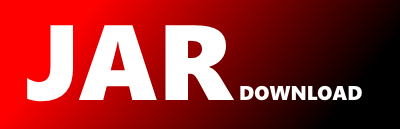
package.dist.index.js Maven / Gradle / Ivy
'use strict';
var domQuery = require('@zag-js/dom-query');
// src/index.ts
var LOCK_CLASSNAME = "data-scroll-lock";
function assignStyle(el, style) {
if (!el) return;
const previousStyle = Object.keys(style).reduce(
(acc, key) => {
acc[key] = el.style.getPropertyValue(key);
return acc;
},
{}
);
Object.assign(el.style, style);
return () => {
Object.assign(el.style, previousStyle);
};
}
function setCSSProperty(el, property, value) {
if (!el) return;
const previousValue = el.style.getPropertyValue(property);
el.style.setProperty(property, value);
return () => {
if (previousValue) {
el.style.setProperty(property, previousValue);
} else {
el.style.removeProperty(property);
}
};
}
function getPaddingProperty(documentElement) {
const documentLeft = documentElement.getBoundingClientRect().left;
const scrollbarX = Math.round(documentLeft) + documentElement.scrollLeft;
return scrollbarX ? "paddingLeft" : "paddingRight";
}
function preventBodyScroll(_document) {
const doc = _document ?? document;
const win = doc.defaultView ?? window;
const { documentElement, body } = doc;
const locked = body.hasAttribute(LOCK_CLASSNAME);
if (locked) return;
body.setAttribute(LOCK_CLASSNAME, "");
const scrollbarWidth = win.innerWidth - documentElement.clientWidth;
const setScrollbarWidthProperty = () => setCSSProperty(documentElement, "--scrollbar-width", `${scrollbarWidth}px`);
const paddingProperty = getPaddingProperty(documentElement);
const setStyle = () => assignStyle(body, {
overflow: "hidden",
[paddingProperty]: `${scrollbarWidth}px`
});
const setIOSStyle = () => {
const { scrollX, scrollY, visualViewport } = win;
const offsetLeft = visualViewport?.offsetLeft ?? 0;
const offsetTop = visualViewport?.offsetTop ?? 0;
const restoreStyle = assignStyle(body, {
position: "fixed",
overflow: "hidden",
top: `${-(scrollY - Math.floor(offsetTop))}px`,
left: `${-(scrollX - Math.floor(offsetLeft))}px`,
right: "0",
[paddingProperty]: `${scrollbarWidth}px`
});
return () => {
restoreStyle?.();
win.scrollTo({ left: scrollX, top: scrollY, behavior: "instant" });
};
};
const cleanups = [setScrollbarWidthProperty(), domQuery.isIos() ? setIOSStyle() : setStyle()];
return () => {
cleanups.forEach((fn) => fn?.());
body.removeAttribute(LOCK_CLASSNAME);
};
}
exports.preventBodyScroll = preventBodyScroll;
© 2015 - 2025 Weber Informatics LLC | Privacy Policy