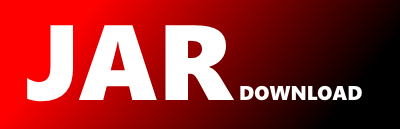
package.build.modules.attributes.js Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of snabbdom Show documentation
Show all versions of snabbdom Show documentation
A virtual DOM library with focus on simplicity, modularity, powerful features and performance.
The newest version!
const xlinkNS = "http://www.w3.org/1999/xlink";
const xmlNS = "http://www.w3.org/XML/1998/namespace";
const colonChar = 58;
const xChar = 120;
function updateAttrs(oldVnode, vnode) {
let key;
const elm = vnode.elm;
let oldAttrs = oldVnode.data.attrs;
let attrs = vnode.data.attrs;
if (!oldAttrs && !attrs)
return;
if (oldAttrs === attrs)
return;
oldAttrs = oldAttrs || {};
attrs = attrs || {};
// update modified attributes, add new attributes
for (key in attrs) {
const cur = attrs[key];
const old = oldAttrs[key];
if (old !== cur) {
if (cur === true) {
elm.setAttribute(key, "");
}
else if (cur === false) {
elm.removeAttribute(key);
}
else {
if (key.charCodeAt(0) !== xChar) {
elm.setAttribute(key, cur);
}
else if (key.charCodeAt(3) === colonChar) {
// Assume xml namespace
elm.setAttributeNS(xmlNS, key, cur);
}
else if (key.charCodeAt(5) === colonChar) {
// Assume xlink namespace
elm.setAttributeNS(xlinkNS, key, cur);
}
else {
elm.setAttribute(key, cur);
}
}
}
}
// remove removed attributes
// use `in` operator since the previous `for` iteration uses it (.i.e. add even attributes with undefined value)
// the other option is to remove all attributes with value == undefined
for (key in oldAttrs) {
if (!(key in attrs)) {
elm.removeAttribute(key);
}
}
}
export const attributesModule = {
create: updateAttrs,
update: updateAttrs,
};
//# sourceMappingURL=attributes.js.map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy