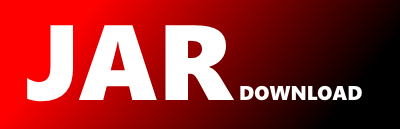
package.dist.development.index.js Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of react-router Show documentation
Show all versions of react-router Show documentation
Declarative routing for React
/**
* react-router v7.0.2
*
* Copyright (c) Remix Software Inc.
*
* This source code is licensed under the MIT license found in the
* LICENSE.md file in the root directory of this source tree.
*
* @license MIT
*/
"use strict";
var __create = Object.create;
var __defProp = Object.defineProperty;
var __getOwnPropDesc = Object.getOwnPropertyDescriptor;
var __getOwnPropNames = Object.getOwnPropertyNames;
var __getProtoOf = Object.getPrototypeOf;
var __hasOwnProp = Object.prototype.hasOwnProperty;
var __export = (target, all) => {
for (var name in all)
__defProp(target, name, { get: all[name], enumerable: true });
};
var __copyProps = (to, from, except, desc) => {
if (from && typeof from === "object" || typeof from === "function") {
for (let key of __getOwnPropNames(from))
if (!__hasOwnProp.call(to, key) && key !== except)
__defProp(to, key, { get: () => from[key], enumerable: !(desc = __getOwnPropDesc(from, key)) || desc.enumerable });
}
return to;
};
var __toESM = (mod, isNodeMode, target) => (target = mod != null ? __create(__getProtoOf(mod)) : {}, __copyProps(
// If the importer is in node compatibility mode or this is not an ESM
// file that has been converted to a CommonJS file using a Babel-
// compatible transform (i.e. "__esModule" has not been set), then set
// "default" to the CommonJS "module.exports" for node compatibility.
isNodeMode || !mod || !mod.__esModule ? __defProp(target, "default", { value: mod, enumerable: true }) : target,
mod
));
var __toCommonJS = (mod) => __copyProps(__defProp({}, "__esModule", { value: true }), mod);
// index.ts
var react_router_exports = {};
__export(react_router_exports, {
Await: () => Await,
BrowserRouter: () => BrowserRouter,
Form: () => Form,
HashRouter: () => HashRouter,
IDLE_BLOCKER: () => IDLE_BLOCKER,
IDLE_FETCHER: () => IDLE_FETCHER,
IDLE_NAVIGATION: () => IDLE_NAVIGATION,
Link: () => Link,
Links: () => Links,
MemoryRouter: () => MemoryRouter,
Meta: () => Meta,
NavLink: () => NavLink,
Navigate: () => Navigate,
NavigationType: () => Action,
Outlet: () => Outlet,
PrefetchPageLinks: () => PrefetchPageLinks,
Route: () => Route,
Router: () => Router,
RouterProvider: () => RouterProvider,
Routes: () => Routes,
Scripts: () => Scripts,
ScrollRestoration: () => ScrollRestoration,
ServerRouter: () => ServerRouter,
StaticRouter: () => StaticRouter,
StaticRouterProvider: () => StaticRouterProvider,
UNSAFE_DataRouterContext: () => DataRouterContext,
UNSAFE_DataRouterStateContext: () => DataRouterStateContext,
UNSAFE_ErrorResponseImpl: () => ErrorResponseImpl,
UNSAFE_FetchersContext: () => FetchersContext,
UNSAFE_FrameworkContext: () => FrameworkContext,
UNSAFE_LocationContext: () => LocationContext,
UNSAFE_NavigationContext: () => NavigationContext,
UNSAFE_RemixErrorBoundary: () => RemixErrorBoundary,
UNSAFE_RouteContext: () => RouteContext,
UNSAFE_ServerMode: () => ServerMode,
UNSAFE_SingleFetchRedirectSymbol: () => SingleFetchRedirectSymbol,
UNSAFE_ViewTransitionContext: () => ViewTransitionContext,
UNSAFE_createBrowserHistory: () => createBrowserHistory,
UNSAFE_createClientRoutes: () => createClientRoutes,
UNSAFE_createClientRoutesWithHMRRevalidationOptOut: () => createClientRoutesWithHMRRevalidationOptOut,
UNSAFE_createRouter: () => createRouter,
UNSAFE_decodeViaTurboStream: () => decodeViaTurboStream,
UNSAFE_deserializeErrors: () => deserializeErrors2,
UNSAFE_getPatchRoutesOnNavigationFunction: () => getPatchRoutesOnNavigationFunction,
UNSAFE_getSingleFetchDataStrategy: () => getSingleFetchDataStrategy,
UNSAFE_invariant: () => invariant,
UNSAFE_mapRouteProperties: () => mapRouteProperties,
UNSAFE_shouldHydrateRouteLoader: () => shouldHydrateRouteLoader,
UNSAFE_useFogOFWarDiscovery: () => useFogOFWarDiscovery,
UNSAFE_useScrollRestoration: () => useScrollRestoration,
createBrowserRouter: () => createBrowserRouter,
createCookie: () => createCookie,
createCookieSessionStorage: () => createCookieSessionStorage,
createHashRouter: () => createHashRouter,
createMemoryRouter: () => createMemoryRouter,
createMemorySessionStorage: () => createMemorySessionStorage,
createPath: () => createPath,
createRequestHandler: () => createRequestHandler,
createRoutesFromChildren: () => createRoutesFromChildren,
createRoutesFromElements: () => createRoutesFromElements,
createRoutesStub: () => createRoutesStub,
createSearchParams: () => createSearchParams,
createSession: () => createSession,
createSessionStorage: () => createSessionStorage,
createStaticHandler: () => createStaticHandler2,
createStaticRouter: () => createStaticRouter,
data: () => data,
generatePath: () => generatePath,
isCookie: () => isCookie,
isRouteErrorResponse: () => isRouteErrorResponse,
isSession: () => isSession,
matchPath: () => matchPath,
matchRoutes: () => matchRoutes,
parsePath: () => parsePath,
redirect: () => redirect,
redirectDocument: () => redirectDocument,
renderMatches: () => renderMatches,
replace: () => replace,
resolvePath: () => resolvePath,
unstable_HistoryRouter: () => HistoryRouter,
unstable_setDevServerHooks: () => setDevServerHooks,
unstable_usePrompt: () => usePrompt,
useActionData: () => useActionData,
useAsyncError: () => useAsyncError,
useAsyncValue: () => useAsyncValue,
useBeforeUnload: () => useBeforeUnload,
useBlocker: () => useBlocker,
useFetcher: () => useFetcher,
useFetchers: () => useFetchers,
useFormAction: () => useFormAction,
useHref: () => useHref,
useInRouterContext: () => useInRouterContext,
useLinkClickHandler: () => useLinkClickHandler,
useLoaderData: () => useLoaderData,
useLocation: () => useLocation,
useMatch: () => useMatch,
useMatches: () => useMatches,
useNavigate: () => useNavigate,
useNavigation: () => useNavigation,
useNavigationType: () => useNavigationType,
useOutlet: () => useOutlet,
useOutletContext: () => useOutletContext,
useParams: () => useParams,
useResolvedPath: () => useResolvedPath,
useRevalidator: () => useRevalidator,
useRouteError: () => useRouteError,
useRouteLoaderData: () => useRouteLoaderData,
useRoutes: () => useRoutes,
useSearchParams: () => useSearchParams,
useSubmit: () => useSubmit,
useViewTransitionState: () => useViewTransitionState
});
module.exports = __toCommonJS(react_router_exports);
// lib/router/history.ts
var Action = /* @__PURE__ */ ((Action2) => {
Action2["Pop"] = "POP";
Action2["Push"] = "PUSH";
Action2["Replace"] = "REPLACE";
return Action2;
})(Action || {});
var PopStateEventType = "popstate";
function createMemoryHistory(options = {}) {
let { initialEntries = ["/"], initialIndex, v5Compat = false } = options;
let entries;
entries = initialEntries.map(
(entry, index2) => createMemoryLocation(
entry,
typeof entry === "string" ? null : entry.state,
index2 === 0 ? "default" : void 0
)
);
let index = clampIndex(
initialIndex == null ? entries.length - 1 : initialIndex
);
let action = "POP" /* Pop */;
let listener = null;
function clampIndex(n) {
return Math.min(Math.max(n, 0), entries.length - 1);
}
function getCurrentLocation() {
return entries[index];
}
function createMemoryLocation(to, state = null, key) {
let location = createLocation(
entries ? getCurrentLocation().pathname : "/",
to,
state,
key
);
warning(
location.pathname.charAt(0) === "/",
`relative pathnames are not supported in memory history: ${JSON.stringify(
to
)}`
);
return location;
}
function createHref2(to) {
return typeof to === "string" ? to : createPath(to);
}
let history = {
get index() {
return index;
},
get action() {
return action;
},
get location() {
return getCurrentLocation();
},
createHref: createHref2,
createURL(to) {
return new URL(createHref2(to), "http://localhost");
},
encodeLocation(to) {
let path = typeof to === "string" ? parsePath(to) : to;
return {
pathname: path.pathname || "",
search: path.search || "",
hash: path.hash || ""
};
},
push(to, state) {
action = "PUSH" /* Push */;
let nextLocation = createMemoryLocation(to, state);
index += 1;
entries.splice(index, entries.length, nextLocation);
if (v5Compat && listener) {
listener({ action, location: nextLocation, delta: 1 });
}
},
replace(to, state) {
action = "REPLACE" /* Replace */;
let nextLocation = createMemoryLocation(to, state);
entries[index] = nextLocation;
if (v5Compat && listener) {
listener({ action, location: nextLocation, delta: 0 });
}
},
go(delta) {
action = "POP" /* Pop */;
let nextIndex = clampIndex(index + delta);
let nextLocation = entries[nextIndex];
index = nextIndex;
if (listener) {
listener({ action, location: nextLocation, delta });
}
},
listen(fn) {
listener = fn;
return () => {
listener = null;
};
}
};
return history;
}
function createBrowserHistory(options = {}) {
function createBrowserLocation(window2, globalHistory) {
let { pathname, search, hash } = window2.location;
return createLocation(
"",
{ pathname, search, hash },
// state defaults to `null` because `window.history.state` does
globalHistory.state && globalHistory.state.usr || null,
globalHistory.state && globalHistory.state.key || "default"
);
}
function createBrowserHref(window2, to) {
return typeof to === "string" ? to : createPath(to);
}
return getUrlBasedHistory(
createBrowserLocation,
createBrowserHref,
null,
options
);
}
function createHashHistory(options = {}) {
function createHashLocation(window2, globalHistory) {
let {
pathname = "/",
search = "",
hash = ""
} = parsePath(window2.location.hash.substring(1));
if (!pathname.startsWith("/") && !pathname.startsWith(".")) {
pathname = "/" + pathname;
}
return createLocation(
"",
{ pathname, search, hash },
// state defaults to `null` because `window.history.state` does
globalHistory.state && globalHistory.state.usr || null,
globalHistory.state && globalHistory.state.key || "default"
);
}
function createHashHref(window2, to) {
let base = window2.document.querySelector("base");
let href = "";
if (base && base.getAttribute("href")) {
let url = window2.location.href;
let hashIndex = url.indexOf("#");
href = hashIndex === -1 ? url : url.slice(0, hashIndex);
}
return href + "#" + (typeof to === "string" ? to : createPath(to));
}
function validateHashLocation(location, to) {
warning(
location.pathname.charAt(0) === "/",
`relative pathnames are not supported in hash history.push(${JSON.stringify(
to
)})`
);
}
return getUrlBasedHistory(
createHashLocation,
createHashHref,
validateHashLocation,
options
);
}
function invariant(value, message) {
if (value === false || value === null || typeof value === "undefined") {
throw new Error(message);
}
}
function warning(cond, message) {
if (!cond) {
if (typeof console !== "undefined") console.warn(message);
try {
throw new Error(message);
} catch (e) {
}
}
}
function createKey() {
return Math.random().toString(36).substring(2, 10);
}
function getHistoryState(location, index) {
return {
usr: location.state,
key: location.key,
idx: index
};
}
function createLocation(current, to, state = null, key) {
let location = {
pathname: typeof current === "string" ? current : current.pathname,
search: "",
hash: "",
...typeof to === "string" ? parsePath(to) : to,
state,
// TODO: This could be cleaned up. push/replace should probably just take
// full Locations now and avoid the need to run through this flow at all
// But that's a pretty big refactor to the current test suite so going to
// keep as is for the time being and just let any incoming keys take precedence
key: to && to.key || key || createKey()
};
return location;
}
function createPath({
pathname = "/",
search = "",
hash = ""
}) {
if (search && search !== "?")
pathname += search.charAt(0) === "?" ? search : "?" + search;
if (hash && hash !== "#")
pathname += hash.charAt(0) === "#" ? hash : "#" + hash;
return pathname;
}
function parsePath(path) {
let parsedPath = {};
if (path) {
let hashIndex = path.indexOf("#");
if (hashIndex >= 0) {
parsedPath.hash = path.substring(hashIndex);
path = path.substring(0, hashIndex);
}
let searchIndex = path.indexOf("?");
if (searchIndex >= 0) {
parsedPath.search = path.substring(searchIndex);
path = path.substring(0, searchIndex);
}
if (path) {
parsedPath.pathname = path;
}
}
return parsedPath;
}
function getUrlBasedHistory(getLocation, createHref2, validateLocation, options = {}) {
let { window: window2 = document.defaultView, v5Compat = false } = options;
let globalHistory = window2.history;
let action = "POP" /* Pop */;
let listener = null;
let index = getIndex();
if (index == null) {
index = 0;
globalHistory.replaceState({ ...globalHistory.state, idx: index }, "");
}
function getIndex() {
let state = globalHistory.state || { idx: null };
return state.idx;
}
function handlePop() {
action = "POP" /* Pop */;
let nextIndex = getIndex();
let delta = nextIndex == null ? null : nextIndex - index;
index = nextIndex;
if (listener) {
listener({ action, location: history.location, delta });
}
}
function push(to, state) {
action = "PUSH" /* Push */;
let location = createLocation(history.location, to, state);
if (validateLocation) validateLocation(location, to);
index = getIndex() + 1;
let historyState = getHistoryState(location, index);
let url = history.createHref(location);
try {
globalHistory.pushState(historyState, "", url);
} catch (error) {
if (error instanceof DOMException && error.name === "DataCloneError") {
throw error;
}
window2.location.assign(url);
}
if (v5Compat && listener) {
listener({ action, location: history.location, delta: 1 });
}
}
function replace2(to, state) {
action = "REPLACE" /* Replace */;
let location = createLocation(history.location, to, state);
if (validateLocation) validateLocation(location, to);
index = getIndex();
let historyState = getHistoryState(location, index);
let url = history.createHref(location);
globalHistory.replaceState(historyState, "", url);
if (v5Compat && listener) {
listener({ action, location: history.location, delta: 0 });
}
}
function createURL(to) {
let base = window2.location.origin !== "null" ? window2.location.origin : window2.location.href;
let href = typeof to === "string" ? to : createPath(to);
href = href.replace(/ $/, "%20");
invariant(
base,
`No window.location.(origin|href) available to create URL for href: ${href}`
);
return new URL(href, base);
}
let history = {
get action() {
return action;
},
get location() {
return getLocation(window2, globalHistory);
},
listen(fn) {
if (listener) {
throw new Error("A history only accepts one active listener");
}
window2.addEventListener(PopStateEventType, handlePop);
listener = fn;
return () => {
window2.removeEventListener(PopStateEventType, handlePop);
listener = null;
};
},
createHref(to) {
return createHref2(window2, to);
},
createURL,
encodeLocation(to) {
let url = createURL(to);
return {
pathname: url.pathname,
search: url.search,
hash: url.hash
};
},
push,
replace: replace2,
go(n) {
return globalHistory.go(n);
}
};
return history;
}
// lib/router/utils.ts
var immutableRouteKeys = /* @__PURE__ */ new Set([
"lazy",
"caseSensitive",
"path",
"id",
"index",
"children"
]);
function isIndexRoute(route) {
return route.index === true;
}
function convertRoutesToDataRoutes(routes, mapRouteProperties2, parentPath = [], manifest = {}) {
return routes.map((route, index) => {
let treePath = [...parentPath, String(index)];
let id = typeof route.id === "string" ? route.id : treePath.join("-");
invariant(
route.index !== true || !route.children,
`Cannot specify children on an index route`
);
invariant(
!manifest[id],
`Found a route id collision on id "${id}". Route id's must be globally unique within Data Router usages`
);
if (isIndexRoute(route)) {
let indexRoute = {
...route,
...mapRouteProperties2(route),
id
};
manifest[id] = indexRoute;
return indexRoute;
} else {
let pathOrLayoutRoute = {
...route,
...mapRouteProperties2(route),
id,
children: void 0
};
manifest[id] = pathOrLayoutRoute;
if (route.children) {
pathOrLayoutRoute.children = convertRoutesToDataRoutes(
route.children,
mapRouteProperties2,
treePath,
manifest
);
}
return pathOrLayoutRoute;
}
});
}
function matchRoutes(routes, locationArg, basename = "/") {
return matchRoutesImpl(routes, locationArg, basename, false);
}
function matchRoutesImpl(routes, locationArg, basename, allowPartial) {
let location = typeof locationArg === "string" ? parsePath(locationArg) : locationArg;
let pathname = stripBasename(location.pathname || "/", basename);
if (pathname == null) {
return null;
}
let branches = flattenRoutes(routes);
rankRouteBranches(branches);
let matches = null;
for (let i = 0; matches == null && i < branches.length; ++i) {
let decoded = decodePath(pathname);
matches = matchRouteBranch(
branches[i],
decoded,
allowPartial
);
}
return matches;
}
function convertRouteMatchToUiMatch(match, loaderData) {
let { route, pathname, params } = match;
return {
id: route.id,
pathname,
params,
data: loaderData[route.id],
handle: route.handle
};
}
function flattenRoutes(routes, branches = [], parentsMeta = [], parentPath = "") {
let flattenRoute = (route, index, relativePath) => {
let meta = {
relativePath: relativePath === void 0 ? route.path || "" : relativePath,
caseSensitive: route.caseSensitive === true,
childrenIndex: index,
route
};
if (meta.relativePath.startsWith("/")) {
invariant(
meta.relativePath.startsWith(parentPath),
`Absolute route path "${meta.relativePath}" nested under path "${parentPath}" is not valid. An absolute child route path must start with the combined path of all its parent routes.`
);
meta.relativePath = meta.relativePath.slice(parentPath.length);
}
let path = joinPaths([parentPath, meta.relativePath]);
let routesMeta = parentsMeta.concat(meta);
if (route.children && route.children.length > 0) {
invariant(
// Our types know better, but runtime JS may not!
// @ts-expect-error
route.index !== true,
`Index routes must not have child routes. Please remove all child routes from route path "${path}".`
);
flattenRoutes(route.children, branches, routesMeta, path);
}
if (route.path == null && !route.index) {
return;
}
branches.push({
path,
score: computeScore(path, route.index),
routesMeta
});
};
routes.forEach((route, index) => {
if (route.path === "" || !route.path?.includes("?")) {
flattenRoute(route, index);
} else {
for (let exploded of explodeOptionalSegments(route.path)) {
flattenRoute(route, index, exploded);
}
}
});
return branches;
}
function explodeOptionalSegments(path) {
let segments = path.split("/");
if (segments.length === 0) return [];
let [first, ...rest] = segments;
let isOptional = first.endsWith("?");
let required = first.replace(/\?$/, "");
if (rest.length === 0) {
return isOptional ? [required, ""] : [required];
}
let restExploded = explodeOptionalSegments(rest.join("/"));
let result = [];
result.push(
...restExploded.map(
(subpath) => subpath === "" ? required : [required, subpath].join("/")
)
);
if (isOptional) {
result.push(...restExploded);
}
return result.map(
(exploded) => path.startsWith("/") && exploded === "" ? "/" : exploded
);
}
function rankRouteBranches(branches) {
branches.sort(
(a, b) => a.score !== b.score ? b.score - a.score : compareIndexes(
a.routesMeta.map((meta) => meta.childrenIndex),
b.routesMeta.map((meta) => meta.childrenIndex)
)
);
}
var paramRe = /^:[\w-]+$/;
var dynamicSegmentValue = 3;
var indexRouteValue = 2;
var emptySegmentValue = 1;
var staticSegmentValue = 10;
var splatPenalty = -2;
var isSplat = (s) => s === "*";
function computeScore(path, index) {
let segments = path.split("/");
let initialScore = segments.length;
if (segments.some(isSplat)) {
initialScore += splatPenalty;
}
if (index) {
initialScore += indexRouteValue;
}
return segments.filter((s) => !isSplat(s)).reduce(
(score, segment) => score + (paramRe.test(segment) ? dynamicSegmentValue : segment === "" ? emptySegmentValue : staticSegmentValue),
initialScore
);
}
function compareIndexes(a, b) {
let siblings = a.length === b.length && a.slice(0, -1).every((n, i) => n === b[i]);
return siblings ? (
// If two routes are siblings, we should try to match the earlier sibling
// first. This allows people to have fine-grained control over the matching
// behavior by simply putting routes with identical paths in the order they
// want them tried.
a[a.length - 1] - b[b.length - 1]
) : (
// Otherwise, it doesn't really make sense to rank non-siblings by index,
// so they sort equally.
0
);
}
function matchRouteBranch(branch, pathname, allowPartial = false) {
let { routesMeta } = branch;
let matchedParams = {};
let matchedPathname = "/";
let matches = [];
for (let i = 0; i < routesMeta.length; ++i) {
let meta = routesMeta[i];
let end = i === routesMeta.length - 1;
let remainingPathname = matchedPathname === "/" ? pathname : pathname.slice(matchedPathname.length) || "/";
let match = matchPath(
{ path: meta.relativePath, caseSensitive: meta.caseSensitive, end },
remainingPathname
);
let route = meta.route;
if (!match && end && allowPartial && !routesMeta[routesMeta.length - 1].route.index) {
match = matchPath(
{
path: meta.relativePath,
caseSensitive: meta.caseSensitive,
end: false
},
remainingPathname
);
}
if (!match) {
return null;
}
Object.assign(matchedParams, match.params);
matches.push({
// TODO: Can this as be avoided?
params: matchedParams,
pathname: joinPaths([matchedPathname, match.pathname]),
pathnameBase: normalizePathname(
joinPaths([matchedPathname, match.pathnameBase])
),
route
});
if (match.pathnameBase !== "/") {
matchedPathname = joinPaths([matchedPathname, match.pathnameBase]);
}
}
return matches;
}
function generatePath(originalPath, params = {}) {
let path = originalPath;
if (path.endsWith("*") && path !== "*" && !path.endsWith("/*")) {
warning(
false,
`Route path "${path}" will be treated as if it were "${path.replace(/\*$/, "/*")}" because the \`*\` character must always follow a \`/\` in the pattern. To get rid of this warning, please change the route path to "${path.replace(/\*$/, "/*")}".`
);
path = path.replace(/\*$/, "/*");
}
const prefix = path.startsWith("/") ? "/" : "";
const stringify = (p) => p == null ? "" : typeof p === "string" ? p : String(p);
const segments = path.split(/\/+/).map((segment, index, array) => {
const isLastSegment = index === array.length - 1;
if (isLastSegment && segment === "*") {
const star = "*";
return stringify(params[star]);
}
const keyMatch = segment.match(/^:([\w-]+)(\??)$/);
if (keyMatch) {
const [, key, optional] = keyMatch;
let param = params[key];
invariant(optional === "?" || param != null, `Missing ":${key}" param`);
return stringify(param);
}
return segment.replace(/\?$/g, "");
}).filter((segment) => !!segment);
return prefix + segments.join("/");
}
function matchPath(pattern, pathname) {
if (typeof pattern === "string") {
pattern = { path: pattern, caseSensitive: false, end: true };
}
let [matcher, compiledParams] = compilePath(
pattern.path,
pattern.caseSensitive,
pattern.end
);
let match = pathname.match(matcher);
if (!match) return null;
let matchedPathname = match[0];
let pathnameBase = matchedPathname.replace(/(.)\/+$/, "$1");
let captureGroups = match.slice(1);
let params = compiledParams.reduce(
(memo2, { paramName, isOptional }, index) => {
if (paramName === "*") {
let splatValue = captureGroups[index] || "";
pathnameBase = matchedPathname.slice(0, matchedPathname.length - splatValue.length).replace(/(.)\/+$/, "$1");
}
const value = captureGroups[index];
if (isOptional && !value) {
memo2[paramName] = void 0;
} else {
memo2[paramName] = (value || "").replace(/%2F/g, "/");
}
return memo2;
},
{}
);
return {
params,
pathname: matchedPathname,
pathnameBase,
pattern
};
}
function compilePath(path, caseSensitive = false, end = true) {
warning(
path === "*" || !path.endsWith("*") || path.endsWith("/*"),
`Route path "${path}" will be treated as if it were "${path.replace(/\*$/, "/*")}" because the \`*\` character must always follow a \`/\` in the pattern. To get rid of this warning, please change the route path to "${path.replace(/\*$/, "/*")}".`
);
let params = [];
let regexpSource = "^" + path.replace(/\/*\*?$/, "").replace(/^\/*/, "/").replace(/[\\.*+^${}|()[\]]/g, "\\$&").replace(
/\/:([\w-]+)(\?)?/g,
(_, paramName, isOptional) => {
params.push({ paramName, isOptional: isOptional != null });
return isOptional ? "/?([^\\/]+)?" : "/([^\\/]+)";
}
);
if (path.endsWith("*")) {
params.push({ paramName: "*" });
regexpSource += path === "*" || path === "/*" ? "(.*)$" : "(?:\\/(.+)|\\/*)$";
} else if (end) {
regexpSource += "\\/*$";
} else if (path !== "" && path !== "/") {
regexpSource += "(?:(?=\\/|$))";
} else {
}
let matcher = new RegExp(regexpSource, caseSensitive ? void 0 : "i");
return [matcher, params];
}
function decodePath(value) {
try {
return value.split("/").map((v) => decodeURIComponent(v).replace(/\//g, "%2F")).join("/");
} catch (error) {
warning(
false,
`The URL path "${value}" could not be decoded because it is is a malformed URL segment. This is probably due to a bad percent encoding (${error}).`
);
return value;
}
}
function stripBasename(pathname, basename) {
if (basename === "/") return pathname;
if (!pathname.toLowerCase().startsWith(basename.toLowerCase())) {
return null;
}
let startIndex = basename.endsWith("/") ? basename.length - 1 : basename.length;
let nextChar = pathname.charAt(startIndex);
if (nextChar && nextChar !== "/") {
return null;
}
return pathname.slice(startIndex) || "/";
}
function resolvePath(to, fromPathname = "/") {
let {
pathname: toPathname,
search = "",
hash = ""
} = typeof to === "string" ? parsePath(to) : to;
let pathname = toPathname ? toPathname.startsWith("/") ? toPathname : resolvePathname(toPathname, fromPathname) : fromPathname;
return {
pathname,
search: normalizeSearch(search),
hash: normalizeHash(hash)
};
}
function resolvePathname(relativePath, fromPathname) {
let segments = fromPathname.replace(/\/+$/, "").split("/");
let relativeSegments = relativePath.split("/");
relativeSegments.forEach((segment) => {
if (segment === "..") {
if (segments.length > 1) segments.pop();
} else if (segment !== ".") {
segments.push(segment);
}
});
return segments.length > 1 ? segments.join("/") : "/";
}
function getInvalidPathError(char, field, dest, path) {
return `Cannot include a '${char}' character in a manually specified \`to.${field}\` field [${JSON.stringify(
path
)}]. Please separate it out to the \`to.${dest}\` field. Alternatively you may provide the full path as a string in and the router will parse it for you.`;
}
function getPathContributingMatches(matches) {
return matches.filter(
(match, index) => index === 0 || match.route.path && match.route.path.length > 0
);
}
function getResolveToMatches(matches) {
let pathMatches = getPathContributingMatches(matches);
return pathMatches.map(
(match, idx) => idx === pathMatches.length - 1 ? match.pathname : match.pathnameBase
);
}
function resolveTo(toArg, routePathnames, locationPathname, isPathRelative = false) {
let to;
if (typeof toArg === "string") {
to = parsePath(toArg);
} else {
to = { ...toArg };
invariant(
!to.pathname || !to.pathname.includes("?"),
getInvalidPathError("?", "pathname", "search", to)
);
invariant(
!to.pathname || !to.pathname.includes("#"),
getInvalidPathError("#", "pathname", "hash", to)
);
invariant(
!to.search || !to.search.includes("#"),
getInvalidPathError("#", "search", "hash", to)
);
}
let isEmptyPath = toArg === "" || to.pathname === "";
let toPathname = isEmptyPath ? "/" : to.pathname;
let from;
if (toPathname == null) {
from = locationPathname;
} else {
let routePathnameIndex = routePathnames.length - 1;
if (!isPathRelative && toPathname.startsWith("..")) {
let toSegments = toPathname.split("/");
while (toSegments[0] === "..") {
toSegments.shift();
routePathnameIndex -= 1;
}
to.pathname = toSegments.join("/");
}
from = routePathnameIndex >= 0 ? routePathnames[routePathnameIndex] : "/";
}
let path = resolvePath(to, from);
let hasExplicitTrailingSlash = toPathname && toPathname !== "/" && toPathname.endsWith("/");
let hasCurrentTrailingSlash = (isEmptyPath || toPathname === ".") && locationPathname.endsWith("/");
if (!path.pathname.endsWith("/") && (hasExplicitTrailingSlash || hasCurrentTrailingSlash)) {
path.pathname += "/";
}
return path;
}
var joinPaths = (paths) => paths.join("/").replace(/\/\/+/g, "/");
var normalizePathname = (pathname) => pathname.replace(/\/+$/, "").replace(/^\/*/, "/");
var normalizeSearch = (search) => !search || search === "?" ? "" : search.startsWith("?") ? search : "?" + search;
var normalizeHash = (hash) => !hash || hash === "#" ? "" : hash.startsWith("#") ? hash : "#" + hash;
var DataWithResponseInit = class {
constructor(data2, init) {
this.type = "DataWithResponseInit";
this.data = data2;
this.init = init || null;
}
};
function data(data2, init) {
return new DataWithResponseInit(
data2,
typeof init === "number" ? { status: init } : init
);
}
var redirect = (url, init = 302) => {
let responseInit = init;
if (typeof responseInit === "number") {
responseInit = { status: responseInit };
} else if (typeof responseInit.status === "undefined") {
responseInit.status = 302;
}
let headers = new Headers(responseInit.headers);
headers.set("Location", url);
return new Response(null, {
...responseInit,
headers
});
};
var redirectDocument = (url, init) => {
let response = redirect(url, init);
response.headers.set("X-Remix-Reload-Document", "true");
return response;
};
var replace = (url, init) => {
let response = redirect(url, init);
response.headers.set("X-Remix-Replace", "true");
return response;
};
var ErrorResponseImpl = class {
constructor(status, statusText, data2, internal = false) {
this.status = status;
this.statusText = statusText || "";
this.internal = internal;
if (data2 instanceof Error) {
this.data = data2.toString();
this.error = data2;
} else {
this.data = data2;
}
}
};
function isRouteErrorResponse(error) {
return error != null && typeof error.status === "number" && typeof error.statusText === "string" && typeof error.internal === "boolean" && "data" in error;
}
// lib/router/router.ts
var validMutationMethodsArr = [
"POST",
"PUT",
"PATCH",
"DELETE"
];
var validMutationMethods = new Set(
validMutationMethodsArr
);
var validRequestMethodsArr = [
"GET",
...validMutationMethodsArr
];
var validRequestMethods = new Set(validRequestMethodsArr);
var redirectStatusCodes = /* @__PURE__ */ new Set([301, 302, 303, 307, 308]);
var redirectPreserveMethodStatusCodes = /* @__PURE__ */ new Set([307, 308]);
var IDLE_NAVIGATION = {
state: "idle",
location: void 0,
formMethod: void 0,
formAction: void 0,
formEncType: void 0,
formData: void 0,
json: void 0,
text: void 0
};
var IDLE_FETCHER = {
state: "idle",
data: void 0,
formMethod: void 0,
formAction: void 0,
formEncType: void 0,
formData: void 0,
json: void 0,
text: void 0
};
var IDLE_BLOCKER = {
state: "unblocked",
proceed: void 0,
reset: void 0,
location: void 0
};
var ABSOLUTE_URL_REGEX = /^(?:[a-z][a-z0-9+.-]*:|\/\/)/i;
var defaultMapRouteProperties = (route) => ({
hasErrorBoundary: Boolean(route.hasErrorBoundary)
});
var TRANSITIONS_STORAGE_KEY = "remix-router-transitions";
var ResetLoaderDataSymbol = Symbol("ResetLoaderData");
function createRouter(init) {
const routerWindow = init.window ? init.window : typeof window !== "undefined" ? window : void 0;
const isBrowser2 = typeof routerWindow !== "undefined" && typeof routerWindow.document !== "undefined" && typeof routerWindow.document.createElement !== "undefined";
invariant(
init.routes.length > 0,
"You must provide a non-empty routes array to createRouter"
);
let mapRouteProperties2 = init.mapRouteProperties || defaultMapRouteProperties;
let manifest = {};
let dataRoutes = convertRoutesToDataRoutes(
init.routes,
mapRouteProperties2,
void 0,
manifest
);
let inFlightDataRoutes;
let basename = init.basename || "/";
let dataStrategyImpl = init.dataStrategy || defaultDataStrategy;
let patchRoutesOnNavigationImpl = init.patchRoutesOnNavigation;
let future = {
...init.future
};
let unlistenHistory = null;
let subscribers = /* @__PURE__ */ new Set();
let savedScrollPositions2 = null;
let getScrollRestorationKey2 = null;
let getScrollPosition = null;
let initialScrollRestored = init.hydrationData != null;
let initialMatches = matchRoutes(dataRoutes, init.history.location, basename);
let initialErrors = null;
if (initialMatches == null && !patchRoutesOnNavigationImpl) {
let error = getInternalRouterError(404, {
pathname: init.history.location.pathname
});
let { matches, route } = getShortCircuitMatches(dataRoutes);
initialMatches = matches;
initialErrors = { [route.id]: error };
}
if (initialMatches && !init.hydrationData) {
let fogOfWar = checkFogOfWar(
initialMatches,
dataRoutes,
init.history.location.pathname
);
if (fogOfWar.active) {
initialMatches = null;
}
}
let initialized;
if (!initialMatches) {
initialized = false;
initialMatches = [];
let fogOfWar = checkFogOfWar(
null,
dataRoutes,
init.history.location.pathname
);
if (fogOfWar.active && fogOfWar.matches) {
initialMatches = fogOfWar.matches;
}
} else if (initialMatches.some((m) => m.route.lazy)) {
initialized = false;
} else if (!initialMatches.some((m) => m.route.loader)) {
initialized = true;
} else {
let loaderData = init.hydrationData ? init.hydrationData.loaderData : null;
let errors = init.hydrationData ? init.hydrationData.errors : null;
if (errors) {
let idx = initialMatches.findIndex(
(m) => errors[m.route.id] !== void 0
);
initialized = initialMatches.slice(0, idx + 1).every((m) => !shouldLoadRouteOnHydration(m.route, loaderData, errors));
} else {
initialized = initialMatches.every(
(m) => !shouldLoadRouteOnHydration(m.route, loaderData, errors)
);
}
}
let router;
let state = {
historyAction: init.history.action,
location: init.history.location,
matches: initialMatches,
initialized,
navigation: IDLE_NAVIGATION,
// Don't restore on initial updateState() if we were SSR'd
restoreScrollPosition: init.hydrationData != null ? false : null,
preventScrollReset: false,
revalidation: "idle",
loaderData: init.hydrationData && init.hydrationData.loaderData || {},
actionData: init.hydrationData && init.hydrationData.actionData || null,
errors: init.hydrationData && init.hydrationData.errors || initialErrors,
fetchers: /* @__PURE__ */ new Map(),
blockers: /* @__PURE__ */ new Map()
};
let pendingAction = "POP" /* Pop */;
let pendingPreventScrollReset = false;
let pendingNavigationController;
let pendingViewTransitionEnabled = false;
let appliedViewTransitions = /* @__PURE__ */ new Map();
let removePageHideEventListener = null;
let isUninterruptedRevalidation = false;
let isRevalidationRequired = false;
let cancelledFetcherLoads = /* @__PURE__ */ new Set();
let fetchControllers = /* @__PURE__ */ new Map();
let incrementingLoadId = 0;
let pendingNavigationLoadId = -1;
let fetchReloadIds = /* @__PURE__ */ new Map();
let fetchRedirectIds = /* @__PURE__ */ new Set();
let fetchLoadMatches = /* @__PURE__ */ new Map();
let activeFetchers = /* @__PURE__ */ new Map();
let fetchersQueuedForDeletion = /* @__PURE__ */ new Set();
let blockerFunctions = /* @__PURE__ */ new Map();
let unblockBlockerHistoryUpdate = void 0;
let pendingRevalidationDfd = null;
function initialize() {
unlistenHistory = init.history.listen(
({ action: historyAction, location, delta }) => {
if (unblockBlockerHistoryUpdate) {
unblockBlockerHistoryUpdate();
unblockBlockerHistoryUpdate = void 0;
return;
}
warning(
blockerFunctions.size === 0 || delta != null,
"You are trying to use a blocker on a POP navigation to a location that was not created by @remix-run/router. This will fail silently in production. This can happen if you are navigating outside the router via `window.history.pushState`/`window.location.hash` instead of using router navigation APIs. This can also happen if you are using createHashRouter and the user manually changes the URL."
);
let blockerKey = shouldBlockNavigation({
currentLocation: state.location,
nextLocation: location,
historyAction
});
if (blockerKey && delta != null) {
let nextHistoryUpdatePromise = new Promise((resolve) => {
unblockBlockerHistoryUpdate = resolve;
});
init.history.go(delta * -1);
updateBlocker(blockerKey, {
state: "blocked",
location,
proceed() {
updateBlocker(blockerKey, {
state: "proceeding",
proceed: void 0,
reset: void 0,
location
});
nextHistoryUpdatePromise.then(() => init.history.go(delta));
},
reset() {
let blockers = new Map(state.blockers);
blockers.set(blockerKey, IDLE_BLOCKER);
updateState({ blockers });
}
});
return;
}
return startNavigation(historyAction, location);
}
);
if (isBrowser2) {
restoreAppliedTransitions(routerWindow, appliedViewTransitions);
let _saveAppliedTransitions = () => persistAppliedTransitions(routerWindow, appliedViewTransitions);
routerWindow.addEventListener("pagehide", _saveAppliedTransitions);
removePageHideEventListener = () => routerWindow.removeEventListener("pagehide", _saveAppliedTransitions);
}
if (!state.initialized) {
startNavigation("POP" /* Pop */, state.location, {
initialHydration: true
});
}
return router;
}
function dispose() {
if (unlistenHistory) {
unlistenHistory();
}
if (removePageHideEventListener) {
removePageHideEventListener();
}
subscribers.clear();
pendingNavigationController && pendingNavigationController.abort();
state.fetchers.forEach((_, key) => deleteFetcher(key));
state.blockers.forEach((_, key) => deleteBlocker(key));
}
function subscribe(fn) {
subscribers.add(fn);
return () => subscribers.delete(fn);
}
function updateState(newState, opts = {}) {
state = {
...state,
...newState
};
let unmountedFetchers = [];
let mountedFetchers = [];
state.fetchers.forEach((fetcher, key) => {
if (fetcher.state === "idle") {
if (fetchersQueuedForDeletion.has(key)) {
unmountedFetchers.push(key);
} else {
mountedFetchers.push(key);
}
}
});
[...subscribers].forEach(
(subscriber) => subscriber(state, {
deletedFetchers: unmountedFetchers,
viewTransitionOpts: opts.viewTransitionOpts,
flushSync: opts.flushSync === true
})
);
unmountedFetchers.forEach((key) => deleteFetcher(key));
mountedFetchers.forEach((key) => state.fetchers.delete(key));
}
function completeNavigation(location, newState, { flushSync } = {}) {
let isActionReload = state.actionData != null && state.navigation.formMethod != null && isMutationMethod(state.navigation.formMethod) && state.navigation.state === "loading" && location.state?._isRedirect !== true;
let actionData;
if (newState.actionData) {
if (Object.keys(newState.actionData).length > 0) {
actionData = newState.actionData;
} else {
actionData = null;
}
} else if (isActionReload) {
actionData = state.actionData;
} else {
actionData = null;
}
let loaderData = newState.loaderData ? mergeLoaderData(
state.loaderData,
newState.loaderData,
newState.matches || [],
newState.errors
) : state.loaderData;
let blockers = state.blockers;
if (blockers.size > 0) {
blockers = new Map(blockers);
blockers.forEach((_, k) => blockers.set(k, IDLE_BLOCKER));
}
let preventScrollReset = pendingPreventScrollReset === true || state.navigation.formMethod != null && isMutationMethod(state.navigation.formMethod) && location.state?._isRedirect !== true;
if (inFlightDataRoutes) {
dataRoutes = inFlightDataRoutes;
inFlightDataRoutes = void 0;
}
if (isUninterruptedRevalidation) {
} else if (pendingAction === "POP" /* Pop */) {
} else if (pendingAction === "PUSH" /* Push */) {
init.history.push(location, location.state);
} else if (pendingAction === "REPLACE" /* Replace */) {
init.history.replace(location, location.state);
}
let viewTransitionOpts;
if (pendingAction === "POP" /* Pop */) {
let priorPaths = appliedViewTransitions.get(state.location.pathname);
if (priorPaths && priorPaths.has(location.pathname)) {
viewTransitionOpts = {
currentLocation: state.location,
nextLocation: location
};
} else if (appliedViewTransitions.has(location.pathname)) {
viewTransitionOpts = {
currentLocation: location,
nextLocation: state.location
};
}
} else if (pendingViewTransitionEnabled) {
let toPaths = appliedViewTransitions.get(state.location.pathname);
if (toPaths) {
toPaths.add(location.pathname);
} else {
toPaths = /* @__PURE__ */ new Set([location.pathname]);
appliedViewTransitions.set(state.location.pathname, toPaths);
}
viewTransitionOpts = {
currentLocation: state.location,
nextLocation: location
};
}
updateState(
{
...newState,
// matches, errors, fetchers go through as-is
actionData,
loaderData,
historyAction: pendingAction,
location,
initialized: true,
navigation: IDLE_NAVIGATION,
revalidation: "idle",
restoreScrollPosition: getSavedScrollPosition(
location,
newState.matches || state.matches
),
preventScrollReset,
blockers
},
{
viewTransitionOpts,
flushSync: flushSync === true
}
);
pendingAction = "POP" /* Pop */;
pendingPreventScrollReset = false;
pendingViewTransitionEnabled = false;
isUninterruptedRevalidation = false;
isRevalidationRequired = false;
pendingRevalidationDfd?.resolve();
pendingRevalidationDfd = null;
}
async function navigate(to, opts) {
if (typeof to === "number") {
init.history.go(to);
return;
}
let normalizedPath = normalizeTo(
state.location,
state.matches,
basename,
to,
opts?.fromRouteId,
opts?.relative
);
let { path, submission, error } = normalizeNavigateOptions(
false,
normalizedPath,
opts
);
let currentLocation = state.location;
let nextLocation = createLocation(state.location, path, opts && opts.state);
nextLocation = {
...nextLocation,
...init.history.encodeLocation(nextLocation)
};
let userReplace = opts && opts.replace != null ? opts.replace : void 0;
let historyAction = "PUSH" /* Push */;
if (userReplace === true) {
historyAction = "REPLACE" /* Replace */;
} else if (userReplace === false) {
} else if (submission != null && isMutationMethod(submission.formMethod) && submission.formAction === state.location.pathname + state.location.search) {
historyAction = "REPLACE" /* Replace */;
}
let preventScrollReset = opts && "preventScrollReset" in opts ? opts.preventScrollReset === true : void 0;
let flushSync = (opts && opts.flushSync) === true;
let blockerKey = shouldBlockNavigation({
currentLocation,
nextLocation,
historyAction
});
if (blockerKey) {
updateBlocker(blockerKey, {
state: "blocked",
location: nextLocation,
proceed() {
updateBlocker(blockerKey, {
state: "proceeding",
proceed: void 0,
reset: void 0,
location: nextLocation
});
navigate(to, opts);
},
reset() {
let blockers = new Map(state.blockers);
blockers.set(blockerKey, IDLE_BLOCKER);
updateState({ blockers });
}
});
return;
}
await startNavigation(historyAction, nextLocation, {
submission,
// Send through the formData serialization error if we have one so we can
// render at the right error boundary after we match routes
pendingError: error,
preventScrollReset,
replace: opts && opts.replace,
enableViewTransition: opts && opts.viewTransition,
flushSync
});
}
function revalidate() {
if (!pendingRevalidationDfd) {
pendingRevalidationDfd = createDeferred();
}
interruptActiveLoads();
updateState({ revalidation: "loading" });
let promise = pendingRevalidationDfd.promise;
if (state.navigation.state === "submitting") {
return promise;
}
if (state.navigation.state === "idle") {
startNavigation(state.historyAction, state.location, {
startUninterruptedRevalidation: true
});
return promise;
}
startNavigation(
pendingAction || state.historyAction,
state.navigation.location,
{
overrideNavigation: state.navigation,
// Proxy through any rending view transition
enableViewTransition: pendingViewTransitionEnabled === true
}
);
return promise;
}
async function startNavigation(historyAction, location, opts) {
pendingNavigationController && pendingNavigationController.abort();
pendingNavigationController = null;
pendingAction = historyAction;
isUninterruptedRevalidation = (opts && opts.startUninterruptedRevalidation) === true;
saveScrollPosition(state.location, state.matches);
pendingPreventScrollReset = (opts && opts.preventScrollReset) === true;
pendingViewTransitionEnabled = (opts && opts.enableViewTransition) === true;
let routesToUse = inFlightDataRoutes || dataRoutes;
let loadingNavigation = opts && opts.overrideNavigation;
let matches = matchRoutes(routesToUse, location, basename);
let flushSync = (opts && opts.flushSync) === true;
let fogOfWar = checkFogOfWar(matches, routesToUse, location.pathname);
if (fogOfWar.active && fogOfWar.matches) {
matches = fogOfWar.matches;
}
if (!matches) {
let { error, notFoundMatches, route } = handleNavigational404(
location.pathname
);
completeNavigation(
location,
{
matches: notFoundMatches,
loaderData: {},
errors: {
[route.id]: error
}
},
{ flushSync }
);
return;
}
if (state.initialized && !isRevalidationRequired && isHashChangeOnly(state.location, location) && !(opts && opts.submission && isMutationMethod(opts.submission.formMethod))) {
completeNavigation(location, { matches }, { flushSync });
return;
}
pendingNavigationController = new AbortController();
let request = createClientSideRequest(
init.history,
location,
pendingNavigationController.signal,
opts && opts.submission
);
let pendingActionResult;
if (opts && opts.pendingError) {
pendingActionResult = [
findNearestBoundary(matches).route.id,
{ type: "error" /* error */, error: opts.pendingError }
];
} else if (opts && opts.submission && isMutationMethod(opts.submission.formMethod)) {
let actionResult = await handleAction(
request,
location,
opts.submission,
matches,
fogOfWar.active,
{ replace: opts.replace, flushSync }
);
if (actionResult.shortCircuited) {
return;
}
if (actionResult.pendingActionResult) {
let [routeId, result] = actionResult.pendingActionResult;
if (isErrorResult(result) && isRouteErrorResponse(result.error) && result.error.status === 404) {
pendingNavigationController = null;
completeNavigation(location, {
matches: actionResult.matches,
loaderData: {},
errors: {
[routeId]: result.error
}
});
return;
}
}
matches = actionResult.matches || matches;
pendingActionResult = actionResult.pendingActionResult;
loadingNavigation = getLoadingNavigation(location, opts.submission);
flushSync = false;
fogOfWar.active = false;
request = createClientSideRequest(
init.history,
request.url,
request.signal
);
}
let {
shortCircuited,
matches: updatedMatches,
loaderData,
errors
} = await handleLoaders(
request,
location,
matches,
fogOfWar.active,
loadingNavigation,
opts && opts.submission,
opts && opts.fetcherSubmission,
opts && opts.replace,
opts && opts.initialHydration === true,
flushSync,
pendingActionResult
);
if (shortCircuited) {
return;
}
pendingNavigationController = null;
completeNavigation(location, {
matches: updatedMatches || matches,
...getActionDataForCommit(pendingActionResult),
loaderData,
errors
});
}
async function handleAction(request, location, submission, matches, isFogOfWar, opts = {}) {
interruptActiveLoads();
let navigation = getSubmittingNavigation(location, submission);
updateState({ navigation }, { flushSync: opts.flushSync === true });
if (isFogOfWar) {
let discoverResult = await discoverRoutes(
matches,
location.pathname,
request.signal
);
if (discoverResult.type === "aborted") {
return { shortCircuited: true };
} else if (discoverResult.type === "error") {
let boundaryId = findNearestBoundary(discoverResult.partialMatches).route.id;
return {
matches: discoverResult.partialMatches,
pendingActionResult: [
boundaryId,
{
type: "error" /* error */,
error: discoverResult.error
}
]
};
} else if (!discoverResult.matches) {
let { notFoundMatches, error, route } = handleNavigational404(
location.pathname
);
return {
matches: notFoundMatches,
pendingActionResult: [
route.id,
{
type: "error" /* error */,
error
}
]
};
} else {
matches = discoverResult.matches;
}
}
let result;
let actionMatch = getTargetMatch(matches, location);
if (!actionMatch.route.action && !actionMatch.route.lazy) {
result = {
type: "error" /* error */,
error: getInternalRouterError(405, {
method: request.method,
pathname: location.pathname,
routeId: actionMatch.route.id
})
};
} else {
let results = await callDataStrategy(
"action",
state,
request,
[actionMatch],
matches,
null
);
result = results[actionMatch.route.id];
if (request.signal.aborted) {
return { shortCircuited: true };
}
}
if (isRedirectResult(result)) {
let replace2;
if (opts && opts.replace != null) {
replace2 = opts.replace;
} else {
let location2 = normalizeRedirectLocation(
result.response.headers.get("Location"),
new URL(request.url),
basename
);
replace2 = location2 === state.location.pathname + state.location.search;
}
await startRedirectNavigation(request, result, true, {
submission,
replace: replace2
});
return { shortCircuited: true };
}
if (isErrorResult(result)) {
let boundaryMatch = findNearestBoundary(matches, actionMatch.route.id);
if ((opts && opts.replace) !== true) {
pendingAction = "PUSH" /* Push */;
}
return {
matches,
pendingActionResult: [boundaryMatch.route.id, result]
};
}
return {
matches,
pendingActionResult: [actionMatch.route.id, result]
};
}
async function handleLoaders(request, location, matches, isFogOfWar, overrideNavigation, submission, fetcherSubmission, replace2, initialHydration, flushSync, pendingActionResult) {
let loadingNavigation = overrideNavigation || getLoadingNavigation(location, submission);
let activeSubmission = submission || fetcherSubmission || getSubmissionFromNavigation(loadingNavigation);
let shouldUpdateNavigationState = !isUninterruptedRevalidation && !initialHydration;
if (isFogOfWar) {
if (shouldUpdateNavigationState) {
let actionData = getUpdatedActionData(pendingActionResult);
updateState(
{
navigation: loadingNavigation,
...actionData !== void 0 ? { actionData } : {}
},
{
flushSync
}
);
}
let discoverResult = await discoverRoutes(
matches,
location.pathname,
request.signal
);
if (discoverResult.type === "aborted") {
return { shortCircuited: true };
} else if (discoverResult.type === "error") {
let boundaryId = findNearestBoundary(discoverResult.partialMatches).route.id;
return {
matches: discoverResult.partialMatches,
loaderData: {},
errors: {
[boundaryId]: discoverResult.error
}
};
} else if (!discoverResult.matches) {
let { error, notFoundMatches, route } = handleNavigational404(
location.pathname
);
return {
matches: notFoundMatches,
loaderData: {},
errors: {
[route.id]: error
}
};
} else {
matches = discoverResult.matches;
}
}
let routesToUse = inFlightDataRoutes || dataRoutes;
let [matchesToLoad, revalidatingFetchers] = getMatchesToLoad(
init.history,
state,
matches,
activeSubmission,
location,
initialHydration === true,
isRevalidationRequired,
cancelledFetcherLoads,
fetchersQueuedForDeletion,
fetchLoadMatches,
fetchRedirectIds,
routesToUse,
basename,
pendingActionResult
);
pendingNavigationLoadId = ++incrementingLoadId;
if (matchesToLoad.length === 0 && revalidatingFetchers.length === 0) {
let updatedFetchers2 = markFetchRedirectsDone();
completeNavigation(
location,
{
matches,
loaderData: {},
// Commit pending error if we're short circuiting
errors: pendingActionResult && isErrorResult(pendingActionResult[1]) ? { [pendingActionResult[0]]: pendingActionResult[1].error } : null,
...getActionDataForCommit(pendingActionResult),
...updatedFetchers2 ? { fetchers: new Map(state.fetchers) } : {}
},
{ flushSync }
);
return { shortCircuited: true };
}
if (shouldUpdateNavigationState) {
let updates = {};
if (!isFogOfWar) {
updates.navigation = loadingNavigation;
let actionData = getUpdatedActionData(pendingActionResult);
if (actionData !== void 0) {
updates.actionData = actionData;
}
}
if (revalidatingFetchers.length > 0) {
updates.fetchers = getUpdatedRevalidatingFetchers(revalidatingFetchers);
}
updateState(updates, { flushSync });
}
revalidatingFetchers.forEach((rf) => {
abortFetcher(rf.key);
if (rf.controller) {
fetchControllers.set(rf.key, rf.controller);
}
});
let abortPendingFetchRevalidations = () => revalidatingFetchers.forEach((f) => abortFetcher(f.key));
if (pendingNavigationController) {
pendingNavigationController.signal.addEventListener(
"abort",
abortPendingFetchRevalidations
);
}
let { loaderResults, fetcherResults } = await callLoadersAndMaybeResolveData(
state,
matches,
matchesToLoad,
revalidatingFetchers,
request
);
if (request.signal.aborted) {
return { shortCircuited: true };
}
if (pendingNavigationController) {
pendingNavigationController.signal.removeEventListener(
"abort",
abortPendingFetchRevalidations
);
}
revalidatingFetchers.forEach((rf) => fetchControllers.delete(rf.key));
let redirect2 = findRedirect(loaderResults);
if (redirect2) {
await startRedirectNavigation(request, redirect2.result, true, {
replace: replace2
});
return { shortCircuited: true };
}
redirect2 = findRedirect(fetcherResults);
if (redirect2) {
fetchRedirectIds.add(redirect2.key);
await startRedirectNavigation(request, redirect2.result, true, {
replace: replace2
});
return { shortCircuited: true };
}
let { loaderData, errors } = processLoaderData(
state,
matches,
loaderResults,
pendingActionResult,
revalidatingFetchers,
fetcherResults
);
if (initialHydration && state.errors) {
errors = { ...state.errors, ...errors };
}
let updatedFetchers = markFetchRedirectsDone();
let didAbortFetchLoads = abortStaleFetchLoads(pendingNavigationLoadId);
let shouldUpdateFetchers = updatedFetchers || didAbortFetchLoads || revalidatingFetchers.length > 0;
return {
matches,
loaderData,
errors,
...shouldUpdateFetchers ? { fetchers: new Map(state.fetchers) } : {}
};
}
function getUpdatedActionData(pendingActionResult) {
if (pendingActionResult && !isErrorResult(pendingActionResult[1])) {
return {
[pendingActionResult[0]]: pendingActionResult[1].data
};
} else if (state.actionData) {
if (Object.keys(state.actionData).length === 0) {
return null;
} else {
return state.actionData;
}
}
}
function getUpdatedRevalidatingFetchers(revalidatingFetchers) {
revalidatingFetchers.forEach((rf) => {
let fetcher = state.fetchers.get(rf.key);
let revalidatingFetcher = getLoadingFetcher(
void 0,
fetcher ? fetcher.data : void 0
);
state.fetchers.set(rf.key, revalidatingFetcher);
});
return new Map(state.fetchers);
}
async function fetch2(key, routeId, href, opts) {
abortFetcher(key);
let flushSync = (opts && opts.flushSync) === true;
let routesToUse = inFlightDataRoutes || dataRoutes;
let normalizedPath = normalizeTo(
state.location,
state.matches,
basename,
href,
routeId,
opts?.relative
);
let matches = matchRoutes(routesToUse, normalizedPath, basename);
let fogOfWar = checkFogOfWar(matches, routesToUse, normalizedPath);
if (fogOfWar.active && fogOfWar.matches) {
matches = fogOfWar.matches;
}
if (!matches) {
setFetcherError(
key,
routeId,
getInternalRouterError(404, { pathname: normalizedPath }),
{ flushSync }
);
return;
}
let { path, submission, error } = normalizeNavigateOptions(
true,
normalizedPath,
opts
);
if (error) {
setFetcherError(key, routeId, error, { flushSync });
return;
}
let match = getTargetMatch(matches, path);
let preventScrollReset = (opts && opts.preventScrollReset) === true;
if (submission && isMutationMethod(submission.formMethod)) {
await handleFetcherAction(
key,
routeId,
path,
match,
matches,
fogOfWar.active,
flushSync,
preventScrollReset,
submission
);
return;
}
fetchLoadMatches.set(key, { routeId, path });
await handleFetcherLoader(
key,
routeId,
path,
match,
matches,
fogOfWar.active,
flushSync,
preventScrollReset,
submission
);
}
async function handleFetcherAction(key, routeId, path, match, requestMatches, isFogOfWar, flushSync, preventScrollReset, submission) {
interruptActiveLoads();
fetchLoadMatches.delete(key);
function detectAndHandle405Error(m) {
if (!m.route.action && !m.route.lazy) {
let error = getInternalRouterError(405, {
method: submission.formMethod,
pathname: path,
routeId
});
setFetcherError(key, routeId, error, { flushSync });
return true;
}
return false;
}
if (!isFogOfWar && detectAndHandle405Error(match)) {
return;
}
let existingFetcher = state.fetchers.get(key);
updateFetcherState(key, getSubmittingFetcher(submission, existingFetcher), {
flushSync
});
let abortController = new AbortController();
let fetchRequest = createClientSideRequest(
init.history,
path,
abortController.signal,
submission
);
if (isFogOfWar) {
let discoverResult = await discoverRoutes(
requestMatches,
path,
fetchRequest.signal
);
if (discoverResult.type === "aborted") {
return;
} else if (discoverResult.type === "error") {
setFetcherError(key, routeId, discoverResult.error, { flushSync });
return;
} else if (!discoverResult.matches) {
setFetcherError(
key,
routeId,
getInternalRouterError(404, { pathname: path }),
{ flushSync }
);
return;
} else {
requestMatches = discoverResult.matches;
match = getTargetMatch(requestMatches, path);
if (detectAndHandle405Error(match)) {
return;
}
}
}
fetchControllers.set(key, abortController);
let originatingLoadId = incrementingLoadId;
let actionResults = await callDataStrategy(
"action",
state,
fetchRequest,
[match],
requestMatches,
key
);
let actionResult = actionResults[match.route.id];
if (fetchRequest.signal.aborted) {
if (fetchControllers.get(key) === abortController) {
fetchControllers.delete(key);
}
return;
}
if (fetchersQueuedForDeletion.has(key)) {
if (isRedirectResult(actionResult) || isErrorResult(actionResult)) {
updateFetcherState(key, getDoneFetcher(void 0));
return;
}
} else {
if (isRedirectResult(actionResult)) {
fetchControllers.delete(key);
if (pendingNavigationLoadId > originatingLoadId) {
updateFetcherState(key, getDoneFetcher(void 0));
return;
} else {
fetchRedirectIds.add(key);
updateFetcherState(key, getLoadingFetcher(submission));
return startRedirectNavigation(fetchRequest, actionResult, false, {
fetcherSubmission: submission,
preventScrollReset
});
}
}
if (isErrorResult(actionResult)) {
setFetcherError(key, routeId, actionResult.error);
return;
}
}
let nextLocation = state.navigation.location || state.location;
let revalidationRequest = createClientSideRequest(
init.history,
nextLocation,
abortController.signal
);
let routesToUse = inFlightDataRoutes || dataRoutes;
let matches = state.navigation.state !== "idle" ? matchRoutes(routesToUse, state.navigation.location, basename) : state.matches;
invariant(matches, "Didn't find any matches after fetcher action");
let loadId = ++incrementingLoadId;
fetchReloadIds.set(key, loadId);
let loadFetcher = getLoadingFetcher(submission, actionResult.data);
state.fetchers.set(key, loadFetcher);
let [matchesToLoad, revalidatingFetchers] = getMatchesToLoad(
init.history,
state,
matches,
submission,
nextLocation,
false,
isRevalidationRequired,
cancelledFetcherLoads,
fetchersQueuedForDeletion,
fetchLoadMatches,
fetchRedirectIds,
routesToUse,
basename,
[match.route.id, actionResult]
);
revalidatingFetchers.filter((rf) => rf.key !== key).forEach((rf) => {
let staleKey = rf.key;
let existingFetcher2 = state.fetchers.get(staleKey);
let revalidatingFetcher = getLoadingFetcher(
void 0,
existingFetcher2 ? existingFetcher2.data : void 0
);
state.fetchers.set(staleKey, revalidatingFetcher);
abortFetcher(staleKey);
if (rf.controller) {
fetchControllers.set(staleKey, rf.controller);
}
});
updateState({ fetchers: new Map(state.fetchers) });
let abortPendingFetchRevalidations = () => revalidatingFetchers.forEach((rf) => abortFetcher(rf.key));
abortController.signal.addEventListener(
"abort",
abortPendingFetchRevalidations
);
let { loaderResults, fetcherResults } = await callLoadersAndMaybeResolveData(
state,
matches,
matchesToLoad,
revalidatingFetchers,
revalidationRequest
);
if (abortController.signal.aborted) {
return;
}
abortController.signal.removeEventListener(
"abort",
abortPendingFetchRevalidations
);
fetchReloadIds.delete(key);
fetchControllers.delete(key);
revalidatingFetchers.forEach((r) => fetchControllers.delete(r.key));
let redirect2 = findRedirect(loaderResults);
if (redirect2) {
return startRedirectNavigation(
revalidationRequest,
redirect2.result,
false,
{ preventScrollReset }
);
}
redirect2 = findRedirect(fetcherResults);
if (redirect2) {
fetchRedirectIds.add(redirect2.key);
return startRedirectNavigation(
revalidationRequest,
redirect2.result,
false,
{ preventScrollReset }
);
}
let { loaderData, errors } = processLoaderData(
state,
matches,
loaderResults,
void 0,
revalidatingFetchers,
fetcherResults
);
if (state.fetchers.has(key)) {
let doneFetcher = getDoneFetcher(actionResult.data);
state.fetchers.set(key, doneFetcher);
}
abortStaleFetchLoads(loadId);
if (state.navigation.state === "loading" && loadId > pendingNavigationLoadId) {
invariant(pendingAction, "Expected pending action");
pendingNavigationController && pendingNavigationController.abort();
completeNavigation(state.navigation.location, {
matches,
loaderData,
errors,
fetchers: new Map(state.fetchers)
});
} else {
updateState({
errors,
loaderData: mergeLoaderData(
state.loaderData,
loaderData,
matches,
errors
),
fetchers: new Map(state.fetchers)
});
isRevalidationRequired = false;
}
}
async function handleFetcherLoader(key, routeId, path, match, matches, isFogOfWar, flushSync, preventScrollReset, submission) {
let existingFetcher = state.fetchers.get(key);
updateFetcherState(
key,
getLoadingFetcher(
submission,
existingFetcher ? existingFetcher.data : void 0
),
{ flushSync }
);
let abortController = new AbortController();
let fetchRequest = createClientSideRequest(
init.history,
path,
abortController.signal
);
if (isFogOfWar) {
let discoverResult = await discoverRoutes(
matches,
path,
fetchRequest.signal
);
if (discoverResult.type === "aborted") {
return;
} else if (discoverResult.type === "error") {
setFetcherError(key, routeId, discoverResult.error, { flushSync });
return;
} else if (!discoverResult.matches) {
setFetcherError(
key,
routeId,
getInternalRouterError(404, { pathname: path }),
{ flushSync }
);
return;
} else {
matches = discoverResult.matches;
match = getTargetMatch(matches, path);
}
}
fetchControllers.set(key, abortController);
let originatingLoadId = incrementingLoadId;
let results = await callDataStrategy(
"loader",
state,
fetchRequest,
[match],
matches,
key
);
let result = results[match.route.id];
if (fetchControllers.get(key) === abortController) {
fetchControllers.delete(key);
}
if (fetchRequest.signal.aborted) {
return;
}
if (fetchersQueuedForDeletion.has(key)) {
updateFetcherState(key, getDoneFetcher(void 0));
return;
}
if (isRedirectResult(result)) {
if (pendingNavigationLoadId > originatingLoadId) {
updateFetcherState(key, getDoneFetcher(void 0));
return;
} else {
fetchRedirectIds.add(key);
await startRedirectNavigation(fetchRequest, result, false, {
preventScrollReset
});
return;
}
}
if (isErrorResult(result)) {
setFetcherError(key, routeId, result.error);
return;
}
updateFetcherState(key, getDoneFetcher(result.data));
}
async function startRedirectNavigation(request, redirect2, isNavigation, {
submission,
fetcherSubmission,
preventScrollReset,
replace: replace2
} = {}) {
if (redirect2.response.headers.has("X-Remix-Revalidate")) {
isRevalidationRequired = true;
}
let location = redirect2.response.headers.get("Location");
invariant(location, "Expected a Location header on the redirect Response");
location = normalizeRedirectLocation(
location,
new URL(request.url),
basename
);
let redirectLocation = createLocation(state.location, location, {
_isRedirect: true
});
if (isBrowser2) {
let isDocumentReload = false;
if (redirect2.response.headers.has("X-Remix-Reload-Document")) {
isDocumentReload = true;
} else if (ABSOLUTE_URL_REGEX.test(location)) {
const url = init.history.createURL(location);
isDocumentReload = // Hard reload if it's an absolute URL to a new origin
url.origin !== routerWindow.location.origin || // Hard reload if it's an absolute URL that does not match our basename
stripBasename(url.pathname, basename) == null;
}
if (isDocumentReload) {
if (replace2) {
routerWindow.location.replace(location);
} else {
routerWindow.location.assign(location);
}
return;
}
}
pendingNavigationController = null;
let redirectNavigationType = replace2 === true || redirect2.response.headers.has("X-Remix-Replace") ? "REPLACE" /* Replace */ : "PUSH" /* Push */;
let { formMethod, formAction, formEncType } = state.navigation;
if (!submission && !fetcherSubmission && formMethod && formAction && formEncType) {
submission = getSubmissionFromNavigation(state.navigation);
}
let activeSubmission = submission || fetcherSubmission;
if (redirectPreserveMethodStatusCodes.has(redirect2.response.status) && activeSubmission && isMutationMethod(activeSubmission.formMethod)) {
await startNavigation(redirectNavigationType, redirectLocation, {
submission: {
...activeSubmission,
formAction: location
},
// Preserve these flags across redirects
preventScrollReset: preventScrollReset || pendingPreventScrollReset,
enableViewTransition: isNavigation ? pendingViewTransitionEnabled : void 0
});
} else {
let overrideNavigation = getLoadingNavigation(
redirectLocation,
submission
);
await startNavigation(redirectNavigationType, redirectLocation, {
overrideNavigation,
// Send fetcher submissions through for shouldRevalidate
fetcherSubmission,
// Preserve these flags across redirects
preventScrollReset: preventScrollReset || pendingPreventScrollReset,
enableViewTransition: isNavigation ? pendingViewTransitionEnabled : void 0
});
}
}
async function callDataStrategy(type, state2, request, matchesToLoad, matches, fetcherKey) {
let results;
let dataResults = {};
try {
results = await callDataStrategyImpl(
dataStrategyImpl,
type,
state2,
request,
matchesToLoad,
matches,
fetcherKey,
manifest,
mapRouteProperties2
);
} catch (e) {
matchesToLoad.forEach((m) => {
dataResults[m.route.id] = {
type: "error" /* error */,
error: e
};
});
return dataResults;
}
for (let [routeId, result] of Object.entries(results)) {
if (isRedirectDataStrategyResult(result)) {
let response = result.result;
dataResults[routeId] = {
type: "redirect" /* redirect */,
response: normalizeRelativeRoutingRedirectResponse(
response,
request,
routeId,
matches,
basename
)
};
} else {
dataResults[routeId] = await convertDataStrategyResultToDataResult(
result
);
}
}
return dataResults;
}
async function callLoadersAndMaybeResolveData(state2, matches, matchesToLoad, fetchersToLoad, request) {
let loaderResultsPromise = callDataStrategy(
"loader",
state2,
request,
matchesToLoad,
matches,
null
);
let fetcherResultsPromise = Promise.all(
fetchersToLoad.map(async (f) => {
if (f.matches && f.match && f.controller) {
let results = await callDataStrategy(
"loader",
state2,
createClientSideRequest(init.history, f.path, f.controller.signal),
[f.match],
f.matches,
f.key
);
let result = results[f.match.route.id];
return { [f.key]: result };
} else {
return Promise.resolve({
[f.key]: {
type: "error" /* error */,
error: getInternalRouterError(404, {
pathname: f.path
})
}
});
}
})
);
let loaderResults = await loaderResultsPromise;
let fetcherResults = (await fetcherResultsPromise).reduce(
(acc, r) => Object.assign(acc, r),
{}
);
return {
loaderResults,
fetcherResults
};
}
function interruptActiveLoads() {
isRevalidationRequired = true;
fetchLoadMatches.forEach((_, key) => {
if (fetchControllers.has(key)) {
cancelledFetcherLoads.add(key);
}
abortFetcher(key);
});
}
function updateFetcherState(key, fetcher, opts = {}) {
state.fetchers.set(key, fetcher);
updateState(
{ fetchers: new Map(state.fetchers) },
{ flushSync: (opts && opts.flushSync) === true }
);
}
function setFetcherError(key, routeId, error, opts = {}) {
let boundaryMatch = findNearestBoundary(state.matches, routeId);
deleteFetcher(key);
updateState(
{
errors: {
[boundaryMatch.route.id]: error
},
fetchers: new Map(state.fetchers)
},
{ flushSync: (opts && opts.flushSync) === true }
);
}
function getFetcher(key) {
activeFetchers.set(key, (activeFetchers.get(key) || 0) + 1);
if (fetchersQueuedForDeletion.has(key)) {
fetchersQueuedForDeletion.delete(key);
}
return state.fetchers.get(key) || IDLE_FETCHER;
}
function deleteFetcher(key) {
let fetcher = state.fetchers.get(key);
if (fetchControllers.has(key) && !(fetcher && fetcher.state === "loading" && fetchReloadIds.has(key))) {
abortFetcher(key);
}
fetchLoadMatches.delete(key);
fetchReloadIds.delete(key);
fetchRedirectIds.delete(key);
fetchersQueuedForDeletion.delete(key);
cancelledFetcherLoads.delete(key);
state.fetchers.delete(key);
}
function queueFetcherForDeletion(key) {
let count = (activeFetchers.get(key) || 0) - 1;
if (count <= 0) {
activeFetchers.delete(key);
fetchersQueuedForDeletion.add(key);
} else {
activeFetchers.set(key, count);
}
updateState({ fetchers: new Map(state.fetchers) });
}
function abortFetcher(key) {
let controller = fetchControllers.get(key);
if (controller) {
controller.abort();
fetchControllers.delete(key);
}
}
function markFetchersDone(keys) {
for (let key of keys) {
let fetcher = getFetcher(key);
let doneFetcher = getDoneFetcher(fetcher.data);
state.fetchers.set(key, doneFetcher);
}
}
function markFetchRedirectsDone() {
let doneKeys = [];
let updatedFetchers = false;
for (let key of fetchRedirectIds) {
let fetcher = state.fetchers.get(key);
invariant(fetcher, `Expected fetcher: ${key}`);
if (fetcher.state === "loading") {
fetchRedirectIds.delete(key);
doneKeys.push(key);
updatedFetchers = true;
}
}
markFetchersDone(doneKeys);
return updatedFetchers;
}
function abortStaleFetchLoads(landedId) {
let yeetedKeys = [];
for (let [key, id] of fetchReloadIds) {
if (id < landedId) {
let fetcher = state.fetchers.get(key);
invariant(fetcher, `Expected fetcher: ${key}`);
if (fetcher.state === "loading") {
abortFetcher(key);
fetchReloadIds.delete(key);
yeetedKeys.push(key);
}
}
}
markFetchersDone(yeetedKeys);
return yeetedKeys.length > 0;
}
function getBlocker(key, fn) {
let blocker = state.blockers.get(key) || IDLE_BLOCKER;
if (blockerFunctions.get(key) !== fn) {
blockerFunctions.set(key, fn);
}
return blocker;
}
function deleteBlocker(key) {
state.blockers.delete(key);
blockerFunctions.delete(key);
}
function updateBlocker(key, newBlocker) {
let blocker = state.blockers.get(key) || IDLE_BLOCKER;
invariant(
blocker.state === "unblocked" && newBlocker.state === "blocked" || blocker.state === "blocked" && newBlocker.state === "blocked" || blocker.state === "blocked" && newBlocker.state === "proceeding" || blocker.state === "blocked" && newBlocker.state === "unblocked" || blocker.state === "proceeding" && newBlocker.state === "unblocked",
`Invalid blocker state transition: ${blocker.state} -> ${newBlocker.state}`
);
let blockers = new Map(state.blockers);
blockers.set(key, newBlocker);
updateState({ blockers });
}
function shouldBlockNavigation({
currentLocation,
nextLocation,
historyAction
}) {
if (blockerFunctions.size === 0) {
return;
}
if (blockerFunctions.size > 1) {
warning(false, "A router only supports one blocker at a time");
}
let entries = Array.from(blockerFunctions.entries());
let [blockerKey, blockerFunction] = entries[entries.length - 1];
let blocker = state.blockers.get(blockerKey);
if (blocker && blocker.state === "proceeding") {
return;
}
if (blockerFunction({ currentLocation, nextLocation, historyAction })) {
return blockerKey;
}
}
function handleNavigational404(pathname) {
let error = getInternalRouterError(404, { pathname });
let routesToUse = inFlightDataRoutes || dataRoutes;
let { matches, route } = getShortCircuitMatches(routesToUse);
return { notFoundMatches: matches, route, error };
}
function enableScrollRestoration(positions, getPosition, getKey) {
savedScrollPositions2 = positions;
getScrollPosition = getPosition;
getScrollRestorationKey2 = getKey || null;
if (!initialScrollRestored && state.navigation === IDLE_NAVIGATION) {
initialScrollRestored = true;
let y = getSavedScrollPosition(state.location, state.matches);
if (y != null) {
updateState({ restoreScrollPosition: y });
}
}
return () => {
savedScrollPositions2 = null;
getScrollPosition = null;
getScrollRestorationKey2 = null;
};
}
function getScrollKey(location, matches) {
if (getScrollRestorationKey2) {
let key = getScrollRestorationKey2(
location,
matches.map((m) => convertRouteMatchToUiMatch(m, state.loaderData))
);
return key || location.key;
}
return location.key;
}
function saveScrollPosition(location, matches) {
if (savedScrollPositions2 && getScrollPosition) {
let key = getScrollKey(location, matches);
savedScrollPositions2[key] = getScrollPosition();
}
}
function getSavedScrollPosition(location, matches) {
if (savedScrollPositions2) {
let key = getScrollKey(location, matches);
let y = savedScrollPositions2[key];
if (typeof y === "number") {
return y;
}
}
return null;
}
function checkFogOfWar(matches, routesToUse, pathname) {
if (patchRoutesOnNavigationImpl) {
if (!matches) {
let fogMatches = matchRoutesImpl(
routesToUse,
pathname,
basename,
true
);
return { active: true, matches: fogMatches || [] };
} else {
if (Object.keys(matches[0].params).length > 0) {
let partialMatches = matchRoutesImpl(
routesToUse,
pathname,
basename,
true
);
return { active: true, matches: partialMatches };
}
}
}
return { active: false, matches: null };
}
async function discoverRoutes(matches, pathname, signal) {
if (!patchRoutesOnNavigationImpl) {
return { type: "success", matches };
}
let partialMatches = matches;
while (true) {
let isNonHMR = inFlightDataRoutes == null;
let routesToUse = inFlightDataRoutes || dataRoutes;
let localManifest = manifest;
try {
await patchRoutesOnNavigationImpl({
path: pathname,
matches: partialMatches,
patch: (routeId, children) => {
if (signal.aborted) return;
patchRoutesImpl(
routeId,
children,
routesToUse,
localManifest,
mapRouteProperties2
);
}
});
} catch (e) {
return { type: "error", error: e, partialMatches };
} finally {
if (isNonHMR && !signal.aborted) {
dataRoutes = [...dataRoutes];
}
}
if (signal.aborted) {
return { type: "aborted" };
}
let newMatches = matchRoutes(routesToUse, pathname, basename);
if (newMatches) {
return { type: "success", matches: newMatches };
}
let newPartialMatches = matchRoutesImpl(
routesToUse,
pathname,
basename,
true
);
if (!newPartialMatches || partialMatches.length === newPartialMatches.length && partialMatches.every(
(m, i) => m.route.id === newPartialMatches[i].route.id
)) {
return { type: "success", matches: null };
}
partialMatches = newPartialMatches;
}
}
function _internalSetRoutes(newRoutes) {
manifest = {};
inFlightDataRoutes = convertRoutesToDataRoutes(
newRoutes,
mapRouteProperties2,
void 0,
manifest
);
}
function patchRoutes(routeId, children) {
let isNonHMR = inFlightDataRoutes == null;
let routesToUse = inFlightDataRoutes || dataRoutes;
patchRoutesImpl(
routeId,
children,
routesToUse,
manifest,
mapRouteProperties2
);
if (isNonHMR) {
dataRoutes = [...dataRoutes];
updateState({});
}
}
router = {
get basename() {
return basename;
},
get future() {
return future;
},
get state() {
return state;
},
get routes() {
return dataRoutes;
},
get window() {
return routerWindow;
},
initialize,
subscribe,
enableScrollRestoration,
navigate,
fetch: fetch2,
revalidate,
// Passthrough to history-aware createHref used by useHref so we get proper
// hash-aware URLs in DOM paths
createHref: (to) => init.history.createHref(to),
encodeLocation: (to) => init.history.encodeLocation(to),
getFetcher,
deleteFetcher: queueFetcherForDeletion,
dispose,
getBlocker,
deleteBlocker,
patchRoutes,
_internalFetchControllers: fetchControllers,
// TODO: Remove setRoutes, it's temporary to avoid dealing with
// updating the tree while validating the update algorithm.
_internalSetRoutes
};
return router;
}
function createStaticHandler(routes, opts) {
invariant(
routes.length > 0,
"You must provide a non-empty routes array to createStaticHandler"
);
let manifest = {};
let basename = (opts ? opts.basename : null) || "/";
let mapRouteProperties2 = opts?.mapRouteProperties || defaultMapRouteProperties;
let dataRoutes = convertRoutesToDataRoutes(
routes,
mapRouteProperties2,
void 0,
manifest
);
async function query(request, {
requestContext,
skipLoaderErrorBubbling,
dataStrategy
} = {}) {
let url = new URL(request.url);
let method = request.method;
let location = createLocation("", createPath(url), null, "default");
let matches = matchRoutes(dataRoutes, location, basename);
if (!isValidMethod(method) && method !== "HEAD") {
let error = getInternalRouterError(405, { method });
let { matches: methodNotAllowedMatches, route } = getShortCircuitMatches(dataRoutes);
return {
basename,
location,
matches: methodNotAllowedMatches,
loaderData: {},
actionData: null,
errors: {
[route.id]: error
},
statusCode: error.status,
loaderHeaders: {},
actionHeaders: {}
};
} else if (!matches) {
let error = getInternalRouterError(404, { pathname: location.pathname });
let { matches: notFoundMatches, route } = getShortCircuitMatches(dataRoutes);
return {
basename,
location,
matches: notFoundMatches,
loaderData: {},
actionData: null,
errors: {
[route.id]: error
},
statusCode: error.status,
loaderHeaders: {},
actionHeaders: {}
};
}
let result = await queryImpl(
request,
location,
matches,
requestContext,
dataStrategy || null,
skipLoaderErrorBubbling === true,
null
);
if (isResponse(result)) {
return result;
}
return { location, basename, ...result };
}
async function queryRoute(request, {
routeId,
requestContext,
dataStrategy
} = {}) {
let url = new URL(request.url);
let method = request.method;
let location = createLocation("", createPath(url), null, "default");
let matches = matchRoutes(dataRoutes, location, basename);
if (!isValidMethod(method) && method !== "HEAD" && method !== "OPTIONS") {
throw getInternalRouterError(405, { method });
} else if (!matches) {
throw getInternalRouterError(404, { pathname: location.pathname });
}
let match = routeId ? matches.find((m) => m.route.id === routeId) : getTargetMatch(matches, location);
if (routeId && !match) {
throw getInternalRouterError(403, {
pathname: location.pathname,
routeId
});
} else if (!match) {
throw getInternalRouterError(404, { pathname: location.pathname });
}
let result = await queryImpl(
request,
location,
matches,
requestContext,
dataStrategy || null,
false,
match
);
if (isResponse(result)) {
return result;
}
let error = result.errors ? Object.values(result.errors)[0] : void 0;
if (error !== void 0) {
throw error;
}
if (result.actionData) {
return Object.values(result.actionData)[0];
}
if (result.loaderData) {
return Object.values(result.loaderData)[0];
}
return void 0;
}
async function queryImpl(request, location, matches, requestContext, dataStrategy, skipLoaderErrorBubbling, routeMatch) {
invariant(
request.signal,
"query()/queryRoute() requests must contain an AbortController signal"
);
try {
if (isMutationMethod(request.method)) {
let result2 = await submit(
request,
matches,
routeMatch || getTargetMatch(matches, location),
requestContext,
dataStrategy,
skipLoaderErrorBubbling,
routeMatch != null
);
return result2;
}
let result = await loadRouteData(
request,
matches,
requestContext,
dataStrategy,
skipLoaderErrorBubbling,
routeMatch
);
return isResponse(result) ? result : {
...result,
actionData: null,
actionHeaders: {}
};
} catch (e) {
if (isDataStrategyResult(e) && isResponse(e.result)) {
if (e.type === "error" /* error */) {
throw e.result;
}
return e.result;
}
if (isRedirectResponse(e)) {
return e;
}
throw e;
}
}
async function submit(request, matches, actionMatch, requestContext, dataStrategy, skipLoaderErrorBubbling, isRouteRequest) {
let result;
if (!actionMatch.route.action && !actionMatch.route.lazy) {
let error = getInternalRouterError(405, {
method: request.method,
pathname: new URL(request.url).pathname,
routeId: actionMatch.route.id
});
if (isRouteRequest) {
throw error;
}
result = {
type: "error" /* error */,
error
};
} else {
let results = await callDataStrategy(
"action",
request,
[actionMatch],
matches,
isRouteRequest,
requestContext,
dataStrategy
);
result = results[actionMatch.route.id];
if (request.signal.aborted) {
throwStaticHandlerAbortedError(request, isRouteRequest);
}
}
if (isRedirectResult(result)) {
throw new Response(null, {
status: result.response.status,
headers: {
Location: result.response.headers.get("Location")
}
});
}
if (isRouteRequest) {
if (isErrorResult(result)) {
throw result.error;
}
return {
matches: [actionMatch],
loaderData: {},
actionData: { [actionMatch.route.id]: result.data },
errors: null,
// Note: statusCode + headers are unused here since queryRoute will
// return the raw Response or value
statusCode: 200,
loaderHeaders: {},
actionHeaders: {}
};
}
let loaderRequest = new Request(request.url, {
headers: request.headers,
redirect: request.redirect,
signal: request.signal
});
if (isErrorResult(result)) {
let boundaryMatch = skipLoaderErrorBubbling ? actionMatch : findNearestBoundary(matches, actionMatch.route.id);
let context2 = await loadRouteData(
loaderRequest,
matches,
requestContext,
dataStrategy,
skipLoaderErrorBubbling,
null,
[boundaryMatch.route.id, result]
);
return {
...context2,
statusCode: isRouteErrorResponse(result.error) ? result.error.status : result.statusCode != null ? result.statusCode : 500,
actionData: null,
actionHeaders: {
...result.headers ? { [actionMatch.route.id]: result.headers } : {}
}
};
}
let context = await loadRouteData(
loaderRequest,
matches,
requestContext,
dataStrategy,
skipLoaderErrorBubbling,
null
);
return {
...context,
actionData: {
[actionMatch.route.id]: result.data
},
// action status codes take precedence over loader status codes
...result.statusCode ? { statusCode: result.statusCode } : {},
actionHeaders: result.headers ? { [actionMatch.route.id]: result.headers } : {}
};
}
async function loadRouteData(request, matches, requestContext, dataStrategy, skipLoaderErrorBubbling, routeMatch, pendingActionResult) {
let isRouteRequest = routeMatch != null;
if (isRouteRequest && !routeMatch?.route.loader && !routeMatch?.route.lazy) {
throw getInternalRouterError(400, {
method: request.method,
pathname: new URL(request.url).pathname,
routeId: routeMatch?.route.id
});
}
let requestMatches = routeMatch ? [routeMatch] : pendingActionResult && isErrorResult(pendingActionResult[1]) ? getLoaderMatchesUntilBoundary(matches, pendingActionResult[0]) : matches;
let matchesToLoad = requestMatches.filter(
(m) => m.route.loader || m.route.lazy
);
if (matchesToLoad.length === 0) {
return {
matches,
// Add a null for all matched routes for proper revalidation on the client
loaderData: matches.reduce(
(acc, m) => Object.assign(acc, { [m.route.id]: null }),
{}
),
errors: pendingActionResult && isErrorResult(pendingActionResult[1]) ? {
[pendingActionResult[0]]: pendingActionResult[1].error
} : null,
statusCode: 200,
loaderHeaders: {}
};
}
let results = await callDataStrategy(
"loader",
request,
matchesToLoad,
matches,
isRouteRequest,
requestContext,
dataStrategy
);
if (request.signal.aborted) {
throwStaticHandlerAbortedError(request, isRouteRequest);
}
let context = processRouteLoaderData(
matches,
results,
pendingActionResult,
true,
skipLoaderErrorBubbling
);
let executedLoaders = new Set(
matchesToLoad.map((match) => match.route.id)
);
matches.forEach((match) => {
if (!executedLoaders.has(match.route.id)) {
context.loaderData[match.route.id] = null;
}
});
return {
...context,
matches
};
}
async function callDataStrategy(type, request, matchesToLoad, matches, isRouteRequest, requestContext, dataStrategy) {
let results = await callDataStrategyImpl(
dataStrategy || defaultDataStrategy,
type,
null,
request,
matchesToLoad,
matches,
null,
manifest,
mapRouteProperties2,
requestContext
);
let dataResults = {};
await Promise.all(
matches.map(async (match) => {
if (!(match.route.id in results)) {
return;
}
let result = results[match.route.id];
if (isRedirectDataStrategyResult(result)) {
let response = result.result;
throw normalizeRelativeRoutingRedirectResponse(
response,
request,
match.route.id,
matches,
basename
);
}
if (isResponse(result.result) && isRouteRequest) {
throw result;
}
dataResults[match.route.id] = await convertDataStrategyResultToDataResult(result);
})
);
return dataResults;
}
return {
dataRoutes,
query,
queryRoute
};
}
function getStaticContextFromError(routes, context, error) {
let newContext = {
...context,
statusCode: isRouteErrorResponse(error) ? error.status : 500,
errors: {
[context._deepestRenderedBoundaryId || routes[0].id]: error
}
};
return newContext;
}
function throwStaticHandlerAbortedError(request, isRouteRequest) {
if (request.signal.reason !== void 0) {
throw request.signal.reason;
}
let method = isRouteRequest ? "queryRoute" : "query";
throw new Error(
`${method}() call aborted without an \`AbortSignal.reason\`: ${request.method} ${request.url}`
);
}
function isSubmissionNavigation(opts) {
return opts != null && ("formData" in opts && opts.formData != null || "body" in opts && opts.body !== void 0);
}
function normalizeTo(location, matches, basename, to, fromRouteId, relative) {
let contextualMatches;
let activeRouteMatch;
if (fromRouteId) {
contextualMatches = [];
for (let match of matches) {
contextualMatches.push(match);
if (match.route.id === fromRouteId) {
activeRouteMatch = match;
break;
}
}
} else {
contextualMatches = matches;
activeRouteMatch = matches[matches.length - 1];
}
let path = resolveTo(
to ? to : ".",
getResolveToMatches(contextualMatches),
stripBasename(location.pathname, basename) || location.pathname,
relative === "path"
);
if (to == null) {
path.search = location.search;
path.hash = location.hash;
}
if ((to == null || to === "" || to === ".") && activeRouteMatch) {
let nakedIndex = hasNakedIndexQuery(path.search);
if (activeRouteMatch.route.index && !nakedIndex) {
path.search = path.search ? path.search.replace(/^\?/, "?index&") : "?index";
} else if (!activeRouteMatch.route.index && nakedIndex) {
let params = new URLSearchParams(path.search);
let indexValues = params.getAll("index");
params.delete("index");
indexValues.filter((v) => v).forEach((v) => params.append("index", v));
let qs = params.toString();
path.search = qs ? `?${qs}` : "";
}
}
if (basename !== "/") {
path.pathname = path.pathname === "/" ? basename : joinPaths([basename, path.pathname]);
}
return createPath(path);
}
function normalizeNavigateOptions(isFetcher, path, opts) {
if (!opts || !isSubmissionNavigation(opts)) {
return { path };
}
if (opts.formMethod && !isValidMethod(opts.formMethod)) {
return {
path,
error: getInternalRouterError(405, { method: opts.formMethod })
};
}
let getInvalidBodyError = () => ({
path,
error: getInternalRouterError(400, { type: "invalid-body" })
});
let rawFormMethod = opts.formMethod || "get";
let formMethod = rawFormMethod.toUpperCase();
let formAction = stripHashFromPath(path);
if (opts.body !== void 0) {
if (opts.formEncType === "text/plain") {
if (!isMutationMethod(formMethod)) {
return getInvalidBodyError();
}
let text = typeof opts.body === "string" ? opts.body : opts.body instanceof FormData || opts.body instanceof URLSearchParams ? (
// https://html.spec.whatwg.org/multipage/form-control-infrastructure.html#plain-text-form-data
Array.from(opts.body.entries()).reduce(
(acc, [name, value]) => `${acc}${name}=${value}
`,
""
)
) : String(opts.body);
return {
path,
submission: {
formMethod,
formAction,
formEncType: opts.formEncType,
formData: void 0,
json: void 0,
text
}
};
} else if (opts.formEncType === "application/json") {
if (!isMutationMethod(formMethod)) {
return getInvalidBodyError();
}
try {
let json = typeof opts.body === "string" ? JSON.parse(opts.body) : opts.body;
return {
path,
submission: {
formMethod,
formAction,
formEncType: opts.formEncType,
formData: void 0,
json,
text: void 0
}
};
} catch (e) {
return getInvalidBodyError();
}
}
}
invariant(
typeof FormData === "function",
"FormData is not available in this environment"
);
let searchParams;
let formData;
if (opts.formData) {
searchParams = convertFormDataToSearchParams(opts.formData);
formData = opts.formData;
} else if (opts.body instanceof FormData) {
searchParams = convertFormDataToSearchParams(opts.body);
formData = opts.body;
} else if (opts.body instanceof URLSearchParams) {
searchParams = opts.body;
formData = convertSearchParamsToFormData(searchParams);
} else if (opts.body == null) {
searchParams = new URLSearchParams();
formData = new FormData();
} else {
try {
searchParams = new URLSearchParams(opts.body);
formData = convertSearchParamsToFormData(searchParams);
} catch (e) {
return getInvalidBodyError();
}
}
let submission = {
formMethod,
formAction,
formEncType: opts && opts.formEncType || "application/x-www-form-urlencoded",
formData,
json: void 0,
text: void 0
};
if (isMutationMethod(submission.formMethod)) {
return { path, submission };
}
let parsedPath = parsePath(path);
if (isFetcher && parsedPath.search && hasNakedIndexQuery(parsedPath.search)) {
searchParams.append("index", "");
}
parsedPath.search = `?${searchParams}`;
return { path: createPath(parsedPath), submission };
}
function getLoaderMatchesUntilBoundary(matches, boundaryId, includeBoundary = false) {
let index = matches.findIndex((m) => m.route.id === boundaryId);
if (index >= 0) {
return matches.slice(0, includeBoundary ? index + 1 : index);
}
return matches;
}
function getMatchesToLoad(history, state, matches, submission, location, initialHydration, isRevalidationRequired, cancelledFetcherLoads, fetchersQueuedForDeletion, fetchLoadMatches, fetchRedirectIds, routesToUse, basename, pendingActionResult) {
let actionResult = pendingActionResult ? isErrorResult(pendingActionResult[1]) ? pendingActionResult[1].error : pendingActionResult[1].data : void 0;
let currentUrl = history.createURL(state.location);
let nextUrl = history.createURL(location);
let boundaryMatches = matches;
if (initialHydration && state.errors) {
boundaryMatches = getLoaderMatchesUntilBoundary(
matches,
Object.keys(state.errors)[0],
true
);
} else if (pendingActionResult && isErrorResult(pendingActionResult[1])) {
boundaryMatches = getLoaderMatchesUntilBoundary(
matches,
pendingActionResult[0]
);
}
let actionStatus = pendingActionResult ? pendingActionResult[1].statusCode : void 0;
let shouldSkipRevalidation = actionStatus && actionStatus >= 400;
let navigationMatches = boundaryMatches.filter((match, index) => {
let { route } = match;
if (route.lazy) {
return true;
}
if (route.loader == null) {
return false;
}
if (initialHydration) {
return shouldLoadRouteOnHydration(route, state.loaderData, state.errors);
}
if (isNewLoader(state.loaderData, state.matches[index], match)) {
return true;
}
let currentRouteMatch = state.matches[index];
let nextRouteMatch = match;
return shouldRevalidateLoader(match, {
currentUrl,
currentParams: currentRouteMatch.params,
nextUrl,
nextParams: nextRouteMatch.params,
...submission,
actionResult,
actionStatus,
defaultShouldRevalidate: shouldSkipRevalidation ? false : (
// Forced revalidation due to submission, useRevalidator, or X-Remix-Revalidate
isRevalidationRequired || currentUrl.pathname + currentUrl.search === nextUrl.pathname + nextUrl.search || // Search params affect all loaders
currentUrl.search !== nextUrl.search || isNewRouteInstance(currentRouteMatch, nextRouteMatch)
)
});
});
let revalidatingFetchers = [];
fetchLoadMatches.forEach((f, key) => {
if (initialHydration || !matches.some((m) => m.route.id === f.routeId) || fetchersQueuedForDeletion.has(key)) {
return;
}
let fetcherMatches = matchRoutes(routesToUse, f.path, basename);
if (!fetcherMatches) {
revalidatingFetchers.push({
key,
routeId: f.routeId,
path: f.path,
matches: null,
match: null,
controller: null
});
return;
}
let fetcher = state.fetchers.get(key);
let fetcherMatch = getTargetMatch(fetcherMatches, f.path);
let shouldRevalidate = false;
if (fetchRedirectIds.has(key)) {
shouldRevalidate = false;
} else if (cancelledFetcherLoads.has(key)) {
cancelledFetcherLoads.delete(key);
shouldRevalidate = true;
} else if (fetcher && fetcher.state !== "idle" && fetcher.data === void 0) {
shouldRevalidate = isRevalidationRequired;
} else {
shouldRevalidate = shouldRevalidateLoader(fetcherMatch, {
currentUrl,
currentParams: state.matches[state.matches.length - 1].params,
nextUrl,
nextParams: matches[matches.length - 1].params,
...submission,
actionResult,
actionStatus,
defaultShouldRevalidate: shouldSkipRevalidation ? false : isRevalidationRequired
});
}
if (shouldRevalidate) {
revalidatingFetchers.push({
key,
routeId: f.routeId,
path: f.path,
matches: fetcherMatches,
match: fetcherMatch,
controller: new AbortController()
});
}
});
return [navigationMatches, revalidatingFetchers];
}
function shouldLoadRouteOnHydration(route, loaderData, errors) {
if (route.lazy) {
return true;
}
if (!route.loader) {
return false;
}
let hasData = loaderData != null && loaderData[route.id] !== void 0;
let hasError = errors != null && errors[route.id] !== void 0;
if (!hasData && hasError) {
return false;
}
if (typeof route.loader === "function" && route.loader.hydrate === true) {
return true;
}
return !hasData && !hasError;
}
function isNewLoader(currentLoaderData, currentMatch, match) {
let isNew = (
// [a] -> [a, b]
!currentMatch || // [a, b] -> [a, c]
match.route.id !== currentMatch.route.id
);
let isMissingData = !currentLoaderData.hasOwnProperty(match.route.id);
return isNew || isMissingData;
}
function isNewRouteInstance(currentMatch, match) {
let currentPath = currentMatch.route.path;
return (
// param change for this match, /users/123 -> /users/456
currentMatch.pathname !== match.pathname || // splat param changed, which is not present in match.path
// e.g. /files/images/avatar.jpg -> files/finances.xls
currentPath != null && currentPath.endsWith("*") && currentMatch.params["*"] !== match.params["*"]
);
}
function shouldRevalidateLoader(loaderMatch, arg) {
if (loaderMatch.route.shouldRevalidate) {
let routeChoice = loaderMatch.route.shouldRevalidate(arg);
if (typeof routeChoice === "boolean") {
return routeChoice;
}
}
return arg.defaultShouldRevalidate;
}
function patchRoutesImpl(routeId, children, routesToUse, manifest, mapRouteProperties2) {
let childrenToPatch;
if (routeId) {
let route = manifest[routeId];
invariant(
route,
`No route found to patch children into: routeId = ${routeId}`
);
if (!route.children) {
route.children = [];
}
childrenToPatch = route.children;
} else {
childrenToPatch = routesToUse;
}
let uniqueChildren = children.filter(
(newRoute) => !childrenToPatch.some(
(existingRoute) => isSameRoute(newRoute, existingRoute)
)
);
let newRoutes = convertRoutesToDataRoutes(
uniqueChildren,
mapRouteProperties2,
[routeId || "_", "patch", String(childrenToPatch?.length || "0")],
manifest
);
childrenToPatch.push(...newRoutes);
}
function isSameRoute(newRoute, existingRoute) {
if ("id" in newRoute && "id" in existingRoute && newRoute.id === existingRoute.id) {
return true;
}
if (!(newRoute.index === existingRoute.index && newRoute.path === existingRoute.path && newRoute.caseSensitive === existingRoute.caseSensitive)) {
return false;
}
if ((!newRoute.children || newRoute.children.length === 0) && (!existingRoute.children || existingRoute.children.length === 0)) {
return true;
}
return newRoute.children.every(
(aChild, i) => existingRoute.children?.some((bChild) => isSameRoute(aChild, bChild))
);
}
async function loadLazyRouteModule(route, mapRouteProperties2, manifest) {
if (!route.lazy) {
return;
}
let lazyRoute = await route.lazy();
if (!route.lazy) {
return;
}
let routeToUpdate = manifest[route.id];
invariant(routeToUpdate, "No route found in manifest");
let routeUpdates = {};
for (let lazyRouteProperty in lazyRoute) {
let staticRouteValue = routeToUpdate[lazyRouteProperty];
let isPropertyStaticallyDefined = staticRouteValue !== void 0 && // This property isn't static since it should always be updated based
// on the route updates
lazyRouteProperty !== "hasErrorBoundary";
warning(
!isPropertyStaticallyDefined,
`Route "${routeToUpdate.id}" has a static property "${lazyRouteProperty}" defined but its lazy function is also returning a value for this property. The lazy route property "${lazyRouteProperty}" will be ignored.`
);
if (!isPropertyStaticallyDefined && !immutableRouteKeys.has(lazyRouteProperty)) {
routeUpdates[lazyRouteProperty] = lazyRoute[lazyRouteProperty];
}
}
Object.assign(routeToUpdate, routeUpdates);
Object.assign(routeToUpdate, {
// To keep things framework agnostic, we use the provided `mapRouteProperties`
// function to set the framework-aware properties (`element`/`hasErrorBoundary`)
// since the logic will differ between frameworks.
...mapRouteProperties2(routeToUpdate),
lazy: void 0
});
}
async function defaultDataStrategy({
matches
}) {
let matchesToLoad = matches.filter((m) => m.shouldLoad);
let results = await Promise.all(matchesToLoad.map((m) => m.resolve()));
return results.reduce(
(acc, result, i) => Object.assign(acc, { [matchesToLoad[i].route.id]: result }),
{}
);
}
async function callDataStrategyImpl(dataStrategyImpl, type, state, request, matchesToLoad, matches, fetcherKey, manifest, mapRouteProperties2, requestContext) {
let loadRouteDefinitionsPromises = matches.map(
(m) => m.route.lazy ? loadLazyRouteModule(m.route, mapRouteProperties2, manifest) : void 0
);
let dsMatches = matches.map((match, i) => {
let loadRoutePromise = loadRouteDefinitionsPromises[i];
let shouldLoad = matchesToLoad.some((m) => m.route.id === match.route.id);
let resolve = async (handlerOverride) => {
if (handlerOverride && request.method === "GET" && (match.route.lazy || match.route.loader)) {
shouldLoad = true;
}
return shouldLoad ? callLoaderOrAction(
type,
request,
match,
loadRoutePromise,
handlerOverride,
requestContext
) : Promise.resolve({ type: "data" /* data */, result: void 0 });
};
return {
...match,
shouldLoad,
resolve
};
});
let results = await dataStrategyImpl({
matches: dsMatches,
request,
params: matches[0].params,
fetcherKey,
context: requestContext
});
try {
await Promise.all(loadRouteDefinitionsPromises);
} catch (e) {
}
return results;
}
async function callLoaderOrAction(type, request, match, loadRoutePromise, handlerOverride, staticContext) {
let result;
let onReject;
let runHandler = (handler) => {
let reject;
let abortPromise = new Promise((_, r) => reject = r);
onReject = () => reject();
request.signal.addEventListener("abort", onReject);
let actualHandler = (ctx) => {
if (typeof handler !== "function") {
return Promise.reject(
new Error(
`You cannot call the handler for a route which defines a boolean "${type}" [routeId: ${match.route.id}]`
)
);
}
return handler(
{
request,
params: match.params,
context: staticContext
},
...ctx !== void 0 ? [ctx] : []
);
};
let handlerPromise = (async () => {
try {
let val = await (handlerOverride ? handlerOverride((ctx) => actualHandler(ctx)) : actualHandler());
return { type: "data", result: val };
} catch (e) {
return { type: "error", result: e };
}
})();
return Promise.race([handlerPromise, abortPromise]);
};
try {
let handler = match.route[type];
if (loadRoutePromise) {
if (handler) {
let handlerError;
let [value] = await Promise.all([
// If the handler throws, don't let it immediately bubble out,
// since we need to let the lazy() execution finish so we know if this
// route has a boundary that can handle the error
runHandler(handler).catch((e) => {
handlerError = e;
}),
loadRoutePromise
]);
if (handlerError !== void 0) {
throw handlerError;
}
result = value;
} else {
await loadRoutePromise;
handler = match.route[type];
if (handler) {
result = await runHandler(handler);
} else if (type === "action") {
let url = new URL(request.url);
let pathname = url.pathname + url.search;
throw getInternalRouterError(405, {
method: request.method,
pathname,
routeId: match.route.id
});
} else {
return { type: "data" /* data */, result: void 0 };
}
}
} else if (!handler) {
let url = new URL(request.url);
let pathname = url.pathname + url.search;
throw getInternalRouterError(404, {
pathname
});
} else {
result = await runHandler(handler);
}
} catch (e) {
return { type: "error" /* error */, result: e };
} finally {
if (onReject) {
request.signal.removeEventListener("abort", onReject);
}
}
return result;
}
async function convertDataStrategyResultToDataResult(dataStrategyResult) {
let { result, type } = dataStrategyResult;
if (isResponse(result)) {
let data2;
try {
let contentType = result.headers.get("Content-Type");
if (contentType && /\bapplication\/json\b/.test(contentType)) {
if (result.body == null) {
data2 = null;
} else {
data2 = await result.json();
}
} else {
data2 = await result.text();
}
} catch (e) {
return { type: "error" /* error */, error: e };
}
if (type === "error" /* error */) {
return {
type: "error" /* error */,
error: new ErrorResponseImpl(result.status, result.statusText, data2),
statusCode: result.status,
headers: result.headers
};
}
return {
type: "data" /* data */,
data: data2,
statusCode: result.status,
headers: result.headers
};
}
if (type === "error" /* error */) {
if (isDataWithResponseInit(result)) {
if (result.data instanceof Error) {
return {
type: "error" /* error */,
error: result.data,
statusCode: result.init?.status
};
}
result = new ErrorResponseImpl(
result.init?.status || 500,
void 0,
result.data
);
}
return {
type: "error" /* error */,
error: result,
statusCode: isRouteErrorResponse(result) ? result.status : void 0
};
}
if (isDataWithResponseInit(result)) {
return {
type: "data" /* data */,
data: result.data,
statusCode: result.init?.status,
headers: result.init?.headers ? new Headers(result.init.headers) : void 0
};
}
return { type: "data" /* data */, data: result };
}
function normalizeRelativeRoutingRedirectResponse(response, request, routeId, matches, basename) {
let location = response.headers.get("Location");
invariant(
location,
"Redirects returned/thrown from loaders/actions must have a Location header"
);
if (!ABSOLUTE_URL_REGEX.test(location)) {
let trimmedMatches = matches.slice(
0,
matches.findIndex((m) => m.route.id === routeId) + 1
);
location = normalizeTo(
new URL(request.url),
trimmedMatches,
basename,
location
);
response.headers.set("Location", location);
}
return response;
}
function normalizeRedirectLocation(location, currentUrl, basename) {
if (ABSOLUTE_URL_REGEX.test(location)) {
let normalizedLocation = location;
let url = normalizedLocation.startsWith("//") ? new URL(currentUrl.protocol + normalizedLocation) : new URL(normalizedLocation);
let isSameBasename = stripBasename(url.pathname, basename) != null;
if (url.origin === currentUrl.origin && isSameBasename) {
return url.pathname + url.search + url.hash;
}
}
return location;
}
function createClientSideRequest(history, location, signal, submission) {
let url = history.createURL(stripHashFromPath(location)).toString();
let init = { signal };
if (submission && isMutationMethod(submission.formMethod)) {
let { formMethod, formEncType } = submission;
init.method = formMethod.toUpperCase();
if (formEncType === "application/json") {
init.headers = new Headers({ "Content-Type": formEncType });
init.body = JSON.stringify(submission.json);
} else if (formEncType === "text/plain") {
init.body = submission.text;
} else if (formEncType === "application/x-www-form-urlencoded" && submission.formData) {
init.body = convertFormDataToSearchParams(submission.formData);
} else {
init.body = submission.formData;
}
}
return new Request(url, init);
}
function convertFormDataToSearchParams(formData) {
let searchParams = new URLSearchParams();
for (let [key, value] of formData.entries()) {
searchParams.append(key, typeof value === "string" ? value : value.name);
}
return searchParams;
}
function convertSearchParamsToFormData(searchParams) {
let formData = new FormData();
for (let [key, value] of searchParams.entries()) {
formData.append(key, value);
}
return formData;
}
function processRouteLoaderData(matches, results, pendingActionResult, isStaticHandler = false, skipLoaderErrorBubbling = false) {
let loaderData = {};
let errors = null;
let statusCode;
let foundError = false;
let loaderHeaders = {};
let pendingError = pendingActionResult && isErrorResult(pendingActionResult[1]) ? pendingActionResult[1].error : void 0;
matches.forEach((match) => {
if (!(match.route.id in results)) {
return;
}
let id = match.route.id;
let result = results[id];
invariant(
!isRedirectResult(result),
"Cannot handle redirect results in processLoaderData"
);
if (isErrorResult(result)) {
let error = result.error;
if (pendingError !== void 0) {
error = pendingError;
pendingError = void 0;
}
errors = errors || {};
if (skipLoaderErrorBubbling) {
errors[id] = error;
} else {
let boundaryMatch = findNearestBoundary(matches, id);
if (errors[boundaryMatch.route.id] == null) {
errors[boundaryMatch.route.id] = error;
}
}
if (!isStaticHandler) {
loaderData[id] = ResetLoaderDataSymbol;
}
if (!foundError) {
foundError = true;
statusCode = isRouteErrorResponse(result.error) ? result.error.status : 500;
}
if (result.headers) {
loaderHeaders[id] = result.headers;
}
} else {
loaderData[id] = result.data;
if (result.statusCode && result.statusCode !== 200 && !foundError) {
statusCode = result.statusCode;
}
if (result.headers) {
loaderHeaders[id] = result.headers;
}
}
});
if (pendingError !== void 0 && pendingActionResult) {
errors = { [pendingActionResult[0]]: pendingError };
loaderData[pendingActionResult[0]] = void 0;
}
return {
loaderData,
errors,
statusCode: statusCode || 200,
loaderHeaders
};
}
function processLoaderData(state, matches, results, pendingActionResult, revalidatingFetchers, fetcherResults) {
let { loaderData, errors } = processRouteLoaderData(
matches,
results,
pendingActionResult
);
revalidatingFetchers.forEach((rf) => {
let { key, match, controller } = rf;
let result = fetcherResults[key];
invariant(result, "Did not find corresponding fetcher result");
if (controller && controller.signal.aborted) {
return;
} else if (isErrorResult(result)) {
let boundaryMatch = findNearestBoundary(state.matches, match?.route.id);
if (!(errors && errors[boundaryMatch.route.id])) {
errors = {
...errors,
[boundaryMatch.route.id]: result.error
};
}
state.fetchers.delete(key);
} else if (isRedirectResult(result)) {
invariant(false, "Unhandled fetcher revalidation redirect");
} else {
let doneFetcher = getDoneFetcher(result.data);
state.fetchers.set(key, doneFetcher);
}
});
return { loaderData, errors };
}
function mergeLoaderData(loaderData, newLoaderData, matches, errors) {
let mergedLoaderData = Object.entries(newLoaderData).filter(([, v]) => v !== ResetLoaderDataSymbol).reduce((merged, [k, v]) => {
merged[k] = v;
return merged;
}, {});
for (let match of matches) {
let id = match.route.id;
if (!newLoaderData.hasOwnProperty(id) && loaderData.hasOwnProperty(id) && match.route.loader) {
mergedLoaderData[id] = loaderData[id];
}
if (errors && errors.hasOwnProperty(id)) {
break;
}
}
return mergedLoaderData;
}
function getActionDataForCommit(pendingActionResult) {
if (!pendingActionResult) {
return {};
}
return isErrorResult(pendingActionResult[1]) ? {
// Clear out prior actionData on errors
actionData: {}
} : {
actionData: {
[pendingActionResult[0]]: pendingActionResult[1].data
}
};
}
function findNearestBoundary(matches, routeId) {
let eligibleMatches = routeId ? matches.slice(0, matches.findIndex((m) => m.route.id === routeId) + 1) : [...matches];
return eligibleMatches.reverse().find((m) => m.route.hasErrorBoundary === true) || matches[0];
}
function getShortCircuitMatches(routes) {
let route = routes.length === 1 ? routes[0] : routes.find((r) => r.index || !r.path || r.path === "/") || {
id: `__shim-error-route__`
};
return {
matches: [
{
params: {},
pathname: "",
pathnameBase: "",
route
}
],
route
};
}
function getInternalRouterError(status, {
pathname,
routeId,
method,
type,
message
} = {}) {
let statusText = "Unknown Server Error";
let errorMessage = "Unknown @remix-run/router error";
if (status === 400) {
statusText = "Bad Request";
if (method && pathname && routeId) {
errorMessage = `You made a ${method} request to "${pathname}" but did not provide a \`loader\` for route "${routeId}", so there is no way to handle the request.`;
} else if (type === "invalid-body") {
errorMessage = "Unable to encode submission body";
}
} else if (status === 403) {
statusText = "Forbidden";
errorMessage = `Route "${routeId}" does not match URL "${pathname}"`;
} else if (status === 404) {
statusText = "Not Found";
errorMessage = `No route matches URL "${pathname}"`;
} else if (status === 405) {
statusText = "Method Not Allowed";
if (method && pathname && routeId) {
errorMessage = `You made a ${method.toUpperCase()} request to "${pathname}" but did not provide an \`action\` for route "${routeId}", so there is no way to handle the request.`;
} else if (method) {
errorMessage = `Invalid request method "${method.toUpperCase()}"`;
}
}
return new ErrorResponseImpl(
status || 500,
statusText,
new Error(errorMessage),
true
);
}
function findRedirect(results) {
let entries = Object.entries(results);
for (let i = entries.length - 1; i >= 0; i--) {
let [key, result] = entries[i];
if (isRedirectResult(result)) {
return { key, result };
}
}
}
function stripHashFromPath(path) {
let parsedPath = typeof path === "string" ? parsePath(path) : path;
return createPath({ ...parsedPath, hash: "" });
}
function isHashChangeOnly(a, b) {
if (a.pathname !== b.pathname || a.search !== b.search) {
return false;
}
if (a.hash === "") {
return b.hash !== "";
} else if (a.hash === b.hash) {
return true;
} else if (b.hash !== "") {
return true;
}
return false;
}
function isDataStrategyResult(result) {
return result != null && typeof result === "object" && "type" in result && "result" in result && (result.type === "data" /* data */ || result.type === "error" /* error */);
}
function isRedirectDataStrategyResult(result) {
return isResponse(result.result) && redirectStatusCodes.has(result.result.status);
}
function isErrorResult(result) {
return result.type === "error" /* error */;
}
function isRedirectResult(result) {
return (result && result.type) === "redirect" /* redirect */;
}
function isDataWithResponseInit(value) {
return typeof value === "object" && value != null && "type" in value && "data" in value && "init" in value && value.type === "DataWithResponseInit";
}
function isResponse(value) {
return value != null && typeof value.status === "number" && typeof value.statusText === "string" && typeof value.headers === "object" && typeof value.body !== "undefined";
}
function isRedirectStatusCode(statusCode) {
return redirectStatusCodes.has(statusCode);
}
function isRedirectResponse(result) {
return isResponse(result) && isRedirectStatusCode(result.status) && result.headers.has("Location");
}
function isValidMethod(method) {
return validRequestMethods.has(method.toUpperCase());
}
function isMutationMethod(method) {
return validMutationMethods.has(method.toUpperCase());
}
function hasNakedIndexQuery(search) {
return new URLSearchParams(search).getAll("index").some((v) => v === "");
}
function getTargetMatch(matches, location) {
let search = typeof location === "string" ? parsePath(location).search : location.search;
if (matches[matches.length - 1].route.index && hasNakedIndexQuery(search || "")) {
return matches[matches.length - 1];
}
let pathMatches = getPathContributingMatches(matches);
return pathMatches[pathMatches.length - 1];
}
function getSubmissionFromNavigation(navigation) {
let { formMethod, formAction, formEncType, text, formData, json } = navigation;
if (!formMethod || !formAction || !formEncType) {
return;
}
if (text != null) {
return {
formMethod,
formAction,
formEncType,
formData: void 0,
json: void 0,
text
};
} else if (formData != null) {
return {
formMethod,
formAction,
formEncType,
formData,
json: void 0,
text: void 0
};
} else if (json !== void 0) {
return {
formMethod,
formAction,
formEncType,
formData: void 0,
json,
text: void 0
};
}
}
function getLoadingNavigation(location, submission) {
if (submission) {
let navigation = {
state: "loading",
location,
formMethod: submission.formMethod,
formAction: submission.formAction,
formEncType: submission.formEncType,
formData: submission.formData,
json: submission.json,
text: submission.text
};
return navigation;
} else {
let navigation = {
state: "loading",
location,
formMethod: void 0,
formAction: void 0,
formEncType: void 0,
formData: void 0,
json: void 0,
text: void 0
};
return navigation;
}
}
function getSubmittingNavigation(location, submission) {
let navigation = {
state: "submitting",
location,
formMethod: submission.formMethod,
formAction: submission.formAction,
formEncType: submission.formEncType,
formData: submission.formData,
json: submission.json,
text: submission.text
};
return navigation;
}
function getLoadingFetcher(submission, data2) {
if (submission) {
let fetcher = {
state: "loading",
formMethod: submission.formMethod,
formAction: submission.formAction,
formEncType: submission.formEncType,
formData: submission.formData,
json: submission.json,
text: submission.text,
data: data2
};
return fetcher;
} else {
let fetcher = {
state: "loading",
formMethod: void 0,
formAction: void 0,
formEncType: void 0,
formData: void 0,
json: void 0,
text: void 0,
data: data2
};
return fetcher;
}
}
function getSubmittingFetcher(submission, existingFetcher) {
let fetcher = {
state: "submitting",
formMethod: submission.formMethod,
formAction: submission.formAction,
formEncType: submission.formEncType,
formData: submission.formData,
json: submission.json,
text: submission.text,
data: existingFetcher ? existingFetcher.data : void 0
};
return fetcher;
}
function getDoneFetcher(data2) {
let fetcher = {
state: "idle",
formMethod: void 0,
formAction: void 0,
formEncType: void 0,
formData: void 0,
json: void 0,
text: void 0,
data: data2
};
return fetcher;
}
function restoreAppliedTransitions(_window, transitions) {
try {
let sessionPositions = _window.sessionStorage.getItem(
TRANSITIONS_STORAGE_KEY
);
if (sessionPositions) {
let json = JSON.parse(sessionPositions);
for (let [k, v] of Object.entries(json || {})) {
if (v && Array.isArray(v)) {
transitions.set(k, new Set(v || []));
}
}
}
} catch (e) {
}
}
function persistAppliedTransitions(_window, transitions) {
if (transitions.size > 0) {
let json = {};
for (let [k, v] of transitions) {
json[k] = [...v];
}
try {
_window.sessionStorage.setItem(
TRANSITIONS_STORAGE_KEY,
JSON.stringify(json)
);
} catch (error) {
warning(
false,
`Failed to save applied view transitions in sessionStorage (${error}).`
);
}
}
}
function createDeferred() {
let resolve;
let reject;
let promise = new Promise((res, rej) => {
resolve = async (val) => {
res(val);
try {
await promise;
} catch (e) {
}
};
reject = async (error) => {
rej(error);
try {
await promise;
} catch (e) {
}
};
});
return {
promise,
//@ts-ignore
resolve,
//@ts-ignore
reject
};
}
// lib/components.tsx
var React3 = __toESM(require("react"));
// lib/context.ts
var React = __toESM(require("react"));
var DataRouterContext = React.createContext(null);
DataRouterContext.displayName = "DataRouter";
var DataRouterStateContext = React.createContext(null);
DataRouterStateContext.displayName = "DataRouterState";
var ViewTransitionContext = React.createContext({
isTransitioning: false
});
ViewTransitionContext.displayName = "ViewTransition";
var FetchersContext = React.createContext(
/* @__PURE__ */ new Map()
);
FetchersContext.displayName = "Fetchers";
var AwaitContext = React.createContext(null);
AwaitContext.displayName = "Await";
var NavigationContext = React.createContext(
null
);
NavigationContext.displayName = "Navigation";
var LocationContext = React.createContext(
null
);
LocationContext.displayName = "Location";
var RouteContext = React.createContext({
outlet: null,
matches: [],
isDataRoute: false
});
RouteContext.displayName = "Route";
var RouteErrorContext = React.createContext(null);
RouteErrorContext.displayName = "RouteError";
// lib/hooks.tsx
var React2 = __toESM(require("react"));
var ENABLE_DEV_WARNINGS = true;
function useHref(to, { relative } = {}) {
invariant(
useInRouterContext(),
// TODO: This error is probably because they somehow have 2 versions of the
// router loaded. We can help them understand how to avoid that.
`useHref() may be used only in the context of a component.`
);
let { basename, navigator: navigator2 } = React2.useContext(NavigationContext);
let { hash, pathname, search } = useResolvedPath(to, { relative });
let joinedPathname = pathname;
if (basename !== "/") {
joinedPathname = pathname === "/" ? basename : joinPaths([basename, pathname]);
}
return navigator2.createHref({ pathname: joinedPathname, search, hash });
}
function useInRouterContext() {
return React2.useContext(LocationContext) != null;
}
function useLocation() {
invariant(
useInRouterContext(),
// TODO: This error is probably because they somehow have 2 versions of the
// router loaded. We can help them understand how to avoid that.
`useLocation() may be used only in the context of a component.`
);
return React2.useContext(LocationContext).location;
}
function useNavigationType() {
return React2.useContext(LocationContext).navigationType;
}
function useMatch(pattern) {
invariant(
useInRouterContext(),
// TODO: This error is probably because they somehow have 2 versions of the
// router loaded. We can help them understand how to avoid that.
`useMatch() may be used only in the context of a component.`
);
let { pathname } = useLocation();
return React2.useMemo(
() => matchPath(pattern, decodePath(pathname)),
[pathname, pattern]
);
}
var navigateEffectWarning = `You should call navigate() in a React.useEffect(), not when your component is first rendered.`;
function useIsomorphicLayoutEffect(cb) {
let isStatic = React2.useContext(NavigationContext).static;
if (!isStatic) {
React2.useLayoutEffect(cb);
}
}
function useNavigate() {
let { isDataRoute } = React2.useContext(RouteContext);
return isDataRoute ? useNavigateStable() : useNavigateUnstable();
}
function useNavigateUnstable() {
invariant(
useInRouterContext(),
// TODO: This error is probably because they somehow have 2 versions of the
// router loaded. We can help them understand how to avoid that.
`useNavigate() may be used only in the context of a component.`
);
let dataRouterContext = React2.useContext(DataRouterContext);
let { basename, navigator: navigator2 } = React2.useContext(NavigationContext);
let { matches } = React2.useContext(RouteContext);
let { pathname: locationPathname } = useLocation();
let routePathnamesJson = JSON.stringify(getResolveToMatches(matches));
let activeRef = React2.useRef(false);
useIsomorphicLayoutEffect(() => {
activeRef.current = true;
});
let navigate = React2.useCallback(
(to, options = {}) => {
warning(activeRef.current, navigateEffectWarning);
if (!activeRef.current) return;
if (typeof to === "number") {
navigator2.go(to);
return;
}
let path = resolveTo(
to,
JSON.parse(routePathnamesJson),
locationPathname,
options.relative === "path"
);
if (dataRouterContext == null && basename !== "/") {
path.pathname = path.pathname === "/" ? basename : joinPaths([basename, path.pathname]);
}
(!!options.replace ? navigator2.replace : navigator2.push)(
path,
options.state,
options
);
},
[
basename,
navigator2,
routePathnamesJson,
locationPathname,
dataRouterContext
]
);
return navigate;
}
var OutletContext = React2.createContext(null);
function useOutletContext() {
return React2.useContext(OutletContext);
}
function useOutlet(context) {
let outlet = React2.useContext(RouteContext).outlet;
if (outlet) {
return /* @__PURE__ */ React2.createElement(OutletContext.Provider, { value: context }, outlet);
}
return outlet;
}
function useParams() {
let { matches } = React2.useContext(RouteContext);
let routeMatch = matches[matches.length - 1];
return routeMatch ? routeMatch.params : {};
}
function useResolvedPath(to, { relative } = {}) {
let { matches } = React2.useContext(RouteContext);
let { pathname: locationPathname } = useLocation();
let routePathnamesJson = JSON.stringify(getResolveToMatches(matches));
return React2.useMemo(
() => resolveTo(
to,
JSON.parse(routePathnamesJson),
locationPathname,
relative === "path"
),
[to, routePathnamesJson, locationPathname, relative]
);
}
function useRoutes(routes, locationArg) {
return useRoutesImpl(routes, locationArg);
}
function useRoutesImpl(routes, locationArg, dataRouterState, future) {
invariant(
useInRouterContext(),
// TODO: This error is probably because they somehow have 2 versions of the
// router loaded. We can help them understand how to avoid that.
`useRoutes() may be used only in the context of a component.`
);
let { navigator: navigator2 } = React2.useContext(NavigationContext);
let { matches: parentMatches } = React2.useContext(RouteContext);
let routeMatch = parentMatches[parentMatches.length - 1];
let parentParams = routeMatch ? routeMatch.params : {};
let parentPathname = routeMatch ? routeMatch.pathname : "/";
let parentPathnameBase = routeMatch ? routeMatch.pathnameBase : "/";
let parentRoute = routeMatch && routeMatch.route;
if (ENABLE_DEV_WARNINGS) {
let parentPath = parentRoute && parentRoute.path || "";
warningOnce(
parentPathname,
!parentRoute || parentPath.endsWith("*") || parentPath.endsWith("*?"),
`You rendered descendant (or called \`useRoutes()\`) at "${parentPathname}" (under ) but the parent route path has no trailing "*". This means if you navigate deeper, the parent won't match anymore and therefore the child routes will never render.
Please change the parent to .`
);
}
let locationFromContext = useLocation();
let location;
if (locationArg) {
let parsedLocationArg = typeof locationArg === "string" ? parsePath(locationArg) : locationArg;
invariant(
parentPathnameBase === "/" || parsedLocationArg.pathname?.startsWith(parentPathnameBase),
`When overriding the location using \`\` or \`useRoutes(routes, location)\`, the location pathname must begin with the portion of the URL pathname that was matched by all parent routes. The current pathname base is "${parentPathnameBase}" but pathname "${parsedLocationArg.pathname}" was given in the \`location\` prop.`
);
location = parsedLocationArg;
} else {
location = locationFromContext;
}
let pathname = location.pathname || "/";
let remainingPathname = pathname;
if (parentPathnameBase !== "/") {
let parentSegments = parentPathnameBase.replace(/^\//, "").split("/");
let segments = pathname.replace(/^\//, "").split("/");
remainingPathname = "/" + segments.slice(parentSegments.length).join("/");
}
let matches = matchRoutes(routes, { pathname: remainingPathname });
if (ENABLE_DEV_WARNINGS) {
warning(
parentRoute || matches != null,
`No routes matched location "${location.pathname}${location.search}${location.hash}" `
);
warning(
matches == null || matches[matches.length - 1].route.element !== void 0 || matches[matches.length - 1].route.Component !== void 0 || matches[matches.length - 1].route.lazy !== void 0,
`Matched leaf route at location "${location.pathname}${location.search}${location.hash}" does not have an element or Component. This means it will render an with a null value by default resulting in an "empty" page.`
);
}
let renderedMatches = _renderMatches(
matches && matches.map(
(match) => Object.assign({}, match, {
params: Object.assign({}, parentParams, match.params),
pathname: joinPaths([
parentPathnameBase,
// Re-encode pathnames that were decoded inside matchRoutes
navigator2.encodeLocation ? navigator2.encodeLocation(match.pathname).pathname : match.pathname
]),
pathnameBase: match.pathnameBase === "/" ? parentPathnameBase : joinPaths([
parentPathnameBase,
// Re-encode pathnames that were decoded inside matchRoutes
navigator2.encodeLocation ? navigator2.encodeLocation(match.pathnameBase).pathname : match.pathnameBase
])
})
),
parentMatches,
dataRouterState,
future
);
if (locationArg && renderedMatches) {
return /* @__PURE__ */ React2.createElement(
LocationContext.Provider,
{
value: {
location: {
pathname: "/",
search: "",
hash: "",
state: null,
key: "default",
...location
},
navigationType: "POP" /* Pop */
}
},
renderedMatches
);
}
return renderedMatches;
}
function DefaultErrorComponent() {
let error = useRouteError();
let message = isRouteErrorResponse(error) ? `${error.status} ${error.statusText}` : error instanceof Error ? error.message : JSON.stringify(error);
let stack = error instanceof Error ? error.stack : null;
let lightgrey = "rgba(200,200,200, 0.5)";
let preStyles = { padding: "0.5rem", backgroundColor: lightgrey };
let codeStyles = { padding: "2px 4px", backgroundColor: lightgrey };
let devInfo = null;
if (ENABLE_DEV_WARNINGS) {
console.error(
"Error handled by React Router default ErrorBoundary:",
error
);
devInfo = /* @__PURE__ */ React2.createElement(React2.Fragment, null, /* @__PURE__ */ React2.createElement("p", null, "\u{1F4BF} Hey developer \u{1F44B}"), /* @__PURE__ */ React2.createElement("p", null, "You can provide a way better UX than this when your app throws errors by providing your own ", /* @__PURE__ */ React2.createElement("code", { style: codeStyles }, "ErrorBoundary"), " or", " ", /* @__PURE__ */ React2.createElement("code", { style: codeStyles }, "errorElement"), " prop on your route."));
}
return /* @__PURE__ */ React2.createElement(React2.Fragment, null, /* @__PURE__ */ React2.createElement("h2", null, "Unexpected Application Error!"), /* @__PURE__ */ React2.createElement("h3", { style: { fontStyle: "italic" } }, message), stack ? /* @__PURE__ */ React2.createElement("pre", { style: preStyles }, stack) : null, devInfo);
}
var defaultErrorElement = /* @__PURE__ */ React2.createElement(DefaultErrorComponent, null);
var RenderErrorBoundary = class extends React2.Component {
constructor(props) {
super(props);
this.state = {
location: props.location,
revalidation: props.revalidation,
error: props.error
};
}
static getDerivedStateFromError(error) {
return { error };
}
static getDerivedStateFromProps(props, state) {
if (state.location !== props.location || state.revalidation !== "idle" && props.revalidation === "idle") {
return {
error: props.error,
location: props.location,
revalidation: props.revalidation
};
}
return {
error: props.error !== void 0 ? props.error : state.error,
location: state.location,
revalidation: props.revalidation || state.revalidation
};
}
componentDidCatch(error, errorInfo) {
console.error(
"React Router caught the following error during render",
error,
errorInfo
);
}
render() {
return this.state.error !== void 0 ? /* @__PURE__ */ React2.createElement(RouteContext.Provider, { value: this.props.routeContext }, /* @__PURE__ */ React2.createElement(
RouteErrorContext.Provider,
{
value: this.state.error,
children: this.props.component
}
)) : this.props.children;
}
};
function RenderedRoute({ routeContext, match, children }) {
let dataRouterContext = React2.useContext(DataRouterContext);
if (dataRouterContext && dataRouterContext.static && dataRouterContext.staticContext && (match.route.errorElement || match.route.ErrorBoundary)) {
dataRouterContext.staticContext._deepestRenderedBoundaryId = match.route.id;
}
return /* @__PURE__ */ React2.createElement(RouteContext.Provider, { value: routeContext }, children);
}
function _renderMatches(matches, parentMatches = [], dataRouterState = null, future = null) {
if (matches == null) {
if (!dataRouterState) {
return null;
}
if (dataRouterState.errors) {
matches = dataRouterState.matches;
} else if (parentMatches.length === 0 && !dataRouterState.initialized && dataRouterState.matches.length > 0) {
matches = dataRouterState.matches;
} else {
return null;
}
}
let renderedMatches = matches;
let errors = dataRouterState?.errors;
if (errors != null) {
let errorIndex = renderedMatches.findIndex(
(m) => m.route.id && errors?.[m.route.id] !== void 0
);
invariant(
errorIndex >= 0,
`Could not find a matching route for errors on route IDs: ${Object.keys(
errors
).join(",")}`
);
renderedMatches = renderedMatches.slice(
0,
Math.min(renderedMatches.length, errorIndex + 1)
);
}
let renderFallback = false;
let fallbackIndex = -1;
if (dataRouterState) {
for (let i = 0; i < renderedMatches.length; i++) {
let match = renderedMatches[i];
if (match.route.HydrateFallback || match.route.hydrateFallbackElement) {
fallbackIndex = i;
}
if (match.route.id) {
let { loaderData, errors: errors2 } = dataRouterState;
let needsToRunLoader = match.route.loader && !loaderData.hasOwnProperty(match.route.id) && (!errors2 || errors2[match.route.id] === void 0);
if (match.route.lazy || needsToRunLoader) {
renderFallback = true;
if (fallbackIndex >= 0) {
renderedMatches = renderedMatches.slice(0, fallbackIndex + 1);
} else {
renderedMatches = [renderedMatches[0]];
}
break;
}
}
}
}
return renderedMatches.reduceRight((outlet, match, index) => {
let error;
let shouldRenderHydrateFallback = false;
let errorElement = null;
let hydrateFallbackElement = null;
if (dataRouterState) {
error = errors && match.route.id ? errors[match.route.id] : void 0;
errorElement = match.route.errorElement || defaultErrorElement;
if (renderFallback) {
if (fallbackIndex < 0 && index === 0) {
warningOnce(
"route-fallback",
false,
"No `HydrateFallback` element provided to render during initial hydration"
);
shouldRenderHydrateFallback = true;
hydrateFallbackElement = null;
} else if (fallbackIndex === index) {
shouldRenderHydrateFallback = true;
hydrateFallbackElement = match.route.hydrateFallbackElement || null;
}
}
}
let matches2 = parentMatches.concat(renderedMatches.slice(0, index + 1));
let getChildren = () => {
let children;
if (error) {
children = errorElement;
} else if (shouldRenderHydrateFallback) {
children = hydrateFallbackElement;
} else if (match.route.Component) {
children = /* @__PURE__ */ React2.createElement(match.route.Component, null);
} else if (match.route.element) {
children = match.route.element;
} else {
children = outlet;
}
return /* @__PURE__ */ React2.createElement(
RenderedRoute,
{
match,
routeContext: {
outlet,
matches: matches2,
isDataRoute: dataRouterState != null
},
children
}
);
};
return dataRouterState && (match.route.ErrorBoundary || match.route.errorElement || index === 0) ? /* @__PURE__ */ React2.createElement(
RenderErrorBoundary,
{
location: dataRouterState.location,
revalidation: dataRouterState.revalidation,
component: errorElement,
error,
children: getChildren(),
routeContext: { outlet: null, matches: matches2, isDataRoute: true }
}
) : getChildren();
}, null);
}
function getDataRouterConsoleError(hookName) {
return `${hookName} must be used within a data router. See https://reactrouter.com/en/main/routers/picking-a-router.`;
}
function useDataRouterContext(hookName) {
let ctx = React2.useContext(DataRouterContext);
invariant(ctx, getDataRouterConsoleError(hookName));
return ctx;
}
function useDataRouterState(hookName) {
let state = React2.useContext(DataRouterStateContext);
invariant(state, getDataRouterConsoleError(hookName));
return state;
}
function useRouteContext(hookName) {
let route = React2.useContext(RouteContext);
invariant(route, getDataRouterConsoleError(hookName));
return route;
}
function useCurrentRouteId(hookName) {
let route = useRouteContext(hookName);
let thisRoute = route.matches[route.matches.length - 1];
invariant(
thisRoute.route.id,
`${hookName} can only be used on routes that contain a unique "id"`
);
return thisRoute.route.id;
}
function useRouteId() {
return useCurrentRouteId("useRouteId" /* UseRouteId */);
}
function useNavigation() {
let state = useDataRouterState("useNavigation" /* UseNavigation */);
return state.navigation;
}
function useRevalidator() {
let dataRouterContext = useDataRouterContext("useRevalidator" /* UseRevalidator */);
let state = useDataRouterState("useRevalidator" /* UseRevalidator */);
return React2.useMemo(
() => ({
async revalidate() {
await dataRouterContext.router.revalidate();
},
state: state.revalidation
}),
[dataRouterContext.router, state.revalidation]
);
}
function useMatches() {
let { matches, loaderData } = useDataRouterState(
"useMatches" /* UseMatches */
);
return React2.useMemo(
() => matches.map((m) => convertRouteMatchToUiMatch(m, loaderData)),
[matches, loaderData]
);
}
function useLoaderData() {
let state = useDataRouterState("useLoaderData" /* UseLoaderData */);
let routeId = useCurrentRouteId("useLoaderData" /* UseLoaderData */);
return state.loaderData[routeId];
}
function useRouteLoaderData(routeId) {
let state = useDataRouterState("useRouteLoaderData" /* UseRouteLoaderData */);
return state.loaderData[routeId];
}
function useActionData() {
let state = useDataRouterState("useActionData" /* UseActionData */);
let routeId = useCurrentRouteId("useLoaderData" /* UseLoaderData */);
return state.actionData ? state.actionData[routeId] : void 0;
}
function useRouteError() {
let error = React2.useContext(RouteErrorContext);
let state = useDataRouterState("useRouteError" /* UseRouteError */);
let routeId = useCurrentRouteId("useRouteError" /* UseRouteError */);
if (error !== void 0) {
return error;
}
return state.errors?.[routeId];
}
function useAsyncValue() {
let value = React2.useContext(AwaitContext);
return value?._data;
}
function useAsyncError() {
let value = React2.useContext(AwaitContext);
return value?._error;
}
var blockerId = 0;
function useBlocker(shouldBlock) {
let { router, basename } = useDataRouterContext("useBlocker" /* UseBlocker */);
let state = useDataRouterState("useBlocker" /* UseBlocker */);
let [blockerKey, setBlockerKey] = React2.useState("");
let blockerFunction = React2.useCallback(
(arg) => {
if (typeof shouldBlock !== "function") {
return !!shouldBlock;
}
if (basename === "/") {
return shouldBlock(arg);
}
let { currentLocation, nextLocation, historyAction } = arg;
return shouldBlock({
currentLocation: {
...currentLocation,
pathname: stripBasename(currentLocation.pathname, basename) || currentLocation.pathname
},
nextLocation: {
...nextLocation,
pathname: stripBasename(nextLocation.pathname, basename) || nextLocation.pathname
},
historyAction
});
},
[basename, shouldBlock]
);
React2.useEffect(() => {
let key = String(++blockerId);
setBlockerKey(key);
return () => router.deleteBlocker(key);
}, [router]);
React2.useEffect(() => {
if (blockerKey !== "") {
router.getBlocker(blockerKey, blockerFunction);
}
}, [router, blockerKey, blockerFunction]);
return blockerKey && state.blockers.has(blockerKey) ? state.blockers.get(blockerKey) : IDLE_BLOCKER;
}
function useNavigateStable() {
let { router } = useDataRouterContext("useNavigate" /* UseNavigateStable */);
let id = useCurrentRouteId("useNavigate" /* UseNavigateStable */);
let activeRef = React2.useRef(false);
useIsomorphicLayoutEffect(() => {
activeRef.current = true;
});
let navigate = React2.useCallback(
async (to, options = {}) => {
warning(activeRef.current, navigateEffectWarning);
if (!activeRef.current) return;
if (typeof to === "number") {
router.navigate(to);
} else {
await router.navigate(to, { fromRouteId: id, ...options });
}
},
[router, id]
);
return navigate;
}
var alreadyWarned = {};
function warningOnce(key, cond, message) {
if (!cond && !alreadyWarned[key]) {
alreadyWarned[key] = true;
warning(false, message);
}
}
// lib/server-runtime/warnings.ts
var alreadyWarned2 = {};
function warnOnce(condition, message) {
if (!condition && !alreadyWarned2[message]) {
alreadyWarned2[message] = true;
console.warn(message);
}
}
// lib/components.tsx
var ENABLE_DEV_WARNINGS2 = true;
function mapRouteProperties(route) {
let updates = {
// Note: this check also occurs in createRoutesFromChildren so update
// there if you change this -- please and thank you!
hasErrorBoundary: route.hasErrorBoundary || route.ErrorBoundary != null || route.errorElement != null
};
if (route.Component) {
if (ENABLE_DEV_WARNINGS2) {
if (route.element) {
warning(
false,
"You should not include both `Component` and `element` on your route - `Component` will be used."
);
}
}
Object.assign(updates, {
element: React3.createElement(route.Component),
Component: void 0
});
}
if (route.HydrateFallback) {
if (ENABLE_DEV_WARNINGS2) {
if (route.hydrateFallbackElement) {
warning(
false,
"You should not include both `HydrateFallback` and `hydrateFallbackElement` on your route - `HydrateFallback` will be used."
);
}
}
Object.assign(updates, {
hydrateFallbackElement: React3.createElement(route.HydrateFallback),
HydrateFallback: void 0
});
}
if (route.ErrorBoundary) {
if (ENABLE_DEV_WARNINGS2) {
if (route.errorElement) {
warning(
false,
"You should not include both `ErrorBoundary` and `errorElement` on your route - `ErrorBoundary` will be used."
);
}
}
Object.assign(updates, {
errorElement: React3.createElement(route.ErrorBoundary),
ErrorBoundary: void 0
});
}
return updates;
}
function createMemoryRouter(routes, opts) {
return createRouter({
basename: opts?.basename,
future: opts?.future,
history: createMemoryHistory({
initialEntries: opts?.initialEntries,
initialIndex: opts?.initialIndex
}),
hydrationData: opts?.hydrationData,
routes,
mapRouteProperties,
dataStrategy: opts?.dataStrategy,
patchRoutesOnNavigation: opts?.patchRoutesOnNavigation
}).initialize();
}
var Deferred = class {
constructor() {
this.status = "pending";
this.promise = new Promise((resolve, reject) => {
this.resolve = (value) => {
if (this.status === "pending") {
this.status = "resolved";
resolve(value);
}
};
this.reject = (reason) => {
if (this.status === "pending") {
this.status = "rejected";
reject(reason);
}
};
});
}
};
function RouterProvider({
router,
flushSync: reactDomFlushSyncImpl
}) {
let [state, setStateImpl] = React3.useState(router.state);
let [pendingState, setPendingState] = React3.useState();
let [vtContext, setVtContext] = React3.useState({
isTransitioning: false
});
let [renderDfd, setRenderDfd] = React3.useState();
let [transition, setTransition] = React3.useState();
let [interruption, setInterruption] = React3.useState();
let fetcherData = React3.useRef(/* @__PURE__ */ new Map());
let setState = React3.useCallback(
(newState, { deletedFetchers, flushSync, viewTransitionOpts }) => {
deletedFetchers.forEach((key) => fetcherData.current.delete(key));
newState.fetchers.forEach((fetcher, key) => {
if (fetcher.data !== void 0) {
fetcherData.current.set(key, fetcher.data);
}
});
warnOnce(
flushSync === false || reactDomFlushSyncImpl != null,
'You provided the `flushSync` option to a router update, but you are not using the `` from `react-router/dom` so `ReactDOM.flushSync()` is unavailable. Please update your app to `import { RouterProvider } from "react-router/dom"` and ensure you have `react-dom` installed as a dependency to use the `flushSync` option.'
);
let isViewTransitionAvailable = router.window != null && router.window.document != null && typeof router.window.document.startViewTransition === "function";
warnOnce(
viewTransitionOpts == null || isViewTransitionAvailable,
"You provided the `viewTransition` option to a router update, but you do not appear to be running in a DOM environment as `window.startViewTransition` is not available."
);
if (!viewTransitionOpts || !isViewTransitionAvailable) {
if (reactDomFlushSyncImpl && flushSync) {
reactDomFlushSyncImpl(() => setStateImpl(newState));
} else {
React3.startTransition(() => setStateImpl(newState));
}
return;
}
if (reactDomFlushSyncImpl && flushSync) {
reactDomFlushSyncImpl(() => {
if (transition) {
renderDfd && renderDfd.resolve();
transition.skipTransition();
}
setVtContext({
isTransitioning: true,
flushSync: true,
currentLocation: viewTransitionOpts.currentLocation,
nextLocation: viewTransitionOpts.nextLocation
});
});
let t = router.window.document.startViewTransition(() => {
reactDomFlushSyncImpl(() => setStateImpl(newState));
});
t.finished.finally(() => {
reactDomFlushSyncImpl(() => {
setRenderDfd(void 0);
setTransition(void 0);
setPendingState(void 0);
setVtContext({ isTransitioning: false });
});
});
reactDomFlushSyncImpl(() => setTransition(t));
return;
}
if (transition) {
renderDfd && renderDfd.resolve();
transition.skipTransition();
setInterruption({
state: newState,
currentLocation: viewTransitionOpts.currentLocation,
nextLocation: viewTransitionOpts.nextLocation
});
} else {
setPendingState(newState);
setVtContext({
isTransitioning: true,
flushSync: false,
currentLocation: viewTransitionOpts.currentLocation,
nextLocation: viewTransitionOpts.nextLocation
});
}
},
[router.window, reactDomFlushSyncImpl, transition, renderDfd]
);
React3.useLayoutEffect(() => router.subscribe(setState), [router, setState]);
React3.useEffect(() => {
if (vtContext.isTransitioning && !vtContext.flushSync) {
setRenderDfd(new Deferred());
}
}, [vtContext]);
React3.useEffect(() => {
if (renderDfd && pendingState && router.window) {
let newState = pendingState;
let renderPromise = renderDfd.promise;
let transition2 = router.window.document.startViewTransition(async () => {
React3.startTransition(() => setStateImpl(newState));
await renderPromise;
});
transition2.finished.finally(() => {
setRenderDfd(void 0);
setTransition(void 0);
setPendingState(void 0);
setVtContext({ isTransitioning: false });
});
setTransition(transition2);
}
}, [pendingState, renderDfd, router.window]);
React3.useEffect(() => {
if (renderDfd && pendingState && state.location.key === pendingState.location.key) {
renderDfd.resolve();
}
}, [renderDfd, transition, state.location, pendingState]);
React3.useEffect(() => {
if (!vtContext.isTransitioning && interruption) {
setPendingState(interruption.state);
setVtContext({
isTransitioning: true,
flushSync: false,
currentLocation: interruption.currentLocation,
nextLocation: interruption.nextLocation
});
setInterruption(void 0);
}
}, [vtContext.isTransitioning, interruption]);
let navigator2 = React3.useMemo(() => {
return {
createHref: router.createHref,
encodeLocation: router.encodeLocation,
go: (n) => router.navigate(n),
push: (to, state2, opts) => router.navigate(to, {
state: state2,
preventScrollReset: opts?.preventScrollReset
}),
replace: (to, state2, opts) => router.navigate(to, {
replace: true,
state: state2,
preventScrollReset: opts?.preventScrollReset
})
};
}, [router]);
let basename = router.basename || "/";
let dataRouterContext = React3.useMemo(
() => ({
router,
navigator: navigator2,
static: false,
basename
}),
[router, navigator2, basename]
);
return /* @__PURE__ */ React3.createElement(React3.Fragment, null, /* @__PURE__ */ React3.createElement(DataRouterContext.Provider, { value: dataRouterContext }, /* @__PURE__ */ React3.createElement(DataRouterStateContext.Provider, { value: state }, /* @__PURE__ */ React3.createElement(FetchersContext.Provider, { value: fetcherData.current }, /* @__PURE__ */ React3.createElement(ViewTransitionContext.Provider, { value: vtContext }, /* @__PURE__ */ React3.createElement(
Router,
{
basename,
location: state.location,
navigationType: state.historyAction,
navigator: navigator2
},
/* @__PURE__ */ React3.createElement(
MemoizedDataRoutes,
{
routes: router.routes,
future: router.future,
state
}
)
))))), null);
}
var MemoizedDataRoutes = React3.memo(DataRoutes);
function DataRoutes({
routes,
future,
state
}) {
return useRoutesImpl(routes, void 0, state, future);
}
function MemoryRouter({
basename,
children,
initialEntries,
initialIndex
}) {
let historyRef = React3.useRef();
if (historyRef.current == null) {
historyRef.current = createMemoryHistory({
initialEntries,
initialIndex,
v5Compat: true
});
}
let history = historyRef.current;
let [state, setStateImpl] = React3.useState({
action: history.action,
location: history.location
});
let setState = React3.useCallback(
(newState) => {
React3.startTransition(() => setStateImpl(newState));
},
[setStateImpl]
);
React3.useLayoutEffect(() => history.listen(setState), [history, setState]);
return /* @__PURE__ */ React3.createElement(
Router,
{
basename,
children,
location: state.location,
navigationType: state.action,
navigator: history
}
);
}
function Navigate({
to,
replace: replace2,
state,
relative
}) {
invariant(
useInRouterContext(),
// TODO: This error is probably because they somehow have 2 versions of
// the router loaded. We can help them understand how to avoid that.
` may be used only in the context of a component.`
);
let { static: isStatic } = React3.useContext(NavigationContext);
warning(
!isStatic,
` must not be used on the initial render in a . This is a no-op, but you should modify your code so the is only ever rendered in response to some user interaction or state change.`
);
let { matches } = React3.useContext(RouteContext);
let { pathname: locationPathname } = useLocation();
let navigate = useNavigate();
let path = resolveTo(
to,
getResolveToMatches(matches),
locationPathname,
relative === "path"
);
let jsonPath = JSON.stringify(path);
React3.useEffect(() => {
navigate(JSON.parse(jsonPath), { replace: replace2, state, relative });
}, [navigate, jsonPath, relative, replace2, state]);
return null;
}
function Outlet(props) {
return useOutlet(props.context);
}
function Route(_props) {
invariant(
false,
`A is only ever to be used as the child of element, never rendered directly. Please wrap your in a .`
);
}
function Router({
basename: basenameProp = "/",
children = null,
location: locationProp,
navigationType = "POP" /* Pop */,
navigator: navigator2,
static: staticProp = false
}) {
invariant(
!useInRouterContext(),
`You cannot render a inside another . You should never have more than one in your app.`
);
let basename = basenameProp.replace(/^\/*/, "/");
let navigationContext = React3.useMemo(
() => ({
basename,
navigator: navigator2,
static: staticProp,
future: {}
}),
[basename, navigator2, staticProp]
);
if (typeof locationProp === "string") {
locationProp = parsePath(locationProp);
}
let {
pathname = "/",
search = "",
hash = "",
state = null,
key = "default"
} = locationProp;
let locationContext = React3.useMemo(() => {
let trailingPathname = stripBasename(pathname, basename);
if (trailingPathname == null) {
return null;
}
return {
location: {
pathname: trailingPathname,
search,
hash,
state,
key
},
navigationType
};
}, [basename, pathname, search, hash, state, key, navigationType]);
warning(
locationContext != null,
` is not able to match the URL "${pathname}${search}${hash}" because it does not start with the basename, so the won't render anything.`
);
if (locationContext == null) {
return null;
}
return /* @__PURE__ */ React3.createElement(NavigationContext.Provider, { value: navigationContext }, /* @__PURE__ */ React3.createElement(LocationContext.Provider, { children, value: locationContext }));
}
function Routes({
children,
location
}) {
return useRoutes(createRoutesFromChildren(children), location);
}
function Await({
children,
errorElement,
resolve
}) {
return /* @__PURE__ */ React3.createElement(AwaitErrorBoundary, { resolve, errorElement }, /* @__PURE__ */ React3.createElement(ResolveAwait, null, children));
}
var AwaitErrorBoundary = class extends React3.Component {
constructor(props) {
super(props);
this.state = { error: null };
}
static getDerivedStateFromError(error) {
return { error };
}
componentDidCatch(error, errorInfo) {
console.error(
" caught the following error during render",
error,
errorInfo
);
}
render() {
let { children, errorElement, resolve } = this.props;
let promise = null;
let status = 0 /* pending */;
if (!(resolve instanceof Promise)) {
status = 1 /* success */;
promise = Promise.resolve();
Object.defineProperty(promise, "_tracked", { get: () => true });
Object.defineProperty(promise, "_data", { get: () => resolve });
} else if (this.state.error) {
status = 2 /* error */;
let renderError = this.state.error;
promise = Promise.reject().catch(() => {
});
Object.defineProperty(promise, "_tracked", { get: () => true });
Object.defineProperty(promise, "_error", { get: () => renderError });
} else if (resolve._tracked) {
promise = resolve;
status = "_error" in promise ? 2 /* error */ : "_data" in promise ? 1 /* success */ : 0 /* pending */;
} else {
status = 0 /* pending */;
Object.defineProperty(resolve, "_tracked", { get: () => true });
promise = resolve.then(
(data2) => Object.defineProperty(resolve, "_data", { get: () => data2 }),
(error) => Object.defineProperty(resolve, "_error", { get: () => error })
);
}
if (status === 2 /* error */ && !errorElement) {
throw promise._error;
}
if (status === 2 /* error */) {
return /* @__PURE__ */ React3.createElement(AwaitContext.Provider, { value: promise, children: errorElement });
}
if (status === 1 /* success */) {
return /* @__PURE__ */ React3.createElement(AwaitContext.Provider, { value: promise, children });
}
throw promise;
}
};
function ResolveAwait({
children
}) {
let data2 = useAsyncValue();
let toRender = typeof children === "function" ? children(data2) : children;
return /* @__PURE__ */ React3.createElement(React3.Fragment, null, toRender);
}
function createRoutesFromChildren(children, parentPath = []) {
let routes = [];
React3.Children.forEach(children, (element, index) => {
if (!React3.isValidElement(element)) {
return;
}
let treePath = [...parentPath, index];
if (element.type === React3.Fragment) {
routes.push.apply(
routes,
createRoutesFromChildren(element.props.children, treePath)
);
return;
}
invariant(
element.type === Route,
`[${typeof element.type === "string" ? element.type : element.type.name}] is not a component. All component children of must be a or `
);
invariant(
!element.props.index || !element.props.children,
"An index route cannot have child routes."
);
let route = {
id: element.props.id || treePath.join("-"),
caseSensitive: element.props.caseSensitive,
element: element.props.element,
Component: element.props.Component,
index: element.props.index,
path: element.props.path,
loader: element.props.loader,
action: element.props.action,
hydrateFallbackElement: element.props.hydrateFallbackElement,
HydrateFallback: element.props.HydrateFallback,
errorElement: element.props.errorElement,
ErrorBoundary: element.props.ErrorBoundary,
hasErrorBoundary: element.props.hasErrorBoundary === true || element.props.ErrorBoundary != null || element.props.errorElement != null,
shouldRevalidate: element.props.shouldRevalidate,
handle: element.props.handle,
lazy: element.props.lazy
};
if (element.props.children) {
route.children = createRoutesFromChildren(
element.props.children,
treePath
);
}
routes.push(route);
});
return routes;
}
var createRoutesFromElements = createRoutesFromChildren;
function renderMatches(matches) {
return _renderMatches(matches);
}
// lib/dom/lib.tsx
var React10 = __toESM(require("react"));
// lib/dom/dom.ts
var defaultMethod = "get";
var defaultEncType = "application/x-www-form-urlencoded";
function isHtmlElement(object) {
return object != null && typeof object.tagName === "string";
}
function isButtonElement(object) {
return isHtmlElement(object) && object.tagName.toLowerCase() === "button";
}
function isFormElement(object) {
return isHtmlElement(object) && object.tagName.toLowerCase() === "form";
}
function isInputElement(object) {
return isHtmlElement(object) && object.tagName.toLowerCase() === "input";
}
function isModifiedEvent(event) {
return !!(event.metaKey || event.altKey || event.ctrlKey || event.shiftKey);
}
function shouldProcessLinkClick(event, target) {
return event.button === 0 && // Ignore everything but left clicks
(!target || target === "_self") && // Let browser handle "target=_blank" etc.
!isModifiedEvent(event);
}
function createSearchParams(init = "") {
return new URLSearchParams(
typeof init === "string" || Array.isArray(init) || init instanceof URLSearchParams ? init : Object.keys(init).reduce((memo2, key) => {
let value = init[key];
return memo2.concat(
Array.isArray(value) ? value.map((v) => [key, v]) : [[key, value]]
);
}, [])
);
}
function getSearchParamsForLocation(locationSearch, defaultSearchParams) {
let searchParams = createSearchParams(locationSearch);
if (defaultSearchParams) {
defaultSearchParams.forEach((_, key) => {
if (!searchParams.has(key)) {
defaultSearchParams.getAll(key).forEach((value) => {
searchParams.append(key, value);
});
}
});
}
return searchParams;
}
var _formDataSupportsSubmitter = null;
function isFormDataSubmitterSupported() {
if (_formDataSupportsSubmitter === null) {
try {
new FormData(
document.createElement("form"),
// @ts-expect-error if FormData supports the submitter parameter, this will throw
0
);
_formDataSupportsSubmitter = false;
} catch (e) {
_formDataSupportsSubmitter = true;
}
}
return _formDataSupportsSubmitter;
}
var supportedFormEncTypes = /* @__PURE__ */ new Set([
"application/x-www-form-urlencoded",
"multipart/form-data",
"text/plain"
]);
function getFormEncType(encType) {
if (encType != null && !supportedFormEncTypes.has(encType)) {
warning(
false,
`"${encType}" is not a valid \`encType\` for \`
© 2015 - 2025 Weber Informatics LLC | Privacy Policy