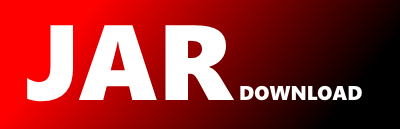
package.task.build_new.js Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mikado Show documentation
Show all versions of mikado Show documentation
Web's fastest template library to build user interfaces.
The newest version!
const child_process = require('child_process');
const { version } = require("../package.json");
const fs = require('fs');
console.log("Start build .....");
console.log();
fs.existsSync("log") || fs.mkdirSync("log");
fs.existsSync("tmp") || fs.mkdirSync("tmp");
fs.existsSync("dist") || fs.mkdirSync("dist");
let flag_str = "";
let language_out;
let use_polyfill;
var options = (function(argv){
const arr = {};
let count = 0;
argv.forEach(function(val, index) {
if(++count > 2){
index = val.split('=');
val = index[1];
index = index[0].toUpperCase();
if(index === "LANGUAGE_OUT"){
language_out = val;
}
/*
else if(index === "USE_POLYFILL"){
use_polyfill = val;
}
*/
else{
flag_str += " --define='" + index + "=" + val + "'";
arr[index] = val;
}
if(count > 3) console.log(index + ': ' + val);
}
});
console.log('RELEASE: ' + (arr['RELEASE'] || 'custom'));
return arr;
})(process.argv);
const light_version = (options["RELEASE"] === "light") || (process.argv[2] === "--light");
const es5_version = (options["RELEASE"] === "es5") || (process.argv[2] === "--es5");
const module_version = (options["RELEASE"] === "module") || (process.argv[2] === "--module");
const parameter = (function(opt){
let parameter = '';
for(let index in opt){
if(opt.hasOwnProperty(index)){
parameter += ' --' + index + '=' + opt[index];
}
}
return parameter;
})({
compilation_level: "ADVANCED_OPTIMIZATIONS", // "SIMPLE" // "WHITESPACE"
use_types_for_optimization: true,
//new_type_inf: true,
//jscomp_warning: "newCheckTypes",
//jscomp_error: "strictCheckTypes",
//jscomp_error: "newCheckTypesExtraChecks",
generate_exports: true,
export_local_property_definitions: true,
language_in: "ECMASCRIPT_2017",
language_out: "STABLE",
process_closure_primitives: true,
summary_detail_level: 3,
warning_level: "VERBOSE",
emit_use_strict: true,
output_manifest: "log/manifest.log",
//output_module_dependencies: "log/module_dependencies.log",
property_renaming_report: "log/renaming_report.log",
//transform_amd_modules: true,
process_common_js_modules: true,
js_module_root: "node_modules/",
module_resolution: "BROWSER",
dependency_mode: "PRUNE",
entry_point: "./src/bundle.js",
rewrite_polyfills: false,
isolation_mode: "IIFE"
//rename_prefix_namespace: true
//output_wrapper: "(function(){%output%}).call(this);",
//define: "'ENABLE_CLASS_CACHE=true'"
//formatting: "PRETTY_PRINT"
});
const executable = process.platform === "win32" ? "\"node_modules/google-closure-compiler-windows/compiler.exe\"" :
process.platform === "darwin" ? "\"node_modules/google-closure-compiler-osx/compiler\"" :
"java -jar node_modules/google-closure-compiler-java/compiler.jar"
console.log(parameter)
exec(executable + parameter + " --define='DEBUG=false' --define='SUPPORT_CACHE_HELPERS=false' --externs='src/goog.js' --js='src/**.js' " + flag_str + " --js_output_file='dist/mikado-new.min.js' && exit 0", function(){
//exec("java -jar node_modules/google-closure-compiler-java/compiler.jar" + parameter + " --js='src/js/**.js' --js='!src/js/lib/**.js' --js='!src/js/*build.js' --js_output_file='public/js/build.js' && exit 0", function(){
//let build = fs.readFileSync("public/js/build.js", "utf8");
//build += fs.readFileSync("src/lib/litepicker.js");
//build = build.replace("${VERSION_JS}", version);
//fs.writeFileSync("public/js/build.js", build);
console.log("Build Complete.");
});
function exec(prompt, callback){
const child = child_process.exec(prompt, function(err, stdout, stderr){
if(err){
console.error(err);
}
else{
if(callback){
callback();
}
}
});
child.stdout.pipe(process.stdout);
child.stderr.pipe(process.stderr);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy