "function") r = Reflect.decorate(decorators, target, key, desc);
else for (var i = decorators.length - 1; i >= 0; i--) if (d = decorators[i]) r = (c < 3 ? d(r) : c > 3 ? d(target, key, r) : d(target, key)) || r;
return c > 3 && r && Object.defineProperty(target, key, r), r;
};
import { html } from 'lit';
import { customElement, property, query, queryAssignedElements, } from 'lit/decorators.js';
import { VscElement } from '../includes/VscElement.js';
import styles from './vscode-form-container.styles.js';
var FormGroupLayout;
(function (FormGroupLayout) {
FormGroupLayout["HORIZONTAL"] = "horizontal";
FormGroupLayout["VERTICAL"] = "vertical";
})(FormGroupLayout || (FormGroupLayout = {}));
const isTextInput = (el) => {
return ['vscode-textfield', 'vscode-textarea'].includes(el.tagName.toLocaleLowerCase());
};
const isSingleSelect = (el) => {
return el.tagName.toLocaleLowerCase() === 'vscode-single-select';
};
const isMultiSelect = (el) => {
return el.tagName.toLocaleLowerCase() === 'vscode-multi-select';
};
const isCheckbox = (el) => {
return el.tagName.toLocaleLowerCase() === 'vscode-checkbox';
};
const isRadio = (el) => {
return el.tagName.toLocaleLowerCase() === 'vscode-radio';
};
let VscodeFormContainer = class VscodeFormContainer extends VscElement {
constructor() {
super(...arguments);
this.breakpoint = 490;
this._responsive = false;
this._firstUpdateComplete = false;
this._resizeObserverCallbackBound = this._resizeObserverCallback.bind(this);
}
set responsive(isResponsive) {
this._responsive = isResponsive;
if (this._firstUpdateComplete) {
if (isResponsive) {
this._activateResponsiveLayout();
}
else {
this._deactivateResizeObserver();
}
}
}
get responsive() {
return this._responsive;
}
/** @deprecated - Use the native `
© 2015 - 2025 Weber Informatics LLC | Privacy Policy