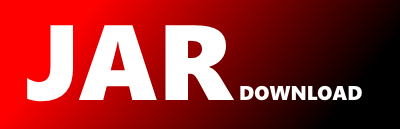
package.ssr.cache.js Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mikado Show documentation
Show all versions of mikado Show documentation
Web's fastest template library to build user interfaces.
The newest version!
function Cache1(){
this.map = new Map();
}
Cache1.prototype.add = function(key, value){
this.map.set(key, value);
if(this.map.size > 100) this.map.delete(this.map.keys().next().value)
}
Cache1.prototype.get = function(key){
return this.map.get(key);
}
function Item(key, value, prev){
this.key = key;
this.value = value;
this.prev = prev || null;
this.next = null;
}
function Cache2(){
this.map = Object.create(null);
this.first = null;
this.last = null;
this.length = 0;
}
Cache2.prototype.add = function(key, value){
if(this.map[key]){
this.map[key] = value;
}
else{
let tmp;
this.map[key] = tmp = new Item(key, value, this.last);
this.first || (this.first = tmp);
this.last && (this.last.next = tmp);
this.last = tmp;
if(this.length >= 100){
this.map[this.first.key] = null;
this.first = this.first.next;
}
else{
this.length++;
}
}
}
Cache2.prototype.get = function(key){
return this.map[key];
}
const cache_1 = new Cache1();
const cache_2 = new Cache2();
console.time("1");
for(let i = 0; i < 4999999; i++) {
cache_1.add("id-"+i, "test " + (i % 50));
cache_1.get("id-"+i);
}
console.timeEnd("1");
console.time("2");
for(let i = 0; i < 4999999; i++) {
cache_2.add("id-"+i, "test " + (i % 50));
cache_2.get("id-"+i);
}
console.timeEnd("2");
© 2015 - 2025 Weber Informatics LLC | Privacy Policy